Spring Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Spring developers for your organization.
Spring
Spring is a Java-based back-end framework popular for its modular architecture, feature-rich dependency injection, and comprehensive ecosystem with projects like Spring Boot, Spring Security, and Spring Data.
According to the CoderPad 2024 Developer survey, Spring is the 2nd most in-demand back-end framework among technical recruiters and hiring managers.
To evaluate the Spring expertise of developers during coding interviews, below you’ll find hands-on coding challenges and interview questions.
Additionally, we have outlined a set of suggested practices to ensure that your interview questions accurately measure the candidates’ Spring skillset.
Table of Contents
Spring example question
Create a Spring CRUD API
The goal of this exercise is to retrieve data from an external source, store it in an appropriate database structure, and create a CRUD RESTful API to interface with the database
Goals
1. Read the data from this graphql endpoint: https://swapi-graphql.netlify.app/.netlify/functions/index with the following query:
query Query {allPlanets{planets{name population terrains climates}}}
(You can view the shape of the data here.)
2. Store the data from the graphql endpoint into the database and create appropriate models
3. Write RESTful Create, Read, Update, and Delete endpoints to interact with the database
Spring skills to assess
Jobs using Spring
Junior Spring interview questions
Question:
Explain the concept of Inversion of Control (IoC) and Dependency Injection (DI) in the context of the Spring framework.
Answer:
Inversion of Control (IoC) and Dependency Injection (DI) are fundamental concepts in the Spring framework.
IoC is a design principle that shifts the responsibility of object creation and management from the application to a container or framework. In traditional programming, objects are responsible for creating and managing their dependencies. In contrast, with IoC, the framework takes charge of creating and managing objects (beans) and their dependencies.
Dependency Injection is an implementation of IoC, where the dependencies of a class (e.g., other classes or services it relies on) are “injected” into the class instead of being created by the class itself. Spring provides various ways of performing DI, such as constructor injection, setter injection, and field injection.
With DI, classes become more loosely coupled, as they don’t need to know how their dependencies are created. This promotes better separation of concerns and makes testing and maintenance easier.
Question:
Provide an example of how to configure a simple bean in Spring using XML configuration.
Answer:
Here’s an example of configuring a simple bean named “userService” in Spring using XML configuration:
<!-- applicationContext.xml -->
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- Define the userService bean -->
<bean id="userService" class="com.example.UserService">
<!-- Setter injection for dependency -->
<property name="userRepository" ref="userRepository"/>
</bean>
<!-- Define the userRepository bean -->
<bean id="userRepository" class="com.example.UserRepository"/>
</beans>
Code language: HTML, XML (xml)
In this example, we define two beans: “userService” and “userRepository”. The “userService” bean depends on the “userRepository” bean, which is injected using setter injection.
Question:
Explain the purpose of the Spring MVC framework and its components.
Answer:
Spring MVC (Model-View-Controller) is a framework provided by Spring for building web applications in Java. Its purpose is to separate the concerns of web application development by using the MVC architectural pattern.
The components of Spring MVC are as follows:
- Model: Represents the application data and business logic. It holds the state of the application and provides data to the View for rendering.
- View: Represents the presentation layer of the application. It is responsible for rendering data from the Model to the user. In Spring MVC, the View is often implemented using JSP (JavaServer Pages), Thymeleaf, or other templating engines.
- Controller: Handles user requests and orchestrates the interaction between the Model and the View. It receives requests from the user, processes them, and updates the Model accordingly. Then, it selects the appropriate View to render the updated Model data to the user.
By separating concerns in this way, Spring MVC promotes a clean and modular design, making it easier to maintain and extend web applications.
Question:
Provide an example of a Spring MVC Controller that handles an HTTP GET request to fetch user details.
Answer:
Here’s an example of a Spring MVC Controller that handles an HTTP GET request to fetch user details:
@Controller
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/{id}")
public String getUserDetails(@PathVariable Long id, Model model) {
User user = userService.getUserById(id);
model.addAttribute("user", user);
return "user-details";
}
}
Code language: PHP (php)
In this example, we define a UserController
class as a Spring @Controller
, which handles requests mapped to the “/user” path. The getUserDetails()
method handles the HTTP GET requests with an ID path variable.
