Frontend Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified frontend developers for your organization.
Frontend
The continually sought-after frontend developer harbors a specialized comprehension of user-facing technologies, making them an indispensable asset in virtually every application team.
According to the CoderPad 2024 Developer survey, frontend developers are the third-most in-demand job role technical recruiters are looking to fill.
The sections that follow furnish a range of hands-on coding exercises and interview inquiries devised to scrutinize a candidate’s prowess in frontend development during technical screenings.
Moreover, an assemblage of recommended best practices has been incorporated to facilitate a trustworthy appraisal of candidates’ frontend expertise through your interview questions.
Table of Contents
Frontend example questions
Question 1: Create a React Todo List
The goal of this exercise is to create a working todo list with persistent data storage.
To start with, we have a styled todo list that supports adding todos. We also have pre-made styles for completed todo items. Although there’s no working mechanism for “completing” a todo.
Requirements
- Clicking on a todo item should toggle the “checked” state.
- The todo list state should be saved and loaded from local storage.
- Checked items should sink to the bottom of the list automatically
Stretch Goals
- Allow todos to be deleted. When you hover your mouse over a todo, an X should appear on the far right side, clicking the X should remove it from the list.
- Add hidden timestamps to todos (
created_at
,completed_at
), these will be used for sorting - The active todos should be sorted by
created_at
descending - The completed todos should be sorted by
completed_at
ascending
Question 2: Angular directives project
You have two tasks to perform on this simple Angular project:
- Create a method in
src/directives/textchange.directive.ts
to add an element to the DOM whose text is a random number. - Create a method in
src/directives/basiccol.directive.ts
to create a box beneath that becomes visible upon generating a random number. There should be a color change when the box is hovered over.
Hint: the Renderer2
class is Angular’s most recommended method for manipulating the DOM. Ensure to use it while creating your functions.
Frontend skills to assess
Frontend job roles
Junior frontend interview questions
Question: What is the Document Object Model (DOM)?
Answer: The DOM is a programming interface for web documents. It represents the structure of a document as a tree of objects, where each object corresponds to a part of the document, such as an element or attribute. Through the DOM, these parts can be accessed and manipulated programmatically.
Question: Examine this HTML code and mention any potential issues.
<div>
<p id="message">Hello, World!</h2>
</div>
Code language: HTML, XML (xml)
Answer: The element with id
“message” starts with a <p>
tag but ends with a </h2>
tag. The tags are mismatched, which would cause rendering issues and potential JavaScript errors if this element is targeted by scripts.
Question: Explain the difference between ==
and ===
in JavaScript.
Answer:
==
(double equals) is an equality operator that performs type coercion if the types of the two operands are different. For instance,"5" == 5
will returntrue
.===
(triple equals) is a strict equality operator and does not perform type coercion. It returnstrue
only if the operands are of the same type and have the same value. In the previous example,"5" === 5
will returnfalse
.
Question: Given the following CSS, why might the .highlight
class not display as expected?
p {
color: blue;
}
.highlight {
color: yellow !important;
}
Code language: CSS (css)
Answer: On its own, the .highlight
class should work as expected due to the !important
declaration, which overrides other styles. However, if the problem persists, potential issues could be:
- Another
!important
rule further down the stylesheet or in a different stylesheet that overrides it. - A more specific selector elsewhere in the CSS that targets the same elements and overrides the style.
- Alternatively, since the use of
important
in CSS is considered a bad practice, it may be best to avoid it all together.
Question: What is responsive web design?
Answer: Responsive web design is an approach to designing and developing web pages so that they render well on a variety of devices and screen sizes. It often involves using flexible grids, fluid layouts, and CSS media queries to adjust the design based on the device’s characteristics, such as its width, height, or orientation.
Question: Why might the JavaScript alert not work in this HTML?
<button onclick="displayAlert()">Click me!</button>
<script>
function dispalyAlert() {
alert("Button clicked!");
}
</script>
Code language: HTML, XML (xml)
Answer: There’s a typo in the function name. The function is defined as dispalyAlert
but the button’s onclick
handler references a non-existent displayAlert
function.
