Vue Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Vue developers for your organization.
Vue
Known among developers for its extremely low learning curve and flexibility, Vue continues to attract users to its component-based architecture, reactive data binding, and robust ecosystem.
According to the CoderPad 2024 Developer survey, Vue is the third most in-demand front-end framework among technical recruiters.
Outlined below are hands-on coding tasks and interview inquiries designed to assess developers’ proficiency in Vue during technical interviews. Furthermore, a compilation of best practices is provided to guarantee that your interview questions accurately measure the Vue abilities of your candidates.
Table of Contents
Vue example question
Create a reusable input component
Complete the simple input component implemented in components/BaseInput.vue
to make this page work. Your goals:
- Implement two-way data binding so the
BaseInput.vue
can be used for entering data by using the v-model directive. - Conditionally render the value that has been entered into the input when it is not empty.
Vue skills to assess
Jobs using Vue
Junior Vue interview questions
Question:
Explain the concept of data binding in Vue.js and provide an example of two-way data binding.
Answer:
Data binding in Vue.js is the process of establishing a connection between the data in the Vue instance and the DOM elements. It allows for automatic synchronization between the data and the user interface. When the data changes, the UI updates accordingly, and vice versa.
An example of two-way data binding in Vue.js is using the v-model
directive. It binds a form input element to a Vue instance data property, allowing changes in the input to update the data property and changes in the data property to update the input.
<template>
<input v-model="message" type="text">
<p>{{ message }}</p>
</template>
<script>
export default {
data() {
return {
message: ""
};
}
};
</script>
Code language: HTML, XML (xml)
In this example, the value entered in the input field will be immediately displayed in the paragraph below it due to the two-way data binding using v-model
.
Question:
Explain the purpose of Vue.js directives and provide an example of a custom directive.
Answer:
Directives in Vue.js are special attributes that provide additional functionalities to the DOM elements they are attached to. They start with the v-
prefix and are used to perform various tasks, such as data binding, conditional rendering, event handling, and more.
Here’s an example of a custom directive that adds a “shake” effect to an element when a certain condition is met:
<template>
<button v-shake="shouldShake">Click Me</button>
</template>
<script>
export default {
data() {
return {
shouldShake: false
};
},
directives: {
shake: {
bind(el, binding) {
el.style.transition = "transform 0.2s";
},
update(el, binding) {
if (binding.value) {
el.style.transform = "translateX(10px)";
} else {
el.style.transform = "translateX(0)";
}
}
}
}
};
</script>
Code language: HTML, XML (xml)
In this example, we create a custom directive called v-shake
. The directive is bound to a button element and checks for changes in the shouldShake
data property. When shouldShake
becomes true, the button shakes by translating it 10 pixels to the right.
Question:
Explain the concept of components in Vue.js and provide an example of creating and using a component.
Answer:
Components in Vue.js are reusable and self-contained units of code that encapsulate the template, logic, and styles for a specific UI element. They enable better code organization and reusability by breaking the application into smaller, manageable pieces.
Here’s an example of creating and using a simple component in Vue.js:
<template>
<div>
<h1>{{ title }}</h1>
<p>{{ content }}</p>
</div>
</template>
<script>
export default {
props: {
title: String,
content: String
}
};
</script>
Code language: HTML, XML (xml)
To use this component in another Vue instance:
<template>
<div>
<my-component title="Hello World" content="Welcome to my Vue.js app"></my-component>
</div>
</template>
<script>
import MyComponent from "@/components/MyComponent.vue";
export default {
components: {
MyComponent
}
};
</script>
Code language: HTML, XML (xml)
In this example, we create a component named MyComponent
with two props: title
and content
. The component receives these props from the parent Vue instance and displays them in the template.
Question:
Explain the purpose of Vue.js computed properties and provide an example of using a computed property.
Answer:
Computed properties in Vue.js are properties that are calculated based on the values of other data properties. They help in creating derived data from the existing data without modifying the original data.
