Tcl Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Tcl developers for your organization.
Tcl
Tool Command Language — Tcl — is a general purpose scripting tool commonly used for automation, creating cross-platform graphical user interfaces (GUIs), and embedded systems scripts.
Tcl was developed in 1988 by John Ousterhout at the University of California, Berkeley. He designed the language as a simple, embeddable scripting language for applications that required extensibility and customization.
https://www.tcl.tk/
To assess the proficiency of developers in Tcl during programming interviews, we offer a collection of practical coding tasks and interview queries below. Moreover, we’ve devised a serie of recommended approaches to guarantee that your interview questions effectively gauge the candidates’ Tcl abilities.
Table of Contents
Tcl example question
Help us design a parking lot app
Hey candidate! Welcome to your interview. Boilerplate is provided. Feel free to change the code as you see fit. To run the code at any time, please hit the run button located in the top left corner.
Goals: Design a parking lot using object-oriented principles
Here are a few methods that you should be able to run:
- Tell us how many spots are remaining
- Tell us how many total spots are in the parking lot
- Tell us when the parking lot is full
- Tell us when the parking lot is empty
- Tell us when certain spots are full e.g. when all motorcycle spots are taken
- Tell us how many spots vans are taking up
Assumptions:
- The parking lot can hold motorcycles, cars and vans
- The parking lot has motorcycle spots, car spots and large spots
- A motorcycle can park in any spot
- A car can park in a single compact spot, or a regular spot
- A van can park, but it will take up 3 regular spots
- These are just a few assumptions. Feel free to ask your interviewer about more assumptions as needed
Tcl skills to assess
Jobs using Tcl
Junior Tcl interview questions
Question:
Explain what Tcl (Tool Command Language) is and list some real-world applications where Tcl is commonly used.
Answer:
Tcl (Tool Command Language) is a dynamic scripting language commonly used for automation, rapid prototyping, and integration tasks. It provides a simple syntax and powerful built-in commands that make it easy to create and manipulate data structures, interact with files, and control applications.
Real-world applications where Tcl is commonly used include:
- Network device configuration: Tcl is widely used to automate the configuration and management of network devices, such as routers and switches.
- GUI development: Tcl’s Tk extension provides a simple and effective way to create graphical user interfaces (GUIs) for applications.
- Embedded systems: Tcl’s small footprint and ease of integration make it suitable for use in embedded systems and IoT devices.
- Electronic Design Automation (EDA): Tcl is used in EDA tools for tasks like simulation, synthesis, and verification.
- Web development: Tcl can be used in web server applications, especially when integrated with web server technologies.
- Test automation: Tcl is often used for test automation, allowing for the creation of test scripts to automate software testing.
- System administration: Tcl is useful for automating system administration tasks and managing resources in Unix-like environments.
Question:
The following Tcl code is intended to calculate the factorial of a given number. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
proc factorial {n} {
set result 1
while {$n > 0} {
set result $result * $n
incr n -1
}
return $result
}
Code language: PHP (php)
Answer:
The logical error in the code is that the condition $n > 0
in the while
loop should be changed to $n >= 1
to correctly calculate the factorial. The correct code is as follows:
proc factorial {n} {
set result 1
while {$n >= 1} {
set result $result * $n
incr n -1
}
return $result
}
Code language: PHP (php)
In this corrected code, the while
loop continues until $n
reaches 0, ensuring the correct calculation of the factorial.
Question:
Explain the concept of Tcl namespaces and why they are useful.
Answer:
In Tcl, namespaces are a way to organize commands and variables into separate contexts or scopes. They provide a mechanism for preventing name collisions and making code modular and reusable.
Tcl namespaces are useful for several reasons:
- Encapsulation: Namespaces allow you to encapsulate commands and variables within a distinct namespace, reducing the risk of conflicts with other code.
- Code organization: By placing related commands and variables within a namespace, the code becomes more organized and easier to maintain.
- Modularity: Namespaces enable modularity, allowing you to package functionality into namespaces and reuse them across different parts of your application.
- Better code sharing: Namespaces facilitate code sharing in a controlled manner, where only specific commands and variables can be accessed from outside the namespace.
- Library management: When using packages and extensions, namespaces help manage the scope and visibility of commands and variables from different libraries.
