JavaScript Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified JavaScript developers for your organization.
JavaScript
JavaScript is widely regarded as one of the most sought-after programming languages globally, owing to its broad adoption in both front-end and back-end frameworks and its ease of learning.
According to the CoderPad 2024 Developer survey, JavaScript is the second-most in-demand language among technical recruiters and hiring managers.
To evaluate the JavaScript skills of developers during coding interviews, we’ve provided realistic coding exercises and interview questions below. Additionally, we’ve outlined a set of best practices to ensure that your interview questions accurately assess the candidates’ JavaScript skills.
Table of Contents
JavaScript example question
Create a JavaScript function to beat me at poker
I like playing poker, but I need to improve at shuffling cards.
Here is my shuffling method :
- I take the card from the top to start a second pile.
- I take the next card from the top of the pile and put it under the second pile.
- I take the next card from the top of the pile and put it on top of the second pile.
- I repeat the last two steps until no card is in the first pile.
Given the initial pile of cards, can you guess the order after my shuffling?
Cards will be defined as strings: “Ace of Spades”, “5 of Hearts”, …
JavaScript skills to assess
Jobs using JavaScript
Junior interview questions
Sure, here are 10 questions for a junior JavaScript developer:
Question: Fix the code:
let x = 10;
if (x = 5) {
console.log(x);
}
Code language: JavaScript (javascript)
What will be printed to the console if you run this code?
Answer: The code will print 5
to the console.
Question: Theory: What is the difference between var
and let
in JavaScript?
Answer: var
is function-scoped, while let
is block-scoped. Variables declared with var
are hoisted to the top of their function scope, while variables declared with let
are not hoisted.
Question: Fix the code:
let arr = [1, 2, 3];
arr.push(4);
console.log(arr);
Code language: JavaScript (javascript)
What will be printed to the console if you run this code?
Answer: The code will print [1, 2, 3, 4]
to the console.
Question: Theory: What is a function declaration in JavaScript?
Answer: A function declaration is a way to define a function in JavaScript using the function
keyword followed by a function name, a set of parentheses, and a set of curly braces.
Question: Fix the code:
let arr = [1, 2, 3];
for (let i = 0; i < arr.length; i++) {
console.log(arr[i]);
}
Code language: JavaScript (javascript)
What will be printed to the console if you run this code?
Answer: The code will print 1
, 2
, and 3
to the console.
Question: Theory: What is an array in JavaScript?
Answer: An array is a data structure that stores a collection of elements, which can be of any data type. Arrays in JavaScript are dynamic and can grow or shrink in size as needed.
Question: Fix the code:
function greet(name) {
console.log("Hello, " + name + "!");
}
greet("John");
Code language: JavaScript (javascript)
What will be printed to the console if you run this code?
Answer: The code will print Hello, John!
to the console.
Question: Theory: What is the this
keyword in JavaScript?
Answer: The this
keyword refers to the object that the function is a method of, or to the global object if the function is not a method of any object.
Question: Fix the code:
let x = 5;
let y = 10;
if (x < y) {
console.log("x is less than y");
} else {
console.log("x is greater than or equal to y");
}
Code language: JavaScript (javascript)
What will be printed to the console if you run this code?
Answer: The code will print x is less than y
to the console.
Question: Theory: What is an object in JavaScript?
Answer: An object is a collection of key-value pairs, where the keys are strings and the values can be of any data type. Objects in JavaScript are dynamic and can have properties added or removed at runtime.
Intermediate JavaScript interview questions
Sure, here are 10 questions for an intermediate JavaScript developer:
Question: Fix the code:
function double(arr) {
arr.forEach(function(val) {
val * 2;
});
return arr;
}
console.log(double([1, 2, 3]));
Code language: JavaScript (javascript)
What will be printed to the console if you run this code?
Answer: The code will print [1, 2, 3]
to the console.
Question: What is a higher-order function in JavaScript?
Answer: A higher-order function is a function that takes one or more functions as arguments and/or returns a function as its result. Examples of higher-order functions include map
, filter
, and reduce
.
Question: Fix the code:
let arr = [1, 2, 3];
for (var i = 0; i < arr.length; i++) {
setTimeout(function() {
console.log(arr[i]);
}, 1000);
}
Code language: JavaScript (javascript)
What will be printed to the console if you run this code?
Answer: The code will print undefined
three times to the console, with a delay of one second between each print.
Question: What is a closure in JavaScript? Provide an example.
Answer: A closure is a function that has access to variables in its outer (enclosing) function, even after the outer function has returned. Here’s an example:
function outerFunction() {
let outerVariable = "Hello";
return function() {
console.log(outerVariable);
}
}
Code language: JavaScript (javascript)
Question: Fix the code:
let arr = [1, 2, 3];
let result = arr.map(function(val) {
return val * 2;
});
console.log(result);
Code language: JavaScript (javascript)
What will be printed to the console if you run this code?
Answer: The code will print [2, 4, 6]
to the console.
Question: Theory: What is the difference between a synchronous and asynchronous function in JavaScript?
Answer: A synchronous function blocks further execution until it has completed its task, while an asynchronous function does not. Asynchronous functions typically use callbacks, promises, or async/await to signal completion.
Question: Fix the code:
function add(a, b) {
return a + b;
}
let result = add(2, 3);
console.log(result);
Code language: JavaScript (javascript)
What will be printed to the console if you run this code?