Here’s an example of using a computed property to calculate the total price of items in a shopping cart:
<template>
<div>
<div v-for="(item, index) in cart" :key="index">
<span>{{ item.name }}</span>
<span>{{ item.price }}</span>
</div>
<p>Total: {{ totalPrice }}</p>
</div>
</template>
<script>
export default {
data() {
return {
cart: [
{ name: "Product A", price: 10 },
{ name: "Product B", price: 20 },
{ name: "Product C", price: 15 }
]
};
},
computed: {
totalPrice() {
return this.cart.reduce((total, item) => total + item.price, 0);
}
}
};
</script>
Code language: HTML, XML (xml)
In this example, the computed property totalPrice
calculates the total price of all items in the cart
data array. It is automatically updated whenever any item’s price changes or new items are added to the cart.
Question:
Explain the concept of Vue.js lifecycle hooks and provide an example of using a lifecycle hook.
Answer:
Vue.js lifecycle hooks are predefined methods that allow you to execute code at specific stages of a component’s lifecycle. These hooks enable you to perform actions like initializing data, making API calls, and cleaning up resources during different stages of a component’s existence.
Here’s an example of using the created
lifecycle hook to fetch data from an API when the component is created:
<template>
<div>
<p v-if="loading">Loading data...</p>
<ul v-else>
<li v-for="user in users" :key="user.id">{{ user.name }}</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
loading: true,
users: []
};
},
created() {
fetch("https://jsonplaceholder.typicode.com/users")
.then(response => response.json())
.then(data => {
this.users = data;
this.loading = false;
});
}
};
</script>
Code language: HTML, XML (xml)
In this example, the created
hook is used to fetch user data from a remote API (JSONPlaceholder). When the component is created, it sets the loading
flag to true
to indicate that the data is being fetched. After the data is fetched, it populates the users
array and sets loading
to false
to display the user names.
Question:
Explain the purpose of Vue.js watchers and provide an example of using a watcher.
Answer:
Watchers in Vue.js are used to perform specific actions or computations based on changes in a data property or a computed property. They are useful when you need to react to data changes with custom logic.
Here’s an example of using a watcher to log a message whenever the counter
data property changes:
<template>
<div>
<button @click="incrementCounter">Increment</button>
<p>Counter: {{ counter }}</p>
</div>
</template>
<script>
export default {
data() {
return {
counter: 0
};
},
methods:
{
incrementCounter() {
this.counter++;
}
},
watch: {
counter(newValue, oldValue) {
console.log(`Counter changed from ${oldValue} to ${newValue}`);
}
}
};
</script>
Code language: HTML, XML (xml)
In this example, the watcher is defined within the component using the watch
option. Whenever the counter
property changes, the watcher function is triggered, and it logs a message with the old and new values of the counter
.
Question:
Explain the concept of Vue.js event handling and provide an example of handling a custom event.
Answer:
In Vue.js, event handling is the process of responding to user interactions, such as clicks, keystrokes, and form submissions. Vue.js allows you to handle both built-in DOM events and custom events emitted by child components.
Here’s an example of handling a custom event emitted by a child component:
Child Component – ChildComponent.vue
:
<template>
<button @click="emitCustomEvent">Click Me</button>
</template>
<script>
export default {
methods: {
emitCustomEvent() {
this.$emit("customEvent", "Custom event emitted from child component");
}
}
};
</script>
Code language: HTML, XML (xml)
Parent Component – ParentComponent.vue
:
<template>
<div>
<child-component @customEvent="handleCustomEvent"></child-component>
<p>{{ message }}</p>
</div>
</template>
<script>
import ChildComponent from "@/components/ChildComponent.vue";
export default {
components: {
ChildComponent
},
data() {
return {
message: ""
};
},
methods: {
handleCustomEvent(data) {
this.message = data;
}
}
};
</script>
Code language: HTML, XML (xml)
In this example, the child component emits a custom event named customEvent
when the button is clicked. The parent component listens for this event using @customEvent="handleCustomEvent"
and responds by updating the message
data property with the data emitted by the child component.
Question:
Explain the concept of Vue.js mixins and provide an example of using a mixin.
Answer:
Mixins in Vue.js are a way to encapsulate reusable functionality that can be shared across multiple components. They allow you to extract common logic, methods, data, and lifecycle hooks into a separate object, which can then be mixed into any component.
