HTML/CSS/JS Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified HTML/CSS/JS developers for your organization.
HTML/CSS/JS
Despite the prevalence of frameworks like Angular, React, and Vue, using vanilla JavaScript with HTML and CSS still remains a popular way to create beautiful user interfaces. This approach is often simpler, provides greater control over the code, and often comes with smaller file sizes as you’re not importing a ton of unused libraries.
One of the biggest benefits of using HTML 5 is the addition of semantic markup, which has improved accessibility for users and readability for developers.
The following sections offer a collection of pragmatic coding tasks and interview inquiries designed to assess a developer’s proficiency in HTML/CSS/JS during coding interviews.
Furthermore, a compilation of recommended practices is incorporated to guarantee the accurate measurement of candidates’ HTML/CSS/JS expertise through your interview questions.
Table of Contents
HTML/CSS/JS example question
Add semantic markup
You have a single task to perform on this simple webpage:
- To edit the HTML source code made up of
divs
and unsemantic elements with appropriate semantic tags where they make sense. - Do this by identify the relevant
divs
and replace them with appropriate semantic tags such asnav
,main
,footer
etc. as well as including HTML attributes such asalt
,role
,aria-label
that improves accessibility - In the end, the web page’s accessibility score on Lighthouse should go from the current score of 82 to the official solution’s Accessibility score of 98.
HTML/CSS/JS skills to assess
Jobs using HTML/CSS/JS
Junior HTML/CSS/JS interview questions
Question: What is the purpose of the <div>
element in HTML, and how is it commonly used?
Answer:
The <div>
element in HTML is a generic container used to group and style other elements or sections of a web page. It does not have any specific semantic meaning and is primarily used for layout purposes and organizing content.
Question: In the code block below, there is a bug that prevents the paragraph text from appearing. Identify the bug and provide a fix.
<div>
<p>Some text here</p>
</div>
Code language: HTML, XML (xml)
Answer:
The bug is that the closing </div>
tag is missing a forward slash (“/”). The correct code should be:
<div>
<p>Some text here</p>
</div>
Code language: HTML, XML (xml)
Question: Explain the CSS Box Model and its components.
Answer:
The CSS Box Model is a fundamental concept in CSS that describes how elements are rendered and sized in a web page. It consists of four components: content, padding, border, and margin. The content area is where the actual content of the element is displayed. The padding area surrounds the content and provides space between the content and the border. The border is a line that goes around the padding and content area, and the margin is the space outside the border.
Question: In the code block below, the CSS selector is incorrect. Identify the error and provide a fix.
.styed-button {
background-color: blue;
color: white;
padding: 10px;
}
Code language: CSS (css)
Answer:
The error is in the CSS selector. It should be .styled-button
instead of .styed-button
. The correct code should be:
.styled-button {
background-color: blue;
color: white;
padding: 10px;
}
Code language: CSS (css)
Question: Explain the purpose of the querySelector
method in JavaScript, and provide an example of how to use it.
Answer:
The querySelector
method in JavaScript allows you to select elements from the DOM using CSS-style selectors. It returns the first element that matches the selector. Here’s an example:
const element = document.querySelector(".my-class");
Code language: JavaScript (javascript)
This example selects the first element with the class “my-class” and assigns it to the element
variable.
Question: In the code block below, there is a syntax error preventing the JavaScript function from executing. Identify the error and provide a fix.
function sayHello() {
console.log("Hello, world!")
}
Code language: JavaScript (javascript)
Answer:
The error is the missing closing parenthesis “)” at the end of the function definition. The correct code should be:
function sayHello() {
console.log("Hello, world!");
}
Code language: JavaScript (javascript)
Question: Explain the purpose of the href
attribute in an <a>
element in HTML, and how it is used.
Answer:
The href
attribute in an <a>
element is used to specify the URL or destination that the link should navigate to when clicked. It is an essential attribute for creating hyperlinks in HTML. The value of the href
attribute can be an absolute URL, a relative URL, or an anchor reference within the same page.
Question: In the code block below, the CSS property is missing. Identify the missing property and provide a fix.