Question:
The following Tcl code is intended to check if a given list contains any duplicate elements. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
proc has_duplicates {lst} {
set elements [array names lst]
foreach element $elements {
if {$lst($element) > 1} {
return 1
}
}
return 0
}
Code language: PHP (php)
Answer:
The logical error in the code is that it checks the occurrence count of elements in the associative array, which may not represent duplicate elements correctly. The correct code is as follows:
proc has_duplicates {lst} {
set unique_elements [lsort -unique $lst]
if {[llength $unique_elements] != [llength $lst]} {
return 1
}
return 0
}
Code language: PHP (php)
In this corrected code, the lsort -unique
command is used to get a list of unique elements from the input list $lst
. The code then compares the lengths of the unique list and the original list to determine if there are any duplicates.
Question:
Explain the concept of event-driven programming in Tcl and its significance in GUI applications.
Answer:
Event-driven programming in Tcl is a programming paradigm where the flow of the program is determined by events, such as user interactions or external signals, rather than following a sequential execution flow. Tcl’s event-driven nature is particularly significant in GUI applications.
In GUI applications, the user interacts with the graphical elements, such as buttons, menus, and mouse clicks. These interactions generate events that are captured by the Tcl event loop. The event loop continuously waits for events to occur, and when an event occurs, it triggers the corresponding event handler or callback procedure.
Event-driven programming in Tcl GUI applications is crucial because it:
- Enables responsiveness: The event loop allows the GUI to respond immediately to user interactions, providing a smooth and interactive user experience.
- Separates concerns: Event-driven programming allows GUI logic to be separated from other application logic, making the code more modular and maintainable.
- Supports non-blocking behavior: Tcl’s event loop can handle multiple events simultaneously, enabling non-blocking behavior even while waiting for user input.
- Facilitates asynchronous operations: Asynchronous operations, such as network requests or file I/O, can be efficiently handled by the event loop without blocking the GUI.
Question:
The following Tcl code is intended to reverse a given list. However, it contains a syntax error and doesn’t run correctly. Identify the error and fix the code.
proc reverse_list {lst} {
set reversed {}
for {set i [llength $lst]} {$i >= 0} {incr i -1} {
lappend reversed [lindex $lst $i]
}
return $reversed
}
Code language: PHP (php)
Answer:
The syntax error in the code is that the loop variable $i
starts from the length of the list $lst
, which is an out-of-bounds index. The correct code is as follows:
proc reverse_list {lst} {
set reversed {}
for {set i [expr {[llength $lst] - 1}]} {$i >= 0} {incr i -1} {
lappend reversed [lindex $lst $i]
}
return $reversed
}
Code language: PHP (php)
In this corrected code, the loop variable $i
starts from the index [llength $lst] - 1
, ensuring the correct reversal of the list.
Question:
Explain the concept of error handling in Tcl and describe the mechanisms available for handling errors.
Answer:
In Tcl, error handling refers to the process of gracefully handling errors and exceptions that may occur during the execution of a script. Tcl provides several mechanisms for error handling:
- Return codes: Many Tcl commands return error codes to indicate whether the command executed successfully or encountered an error. The
if
andswitch
statements can be used to check for error codes and handle errors accordingly. - catch command: The
catch
command is used to capture and handle errors that occur within a script
. It allows you to execute a block of code and intercept any errors that may occur during its execution. The result of the catch
command is a boolean value indicating whether an error occurred (1 for error, 0 for success) and the error message if applicable.
- error command: The
error
command is used to explicitly generate an error and terminate the script’s execution. It allows you to specify a custom error message and an error code, which can be caught and handled using thecatch
command. - trap command: The
trap
command is used to set up error handlers that will be invoked when certain types of errors occur. It allows you to define custom procedures to handle specific error conditions.
By combining these error handling mechanisms, Tcl scripts can gracefully handle errors, recover from exceptional conditions, and provide meaningful feedback to users when something unexpected happens.
Question:
The following Tcl code is intended to find the maximum value in a list. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
proc find_max {lst} {
set max 0
foreach element $lst {
if {$element > $max} {
set max $element
}
}
return $max
}
Code language: PHP (php)
Answer:
The logical error in the code is that it initializes the variable $max
to 0, which may not be the correct initial value, especially if the list contains negative values. The correct code is as follows:
proc find_max {lst} {
set max [lindex $lst 0]
foreach element $lst {
if {$element > $max} {
set max $element
}
}
return $max
}
Code language: PHP (php)
In this corrected code, the variable $max
is initialized to the first element of the list using [lindex $lst 0]
, ensuring that the initial value is set correctly.
