Angular Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Angular developers for your organization.
Angular
The Google-developed Angular consistently ranks as a top front-end framework due to its built-in TypeScript support, two-way data binding, and powerful CLI.
According to the CoderPad 2024 Developer survey, Angular 2+ is the second-most in-demand front-end framework among technical recruiters.
The following sections offer a collection of pragmatic coding tasks and interview inquiries designed to assess a developer’s proficiency in Angular during coding interviews.
Furthermore, a compilation of recommended practices is incorporated to guarantee the accurate measurement of candidates’ Angular expertise through your interview questions.
Table of Contents
Angular example question
Angular directives project
You have two tasks to perform on this simple Angular project:
- Create a method in
src/directives/textchange.directive.ts
to add an element to the DOM whose text is a random number. - Create a method in
src/directives/basiccol.directive.ts
to create a box beneath that becomes visible upon generating a random number. There should be a color change when the box is hovered over.
Hint: the Renderer2
class is Angular’s most recommended method for manipulating the DOM. Ensure to use it while creating your functions.
Angular skills to assess
Jobs using Angular
Junior Angular interview questions
Question:
The following Angular component is not correctly defining the AppComponent
class. Identify and correct the issue.
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: '<h1>Hello, {{ name }}</h1>',
})
export class AppComponent {
name: string = 'John Doe';
Code language: JavaScript (javascript)
Answer:
The issue is that the AppComponent
class is missing the closing curly brace (}
). To fix it, add the missing curly brace at the end of the class definition as follows:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: '<h1>Hello, {{ name }}</h1>',
})
export class AppComponent {
name: string = 'John Doe';
}
Code language: JavaScript (javascript)
With this correction, the AppComponent
class is properly defined.
Question:
What is dependency injection in Angular and why is it important?
Answer:
Dependency injection is a design pattern used in Angular to manage the dependencies of an application. It allows you to provide instances of classes or services to components or other services that need them. By using dependency injection, you can easily replace dependencies, write more modular and testable code, and promote code reusability.
Question:
The following Angular template is not correctly binding to the items
property of the component. Identify and correct the issue.
import { Component } from '@angular/core';
@Component({
selector: 'app-item-list',
template: `
<ul>
<li *ngFor="let item of items">{{ item }}</li>
</ul>
`,
})
export class ItemListComponent {
items: string[] = ['Item 1', 'Item 2', 'Item 3'];
}
Code language: JavaScript (javascript)
Answer:
The issue is that the items
property is not declared as an input property using the @Input
decorator. To fix it, modify the component as follows:
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-item-list',
template: `
<ul>
<li *ngFor="let item of items">{{ item }}</li>
</ul>
`,
})
export class ItemListComponent {
@Input() items: string[] = ['Item 1', 'Item 2', 'Item 3'];
}
Code language: JavaScript (javascript)
Question:
Explain the concept of Angular modules and their purpose in an application.
Answer:
Angular modules are used to organize and encapsulate related functionality within an Angular application. They serve as containers for components, directives, pipes, and services that belong to a specific feature or functionality. Modules help in organizing the codebase, promoting reusability, and managing dependencies. They also enable lazy loading and improve performance by loading only the required modules on demand.
Question:
The following Angular component has a mistake in the constructor that prevents the service dependency from being injected. Identify and correct the issue.
import { Component } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-my-component',
template: '<h1>Data: {{ data }}</h1>',
})
export class MyComponent {
data: string;
constructor(dataService: DataService) {
this.data = dataService.getData();
}
}
Code language: JavaScript (javascript)
Answer:
The issue is that the dataService
parameter in the constructor is missing the access modifier private
. To fix it, modify the constructor as follows:
import { Component } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-my-component',
template: '<h1>Data: {{ data }}</h1>',
})
export class MyComponent {
data: string;
constructor(private dataService: DataService) {
this.data = dataService.getData();
}
}
Code language: JavaScript (javascript)
Question:
What is Angular routing and how does it work?