Question: Why might the following JavaScript code not change the text of the <p>
element?
<p id="intro">Welcome!</p>
<script>
document.getElementById("Intro").innerText = "Hello, World!";
</script>
Code language: HTML, XML (xml)
Answer: The issue is the case sensitivity of the id
. The <p>
element’s id
is “intro” (lowercase), but the script is trying to get an element with the id
“Intro” (capitalized).
Question: Describe the box model in CSS.
Answer: The CSS box model describes the rectangular boxes generated for elements in the document tree and consists of:
- Content: The actual content of the box, where text and images appear.
- Padding: The space between the content and the border.
- Border: The outline of the box.
- Margin: The space between the box’s border and its surrounding elements.
Thebox-sizing
property can be used to determine whether thewidth
andheight
of the box include the padding and border or just the content.
Question: Why is it important to always implement your checks and sanitization server-side?
Answer: Directly sending user input to the server can expose the application to various security vulnerabilities, such as SQL injection, cross-site scripting (XSS), or other malicious attacks. Always validate and sanitize user input.
Intermediate frontend interview questions
Question: Describe the concept of CSS specificity and its importance.
Answer: CSS specificity determines which CSS rule is applied to an element. It’s calculated based on the number and type of selectors in a rule. The hierarchy (from highest to lowest specificity) is: inline styles, IDs, classes/attributes/pseudo-classes, element/pseudo-elements. If two selectors apply to the same element, the one with higher specificity wins. Understanding specificity prevents unintended styling and reduces the over-reliance on !important
.
Question: Review the following JavaScript code. What might be problematic about this?
for (var i = 0; i < 5; i++) {
setTimeout(function() {
console.log(i);
}, 1000);
}
Code language: JavaScript (javascript)
Answer: Due to the asynchronous nature of setTimeout
and the way var
is function-scoped, the loop will have completed by the time the callbacks execute. As a result, the code will print 5
five times. Using let
instead of var
would solve this issue by providing block scoping.
Question: How do CSS Flexbox and Grid differ in terms of layout creation?
Answer:
- Flexbox: Designed for one-dimensional layouts, either in a row or a column. It’s ideal for distributing space along a single axis.
- Grid: Designed for two-dimensional layouts, both rows and columns. It’s more suitable for complex layouts and alignments.
Question: Given this React component, how could you improve its performance?
class UserProfile extends React.Component {
render() {
return (
<div>
<h1>{this.props.user.name}</h1>
<p>{this.props.user.description}</p>
</div>
);
}
}
Code language: JavaScript (javascript)
Answer: If the parent component re-renders frequently but the user
prop doesn’t change often, the UserProfile
component might be re-rendered unnecessarily. Implementing shouldComponentUpdate
or using React.memo
(for functional components) can prevent unnecessary re-renders by checking prop changes.
Question: Look at the following Vue component and identify potential improvements.
<template>
<button @click="incrementCount">{{ count }}</button>
</template>
<script>
export default {
data() {
return {
count: 0
};
},
methods: {
incrementCount() {
this.count += 1;
}
}
}
</script>
Code language: HTML, XML (xml)
Answer: While the code is functional, a few improvements could be:
- Consider using a computed property if any logic or formatting based on
count
is needed. - If this component is expected to grow in complexity, using Vuex for state management might be considered.
- If there are related styles, they can be encapsulated using a
<style scoped>
tag.
Question: Inspect the following Angular code. What’s missing?
@Component({
selector: 'app-item',
template: `
<div>{{ item.name }}</div>
`
})
export class ItemComponent {
@Input() item: any;
}
Code language: JavaScript (javascript)
Answer: The type of item
is defined as any
, which is not recommended. A better approach would be to define an interface that describes the shape of item
, providing better type checking and documentation of the expected data.
Question: What is the Shadow DOM, and why is it useful?
Answer: The Shadow DOM is a browser feature that enables encapsulation in the DOM. It allows you to bundle CSS, JavaScript, and HTML into a scoped DOM subtree, which is isolated from the main document’s DOM. It’s commonly used in Web Components to provide style and behavior encapsulation.
Question: Review the following SASS code. What’s the potential issue with the nesting?