The UserService
is autowired to fetch user details from the database. The user details are then added to the Model
using model.addAttribute()
and passed to the “user-details” view template for rendering.
Question:
Explain the purpose of Spring Data JPA and its advantages.
Answer:
Spring Data JPA is a subproject of Spring that simplifies the interaction with the database using the Java Persistence API (JPA). It aims to reduce boilerplate code and provide a higher-level abstraction for working with databases.
Advantages of Spring Data JPA include:
- Reduces boilerplate code: Spring Data JPA eliminates the need to write repetitive data access code, such as creating queries and managing transactions.
- Automatic query generation: Spring Data JPA can automatically generate queries based on method names defined in the repository interface. This reduces the need to write explicit SQL queries.
- Abstraction over different data sources: Spring Data JPA abstracts away the underlying data source, allowing developers to switch between different databases (e.g., MySQL, PostgreSQL) easily.
- Pagination and sorting: Spring Data JPA provides built-in support for pagination and sorting of query results.
- Improved testability: By using interfaces for repositories, it becomes easier to mock data access during testing.
Overall, Spring Data JPA simplifies database access and improves developer productivity.
Question:
Provide an example of a Spring Data JPA repository interface that performs a custom query.
Answer:
Here’s an example of a Spring Data JPA repository interface that performs a custom query:
public interface UserRepository extends JpaRepository<User, Long> {
@Query("SELECT u FROM User u WHERE u.age > :age")
List<User> findUsersByAgeGreaterThan(@Param("age") int age);
}
Code language: PHP (php)
In this example, we define a custom query using the @Query
annotation within the UserRepository
interface. The query retrieves users whose age is greater than a specified value. The method name findUsersByAgeGreaterThan
is used to generate the method implementation for this query.
Question:
Explain the concept of AOP (Aspect-Oriented Programming) in the context of Spring and its use cases.
Answer:
Aspect-Oriented Programming (AOP) is a programming paradigm that allows developers to modularize cross-cutting concerns, which are concerns that span multiple parts of an application. In the context of Spring, AOP enables the separation of core business logic from cross-cutting concerns, such as logging, security, and transaction management.
AOP achieves this separation by defining aspects, which are modules that encapsulate cross-cutting concerns. Aspects are then applied to target objects or methods using a technique called weaving. Spring provides various ways to define aspects, including using XML configuration, annotations, or Java configuration.
Use cases for AOP in Spring include:
- Logging: AOP can be used to add logging functionality to methods without modifying their source code.
- Security: AOP can enforce security checks (e.g., authentication, authorization) across multiple methods in a consistent manner.
- Transaction management: AOP can manage transactions by automatically starting, committing, or rolling back transactions based on method execution.
- Caching: AOP can be used to cache method results to improve performance.
- Error handling: AOP can handle exceptions in a central location, providing a consistent error handling mechanism.
Question:
Provide an example of how to use Spring’s @Transactional
annotation to manage transactions in a service class.
Answer:
Here’s an example of how to use Spring’s @Transactional
annotation to manage transactions in a service class:
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Transactional
public User createUser(User user) {
return userRepository.save(user);
}
@Transactional(readOnly = true)
public User getUserById(Long id) {
return userRepository.findById(id).orElse(null);
}
@Transactional
public void deleteUser(Long id) {
userRepository.deleteById(id);
}
}
Code language: CSS (css)
In this example, the UserService
class is annotated with @Service
to indicate that it is a Spring service bean.
The createUser()
and deleteUser()
methods are annotated with @Transactional
to indicate that they are transactional methods. The createUser()
method inserts a new user into the database using the userRepository.save()
method. The deleteUser()
method deletes a user from the database using the userRepository.deleteById()
method.
The getUserById()
method is annotated with @Transactional(readOnly = true)
to indicate that it is a read-only transactional method. It retrieves a user from the database using the userRepository.findById()
method.
Spring will manage transactions for these methods automatically. If an exception occurs during the transaction, Spring will automatically rollback the transaction, ensuring data consistency.
Question:
Explain the purpose of Spring Security and its core features.
Answer:
Spring Security is a powerful security framework that provides authentication, authorization, and other security features for Spring-based applications. Its purpose is to secure web applications and APIs by protecting against various security threats.