.container {
width: 500px;
height: 300px;
background-color: red;
/* Missing CSS property */
}
Code language: CSS (css)
Answer:
The missing CSS property is border
. To fix the code, add the border
property with the desired value, such as:
.container {
width: 500px;
height: 300px;
background-color: red;
border: 1px solid black;
}
Code language: CSS (css)
Question: Explain the concept of event handling in JavaScript and provide an example of attaching an event listener to a button.
Answer:
In JavaScript, event handling involves responding to user actions or events triggered by the browser. Event listeners are functions that listen for specific events and execute code in response. Here’s an example of attaching an event listener to a button:
<button id="myButton">Click me</button>
Code language: HTML, XML (xml)
const button = document.getElementById("myButton");
button.addEventListener("click", () => {
console.log("Button clicked!");
});
Code language: JavaScript (javascript)
In this example, the event listener is attached to the button element with the id “myButton.” When the button is clicked, the anonymous arrow function is executed, and it logs “Button clicked!” to the console.
Question: In the code block below, the JavaScript function contains an error preventing it from executing. Identify the error and provide a fix.
function calculateSum(a, b) {
const sum = a + b;
console.log("The sum is: " + sum);
}
calculateSum(5, 10;
Code language: JavaScript (javascript)
Answer:
The error is the missing closing parenthesis “)” at the end of the function call. The correct code should be:
calculateSum(5, 10);
Intermediate HTML/CSS/JS interview questions
Question: What is the purpose of the <meta>
tag in HTML, and how is it commonly used?
Answer:
The <meta>
tag in HTML is used to provide metadata about the HTML document. It includes information such as character encoding, viewport settings for responsive design, keywords for SEO, and more. Here’s an example of a <meta>
tag for specifying character encoding:
<meta charset="UTF-8">
Code language: HTML, XML (xml)
Question: In the given CSS code block, there is an issue with the positioning of elements. Identify the problem and provide a fix.
.container {
position: relative;
top: 50%;
transform: translateY(-50%);
}
Code language: CSS (css)
Answer:
The issue is that the top
and transform
properties are relative to the containing block’s size, not the actual height of the element itself. To fix this, change the position
property to absolute
and adjust the positioning based on the parent container’s dimensions:
.container {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
Code language: CSS (css)
Question: Explain the concept of event bubbling and event capturing in JavaScript.
Answer:
Event bubbling and event capturing are two phases of event propagation in the DOM tree. In event bubbling, the event is first handled by the innermost element and then propagates up through its ancestors. In event capturing, the event is first captured by the outermost ancestor and then propagates down to the target element.
Event capturing and bubbling can be controlled by using the addEventListener
method with the capture
option. By default, the capturing phase is false
, and the event goes through the bubbling phase. To enable capturing, set the capture
option to true
.
Question: In the given JavaScript code, there is an error preventing the function from executing correctly. Identify the error and provide a fix.
function showInfo() {
console.log(info);
var info = "This is some information.";
}
showInfo();
Code language: JavaScript (javascript)
Answer:
The error is due to variable hoisting. The variable info
is declared after it is used in the console.log
statement. To fix the error, move the variable declaration above the console.log
statement:
function showInfo() {
var info = "This is some information.";
console.log(info);
}
showInfo();
Code language: JavaScript (javascript)
Question: What is CSS specificity, and how is it calculated? Provide an example to illustrate specificity calculation.
Answer:
CSS specificity is a set of rules that determines which styles should be applied to an element when conflicting styles are present. It is calculated based on the combination of selectors used to target an element.
Specificity is calculated by assigning weights to different parts of the selector. Inline styles have the highest weight (1,000), followed by IDs (100), classes and pseudo-classes (10), and elements and pseudo-elements (1). When multiple selectors have the same weight, the last one encountered in the stylesheet takes precedence.
Here’s an example to illustrate specificity calculation:
p {
color: blue;
}
.container p {
color: red;
}
#special {
color: green;
}
Code language: CSS (css)
In this example, the selector #special
has the highest specificity, so it will override the other styles and make the text color green.
Question: In the given HTML code, there is an issue with the layout. Identify the problem and provide a fix.
