JavaScript .some() Method: When and How to Use It
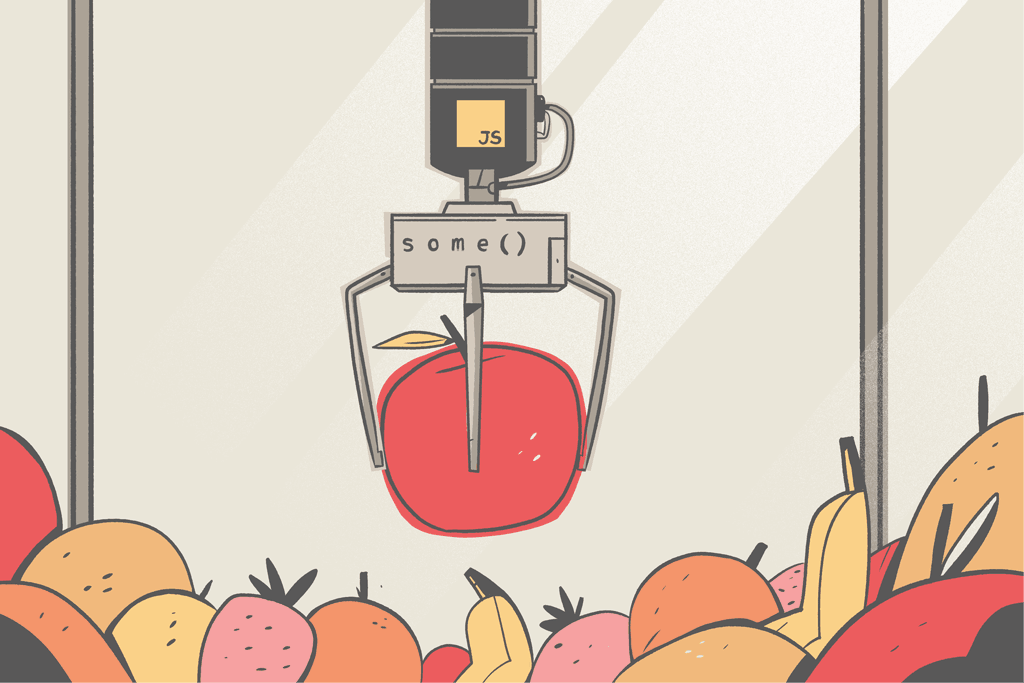
Arrays in JavaScript offer many built-in functionalities that many developers are unaware of, among these is the .some()
method.
The .some()
method comes in handy when the developer needs to check for a condition on an array and wants to know if at least one element fulfills that condition. This condition is passed in as a function that runs for each element of the array and exits execution the moment a true value is returned from the function. You also have access to that particular element as one of the function arguments.
Let’s understand the syntax of the .some()
method.
some((element, index, array) => { /* condition function returns boolean */ } )
Code language: JavaScript (javascript)
Here we have the element in context (the element of the array). The condition function will run on each member of the array unless the array is exhausted or the condition function returns true
. Let’s take a simple example to understand this better.
Understanding some() with an example
Say we run an online direct-from-the-farm fruit delivery service. If a user has any item that costs more than $30.00 added to their cart, we’ll offer them free delivery. We can assume that the cart items in memory exist as per the following basic data structure:
const itemsInCart = [
{ id: 123, name: “Apple”, cost: 10, quantity: 6 },
{ id: 456, name: “Coconut”, cost: 40, quantity: 1 },
{ id: 789, name: “Orange”, cost: 15, quantity: 2 }
]
Code language: JavaScript (javascript)
The first thing we’ll do is set a flag variable isOver30
as false
so that we can set it to true
once our desired condition is fulfilled. We’ll then run a .forEach()
loop on the cart items’ array to keep looking for any item that costs more than $30. If we find an item that costs more than $30, the flag will be set totrue
, and the loop will be exited.
let isOver30= false;
itemsInCart.forEach((item) => {
if (item.cost > 30) {
isOver30= true;
return;
}
});
// use the isOver30 flag to make further decisions
Code language: JavaScript (javascript)
Wait a minute. The .forEach()
method does not let us exit the loop once done. Once the function passes the “coconut” object, our condition would be renderedtrue
. Thus, we don’t need to continue anymore. But .forEach
runs on all the elements of an array. This means it’s overkill in our case.