Core features of Spring Security include:
- Authentication: Spring Security provides various authentication mechanisms, such as form-based authentication, HTTP Basic authentication, and OAuth. It enables users to log in and prove their identity to access protected resources.
- Authorization: Spring Security manages user roles and permissions to control access to different parts of the application. It ensures that only authorized users can perform specific actions or view certain pages.
- Cross-Site Request Forgery (CSRF) protection: Spring Security automatically protects against CSRF attacks by adding CSRF tokens to forms.
- Session management: Spring Security handles user session management, including session fixation protection and session concurrency control.
- Remember-me functionality: Spring Security allows users to be remembered after a successful login using cookies or tokens.
- Method-level security: Spring Security can secure individual methods based on user roles, providing fine-grained access control.
By integrating Spring Security into a Spring application, developers can add robust security features without reinventing the wheel.
Question:
Provide an example of configuring Spring Security to secure a RESTful API with JWT (JSON Web Tokens) authentication.
Answer:
Here’s an example of how to configure Spring Security to secure a RESTful API with JWT authentication:
- Add the necessary dependencies to your project:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
<version>0.9.1</version>
</dependency>
Code language: HTML, XML (xml)
- Create a JWTUtil class to generate and parse JWT tokens:
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
import org.springframework.stereotype.Component;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
@Component
public class JwtUtil {
private final String secret = "your-secret-key";
private final long expirationTime = 3600000; // 1 hour
public String generateToken(String username) {
Date now = new Date();
Date expiryDate = new Date(now.getTime() + expirationTime);
Map<String, Object> claims = new HashMap<>();
claims.put("sub", username);
return Jwts.builder()
.setClaims(claims)
.setIssuedAt(now)
.setExpiration(expiryDate)
.signWith(SignatureAlgorithm.HS512, secret)
.compact();
}
public String getUsernameFromToken(String token) {
Claims claims = Jwts.parser()
.setSigningKey(secret)
.parseClaimsJws(token)
.getBody();
return claims.getSubject();
}
}
Code language: JavaScript (javascript)
- Configure Spring Security to use JWT authentication:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.config.http.SessionCreationPolicy;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private JwtAuthenticationEntryPoint jwtAuthenticationEntryPoint;
@Autowired
private JwtRequestFilter jwtRequestFilter;
@Autowired
private CustomUserDetailsService customUserDetailsService;
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(customUserDetailsService).passwordEncoder(passwordEncoder());
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
@Bean
@Override
public AuthenticationManager authenticationManagerBean() throws Exception {
return super.authenticationManagerBean();
}
@Override
protected void configure(HttpSecurity httpSecurity) throws Exception {
httpSecurity.csrf().disable()
.authorizeRequests().antMatchers("/authenticate").permitAll()
.anyRequest().authenticated()
.and().exceptionHandling().authenticationEntryPoint(jwtAuthenticationEntryPoint)
.and().sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS);
httpSecurity.addFilterBefore(jwtRequestFilter, UsernamePasswordAuthenticationFilter.class);
}
}
Code language: JavaScript (javascript)
In this example, we disable CSRF protection since it’s not needed for stateless JWT authentication. We permit all requests to “/authenticate”, where the client can request a JWT token by providing valid credentials.
The JwtAuthenticationEntryPoint
is a custom class that handles authentication errors and sends appropriate responses.
The JwtRequestFilter
is a custom filter that intercepts incoming requests and validates the JWT token. It sets the authenticated user in the security context if the token is valid.
The CustomUserDetailsService
is a custom implementation of Spring’s UserDetailsService
interface, which loads user details from the database.
With this configuration, your RESTful API is secured using JWT authentication. Clients must include a valid JWT token in the Authorization header to access protected endpoints.
Intermediate Spring interview questions
Question:
Explain the concept of inversion of control (IoC) and dependency injection in the context of the Spring framework.
Answer:
Inversion of Control (IoC) and Dependency Injection (DI) are fundamental concepts in the Spring framework.
IoC is a design pattern where the control over the flow of the application is shifted from the application code to a container. In traditional programming, the application code is responsible for creating and managing the objects it depends on. However, with IoC, the responsibility of object creation and dependency management is delegated to a container, which manages the lifecycle of objects and their dependencies.
Dependency Injection is a specific implementation of IoC. It is the process of providing the dependent objects (dependencies) to a class instead of the class creating them itself. Instead of hard-coding the dependencies, the container injects them at runtime, allowing for loose coupling between classes.
In the Spring framework, DI is achieved through constructor injection or setter injection. Constructor injection involves passing the dependencies as parameters to a class’s constructor, while setter injection involves setting the dependencies using setter methods.
Question:
The following Spring bean configuration is intended to define a bean of the UserService
class and inject a dependency of the UserRepository
class. However, the configuration contains a syntax error and doesn’t work correctly. Identify the error and fix the configuration.
<bean id="userService" class="com.example.UserService">
<property name="userRepository" ref="userRepo" />
</bean>
<bean id="userRepo" class="com.example.UserRepository" />
Code language: HTML, XML (xml)
Answer:
The error in the configuration is that the <property>
element is used to inject the dependency, but the UserService
class doesn’t have a corresponding setter method for the userRepository
property.
To fix the code, we need to use constructor injection instead. Here’s the corrected configuration:
<bean id="userService" class="com.example.UserService">
<constructor-arg ref="userRepo" />
</bean>
<bean id="userRepo" class="com.example.UserRepository" />
Code language: HTML, XML (xml)
In this corrected configuration, we use <constructor-arg>
to inject the dependency into the UserService
constructor.
Question:
Explain the concept of Spring AOP (Aspect-Oriented Programming) and provide an example scenario where AOP can be beneficial.
Answer:
Spring AOP is a programming paradigm that allows cross-cutting concerns (such as logging, security, and transaction management) to be modularized and separated from the core business logic of an application. It achieves this by dynamically applying aspects (modules) to specific join points (points in the application’s execution flow) using proxy-based or bytecode weaving techniques.
An example scenario where AOP can be beneficial is logging. Instead of adding logging code manually to each method in multiple classes, you can use AOP to apply a logging aspect to all methods or specific methods across multiple classes. This way, logging concerns are centralized and kept separate from the core functionality, leading to cleaner and more maintainable code.
Question:
The following Spring Boot application class is intended to define the main application class. However, it contains a logical error, and the application doesn’t run correctly. Identify the error and fix the code.
@SpringBootApplication
public class MyApplication {
public static void main() {
SpringApplication.run(MyApplication.class);
}
}
Code language: PHP (php)
Answer:
The logical error in the code is that the main
method signature is incorrect. The main
method should accept a String[]
argument, which represents the command-line arguments. Also, the run
method should have the MyApplication.class
as the first argument.
Here’s the corrected code:
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
Code language: PHP (php)
In this corrected code, the main
method accepts the String[] args
argument, and the run
method is called with MyApplication.class
and the args
argument.
Question:
Explain the purpose of Spring Bean Scopes and provide an example of each scope.
Answer:
Spring Bean Scopes define the lifecycle and visibility of Spring beans managed by the Spring IoC container. Different bean scopes determine when and how many instances of a bean are created and used within the application context.
The following are the common Spring bean scopes:
- Singleton Scope: It creates a single instance of the bean and shares that instance across the application context. It is the default scope.
@Component // Equivalent to @Scope("singleton")
public class SingletonBean {
// Bean implementation
}
Code language: PHP (php)
- Prototype Scope: It creates a new instance of the bean whenever requested. Each time a bean is injected or retrieved from the container, a new instance is provided.
@Component
@Scope("prototype")
public class PrototypeBean {
// Bean implementation
}
Code language: PHP (php)
- Request Scope: It creates a new instance of the bean for each HTTP request in a web application.
@Component
@Scope("request")
public class RequestBean {
// Bean implementation
}
Code language: PHP (php)
- Session Scope: It creates a single instance of the bean per user session in a web application.
@Component
@Scope("session")
public class SessionBean {
// Bean implementation
}
Code language: PHP (php)
- Application Scope: It creates a single instance of the bean for the entire web application context.
@Component
@Scope("application")
public class ApplicationBean {
// Bean implementation
}
Code language: PHP (php)
Question:
The following Spring Data JPA repository is intended to define a custom query method to retrieve users by their email address. However, it contains a logical error, and the method doesn’t work correctly. Identify the error and fix the code.
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
User findByEmail(String email);
}
Code language: PHP (php)
Answer:
The logical error in the code is that the method name doesn’t follow the correct naming convention for a custom query method.
To fix the code, we need to use the correct naming convention for the custom query method. The method name should start with “find” followed by the property name, and “By” followed by the property name for the criteria.
Here’s the corrected code:
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
User findByEmail(String email);
}
Code language: PHP (php)
In this corrected code, the findByEmail
method follows the correct naming convention and correctly retrieves users by their email address.
Question:
Explain the purpose of the @Transactional
annotation in Spring and its significance in managing database transactions.
Answer:
The @Transactional
annotation in Spring is used to mark a method or class as transactional. It indicates that the annotated method (or all methods within the annotated class) should be executed within a database transaction.
Transactional management ensures that all database operations within a transaction are treated as a single unit of work. If any operation within the transaction fails, the entire transaction is rolled back, and the database returns to its original state, preventing data inconsistency.
When a method is annotated with @Transactional
, Spring dynamically creates a proxy around the method. The proxy intercepts the method calls and handles the transactional behavior, such as beginning a new transaction before the method is invoked
and committing or rolling back the transaction after the method completes.
For example:
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Transactional
public void updateUser(User user) {
// Some business logic
userRepository.save(user);
// Other operations
}
}
Code language: PHP (php)
In this example, the updateUser
method is marked as @Transactional
, so all the operations within this method will be executed within a single transaction.
Question:
The following Spring REST Controller is intended to handle HTTP POST requests to create a new user. However, it contains a logical error, and the request doesn’t work correctly. Identify the error and fix the code.
@RestController
public class UserController {
@Autowired
private UserService userService;
@PostMapping("/users")
public ResponseEntity<String> createUser(@RequestBody User user) {
userService.createUser(user);
return ResponseEntity.ok("User created successfully.");
}
}
Code language: CSS (css)
Answer:
The logical error in the code is that the createUser
method of the userService
is not handling any potential exceptions that might occur during the user creation process.
To fix the code, we need to handle exceptions appropriately and return an appropriate response in case of errors. We can use Spring’s try-catch
mechanism or let Spring handle the exceptions and return an error response automatically.
Here’s a possible way to handle exceptions:
@RestController
public class UserController {
@Autowired
private UserService userService;
@PostMapping("/users")
public ResponseEntity<String> createUser(@RequestBody User user) {
try {
userService.createUser(user);
return ResponseEntity.ok("User created successfully.");
} catch (Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("Failed to create user.");
}
}
}
Code language: PHP (php)
In this corrected code, we added a try-catch
block to handle any exceptions that may occur during user creation. If an exception occurs, we return an internal server error response with an appropriate message.
Question:
Explain the purpose of Spring Security and its significance in securing web applications.
Answer:
Spring Security is a powerful and customizable framework provided by Spring for securing Java-based applications, including web applications. It is designed to handle various aspects of application security, such as authentication, authorization, and protection against common security vulnerabilities.
The key features and benefits of Spring Security include:
- Authentication: Spring Security provides authentication mechanisms to verify the identity of users. It supports various authentication methods, such as form-based login, HTTP basic authentication, and OAuth.
- Authorization: Spring Security enables fine-grained control over what resources a user can access within the application. It supports role-based and permission-based access control.
- Protection against Security Threats: Spring Security helps protect web applications against common security threats, such as Cross-Site Request Forgery (CSRF), Cross-Site Scripting (XSS), and Clickjacking, by providing built-in countermeasures.
- Integration with Spring Framework: Spring Security seamlessly integrates with the Spring ecosystem, making it easier to secure applications that use other Spring modules, such as Spring Boot and Spring Data.
- Customizability: Spring Security is highly customizable and extensible. It allows developers to implement their own authentication and authorization logic and integrate with existing security infrastructures.
By using Spring Security, developers can focus on building application features, knowing that the security concerns are well-handled by the framework.
Question:
The following Spring Boot application.properties file is intended to configure the database connection and JPA settings for a MySQL database. However, it contains a logical error, and the application fails to establish a database connection. Identify the error and fix the code.
# Database Configuration
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=myuser
spring.datasource.password=mypassword
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
# JPA Configuration
spring.jpa.show-sql=true
spring.jpa.hibernate.ddl-auto=create
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL5Dialect
Code language: PHP (php)
Answer:
The logical error in the code is that the spring.datasource.driver-class-name
property is using the incorrect class name for the MySQL driver. The com.mysql.jdbc.Driver
driver class is used for older versions of MySQL Connector/J. For newer versions, the driver class should be com.mysql.cj.jdbc.Driver
.
To fix the code, we need to update the spring.datasource.driver-class-name
property with the correct driver class name.
Here’s the corrected application.properties
:
# Database Configuration
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=myuser
spring.datasource.password=mypassword
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
# JPA Configuration
spring.jpa.show-sql=true
spring.jpa.hibernate.ddl-auto=create
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL5Dialect
Code language: PHP (php)
In this corrected code, the spring.datasource.driver-class-name
property uses the correct driver class name, com.mysql.cj.jdbc.Driver
, ensuring that the application can establish a database connection.
Senior Spring interview questions
Question:
Explain the concept of dependency injection in the context of Spring framework and discuss its benefits in software development.
Answer:
Dependency injection (DI) is a design pattern used in the Spring framework to achieve loose coupling between classes. In DI, the dependencies of a class are injected into it from the outside rather than the class creating its dependencies internally. This allows for more flexibility, maintainability, and testability of the code.
In Spring, DI is achieved through inversion of control (IoC) containers, which manage the creation and lifecycle of beans (objects) and their dependencies. Spring provides different ways to perform DI, such as constructor injection, setter injection, and autowiring.
Benefits of Dependency Injection in software development:
- Decoupling: DI promotes loose coupling between classes, as classes are not responsible for creating their dependencies. This allows components to be easily replaced or modified without affecting the entire system.
- Testability: By injecting dependencies, it becomes easier to mock or stub them during unit testing. This enables isolated testing of components, leading to more reliable and comprehensive test suites.
- Reusability: With DI, components become more independent and can be reused in different contexts or applications.
- Maintainability: DI improves code maintainability by reducing the entanglement between classes. Changes to dependencies can be managed in a centralized manner through the IoC container.
- Configurability: DI allows for external configuration of dependencies, making it easier to adapt the application to different environments or use cases.
- Modularization: DI encourages a modular design, where each component focuses on its specific functionality, promoting better code organization.
Question:
Provide an example of how to define a bean (component) in Spring using annotations.
Answer:
In Spring, you can define a bean (component) using annotations such as @Component
, @Service
, @Repository
, or @Controller
. These annotations are used to indicate that a class should be managed as a Spring bean.
Example of defining a bean using @Component
:
import org.springframework.stereotype.Component;
@Component
public class MyService {
// Class implementation here
}
Code language: JavaScript (javascript)
In this example, the MyService
class is annotated with @Component
, indicating that it should be managed as a Spring bean. The bean will be automatically detected and registered by the Spring IoC container.
Question:
Explain the purpose of the @Autowired
annotation in Spring and provide an example of its usage.
Answer:
The @Autowired
annotation in Spring is used to automatically inject dependencies into a Spring bean. It allows Spring to resolve and inject the required dependencies at runtime, without the need for explicit configuration.
Example of using @Autowired
:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class MyService {
private final MyRepository repository;
@Autowired
public MyService(MyRepository repository) {
this.repository = repository;
}
// Class implementation here
}
Code language: PHP (php)
In this example, the MyService
class is annotated with @Service
, indicating that it is a Spring bean. The constructor of MyService
is annotated with @Autowired
, specifying that an instance of MyRepository
should be injected into the constructor. Spring will automatically resolve the dependency and inject the appropriate MyRepository
bean when creating the MyService
bean.
Question:
Explain the concept of aspect-oriented programming (AOP) in the context of Spring and discuss its benefits.
Answer:
Aspect-Oriented Programming (AOP) is a programming paradigm used in Spring to address cross-cutting concerns, such as logging, security, and transaction management, which often span multiple modules or layers of an application. AOP allows you to modularize these concerns, making the codebase cleaner and more maintainable.
In Spring, AOP is achieved through aspects, which are separate modules that encapsulate behavior. Aspects are woven into the application’s core business logic at runtime, allowing for the separation of concerns without modifying the original code.
Benefits of Aspect-Oriented Programming in Spring:
- Modularity: AOP promotes a modular design, where cross-cutting concerns are isolated from the core business logic. This results in more maintainable and scalable code.
- Reusability: Aspects can be reused across different components, enabling consistent behavior across the application.
- Decoupling: AOP helps in decoupling cross-cutting concerns from the application logic. Changes to aspects do not require modifications to the core business logic.
- Readability: By removing cross-cutting concerns from the main code, the core business logic becomes more focused and easier to read.
- Debugging: AOP allows developers to focus on the core business logic during debugging, as cross-cutting concerns are separated.
Question:
Provide an example of using Spring’s @Transactional
annotation to manage transactions in a service layer.
Answer:
The @Transactional
annotation in Spring is used to manage transactions for methods in the service layer. It ensures that the annotated method runs within a transactional context, and if any exception occurs, the transaction is rolled back.
Example of using @Transactional
:
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class MyService {
private final MyRepository repository;
public MyService(MyRepository repository) {
this.repository = repository;
}
@Transactional
public void updateData(Data data) {
// Perform data update operations using repository methods
repository.save(data);
}
}
Code language: PHP (php)
In this example, the updateData()
method in the MyService
class is annotated with @Transactional
. This means that all database operations performed within this method will be executed within a single transaction. If the method completes successfully, the transaction will be committed. If an exception occurs, the transaction will be rolled back, ensuring data consistency.
Question:
Explain the difference between singleton and prototype bean scopes in Spring.
Answer:
In Spring, beans can have different scopes that define the lifecycle and visibility of the bean instance. The two most commonly used scopes are singleton and prototype.
- Singleton Scope:
- Singleton is the default scope in Spring.
- In singleton scope, Spring creates and maintains a single instance of the bean throughout the application context.
- Every time the bean is requested, Spring returns the same instance.
- This can lead to potential thread safety issues if the bean is mutable and shared across multiple threads.
- Example:
@Scope("singleton")
- Prototype Scope:
- In prototype scope, Spring creates a new instance of the bean every time it is requested.
- The lifecycle of the prototype bean is managed by the client, and Spring does not manage its destruction.
- This scope is useful for stateful or heavy-weight beans that need to be independent of each other.
- Example:
@Scope("prototype")
Example of defining bean scopes in Spring:
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
@Component
@Scope("singleton")
public class SingletonBean {
// Singleton bean implementation
}
@Component
@Scope("prototype")
public class PrototypeBean {
// Prototype bean implementation
}
Code language: JavaScript (javascript)
In this example, SingletonBean
is defined with the @Scope("singleton")
annotation, while PrototypeBean
is defined with the @Scope("prototype")
annotation.
Question:
Explain the purpose of Spring’s @Qualifier
annotation and provide an example of its usage.
Answer:
The @Qualifier
annotation in Spring is used to resolve ambiguities when multiple beans of the same type are defined in the application context. When autowiring dependencies using @Autowired
, Spring needs to know which bean to inject when there are multiple candidates.
Example of using @Qualifier
:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.stereotype.Service;
@Service
public class MyService {
private final MyRepository repository;
@Autowired
public MyService(@Qualifier("myRepositoryImpl") MyRepository repository) {
this.repository = repository;
}
// Class implementation here
}
Code language: JavaScript (javascript)
In this example, suppose there are multiple implementations of the MyRepository
interface in the application context. By using @Qualifier("myRepositoryImpl")
, we specify that the MyService
should be injected with the bean named “myRepositoryImpl.” This resolves the ambiguity and ensures that the correct bean is injected.
Question:
Explain the concept of Spring profiles and provide an example of how to use them in a Spring Boot application.
Answer:
Spring profiles are used to define different configurations for the same application based on specific environments or scenarios. Profiles allow you to segregate bean definitions, properties, and other configuration elements based on the active profile.
Example of using Spring profiles in a Spring Boot application:
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Profile;
@Configuration
public class MyConfiguration {
@Bean
@Profile("dev")
public MyService devService() {
return new MyDevService();
}
@Bean
@Profile("prod")
public MyService prodService() {
return new MyProdService();
}
}
Code language: CSS (css)
In this example, two different implementations of MyService
are defined, one for the “dev” profile and another for the “prod” profile. The @Profile
annotation is used to associate each bean with a specific profile. When the application is started with a specific profile (e.g., using -Dspring.profiles.active=dev
as a command-line argument), only the beans associated with that profile will be created and used.
