How To Use DataProvider In TestNG With Examples
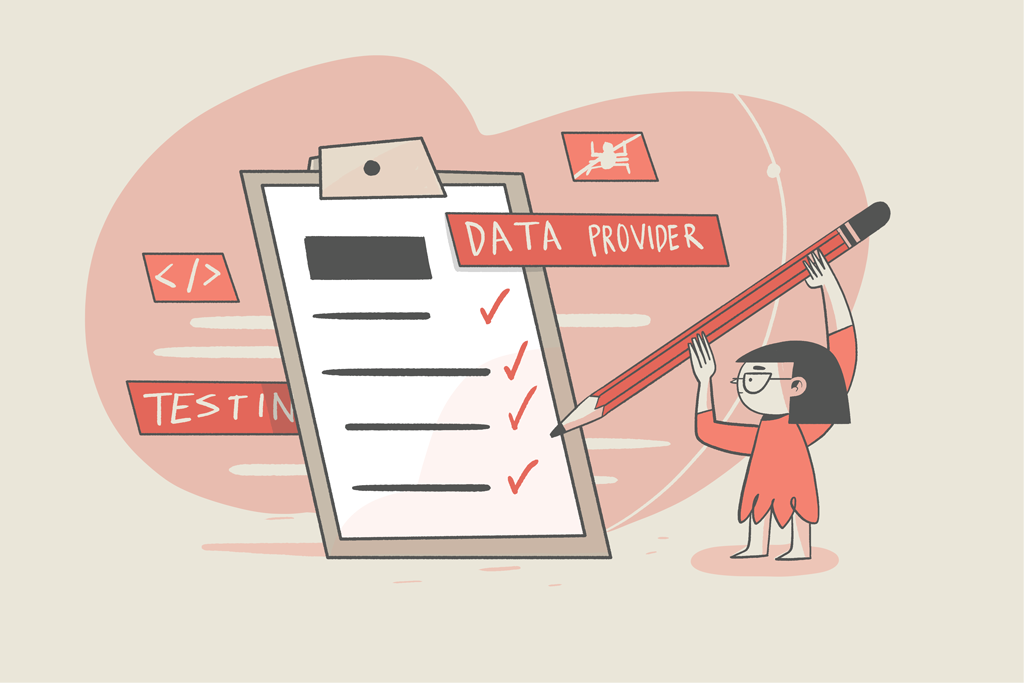
Testing is an essential part of software development, especially in the agile world. By testing in the early stages of development, developers can ensure that their code is of high quality and meets customer expectations.
In the agile world, it’s especially important to test early and often, as changes are made frequently and new features are added constantly. By testing regularly, developers can catch bugs early and prevent them from becoming major issues later on.
There are many tools and techniques available for testing. In this post, we’ll discuss how to use DataProvider with TestNG.
Prerequisites
Before we get started with the guide, it’s important that you fulfill all the prerequisites. This will ensure that you have all the necessary tools and knowledge to complete the guide successfully. You’ll need
- an understanding of the Java programming language,
- Java IDE such as NetBeans (or online IDE like Coderpad), and
- a basic understanding of testing frameworks like JUnit or NUnit.
Once you’ve fulfilled all of the prerequisites, you’re ready to get started with the guide.
What Is TestNG?
TestNG is a testing framework for the Java programming language. The name “TestNG” is a play on the word “testing.” The initials “NG” stand for “next generation.”
The goal of TestNG is to help developers perform a wider range of test cases—unit, functional, end-to-end, integration, etc.—with more ease and flexibility.
TestNG is an open-source project hosted on GitHub and is released under the Apache software license. It was developed by Cédric Beust and was first released in July 2004.
TestNG enables a user to create tests that are more maintainable and easier to understand. It also provides support for a variety of features, such as data-driven testing, parameterized testing, and multi-threaded testing.
Understanding TestNG With an Example
Let’s check out an example of how to use TestNG to write a test case using the following code snippet:
@Test
public void TestLogin(){
WebDriverManager.chromedriver().setup();
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
String username = "username", password = "*****";
driver.get("<https://demosite.executeautomation.com/>");
driver.findElement(By.name("UserName")).sendKeys(username);
driver.findElement(By.name("Password")).sendKeys(password);
driver.findElement(By.name("Login")).submit();
Assert.assertEquals("User Form", driver.findElement(By.tagName("h2")).getText());
driver.quit();
}
Code language: Java (java)
In the above code snippet, we’re going to the URL https://demosite.executeautomation.com, which has a login page that accepts any username and password.
There, we’ll assert user form text from the next page after a successful login.
The goal of the above code is to understand how to use the @Test
annotation from TestNG and write an automation test case using Selenium in Java.
How to Perform Parameterization Using TestNG
To perform parameterization, we use the @Parameters({"parameter1", "parameter2", ..})
annotation.