Even return statements inside .forEach()
act as continue statements—not like break statements, which we require. Well, it’s quite easy to exit out of a good old for
loop. So, let’s give it a shot:
let isOver30= false;
for (let index = 0; index < itemsInCart.length; index++) {
const item = itemsInCart[index];
if (item.cost > 30) {
isOver30 = true;
break;
}
}
// use the isOver30 flag to make further decisions
Code language: JavaScript (javascript)
While this would suffice, it’s not the most readable code.
Making the code cleaner
Now let us use the .some()
method to take care of this logic in a cleaner manner.
const conditionFunc = item => item.cost > 30;
const isEligibleForFreeDelivery = itemsInCart.some(conditionFunc);
Code language: JavaScript (javascript)
Two lines –that’s all it takes. We’ve cut our code length by almost 75% with the .some()
method. We could technically even bring this down to one line, as our conditionFunc
is a straightforward function.
const isEligibleForFreeDelivery = itemsInCart.some((item) => item.cost > 30);
Code language: JavaScript (javascript)
The .some()
method stops execution once our condition function returnstrue
. That means that .some()
does not even operate on all the elements of an array, unlike methods like .forEach()
. This makes .some()
an ideal method when you don’t want to iterate over every element of an array. Apart from the element itself, we also have access to the index and the whole array, as well as inside the conditionFunc
. Developers can use this to solve their problems as they see fit.
Understanding the difference between some() and every()
The .some()
method is built on the same foundation as that of the methods .every()
and .find()
. The .every()
method returnstrue
if every array element passes the provided condition, stops execution, and returns false
the moment even one element does not fulfill the condition.
On the other hand, the .find()
method returns the first element that satisfies the provided condition. Because of this, it’s similar to the functionality of the .some()
method.
Of course, this could all technically be achieved with a for
loop or in a custom manner. However, you’re also required to appropriately document andexplain your functions so that other developers can use them. You have to name them right, add tons of comments, and hope future devs understand what you’re talking about.
You don’t have to worry about that with standardized JavaScript functions like .some()
and .find()
. With these methods, it’s much easier to deduce the purpose of that particular block of code without understanding its actual logic. This is the reason it’s best practice to use a built-in function if there’s one for what a developer is trying to achieve.
Just check out this code snippet to see for yourself:
const users = [
{ name: "John", age: 20 },
{ name: "Sarah", age: 19 },
{ name: "Bob", age: 30 },
{ name: "Alice", age: 25 },
];
// Using some() functions to check if any of the users are older than 20
const olderThan20 = users.some((user) => user.age > 20);
console.log(olderThan20); // true
// Using every() function to check if all users are older than 20
const allOlderThan20 = users.every((user) => user.age > 20);
console.log(allOlderThan20); //false
// Using find() function to find the first user older than 20
const firstOlderThan20 = users.find((user) => user.age > 20);
// Using find() function to find the first user older than 20 and return the name
const firstOlderThan20Name = users.find((user) => user.age > 20).name;
Code language: JavaScript (javascript)
Best practices for using some() in JavaScript
The advantage of using .some()
over other methods for similar tasks is that the final code is cleaner than the conventional approaches we discussed. This will allow future devs to understand this code’s purpose and extend it accordingly.
There are a few instances when we should stick to the for
loops:
- If the conditions are more complex than in our example above
- If we need to operate on multiple arrays
- If there are multiple exit conditions, which will then make your functions highly tailored to your problem and not a one-size-fits-all approach
It all depends on your use case. If you need to run condition checks on elements of an array and need at least one of them to be true, then .some()
is the way to go.
Wrapping up
JavaScript keeps coming up with practical built-in methods that offer ease and convenience for current and future developers of any given codebase, thus allowing everyone to build solutions rapidly. The .some()
array is another method that solves a particular problem. It won’t be the solution to all our problems, but whenever it is, it can render a final code output that is extremely clean and high quality.
This post was written by Keshav Malik. Keshav is a full-time developer who loves to build and break stuff. He is constantly looking for new and exciting technologies and enjoys working with diverse technologies in his spare time. He loves music and plays badminton whenever the opportunity presents itself.