Node.js Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Node developers for your organization.
Node.js
The premier back-end JavaScript framework, Node continues to rise in popularity due to its low learning curve, extensive library support, and excellent performance due to its non-blocking input/output design.
According to the CoderPad 2024 Developer survey, Node is THE most in-demand back-end framework among technical recruiters and hiring managers.
To evaluate the Node expertise of developers during coding interviews, we have provided hands-on coding challenges and interview questions below.
Additionally, we have outlined a set of suggested practices to ensure that your interview questions accurately measure the candidates’ Node skillset.
Table of Contents
Node.js example question
Create a NodeJS CRUD API
The goal of this exercise is to retrieve data from an external source, store it in an appropriate database structure, and create a CRUD RESTful API to interface with the database
Goals
1. Read the data from this graphql endpoint: https://swapi-graphql.netlify.app/.netlify/functions/index with the following query:
query Query {allPlanets{planets{name population terrains climates}}}
(View the shape of the data here.)
2. Store the data from the graphql endpoint into the database and create appropriate models
3. Write RESTful Create, Read, Update, and Delete endpoints to interact with the database
Node skills to assess
Jobs using Node
Junior Node.js interview questions
Question:
Explain the concept of Node.js and its role as a server-side JavaScript runtime environment. How does it differ from the client-side JavaScript environment?
Answer:
Node.js is an open-source, cross-platform JavaScript runtime environment that allows developers to run JavaScript code outside the web browser. It is built on the V8 JavaScript engine from Google Chrome and provides a runtime environment for executing JavaScript code on the server-side. Node.js enables developers to build scalable, high-performance, and event-driven applications using JavaScript.
Difference from client-side JavaScript environment:
- Context: In the client-side environment, JavaScript runs within a web browser and has access to the Document Object Model (DOM) to interact with web pages. On the other hand, Node.js runs on the server-side, and it has access to various built-in modules, file system, and network functionalities to perform server-related tasks.
- Execution Environment: Client-side JavaScript is executed by the web browser, which may have limitations and security restrictions due to its sandboxed environment. Node.js, being server-side, runs in a server environment, giving it more access and control over the system resources.
- Application Type: Client-side JavaScript is mainly used for enhancing user interfaces and adding interactivity to web pages. Node.js is used to build server-side applications, APIs, microservices, real-time applications, and other backend services.
- Asynchronous I/O: Node.js utilizes a non-blocking, event-driven I/O model, allowing it to handle concurrent requests efficiently. This is especially useful for applications that deal with multiple concurrent connections, such as web servers and chat applications.
Question:
Explain the significance of the Node Package Manager (NPM) in Node.js development. How do you initialize a new Node.js project and manage dependencies using NPM?
Answer:
The Node Package Manager (NPM) is a crucial tool in Node.js development. It is the default package manager for Node.js, providing a vast ecosystem of reusable packages and modules that developers can use to build applications efficiently. NPM allows developers to manage project dependencies, install and update packages, and share their own packages with the community.
To initialize a new Node.js project and manage dependencies using NPM:
- Initialize a new project: Open your terminal, navigate to the project’s root directory, and run the following command:
npm init
This command will create a package.json
file that holds the project’s metadata and dependencies.
- Install dependencies: After initializing the project, you can use NPM to install external packages. For example, to install the
express
package, run:
npm install express
This will install the express
package and add it as a dependency in the package.json
file.
- Use dependencies in the project: You can now use the installed packages in your Node.js application by requiring them in your code. For example, to use
express
, add the following line in yourapp.js
file:
const express = require('express');
Code language: JavaScript (javascript)
Question:
Explain the concept of modules in Node.js and how they promote code reusability. Provide an example of creating and using a custom module in Node.js.
Answer:
Modules in Node.js are reusable blocks of code that can be encapsulated and exported from one file and imported into another file. They promote code reusability, maintainability, and organization in large Node.js projects.
Here’s an example of creating and using a custom module in Node.js:
- Create a new file named
mathUtils.js
:
// mathUtils.js
exports.add = function (a, b) {
return a + b;
};
exports.subtract = function (a, b) {
return a - b;
};
Code language: JavaScript (javascript)
- Use the custom module in another file:
// main.js
const mathUtils = require('./mathUtils');
const sum = mathUtils.add(10, 5);
const difference = mathUtils.subtract(10, 5);
console.log('Sum:', sum);
console.log('Difference:', difference);
Code language: JavaScript (javascript)
In this example, we create a custom module in mathUtils.js
that exports two functions: add
and subtract
. In main.js
, we require the mathUtils
module and use its functions to perform addition and subtraction operations.