Answer:
Angular routing is a mechanism that allows navigation between different components and views within an application. It enables users to move from one page to another without requiring a full page reload. Angular routing uses the Angular Router module to define routes, map them to components, and handle navigation. Routes are configured with specific URLs, and when a user navigates to a URL, Angular Router loads the associated component and updates the view.
Question:
The following Angular template has a typo that causes a compilation error. Identify and correct the issue.
import { Component } from '@angular/core';
@Component({
selector: 'app-counter',
template: '<p>Counter: {{ count }}<p>',
})
export class CounterComponent {
count = 0;
}
Code language: JavaScript (javascript)
Answer:
The issue is that the closing </p>
tag is missing the forward slash /
. To fix it, modify the template as follows:
<p>Counter: {{ count }}</p>
Code language: HTML, XML (xml)
Question:
What are Angular directives? Provide examples of built-in directives and explain their usage.
Answer:
Angular directives are markers on DOM elements that tell Angular to attach specific behaviors or apply transformations to those elements. They are used to manipulate the structure and behavior of components and templates.
Examples of built-in directives in Angular include:
ngIf
: Conditionally renders an element based on a condition.ngFor
: Iterates over a collection and generates DOM elements for each item.ngStyle
: Binds CSS styles to an element based on specified expressions.ngClass
: Binds CSS classes to an element based on specified conditions.
These directives are used within templates to dynamically control the rendering, styling, and behavior of elements based on application logic.
Question:
The following Angular component is missing the necessary imports for Component
and Input
. Identify and correct the issue.
@Component({
selector: 'app-product',
template: '<h1>{{ name }}</h1>',
})
export class ProductComponent {
@Input() name: string;
}
Code language: JavaScript (javascript)
Answer:
The issue is that the Component
and Input
imports are missing. To fix it, add the necessary import statements as follows:
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-product',
template: '<h1>{{ name }}</h1>',
})
export class ProductComponent {
@Input() name: string;
}
Code language: JavaScript (javascript)
Question:
Theoretical: What is the purpose of Angular services and how are they used within components?
Answer:
Angular services are used to organize and share code across multiple components or throughout an application. They provide a way to encapsulate and manage application logic, data retrieval, communication with servers, and other common tasks. Services are typically used within components by injecting them as dependencies. This allows components to access the functionality and data provided by the service, promoting code separation, reusability, and testability.
Intermediate Angular interview questions
Question:
The following Angular component is not correctly subscribing to a data service and fetching the data. Identify and correct the issue.
import { Component, OnInit } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-data',
template: '<ul><li *ngFor="let item of items">{{ item.name }}</li></ul>',
})
export class DataComponent implements OnInit {
items: any[];
constructor(private dataService: DataService) {}
ngOnInit() {
this.dataService.getData().subscribe((data: any[]) => {
this.items = data;
});
}
}
Code language: JavaScript (javascript)
Answer:
The issue is that the component is not importing the DataService
correctly. To fix it, ensure that the correct import statement is added at the top of the file:
import { Component, OnInit } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-data',
template: '<ul><li *ngFor="let item of items">{{ item.name }}</li></ul>',
})
export class DataComponent implements OnInit {
items: any[];
constructor(private dataService: DataService) {}
ngOnInit() {
this.dataService.getData().subscribe((data: any[]) => {
this.items = data;
});
}
}
Code language: JavaScript (javascript)
Question:
Theoretical: What is the purpose of Angular CLI? How does it simplify Angular development?
Answer:
Angular CLI (Command Line Interface) is a command-line tool used for developing Angular applications. It provides a set of powerful features that simplify and automate various development tasks, such as creating new projects, generating components, services, and modules, running tests, optimizing the build, and more. Angular CLI abstracts away the complex configuration and setup required for an Angular project, allowing developers to focus on writing code and improving productivity.