Here’s an example of a mixin that provides a logMessage
method to log a message:
// mixins/logger.js
export const loggerMixin = {
methods: {
logMessage(message) {
console.log(message);
}
}
};
Code language: JavaScript (javascript)
To use the mixin in a component:
<template>
<div>
<button @click="logHello">Log Hello</button>
</div>
</template>
<script>
import { loggerMixin } from "@/mixins/logger";
export default {
mixins: [loggerMixin],
methods: {
logHello() {
this.logMessage("Hello from the component!");
}
}
};
</script>
Code language: HTML, XML (xml)
In this example, the loggerMixin
provides the logMessage
method. The component imports the mixin and includes it in the mixins
option. As a result, the component can use the logMessage
method as if it was defined directly in the component.
Question:
Explain the purpose of Vue.js router and provide an example of defining and using routes.
Answer:
The Vue Router is a plugin that provides a way to handle client-side routing in Vue.js applications. It enables the creation of single-page applications (SPAs) by allowing different components to be displayed based on the URL without full page reloads.
Here’s an example of defining and using routes using Vue Router:
Main Vue Instance – main.js
:
import Vue from "vue";
import App from "./App.vue";
import VueRouter from "vue-router";
import Home from "@/components/Home.vue";
import About from "@/components/About.vue";
Vue.use(VueRouter);
const routes = [
{ path: "/", component: Home },
{ path: "/about", component: About }
];
const router = new VueRouter({
routes
});
new Vue({
router,
render: h => h(App)
}).$mount("#app");
Code language: JavaScript (javascript)
Home.vue
:
<template>
<div>
<h1>Welcome to the Home Page</h1>
</div>
</template>
Code language: HTML, XML (xml)
About.vue
:
<template>
<div>
<h1>About Us</h1>
<p>We are a company that provides awesome products.</p>
</div>
</template>
Code language: HTML, XML (xml)
In this example, we import VueRouter
and create a new instance of it with defined routes. The router
is then added to the main Vue instance. The components Home
and About
correspond to the respective routes “/home” and “/about” and will be rendered when the user navigates to the corresponding URLs.
Question:
Explain the concept of Vue.js template refs and provide an example of using a template ref.
Answer:
Vue.js template refs allow you to access and interact with DOM elements directly from the template. They provide a way to get a reference to a specific element and use it in your component’s logic, such as modifying its attributes, styles, or handling events.
Here’s an example of using a template ref to access an input element’s value:
<template>
<div>
<input ref="inputElement" type="text" v-model="message">
<button @click="showMessage">Show Message</button>
</div>
</template>
<script>
export default {
data() {
return {
message: ""
};
},
methods: {
showMessage() {
const inputElement = this.$refs.inputElement;
alert(`You entered: ${inputElement.value}`);
}
}
};
</script>
Code language: HTML, XML (xml)
In this example, we add a ref
attribute to the input element, allowing us to access it using this.$refs.inputElement
in the component’s showMessage
method. When the button is clicked, the method retrieves the value entered in the input and shows it in an alert.
Intermediate Vue interview questions
Question:
Explain the concept of data binding in Vue.js and provide an example of two-way data binding.
Answer:
Data binding in Vue.js is the automatic synchronization of data between the model (data) and the view (UI). It allows changes in the model to be reflected in the UI, and vice versa, without manually updating the DOM. There are two types of data binding in Vue.js: one-way binding and two-way binding.
One-way binding is the default type, where the data flows from the model to the view. For example:
<template>
<div>
<p>{{ message }}</p>
<input type="text" v-bind:value="message" />
</div>
</template>
<script>
export default {
data() {
return {
message: "Hello, Vue!",
};
},
};
</script>
Code language: HTML, XML (xml)
In this example, the message
data property is displayed in a paragraph (<p>
) element using the mustache syntax ({{ message }}
). The value of the message
property is also bound to the value of the input field using the v-bind:value
directive, ensuring that any changes in the data are reflected in the input field.
Question:
Explain the Virtual DOM concept in Vue.js and how it improves performance.
Answer:
The Virtual DOM is a concept in Vue.js (and other modern frontend libraries) that improves performance by reducing the number of actual DOM manipulations. It is an abstraction of the real DOM represented as an in-memory tree-like structure.
When a Vue component’s data changes, Vue creates a virtual representation of how the DOM should look after the update. This virtual representation is then compared to the previous virtual representation to compute the minimum number of changes needed to update the real DOM. This process, called “reconciliation,” is much faster than directly manipulating the entire real DOM.