Question:
Explain the concept of asynchronous programming in Node.js. What is the importance of callbacks and how do they handle asynchronous operations?
Answer:
Asynchronous programming in Node.js is a fundamental concept that allows non-blocking execution of code. It enables Node.js to handle multiple tasks simultaneously without waiting for each operation to complete before moving to the next one. Asynchronous programming is crucial for handling I/O operations, such as reading and writing files or making network requests, without blocking the entire application.
Callbacks play a significant role in handling asynchronous operations in Node.js. They are functions passed as arguments to asynchronous functions, and they get executed once the asynchronous operation is completed. Callbacks allow developers to specify what action should be taken after the asynchronous operation finishes.
Here’s an example of using a callback for an asynchronous file read operation:
const fs = require('fs');
fs.readFile('file.txt', 'utf8', function (err, data) {
if (err) {
console.error('Error:', err);
} else {
console.log('File content:', data);
}
});
Code language: JavaScript (javascript)
In this example, fs.readFile()
is an asynchronous function that reads the content of file.txt
. The third argument is a callback function that handles the result of the file read operation. If there’s an error, it will be passed as the first argument to the callback; otherwise, the data read from the file will be passed as the second argument.
Question:
Explain the purpose of the require
function in Node.js and how it facilitates module loading. How do you load core modules, built-in modules, and local modules using require
?
Answer:
The require
function in Node.js is used to load modules and files. It allows you to include external modules, built-in modules, and custom modules in your Node.js application.
To load core modules or built-in modules provided by Node.js, you can use the require
function directly:
const http = require('http');
const fs = require('fs');
const path = require('path');
Code language: JavaScript (javascript)
Core modules are modules that are included by default in Node.js, and you can use them without installing any additional packages.
To load local modules or custom modules created by you or other developers, you need to provide the file path relative to the current file:
Assume you have a file named mathUtils.js
in the same directory as your current file:
const mathUtils = require('./mathUtils');
Code language: JavaScript (javascript)
In this example, we load the custom module mathUtils.js
using require
. The ./
indicates that the module is in the same directory as the current file.
Question:
Explain the concept of the Node.js Event Loop and how it enables non-blocking I/O operations. How does the Event Loop handle asynchronous events in Node.js?
Answer:
The Node.js Event Loop is a core mechanism that enables non-blocking I/O operations in Node.js. It is responsible for handling and processing asynchronous events such as I/O operations, timers, and callbacks. The Event Loop is what makes Node.js highly performant and scalable.
Here’s a simplified overview of how the Node.js Event Loop works:
- Node.js runs the initial synchronous code, and any asynchronous operations (e.g., reading a file) are delegated to the system.
- Asynchronous operations are initiated and move to a separate thread (known as the Thread Pool) managed by libuv, a library that handles asynchronous I/O operations.
- While waiting for the completion of an asynchronous operation, the main Node.js thread continues executing other code and processing new events.
- When an asynchronous operation is completed, the corresponding callback is added to the Event Queue.
- The Event Loop continuously checks the Event Queue for pending callbacks. If there are any, it processes them one by one, executing their respective callback functions.
- The process repeats, allowing Node.js to handle multiple concurrent operations efficiently, providing a non-blocking I/O model.
This mechanism allows Node.js to manage multiple asynchronous operations simultaneously, making it well-suited for handling concurrent requests in web applications and other I/O-intensive tasks.
Question:
Explain the purpose of the fs
module in Node.js and how it enables file system operations. Provide an example of reading and writing files using the fs
module.
Answer:
The fs
module in Node.js is a built-in module that provides file system-related functionalities, allowing developers to work with files and directories. It enables reading, writing, updating, and deleting files, as well as managing directories.
Here’s an example of reading and writing files using the fs
module:
const fs = require('fs');
// Reading a file
fs.readFile('file.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
} else {
console.log('File content:', data);
}
});
// Writing to a file
const contentToWrite = 'This is some content to write to the file.';
fs.writeFile('output.txt', contentToWrite, 'utf8', (err) => {
if (err) {
console.error('Error writing to file:', err);
} else {
console.log('File has been written successfully.');
}
});
Code language: JavaScript (javascript)
In this example, we use the readFile()
method to read the content of a file named file.txt
in UTF-8 encoding. The content is read asynchronously, and the result is passed to the callback function. Similarly, we use the writeFile()
method to write content to a file named output.txt
. The content is written asynchronously, and the callback is used to handle any errors that may occur during the write operation.
