Introduction to Smart Contracts and Solidity
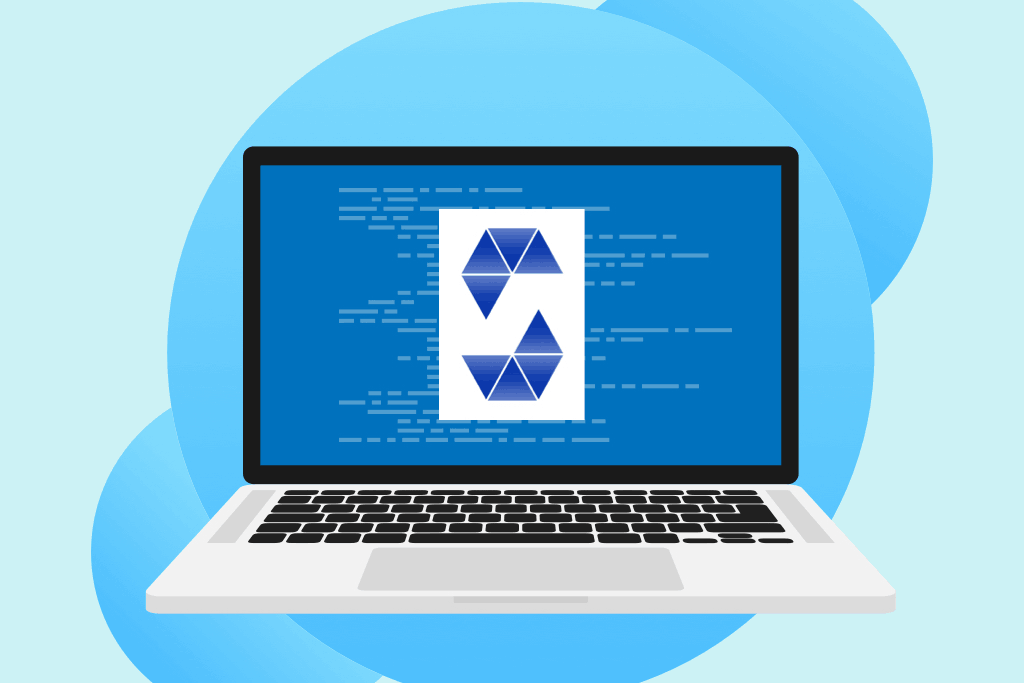
A smart contract is an agreement between one or more parties written as code. The participants define the rules and conditions between them before drafting the contract.
When the conditions established beforehand in the contract are fulfilled between the participants, the contract takes its complete form, and each party must fulfill its obligation.
These contracts are deployed on a blockchain to be executed. The deployment and execution of these contracts cost fees that are paid to miners.
Miners are an essential component of a blockchain. Indeed, it is the miners who make it possible for transactions to take place on the blockchain. They voluntarily provide their computer resources or the space on their hard disk to store and validate transactions. In return, they receive a reward in the form of Ether.
Smart contracts are important because they can be conducted in a dematerialized, secure, and decentralized system without the intervention of a trusted third party or middleman. Developers should learn how to implement them because these tools can potentially impact our personal and professional lives drastically.
This introduction to smart contracts is broken up into the following sections:
How smart contracts work
To understand how smart contracts work, we need to talk about the link between smart contracts and the blockchain.
The blockchain is like a vast database that contains the history of all transactions made between users since its creation. In simple terms, the blockchain is a Peer-To-Peer (P2P) network on which smart contracts are deployed so that they can perform the functions for which they have been written.
There are a multitude of blockchains, but they all work in much the same way. The most popular are Bitcoin and Ethereum blockchains.
This article will focus on the Ethereum blockchain, created by Vitalik Buterin and his team. It is an open-source blockchain that has allowed developers to program and execute their smart contracts since 2015.
Ethereum is based on the Ethereum Virtual Machine (EVM), the environment in which smart contracts will run. Users run their contracts in the EVM in exchange for fees called “gas” expressed in Ether (ETH).
Ether is a kind of cryptographic fuel for the Ethereum network. For every transaction made on the network, a certain amount of gas (energy) is consumed to execute that transaction, and this gas can only be paid for in Ether. This transaction fee that you pay to process your transaction goes to the miners.
Structure of a smart contract
To write smart contracts, you can use one of several languages supported by Ethereum, including Solidity, Vyper, and Low-level Lisp-like Language (LLL). This section will focus on the most used language for writing smart contracts: Solidity.
Solidity is a high-level language oriented to smart contracts, influenced by C++, Python, and JavaScript. The extension of the Solidity contract file is .sol, for example, ExampleContract.sol
.
The basic layout of the Solidity source file contains the following elements in order:
- License: Each source file must start with a comment indicating its license.
- Version pragma: Source files may be annotated with a version pragma to reject compilation with future compiler versions that might introduce incompatible changes. These versions always have versions of the form 0.x.0 or x.0.0.
- Import statements: for importing other contracts/libraries
- Contract definition: to declare a new contract. The name of the contract is prefixed by the keyword
contract
, as shown in the figure below.
