4 Ways to Use JavaScript .slice() Method and How to Do Each
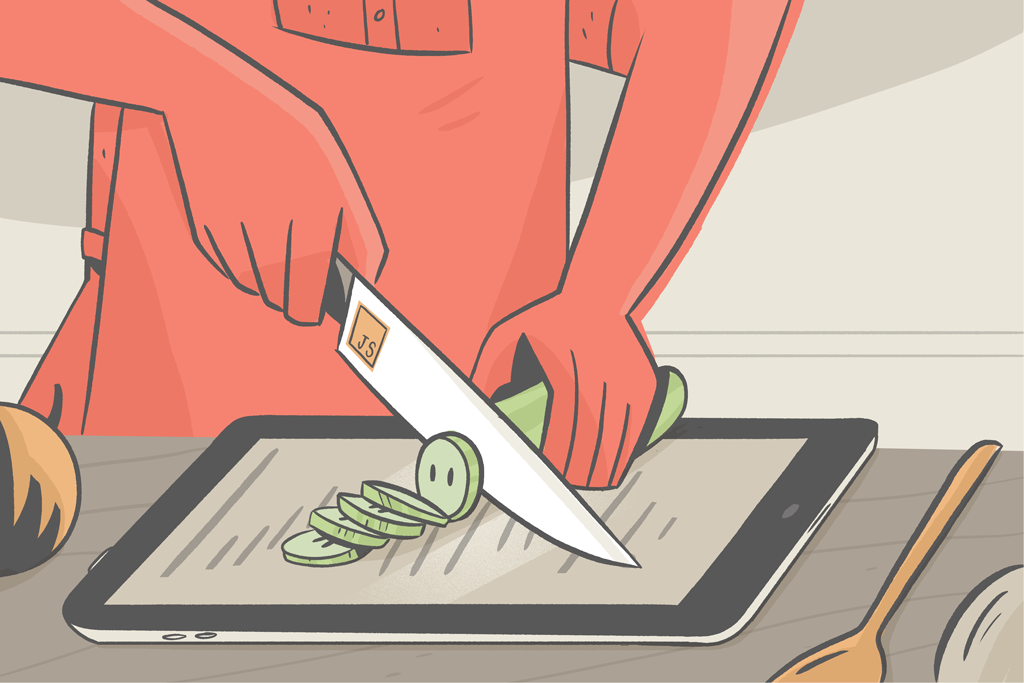
The JavaScript .slice()
method is a common method that’s often used in code but not often understood by novice developers. To help you understand this helpful method, we will discuss exactly what it is and four ways you can use it.
ℹ️ Before getting started, we assume that you understand how functions work in JavaScript. If not, check out the JavaScript functions guide to learn more.
What is the .slice() method?
Put simply, .slice()
returns a portion of an array. It’s implementation looks something like this:
target.slice(start_index, end_index);
Code language: JavaScript (javascript)
It takes two arguments:
- The index of the element to start at.
- The index of the element to end at.
It then returns an array containing elements from the start index to the end index.
The start_index
in the method is inclusive (where start_index
is included), and the end_index
is exclusive (where end_index
is not included). This method creates a shallow copy and does not modify the original array or string.
The .slice()
method can be helpful when working on a big project:
- The method can be used to quickly and easily extract a portion of an array or string. This function can be very helpful when you need to process only a subset of data.
- Developers can use the
.slice()
method to create a new array or string based on an existing one. This can be helpful when you need to manipulate data in a different way than the original.
How to use the .slice() method
The .slice()
method can be used on both arrays and strings (which is just an array of characters). Let’s look at some code examples to understand this better.
const arr = [1,2,3,4,5,6,7,8,9,10];
const newArr = arr.slice(3,6);
console.log(newArr);
Code language: JavaScript (javascript)
Here we have an array of integers named arr
, and we are using the slice method on it and storing the output of the slice method in a new variable named newArr
. In this case, the output of the code will be [4, 5, 6]
. But how?
The start index, in this case, is 3. This means we will start slicing the array from arr[3]
, which is 4, and we still stop at arr[6-1]
, which is 6. If you are wondering why we have subtracted 1 from the end index, it is because the end index is always exclusive, which means the end index is not included.
To better understand this concept, let’s look at another example::
const arr = ["A", "B", "C", "D", "E", "F", "G", "H", "I", "J"];
const newArr = arr.slice(3);
console.log(newArr);
Code language: JavaScript (javascript)
In this code, we don’t have any end index specified because both the start and end indexes are optional, and you can use them per your needs.
When any index is not specified, we assume that the index is either the first element (if the start index is not specified) or the length of the array. Because the index starts from 0, we’ll include all elements until the end of the array ( i.e. where the index is length-1
).
The output, in this case, will be ["D", "E", "F", "G", "H", "I", "J"]
. The start index is 3, and the end index is not mentioned, so we’ll start slicing from arr[3]
, which is “D“. As the end index is not mentioned, we’ll include all elements.
