Prompt Engineering for Technical Interview Questions
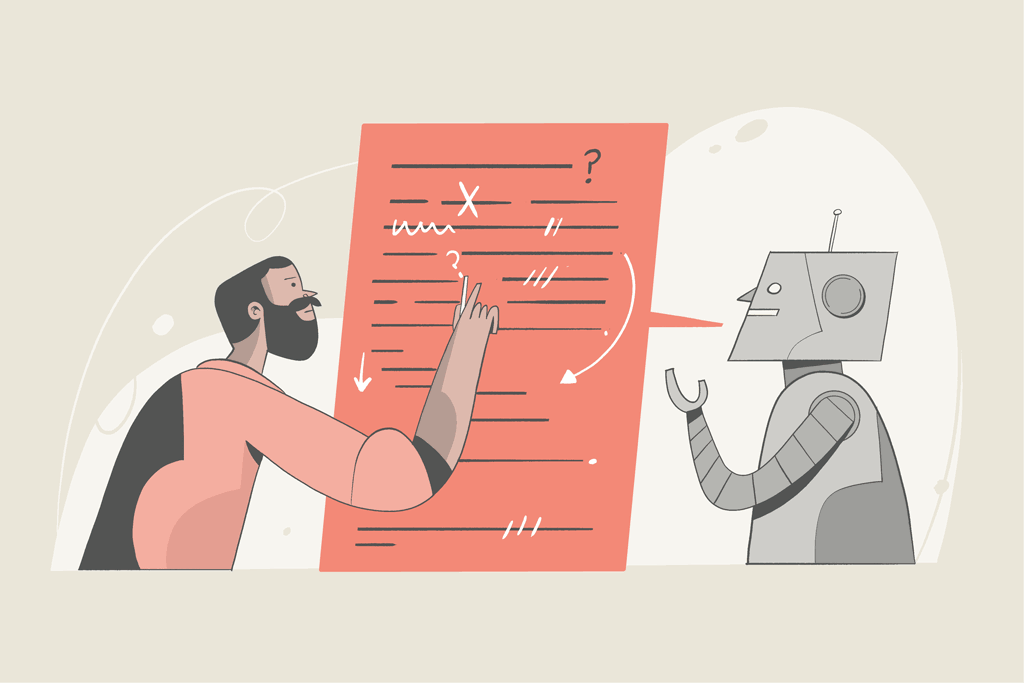
As the use cases for Large Language Model (LLM) Artificial Intelligence (AI) flood the internet as more and more people get access, you’ll start to hear more and more talk on prompt engineering.
One of the lesser-known buzzwords surrounding products like ChatGPT and Google Bard, prompt engineering is creating and refining your input (i.e., prompts) to get the responses you’re looking for.
As advanced as they are, LLM AIs still cannot detect nuance. And much like asking humans to give you answers, you sometimes need to provide feedback to make sure your question is understood so that you can get an appropriate response.
That’s the goal of prompt engineering – to make sure ChatGPT (or whatever product you’re using) understands precisely what you’re asking so you don’t end up with a response that is either incorrect or insufficient for what you want.
The blog post will be broken up into three sections:
- Understanding prompt engineering in the context of technical interviewing
- Implementing prompt engineering to develop technical interview questions
- An example of prompt engineering in creating an interview question
✅ The response below is from CoderPad Interview’s new ChatGPT-based AI Chat. If you’re an existing customer, you can learn how to turn it on here. If you’re not yet using CoderPad for your interviews, you can sign-up for a free trial here to access the AI Chat feature.
Table of Contents
Understanding prompt engineering
Instead of engaging in blog-writer wordplay, we’re going to go straight to the source and let ChatGPT give you a detailed explanation of what precisely prompt engineering entails:
The goal of prompt engineering is to structure an input query in such a way that the language model is likely to produce the desired response.
Prompt engineering can involve several steps or considerations:
Understanding the Model: It’s important to know what the model is capable of, as well as its limitations. For instance, a language model like GPT-4 doesn't "understand" text in the way a human does, it only predicts what comes next in a sequence based on patterns in the data it was trained on. It doesn't have beliefs or desires.
