Svelte Vs. React: Which Is Better?
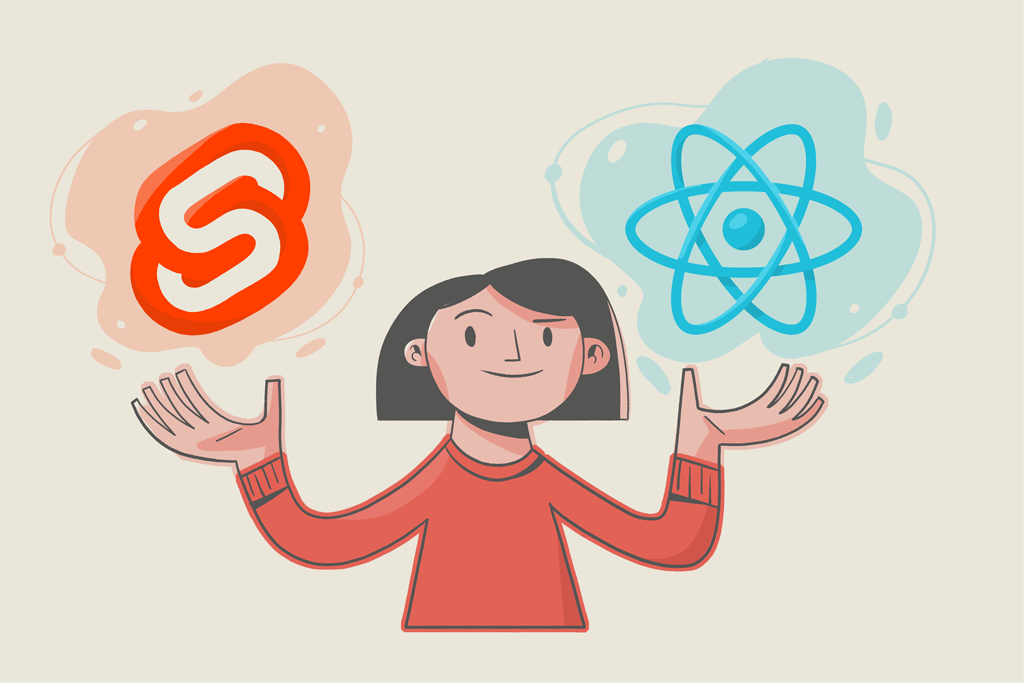
As a front-end developer, you might ask yourself what framework would be the best to use in a project. Should you go with React? Is Svelte a more beginner-friendly choice?
Last year, React was recognized as the most popular front-end framework. However, React had some limitations, so an equally good alternative, Svelte, is also broadly popular in the developer community.
In this post, we’ll compare React and Svelte according to some key factors. By the end of this post, you should be able to make a clear decision as to which library is best for your project.
Primer on React and Svelte
Before getting into the nitty gritty, here’s a high-level overview of both frameworks.
React
React is an open-source library developed and maintained by Facebook and written primarily in JavaScript. It was released in 2013 and has undergone many noticeable changes since then as the front-end ecosystem evolved. It’s used for developing front-end user interfaces for web and mobile apps. Today the React ecosystem itself extends to other frameworks built on top of it, such as Next.js and React Native.
Svelte
Svelte is a free open-source JavaScript compiler. It came out in 2016 and has gained traction rapidly due to its unique approach toward building and shipping front-end web applications. The Svelte source code itself was written in Typescript, a more robust version of JavaScript.
React and Svelte: similarities
Both React and Svelte are used for building web applications. In terms of overall architecture, both are component-based, meaning you break your web application into smaller components, then reuse those components for scalability. For dynamically updating the DOM and sharing data across these components, you create special mutable variables.
React vs Svelte
We’ll compare the two frameworks according to the following criteria:
- Syntax and learning curve
- Developer experience
- Popularity, community support, and documentation
- Ecosystem and tools/libraries
- Performance
Comparing Svelte and React according to the above should help you decide which framework is best suited for you and your project.