Test methods will read these parameters from XML files, as shown below:
<?xml version="1.0" encoding="UTF-8"?>
<suite name="TestSuite">
<parameter name="username" value="username" />
<parameter name="password" value="*****" />
<test name="TestLogin">
<classes>
<class name="DataProvider.TestNg" />
</classes>
</test>
</suite>
Code language: Java (java)
Here, the parameter’s name from the XML file should match the name we mentioned in the annotation in the test method above.
@Test
@Parameters({"username", "password"})
public void TestLogin(String username, String password){
WebDriverManager.chromedriver().setup();
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
driver.get("<https://demosite.executeautomation.com/>");
driver.findElement(By.name("UserName")).sendKeys(username);
driver.findElement(By.name("Password")).sendKeys(password);
driver.findElement(By.name("Login")).submit();
Assert.assertEquals("User Form", driver.findElement(By.tagName("h2")).getText());
driver.quit();
}
Code language: Java (java)
The use of parameterization in TestNG gives you the power to perform data-driven testing more efficiently.
What Is DataProvider in Java?
DataProvider is like a container that passes the data to our test methods so that our single test method can execute itself with multiple data sets.
To make any method act as a DataProvider, we need to use this annotation— @DataProvider
—which will return a 2D array of an object where columns are the arguments needed in one test execution and rows are the number of data passed in each execution.
@Test(dataProvider="LoginDataProvider")
public void TestLogin(String username, String password){
WebDriverManager.chromedriver().setup();
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
driver.get("<https://demosite.executeautomation.com/>");
driver.findElement(By.name("UserName")).sendKeys(username);
driver.findElement(By.name("Password")).sendKeys(password);
driver.findElement(By.name("Login")).submit();
Assert.assertEquals("User Form", driver.findElement(By.tagName("h2")).getText());
driver.quit();
}
@DataProvider(name = "LoginDataProvider")
public Object[][] LoginData(){
return new Object[][]
{
{ "abc", "***" },
{ "xyz", "***" },
{ "mno", "***" }
};
}
Code language: Java (java)
Now, when we run the test above, the same test will run the method TestLogin()
three times as LoginData returns three rows of data.
If you don’t specify any name while declaring the DataProvider method and simply use @DataProvider
while calling it in the test, we need to use the method’s name as a data provider name. In the above case, it’ll be @Test(dataProvider="LoginData")
.
Also, if DataProvider is created in a different class, then we need to include the dataProviderClass argument:
@Test(dataProvider="<name>",dataProviderClass=<dataProvider_ClassFileName>.class)
Code language: Java (java)
Here are a few samples: DataSets.java:
public class DataSets{
@DataProvider(name = "LoginDataProvider")
public Object[][] LoginData(){
return new Object[][] {
{ "abc", "***" },
{ "xyz", "***" },
{ "mno", "***" }
};
}
}
Code language: Java (java)
TestAuthorization.java
public class TestAuthorization{
@Test(dataProvider="LoginDataProvider", dataProviderClass="DataSets.class")
public void TestLogin(String username, String password){
WebDriverManager.chromedriver().setup();
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
driver.get("<https://demosite.executeautomation.com/>");
driver.findElement(By.name("UserName")).sendKeys(username);
driver.findElement(By.name("Password")).sendKeys(password);
driver.findElement(By.name("Login")).submit();
Assert.assertEquals("User Form", driver.findElement(By.tagName("h2")).getText());
driver.quit();
}
}
Code language: Java (java)
Why Use DataProvider in TestNG?
If we have to test the same test case for multiple data (username and password) without using DataProvider, it’ll look as shown below.
@Test
public void TestLogin(){
WebDriverManager.chromedriver().setup();
driver = new ChromeDriver();
driver.manage().window().maximize();
Login("abc", "***");
Login("mno", "***");
Login("xyz", "***");
Login("def", "***");
Login("dod", "***");
driver.quit();
}
public void Login(String username, String password){
driver.get("<https://demosite.executeautomation.com/>");
driver.findElement(By.name("UserName")).sendKeys(username);
driver.findElement(By.name("Password")).sendKeys(password);
driver.findElement(By.name("Login")).submit();
Assert.assertEquals("User Form", driver.findElement(By.tagName("h2")).getText());
}
Code language: Java (java)
Conclusion
We hope you enjoyed reading about DataProvider in testing with examples.
TestNG is a testing framework that you can use with any Java project. It’s used in Agile methodology to test any application’s functionality.
DataProvider is a feature of the TestNG library that allows a developer to run the same suite of test cases with different data sets. It helps the developer to test the application with different values. You can use this to test the business logic of any application.
We hope that you found this post informative and helpful.
This post was written by Keshav Malik. Keshav is a full-time developer who loves to build and break stuff. He is constantly on the lookout for new and interesting technologies and enjoys working with a diverse set of technologies in his spare time. He +loves music and plays badminton whenever the opportunity presents itself.