Clarifying the Objective: What is the goal of the prompt? Do you want a short, factual response? A long narrative? A joke? The type of response you're aiming for will greatly influence how you structure your prompt.
Formulating the Prompt: This is where you actually write the input query. This might involve specifying the format you want the response in, asking the question in several different ways, or providing some sort of context to guide the model. For instance, if you're asking for a joke, you might start with "Tell me a joke about..."
Testing and Refining: It’s unlikely that you’ll get the perfect response on the first try. Testing different prompts, tweaking the wording or structure, and learning from the model's responses is a key part of the process.
Systematizing: Once you’ve found a formula that works, you can create a template or system to produce similar prompts in the future. This can help you get consistent results across a range of queries.
Prompt engineering is a crucial skill in machine learning because the model's output is only as good as the prompts it's given. A poorly designed prompt can result in irrelevant, nonsensical, or even harmful outputs. On the other hand, a well-crafted prompt can guide the model towards the desired result, increasing the usefulness of the model and reducing the risk of harmful or unhelpful outputs.
Prompt engineering in technical interviews
There are several uses for prompt engineering in technical interview questions, including:
- Creating test data
- Creating test cases
- Generating scaffolding code
- Generating the questions themselves
You’ll see in the implementation section below that our prompts will differ depending on the interview factors involved, including the developer level you’re interviewing, the exact role (frontend, backend, full stack, data analyst, etc.), and the languages and technologies involved.
As we see the answers that ChatGPT produces in response to those factors, we’ll continue to refine our input queries until it has everything we need to get a complete question we can use – and reuse – in technical interviews.
Benefits of using prompt engineering for technical interview questions
The ultimate reason for using ChatGPT to generate interview questions is to save time.
Prompt engineering’s part in that is to help you adjust your input query until you have a standard format where you can create a template that you can use to generate future questions.
Once you have a solid query that gives you all the information you need, you can just replace the variable parts of the text to generate more good questions in other domains – different languages, roles, role levels, etc.
For example, once I create a good query for a senior React dev, I can simply replace “React” with “Node.js” if I’m looking to assess a full stack candidate, or I can replace “senior” with “junior” to create a question for a recent college graduate.
Without prompt engineering, you’d generate less-than-ideal questions every time, over and over again, and you’d probably spend more time using LLMs than just writing your own question from scratch.
Implementing Prompt Engineering in Technical Interviews
Creating the prompt
The first – and probably easiest – thing to do in creating a prompt is to define the job role and level you want to review. As an interviewer, you know exactly what role you’re hiring for, so this should be a piece of cake:
Create a technical interview question for a senior React developer.
Next, you’ll need to consider the most important skills you want to assess. This could be specific technologies like utilizing the Pandas library or Express.js for an application– or it could be more general, like displaying best UI design practices or knowledge of API integrations.
Create a technical interview question for a senior React developer that assesses their ability to utilize best UI design practices and knowledge of CSS.
It’d also be beneficial to add a business case context to create a question that reflects the real-world work the candidate would be undertaking in the role. For example, would they be working with an eCommerce application? Processing data via a microservice? Reading/writing from a database to create a dashboard? Use that in your prompt:
Create a technical interview question for a senior React developer that assesses their ability to utilize best UI design practices and knowledge of CSS. The question should be in the form of an application that takes data and turns it into a finance dashboard displaying stock prices for finance professionals.
Testing the prompt
Now it’s time to see how well our prompt creates the response we need and what we may need to fix. Here’s the output from ChatGPT:
Sure! Here's a technical interview question that assesses a senior React developer's ability to utilize best UI design practices and their knowledge of CSS:
**Question: Finance Dashboard Application**
You are tasked with developing a finance dashboard application that displays stock prices for finance professionals. The application will receive data from an API in JSON format, which includes information about different stocks. Your goal is to create a responsive and visually appealing dashboard using React and CSS, adhering to best UI design practices.