Syntax and learning curve: Is Svelte easier to learn than React?
Whether a framework is easy to learn or not depends on its general syntax, its fundamentals, and raw HTML, CSS, and JavaScript code.
Svelte
Any Svelte application has three parts: an HTML template, a JavaScript section, and a CSS section. Svelte imposes this pattern on developers but also provides a shorthand way to write code. However, this pattern is similar to other frameworks like Vue.js, Angular, and (most importantly) vanilla JavaScript applications. Thus, it’s easy to start writing some Svelte code. Here’s an example:
<script>
let name='svelte'
</script>
<h1>You're learning {name}!</h1>
<style>
h1{
text-decoration:underline;
}
</style>
Code language: HTML, XML (xml)
In the script section, we create a JavaScript variable and output it to the HTML template. In the end, we have a style section that will govern the CSS for this page.
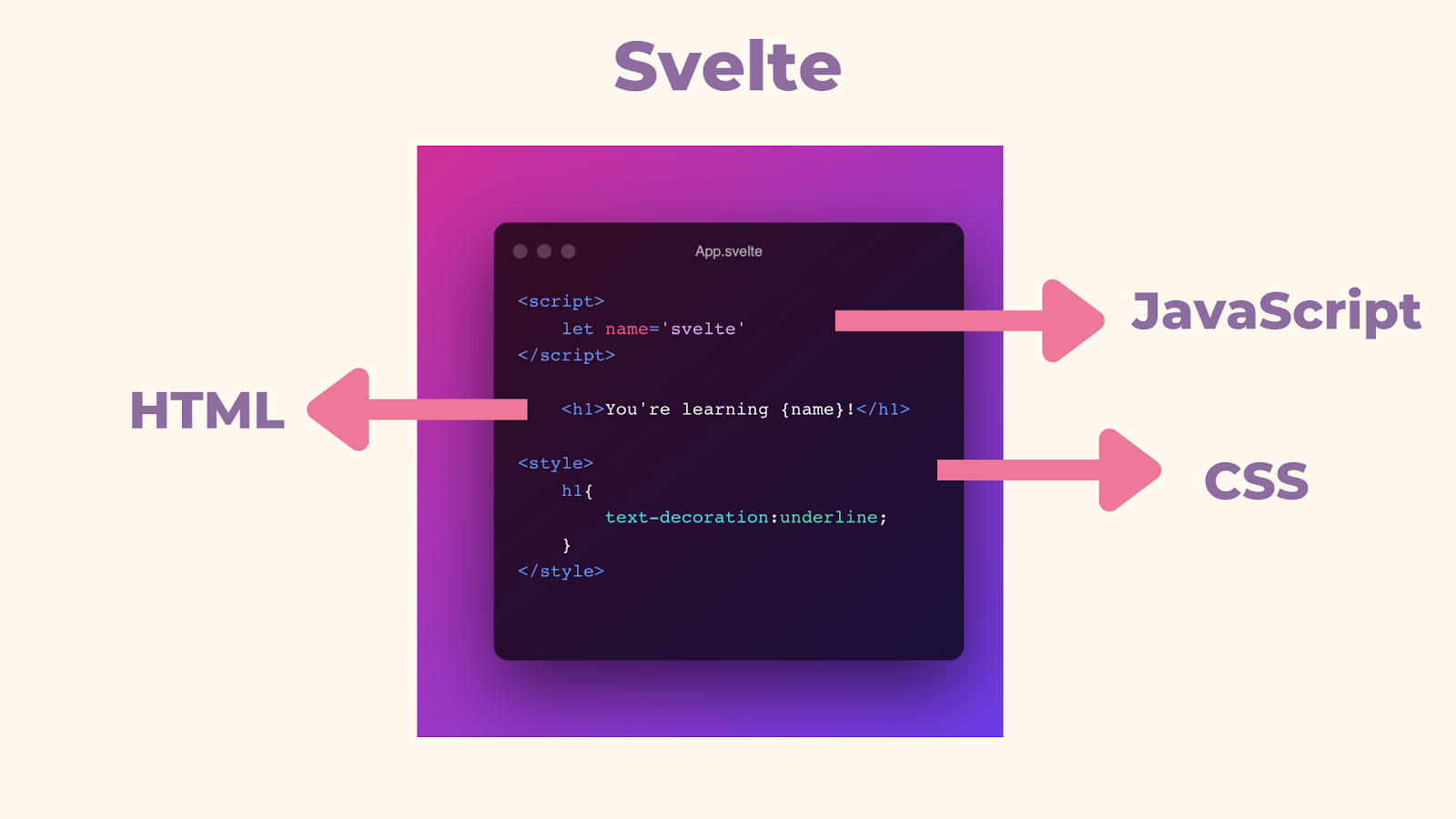
.svelte
file.All Svelte files have a .svelte
extension, which should be self-explanatory.