Question:
The following Angular component is not correctly handling an HTTP error. Identify and correct the issue.
import { Component, OnInit } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-data',
template: '<ul><li *ngFor="let item of items">{{ item.name }}</li></ul>',
})
export class DataComponent implements OnInit {
items: any[];
error: string;
constructor(private http: HttpClient) {}
ngOnInit() {
this.http.get('https://api.example.com/data').subscribe(
(data: any[]) => {
this.items = data;
},
(error) => {
this.error = 'An error occurred while fetching the data.';
}
);
}
}
Code language: JavaScript (javascript)
Answer:
The issue is that the error handling is not properly implemented. To fix it, add the error parameter in the error callback function and assign the error message to the error
property:
import { Component, OnInit } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-data',
template: '<ul><li *ngFor="let item of items">{{ item.name }}</li></ul>',
})
export class DataComponent implements OnInit {
items: any[];
error: string;
constructor(private http: HttpClient) {}
ngOnInit() {
this.http.get('https://api.example.com/data').subscribe(
(data: any[]) => {
this.items = data;
},
(error) => {
this.error = 'An error occurred while fetching the data: ' + error.message;
}
);
}
}
Code language: JavaScript (javascript)
Question:
What are Angular guards and how are they used in routing?
Answer:
Angular guards are a feature of Angular’s routing module that allow developers to control and manage navigation to and from routes. Guards can be used to implement various security and authentication checks, route access permissions, and other pre-navigation checks. There are different types of guards, including CanActivate
, CanActivateChild
, CanDeactivate
, and CanLoad
. They are implemented as classes that can be attached to routes in the route configuration to control access and navigation behavior.
Question:
The following Angular component is not correctly using the async
pipe to handle an asynchronous data stream. Identify and correct the issue.
import { Component } from '@angular/core';
import { Observable } from 'rxjs';
import { DataService } from './data.service';
@Component({
selector: 'app-data',
template: '<ul><li *ngFor="let item of items | async">{{ item.name }}</li></ul>',
})
export class DataComponent {
items: any[];
constructor(private dataService: DataService) {
this.items = this.dataService.getData();
}
}
Code language: JavaScript (javascript)
Answer:
The issue is that the items
property is not correctly assigned an observable value. To fix it, update the assignment to use the getData()
method as an observable:
import { Component } from '@angular/core';
import { Observable } from 'rxjs';
import { DataService } from './data.service';
@Component({
selector: 'app-data',
template: '<ul><li *ngFor="let item of items | async">{{ item.name }}</li></ul>',
})
export class DataComponent {
items: Observable<any[]>;
constructor(private dataService: DataService) {
this.items = this.dataService.getData();
}
}
Code language: JavaScript (javascript)
Question:
Theoretical: What is Angular’s HttpClient
module and how is it used for making HTTP requests?
Answer:
Angular’s HttpClient
module is a built-in module that provides a simplified way to make HTTP requests from an Angular application. It offers a higher-level API compared to the older Http
module. HttpClient provides methods such as get()
, post()
, put()
, delete()
, etc., to perform various HTTP operations. It supports request and response interception, error handling, progress tracking, and configuration options like headers and query parameters. HttpClient returns observables that can be subscribed to in order to retrieve response data.
Question:
The following Angular component is not correctly implementing a reactive form. Identify and correct the issue.
import { Component } from '@angular/core';
import { FormGroup, FormControl } from '@angular/forms';
@Component({
selector: 'app-form',
template: `
<form [formGroup]="form">
<label>Name:</label>
<input formControlName="name" placeholder="Enter your name" />
</form>
<p>You entered: {{ form.controls.name.value }}</p>
`,
})
export class FormComponent {
form: FormGroup;
constructor() {
this.form = new FormGroup({
name: new FormControl(),
});
}
}
Code language: JavaScript (javascript)
Answer:
The issue is that the form control binding is missing the formControlName
attribute in the input element. To fix it, add the formControlName
attribute with the corresponding control name:
import { Component } from '@angular/core';
import { FormGroup, FormControl } from '@angular/forms';
@Component({
selector: 'app-form',
template: `
<form [formGroup]="form">
<label>Name:</label>
<input formControlName="name" placeholder="Enter your name" />
</form>
<p>You entered: {{ form.controls.name.value }}</p>
`,
})
export class FormComponent {
form: FormGroup;
constructor() {
this.form = new FormGroup({
name: new FormControl(),
});
}
}
Code language: JavaScript (javascript)
Question:
Explain the concept of lazy loading in Angular and its benefits in large applications.