Until now, we only looked at the start and end indexes as positive numbers, but these indexes can be negative ones too.
Using .slice() with negative indexes
We have the start and end index as negative numbers in the following code block:
const arr = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const newArr = arr.slice(-3, -1);
console.log(newArr);
Code language: JavaScript (javascript)
The negative index means we’ll count the index from the end of the array. A negative index can be thought of as an offset from the end of the array, list, or string. For example, in an array with five elements, you can access the last element with an index of -1 (like arr[-1]
), the second-to-last element with an index of -2, and so on.
The output, in this case, will be [8, 9]
. The start index is arr[-3]
, which is 8, and the end index is arr[-1]
, which is 10. Since the end index is exclusive, the output will be [8, 9]
.
As we discussed before, both index arguments are optional. Let’s look at a code example with only one negative index argument.
const arr = ["A", "B", "C", "D", "E", "F"];
const newArr = arr.slice(-3);
console.log(newArr);
Code language: JavaScript (javascript)
Here we only have one index specified: -3. We’ll start from arr[-3]
, which is D, and we’ll include all elements until the end. So the output, in this case, will be ["D", "E", "F"]
.
Using .slice() on a nested array
We can use the slice method on a nested array as well:
var nestedArray = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
var newArray = nestedArray.slice(1, 3);
console.log(newArray);
Code language: JavaScript (javascript)
We have a nested array in the above-mentioned code block and are using the slice method on it. In this instance it will print the last two array elements: [[4,5,6],[7,8,9]]
. You can further access elements based on the index directly like this:
var newArray = nestedArray.slice(1, 3)[0]; // Output: [4, 5, 6]
Code language: JavaScript (javascript)
Using .slice() without indexes
Developers can also use the slice method without a start or end index, as both are optional. In this case, the output is the shallow copy of the array. Let’s look at the code to understand this better.
var array = ["a", "b", "c", "d", "e"];
var output = array.slice() // Using slice without arguments
console.log(output);
Code language: PHP (php)
Without using any index arguments, this .slice()
operation would output 'a', 'b', 'c', 'd', 'e'
.
Using .slice() on strings
As discussed earlier, the slice method can also be used on strings:
// Using slice() with any index
const str = "Hello World";
const newStr = str.slice();
console.log(newStr); // Output: "Hello World"
// Using slice() with single index
const newStr2 = str.slice(3); // end index is not specified
console.log(newStr2); // Output: lo World
// Using slice() with start and end index
const newStr3 = str.slice(3, 6);
console.log(newStr3); // Output: lo ( 3rd character is a whitespace )
// Using slice() with one negative & one positive index
const newStr4 = str.slice(0, -1);
console.log(newStr4); // Hello Worl
// Using slice() with only 1 index ( negative )
const newStr5 = str.slice(-3);
console.log(newStr5); // rld
Code language: JavaScript (javascript)
Understanding the difference between .slice() and .splice()
In JavaScript, slice()
and splice()
are both methods that developers can use to manipulate arrays. However, there is a significant difference between the two methods.
The .slice()
method returns a new array containing a subset of the elements from the original array. The elements in the new array are selected from the original array based on a start and end index that you specify.
On the other hand, the .splice()
method modifies the original array.
When you use .splice()
to add or remove elements from an array, the elements you add or remove are selected based on a start index that you specify.
So, if you want to extract a section of an array without modifying the original array, you would use the .slice()
method. And if you want to remove a section of an array and get the removed elements back, you would use the .splice()
method:
.splice(start_index, items_to_remove, item_to_insert_1, item_to_insert_2, ...)
Code language: CSS (css)
With the .splice()
method, the start_index
is a required parameter where all others are optional.
In the following code block, we have an array on which we have used both .splice()
and .slice()
:
const numbers = [1, 2, 3, 4, 5];
const newNumbers = numbers.slice(1, 4);
console.log(newNumbers); // [2, 3, 4]
// Using splice method on the same array
numbers.splice(1, 2);
console.log(numbers); // [1, 4, 5]
Code language: JavaScript (javascript)
When we used the .slice()
method, the start index and the end index are 1 and 4, respectively, which gives the output as [2, 3, 4].
On the other hand, when we used the .splice()
method on the same array, we get the output as [1, 4, 5]
because we are removing two elements from index 1 (i.e., 2 and 3).
Try it out!
Conclusion
In this blog post, we explained how you can use JavaScript’s .slice()
method. This can come in handy when working with arrays and other objects that contain several elements. We’ve also provided a few examples to explain how the method works, using code snippets, as well as how .slice()
and .splice()
differ.
We hope this post has been helpful to you, and if you want to learn more about JavaScript or other topics, check out our blog.
This post was written by Keshav Malik. Keshav is an information security engineer who loves to build and break stuff. He is constantly on the lookout for new and interesting technologies and enjoys working with a diverse set of technologies in his spare time. He loves music and plays badminton whenever the opportunity presents itself.