How to Build a URL Shortener in React with Shrtcode
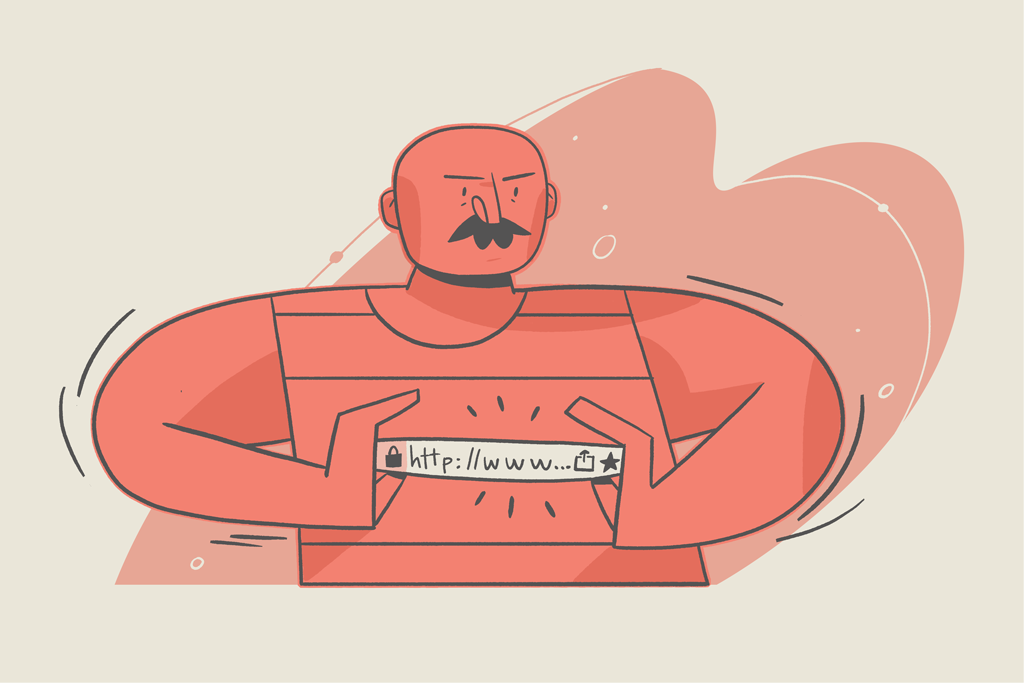
URLs tends to get lengthy, especially when parameters and query strings are attached. This can get annoying when you want to share a link. For example, long URLs can eat into your character limit on social media platforms. Because of this, websites offering URL-shortening services like Bitly, TinyURL, and Short.io have cropped up.
A URL shorterner is a tool that reduces the length of website addresses, creating a short, unique link that redirects to the desired web page when clicked. This makes the URL easier to share, remember, and track. The new unique URL generated usually includes the shortener site’s address and a combination of random letters.
For example, if you want to shorten https://coderpad.io/resources/docs/interview/ with Shrtcode, the resulting URL will look like this: https://shrtco.de/xBcIt where the site’s address is https://shrtco.de and the rest after the slash is the identifier for the link generated by the API.
What is URL shortening?
URL shortening is achieved by storing the origin link along with the random letters attached to the shortened URL in a database. When the short URL is clicked, the server uses the random number to get the original link and then redirects to it. Of course, doing this requires creating a backend.
In this tutorial, we will be using the Shrtcode API to achieve this functionality, so we won’t be doing any of the behind-the-scenes stuff.
Pros and Cons of URL shorteners
As Moneyball author Michael Lewis said, “There’s something bad in everything good and something good in everything bad.” Let’s find out what those are for URL shorteners.
Pros of URL shorteners:
- They promote sharing: Adding shortened URLs on printed materials reduces the number of words readers have to type to copy it. They also make sharing easier in messaging technologies that limit the number of characters in a message.
- Creates a friendlier URL: Most URL shorteners can be used to generate custom links rather than random numbers in order to make URLs intuitive, more appealing, and easy to remember. These custom links act as an abstraction to a more lengthy URL which might include encodings and characters that may be confusing to the average reader.
- Provides analytics data: Most URL shorteners include features to track the performance of links, giving information on click-throughs and traffic sources. Businesses can then use this information to inform their content distribution strategies.
Cons of URL shorteners:
- Dead links: If the server of the tool that was used to shorten the URLs is down, all links will stop working.
- Blocked URLs: Some platforms do not accept shortened URLs and a number of URL shorteners have been included on spam blacklists. Sites that have been blacklisted for sending spam tend to use URL shorteners to bypass the blacklist which has made many URL shorteners meet the same fate.
- Facilitates phishing attacks: Since URLs shorteners obscure the address of a site with random numbers, shortened URLs are used by scammers to drive users to URLs and websites that look legitimate but are specifically designed to collect sensitive information.
What is Shrtcode
Shrtcode is a URL shorterner that offers free use of its shortening services. It puts special emphasis on security, constantly checking that shortened are always pointing to the original site and are free of malware and virus. Shrtcode also offers custom URL generation, QR code generators, password-protected links, and more. However, only the URL shortening features are documented in its docs. To get access to all other features it needs to be requested via email using [email protected].