Question:
Explain the concept of Tcl packages and their role in code organization and reusability.
Answer:
In Tcl, a package is a collection of commands, procedures, variables, and namespaces that are organized together to provide specific functionality. Tcl packages play a crucial role in code organization, modularity, and reusability.
The primary features and benefits of Tcl packages are:
- Namespace encapsulation: Packages use namespaces to encapsulate their contents, preventing name clashes and making it easier to reuse the package in different applications.
- Exported commands: A package can choose to export only specific commands or procedures to the global namespace, hiding implementation details and simplifying the interface.
- Versioning: Packages can have version numbers, allowing users to specify the desired version when loading the package. This ensures compatibility and avoids unexpected behavior due to changes in the package.
- Organized codebase: Packages help keep related code together, promoting a cleaner and more organized codebase.
- Code reuse: Tcl packages allow developers to create reusable components that can be shared across projects, reducing code duplication and development time.
- Loadable extensions: Some packages are implemented as loadable extensions, providing low-level functionality that can be used efficiently from Tcl scripts.
By using Tcl packages, developers can manage large projects more effectively, promote code reuse, and create well-structured, modular, and maintainable applications.
Question:
The following Tcl code is intended to generate a Fibonacci sequence up to a given number of terms. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
proc fibonacci {n} {
set sequence {0 1}
for {set i 2} {$i < $n} {incr i} {
set next_term [expr {[lindex $sequence end] + [lindex $sequence end-1]}]
lappend sequence $next_term
}
return $sequence
}
Code language: JavaScript (javascript)
Answer:
The logical error in the code is that the loop condition should be $i <= $n
instead of $i < $n
to generate the correct number of terms in the Fibonacci sequence. The correct code is as follows:
proc fibonacci {n} {
set sequence {0 1}
for {set i 2} {$i <= $n} {incr i} {
set next_term [expr {[lindex $sequence end] + [lindex $sequence end-1]}]
lappend sequence $next_term
}
return $sequence
}
Code language: JavaScript (javascript)
In this corrected code, the loop condition is changed to $i <= $n
, ensuring that the correct number of terms (n terms) is generated in the Fibonacci sequence.
Intermediate Tcl interview questions
Question:
Explain what Tcl (Tool Command Language) is and highlight its key features.
Answer:
Tcl (Tool Command Language) is a scripting language that is commonly used for various purposes, including application scripting, automation, rapid prototyping, and embedded systems. It was initially developed as a glue language to interact with other software components and tools. Tcl’s simplicity, extensibility, and ease of embedding make it a powerful choice for a wide range of applications.
Key Features of Tcl:
- Simplified Syntax: Tcl has a simple and straightforward syntax, making it easy to learn and use. It uses commands and arguments, where each command is a string representing an action.
- Interpreted Language: Tcl is an interpreted language, which means that the code is executed line-by-line directly by the Tcl interpreter, without the need for compilation.
- Dynamic Typing: Tcl is dynamically typed, allowing variables to change their data type during runtime.
- Extensibility: Tcl is designed to be easily extended through C extensions or other Tcl packages, making it possible to add new commands and features to the language.
- Embeddable: Tcl can be embedded within applications, allowing developers to provide scripting capabilities to their software.
- Cross-Platform: Tcl is platform-independent and runs on various operating systems, making it suitable for cross-platform development.
- Rich Built-in Commands: Tcl comes with a wide range of built-in commands for file I/O, string manipulation, control flow, and more.
- Event-Driven: Tcl supports event-driven programming, enabling the creation of GUI applications and event-driven scripts.
- Tcl Packages: Tcl packages provide additional functionalities and libraries that can be loaded on-demand, enhancing the language’s capabilities.
- Active Community: Tcl has an active community of developers who contribute to the language’s growth, libraries, and extensions.
Question:
Write a Tcl code snippet to read data from a file and store it in a list variable.
Answer:
set filename "data.txt"
set file_handle [open $filename r]
set data_list [split [read $file_handle] "n"]
close $file_handle
Code language: PHP (php)
In this code snippet, we specify the file name using the variable filename
. We then open the file in read mode using open
and store the file handle in the variable file_handle
. The read
command reads the contents of the file, and split
is used to divide the data into a list based on newlines (“n”). Finally, we close the file using close
.
Question:
Explain the concept of procedures in Tcl and provide an example of how to define and call a procedure.