<div class="container">
<img src="image.jpg">
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</p>
</div>
Code language: JavaScript (javascript)
Answer:
The issue is that the elements are displayed vertically by default. To fix the layout and display them horizontally, use CSS flexbox or grid:
<div class="container">
<img src="image.jpg">
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</p>
</div>
Code language: JavaScript (javascript)
.container {
display: flex;
align-items: center;
}
.container img {
margin-right: 10px;
}
Code language: CSS (css)
Question: Explain the concept of closures in JavaScript and provide an example that demonstrates their use.
Answer:
Closures in JavaScript are functions that have access to variables from their outer lexical environment, even after the outer function has finished executing. Closures “remember” the environment in which they were created.
Here’s an example that demonstrates closures:
function createCounter() {
let count = 0;
return function increment() {
count++;
console.log(count);
};
}
const counter = createCounter();
counter(); // Output: 1
counter(); // Output: 2
Code language: JavaScript (javascript)
In this example, the inner function increment
is a closure that retains access to the count
variable defined in its outer scope, even after createCounter
has finished executing. Each time counter
is invoked, it increments and logs the value of count
.
Question: In the given CSS code, there is an issue with the font declaration. Identify the problem and provide a fix.
body {
font: bold 14px Arial, sans-serif;
}
Code language: CSS (css)
Answer:
The issue is with the shorthand font
property. The font-weight
value should be specified separately. To fix the code, separate the font-weight
property from the shorthand declaration:
body {
font-weight: bold;
font-size: 14px;
font-family: Arial, sans-serif;
}
Code language: CSS (css)
Question: What is the purpose of the localStorage
object in JavaScript, and how is it commonly used?
Answer:
The localStorage
object in JavaScript provides a way to store key-value pairs locally in the user’s browser. It allows data to persist even when the browser is closed and reopened. The stored data remains available until it is explicitly cleared or removed.
localStorage
is commonly used for tasks such as saving user preferences, caching data, and implementing offline capabilities in web applications. It provides a simple API with methods like setItem
, getItem
, and removeItem
to store, retrieve, and remove data.
Question: In the given JavaScript code, there is an error that prevents the function from executing correctly. Identify the error and provide a fix.
function fetchData() {
fetch("https://api.example.com/data")
.then((response) => response.json())
.then((data) => {
console.log(data);
})
.catch((error) => {
console.log(error);
});
}
fetchData();
Code language: JavaScript (javascript)
Answer:
The error is due to a network request being blocked by the browser’s same-origin policy. To fix the error, ensure that the API endpoint you are fetching data from allows cross-origin requests (CORS). This typically involves setting appropriate response headers on the server-side to allow requests from different domains. If you have control over the API, you can enable CORS. Otherwise, consider using a proxy server or contacting the API provider for assistance.
Senior HTML/CSS/JS interview questions
Question: Explain the concept of progressive enhancement in web development and how it relates to graceful degradation.
Answer:
Progressive enhancement is an approach to web development that focuses on providing a baseline experience for all users and then enhancing that experience for users with more advanced browsers or devices. It starts with a solid foundation of accessible and semantically meaningful HTML, and then additional layers of functionality, styling, and interactivity are added using CSS and JavaScript.
Graceful degradation, on the other hand, takes the opposite approach. It starts with a fully-featured experience and then ensures that the website or application still functions reasonably well even in older browsers or when certain features are not supported.
Both progressive enhancement and graceful degradation aim to ensure that the user experience is not compromised, regardless of the user’s browser or device capabilities.
Question: In the given JavaScript code, there is a performance issue. Identify the problem and provide a more efficient solution.
const elements = document.getElementsByClassName("item");
for (let i = 0; i < elements.length; i++) {
elements[i].addEventListener("click", function() {
// Perform some action
});
}
Code language: JavaScript (javascript)
Answer:
The performance issue in the code is that a separate event listener is added to each element using a loop. This can lead to poor performance, especially when dealing with a large number of elements.
A more efficient solution would be to use event delegation. Instead of adding event listeners to individual elements, you can add a single event listener to a parent element and then check the event target to determine which specific element was clicked. This way, you only have one event listener, regardless of the number of elements.