**Requirements:**
1. Fetch stock data from the provided API endpoint: `https://api.example.com/stocks`.
2. The dashboard should display a list of stocks, each represented by a card component.
3. Each card should include the following information:
- Stock symbol (e.g., AAPL for Apple Inc.)
- Stock name (e.g., Apple Inc.)
- Current stock price
- Percentage change in stock price (compared to the previous day's closing price)
- Volume of shares traded
4. Implement a color-coded indicator for the percentage change in stock price:
- Green for positive percentage change
- Red for negative percentage change
- No indicator for zero percentage change
5. The dashboard should be responsive and adjust its layout based on the screen size:
- On larger screens, display multiple cards in a row (e.g., 3 cards per row).
- On smaller screens, display a single column of cards.
6. Apply CSS styling to enhance the visual appeal of the dashboard. Consider:
- Font choices, sizes, and weights
- Color scheme
- Card layout and spacing
- Responsive design principles
- Consistent UI design across different browsers
**Deliverables:**
Please create a React application that satisfies the requirements outlined above. You can use any additional libraries or frameworks if needed. Write clean and maintainable code, and feel free to use modern CSS techniques (e.g., CSS modules, CSS-in-JS, etc.) if you prefer.
In addition to the functional implementation, please provide a brief explanation of your design choices, including any CSS frameworks or methodologies you used, and how you ensured the application's responsiveness and browser compatibility.
Good luck!
This is not bad!
Refining the prompt
There are a couple of things I want to fix, though:
- I have no API for them to connect to. I could find one on the internet (or ask ChatGPT to find me one), but for the purpose of time and having them focus on the UI design rather than on API integration, I’m just going to adjust the question so the data comes from a flat file rather than an API.
- Having the candidate write all the boilerplate code for that question would be tedious and not worth my time – I want to see how they work with UI design, not rewrite components they learned about eight years ago. I want ChatGPT to generate the starter code for this question.
Here’s how I’d reframe the prompt to include those points:
Create a technical interview question for a senior React developer that assesses their ability to utilize best UI design practices and knowledge of CSS. The question should be in the form of an application that takes data from a JSON file and turns it into a finance dashboard displaying stock prices for finance professionals. Please also generate the sample data JSON file and provide starter code for the candidate.
Let’s see if we get any improvement:
Here's a technical interview question that assesses a senior React developer's ability to utilize best UI design practices, their knowledge of CSS, and their ability to work with data in a finance dashboard application:
**Question: Finance Dashboard Application**
You are tasked with developing a finance dashboard application that displays stock prices for finance professionals. The application will receive data from a JSON file, which includes information about different stocks. Your goal is to create a responsive and visually appealing dashboard using React and CSS, adhering to best UI design practices.
**Requirements:**
1. Create a React application that reads stock data from a JSON file and displays it on the finance dashboard.
2. The stock data is provided in a file named `stocks.json`. The file follows the structure below:
{
"stocks": [
{
"symbol": "AAPL",
"name": "Apple Inc.",
"price": 150.25,
"change": 1.34,
"volume": 1000000
},
{
"symbol": "GOOGL",
"name": "Alphabet Inc.",
"price": 2500.10,
"change": -0.75,
"volume": 750000
},
{
"symbol": "MSFT",
"name": "Microsoft Corporation",
"price": 300.50,
"change": 0,
"volume": 1200000
}
]
}
Code language: JSON / JSON with Comments (json)
3. Read the stock data from the `stocks.json` file and display it on the finance dashboard.
4. The dashboard should display a list of stocks, each represented by a card component.
5. Each card should include the following information:
- Stock symbol
- Stock name
- Current stock price
- Percentage change in stock price (compared to the previous day's closing price)
- Volume of shares traded
6. Implement a color-coded indicator for the percentage change in stock price:
- Green for positive percentage change
- Red for negative percentage change
- No indicator for zero percentage change
7. The dashboard should be responsive and adjust its layout based on the screen size:
- On larger screens, display multiple cards in a row (e.g., 3 cards per row).