Answer:
In Tcl, procedures are blocks of code that are defined and given a name to be executed as a single unit. They allow developers to group functionality into reusable modules, promoting code organization and maintainability.
Example:
proc greet_user {name} {
puts "Hello, $name!"
}
# Call the procedure
greet_user "John"
Code language: PHP (php)
In this example, we define a procedure named greet_user
that takes one argument name
. Inside the procedure, we use puts
to print a greeting message with the provided name
argument. To call the procedure, we simply use its name followed by the argument in curly braces (“John” in this case).
Question:
How can you handle errors or exceptions in Tcl? Provide an example of using the catch
command.
Answer:
In Tcl, errors or exceptions can be handled using the catch
command. The catch
command allows you to execute a script and capture any errors that occur during its execution. It returns the result of the script and a flag indicating whether an error occurred.
Example:
set data "123"
catch {
set parsed_data [expr {$data * 2}]
} result
if {[catch $result]} {
puts "An error occurred: $result"
} else {
puts "Parsed data: $parsed_data"
}
Code language: JavaScript (javascript)
In this example, we have a variable data
containing a string value “123”. Inside the catch
block, we try to multiply the data by 2 using expr
. Since the data is a string, an error occurs during the multiplication. The catch
command captures this error, and we print an error message inside the if
block using the $result
variable.
Question:
Explain the concept of namespaces in Tcl and why they are important.
Answer:
In Tcl, namespaces are used to organize variables and commands into separate contexts or scopes. They are essential for preventing naming conflicts and providing modularity in large Tcl projects. Namespaces allow developers to encapsulate code and data, making it easier to manage and maintain complex Tcl programs.
Tcl automatically creates a global namespace by default, where global variables and commands reside. However, developers can create their own namespaces using the namespace
command. Variables and procedures defined within a namespace are only accessible within that namespace unless explicitly qualified.
Example:
# Create a new namespace
namespace eval my_namespace {
variable data "This is data in my_namespace"
}
# Access the variable from outside the namespace
puts "Data outside the namespace: $my_namespace::data"
# Access the variable from within the namespace
namespace eval my_namespace {
puts "Data inside the namespace: $data"
}
Code language: PHP (php)
In this example, we create a namespace named my_namespace
using namespace eval
. Inside the namespace, we define a variable data
. Outside the namespace, we can access the variable using the fully qualified name my_namespace::data
. Inside the namespace, we can directly use the variable name data
.
Question:
Write a Tcl code snippet to loop through a list of numbers, filter even numbers, and store them in a new list variable.
Answer:
set number_list {1 2 3 4 5 6 7 8 9}
set even_numbers {}
foreach num $number_list {
if {$num % 2 == 0} {
lappend even_numbers $num
}
}
puts "Even Numbers: $even_numbers"
Code language: JavaScript (javascript)
In this code snippet, we have a list of numbers in number_list
. We initialize an empty list named even_numbers
. The foreach
loop iterates through each number in the number_list
, and the if
condition checks if the number is even using the modulo operator (%). If the number is even, we use lappend
to add it to the even_numbers
list. Finally, we print the even numbers.
Question:
Explain the concept of event-driven programming in Tcl and how it can be used in GUI applications.
Answer:
Event-driven programming in Tcl is a paradigm where the flow of the program is determined by external events or user interactions. Instead of following a sequential execution path, event-driven programs wait for events to occur and then trigger appropriate actions or callbacks associated with those events. This makes Tcl particularly suitable for GUI applications, where user interactions like mouse clicks, button presses, or keystrokes generate events that drive the program’s behavior.
Tcl’s event loop, typically managed by the vwait
command or in event-driven frameworks like tk
, continuously waits for events and dispatches them to the appropriate event handlers.
Example:
# Create a simple GUI application
package require Tk
# Define a function to handle button click event
proc button_click {} {
puts "Button Clicked!"
}
# Create the main application window
wm title . "Event-Driven GUI"
# Create a button with a callback
button .btn -text "Click Me" -command button_click
# Place the button in the main window
pack .btn
Code language: PHP (php)
In this example, we use the Tk
package to create a simple GUI application. We define a procedure button_click
to handle the button click event. When the user clicks the button, the button_click
procedure will be executed, and it will print “Button Clicked!” to the console.
Question:
Explain the concept of file handling in Tcl and provide an example of reading and writing to a file.