React
In React, you have to write your code in a special JavaScript extension language called JSX. Therefore, all React files have a .jsx
extension. The HTML template is returned by a JavaScript function, and all its logic resides inside it. Here’s the same code example as before but in React syntax:
import React from 'react';
import './style.css';
export default function App() {
const name = 'react';
return (
<div>
<h1>You're learning {name}!</h1>
</div>
);
}
Code language: JavaScript (javascript)
Notice that we import the styles.css
file at the top. This is where you can declare some CSS for your React templates:
h1 {
text-decoration:underline;
}
Code language: CSS (css)
You can also do inline styling using JSX, which is more intuitive in Svelte as it’s closer to the native HTML inline styling. Here’s the general way you structure your React code:
Verdict
React has a steeper learning curve than Svelte due to JSX. Svelte is easier to work with, especially for beginners due to its relatedness with vanilla JavaScript and other frameworks.
Developer experience
Developer experience describes how much you enjoy working, building, and maintaining applications in that framework over the long term. If a framework doesn’t make building web apps simple for you, it’s probably not the best choice.
Both React and Svelte come with their own compilers that you can use for hot reloading and building the application bundle. But can both do the same work using the same lines of code? Does one offer extra help in writing code and boilerplate? You’re about to find out.
React
Here is some simple code in React that binds the value of an input field to a variable and prints it:
import React,{ useState } from 'react';
export default function App() {
const [name,setName]=useState('')
const handleChange=(e)=>setName(e.target.value)
return (
<>
<input type="text" value={name} onChange={handleChange} />
<h1>Name: {name}</h1>
</>
);
}
Code language: JavaScript (javascript)
The App
component returns an input field and a heading. We then have a state variable called name
that binds to the input field using the value
property. In order to update this state variable on the change of an input field, we fire the handleChange
function and update the name
state variable with the value of the input field. This is the result:
Svelte
Now let’s write some code in Svelte that does the same thing as above:
<script>
let name='';
</script>
<input type="text" bind:value={name} />
<h1>Name: {name} </h1>
Code language: HTML, XML (xml)
We have a name
variable that we directly bind to the input field and then output it under a heading. Here’s what that looks like:
Verdict
The same behavior takes five lines of code in Svelte and roughly ten lines in React. React has more boilerplate code than Svelte that includes creating a function and putting a return statement to output the HTML. Also, notice how in the above code Svelte required absolutely no import statements, unlike React.
Clearly Svelte offers a more concise and simple way to write code with less boilerplate, offering a better developer experience than React.
React vs Svelte: popularity, community support, and documentation
The fate of any open-source project is decided by its community. You’ll want to choose a framework with ample resources for learning, blogs and tutorials that can guide you, and solid documentation with great online community support.