By using the Virtual DOM, Vue.js optimizes the rendering process and reduces unnecessary DOM operations, resulting in faster and more efficient updates to the user interface.
Question:
The following Vue component is intended to display a list of items fetched from an API. However, it contains a logical error and doesn’t display the items correctly. Identify the error and fix the code.
<template>
<div>
<ul>
<li v-for="item in items" :key="item.id">{{ item.name }}</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
items: [],
};
},
mounted() {
fetch("https://api.example.com/items")
.then((response) => response.json())
.then((data) => (this.items = data));
},
};
</script>
Code language: HTML, XML (xml)
Answer:
The logical error in the code is that the API call is made in the mounted()
hook, but the items
array is not updated with the fetched data correctly. The correct code is as follows:
<template>
<div>
<ul>
<li v-for="item in items" :key="item.id">{{ item.name }}</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
items: [],
};
},
mounted() {
fetch("https://api.example.com/items")
.then((response) => response.json())
.then((data) => (this.items = data.items));
},
};
</script>
Code language: HTML, XML (xml)
In this corrected code, the API response data is an object containing an array of items, so we need to update the items
array with data.items
.
Question:
What is Vuex in Vue.js, and why is it used?
Answer:
Vuex is the official state management library for Vue.js applications. It serves as a centralized store to manage the state of the entire application and provides a set of rules to ensure state changes are predictable and consistent.
Vuex is used for the following reasons:
- Centralized State: Instead of managing state in multiple components, Vuex allows you to store all the application’s state in a single store, making it easier to track and manage changes.
- Predictable State Changes: State changes are strictly controlled through mutations, making it easier to debug and trace how and when the state changes occur.
- Communication between Components: Vuex facilitates communication between components by allowing any component to access the state from the store.
- Time-Travel Debugging: Vuex supports time-travel debugging, enabling developers to review and replay state changes to track application behavior more efficiently.
- Plugins and Middleware: Vuex provides a plugin system for additional features and middleware to intercept and augment store actions.
Question:
The following Vue component is intended to show a “Hello, Guest” message when the isLoggedIn
data property is false
, and a “Welcome, User” message when it’s true
. However, it contains a syntax error and doesn’t render correctly. Identify the error and fix the code.
<template>
<div>
<p v-if="isLoggedIn">Welcome, User</p>
<p v-else>Hello, Guest</p>
</div>
</template>
<script>
export default {
data() {
return {
isLoggedIn: true,
};
},
};
</script>
Code language: HTML, XML (xml)
Answer:
The logical error in the code is that the isLoggedIn
data property is set to true
, which always shows the “Welcome, User” message, regardless of its value. The correct code is as follows:
<template>
<div>
<p v-if="isLoggedIn">Welcome, User</p>
<p v-else>Hello, Guest</p>
</div>
</template>
<script>
export default {
data() {
return {
isLoggedIn: false,
};
},
};
</script>
Code language: HTML, XML (xml)
In this corrected code, the isLoggedIn
data property is set to false
, so the “Hello, Guest” message is shown when the value is false
.
Question:
Explain the concept of Vue mixins and when you would use them in a Vue application.
Answer:
Vue mixins are a way to encapsulate reusable logic and options that can be added to multiple Vue components. A mixin is an object containing component options, such as data, methods, and lifecycle hooks, that can be merged into one or more components.
Mixins are used when you have logic or behavior that needs to be shared across multiple components. Instead of duplicating the code in each component, you can create a mixin and apply it to the components that need the shared functionality.
For example, if you have several components that require a similar method to fetch data from an API, you can create a data-fetching mixin and include it in those components. This allows you to keep your code DRY (Don’t Repeat Yourself) and makes it easier to maintain and update the shared logic.
Question:
The following Vue component is intended to show an alert message when the “Show Alert” button is clicked. However, it contains a logical error and doesn’t work as expected. Identify the error and fix the code.
