3 Spectacular Interview Questions for Junior Backend Developers
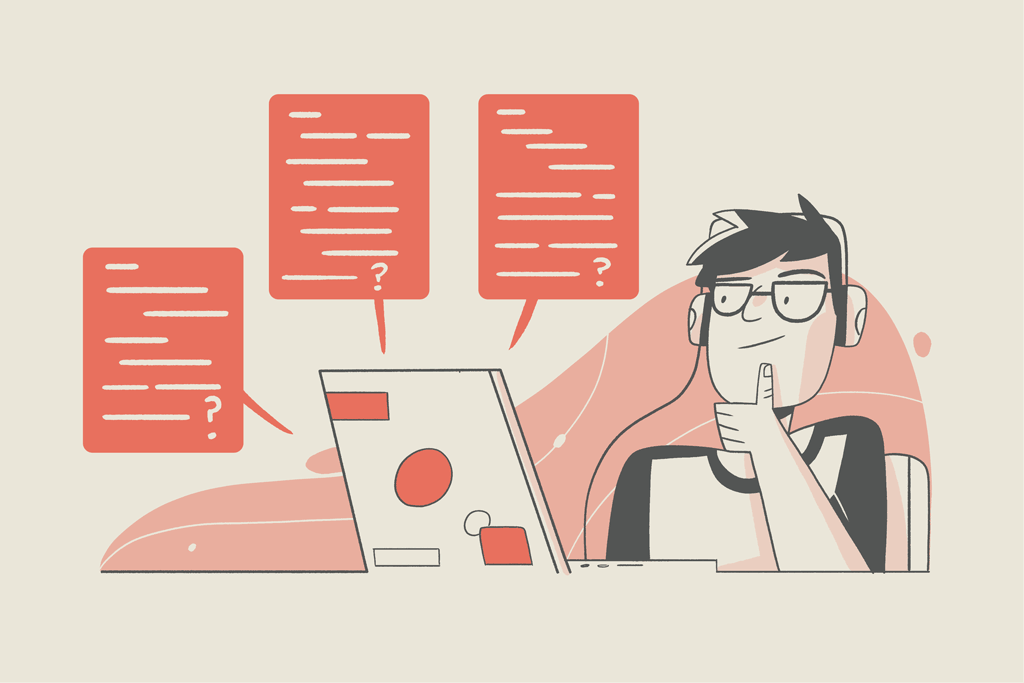
Too often, technical interview sessions become mundane rituals rather than insightful evaluations. While this can take its toll on the morale of developers of all skill levels, it’s particularly damaging to junior developers who may become permanently cynical when it comes to following through with future interview processes.
For junior roles, it’s vital to strike the right balance between interview questions that challenging enough to keep them interested with making sure the right skills are being assessed. Overly complex problems may not be the best litmus test. Instead, gauging their understanding of basic backend principles and assessing their problem-solving skills can offer more insightful results.
In this blog post, we will introduce three handpicked coding interview questions. These questions are specifically tailored for junior backend developers, offering a harmonious blend of challenge and feasibility.
By employing these questions, hiring managers and interviewers can get a clear glimpse into a candidate’s coding acumen. More importantly, it provides an opportunity to evaluate their potential to evolve and adapt in a constantly changing tech landscape.
✅ The questions below are written with Django/Python and Node.js. However, you can easily tailor them to suit your own backend frameworks.
Question 1: Basic CRUD API
Description:
Implement a basic RESTful API for managing a list of products. Each product should have a unique identifier, a name, a description, and a price. Without changing anything in the code, you can already test the API by creating a product with POST and retrieving with a GET.
Requirements:
- Implement the endpoint and route for the update feature.
- Implement the endpoint, route, and corresponding
model.py
function for the delete feature. - Ensure that the API handles potential errors, such as trying to update a non-existent product.
Bonus:
- Implement filtering on the Read endpoint to find products based on partial name matches.
- Implement pagination for the list of products.
Evaluation Criteria:
- Proper use of HTTP methods and status codes.
- Code organization and clarity.
- Error handling.
Question 2: User Authentication System
Description:
Develop a backend system that allows user registration and authentication for an application.
Requirements:
- Implement a registration endpoint where users can sign up using their email and password.
- Implement a login endpoint that authenticates users based on the provided email and password.
- Store user credentials securely in the database (e.g., hash the passwords).
- Upon successful login, generate and return a token (e.g., JWT) to the client.
Bonus:
- Implement a middleware function that validates the token for protected routes.
- Allow users to reset their password by sending a reset link to their email.
Evaluation Criteria:
- Security measures implemented (e.g., password hashing, secure token handling).
- Proper database schema design and interactions.
- Error handling and clear response messages.
Question 3: Rate limiting
Description:
Implement a simple rate limiter middleware for a web server. The rate limiter should prevent any IP from making more than 10 requests in a 1-minute window.
Requirements:
- Create a middleware function that checks the number of requests made by an IP address.
- If the IP has made more than 10 requests in the last minute, return a 429 (Too Many Requests) HTTP status code.
- If the IP is below the limit, allow the request to proceed.
- Include a reset header in the response indicating the time when the IP can make requests again.
Note: You can use basic in-memory storage like arrays or maps. For added complexity, consider integrating a database or caching solution like Redis.
Evaluation Criteria:
- Correctness and Efficiency: Does the solution accurately limit requests per IP within the given timeframe, and does it do so efficiently, even with many IP addresses making requests?
- Code Quality and Extensibility: Is the code well-organized, readable, and can it be easily adapted for different rate limits or time windows?
- Testing and Robustness: Has the solution been tested for various scenarios, and does it handle edge cases and potential high-traffic conditions gracefully?
Some parts of this blog post were written with the assistance of ChatGPT.