Django Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Django developers for your organization.
Django
Django is a Python-based back-end framework known for it’s emphasis on promoting code reusability (the Don’t Repeat Yourself principle), clear & extensive developer documentation, and ability to quickly scale.
According to the CoderPad 2024 Developer survey, Django is the 3rd most in-demand back-end framework among technical recruiters and hiring managers.
To evaluate the Django expertise of developers during coding interviews, below you’ll find hands-on coding challenges and interview questions. Additionally, we have outlined a set of suggested practices to ensure that your interview questions accurately measure the candidates’ Django skillset.
Table of Contents
Django example question
Create a polling application
Here is a simple but realistic Django project, which allows people to vote on polls.
You have two simple tasks to perform on this project:
- Add a database migration to populate the database with a poll of your choice.
- Edit the source code so that people can vote for multiple options instead of just one.
Feel free to take some time to familiarize yourself with the environment before starting the assignment.
Django skills to assess
Jobs using Django
Junior Django interview questions
Question:
The following Django view function is giving an error. Identify and fix the issue.
from django.http import HttpResponse
def my_view(request):
return HttpResponse("Hello, Django!")
Code language: JavaScript (javascript)
Answer:
The code seems fine. However, make sure that you have the necessary Django dependencies installed and that the urlpatterns
in your project’s urls.py
file is properly configured to route the request to this view function.
Question:
What is the purpose of Django’s ORM (Object-Relational Mapping)?
Answer:
Django’s ORM provides a high-level, Pythonic way to interact with databases. It allows developers to work with database records as Python objects, abstracting away the complexities of SQL queries and database management.
Question:
The following Django model has a mistake. Identify and fix the issue.
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=200)
author = models.ForeignKey('Author', on_delete=models.CASCADE)
publication_date = models.DateField()
Answer:
The code is missing the definition of the Author
model. Make sure to import and define the Author
model before using it as a foreign key in the Book
model.
Question:
Explain the concept of Django’s Migrations.
Answer:
Django’s Migrations are a built-in feature that allows developers to manage database schema changes over time. Migrations help in creating, updating, and deleting database tables, fields, and relationships without manually writing SQL scripts.
Question:
The following Django template code is not rendering the variable correctly. Identify and fix the issue.
<!DOCTYPE html>
<html>
<head>
<title>My Website</title>
</head>
<body>
<h1>Welcome, {{ name }}</h1>
</body>
</html>
Code language: HTML, XML (xml)
Answer:
To render a variable in a Django template, you need to pass a context dictionary to the template rendering function, where the variable name
is defined. Make sure you are passing the context correctly while rendering the template.
Question:
What is the purpose of Django’s URLconf?
Answer:
Django’s URLconf is a module that defines the mapping between URLs and view functions. It helps in routing incoming HTTP requests to the appropriate view functions or class-based views, allowing developers to create clean and maintainable URL structures.
Question:
Fix the code: The following Django form is not saving data to the database. Identify and fix the issue.
from django import forms
from .models import Person
class PersonForm(forms.ModelForm):
class Meta:
model = Person
fields = ('name', 'age', 'email')
Answer:
The code is missing the save()
method in the PersonForm
class. Add the save()
method to save the form data to the database. For example:
class PersonForm(forms.ModelForm):
class Meta:
model = Person
fields = ('name', 'age', 'email')
def save(self, commit=True):
person = super().save(commit=False)
if commit:
person.save()
return person
Question:
What is the purpose of Django’s middleware?
Answer:
Django’s middleware is a mechanism that enables developers to process requests and responses globally across the entire Django application. Middleware can perform operations such as authentication, request/response modification, and error handling.
Question:
The following Django view is not retrieving data from the database correctly. Identify and fix the issue.
from
django.shortcuts import render
from .models import Product
def product_list(request):
products = Product.objects.filter(category='Electronics')
return render(request, 'product_list.html', {'products': products})
Code language: JavaScript (javascript)
Answer:
The code is filtering the Product
objects incorrectly. When comparing string values, use double underscores (__
) to indicate the field lookup. Modify the filter line to the following:
products = Product.objects.filter(category__exact='Electronics')
Code language: JavaScript (javascript)
Question:
Explain the role of Django’s template engine.