<template>
<div>
<button @click="showAlert">Show Alert</button>
<div v-if
="isAlertVisible">
<p>{{ alertMessage }}</p>
</div>
</div>
</template>
<script>
export default {
data() {
return {
isAlertVisible: false,
alertMessage: "This is an alert!",
};
},
methods: {
showAlert() {
this.isAlertVisible = true;
},
},
};
</script>
Code language: HTML, XML (xml)
Answer:
The logical error in the code is that the “Show Alert” button does not trigger the showAlert()
method. The correct code is as follows:
<template>
<div>
<button @click="showAlert">Show Alert</button>
<div v-if="isAlertVisible">
<p>{{ alertMessage }}</p>
</div>
</div>
</template>
<script>
export default {
data() {
return {
isAlertVisible: false,
alertMessage: "This is an alert!",
};
},
methods: {
showAlert() {
this.isAlertVisible = !this.isAlertVisible;
},
},
};
</script>
Code language: HTML, XML (xml)
In this corrected code, the showAlert()
method toggles the value of isAlertVisible
when the “Show Alert” button is clicked, making the alert message show and hide accordingly.
Question:
Explain the concept of Vue directives and provide examples of two built-in directives.
Answer:
Vue directives are special attributes that start with the v-
prefix and are used to apply reactive behavior to the DOM elements. They are used in templates to manipulate the DOM, conditionally render elements, and apply special behaviors.
Two commonly used built-in directives in Vue are:
v-if
: Thev-if
directive conditionally renders an element based on the truthiness of the provided expression. If the expression evaluates totrue
, the element is rendered; otherwise, it is removed from the DOM.
<template>
<div>
<p v-if="showMessage">This is a message.</p>
</div>
</template>
<script>
export default {
data() {
return {
showMessage: true,
};
},
};
</script>
Code language: HTML, XML (xml)
v-for
: Thev-for
directive is used to render a list of items based on an array or object. It iterates over each item and creates a new element for each iteration.
<template>
<div>
<ul>
<li v-for="item in items" :key="item.id">{{ item.name }}</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
items: [
{ id: 1, name: "Item 1" },
{ id: 2, name: "Item 2" },
{ id: 3, name: "Item 3" },
],
};
},
};
</script>
Code language: HTML, XML (xml)
In the first example, the v-if
directive is used to conditionally render the <p>
element based on the value of showMessage
. In the second example, the v-for
directive is used to render a list of items stored in the items
data property.
Question:
The following Vue component is intended to show an image from a given imageUrl
. However, it contains a logical error and doesn’t display the image correctly. Identify the error and fix the code.
<template>
<div>
<img :src="imageUrl" alt="Image" />
</div>
</template>
<script>
export default {
data() {
return {
imageUrl: "https://example.com/image.jpg",
};
},
};
</script>
Code language: HTML, XML (xml)
Answer:
The logical error in the code is that the image URL is not valid or accessible. To fix the code, replace the imageUrl
with a valid URL that points to an accessible image.
<template>
<div>
<img :src="imageUrl" alt="Image" />
</div>
</template>
<script>
export default {
data() {
return {
imageUrl: "https://example.com/valid-image.jpg",
};
},
};
</script>
Code language: HTML, XML (xml)
In this corrected code, the imageUrl
points to a valid image URL, ensuring the image is displayed correctly.
Question:
Explain the concept of Vue single-file components (SFCs) and their benefits.
Answer:
Vue single-file components (SFCs) are a way to encapsulate the entire component’s code, including the template, script, and styles, in a single file with the .vue
extension. SFCs promote a more organized and maintainable structure for Vue applications.
Benefits of Vue single-file components include:
- Clarity and Readability: SFCs make it easier to read and understand a component’s code, as all parts of the component are in one place.
- Separation of Concerns: SFCs allow developers to separate the template, script, and styles, promoting a clear separation of concerns between different parts of the component.
- Scoped Styles: SFCs support scoped styles by default, preventing styles from affecting other components in the application.
- Build Tools Integration: SFCs can be easily processed by build tools like webpack and Vue CLI, which compile and bundle them for use in the application.
- Custom Blocks: SFCs support custom blocks, such as
<script setup>
for a simplified script setup and<i18n>
for internationalization.
Here’s an example of a simple Vue SFC:
<template>
<div>
<h1>{{ message }}</h1>
<button @click="incrementCounter">Increment</button>
</div>
</template>
<script>
export default {
data() {
return {
message: "Hello, Vue!",
counter: 0,
};
},
methods: {
incrementCounter() {
this.counter++;
},
},
};
</script>
<style>
h1 {
color: blue;
}
</style>
Code language: HTML, XML (xml)
In this example, the template, script, and styles of the component are all in one file, making it easy to manage and understand the component’s logic.