Question:
Explain the concept of the Node.js http
module and how it enables building web servers. Provide an example of creating a basic HTTP server using the http
module.
Answer:
The http
module in Node.js is a built-in module that provides functionality to create HTTP servers and make HTTP requests. It enables developers to build web servers that can handle incoming HTTP requests and send responses to clients.
Here’s an example of creating a basic HTTP server using the http
module:
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, Node.js!');
});
const port = 3000;
server.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
Code language: JavaScript (javascript)
In this example, we create an HTTP server using the createServer()
method of the http
module. The server responds to all incoming requests with a simple “Hello, Node.js!” message. The server listens on port 3000, and when it starts, the message “Server is running on http://localhost:3000” is logged to the console.
Question:
Explain the purpose of the npm
command-line tool and its role in managing Node.js packages. How do you use npm
to install, update, and remove packages?
Answer:
The npm
command-line tool is the Node Package Manager, used to manage Node.js packages and modules. It comes bundled with Node.js installation and provides a convenient way to install, update, and remove packages for Node.js projects.
Here’s how you can use npm
to manage packages:
- Install a package: To install a package and add it as a dependency to your project, use the
npm install
command. For example, to install theexpress
package:
npm install express
The package will be downloaded and saved to the node_modules
directory in your project.
- Install a package globally: To install a package globally on your system, use the
-g
flag. For example, to installnodemon
globally:
npm install -g nodemon
Global packages are typically used for development tools and utilities.
- Update a package: To update a package to the latest version, use the
npm update
command followed by the package name. For example, to update theexpress
package:
npm update express
- Remove a package: To remove a package from your project’s dependencies, use the
npm uninstall
command followed by the package name. For example, to remove theexpress
package:
npm uninstall express
These are some of the basic npm
commands that you can use to manage packages in your Node.js projects effectively.
Question:
Explain the concept of middleware in Node.js, and its role in handling HTTP requests in frameworks like Express.js. Provide an example of using middleware in an Express.js application.
Answer:
Middleware in Node.js is a function that sits between the server and the route handlers in a web application. It intercepts HTTP requests and can perform various tasks such as authentication, logging, data parsing, and error handling. Middleware functions have access to the request (req
) and response (res
) objects, and they can modify the request or response before passing it to the next middleware or the route handler.
In Express.js, middleware plays a crucial role in processing incoming requests and preparing the response before sending it back to the client.
Here’s an example of using middleware in an Express.js application:
const express = require('express');
const app = express();
// Logger Middleware
app.use((req, res, next) => {
console.log(`[${new Date().toISOString()}] ${req.method} ${req.url}`);
next();
});
// Body Parser Middleware
app.use(express.json());
// Route Handler
app.get('/', (req, res) => {
res.send('Hello, Express!');
});
// Error Handling Middleware
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something went wrong!');
});
const port = 3000;
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
Code language: JavaScript (javascript)
In this example, we have three middleware functions:
- Logger Middleware: Logs the incoming requests to the console with the current timestamp.
- Body Parser Middleware: Parses the request body as JSON if the content type is ‘application/json’.
- Error Handling Middleware: Captures any errors that occur during request processing and responds with a generic error message and a 500 status code.
Middleware functions are executed in the order they are declared using app.use()
, and they can modify the request, response, or perform actions before passing the control to the next middleware or route handler. Middleware makes Express.js flexible and powerful for building web applications with various functionalities.
Intermediate Node.js interview questions
Question:
Explain the concept of asynchronous programming in Node.js. Provide an example of how you can use callbacks to handle asynchronous operations.
Answer:
Asynchronous programming in Node.js allows executing tasks without blocking the execution of the main program. Instead of waiting for a task to complete before moving on to the next one, Node.js utilizes callbacks and event loops to handle asynchronous operations.
Here’s an example of how you can use callbacks for asynchronous file reading in Node.js:
const fs = require('fs');
// Asynchronous file reading with a callback
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading the file:', err);
} else {
console.log('File content:', data);
}
});
Code language: JavaScript (javascript)
In this example, fs.readFile()
asynchronously reads the content of ‘example.txt’ and calls the provided callback function when the operation is complete. The callback function receives an error (if any) and the file content. This way, the rest of the program can continue executing while the file is being read, enhancing overall performance.
Question:
What is npm in the context of Node.js development? Explain its significance and provide an example of how you can use npm packages in a Node.js application.
Answer:
npm (Node Package Manager) is a package manager for Node.js that allows developers to discover, install, and manage reusable code packages, also known as modules or packages. npm simplifies the process of managing dependencies and enables easy integration of third-party code into Node.js applications.