Answer:
Django’s template engine is responsible for rendering dynamic web pages by combining templates (HTML files with placeholders) with data from the view functions. It allows developers to separate the presentation logic from the business logic and enables the reuse of templates across different views.
Intermediate Django interview questions
Question:
The following Django view function is throwing an error. Identify and fix the issue.
from django.shortcuts import render
from .models import Post
def post_detail(request, post_id):
post = Post.objects.get(id=post_id)
return render(request, 'post_detail.html', {'post': post})
Code language: JavaScript (javascript)
Answer:
The code is assuming that a Post
object with the given post_id
exists in the database. To handle the case when the Post
object does not exist, you can use the get_object_or_404
shortcut from django.shortcuts
. Import it and modify the view function as follows:
from django.shortcuts import render, get_object_or_404
from .models import Post
def post_detail(request, post_id):
post = get_object_or_404(Post, id=post_id)
return render(request, 'post_detail.html', {'post': post})
Code language: JavaScript (javascript)
Question:
Explain the purpose of Django’s authentication system.
Answer:
Django’s authentication system provides a secure and flexible way to manage user authentication and authorization. It includes features such as user registration, login, logout, password management, and permission-based access control.
Question:
The following Django model has a mistake that is causing a database error. Identify and fix the issue.
from django.db import models
class Category(models.Model):
name = models.CharField(max_length=50, unique=True)
parent = models.ForeignKey('Category', on_delete=models.CASCADE, null=True, blank=True)
Answer:
The ForeignKey
relationship in the Category
model is self-referential, indicating a hierarchical structure. However, the on_delete=models.CASCADE
is not suitable in this case because it would cause the deletion of parent categories to also delete their child categories. Change the on_delete
argument to models.PROTECT
to prevent accidental deletion of parent categories.
parent = models.ForeignKey('Category', on_delete=models.PROTECT, null=True, blank=True)
Code language: PHP (php)
Question:
What are Django signals, and how are they used?
Answer:
Django signals are decoupled notifications that allow certain senders to notify a set of receivers when a particular action occurs. Signals are used to enable decoupled applications, allowing different parts of an application to communicate and respond to events without being tightly coupled to each other.
Question:
The following Django template code is not displaying the looped data correctly. Identify and fix the issue.
<ul>
{% for category in categories %}
<li>{{ category.name }}</li>
{% endfor %}
</ul>
Code language: HTML, XML (xml)
Answer:
The code assumes that the categories
variable in the template context is a list. However, if it is a queryset, you need to iterate over it using the .all
method. Modify the template code as follows:
<ul>
{% for category in categories.all %}
<li>{{ category.name }}</li>
{% endfor %}
</ul>
Code language: HTML, XML (xml)
Question:
Explain the purpose of Django’s middleware classes.
Answer:
Django’s middleware classes provide hooks for processing requests and responses globally across the entire Django application. They allow developers to modify request and response objects, perform authentication, caching, compression, and other operations at various stages of the request/response lifecycle.
Question:
Fix the code: The following Django form is not saving data correctly. Identify and fix the issue.
from django import forms
from .models import UserProfile
class UserProfileForm(forms.ModelForm):
class Meta:
model = UserProfile
fields = ('name', 'email')
def save(self, commit=True):
user_profile = super().save(commit=False)
if commit:
user_profile.save()
return user_profile
Answer:
The code correctly defines the save()
method, but it is missing the commit
argument in the super().save()
call. Modify the save()
method as follows:
def save(self, commit=True):
user_profile = super().save(commit=commit)
return user_profile
Code language: PHP (php)
Question:
What are Django’s class-based views, and what advantages do they offer over function-based views?
Answer:
Django’s class-based views are an alternative way to define views using Python classes instead of functions. They offer advantages such as code reuse, modularity, and the ability to override specific methods to customize behavior at different stages of the view lifecycle. Class-based views also provide built-in mixins for common functionalities, making them highly flexible.