- On smaller screens, display a single column of cards.
8. Apply CSS styling to enhance the visual appeal of the dashboard. Consider:
- Font choices, sizes, and weights
- Color scheme
- Card layout and spacing
- Responsive design principles
- Consistent UI design across different browsers
**Starter Code:**
Use the following starter code as a basis for your React application:
import React, { useEffect, useState } from 'react';
import stocksData from './stocks.json';
function FinanceDashboard() {
const
[stocks, setStocks] = useState([]);
useEffect(() => {
// Read stock data from the JSON file
setStocks(stocksData.stocks);
}, []);
return (
<div className="finance-dashboard">
{/* Display the stock cards here */}
</div>
);
}
export default FinanceDashboard;
Code language: JavaScript (javascript)
Feel free to modify the starter code as needed and add any additional components or styles to complete the finance dashboard application.
Much better. You could continue to modify it to add test cases or generate starter code for multiple languages, but for simplicity’s sake, we’ll stick with what we have.
Abstract the prompt to create a template
Barring any other changes you may want to make to create your ideal interview question, we now have a specific prompt that we can generalize to generate a template for creating future interview questions for different roles, technologies, job levels, etc.
Using our example above, our final prompt was:
Create a technical interview question for a senior React developer that assesses their ability to utilize best UI design practices and knowledge of CSS. The question should be in the form of an application that takes data from a JSON file and turns it into a finance dashboard displaying stock prices for finance professionals. Please also generate the sample data JSON file and provide starter code for the candidate.
A generalized template for that would look like this:
Create a technical interview question for a [role level] [job title] that assesses [skills you want to assess]. The question should be in the form of an application that [functionality of the application the candidate would be working on with your team]. Please also generate the sample data [data structure type] file and provide starter code for the candidate.
So if we wanted to create a question for a junior full-stack developer who would make the admin dashboard for that finance application, we would just fill in the template like so:
Create a technical interview question for a junior full-stack developer that assesses their ability to populate a user interface in Angular with customer information from a MySQL database. The question should be in the form of an application that displays an admin dashboard where users can update their personal information. Please also generate the sample customer data SQL file and provide starter code for the candidate.
Here’s ChatGPT’s response to that:
Question
:
You have been assigned a task to create an admin dashboard in Angular that allows users to update their personal information, which is stored in a MySQL database. Your task is to populate the user interface with customer information retrieved from the database and implement the necessary functionality to update the data.
You need to create a single-page application that consists of the following components:
1. Admin Dashboard Component: This component serves as the main container for the admin dashboard. It should display a table listing all the customers and provide options to edit their information.
2. Customer Table Component: This component should display the customer data in a table format. Each row of the table should represent a customer, and each column should display a specific attribute of the customer (e.g., name, email, phone number, address). Include an "Edit" button in each row to allow users to update the information.
3. Edit Customer Component: This component should be displayed when a user clicks the "Edit" button for a specific customer. It should show a form where the user can modify the customer's information.
Your task is to implement the necessary Angular components, services, and routing configuration to fulfill the requirements mentioned above. Additionally, you need to retrieve the customer information from a MySQL database and populate the user interface with the retrieved data.
To assist you, here's a sample customer data SQL file (customers.sql) that you can import into your MySQL database:
-- customers.sql
CREATE TABLE customers (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(255) NOT NULL,
email VARCHAR(255) NOT NULL,
phone VARCHAR(20) NOT NULL,
address VARCHAR(255) NOT NULL
);
INSERT INTO customers (name, email, phone, address)
VALUES
('John Doe', '[email protected]', '1234567890', '123 Main St'),
('Jane Smith', '[email protected]', '0987654321', '456 Elm St'),
('Mike Johnson', '[email protected]', '5555555555', '789 Oak St');
Code language: SQL (Structured Query Language) (sql)
Please provide the starter code for the candidate to work with.