Answer:
Lazy loading is a technique in Angular where modules are loaded only when they are needed, rather than loading the entire application upfront. In large applications, lazy loading can significantly improve performance by reducing the initial bundle size and decreasing the load time. It allows splitting the application into smaller feature modules that can be loaded on-demand, as the user navigates to specific routes. Lazy loading helps optimize resource utilization, improves the user experience, and reduces the time required for the initial application load.
Question:
The following Angular component is not correctly implementing a custom directive. Identify and correct the issue.
import { Directive, ElementRef } from '@angular/core';
@Directive({
selector: '[appCustomDirective]',
})
export class CustomDirective {
constructor(private elementRef: ElementRef) {
elementRef.nativeElement.style.backgroundColor = 'red';
elementRef.nativeElement.style.color = 'white';
}
}
Code language: JavaScript (javascript)
Answer:
The issue is that the code is missing the necessary import statement for ElementRef
. To fix it, add the import statement at the top of the file:
import { Directive, ElementRef } from '@angular/core';
@Directive({
selector: '[appCustomDirective]',
})
export class CustomDirective {
constructor(private elementRef: ElementRef) {
elementRef.nativeElement.style.backgroundColor = 'red';
elementRef.nativeElement.style.color = 'white';
}
}
Code language: JavaScript (javascript)
Question:
What are Angular templates and how are they used to define the user interface in Angular applications?
Answer:
Angular templates are HTML-based views that define the user interface of an Angular component. They provide a way to structure and render the desired content on the screen. Angular templates can include HTML elements, data bindings, directives, event bindings, and template expressions. They are used in conjunction with component classes to define the behavior and presentation logic of the application. Templates support features like structural directives (*ngFor
, *ngIf
), property binding ([property]
), event binding ((event)
), and interpolation ({{ expression }}
), enabling dynamic and interactive rendering of content.
Senior Angular interview questions
Question:
The following Angular component is not correctly implementing lazy loading for a feature module. Identify and correct the issue.
import { Component } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
@Component({
selector: 'app-root',
template: `
<router-outlet></router-outlet>
`,
})
export class AppComponent {}
const routes: Routes = [
{
path: 'dashboard',
loadChildren: './dashboard/dashboard.module',
},
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule],
})
export class AppRoutingModule {}
Code language: JavaScript (javascript)
Answer:
The issue is that the loadChildren
property is not correctly specifying the path to the feature module. To fix it, update the loadChildren
value to include the correct module path:
const routes: Routes = [
{
path: 'dashboard',
loadChildren: () => import('./dashboard/dashboard.module').then(m => m.DashboardModule),
},
];
Code language: JavaScript (javascript)
Question:
Explain the concept of AOT (Ahead-of-Time) compilation in Angular and its benefits.
Answer:
AOT (Ahead-of-Time) compilation is a compilation technique used in Angular to convert Angular templates and components into highly optimized JavaScript code during the build process. Unlike JIT (Just-in-Time) compilation, which compiles templates at runtime in the browser, AOT compilation precompiles templates and components before the application is deployed. The benefits of AOT compilation include improved application performance, smaller bundle sizes, early error detection, and better security by eliminating the need to ship the Angular compiler to the client.
Question:
The following Angular component is not correctly implementing change detection. Identify and correct the issue.
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-user',
template: '<h1>{{ user.name }}</h1>',
})
export class UserComponent {
@Input() user: any;
}
Code language: JavaScript (javascript)
Answer:
The issue is that the UserComponent
is missing the change detection strategy. To fix it, add the ChangeDetectionStrategy
property to the component and specify the desired strategy:
import { Component, Input, ChangeDetectionStrategy } from '@angular/core';
@Component({
selector: 'app-user',
template: '<h1>{{ user.name }}</h1>',
changeDetection: ChangeDetectionStrategy.OnPush,
})
export class UserComponent {
@Input() user: any;
}
Code language: JavaScript (javascript)
Question:
What are Angular schematics? How can they be used to generate and modify code in an Angular project?