Answer:
File handling in Tcl involves operations like reading data from files, writing data to files, and manipulating file attributes. Tcl provides various commands to interact with files, such as open
, read
, write
, and close
.
Example – Reading from a File:
set filename "data.txt"
set file_handle [open $filename r]
set data [read $file_handle]
close $file_handle
puts $data
Code language: PHP (php)
In this example, we specify the file name using the variable filename
. We use the open
command to open the file in read mode (r
) and store the file handle in the variable file_handle
. The read
command reads the contents of the file into the variable data
. Finally, we close the file using the close
command and print the data to the console.
Example – Writing to a File:
set filename "output.txt"
set file_handle [open $filename w]
set data "This is some data to write to the file."
puts $file_handle $data
close $file_handle
Code language: PHP (php)
In this example, we specify the file name using the variable filename
. We use the open
command to open the file in write mode (w
) and store the file handle in the variable file_handle
. The puts
command writes the data to the file. Finally, we close the file using the close
command.
Question:
Explain the concept of sockets in Tcl and provide an example of how to create a simple TCP server.
Answer:
In Tcl, sockets are used to establish network connections and facilitate communication over the network. Sockets are a fundamental part of client-server communication, where a server listens for incoming connections from clients.
Example – Creating a TCP Server:
# Create a TCP server
set server_socket [socket -server accept_connection 8888]
# Procedure to handle incoming connections
proc accept_connection {channel addr port} {
puts "Received connection from $addr on port $port"
puts $channel "Hello, client! Welcome to the server."
flush $channel
close $channel
}
Code language: PHP (php)
In this example, we use the socket
command with the -server
option to create a TCP server on port 8888. The server listens for incoming connections and, when a connection is established, calls the accept_connection
procedure to handle the connection. The accept_connection
procedure prints a welcome message to the client and closes the connection after responding.
To test the server, clients can connect to it using a TCP client, such as Telnet or using Tcl’s socket
command.
Question:
Explain the concept of object-oriented programming (OOP) in Tcl and how it can be implemented using namespaces.
Answer:
While Tcl does not have built-in native support for object-oriented programming (OOP), it can be emulated using namespaces and procedures. Namespaces provide a way to encapsulate code and data, acting as objects, and procedures can be used as methods that operate on the object’s data.
A common approach to implementing OOP in Tcl is by defining a namespace as a class and procedures within that namespace as methods. Data is stored as variables within the namespace, simulating instance variables.
Example:
# Define a namespace as a class
namespace eval Person {
variable name ""
variable age 0
# Constructor method
proc new {name age} {
set person [namespace current]
variable name $name
variable age $age
return $person
}
# Method to get the name
proc get_name {} {
variable name
return $name
}
# Method to get the age
proc get_age {} {
variable age
return $age
}
}
# Create a new person object
set john [Person::new "John Doe" 30]
# Access methods
puts "Name: [Person::get_name]"
puts "Age: [Person::get_age]"
Code language: PHP (php)
In this example, we create a namespace Person
, representing the class. Inside the namespace, we have variables name
and age
, which act as instance variables. The new
procedure is the constructor method that initializes the object’s state. The get_name
and get_age
procedures are methods to access the object’s data.
We then create a new Person
object named john
, set its name and age using the constructor, and call the methods to retrieve the data.
Senior Tcl interview questions
Question:
Explain the concept of namespaces in Tcl and how they are used to avoid naming conflicts in large projects.
Answer:
In Tcl, namespaces are used to organize variables, procedures, and other commands into separate contexts or scopes to avoid naming conflicts in large projects. Namespaces allow developers to create distinct containers where commands with the same name can coexist without interfering with each other.
To define a namespace, you can use the namespace
command:
namespace eval my_namespace {
variable my_var 42
proc my_proc {} {
puts "Hello from my_proc"
}
}
Code language: JavaScript (javascript)
In this example, we create a namespace named my_namespace
. Within this namespace, we define a variable my_var
and a procedure my_proc
. The variables and procedures defined in the namespace can be accessed using the ::
syntax:
puts $::my_namespace::my_var
my_namespace::my_proc
Code language: PHP (php)
Question:
The following Tcl code is intended to read a file and print its content. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
set file_handle [open "myfile.txt"]
set content [read $file_handle]
close $file_handle
puts content
Code language: PHP (php)
Answer:
The logical error in the code is that the puts
command is used without the dollar sign ($
) to access the content
variable. The correct code is as follows:
set file_handle [open "myfile.txt"]
set content [read $file_handle]
close $file_handle
puts $content
Code language: PHP (php)
In this corrected code, the puts
command correctly uses the $content
variable to print the content of the file.
