Example React Interview Questions
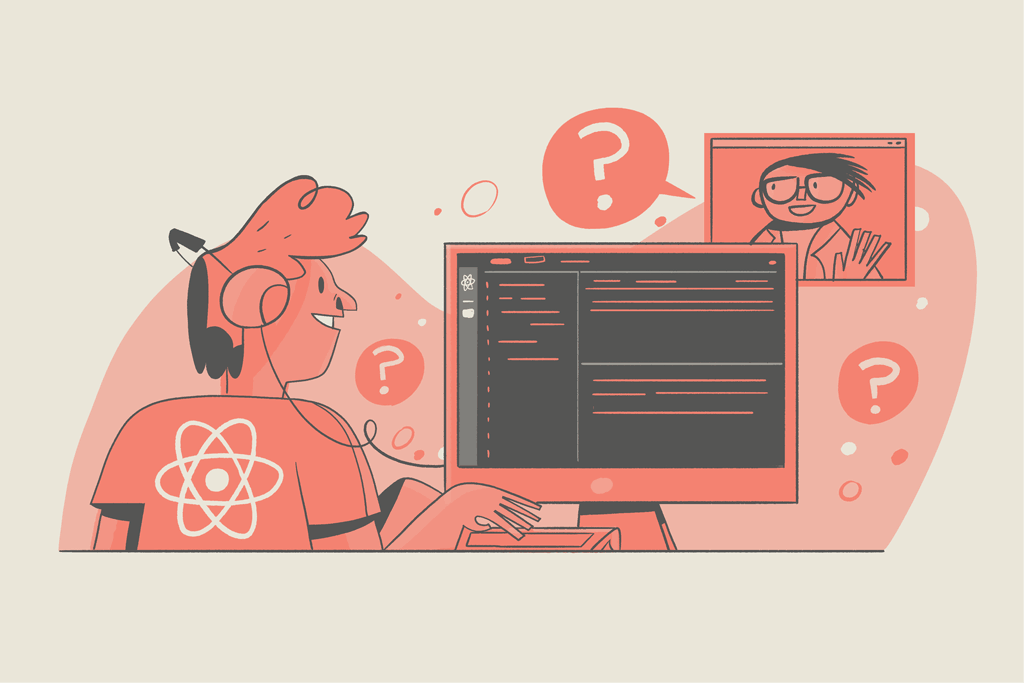
Looking to hire a React developer? Get in line…
React developers are some of the most in-demand front-end developers.
So what can you do to compete?
Create a great interview experience! And one of the best ways to do that is to create technical interview questions that allow you to assess a candidate’s technical skills in a way that’s both fun and challenging to the candidate.
Try using the example React interview questions below to create a creative, engaging interview experience.
React interview question 1: Virtualized list
This exercise aims to have the candidate render every word in the English language in a single, scrollable, performant list. To complete it, candidates will need to demonstrate their skills in render optimization fundamentals. You’ll also be able to assess their problem-solving skills and see how they communicate their approach to the challenge.
In the example question below, you’ll see that we’ve added an App.tsx
file, where there’s a useDictionary()
hook already written. This hook will fetch the entire dictionary and return it in a nice array.
The dictionary has nearly 400K words, far too many elements to write to the DOM at once. It will almost certainly crash web browsers. For this reason, we added a component called <SafelyRenderChildren
> that puts an artificial limit on the number of children you can render at once to ensure that there’s no chance of the candidate crashing their browsers during the interview.
Create learning opportunities
Your candidates may or may not be familiar with virtualization for element rendering. As such, we’ve included a brief description of what virtualization is for the candidates:
What is virtualization?
Virtualization in client-side development, deals with the concept of only rendering elements that are critical for providing a seamless user experience, and culling every element that is off the screen.
So instead of rendering 500K list items, we might start with only rendering 500 items, and then progressively adding/removing list items as the user scrolls.
The goal is to deliver a user experience that feels as seamless as possible.
If they already know what it is –great! If not, we hope to help enhance their interview experience by teaching them about something new and increasing their chances of success with the exercise.