Here’s an example of how you can use an npm package in a Node.js application:
- Install the npm package:
npm install axios
- Use the package in your Node.js application:
const axios = require('axios');
axios.get('https://api.example.com/data')
.then(response => {
console.log('API response:', response.data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
Code language: JavaScript (javascript)
In this example, we first install the axios
package using npm install axios
. Then, in our Node.js application, we require the axios
module and use it to make an HTTP GET request to an example API. The response data is logged to the console, and any errors are handled with the .catch()
method.
Question:
Explain the concept of middleware in the context of Node.js and how it is commonly used in web applications.
Answer:
Middleware in Node.js refers to functions that are executed in the request-response cycle of a web application. Middleware functions have access to the request and response objects, and they can perform tasks, modify request/response objects, or terminate the request-response cycle.
In web applications, middleware is commonly used for tasks such as authentication, logging, error handling, parsing request bodies, and handling CORS (Cross-Origin Resource Sharing) headers.
Here’s an example of how middleware can be used in a simple Express.js application:
const express = require('express');
const app = express();
// Middleware to log incoming requests
app.use((req, res, next) => {
console.log(`${req.method} ${req.url}`);
next();
});
// Route handling
app.get('/', (req, res) => {
res.send('Hello, World!');
});
// Error handling middleware
app.use((err, req, res, next) => {
console.error('Error:', err);
res.status(500).send('Something went wrong.');
});
app.listen(3000, () => {
console.log('Server is running on port 3000.');
});
Code language: JavaScript (javascript)
In this example, the first middleware logs the incoming requests, and the second middleware handles errors. The next()
function is called to pass control to the next middleware in the chain or the route handler. Middleware allows for modularizing application logic and making the code more maintainable.
Question:
What is the role of the “Event Loop” in Node.js, and how does it enable non-blocking I/O operations?
Answer:
The Event Loop is a crucial component of Node.js that enables non-blocking I/O operations and asynchronous programming. It’s responsible for handling and dispatching events and callbacks in a single-threaded manner, which allows Node.js to efficiently manage multiple I/O operations concurrently without blocking the execution of the main program.
The Event Loop continuously listens for events, such as incoming HTTP requests or file read completions, and adds the associated callbacks to the callback queue. When the current execution stack is empty, Node.js picks up the callbacks from the callback queue and processes them one by one. This process allows the application to be highly responsive and handle multiple requests simultaneously without creating new threads for each request.
Overall, the Event Loop is the key to Node.js’ ability to handle a large number of I/O operations with low overhead, making it well-suited for scalable and high-performance applications.
Question:
Explain the concept of Promises in Node.js and how they help in handling asynchronous operations. Provide an example of using Promises in a Node.js application.
Answer:
Promises in Node.js are a way to handle asynchronous operations in a more organized and readable manner. A Promise represents the eventual completion (or failure) of an asynchronous operation and allows you to attach callbacks to handle the result or error once the operation completes.
Here’s an example of using Promises to read a file asynchronously in Node.js:
const fs = require('fs');
function readFileAsync(filePath) {
return new Promise((resolve, reject) => {
fs.readFile(filePath, 'utf8', (err, data) => {
if (err) {
reject(err);
} else {
resolve(data);
}
});
});
}
readFileAsync('example.txt')
.then(data => {
console.log('File content:', data);
})
.catch(error => {
console.error('Error reading the file:', error);
});
Code language: JavaScript (javascript)
In this example, we create a function readFileAsync()
that returns a Promise. The Promise wraps the fs.readFile()
operation, and when the operation completes, it either resolves with the file content or rejects with an error. We can then use .then()
to handle the successful result and .catch()
to handle any errors, making the code more readable and organized.
Question:
Explain the concept of streams in Node.js and their significance in handling large data efficiently. Provide an example of reading a large file using streams in Node.js.
Answer:
Streams in Node.js are a way to handle large amounts of data efficiently, especially when dealing with files or network communication. A stream is a continuous flow of data that can be processed incrementally, in small chunks, without loading the entire data into memory.
Streams in Node.js can be of four types: Readable, Writable, Duplex, and Transform. Readable streams are used to read data, Writable streams for writing data, Duplex streams for both reading and writing, and Transform streams for modifying data while reading or writing.