Question:
Explain what a Tcl list is and how you can manipulate and access elements in a list.
Answer:
In Tcl, a list is a data structure with a sequence of elements. Lists are represented as strings where individual elements are separated by spaces or other specified characters. Tcl provides commands to manipulate and access elements in a list easily.
To create a list, you can use curly braces {}
or the list
command:
set my_list {apple banana orange}
# or
set my_list [list apple banana orange]
Code language: PHP (php)
To access elements in a list, you can use the lindex
command:
puts [lindex $my_list 0] ;# Output: apple
puts [lindex $my_list 1] ;# Output: banana
Code language: PHP (php)
To append elements to a list, you can use the lappend
command:
lappend my_list "grape" "mango"
# my_list is now: {apple banana orange grape mango}
Code language: PHP (php)
You can also use foreach
to iterate through the elements of a list:
foreach item $my_list {
puts $item
}
Code language: PHP (php)
Question:
The following Tcl code is intended to find the factorial of a given number. However, it contains a syntax error and doesn’t produce the correct result. Identify the error and fix the code.
proc factorial {n} {
if {$n == 0} {
return 1
} else {
return $n * factorial $n-1
}
}
Code language: PHP (php)
Answer:
The syntax error in the code is the missing curly braces around the factorial $n-1
argument. The correct code is as follows:
proc factorial {n} {
if {$n == 0} {
return 1
} else {
return [expr {$n * [factorial [expr {$n-1}]]}]
}
}
Code language: PHP (php)
In this corrected code, we use [expr {}]
to evaluate the expression $n-1
before passing it to the factorial
function.
Question:
Explain the concept of Tcl event-driven programming and how it’s used to handle asynchronous events.
Answer:
Tcl supports event-driven programming, which allows handling asynchronous events such as user input, timers, or network data. In event-driven programming, the program doesn’t follow a linear flow but instead waits for specific events to occur and responds to them accordingly.
Tcl provides the vwait
and after
commands to handle event-driven programming:
vwait
: Thevwait
command waits for the variable to be set, which usually happens when an event occurs, such as user input.
proc on_button_click {} {
puts "Button clicked!"
}
button .b -text "Click Me" -command on_button_click
pack .b
vwait forever ;# Wait indefinitely for an event
Code language: PHP (php)
after
: Theafter
command schedules a procedure to run after a specified time delay, allowing you to implement timers and other asynchronous tasks.
proc print_message {} {
puts "This message appears after 3 seconds."
}
after 3000 print_message ;# Schedule print_message to run after 3000 milliseconds (3 seconds)
Code language: PHP (php)
Question:
The following Tcl code is intended to check if a given string is a palindrome. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
proc is_palindrome {str} {
set reversed [string reverse $str]
return [string equal $str $reversed]
}
Code language: PHP (php)
Answer:
The logical error in the code is that the string equal
command returns a boolean value (1
or 0
) instead of the result of the palindrome check. The correct code is as follows:
proc is_palindrome {str} {
set reversed [string reverse $str]
return [expr {[string equal $str $reversed] ? 1 : 0}]
}
Code language: PHP (php)
In this corrected code, we use expr
to explicitly return 1
if the strings are equal (i.e., it’s a palindrome) and 0
if they are not.
Question:
Explain what Tcl’s coroutine
is and how it can be used to implement cooperative multitasking.
Answer:
Tcl’s coroutine
is a powerful feature that allows you to implement cooperative multitasking, where multiple execution contexts (coroutines) can yield control to each other voluntarily. Unlike traditional multithreading, cooperative multitasking does not involve preemptive context switching; instead, coroutines yield control explicitly to other coroutines.
You can create a coroutine using the coroutine
command:
coroutine my_coroutine {
# Coroutine body
}
Code language: PHP (php)
Inside a coroutine, you can use the yield
command to suspend the coroutine’s execution and allow other coroutines to run:
coroutine my_coroutine {
puts "Coroutine starts"
yield
puts "Coroutine resumes"
}
Code language: JavaScript (javascript)
To schedule coroutines and allow them to cooperate, you can use the coroutine::run
command:
coroutine my_coroutine {
puts "Coroutine starts"
yield
puts "Coroutine resumes"
}
coroutine::run my_coroutine
puts "Back in the
main thread"
Code language: PHP (php)
In this example, the coroutine my_coroutine
is created and scheduled to run. It prints “Coroutine starts,” yields control, and then resumes its execution to print “Coroutine resumes.” The main thread also continues to execute and prints “Back in the main thread.”