Starter Code:
Here's the starter code for the Angular application:
1. AdminDashboardComponent (admin-dashboard.component.html):
<!-- admin-dashboard.component.html -->
<h1>Admin Dashboard</h1>
<app-customer-table></app-customer-table>
Code language: HTML, XML (xml)
2. CustomerTableComponent (customer-table.component.html):
<!-- customer-table.component.html -->
<table>
<thead>
<tr>
<th>Name</th>
<th>Email</th>
<th>Phone</th>
<th>Address</th>
<th>Action</th>
</tr>
</thead>
<tbody>
<!-- Display customer data here -->
</tbody>
</table>
Code language: HTML, XML (xml)
3. EditCustomerComponent (edit-customer.component.html):
<!-- edit-customer.component.html -->
<form>
<!-- Display editable fields for customer information here -->
<button type="submit">Save</button>
</form>
Code language: HTML, XML (xml)
Please use the provided code as a starting point and build upon it to complete the task. You may use any additional dependencies or libraries as needed. Good luck!
That’s got everything we need!
Common mistakes in prompt engineering
The use of AI in interviews is new enough that it’s not without risks.
First and foremost – always review the response! There are multiple stories of ChatGPT and Google Bard making up information or even just getting it wrong.
Ensure you’re reviewing not only the code snippets but also the assumptions ChatGPT makes when creating the response based on your input. As you saw above, for example, its first idea for where to get the data for the dashboard was via an API. If you have one ready, great; otherwise, you might want to look for quicker options.
It’d also be a good idea to answer the question yourself. You can ask ChatGPT to generate a solution, although it currently struggles to answer software development questions involving multiple files. But at the very least, make sure YOU can answer it before you give it to a candidate.
You also want to avoid overly complex or vague prompts. The former will confuse the LLM and cause it to return a wrong or no answer. The latter will lead you to spend lots of time querying ChatGPT to refine your request to a usable point.
Final thoughts
By now, you have a thorough understanding of why prompt engineering is essential for utilizing ChatGPT and how you can use it to enhance your technical interview process by generating technical interview questions.
Here are a couple more suggestions for getting the most out of using LLMs in your interviewing.
Keep on experimenting
The technical interview prompt we created represents just a small set of elements that you may want in your technical interview.
For example, I asked ChatGPT to also generate a file with test cases for our senior React developer question by adding this line to the prompt:
In addition, create a file that also has test cases.
When it regenerated the response, it also added this:
**Test Cases**
Here is an example of test case file for your dashboard (`App.test.js`):
import { render, screen } from '@testing-library/react';
import App from './App';
test('renders stock data', async () => {
render(<App />);
const linkElement = await screen.findByText(/AAPL/i);
expect(linkElement).toBeInTheDocument();
});
test('renders loading state', () => {
render(<App />);
const loadingElement = screen.getByText(/loading/i);
expect(loadingElement).toBeInTheDocument();
});
test('handles error state', async () => {
global.fetch = jest.fn(() => Promise.reject("API is down"));
render(<App />);
const errorElement = await screen.findByText(/error/i);
expect(errorElement).toBeInTheDocument();
});
Code language: JavaScript (javascript)
Remember to add your own test cases to check for the presence and functionality of all the important components and states of the app.
You can also have it generate starter code in other languages, CSS files with the styling you want the candidate to use, and more – the limit is your imagination.
Don’t forget to practice
Whether you use ChatGPT or Google Bard, getting good at prompt engineering with LLMs is the kind of thing that takes practice.
Both Bard and ChatGPT have free offerings with limitations on the number of queries you can submit. As of this writing, ChatGPT also offers monthly and annual subscriptions.