Here’s an example of reading a large file using streams in Node.js:
const fs = require('fs');
const readableStream = fs.createReadStream('largefile.txt', 'utf8');
readableStream.on('data', chunk => {
console.log('Received a chunk of data:', chunk);
});
readableStream.on('end', () => {
console.log('Reading finished.');
});
readableStream.on('
error', err => {
console.error('Error while reading:', err);
});
Code language: PHP (php)
In this example, we create a Readable stream using fs.createReadStream()
. As the file is read, the 'data'
event is emitted with chunks of data that can be processed immediately, without waiting for the whole file to be read. The 'end'
event is emitted when the reading is finished, and the 'error'
event is emitted if an error occurs during the reading process.
By using streams, Node.js can efficiently process large files, as only a small chunk of data is held in memory at any given time, reducing memory consumption and enabling faster data processing.
Question:
Explain the purpose of the Express.js framework in Node.js and its significance in building web applications. Provide an example of creating a simple web server using Express.js.
Answer:
Express.js is a popular and minimalist web framework for Node.js that simplifies the process of building web applications and APIs. It provides a robust set of features for handling routes, middleware, templates, and much more, making it an excellent choice for building web applications with Node.js.
Here’s an example of creating a simple web server using Express.js:
const express = require('express');
const app = express();
const port = 3000;
// Route handling
app.get('/', (req, res) => {
res.send('Hello, World!');
});
// Start the server
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
Code language: JavaScript (javascript)
In this example, we create an Express application using express()
. We define a route for the root URL '/'
, and when a GET request is made to this route, it responds with the message 'Hello, World!'
. We then start the server on port 3000 using app.listen()
. Express handles the HTTP server setup and provides an easy-to-use interface for handling routes and responding to requests.
Express.js significantly reduces boilerplate code and provides a straightforward and flexible structure for building web applications, allowing developers to focus on implementing business logic and features.
Question:
Explain the concept of Authentication and Authorization in the context of web applications. Provide an example of how you can implement token-based authentication in a Node.js application.
Answer:
Authentication and Authorization are two essential aspects of web applications for ensuring security and controlling access to resources.
Authentication is the process of verifying the identity of a user, typically by providing credentials (such as username and password). Once the user is authenticated, they receive an authentication token, which is used to identify them in subsequent requests.
Authorization, on the other hand, is the process of determining what actions a user is allowed to perform on the application’s resources based on their identity and assigned roles or permissions.
Here’s an example of implementing token-based authentication in a Node.js application using JSON Web Tokens (JWT):
const express = require('express');
const jwt = require('jsonwebtoken');
const app = express();
const secretKey = 'your-secret-key';
// Middleware to check for authentication token
function authenticateToken(req, res, next) {
const token = req.header('Authorization')?.split(' ')[1];
if (!token) {
return res.sendStatus(401);
}
jwt.verify(token, secretKey, (err, user) => {
if (err) {
return res.sendStatus(403);
}
req.user = user;
next();
});
}
// Login route
app.post('/login', (req, res) => {
// Assuming you have a user authentication logic here
const user = { id: 1, username: 'exampleUser' };
const token = jwt.sign(user, secretKey);
res.json({ token });
});
// Protected route
app.get('/protected', authenticateToken, (req, res) => {
res.json({ message: 'Protected route accessed!', user: req.user });
});
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
Code language: PHP (php)
In this example, when a user successfully logs in ('/login'
route), we generate a JWT token using the jwt.sign()
method, which includes the user information (e.g., user ID and username). The token is then sent to the client as a response. On subsequent requests to the protected route ('/protected'
), the token is passed in the Authorization
header. The authenticateToken
middleware verifies the token using jwt.verify()
and allows access to the protected route only if the token is valid. If the token is missing or invalid, appropriate status codes (401 or 403) are sent in response, indicating unauthorized access.
Question:
Explain the concept of sessions and cookies in the context of web applications. Provide an example of how you can implement session-based authentication using cookies in a Node.js application.
Answer:
Sessions and cookies are mechanisms used to maintain user state and enable authentication in web applications.
A session is a logical unit of user state on the server side. When a user logs in, a unique session identifier is generated, and this identifier is stored on the server along with user-specific data. The session identifier is typically sent to the client as a cookie, allowing the server to recognize the user across multiple requests.