React
The official React library has 198,000 stars and 41,000 forks. If you search for questions and answers tagged with React on Stack Overflow, you’ll get more than 700,000 results. It’s also one of the most popular web frameworks according to the Stack Overflow 2022 developer survey:
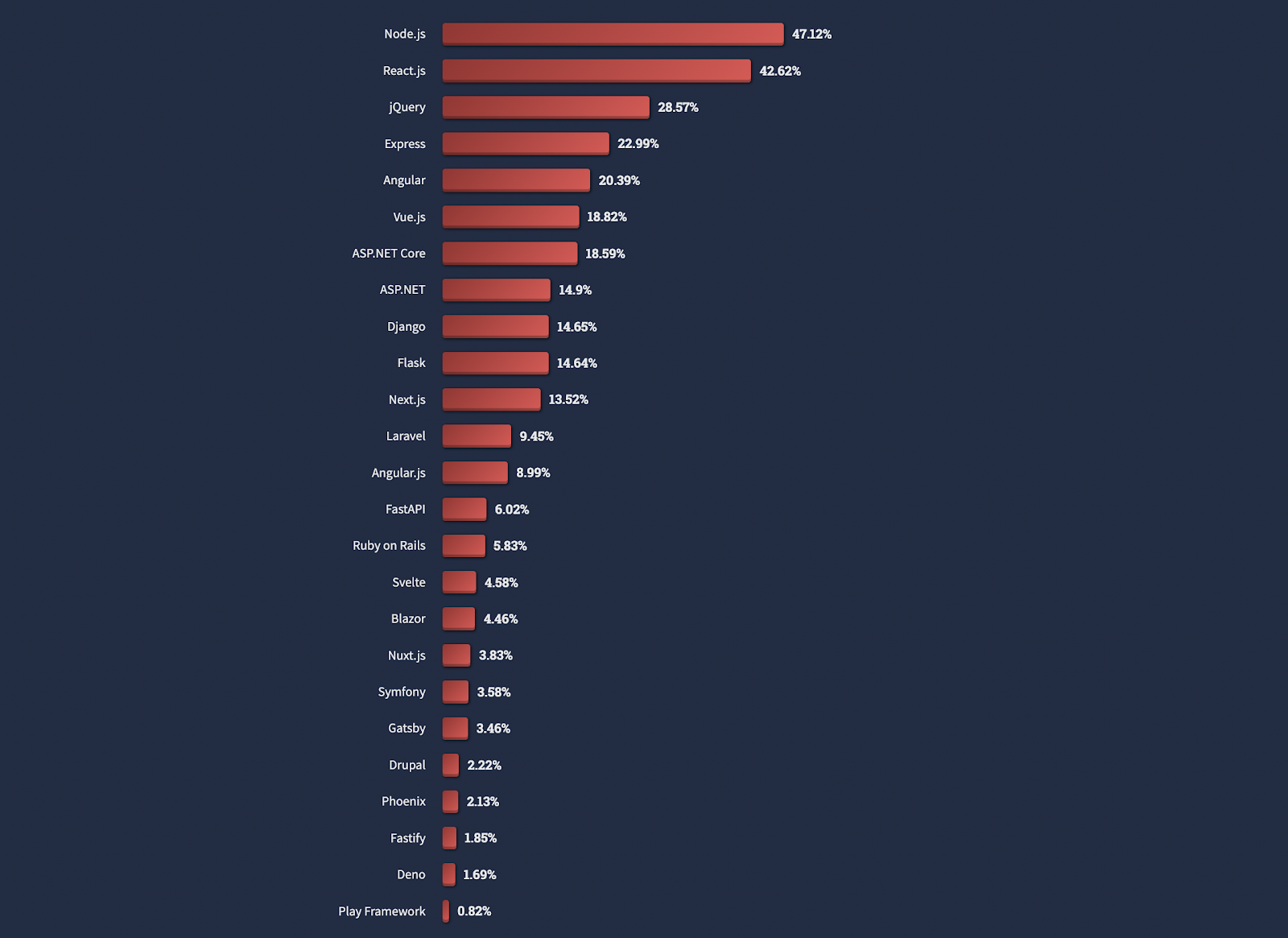
Further, it was also the most wanted web framework in the Stack Overflow 2022 developer survey:
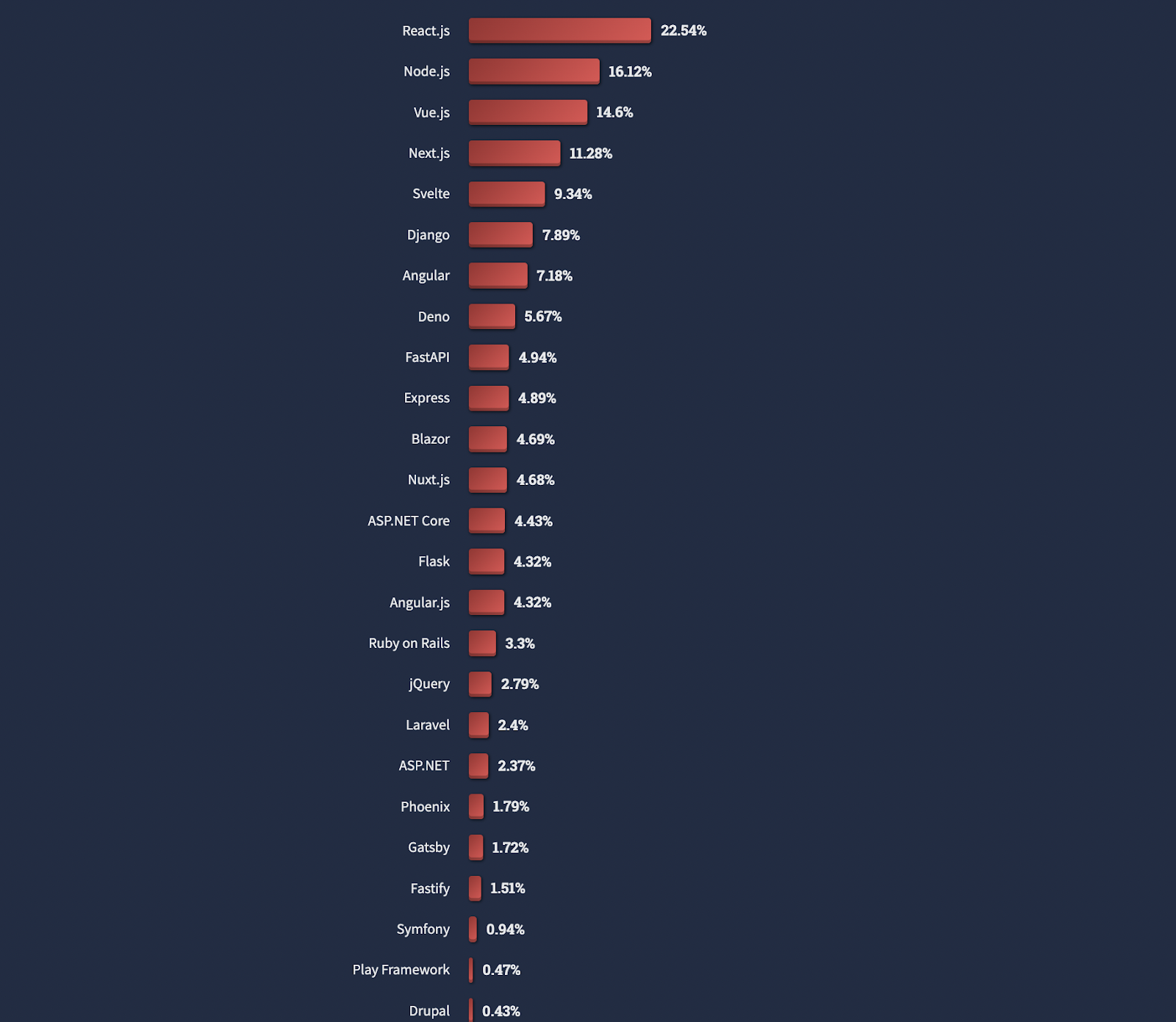
The community support for React is as good as can be. You’ll find third-party packages for almost everything, a wide range of blogs and tutorials, and how-to guides to help you out. React’s official documentation is fairly easy, but it can be a challenge if you’re a beginner. A built-in editor where you can try out tutorials and example snippets is a must, but it’s missing from the official docs website.