Answer: The code will print 5
to the console.
Question: What is the difference between let
and const
in JavaScript?
Answer: let
is used to declare variables that can be reassigned, while const
is used to declare variables that cannot be reassigned.
Question: Fix the code:
let arr = [1, 2, 3];
let sum = 0;
for (let i = 0; i < arr.length; i++) {
sum += arr[i];
}
console.log(sum);
Code language: JavaScript (javascript)
What will be printed to the console if you run this code?
Answer: The code will print 6
to the console.
Question: What is the difference between null
and undefined
in JavaScript?
A: null
represents an intentional absence of any object value, while undefined
represents a lack of a defined value. null
is typically used to represent an absence of a value that should be present, while undefined
is typically used to represent an uninitialized variable or an unknown value.
Senior JavaScript interview questions
Sure, here are 10 questions for a senior JavaScript developer:
Question: Fix the code:
function multiply(a, b) {
let result = a * b;
}
console.log(multiply(2, 3));
Code language: JavaScript (javascript)
What will be printed to the console if you run this code?
Answer: undefined
will be printed to the console.
Question: What is the difference between the ==
and ===
operators in JavaScript?
Answer: The ==
operator compares two values for equality, but performs type coercion if the values have different types. The ===
operator, on the other hand, compares two values for equality without performing type coercion.
Question: Fix the code:
let myArray = [1, 2, 3, 4, 5];
let result = myArray.reduce(function(accumulator, currentValue) {
return accumulator + currentValue;
});
console.log(result);
Code language: JavaScript (javascript)
What will be printed to the console if you run this code?
Answer: The code will print 15
to the console.
Question: Theory: What is lexical scoping in JavaScript?
Answer: Lexical scoping is a way of determining the value of a variable based on where it is defined in the code. In JavaScript, variables are resolved using lexical scoping, which means that a variable declared in an outer function can be accessed by an inner function, but not vice versa.
Question: Fix the code:
let myArray = [1, 2, 3, 4, 5];
let result = myArray.filter(function(element) {
return element % 2 === 0;
});
console.log(result);
Code language: JavaScript (javascript)
What will be printed to the console if you run this code?
Answer: The code will print [2, 4]
to the console.
Question: Theory: What is hoisting in JavaScript?
Answer: Hoisting is a JavaScript behavior where variable and function declarations are moved to the top of their respective scopes. This means that you can use a variable or function before it has been declared, but the value of the variable will be undefined
until it is assigned a value, and the function will not be callable until it has been declared.
Question: Fix the code:
let myArray = [1, 2, 3, 4, 5];
let result = myArray.reduce(function(accumulator, currentValue) {
return accumulator * currentValue;
}, 1);
console.log(result);
Code language: JavaScript (javascript)
What will be printed to the console if you run this code?
Answer: The code will print 120
to the console.
Question: What is the this
keyword in JavaScript?
Answer: The this
keyword in JavaScript refers to the object that the function is a method of. The value of this
depends on how the function is called.
Question: Fix the code:
let myObject = {
firstName: "John",
lastName: "Doe",
fullName: function() {
return this.firstName + " " + this.lastName;
}
};
console.log(myObject.fullName());
Code language: JavaScript (javascript)
What will be printed to the console if you run this code?
Answer: The code will print "John Doe"
to the console.
Question: What is a closure in JavaScript? Provide an example.
Answer: A closure is a function that has access to variables in its outer (enclosing) function, even after the outer function has returned. Here’s an example:
function outerFunction() {
let outerVariable = "Hello";
return function() {
console.log(outerVariable);
}
}
Code language: JavaScript (javascript)
More JavaScript interview resources
For more guides on improving your knowledge of JavaScript and acing interviews, we have outlined helpful blog posts below:
- 4 Ways to Use JavaScript .slice() Method and How to Do Each
- 21 Junior Front-End Developer Interview Questions
- A Thorough Guide To JavaScript Array Methods with Examples
- Guide to Sorting in JavaScript
- JavaScript .some() Method: When and How to Use It
- Javascript Interview Questions For Candidates
- CodinGame JavaScript Interview Questions
- How to Find & Hire Javascript Developers in 2021
1,000 Companies use CoderPad to Screen and Interview Developers
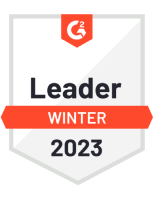
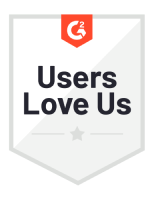
Interview best practices for JavaScript roles
To conduct effective JavaScript interviews, it’s important to consider various factors such as the candidate’s experience level and the engineering role. To ensure that your JavaScript interview questions yield the best results, we recommend following these best practices when working with candidates:
- Craft technical questions that are aligned with actual business cases within your organization. This will not only be more engaging for the candidate but also enable you to better assess the candidate’s suitability for your team.
- Encourage the candidate to ask questions during the interview and foster a collaborative environment.
- If you’re using JavaScript as part of front-end development, also ensure the candidate has a decent understanding of HTML and CSS.
- JavaScript comes with a constantly evolving ecosystem of frameworks. It may be useful to see which ones the candidate knows about or has interest in.
In addition, it’s important to adhere to standard interview etiquette when conducting JavaScript interviews. This includes adjusting the question complexity to the candidate’s level of development skills, providing timely feedback to candidates about their application status, and allowing candidates to ask questions about the assessment or working with you and your team.