Here’s an example of implementing session-based authentication using cookies in a Node.js application:
const express = require('express');
const cookieParser = require('cookie-parser');
const app = express();
const secretKey = 'your-secret-key';
const users = [
{ id: 1, username: 'exampleUser', password: 'password123' }
];
app.use(cookieParser());
// Middleware to check for a valid session
function authenticateSession(req, res, next) {
const sessionId = req.cookies.sessionId;
if (!sessionId) {
return res.sendStatus(401);
}
// Assuming you have a session store here (e.g., in-memory or database)
const user = sessionStore[sessionId];
if (!user) {
return res.sendStatus(403);
}
req.user = user;
next();
}
// Login route
app.post('/login', (req, res) => {
const { username, password } = req.body;
const user = users.find(u => u.username === username && u.password === password);
if (!user) {
return res.sendStatus(401);
}
// Generate a random session ID and store user data
const sessionId = Math.random().toString(36).substr(2);
sessionStore[sessionId] = user;
// Set the session ID as a cookie
res.cookie('sessionId', sessionId, { httpOnly: true });
res.json({ message: 'Login successful!' });
});
// Protected route
app.get('/protected', authenticateSession, (req, res) => {
res.json({ message: 'Protected route accessed!', user: req.user });
});
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
Code language: PHP (php)
In this example, when a user successfully logs in ('/login'
route), a random session ID is generated and associated with the user data in the session store. The session ID is then sent to the client as a cookie with the res.cookie()
method. On subsequent requests, the client sends the session ID in the Cookie
header, allowing the server to identify the
user by looking up the session ID in the session store. If the session ID is not valid or missing, appropriate status codes (401 or 403) are sent in response, indicating unauthorized access.
Senior Node.js interview questions
Question:
Explain the concept of asynchronous programming in Node.js and how it helps in building scalable applications. Provide an example of asynchronous code using callbacks.
Answer:
Asynchronous programming in Node.js allows executing multiple tasks concurrently without blocking the execution of the main program. It is essential for building scalable applications as it allows the server to handle a large number of concurrent connections efficiently, without getting stuck waiting for slow I/O operations.
Example of asynchronous code using callbacks:
const fs = require('fs');
// Asynchronous file read operation
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error:', err);
} else {
console.log('File contents:', data);
}
});
console.log('Async operation is in progress...');
Code language: JavaScript (javascript)
In this example, the readFile()
function reads the contents of a file asynchronously. The callback function is executed once the file read operation is completed. While the file is being read, the program continues executing the console.log('Async operation is in progress...')
statement, showing the non-blocking nature of the asynchronous operation.
Question:
Explain the concept of the event loop in Node.js and how it enables non-blocking I/O operations. How can developers avoid blocking the event loop?
Answer:
The event loop is a critical part of Node.js that allows it to handle asynchronous I/O operations efficiently. The event loop is a loop that continuously checks for pending events, such as I/O operations, timers, and callbacks, and executes their corresponding event handlers.
Node.js relies on non-blocking I/O operations to avoid blocking the event loop. When a function initiates an I/O operation, instead of waiting for it to complete, Node.js continues executing other tasks while the I/O operation is handled in the background. Once the I/O operation is completed, its callback is added to the event queue, and the event loop executes it when all other tasks in the current event loop iteration are done.
Developers can avoid blocking the event loop by using asynchronous patterns, such as callbacks, Promises, or async/await, for I/O operations and CPU-intensive tasks. By doing so, they ensure that long-running operations don’t block the event loop, allowing the server to remain responsive to incoming requests.
Question:
In Node.js, what is the purpose of the module.exports
object, and how does it facilitate module-based development? Provide an example of exporting and importing modules in Node.js.
Answer:
In Node.js, the module.exports
object is used to define what parts of a module should be accessible and usable by other modules that require it. It facilitates module-based development by encapsulating code into individual modules, making the code modular, maintainable, and reusable.
Example of exporting and importing modules:
Module: mathOperations.js
const add = (a, b) => a + b;
const subtract = (a, b) => a - b;
module.exports = {
add,
subtract
};
Code language: JavaScript (javascript)
Module: main.js
const mathOps = require('./mathOperations');
console.log(mathOps.add(5, 3)); // Output: 8
console.log(mathOps.subtract(10, 5)); // Output: 5
Code language: JavaScript (javascript)
In this example, we have two modules. The mathOperations.js
module exports the add
and subtract
functions using module.exports
. The main.js
module imports those functions using require
and can use them as if they were defined locally. This illustrates how module.exports
enables us to share functionality between modules in Node.js.
Question:
Explain the purpose of middleware in Express.js. Provide an example of how to use middleware to handle HTTP requests.
Answer:
Middleware in Express.js is a function or a set of functions that are executed in a sequence during the lifecycle of an HTTP request. Middleware functions have access to the request (req
) and response (res
) objects and can perform tasks such as logging, authentication, data parsing, and error handling. Middleware provides a way to modularize and reuse common functionality across routes in an Express application.
Example of using middleware in Express.js:
const express = require('express');
const app = express();
// Middleware for logging the request method and URL
const loggerMiddleware = (req, res, next) => {
console.log(`${req.method} ${req.url}`);
next(); // Move to the next middleware or route handler
};
// Middleware for parsing JSON data
app.use(express.json());
// Apply the loggerMiddleware to all routes
app.use(loggerMiddleware);
// Route handler
app.get('/', (req, res) => {
res.send('Hello, world!');
});
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
Code language: JavaScript (javascript)
In this example, we have defined two middleware functions: loggerMiddleware
for logging requests and express.json()
middleware for parsing JSON data. The app.use()
function is used to apply middleware to all routes, and the next()
function is called within each middleware to pass control to the next middleware or route handler.
Question:
Explain the concept of streams in Node.js and how they help in handling large datasets efficiently. Provide an example of using readable and writable streams in Node.js.
Answer:
Streams in Node.js are an essential feature that allows reading or writing data in chunks, rather than loading the entire dataset into memory at once. Streams are beneficial when handling large datasets as they enable efficient memory usage and reduced processing time.
Example of using readable and writable streams:
Read a file using readable stream:
const fs = require('fs');
const readableStream = fs.createReadStream('largeFile.txt', 'utf8');
readableStream.on('data', (chunk) => {
console.log(`Received ${chunk.length} bytes of data.`);
});
readableStream.on('end', () => {
console.log('Reading file completed.');
});
Code language: JavaScript (javascript)
Write data to a file using writable stream:
const fs = require('fs');
const writableStream = fs.createWriteStream('outputFile.txt');
writableStream.write('This is the first line.n');
writableStream.write('This is the second line.n');
writableStream.end('Writing data completed.');
Code language: JavaScript (javascript)
In the first example, a readable stream is created using fs.createReadStream()
, and it reads a large file in chunks. The data
event is emitted for each chunk, allowing you to process the data in smaller portions.
In the second example, a writable stream is created using fs.createWriteStream()
, and it writes data to an output file in chunks. The write()
method allows you to write data in smaller chunks, reducing memory consumption and improving performance.
Question:
Explain the role of the Node.js util.promisify
function and how it simplifies working with asynchronous functions that use callbacks. Provide an example of using util.promisify
to convert a callback-based function into a Promise-based function.
Answer:
The util.promisify
function in Node.js is part of the util
module, and it is used to convert functions that use callbacks into functions that return Promises. This simplifies working with asynchronous functions, as it allows you to use async/await
or .then()
syntax to handle asynchronous operations.
Example of using util.promisify
:
const util = require('util');
const fs = require('fs');
// Callback-based function
const readFileCallback = (file, callback) => {
fs.readFile(file, 'utf8', callback);
};
// Promise-based function using util.promisify
const readFilePromise = util.promisify(readFileCallback);
// Usage with async/await
(async () => {
try {
const data = await readFilePromise('example.txt');
console.log('File contents:', data);
} catch (err) {
console.error('Error:', err);
}
})();
Code language: JavaScript (javascript)
In this example, we have a callback-based function readFileCallback
, which reads a file and returns the data through the callback. Using util.promisify
, we convert readFileCallback
into readFilePromise
, a Promise-based function that returns a Promise that resolves with the file data.
With readFilePromise
, we can use async/await
to handle the asynchronous operation more concisely and with better error handling.
Question:
Explain the concept of WebSocket in Node.js and how it enables bidirectional communication between clients and servers. Provide an example of implementing a WebSocket server in Node.js using the ws
library.
Answer:
WebSocket is a communication protocol that enables full-duplex, bidirectional communication between clients and servers over a single, long-lived connection. Unlike traditional HTTP, which is request-response-based, WebSocket allows real-time, low-latency communication, making it ideal for applications that require frequent data exchange, such as chat applications, real-time gaming, and live data updates.
Example of implementing a WebSocket server using the ws
library:
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
// Event handler for new connections
wss.on('connection', (ws) => {
console.log('New client connected.');
// Event handler for receiving messages from clients
ws.on('message', (message) => {
console.log('Received message:', message);
// Send a response back to the client
ws.send(`Server received: ${message}`);
});
// Event handler for client disconnection
ws.on('close', () => {
console.log('Client disconnected.');
});
});
Code language: JavaScript (javascript)
In this example, we use the ws
library to create a WebSocket server. When a client connects ('connection'
event), the server logs a message. When the server receives a message from a client ('message'
event), it logs the message and sends a response back to the client using ws.send()
. If a client disconnects ('close'
event), the server logs a disconnection message.
Question:
Explain the concept of error handling in Node.js, and describe how the try-catch
mechanism can be used to handle errors in asynchronous code. Provide an example of error handling in asynchronous code.