Goals and stretch goals
Once the background information is laid out, you can proceed with laying out the goals for this particular technical exercise.
Here are the goals we will set for the candidates to test their understanding of the task and implementing virtualization:
- When loading the page, we should see a list that is filled with words, the scrollbar should be as long as the entirety of the dictionary, appearing as if every word is already present in the list.
- Scrolling using the mouse wheel should feel completely seamless. The elements should stay positioned correctly and not jump around as elements load/unload.
- We should be able to drag the scrollbar to any position in the list and have the correct items be visible.
Some candidates will spend the whole time working on meeting those goals – and that’s fine. For candidates that can complete the main tasks, you can throw in a stretch goal, like adding a search box to the list.
Constraints
Like building any real-life application, this React exercise has its own requirements and constraints. In particular:
- Each list item must have a known height (30px).
<SafelyRenderChildren/>
should limit the maximum list items to 2,500.
Final tips and advice
Before letting the candidate jump into coding, let’s offer them some further advice on how to be successful with this exercise:
- The amount of items you render at one time is completely up to you, and should be tuned for a balance of performance and usability – i.e. we want no visual evidence of loading when mouse wheel scrolling, and minimal evidence of loading when scrolling large distances at one time.
- There is an optional included
useScrollPosition
hook that you can use to quickly get the current scroll position.
What the candidate sees
If we put this all together into a CoderPad, we can see what the experience would be like for you and the candidate. You can even try out the exercise yourself:
React interview question 2: File tree
This question tests a candidate’s ability to generate a complex UI from a simple input and ability to read and render data structures.
In this challenge, they will build an arbitrarily-deep file tree explorer given a list of file objects consisting of a path
and the contents
of the file.
Goals and stretch goals
More specifically, the candidate:
- Must display files in a nested structure, with entries for each folder and file
- Must be able to handle arbitrarily-deep file structures
If they solve this challenge and still have more time, their follow-on task is to implement the ability to add new files to the file tree.
When it comes to assessing how the candidate did in achieving these goals, its helpful to ask the following questions:
- How efficient is their algorithm? How many times do they loop over the files list?
- How accurate is their solution? Are they sorting things correctly according to the requirements? Does the solution handle edge cases?
- How do they get to the answer? Do they immediately know how to solve and just jump into writing code? If they don’t know the answer right away, do they know how to utilize appropriate resources (e.g., Google, StackOverflow, you, etc.) to find a suitable solution?
- Do they test their code?
Constraints
In sorting the file tree, the following rules must be followed, which mirror the experience we would want the end user to have:
- Folders are shown before files
- All items are sorted alphabetically (case-insensitive)
What the candidate sees
This CoderPad demonstrates how the question would appear to a candidate in a technical interview:
React interview question 3: To-do list
In this challenge the candidate will display their knowledge of object-oriented programming. The goal of the exercise is to create a working todo list with persistent data storage.
The candidate is presented with starter code that creates a styled todo list that supports adding “todo” tasks. There are also premade styles for completed todo items.
However you will notice that there’s no working mechanism for “completing” a todo list item…
Goals and stretch goals
The candidate should complete the following tasks in order be successful at this exercise:
- Clicking on a todo item should toggle the “checked” state.
- The todo list state should be saved and loaded from local storage.
- Checked items should sink to the bottom of the list automatically.
If the candidate is able to complete these goals, they can then attempt the following stretch goals:
- Allow todo items to be deleted. When you hover your mouse over a todo, an “X” should appear on the far right side, clicking the “X” should remove it from the list.
- Add hidden timestamps to todo list items (
created_at
,completed_at
), these will be used for sorting:- The active todos should be sorted by
created_at
-> descending - The completed todos should be sorted by
completed_at
-> ascending
- The active todos should be sorted by
What the candidate sees
More interviewing resources
✅ Interested in using this question in a CoderPad Live interview? It’s easy, simply follow the instructions here.
Even for the most experienced managers, hiring the right engineers can be tricky.
To make it easier, here are some more resources – more example questions and interview tips – to help make your hiring process as smooth as possible: