Backend Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified backend developers for your organization.
Backend
Backend developers, renowned for their in-depth mastery of server-side technologies, stand as vital components in nearly all application development teams.
According to the CoderPad 2023 Developer survey, backend developers are the THE most in-demand job role technical recruiters are looking to fill.
In the subsequent sections, you will find an array of practical coding tasks and interview questions designed to assess the technical competence of backend developer candidates during evaluations.
Additionally, we have included a selection of endorsed best practices to aid in a reliable assessment of candidates’ backend skills through your interview queries.
Table of Contents
Backend example questions
Question 1: Create a CRUD API
The goal of this exercise is to retrieve data from an external source, store it in an appropriate database structure, and create a CRUD RESTful API to interface with the database
Goals
1. Read the data from this graphql endpoint: https://swapi-graphql.netlify.app/.netlify/functions/index with the following query:
query Query {allPlanets{planets{name population terrains climates}}}
(View the shape of the data here.)
2. Store the data from the graphql endpoint into the database and create appropriate models
3. Write RESTful Create, Read, Update, and Delete endpoints to interact with the database
Question 2: Shopping List
This question tests a candidate’s ability to create model(s) for the data structure, insert hard-coded data into a database, modify tables and fields in a database, and write endpoints to interact with data.
Part 1
Create a model for the shopping lists and items located in app/data/data.ts.
Part 2
Store the shopping lists and items located in app/data/data.ts in the database.
Part 3
Modify the database such that a price field can be stored for each item.
Part 4
Multiply the quantity of each item in the shopping lists by two.
Part 5
Create API endpoints to dynamically get a list of shopping lists and items.
Assessing the candidate
The candidate should create models for a shopping list and items. They should then write code to create the tables necessary and insert the data into the tables. Additionally, the candidate can be asked to edit the data in the tables and add additional fields. Finally, endpoints to interact with the data can be created.
Backend skills to assess
Backend job roles
Junior backend interview questions
Question: What’s the difference between a framework and a library?
Answer: A library is a collection of functions/methods/classes that you can call from your own code. You’re in charge of the flow of the application; you decide when to call the library. In contrast, with a framework, the flow is determined by the framework itself, invoking your code at specific points (Inversion of Control). Examples include Flask as a framework and NumPy as a library in Python.
Question: Consider the following Python code snippet. Can you identify what’s wrong with it?
def add_numbers(a, b):
return a + b
result = add_numbers("5", 3)
Code language: JavaScript (javascript)
Answer: The function add_numbers
is trying to add a string (“5”) and an integer (3) together, which will raise a TypeError in Python. To fix it, one must ensure that both variables are of the same datatype, by converting the string to an integer.
Question: Explain what a JOIN
operation is in SQL.
Answer: A JOIN
operation in SQL is used to combine rows from two or more tables based on a related column between them. The most common types of JOINs include INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL JOIN.
Question: Look at this SQL query and explain if there is any vulnerability:
query = "SELECT * FROM users WHERE username = '" + username + "' AND password = '" + password + "';"
Code language: JavaScript (javascript)
Answer: This query is vulnerable to SQL injection. An attacker can provide malicious input in username
or password
to modify the query and potentially access or harm the database. To prevent this, one should use parameterized queries or prepared statements.
Question: Describe the concept of statelessness in RESTful APIs.
Answer: Statelessness means that each HTTP request from a client to a server must contain all the information needed to understand and process the request. The server should not store any context between requests, ensuring that each request can be understood in isolation.
Question: What’s the output of the following Python function?
def mystery_function(a, b=[]):
b.append(a)
return b
print(mystery_function(1))
print(mystery_function(2))
Code language: PHP (php)
Answer: The output will be:
[1]
[1, 2]
Code language: JSON / JSON with Comments (json)
The mutable default argument (b=[]
) is a common Python gotcha. The list b
is created only once when the function is defined, so successive calls to the function reuse the same list.
Question: What is the most Pythonic way to check if a list is empty (considering you are sure the variable to test is a list) ?
Answer:
if my_list:
# do something when list is not empty
else:
# do something when list is empty
Code language: PHP (php)
It is not necessary to compare it with == []
or to call len(my_list)
. When used in a condition, the empty tuples, lists, dictionaries and sets are treated as False
.
Question: Given this simple express.js middleware, what is its purpose?
app.use((req, res, next) => {
res.setHeader('Cache-Control', 'no-cache');
next();
});
Code language: JavaScript (javascript)
Answer: This middleware sets the Cache-Control
header to no-cache
for all responses. It instructs the browser (or caching servers) not to cache the content of the response, ensuring that the client always fetches the latest content from the server.
Question: How can you ensure data integrity in a relational database?
Answer: Data integrity can be maintained through the use of constraints such as primary keys, foreign keys, unique constraints, and check constraints. Regular backups, validations, and normalization can also help maintain data integrity.
Question: In the following Node.js code, what issue might arise and how can you fix it?
const fs = require('fs');
fs.readFile('file.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
fs.unlinkSync('file.txt');
Code language: JavaScript (javascript)
Answer: The code is trying to read the content of ‘file.txt’ asynchronously while it’s being deleted synchronously. There’s a race condition; the file might be deleted before the readFile operation completes, leading to an error. To fix it, the deletion should be moved inside the callback of readFile
to ensure the read operation completes before the file is deleted.
Intermediate backend developer questions
Question: Describe the differences between horizontal and vertical scaling.
Answer:
- Horizontal scaling means adding more machines to a system. This is often referred to as scaling out. For example, adding more nodes to a distributed database or adding more servers in a load-balanced environment.
- Vertical scaling means increasing the resources of an existing machine, such as adding more RAM, CPU, or storage. This is often referred to as scaling up.
Question: Analyze the potential issues with this piece of code handling database connections:
def get_data(query):
connection = create_db_connection()
data = connection.execute(query)
connection.close()
return data
Code language: JavaScript (javascript)
Answer: There are a couple potential issues:
- There’s no error handling. If the connection fails or the query has issues, the code could break.
- Each time data is fetched, a new database connection is established and closed. This is resource-intensive and can slow down applications, especially if
get_data
is called frequently.
Question: What are microservices, and what are their benefits over a monolithic architecture?
Answer: Microservices is an architectural style that structures an application as a collection of loosely coupled, independently deployable services. Each service corresponds to a business capability and often has its own database. Benefits include better scalability, flexibility in technology choices for each service, easier maintenance and upgrades, and enhanced fault isolation.
Question: Examine the following endpoint in a Flask application and suggest improvements:
@app.route('/users/<id>', methods=['GET'])
def get_user(id):
user = db.session.query(User).filter_by(id=id).first()
return jsonify(user)
Code language: JavaScript (javascript)
Answer:
- The function doesn’t handle the case where the user might not be found, potentially leading to a
None
object being passed tojsonify
. To fix this, Replace the functionfirst
by the functionone
. Exceptions will be raised and handled by the rest of the code if there is not exactly one record found. - Directly serializing the ORM object may expose sensitive fields. It’s better to use a serialization method or library to ensure only required fields are exposed.
- No input validation or type checking for
id
.
Question: What is the potential bottleneck in the following database query?
SELECT * FROM orders ORDER BY creation_date DESC LIMIT 10;
Answer: If there is no index on the creation_date
column, sorting the table can be resource-intensive, especially as the orders
table grows in size. To improve this, an index on the creation_date
column should be considered.
Question: Explain the concept of eventual consistency.
Answer: Eventual consistency refers to a system state where all replicas of data might not be immediately consistent but will become consistent over time. It is a relaxation of the strong consistency model to achieve higher availability and scalability in distributed systems.
Question: Consider the following asynchronous Node.js function. What’s wrong with it?
async function fetchData() {
let data = await someAsyncFunction();
if (!data) {
throw new Error("Data not found");
}
return processData(data);
}
Code language: JavaScript (javascript)
Answer: The function lacks error handling for potential failures of someAsyncFunction()
. A try-catch block should be added around the await
statement to handle any errors that might arise from that asynchronous call.
Question: Look at this Redis caching function and identify any issues:
def cache_data(key, value):
redis_client = redis.StrictRedis(host='localhost', port=6379, db=0)
redis_client.set(key, value)
Code language: JavaScript (javascript)
Answer: The function initializes a new Redis connection each time it’s called. This can be inefficient, especially if caching operations are frequent. It’s better to maintain a persistent Redis connection or use a connection pool.
Question: Analyze this Django ORM query for optimization:
books = Book.objects.all()
for book in books:
print(book.author.name)
Code language: PHP (php)
Answer: This code suffers from the “N+1 queries problem”. For each book, a separate query is made to fetch the author’s name, leading to N+1 queries in total (1 to fetch all books + N to fetch the authors). The optimized version would use select_related
:
books = Book.objects.select_related('author').all()
for book in books:
print(book.author.name)
Code language: PHP (php)
Question: How does a message queue like RabbitMQ or Kafka improve system architecture?
Answer: Message queues decouple components in a system, allowing for asynchronous processing. This means that a component can send a message to the queue without waiting for it to be processed. This has several benefits:
- Improved performance and responsiveness, as components don’t block waiting for tasks to complete.
- Enhanced system reliability, as the message queue can act as a buffer during high traffic or if a component fails and needs to restart.
- Better scalability, as workers can be easily added or removed based on the workload.
Senior backend developer questions
Question: Explain the principles and benefits of the Twelve-Factor App methodology.
Answer: The Twelve-Factor App is a set of best practices for building modern, scalable, maintainable software-as-a-service apps. Some key principles include:
Certainly! Here are all 12 principles of the 12 Factor App methodology, presented in a consistent format:
- Codebase: One codebase tracked in revision control, with many deploys.
- Dependencies: Explicitly declare and isolate dependencies.
- Config: Store configuration in the environment.
- Backing Services: Treat backing services as attached resources.
- Build, Release, Run: Strictly separate build and run stages.
- Processes: Execute the app as one or more stateless processes.
- Port Binding: Export services via port binding.
- Concurrency: Scale out via the process model.
- Disposability: Maximize robustness with fast startup and graceful shutdown.
- Dev/Prod Parity: Keep development, staging, and production as similar as possible.
- Logs: Treat logs as event streams.
- Admin Processes: Run admin/management tasks as one-off processes.
Benefits include portability across execution environments, minimized divergence between development and production, and scalability.
Question: Explain the CAP theorem and its implications in distributed systems design.
Answer: The CAP theorem states that it’s impossible for a distributed system to simultaneously provide all three of the following guarantees:
- Consistency: Every read receives the most recent write.
- Availability: Every request receives a (non-error) response, without a guarantee that it contains the most recent version.
- Partition tolerance: The system continues to operate despite arbitrary message loss or failure of part of the system.
In the face of a network partition, a system must choose between consistency and availability. This theorem guides the design and trade-offs of distributed databases and systems.
Question: Examine the following piece of Python code and identify potential issues related to concurrency:
counter = 0
def increment_counter():
global counter
for _ in range(1000000):
counter += 1
Code language: PHP (php)
Answer: If increment_counter
is called concurrently from multiple threads or processes, there could be race conditions leading to the counter
not being accurately incremented. A synchronization mechanism like locks or semaphores should be employed to protect the critical section.
Question: Given the following SQL query, how can it be optimized for a large dataset?
SELECT orders.id, products.name
FROM orders
INNER JOIN products ON orders.product_id = products.id
WHERE products.category = 'electronics';
Code language: JavaScript (javascript)
Answer:
- Ensure there are indices on the columns involved in the JOIN operation (
orders.product_id
andproducts.id
). - Add an index on
products.category
since it’s used in the WHERE clause. - Consider denormalization or materialized views if this is a frequently executed query.
- Think about reworking the database schema. The field
products.category
could be changed to a numeric field and the correspondance between num_category and str_category could be stored in an intermediate table.
Question: Analyze this cache invalidation strategy and suggest improvements:
def update_product(product):
db.save(product)
cache.delete(f'product-{product.id}')
Code language: JavaScript (javascript)
Answer: While the function updates a product in the database and invalidates the cache, a race condition could occur where the cache is repopulated with old data before the database update completes. A better strategy might be to use cache versioning or to update the cache with the new data directly after the database update, ensuring fresh data is always available.
Question: How do you handle versioning in RESTful APIs?
Answer: There are multiple strategies:
- URI Versioning: Include the version in the URI, e.g.,
/v1/users
. - Header Versioning: Use custom headers, e.g.,
Accept-version: v1
. - Accept Header: Use the
Accept
header with a versioned media type, e.g.,Accept: application/json; Version=2
. - Query Parameter: Include the version in a query parameter, e.g.,
/users?version=1
.
It’s essential to choose a method that aligns with the organization’s goals, and it’s also vital to provide proper documentation for clients.
Question: Here’s a simplified code to manage tasks in a queue. Identify potential pitfalls:
tasks = []
def add_task(task):
tasks.append(task)
def process_next_task():
if tasks:
task = tasks.pop(0)
# process task
Code language: PHP (php)
Answer:
- The list data structure in Python is not efficient for pop operations from the start of the list. This operation is O(n). Using a data structure like
deque
from thecollections
module would be more efficient. - If used in a multithreaded environment, race conditions can occur, leading to tasks being processed multiple times or not at all. Proper synchronization or thread-safe collections should be used.
Question: Consider the following Kafka consumer code snippet. What could be problematic about this?
consumer = KafkaConsumer('my_topic')
for message in consumer:
process_message(message)
consumer.commit()
Code language: JavaScript (javascript)
Answer: The consumer commits after processing each message, which can be inefficient and slow down the message processing rate. Depending on the process_message
function, failures might result in message loss if a message is committed but not successfully processed. It might be better to batch the commits or use a commit strategy based on time or message count.
Question: Describe the advantages and challenges of transitioning a monolithic application to a microservices architecture.
Answer:
Advantages:
- Scalability: Each service can be scaled independently based on its requirements.
- Flexibility: Different services can use different technologies.
- Maintainability: Smaller codebases are easier to understand and maintain.
- Faster Time to Market: Teams can work on different services simultaneously, leading to faster feature releases.
Challenges:
- Network Complexity: Increased inter-service communication can introduce latency and complexity.
- Data Consistency: With services having their own databases, ensuring data consistency can be challenging.
- Operational Overhead: More services mean more things to deploy, monitor, and manage.
- Service Discovery: Services need to discover and communicate with each other.
More backend interview resources
For more guides on improving your knowledge of software engineer hiring, we have outlined helpful blog posts below:
- Hire Back-End Developers: 11 strategies and niche job boards
- Software Developers Want to Leave Their Jobs. Is Your Company Ready to Recruit?
- Prompt Engineering for Technical Interview Questions
Additionally, we offer the following backend framework interview questions:
1,000 Companies use CoderPad to Screen and Interview Developers
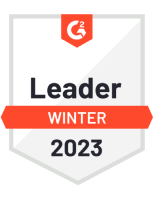
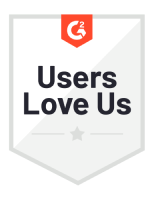
Best interview practices for backend roles
The backend interview process can often be quite diverse, influenced by various elements including the specific engineering role in focus and the applicant’s level of expertise in backend technologies. To enhance the effectiveness of your backend interview questions, consider adopting the following strategies when interacting with potential recruits:
- Develop technical queries that reflect actual scenarios encountered in your organization – this approach is not only more engaging for the candidate but also serves as a more accurate gauge of how their skill set aligns with your team’s needs.
- Encourage a participatory environment by allowing the candidate to pose questions throughout the process.
- Given that backend candidates may sometimes need to collaborate with front-end teams, it is advantageous for them to have a foundational understanding of the principles that govern user interface and user experience design.
Furthermore, adhering to standard interview protocols remains crucial during backend interview sessions – adjust the complexity of interview questions based on the candidate’s evolving expertise, provide timely feedback regarding their status in the recruitment process, and offer sufficient opportunities for candidates to discuss the evaluation or delve into the nuances of collaborating with your team.