Question:
Explain the purpose of Spring Boot Actuator and provide an example of how to enable and use it in a Spring Boot application.
Answer:
Spring Boot Actuator is a subproject of Spring Boot that provides several production-ready features to help monitor and manage Spring Boot applications. It exposes various endpoints that provide useful information and insights into the application’s internals, such as health status, metrics, environment properties, and more.
To enable Spring Boot Actuator, you need to include the spring-boot-starter-actuator
dependency in your application’s pom.xml
or build.gradle
.
Example of enabling Spring Boot Actuator in a Spring Boot application:
- Add the
spring-boot-starter-actuator
dependency to yourpom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Code language: HTML, XML (xml)
- By default, all Actuator endpoints are secured. To allow access to the Actuator endpoints without authentication, add the following to your
application.properties
:
management.endpoints.web.exposure.include=*
Code language: PHP (php)
After enabling Actuator, you can access various endpoints, for example:
/actuator/health
: Provides information about the health of the application./actuator/metrics
: Provides various metrics about the application, such as memory usage and request counts./actuator/env
: Provides information about the environment properties./actuator/info
: Provides custom application information defined inapplication.properties
.
For example, to access the health endpoint, you can make a GET request to http://localhost:8080/actuator/health
.
Question:
Explain the concept of Spring Security and its role in securing a Spring Boot application.
Answer:
Spring Security is a powerful framework provided by Spring to handle security aspects of a Spring-based application. It offers comprehensive security features, such as authentication, authorization, and protection against common security vulnerabilities like Cross-Site Request Forgery (CSRF) and Cross-Site Scripting (XSS).
Role of Spring Security in securing a Spring Boot application:
- Authentication: Spring Security provides authentication mechanisms to verify the identity of users. It supports various authentication methods, such as form-based login, HTTP Basic authentication, and OAuth.
- Authorization: Once authenticated, Spring Security enables you to define access control rules based on roles and permissions. It ensures that only authorized users can access specific resources.
- Protection against common attacks: Spring Security helps protect the application against security vulnerabilities like CSRF attacks, XSS attacks, and Clickjacking.
- User session management: Spring Security manages user sessions and provides options for session fixation protection and session invalidation.
- Secure password handling: Spring Security provides utilities for securely storing and handling user passwords, such as password hashing and salting.
Example of configuring Spring Security in a Spring Boot application:
To enable Spring Security in a Spring Boot application, you need to include the spring-boot-starter-security
dependency in your pom.xml
or build.gradle
. After enabling Spring Security, it automatically secures your application with basic authentication, and you will need to provide a username and password to access your application.
By default, Spring Security will generate a random password during startup and log it. You can find the generated password in the application logs and use it to log in. For production applications, it is essential to set a secure password explicitly.
Additional configuration for customizing authentication and authorization can be done through Java-based configuration or using Spring Security’s configuration properties in application.properties
or application.yml
.
1,000 Companies use CoderPad to Screen and Interview Developers
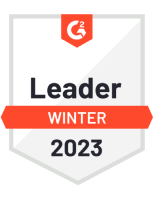
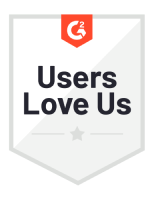
Interview best practices for Spring roles
For successful Spring interviews, it’s important to consider various factors, such as the candidate’s experience level and the engineering role. To ensure that your Spring interview questions yield the best results, we recommend following these best practices when working with candidates:
- Create technical questions that align with actual business cases within your organization. This will not only be more engaging for the candidate but also enable you to better assess the candidate’s suitability for your team.
- Encourage the candidate to ask questions during the interview and foster a collaborative environment.
- Make sure your Spring candidates are familiar with using application servers like Apache Tomcat, JBoss, or WebLogic.
- Verify that the candidate has a minimal knowledge of all the different Spring layers (Core Container, Data Access/Integration, Web, AOP and Instrumentation, …).
Additionally, adhering to standard interview etiquette when conducting Spring interviews is essential. This includes adjusting the question complexity to the candidate’s level of development skills, providing timely feedback to candidates about their application status, and allowing candidates to ask questions about the assessment or working with you and your team.