.header {
font-size: 24px;
.title {
margin: 10px;
.subtitle {
color: red;
}
}
}
Answer: Deep nesting in SASS can lead to overly specific CSS selectors when compiled. This can make styles hard to override and lead to unintentional styling due to high specificity. It’s a best practice to avoid deep nesting and ensure that generated selectors are as flat and reusable as possible.
Question: Given this Webpack configuration snippet, what does it do?
module.exports = {
module: {
rules: [
{
test: /.js$/,
exclude: /node_modules/,
use: {
loader: "babel-loader"
}
}
]
}
};
Code language: JavaScript (javascript)
Answer: This configuration tells Webpack to use the babel-loader
for all .js
files (except those in node_modules
). This means it will transpile ES6+ JavaScript into ES5, allowing the code to run in older browsers.
Senior frontend interviews questions
Question: Explain the concept and benefits of Server-Side Rendering (SSR).
Answer: SSR involves rendering a client-side or universally coded application on the server and sending a fully rendered page to the client. Benefits include:
- Faster Initial Page Load: The browser can start displaying the page as soon as it receives the first byte from the server.
- SEO: Search engines can crawl the site for better SEO because the website’s content is fully rendered.
- Optimized Performance: Reduced initial load on client devices, especially beneficial for low-powered devices.
Question: Explain what is happening in this React hook.
function useCustomHook(initialValue) {
const [value, setValue] = useState(initialValue);
useEffect(() => {
const intervalId = setInterval(() => {
setValue(prevValue => prevValue + 1);
}, 1000);
return () => clearInterval(intervalId);
}, []);
return value;
}
Code language: JavaScript (javascript)
Answer: The hook initializes a setInterval inside a useEffect
with an empty dependency array, meaning it only sets the interval once when the component mounts. The cleanup function correctly clears the interval when the component is unmounted. The code appears to be well-structured for its intention, which is to increment the value every second.
Question: Describe the benefits and drawbacks of CSS-in-JS solutions, such as styled-components or Emotion.
Answer:
Benefits:
- Scoped Styles: Avoid global namespace and specificity conflicts.
- Dynamic Styling: Easy to use component props and state to dynamically generate styles.
- Component-based: Helps maintain styles in componentized architectures like React.
Drawbacks:
- Performance: Depending on implementation, there could be a runtime cost.
- Barrier of Entry: Another thing to learn for new developers.
- Potentially Larger Bundles: Depending on usage, bundles might be larger due to injected styles.
Question: Examine this Vue component code and describe what it does and any issues you see:
<template>
<div v-if="isLoading">Loading...</div>
<div v-else>{{ data.title }}</div>
</template>
<script>
export default {
data() {
return {
isLoading: true,
data: {},
};
},
mounted() {
fetchData().then((response) => {
this.data = response;
this.isLoading = false;
});
},
methods: {
fetchData() {
return new Promise((resolve, reject) => {
// Simulating an asynchronous operation, such as an API call.
// The data returned by the promise could potentially be anything.
// The API call could also fail. In that case, the code would call "reject"
setTimeout(() => {
const data = { title: "this is my title" };
resolve(data);
}, 1000);
});
},
},
};
</script>
Code language: HTML, XML (xml)
Answer:
- Error handling is missing for the asynchronous data fetching operation. Consider adding a
.catch
to handle any errors that arise during data fetching. - The component doesn’t handle potential issues if
response
doesn’t have atitle
property.
Question: What are Progressive Web Apps (PWAs) and how do they enhance web applications?
Answer: PWAs are web applications that use modern web capabilities to provide an app-like experience to users. They can work offline, be installed on the home screen, and even send push notifications. Enhancements include:
- Reliability: Load instantly regardless of the network state.
- Performance: Respond quickly to user interactions.
- Engagement: Feel like a natural app on the device, with immersive user experiences.
Question: Review this TypeScript code:
type User = {
id: number;
name: string;
age?: number;
}
function greet(user: User) {
return `Hello, ${user.name}! You are ${user.age} years old.`;
}
Code language: JavaScript (javascript)
Answer: The age
property on the User
type is optional (age?