Question:
The following Tcl code is intended to calculate the sum of integers from 1 to n using a loop. However, it contains a syntax error and doesn’t produce the correct result. Identify the error and fix the code.
proc sum_up_to_n {n} {
set sum 0
for {set i 1} {$i <= n} {incr i} {
set sum + i
}
return $sum
}
Code language: JavaScript (javascript)
Answer:
The syntax error in the code is that the set sum + i
line does not correctly update the sum
variable. The correct code is as follows:
proc sum_up_to_n {n} {
set sum 0
for {set i 1} {$i <= $n} {incr i} {
incr sum $i
}
return $sum
}
Code language: JavaScript (javascript)
In this corrected code, we use incr sum $i
to add the value of i
to the sum
variable in each iteration of the loop.
Question:
Explain the concept of Tcl’s safe interpreter and how it provides a sandboxed execution environment for untrusted scripts.
Answer:
In Tcl, a safe interpreter is a separate interpreter instance that provides a sandboxed execution environment for untrusted scripts. It allows you to execute potentially unsafe scripts in a restricted environment, preventing them from accessing critical resources and commands in the main interpreter.
To create a safe interpreter, you can use the safe
command:
set safe_interp [safe interp]
Code language: JavaScript (javascript)
The safe interpreter has the following restrictions:
- Limited commands: The safe interpreter can only access a predefined set of safe commands and cannot execute arbitrary Tcl commands or external commands.
- No access to global variables: The safe interpreter cannot access global variables of the main interpreter, ensuring data isolation.
- Restricted file access: The safe interpreter may have limited or no file system access to prevent reading or writing sensitive files.
The safe interpreter can be used to evaluate untrusted scripts or perform certain tasks that require restricted execution:
set script {
proc dangerous_proc {} {
file delete / ;# Try to delete the root directory
}
}
safe_interp eval $script
Code language: PHP (php)
In this example, the dangerous_proc
procedure attempts to delete the root directory, but since it is executed within the safe interpreter, it is restricted from performing such operations.
Question:
The following Tcl code is intended to read a CSV file and store its contents in a list of dictionaries, where each dictionary represents a row. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
set file_handle [open "data.csv"]
set rows {}
while {[gets $file_handle line] != -1} {
lassign [split $line ,] col1 col2 col3
dict set row col1 $col1 col2 $col2 col3 $col3
lappend rows $row
}
close $file_handle
Code language: PHP (php)
Answer:
The logical error in the code is that the dict set
command is not used correctly to create dictionaries for each row. The correct code is as follows:
set file_handle [open "data.csv"]
set rows {}
while {[gets $file_handle line] != -1} {
lassign [split $line ,] col1 col2 col3
set row [dict create col1 $col1 col2 $col2 col3 $col3]
lappend rows $row
}
close $file_handle
Code language: PHP (php)
In this corrected code, we use dict create
to create a new dictionary row
for each row in the CSV file. The dictionaries are then appended to the list rows
to store the entire dataset.
1,000 Companies use CoderPad to Screen and Interview Developers
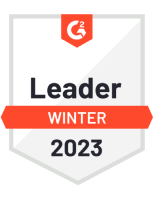
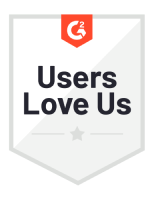
Interview best practices for Tcl roles
In order to conduct effective Tcl interviews, it’s essential to take into account various factors, such as the candidates’ experience levels and the specific engineering role they’re pursuing. To guarantee that your Tcl interview questions generate optimal outcomes, we suggest following these best practices when engaging with candidates:
- Create technical questions that reflect real-world situations within your organization. This strategy will not only captivate the candidate but also enable you to more accurately assess their suitability for your team.
- Foster a collaborative environment by inviting candidates to ask questions throughout the interview.
- Candidates should be familiar with common Tcl libraries that you use — like Tk for GUIs and Expect for application automation.
Additionally, it’s vital to adhere to conventional interview practices when carrying out Tcl interviews. This involves tailoring question difficulty according to the candidate’s ability, offering prompt feedback on their application status, and permitting candidates to inquire about the evaluation or collaborate with you and your team.