Here’s an example of using event delegation to handle click events:
const parentElement = document.getElementById("parent");
parentElement.addEventListener("click", function(event) {
if (event.target.classList.contains("item")) {
// Perform some action
}
});
Code language: JavaScript (javascript)
Question: Explain the concept of CSS preprocessors and their benefits in CSS development. Provide an example of a popular CSS preprocessor and explain its key features.
Answer:
CSS preprocessors are tools that extend the capabilities of CSS by adding features like variables, nesting, mixins, and functions. They allow developers to write more modular and maintainable CSS code and provide an abstraction layer on top of raw CSS.
One popular CSS preprocessor is Sass (Syntactically Awesome Style Sheets). Sass introduces features like variables, nesting, mixins, functions, and more. It provides benefits like code reusability, improved maintainability, easier theming, and more concise and readable code.
Here’s an example of using Sass:
$primary-color: #007bff;
.button {
background-color: $primary-color;
color: white;
&:hover {
background-color: darken($primary-color, 10%);
}
}
Code language: PHP (php)
In this example, Sass variables ($primary-color
) are used to define reusable values, nesting is used to scope styles under the .button
class, and the darken
function is used to darken the background color on hover.
Question: Explain the concept of object-oriented CSS (OOCSS) and how it can improve CSS maintainability and reusability.
Answer:
Object-oriented CSS (OOCSS) is an approach to writing CSS that focuses on separating structure (objects) from skin (visual styles). It involves creating reusable CSS classes that represent objects or components rather than styling specific elements.
By separating structure and skin, OOCSS promotes code reusability and maintainability. Objects can be reused across different elements, reducing code duplication. Skin classes provide visual styles and can be applied to multiple objects, allowing for consistent styling across the website or application.
OOCSS also enables easier updates and modifications. By isolating structure and skin, changes to the visual styles can be made without affecting the underlying structure or layout, making maintenance and updates more efficient.
Question: In the given JavaScript code, there is a problem with variable scope. Identify the issue and provide a fix.
function printNumbers() {
for (var i = 0; i < 5; i++) {
setTimeout(function() {
console.log(i);
}, 1000);
}
}
printNumbers();
Code language: JavaScript (javascript)
Answer:
The issue is that the variable i
is declared using var
, which has function scope, not block scope. As a result, all the setTimeout
callbacks reference the same variable i
, which has the value of 5 at the end of the loop.
To fix the problem, you can use a block-scoped variable by replacing var
with let
:
function printNumbers() {
for (let i = 0; i < 5; i++) {
setTimeout(function() {
console.log(i);
}, 1000);
}
}
printNumbers();
Code language: JavaScript (javascript)
Using let
creates a new lexical scope for each iteration of the loop, ensuring that each setTimeout
callback captures the correct value of i
.
Question: Explain the concept of the Document Object Model (DOM) and how it relates to web development.
Answer:
The Document Object Model (DOM) is a programming interface for HTML and XML documents. It represents the structure and content of a web page as a hierarchical tree of objects. Each element, attribute, and text node in the HTML document is represented as an object in the DOM tree, allowing developers to access and manipulate the document’s structure, content, and styles using JavaScript or other programming languages.
The DOM provides methods and properties to dynamically create, modify, or remove elements, update styles and classes, handle events, and traverse the document tree. It plays a vital role in web development, enabling interactive and dynamic web pages through the manipulation of the document’s structure and content.
Question: In the given CSS code, there is a specificity issue that prevents the styles from being applied correctly. Identify the problem and provide a fix.
.container p {
color: red;
}
p {
color: blue;
}
Code language: CSS (css)
Answer:
The issue is that the first CSS rule has higher specificity due to the combination of the class selector (.container
) and the element selector (p
). As a result, the color “red” is applied to all paragraphs inside the .container
class, overriding the color “blue” specified by the second rule.
To fix the specificity issue, you can increase the specificity of the second rule by adding a class or ID selector to target specific paragraphs:
.container p {
color: red;
}
.special-paragraph {
color: blue;
}
Code language: CSS (css)
By adding the .special-paragraph
class to specific paragraphs, you ensure that the styles defined in the second rule are applied with higher specificity.
Question: Explain the concept of reflow and repaint in relation to browser rendering and performance optimization.
Answer:
Reflow and repaint are two critical aspects of browser rendering and performance optimization.
Reflow (or layout) is the process of calculating the position and size of elements on a web page. It occurs when changes are made to the layout, such as modifying dimensions, adding or removing elements, or changing styles that affect the document’s flow. Reflow is computationally expensive and can impact performance, especially if triggered frequently or on large parts of the page.
Repaint (or redraw) is the process of updating the visual representation of elements on the screen. It occurs when changes are made to the visual appearance of elements that don’t affect their layout properties, such as modifying colors, borders, or visibility. Repaint is less computationally expensive than reflow but can still impact performance, especially when applied to numerous elements or with complex styles.
Optimizing reflow and repaint is crucial for improving page performance. Minimizing layout changes, using efficient CSS properties, reducing unnecessary DOM manipulation, and leveraging techniques like CSS transforms and hardware acceleration can help mitigate the performance impact of reflow and repaint.
Question: In the given JavaScript code, there is an error that prevents the function from executing correctly. Identify the error and provide a fix.
function fetchData() {
return fetch("https://api.example.com/data")
.then((response) => response.json())
.then((data) => {
console.log(data);
})
.catch((error) => {
console.log(error);
});
}
async function processData() {
try {
const data = await fetchData();
// Perform further processing
} catch (error) {
console.log(error);
}
}
processData();
Code language: JavaScript (javascript)
Answer:
The error is that the fetchData
function returns a promise, but the await
keyword is not used to handle the promise in the processData
function. To fix the error, you can modify the processData
function to use await
when calling fetchData
:
async function processData() {
try {
const data = await fetchData();
// Perform further processing
} catch (error) {
console.log(error);
}
}
processData();
Code language: JavaScript (javascript)
By adding the await
keyword before fetchData()
, the processData
function will wait for the promise to resolve and assign the result to the data
variable.
Question: Explain the concept of responsive web design and the techniques used to achieve it.
Answer:
Responsive web design is an approach to building websites that ensures optimal user experience across different devices and screen sizes. It involves designing and developing websites that automatically adapt their layout, content, and functionality based on the device’s capabilities and screen size.
The key techniques used in responsive web design include:
- Fluid Grids: Designing layouts based on relative units (e.g., percentages) rather than fixed units (e.g., pixels) to allow elements to scale proportionally.
- Flexible Images: Using CSS techniques like
max-width: 100%
to ensure images adapt to the container size and avoid overflowing or distorting the layout. - Media Queries: Applying different CSS styles based on the device’s characteristics (e.g., screen width, device orientation) using media queries.
- Breakpoints: Defining specific screen widths at which the layout should change to accommodate different devices, and applying appropriate CSS rules accordingly.
- Mobile-First Approach: Designing and developing websites starting from the mobile layout and progressively enhancing the experience for larger screens.
By combining these techniques, responsive web design enables websites to provide a consistent and user-friendly experience, regardless of the device or screen size used to access them.
1,000 Companies use CoderPad to Screen and Interview Developers
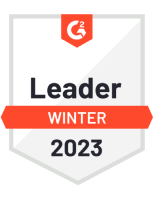
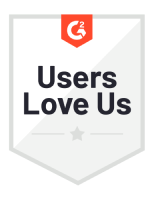
Best interview practices for HTML/CSS/JS roles
The HTML/CSS/JS interview process can differ significantly based on factors such as the specific engineering role and the candidate’s expertise level. To enhance the effectiveness of your HTML/CSS/JS interview questions, we suggest following these best practices when engaging with your candidates:
- Develop technical questions related to real-world scenarios within your organization – this approach is not only more engaging for the candidate but also better demonstrates the compatibility of their skills with your team.
- Foster a collaborative environment by inviting the candidate to ask questions throughout the exercise.
- If you’re assessing HTML/CSS/JS as part of a full-stack position, confirm that candidates are comfortable with integrating front-end aspects into the rest of the stack.
Furthermore, it is crucial to adhere to standard interview practices when conducting HTML/CSS/JS interviews – adjust the complexity of interview questions based on the candidate’s development skill level, provide timely feedback on their standing in the hiring process, and allocate sufficient time for candidates to inquire about the evaluation or the experience of working with you and your team.