). However, the greet
function assumes user.age
will always exist, which could lead to the output: “Hello, Alice! You are undefined years old.”. A check should be implemented to handle cases when age
is not provided.
Question: Analyze the following Redux reducer:
function postReducer(state = [], action) {
switch (action.type) {
case 'ADD_POST':
return [...state, action.payload];
case 'DELETE_POST':
return state.filter(post => post.id !== action.payload.id);
default:
return state;
}
}
Code language: JavaScript (javascript)
Answer: The reducer seems to handle adding and deleting posts from the state. Here are some observations:
- It uses the spread operator and
filter
method to return new state objects, adhering to Redux’s immutability requirement. - There might be a potential issue if action creators dispatching ‘DELETE_POST’ do not send the
id
correctly in the payload. This could lead to unintended deletions or no action at all. - Consider adding type checks or using TypeScript for better type safety.
Question: How do virtual DOM algorithms, like the one in React, improve performance?
Answer: Virtual DOM acts as a middle layer between the state of an application and the real DOM. On state changes:
- A new virtual DOM tree is created.
- This new tree is compared (diffed) to the previous one.
- Only the actual differences (changes) are updated in the real DOM, often in a single batch, minimizing direct DOM manipulations.
This process optimizes rendering performance, making it more predictable and smoother.
Question: Examine this GraphQL query:
query GetUser($id: ID!) {
user(id: $id) {
name
friends {
name
}
}
}
Code language: PHP (php)
Answer: This GraphQL query fetches a user by an ID and retrieves the user’s name and the names of their friends. Observations:
- The query uses a variable
$id
of typeID!
. The!
indicates that it’s a non-nullable type, meaning the query requires anid
to be provided. - It fetches nested data (friends) in a single query, showcasing one of the strengths of GraphQL in reducing the need for multiple round trips to the server.
Question: Inspect this Web Component:
class CustomButton extends HTMLElement {
connectedCallback() {
this.innerHTML = `<button>Click me</button>`;
this.querySelector('button').addEventListener('click', () => {
alert('Button clicked!');
});
}
}
customElements.define('custom-button', CustomButton);
Code language: JavaScript (javascript)
Answer: This code defines a Web Component for a custom button that displays an alert when clicked. Potential improvements and observations:
- It lacks a
disconnectedCallback
to remove the event listener, which could lead to memory leaks. - The component directly alters its own innerHTML in the
connectedCallback
. Consider using a Shadow DOM to encapsulate the component’s style and behavior.
More frontend interview resources
For more guides on improving your knowledge of frontend hiring, we have outlined helpful blog posts below:
- Interview Front-End Developers. A Guide for Hiring Managers
- How to Run Front-End Developer Interviews That Don’t Suck
- 3 Design Skills You Should Look For In Front-End Candidates
Additionally, we offer the following frontend framework interview questions:
1,000 Companies use CoderPad to Screen and Interview Developers
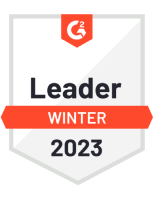
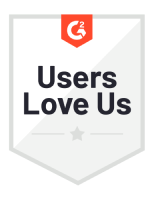
Best interview practices for frontend roles
The frontend interview procedure can vary greatly, influenced by factors such as the particular engineering role and the applicant’s extent of knowledge. To optimize the efficacy of your frontend interview questions, it’s recommended to observe the following guidelines when communicating with prospective hires:
- Formulate technical questions that mirror real-life situations within your organization – this method is not only more captivating for the candidate but also serves to better illustrate the alignment of their abilities with your team’s requirements.
- Cultivate a collaborative space by encouraging the candidate to formulate questions throughout the activity.
- Given that frontend candidates are presumed to handle front-end facets, a strong comprehension of essential UI/UX design concepts is expected.
Moreover, it’s essential to maintain traditional interview standards during frontend sessions – modify the level of difficulty of interview questions according to the candidate’s growth in expertise, convey feedback promptly concerning their position in the hiring trajectory, and grant ample space for candidates to discuss the assessment or explore the dynamics of working alongside you and your unit