Answer:
Angular schematics are generators and transformers of code and files in an Angular project. They provide a way to automate common development tasks, such as generating components, services, modules, and other artifacts, as well as modifying existing code. Schematics can be executed using the Angular CLI or directly through the Schematics API. They allow developers to define custom blueprints and templates, apply code transformations, and enforce project-specific conventions and best practices, thus enhancing development efficiency and consistency.
Question:
The following Angular component is not correctly using RxJS operators to handle data streams. Identify and correct the issue.
import { Component } from '@angular/core';
import { Observable } from 'rxjs/Observable';
import { map } from 'rxjs';
@Component({
selector: 'app-data',
template: '<ul><li *ngFor="let item of
items$ | async">{{ item }}</li></ul>',
})
export class DataComponent {
items$: Observable<string[]>;
constructor() {
this.items$ = this.getData().pipe(
map((data: any[]) => {
return data.map(item => item.name);
})
);
}
getData(): Observable<any[]> {
// Simulated HTTP request
return new Observable(observer => {
setTimeout(() => {
observer.next([{ name: 'Item 1' }, { name: 'Item 2' }, { name: 'Item 3' }]);
observer.complete();
}, 1000);
});
}
}
Code language: PHP (php)
Answer:
The issue is that the import statement for the map
operator is incorrect. To fix it, update the import statement to import map
from the correct location:
import { Component } from '@angular/core';
import { Observable } from 'rxjs';
import { map } from 'rxjs/operators';
@Component({
selector: 'app-data',
template: '<ul><li *ngFor="let item of items$ | async">{{ item }}</li></ul>',
})
export class DataComponent {
items$: Observable<string[]>;
constructor() {
this.items$ = this.getData().pipe(
map((data: any[]) => {
return data.map(item => item.name);
})
);
}
getData(): Observable<any[]> {
// Simulated HTTP request
return new Observable(observer => {
setTimeout(() => {
observer.next([{ name: 'Item 1' }, { name: 'Item 2' }, { name: 'Item 3' }]);
observer.complete();
}, 1000);
});
}
}
Code language: JavaScript (javascript)
Question:
What is NgRx and how does it facilitate state management in Angular applications?
Answer:
NgRx is a library for reactive state management in Angular applications. It is based on the principles of Redux, a popular state management pattern and library. NgRx provides a set of building blocks, including actions, reducers, selectors, and effects, to manage the state of an application in a predictable and scalable way. It enables developers to centralize and manage application state, make state changes explicit and traceable, handle complex asynchronous operations, and easily share state between components. NgRx follows a unidirectional data flow and encourages immutability and pure functions for state updates.
Question:
The following Angular component is not correctly implementing server-side rendering (SSR). Identify and correct the issue.
import { Component, OnInit } from '@angular/core';
import { TransferState, makeStateKey } from '@angular/platform-browser';
@Component({
selector: 'app-home',
template: '<h1>Welcome to {{ title }}</h1>',
})
export class HomeComponent implements OnInit {
title: string;
constructor(private transferState: TransferState) {}
ngOnInit() {
const key = makeStateKey<string>('title');
this.title = this.transferState.get(key, '');
this.transferState.set(key, 'Angular SSR Example');
}
}
Code language: JavaScript (javascript)
Answer:
The issue is that the code is missing the necessary import statement for TransferState
and makeStateKey
. To fix it, add the import statements at the top of the file:
import { Component, OnInit } from '@angular/core';
import { TransferState, makeStateKey } from '@angular/platform-browser';
@Component({
selector: 'app-home',
template: '<h1>Welcome to {{ title }}</h1>',
})
export class HomeComponent implements OnInit {
title: string;
constructor(private transferState: TransferState) {}
ngOnInit() {
const key = makeStateKey<string>('title');
this.title = this.transferState.get(key, '');
this.transferState.set(key, 'Angular SSR Example');
}
}
Code language: JavaScript (javascript)
Question:
What are Angular interceptors and how are they used in HTTP requests?