Senior Vue interview questions
Question:
Explain the concept of reactivity in Vue.js and how it simplifies the development process. Provide an example of using reactivity in a Vue component.
Answer:
Reactivity in Vue.js is a core feature that enables automatic updates of the user interface whenever the underlying data changes. When data properties are declared in the Vue instance or component, Vue automatically sets up getter and setter functions for these properties. When a property is accessed or modified, Vue tracks the dependencies and triggers re-rendering of the affected parts of the UI, keeping it in sync with the data.
Here’s an example of using reactivity in a Vue component:
<template>
<div>
<p>Count: {{ count }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script>
export default {
data() {
return {
count: 0,
};
},
methods: {
increment() {
this.count++;
},
},
};
</script>
Code language: HTML, XML (xml)
In this example, the count
data property is declared in the component’s data()
function. When the “Increment” button is clicked, the increment()
method is called, updating the count
property. Vue’s reactivity system then automatically updates the UI to display the new count value without the need for explicit DOM manipulation.
Question:
Explain the purpose of computed properties in Vue.js and how they differ from methods. Provide an example of using computed properties in a Vue component.
Answer:
Computed properties in Vue.js are properties that are derived from the underlying data but are cached and updated only when their dependencies change. They are used to perform complex data transformations or calculations, and they provide better performance compared to methods because they cache their results until their dependencies change.
Here’s an example of using computed properties in a Vue component:
<template>
<div>
<p>Radius: {{ radius }}</p>
<p>Area: {{ area }}</p>
</div>
</template>
<script>
export default {
data() {
return {
radius: 5,
};
},
computed: {
area() {
return Math.PI * Math.pow(this.radius, 2);
},
},
};
</script>
Code language: HTML, XML (xml)
In this example, the area
computed property calculates the area of a circle based on the radius
data property. Whenever the radius
changes, Vue automatically updates the area
without explicitly calling a method, providing a reactive and performant solution.
Question:
Explain the concept of Vue directives and provide examples of two commonly used directives in Vue.js.
Answer:
Vue directives are special attributes that allow you to apply reactive behavior to the DOM elements when rendering the Vue components. They start with the v-
prefix and are used to manipulate the DOM, apply conditional rendering, and bind data to the UI.
Two commonly used directives in Vue.js are:
v-if
: This directive conditionally renders an element based on the truthiness of the provided expression. If the expression evaluates to true, the element is rendered; otherwise, it is removed from the DOM.
<template>
<div>
<p v-if="showMessage">Hello, Vue developer!</p>
</div>
</template>
<script>
export default {
data() {
return {
showMessage: true,
};
},
};
</script>
Code language: HTML, XML (xml)
In this example, the p
element is rendered only when the showMessage
data property is true.
v-for
: This directive is used to render a block of elements based on an array or object data.
<template>
<div>
<ul>
<li v-for="item in items" :key="item.id">{{ item.name }}</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
items: [
{ id: 1, name: "Item 1" },
{ id: 2, name: "Item 2" },
{ id: 3, name: "Item 3" },
],
};
},
};
</script>
Code language: HTML, XML (xml)
In this example, the v-for
directive renders a list of li
elements based on the items
data array.
Question:
Explain the concept of Vue Mixins and how they promote code reuse in Vue.js. Provide an example of using a mixin in a Vue component.
Answer:
Vue Mixins are a way to encapsulate reusable logic and options that can be mixed into Vue components. They allow you to share functionality across multiple components, promoting code reuse and modularity in Vue.js applications.
Here’s an example of using a mixin in a Vue component:
<template>
<div>
<p>Count: {{ count }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script>
const counterMixin = {
data() {
return {
count: 0,
};
},
methods: {
increment() {
this.count++;
},
},
};
export default {
mixins: [counterMixin],
};
</script>
Code language: HTML, XML (xml)
In this example, the counterMixin
contains the data and methods for managing a counter. The Vue component uses the mixins
option to include the counterMixin
, enabling the component to share the counter logic and functionality.
Question:
Explain the purpose of the Vue Router and how it enables client-side routing in Vue.js applications. Provide an example of defining and using routes with Vue Router.