Svelte
The official Svelte GitHub repository has 63,200 stars but only 3,100 forks. Looks like not a lot of people have tried to fork and play around with it. Stack Overflow has roughly 9,500 results for questions and answers tagged with Svelte. It has a small yet evolving ecosystem, but the most essential packages and dependencies are out there and are well-maintained.
Interestingly, among other web frameworks, it had the best love-to-hate ratio under the “Loved vs Dreaded” category of frameworks in theStack Overflow 2022 developer survey:
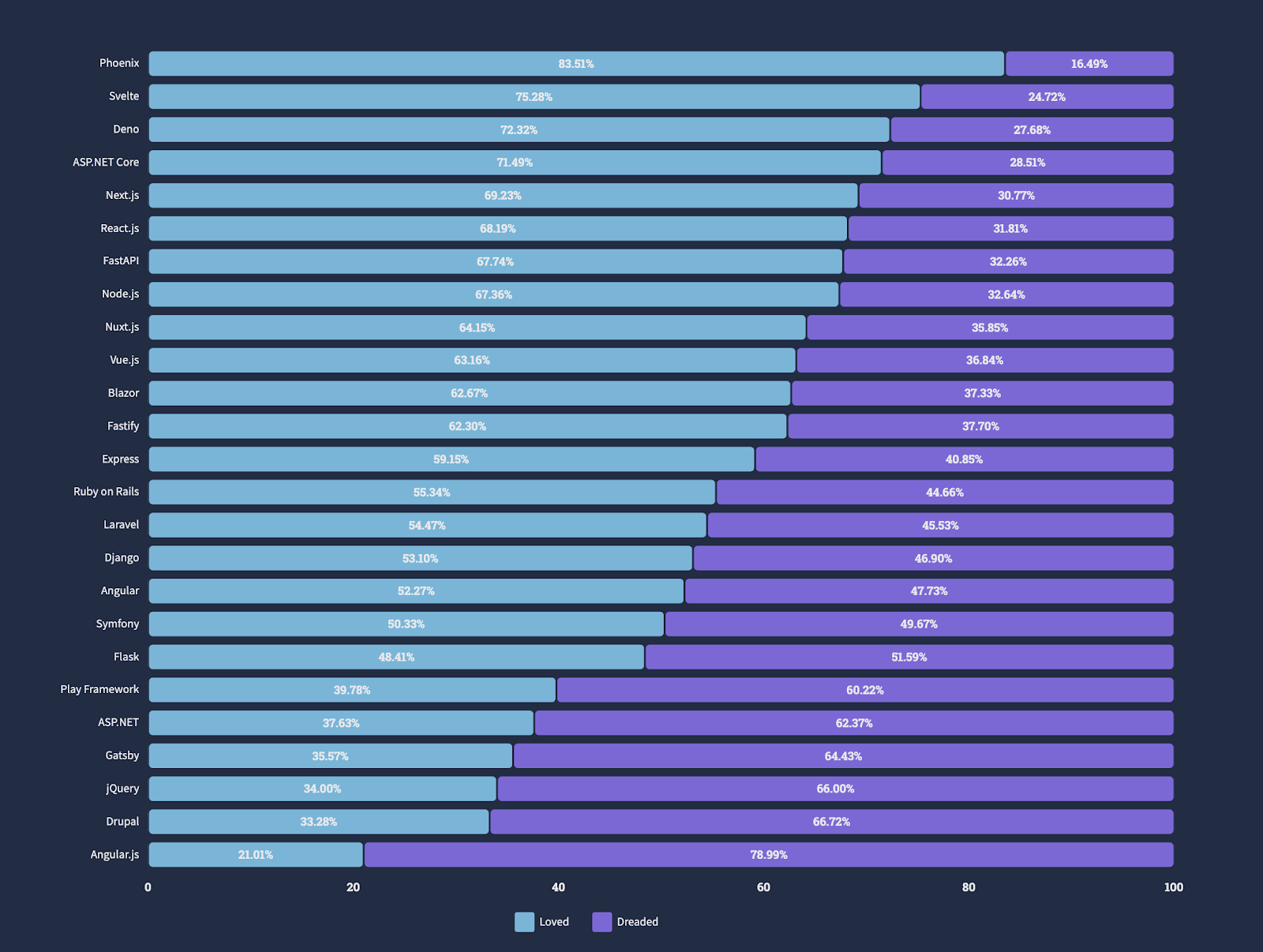
Svelte’s documentation is beginner friendly, and the built-in Svelte editor makes it even easier to understand tutorials and examples.