CoderPad also allows you to use the ChatGPT-powered AI Chat in your interview pads. That way, you can practice engineering your prompt through the AI Chat and then set up your files and code when you get the response you want from ChatGPT:
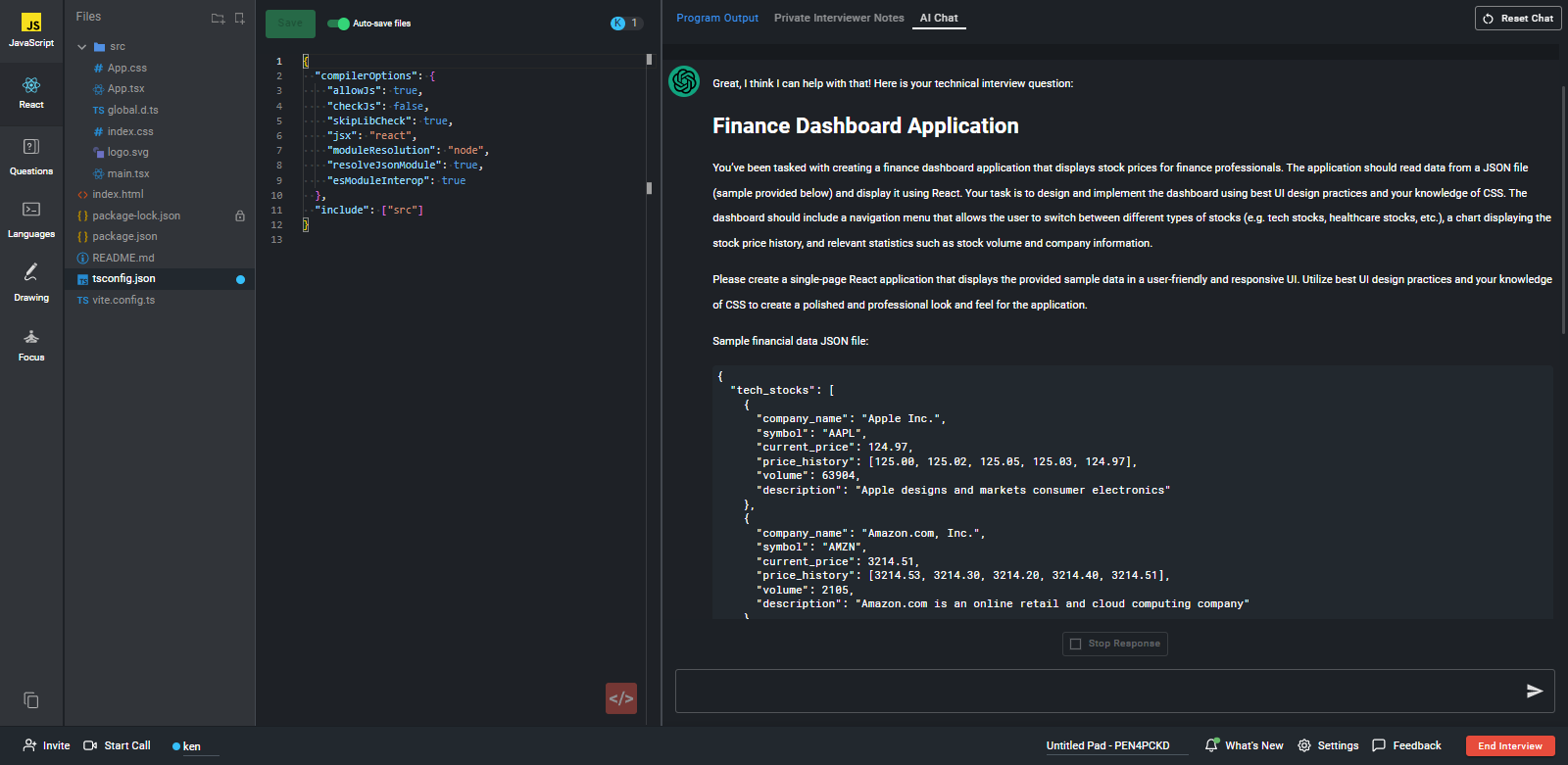
Read more on how to use ChatGPT in interviews
As access to LLMs continues to increase, you’ll start reading about more and more use cases for it that will help make your life a whole lot easier.
Given that we’re a leading interview and assessment platform, we’ll continue showing you how to use them to improve your hiring process.
You can check out the resources in the Related Posts section below to learn more about improving your interview processes with ChatGPT.