//SPDX-License-Identifier:GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Authenticator {
}
Code language: JavaScript (javascript)
Solidity contracts can be compared to classes as you’d find in Java. These contracts can contain several elements more or less familiar to programming languages, namely:
- State variables (string, uint, address, etc.)
- Functions
- Function modifier
- Events.
You can check out the Solidity documentation for more details on these elements.
Let’s implement your first smart contract
We will develop a contract to “certify” products for our demo. The contract owner will be responsible for adding their products. Once the products are added, the product owners can check if their product is authentic using its barcode.
If the product is genuine, they will receive all its information. If not, they will not receive anything.
Development
Before we begin, please note that instructions in the Solidity language always end with a semicolon.
First, we will create a file Authenticator.sol
inside the contract folder.
Inside the Authenticator.sol
file, we start by declaring the license, the pragma version, and our contract class.
//SPDX-License-Identifier:GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Authenticator {
}
Code language: JavaScript (javascript)
Now let’s move on to our state variables.
Step 1: Define variables
//SPDX-License-Identifier:GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Authenticator {
address public chairperson;
uint public productCount;
struct Product {
uint id;
string name;
uint barCode;
}
mapping (uint => Product) private products;
}
Code language: PHP (php)
We start by declaring the variable chairperson
; it is of type address and will correspond to the address of the person who will have the right to add products.
Then we will declare another variable of type uint
; which is basically an integer. This uint
will be called productCount
, corresponding to the total number of products added.
The public
attribute added to the variable allows us to access the value of productCount
outside the contract.
The third state variable here will be a Structure (struct
). To make it simple, it looks a bit like objects in JavaScript, and just like JavaScript objects, we can store properties in structures. Structures are used to store more complex data than strings
or uint
.
So we created a struct named product, which will have properties: id
, name
, and barCode
.
Then we created a mapping that we called products. This mapping will allow us to associate a key type uint
(the barcode) to a value type Product
. Product
corresponds to the struct
we just created just before.
The mappings act like hash tables that consist of key types and corresponding value type pairs.
We are now done with the variables of our contract!
Step 2: Use a constructor to create a new instance of the contract
//SPDX-License-Identifier:GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Authenticator {
address public chairperson;
uint public productCount;
struct Product {
uint id;
string name;
uint barCode;
}
mapping (uint => Product) private products;
constructor(){
chairperson = msg.sender;
productCount = 0;
}
}
Code language: JavaScript (javascript)
In the constructor
, we initialize the chairperson
by the person who creates the instance with msg.sender
.
msg
is a global variable of Solidity that has properties to access the blockchain. msg.sender
refers to the address where the function call comes from.
We also initialize the number of products to 0. So once the contract is deployed, these variables defined in the constructor
will automatically take the values assigned to them.
Step 3: Add products function
//SPDX-License-Identifier:GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Authenticator {
address public chairperson;
uint public productCount;
struct Product {
uint id;
string name;
uint barCode;
}
mapping (uint => Product) private products;
constructor(){
chairperson = msg.sender;
productCount = 0;
}
function addProduct (string memory _name, uint _barCode) public {
require (msg.sender == chairperson);
products[_barCode] = Product (productCount, _name, _barCode);
productCount ++;
}
}
Code language: JavaScript (javascript)
We now arrive at the functions of our contract. The addProduct
function is the function that will allow the contract holder to add products.
This function will take the product name and barcode as parameters. By convention, function parameters in Solidity are preceded by an underscore ( _
) to differentiate them from state variables.
We give our function public visibility so it can be accessed outside the contract.
The addProduct
function can only be called by the chairperson
. We use the require
to do this. Once the products are added, the productCount
is updated.
Step 4: Retrieve products functions
//SPDX-License-Identifier:GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Authenticator {
address public chairperson;
uint public productCount;
struct Product {
uint id;
string name;
uint barCode;
}
mapping (uint => Product) private products;
constructor(){
chairperson = msg.sender;
productCount = 0;
}
function addProduct (string memory _name, uint _barCode) public {
require (msg.sender == chairperson);
products[_barCode] = Product (productCount, _name, _barCode);
productCount ++;
}
function getProduct(uint _productBarcode) public view returns (Product memory){
return products[_productBarcode];
}
}
Code language: JavaScript (javascript)
Now that we can add a product, we can set up the function that checks the authenticity of the products. The goal is to create a function that takes a barcode as a parameter. If the product exists, the function will return its information. Otherwise, it will return nothing.
So we will create the function getProduct
. We use the _productBarCode
as the product’s barcode so that the function returns the product information.
This function is of type View
because it has a method that executes without modifying the state of the contract.
Step 5: Test it out
So we are done with our contract! You can easily run it in CoderPad’s sandbox:
Once the code is run we will see our contract displayed in the Program Output.
So we can add a product by passing to the method the name and the barcode of the product; to check the product’s existence, we just have to pass the product’s barcode to the method getProduct
.
If the product exists, it will return some information—otherwise, it will return nothing.
Congratulations, you created your first smart contract!
Henok Adeyama is an eternal learner. Passionate about programming, he enjoys sharing his knowledge with others. Find him on GitHub and Twitter.