Answer:
Error handling in Node.js is crucial for gracefully handling unexpected situations and preventing the application from crashing. Errors in asynchronous code can be handled using the try-catch
mechanism combined with Promises or async/await
.
Example of error handling in asynchronous code with async/await
:
const fs = require('fs').promises;
async function readFileAsync(file) {
try {
const data = await fs.readFile(file, 'utf8');
console.log('File contents:', data);
} catch (err) {
console.error('Error:', err.message);
}
}
readFileAsync('nonExistentFile.txt');
Code language: JavaScript (javascript)
In this example, the readFileAsync
function reads a file using fs.readFile
with await
. If the file does not exist, an error will be thrown. The try-catch
block allows us to catch and handle the error gracefully, logging the error message without crashing the application.
Question:
Explain the concept of the cluster module in Node.js and how it helps in scaling applications across multiple CPU cores. Provide an example of using the cluster module to create a cluster of worker processes.
Answer:
The cluster module in Node.js allows scaling applications across multiple CPU cores by creating a cluster of worker processes. Each worker process runs in its own thread and handles incoming requests independently. This approach enables applications to utilize the full processing power of the server and handle more concurrent connections efficiently.
Example of using the cluster module to create a cluster of worker processes:
const cluster = require('cluster');
const http = require('http');
const numCPUs = require('os').cpus().length;
if (cluster.isMaster) {
console.log(`Master ${process.pid} is running`);
// Fork workers based on the number of CPU cores
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
cluster.on('exit', (worker, code, signal) => {
console.log(`Worker ${worker.process.pid} died`);
// Replace the dead worker
cluster.fork();
});
} else {
// Workers will handle HTTP server connections here
http.createServer((req, res) => {
res.writeHead(200);
res.end('Hello, World!');
}).listen(8000);
console.log(`Worker ${process.pid} started`);
}
Code language: JavaScript (javascript)
In this example, the master process (cluster.isMaster
) creates a cluster of worker processes using cluster.fork()
. Each worker process listens on the same port and handles incoming HTTP server connections. If a worker process dies ('exit'
event), the master process replaces it with a new worker using cluster.fork()
. This way, the application can gracefully recover from worker failures and maintain high availability.
Question:
Explain the purpose of the npm
package manager in Node.js and how it simplifies dependency management. Provide an example of using npm
to initialize a new project and install external packages.
Answer:
The npm
(Node Package Manager) is the default package manager for Node.js, used for installing, managing, and sharing reusable JavaScript packages and libraries. It simplifies dependency management by allowing developers to specify project dependencies in a package.json
file and then automatically installing all required dependencies with a single command.
Example of using npm
to initialize a new project and install external packages:
- Create a new directory for your project:
mkdir my-node-project
cd my-node-project
- Initialize a new Node.js project and create a
package.json
file:
npm init
Follow the prompts to provide details about your project. You can also use npm init -y
to accept all the default values.
- Install external packages using
npm
:
npm install express
This command installs the express
package and adds it to the dependencies
section of your package.json
file.
- Install additional packages and save them as dev dependencies:
npm install nodemon --save-dev
This command installs nodemon
and adds it to the devDependencies
section of your package.json
file. Dev dependencies are used during development and are not required in production.
After installing the packages, you can start using them in your Node.js project by requiring them in your code. For example, const express = require('express');
will now allow you to use the express
package in your code.
1,000 Companies use CoderPad to Screen and Interview Developers
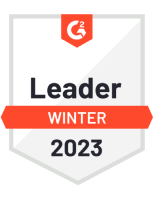
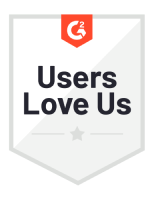
Interview best practices for Node roles
For successful Node.js interviews, it’s important to consider various factors such as the candidate’s experience level and the engineering role they are applying for. To ensure that your Node.js interview questions yield the best results, we recommend following these best practices when working with candidates:
- Devise technical queries that correspond with real-world business scenarios within your organization. This approach not only makes the interview more captivating for the applicant but also allows you to more effectively gauge their fit for your team.
- Encourage the candidate to pose questions throughout the interview and cultivate a cooperative atmosphere.
- Ensure that your candidates possess a comprehensive understanding of multi-threading and asynchronous request concepts, such as promises.
Additionally, adhering to standard interview etiquette when conducting Node.js interviews is essential. This includes adjusting the question complexity to the candidate’s level of development skills, providing timely feedback to candidates about their application status, and allowing candidates to ask questions about the assessment or working with you and your team.