Question:
The following Django view is not handling form validation errors correctly. Identify and fix the issue.
from django.shortcuts import render
from .forms import ContactForm
def contact_view(request):
if request.method == 'POST':
form = ContactForm(request.POST)
if form.is_valid():
# Process the form data
return render(request, 'success.html')
else:
form = ContactForm()
return render(request, 'contact.html', {'form': form})
Code language: PHP (php)
Answer:
The code is missing the case to handle form validation errors. If the form is not valid, it should be re-rendered with the validation errors. Modify the code as follows:
from django.shortcuts import render
from .forms import ContactForm
def contact_view(request):
if request.method == 'POST':
form = ContactForm(request.POST)
if form.is_valid():
# Process the form data
return render(request, 'success.html')
else:
form = ContactForm()
return render(request, 'contact.html', {'form': form})
Code language: PHP (php)
Question:
What is Django’s caching framework, and how can it improve the performance of a web application?
Answer:
Django’s caching framework provides a flexible way to store and retrieve dynamic data, such as database query results or rendered templates, in memory or other caching backends. Caching can significantly improve the performance of a web application by reducing the time required to generate responses and minimizing the load on the underlying resources.
Senior Django interview questions
Question:
The following Django view is performing multiple database queries, which can be optimized. Identify and fix the issue.
from django.shortcuts import render
from .models import Category, Product
def category_products(request, category_id):
category = Category.objects.get(id=category_id)
products = Product.objects.filter(category=category)
return render(request, 'category_products.html', {'category': category, 'products': products})
Code language: JavaScript (javascript)
Answer:
The code can be optimized to perform a single database query using Django’s select_related
method. Modify the view function as follows:
from django.shortcuts import render
from .models import Category, Product
def category_products(request, category_id):
category = Category.objects.select_related('product_set').get(id=category_id)
products = category.product_set.all()
return render(request, 'category_products.html', {'category': category, 'products': products})
Code language: JavaScript (javascript)
Question:
What is Django REST framework, and what are its main features?
Answer:
Django REST framework is a powerful toolkit for building Web APIs in Django. Its main features include support for serialization, authentication, permissions, viewsets, routers, and content negotiation. It simplifies the process of building APIs by providing a set of reusable components and enforcing best practices.
Question:
The following Django model has a mistake that is causing a performance issue. Identify and fix the issue.
from django.db import models
class Order(models.Model):
user = models.ForeignKey('User', on_delete=models.CASCADE)
products = models.ManyToManyField('Product')
created_at = models.DateTimeField(auto_now_add=True)
Answer:
The Order
model is missing an index on the user
field, which could cause performance issues when querying orders by user. Add the db_index=True
option to the user
field to create an index.
user = models.ForeignKey('User', on_delete=models.CASCADE, db_index=True)
Code language: PHP (php)
Question:
Explain the concept of Django’s middleware hooks.
Answer:
Django’s middleware hooks allow developers to process requests and responses at various stages of the request/response lifecycle. Middleware classes can intercept and modify requests before they reach the view, as well as modify responses before they are sent back to the client. Middleware hooks provide a flexible way to implement cross-cutting concerns, such as authentication, caching, and request/response manipulation.
Question:
The following Django template code is not rendering the date correctly. Identify and fix the issue.
<p>The current date is: {{ current_date }}</p>
Code language: HTML, XML (xml)
Answer:
To render the date correctly in the template, use Django’s template tags and filters. Modify the template code as follows:
{% load tz %}
<p>The current date is: {{ current_date|timezone:"America/New_York" }}</p>
Code language: HTML, XML (xml)
This example assumes that you want to display the current date in the “America/New_York” timezone. Adjust the timezone filter to your desired timezone.
Question:
What are Django’s database transactions, and how can they ensure data consistency?
Answer:
Django’s database transactions provide a way to group multiple database operations into a single atomic unit. Transactions ensure data consistency by guaranteeing that either all the operations within the transaction are applied, or none of them are. In case of failures or errors, transactions can be rolled back to restore the database to its original state.
Question:
The following Django form is not properly handling file uploads. Identify and fix the issue.
from django import forms
from .models
import Document
class DocumentForm(forms.ModelForm):
class Meta:
model = Document
fields = ('title', 'file')
Answer:
To properly handle file uploads, the enctype
attribute of the HTML form needs to be set to 'multipart/form-data'
. Modify the form class as follows:
from django import forms
from .models import Document
class DocumentForm(forms.ModelForm):
class Meta:
model = Document
fields = ('title', 'file')
widgets = {
'file': forms.ClearableFileInput(attrs={'multiple': True})
}
In the template, make sure to add the enctype
attribute to the form tag:
<form method="POST" enctype="multipart/form-data">
<!-- Form fields go here -->
</form>
Code language: HTML, XML (xml)
Question:
Explain the purpose of Django’s context processors and provide an example use case.
Answer:
Django’s context processors are functions that add variables to the context of every template rendered. They provide a convenient way to include common context variables across multiple templates. For example, a context processor can add the current user or the site’s configuration settings to the template context, eliminating the need to manually include these variables in each view.
Question:
The following Django view is performing a potentially expensive calculation repeatedly. Identify and fix the issue.
from django.shortcuts import render
def calculate_stats(request):
data = get_expensive_data() # Expensive calculation
stats = {
'average': calculate_average(data),
'max': calculate_max(data),
'min': calculate_min(data),
}
return render(request, 'stats.html', {'stats': stats})
Code language: PHP (php)
Answer:
To avoid performing the expensive calculation multiple times, you can cache the result using Django’s caching framework. Modify the view function as follows:
from django.shortcuts import render
from django.core.cache import cache
def calculate_stats(request):
data = cache.get('expensive_data')
if data is None:
data = get_expensive_data() # Expensive calculation
cache.set('expensive_data', data, 3600) # Cache for 1 hour
stats = {
'average': calculate_average(data),
'max': calculate_max(data),
'min': calculate_min(data),
}
return render(request, 'stats.html', {'stats': stats})
Code language: PHP (php)
This example caches the result of the expensive calculation for 1 hour using Django’s default cache backend. Adjust the caching parameters as per your requirements.
Question:
What are Django’s management commands, and how can they be used?
Answer:
Django’s management commands are custom scripts that can be executed from the command line to perform administrative tasks. They provide a convenient way to automate common operations such as database migrations, data imports/exports, and cron-like tasks. Management commands can be created using Django’s command-line utility (manage.py
) and can be integrated into deployment scripts or scheduled with tools like cron
or Celery
.
More Django interview resources
For more guides on improving your knowledge of Django and acing interviews, we have outlined helpful blog posts below:
- Code Challenge: Django Models
- How To Get Up And Running With Django Migrations: A Guide
- Code Challenge: Django Search Filter
- Logging in Django
- Code Challenge: Django Todo App
- A Guide To API Rate Limiting In Django
1,000 Companies use CoderPad to Screen and Interview Developers
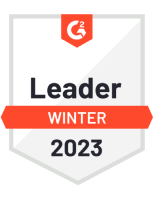
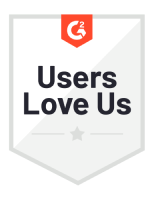
Interview best practices for Django roles
For successful Django interviews, taking into account multiple aspects such as the applicant’s experience level and the engineering position is crucial. To guarantee that your Django interview inquiries produce optimal outcomes, we suggest adhering to the following best practices when engaging with candidates:
- Devise technical queries that correspond with real-world business scenarios within your organization. This approach not only makes the interview more captivating for the applicant but also allows you to more effectively gauge their fit for your team.
- Encourage the candidate to pose questions throughout the interview and cultivate a cooperative atmosphere.
- If using Django as part of a full stack, ensure that your developers also have understanding of front-end technologies like HTML/CSS and of database knowledge, especially with the module Django ORM (object-relational mapper).
Moreover, it is essential to follow conventional interview practices when carrying out Django interviews. This encompasses tailoring the question difficulty according to the applicant’s development skill level, offering prompt feedback regarding their application status, and permitting candidates to inquire about the evaluation or collaborating with you and your team.