Answer:
Angular interceptors are a feature of Angular’s HttpClient module that allow developers to intercept and modify HTTP requests and responses. Interceptors provide a way to add custom logic or behaviors, such as request authentication, error handling, caching, logging, or adding headers, to HTTP communications. They can be used to implement cross-cutting concerns and avoid duplicating code across multiple HTTP requests. Interceptors are implemented as classes that can be registered globally or locally in the HttpClient
configuration to intercept HTTP requests and responses at various stages of the communication process.
Question:
The following Angular component is not correctly implementing component communication using a service. Identify and correct the issue.
import { Component } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-parent',
template: `
<h1>Parent Component</h1>
<button (click)="updateData()">Update Data</button>
`,
})
export class ParentComponent {
constructor(private dataService: DataService) {}
updateData() {
this.dataService.setData('New data');
}
}
@Component({
selector: 'app-child',
template: '<h2>{{ data }}</h2>',
})
export class ChildComponent {
data: string;
constructor(private dataService: DataService) {}
ngOnInit() {
this.data = this.dataService.getData();
}
}
@Injectable()
export class DataService {
private data: string;
setData(value: string) {
this.data = value;
}
getData(): string {
return this.data;
}
}
Code language: JavaScript (javascript)
Answer:
The issue is that the DataService
is not registered as a provider in the module. To fix it, add the DataService
to the providers array in the module:
import { Component, Injectable } from '@angular/core';
@Injectable()
export class DataService {
private data: string;
setData(value: string) {
this.data = value;
}
getData(): string {
return this.data;
}
}
@Component({
selector: 'app-parent',
template: `
<h1>Parent Component</h1>
<button (click)="updateData()">Update Data</button>
`,
})
export class ParentComponent {
constructor(private dataService: DataService) {}
updateData() {
this.dataService.setData('New data');
}
}
@Component({
selector: 'app-child',
template: '<h2>{{ data }}</h2>',
})
export class ChildComponent {
data: string;
constructor(private dataService: DataService) {}
ngOnInit() {
this.data = this.dataService.getData();
}
}
Code language: JavaScript (javascript)
Question:
Explain the concept of Angular modules and their role in organizing and structuring an Angular application.
Answer:
Angular modules are a way to organize and structure an Angular application into logical and reusable units. Modules encapsulate related components, directives, services, and other artifacts, providing a boundary for their scope and visibility. They help manage dependencies, define the compilation context, and enable modularization of an application. Angular modules can be used for feature modules that encapsulate specific functionality, as well as for shared modules that provide common functionality and services across the application. Modules are defined using the @NgModule
decorator and can be imported and exported to establish relationships between modules.
More Angular interview resources
For more guides on improving your knowledge of Angular and acing interviews, we have outlined helpful blog posts below:
- Code Challenge: Directives in Angular
- Dev Discussions: How to Reduce your Angular Bundle Size with Google Developers Expert Santosh Yadav
- How to Find & Hire an AngularJS Developer in 2021
1,000 Companies use CoderPad to Screen and Interview Developers
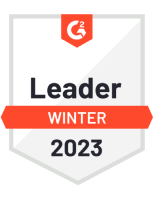
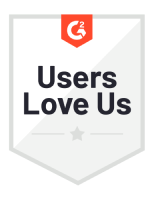
Best interview practices for Angular roles
The Angular interview experience can vary greatly depending on factors such as the particular engineering role and the candidate’s level of expertise. To optimize the efficacy of your Angular interview questions, we recommend adhering to these best practices when interacting with your candidates:
- Formulate technical questions that pertain to real-life situations within your organization – this method is not only more captivating for the candidate but also more effectively showcases the alignment of their skills with your team.
- Cultivate a cooperative atmosphere by encouraging the candidate to ask questions throughout the exercise.
- If you’re evaluating Angular as part of a full-stack role, ensure that candidates are familiar with integrating the front end specificities in the rest of the stack.
Additionally, it is vital to observe standard interview procedures when conducting Angular interviews – tailor the difficulty of interview questions based on the candidate’s development skill level, offer prompt feedback about their position in the hiring process, and set aside ample time for candidates to ask questions about the assessment or the experience of collaborating with you and your team.