Answer:
The Vue Router is a plugin that provides client-side routing in Vue.js applications. It allows you to define different routes for your application and enables navigation between different views without requiring full-page reloads. The router maps URLs to specific Vue components, allowing you to build single-page applications with multiple views.
Here’s an example of defining and using routes with Vue Router:
// main.js
import Vue from "vue";
import VueRouter from "vue-router";
import App from "./App.vue";
import Home from "./views/Home.vue";
import About from "./views/About.vue";
Vue.use(VueRouter);
const routes = [
{ path: "/", component: Home },
{ path: "/about", component: About },
];
const router = new VueRouter({
routes,
});
new Vue({
render: (h) => h(App),
router,
}).$mount("#app");
Code language: JavaScript (javascript)
<!-- App.vue -->
<template>
<div>
<router-link to="/">Home</router-link>
<router-link to="/about">About</router-link>
<router-view></router-view>
</div>
</template>
<script>
export default {};
</script>
Code language: HTML, XML (xml)
In this example, we define two routes: one for the Home component and one for the About component. We use router-link
to create navigation links, and router-view
to render the corresponding components based on the current URL.
Question:
Explain the concept of Vuex and its role in managing state in Vue.js applications. Provide an example of a Vuex store and how it is used in a Vue component.
Answer:
Vuex is a state management pattern and library for Vue.js applications. It serves as a
centralized store for managing the state of the application, making it easier to manage and share data between components. Vuex follows a unidirectional data flow, and all changes to the state are done through mutations, ensuring predictability and consistency in large-scale applications.
Here’s an example of a Vuex store and how it is used in a Vue component:
// store.js
import Vue from "vue";
import Vuex from "vuex";
Vue.use(Vuex);
export default new Vuex.Store({
state: {
count: 0,
},
mutations: {
increment(state) {
state.count++;
},
},
});
Code language: JavaScript (javascript)
<!-- App.vue -->
<template>
<div>
<p>Count: {{ count }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script>
import { mapState, mapMutations } from "vuex";
export default {
computed: {
...mapState(["count"]),
},
methods: {
...mapMutations(["increment"]),
},
};
</script>
Code language: HTML, XML (xml)
In this example, we define a Vuex store with a count
state and a mutation to increment the count. The App.vue
component uses mapState
to map the count
state from the store to its computed properties, and mapMutations
to map the increment
mutation to its methods. This allows the component to access and update the state through the store without directly modifying it.
Question:
Explain the concept of Vue Transitions and provide an example of how to apply a transition to elements in a Vue component.
Answer:
Vue Transitions allow you to apply animations and transitions to elements when they are inserted, updated, or removed from the DOM. Transitions provide a smooth and visually pleasing user experience, enhancing the overall look and feel of a Vue.js application.
Here’s an example of how to apply a transition to elements in a Vue component:
<template>
<div>
<button @click="showMessage = !showMessage">Toggle Message</button>
<transition name="fade">
<p v-if="showMessage">Hello, Vue developer!</p>
</transition>
</div>
</template>
<script>
export default {
data() {
return {
showMessage: false,
};
},
};
</script>
<style>
.fade-enter-active,
.fade-leave-active {
transition: opacity 0.5s;
}
.fade-enter,
.fade-leave-to /* .fade-leave-active in <2.1.8 */ {
opacity: 0;
}
</style>
Code language: HTML, XML (xml)
In this example, we use the transition
element to apply a fade transition to the p
element. When the “Toggle Message” button is clicked, the v-if
directive controls the visibility of the p
element, and the fade transition is smoothly applied when the element enters or leaves the DOM.
Question:
Explain the concept of Vue Slots and how they facilitate component composition. Provide an example of using named slots in a Vue component.
Answer:
Vue Slots are a way to pass content from the parent component to its child components. They allow for more flexible and dynamic component composition, where the parent component can inject content into predefined slots in the child component’s template. Slots enable reusable components to be more versatile and adaptable to different use cases.
Here’s an example of using named slots in a Vue component:
<!-- ParentComponent.vue -->
<template>
<div>
<ChildComponent>
<template v-slot:title>
<h2>Welcome to Vue World!</h2>
</template>
<template v-slot:content>
<p>Vue is an awesome JavaScript framework.</p>
</template>
</ChildComponent>
</div>
</template>
Code language: HTML, XML (xml)
<!-- ChildComponent.vue -->
<template>
<div>
<slot name="title"></slot>
<slot name="content"></slot>
</div>
</template>
Code language: HTML, XML (xml)
In this example, the ParentComponent
provides content to the ChildComponent
using named slots. The content is passed within <template>
elements with the v-slot
directive. In the ChildComponent
, the slots are rendered using <slot>
elements with the corresponding name
attribute, effectively composing the content provided by the parent.
Question:
Explain the concept of Vue’s Custom Directives and how they allow for creating custom behavior for DOM elements. Provide an example of creating a custom directive in Vue.js.
Answer:
Vue’s Custom Directives are a way to extend Vue’s capabilities by creating custom behavior for DOM elements. They allow you to directly interact with the DOM at a lower level and apply custom functionality without the need for a full-fledged component.
Here’s an example of creating a custom directive in Vue.js:
// main.js
import Vue from "vue";
import App from "./App.vue";
Vue.directive("focus", {
inserted: (el) => {
el.focus();
},
});
new Vue({
render: (h) => h(App),
}).$mount("#app");
Code language: JavaScript (javascript)
<!-- App.vue -->
<template>
<div>
<input v-focus />
</div>
</template>
<script>
export default {};
</script>
Code language: HTML, XML (xml)
In this example, we define a custom directive called “focus” that focuses the input element when it is inserted into the DOM. The custom directive is used in the App.vue
component by simply adding the v-focus
attribute to the input element.
Question:
Explain the concept of Vue Server-Side Rendering (SSR) and its benefits. Provide an overview of how SSR works in Vue.js.
Answer:
Vue Server-Side Rendering (SSR) is a technique where the Vue application is rendered on the server before being sent to the client’s browser. With SSR, the initial HTML is generated on the server-side, and then the Vue application is hydrated on the client-side to enable interactivity.
Benefits of Vue SSR:
- Improved SEO: Search engines can crawl and index server-rendered pages more effectively, leading to better search engine rankings.
- Faster Time-to-Interactive (TTI): SSR reduces the time required to load and render the initial page, as the server sends a pre-rendered HTML to the client.
- Better Performance on Low-End Devices: SSR reduces the workload on the client-side, making the application more responsive, especially on low-end devices with limited processing power.
- Enhanced User Experience: The pre-rendered content is available before the client-side JavaScript loads, providing a faster perceived page load time.
Overview of how SSR works in Vue.js:
- Request: When a user requests a page, the server receives the request.
- Render: The server-side application generates the initial HTML, including the Vue components.
- Hydrate: The generated HTML is sent to the client, where the Vue application is “hydrated.” Hydration refers to the process of attaching event listeners and client-side interactivity to the existing server-rendered DOM.
- Interactivity: The client-side application takes over from this point, providing a fully interactive user experience, while still benefiting from the pre-rendered content and improved SEO.
SSR in Vue.js can be achieved using the Vue SSR framework, which takes care of rendering the Vue components on the server and hydrating them on the client. This enables developers to create Vue applications that offer both SEO advantages and improved user experiences.
1,000 Companies use CoderPad to Screen and Interview Developers
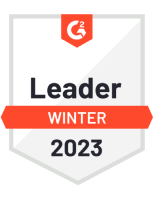
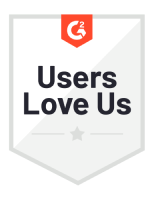
Best interview practices for Vue roles
Interview experiences for Vue candidates can vary greatly depending on factors like the specific engineering position and the applicant’s level of expertise. To optimize the efficacy of your Vue interview questions, we recommend adhering to these best practices when interacting with your candidates:
- Frame technical questions around real-world situations within your organization – this not only makes the interview more engaging for the applicant but also better illustrates the suitability of their skills for your team.
- Encourage a cooperative atmosphere by welcoming the candidate to ask questions during the exercise.
- In the case of evaluating Vue for a full-stack role, ensure that candidates understand how to incorporate the front-end features and requirements in the rest of the stack.
Furthermore, it’s vital to adhere to standard interview guidelines while conducting Vue interviews – tailor the difficulty of interview questions to the applicant’s development skill level, offer prompt feedback about their status in the recruitment process, and set aside ample time for candidates to ask about the assessment or the experience of collaborating with you and your team.