Verdict
Clearly, React has more powerful community support and is much more popular than Svelte, which more or less compensates for the lack of better documentation.
Tools and libraries
Neither React nor Svelte are full-blown frameworks. Nothing comes with everything you need out of the box. So, let’s look at how their tooling and library ecosystems compare.
React
React relies heavily on the open-source community for additional functionality and capabilities. Since it has robust community support, there are popular tools and libraries like React Router for routing, Redux, built-in Context API for state management, UI libraries like Material UI and React Bootstrap, etc. Almost all popular libraries have a React-specific abstraction library available.
For more specific use cases like server-side rendering or static site generation, there are frameworks like Next.js and Gatsby built on top of React.
Svelte
Svelte also has similar tools and libraries for specific use cases. For instance, you can use SvelteNavigator for routing. Svelte has built-in state management that’s fairly easy to use out of the box. For server-side rendering and hot reloading, Svelte has SvelteKit, which is often a choice for developers planning a long-term project.
Verdict
Even though Svelte has enough tooling and libraries, it can’t compete with the extensive open-source ecosystem React offers.
Performance
What good is a framework if it doesn’t help you to create a fast and high-performant web application? Let’s look at how React compares to Svelte in terms of overall performance.
React
React itself ships a 42.2 KB bundle, and there are additional libraries and third-party packages you can use in your application. Internally, React uses something called a virtual DOM to manipulate the DOM in real time. The virtual DOM itself is a copy of the real HTML DOM, which only updates the required HTML nodes in a render cycle.
Svelte
Unlike React, Svelte is merely a JavaScript compiler. This means it doesn’t ship any additional bundles of code of its own like React does. Svelte converts your app into a vanilla JavaScript application for production. So, you’re only shipping the code you write without any additional code from a library.
Svelte uses direct DOM manipulation. It looks through the variables in your code that have changed and updates the DOM entities that rely on those variables.
Verdict
Svelte ships a lighter and faster overall bundle of the final application. However, React can be faster and perform DOM manipulations more effectively. Both are winners in this category provided you know the scope and scale of the application you’re comparing them against.
React vs Svelte: which is better?
It’s time for the final verdict. But first, let’s summarize:
Are you a beginner who wants to build a small project? Maybe a developer portfolio, a blogging website, or a personal project? Go with Svelte. If you wish to develop something more serious but want to do it quicker, like an MVP for a product, go with Svelte. You’ll be able to develop a fast application in little time without much overengineering or complicated boilerplate.
However, if you want to develop across multiple platforms and build a highly flexible and scalable application targeting millions of users, go with React. If you’re developing a complex web application, React should be your preferred choice. Its popularity, strong ecosystem, and extensive set of tools and libraries will help you build apps that cater to complex use cases.
This post was written by Siddhant Varma. Siddhant is a full stack JavaScript developer with expertise in frontend engineering. He’s worked with scaling multiple startups in India and has experience building products in the Ed-Tech and healthcare industries. Siddhant has a passion for teaching and a knack for writing. He’s also taught programming to many graduates, helping them become better future developers.