How to build a URL shortener with Shrtcode
The URL shortener we will build will have an input field to enter the original, long URL and a section to view the shortened URL. It will also include functionality to copy the shortened URL to the clipboard, which will be added using react-copy-to-clipboard. Let’s get started:
Set up React
Spin up a React sandbox on CoderPad:
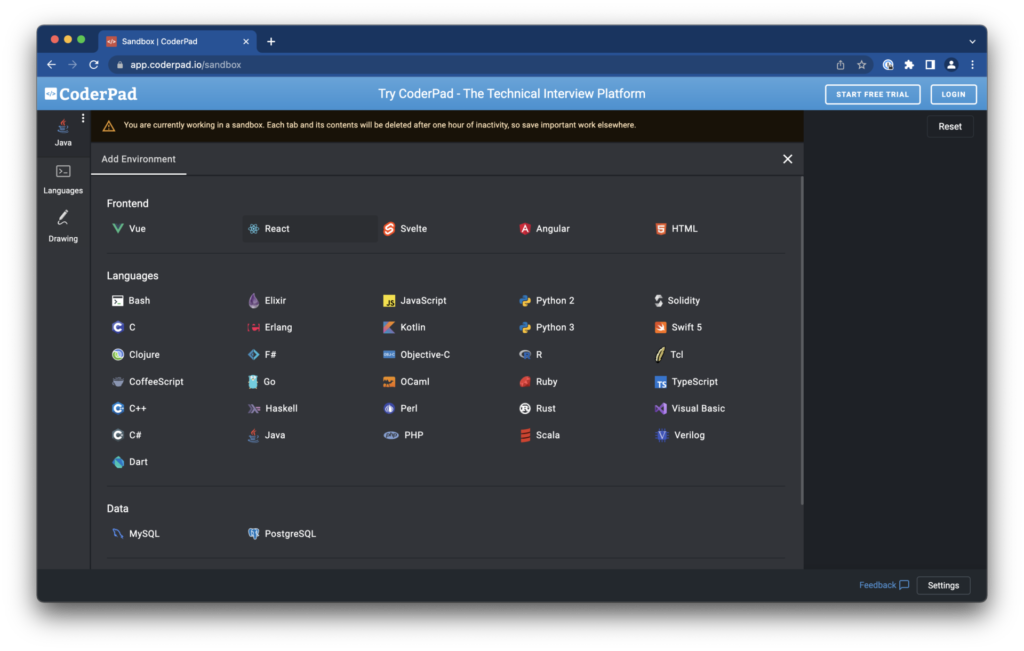
💡 CoderPad sandboxes gives you the native code editor experience when building applications and conducting interviews.
In the newly created sandbox, replace the content in App.tsx
:
import { useState } from 'react';
import './App.css';
function App() {
const [url, setUrl] = useState()
const [shortendUrl, setShortenedUrl] = useState('')
return (
<div className="app">
<div className='shortener'>
<h2>URL shortener</h2>
{/* form to enter URL to be shortened */}
<form>
<input
placeholder='Enter URL'
value={url}
onChange={(e) => setUrl(e.target.value)}/>
<button>Submit</button>
</form>
{/* Section to view shortened URLS */}
{shortendUrl &&
<div className='shortener__viewShot'>
{shortendUrl}
</div>
}
</div>
</div>
);
}
export default App;
Code language: JavaScript (javascript)
In the code block above, we created a url
used to make the input field controlled and a shortendUrl
state which will hold the shortened URL.
Next, modify App.css
:
* {
box-sizing: border-box;
}
.shortener {
width: fit-content;
margin: 0 auto;
text-align: center;
}
.shortener input, button {
height: 30px;
padding: 0 10px;
}
.shortener input {
border: 1px solid gray;
color: rgb(64, 64, 66);
}
.shortener input:focus {
border: 1px solid rgb(95, 180, 245);
outline: none;
}
.shortener form > button {
background-color: rgb(95, 180, 245);
border: none;
color: white;
}
.shortener form > button:hover {
background-color: rgb(51, 162, 246);
}
.shortener__viewShot {
margin-top: 20px;
border: 1px solid gainsboro;
display: flex;
justify-content: space-between;
padding-left: 10px;
color: rgb(64, 64, 66);
align-items: center;
}
.shortener__viewShot button {
background-color: transparent;
border: none;
color: gray;
}
.shortener__viewShot button:hover {
color: rgb(64, 64, 66);
}
Code language: CSS (css)
The sandbox builds the changes and the URL shortener application can be viewed in the preview panel:

How to shorten URLs with Shrtcode
To shorten URLs with Shrtcode, we just need to pass the URL as a query to the API endpoint as described in their docs. Head over to the App.tsx
and add the following function after the shortendUrl
state in the App
component:
const shortenUrl = async (e) => {
e.preventDefault();
try {
const response = await fetch(
`https://api.shrtco.de/v2/shorten?url=${url}`
)
const data = await response.json()
setShortenedUrl(data.result.full_short_link);
} catch (e) {
alert(e);
}
};
Code language: JavaScript (javascript)
In the above function we have attached the original URL as a query to Shrtcode’s shortening endpoint and send a GET request to that endpoint. Then when the response returns, it gets stored in the shortendUrl
state variable.
Next, we need to call this function when the Submit button is clicked. For this, modify the opening form tag in the App
component to the following:
<form onSubmit={shortenUrl}>
Code language: HTML, XML (xml)
With this, in our app, when we enter a URL in the input and click the Submit button, we will see the result like the following:

https://www.npmjs.com/pack
shortened using Shrtcode’s API is returned beneath the input box.Copy shortened URL to the clipboard
Now let’s make it so that we can copy the shortened URL to the clipboard when we click the copy button. For this, we will be using react-copy-to-clipboard. In the shell console in the sandbox, install the library:
npm i react-copy-to-clipboard
Next, we need to pass the button to trigger the copying feature as a child to the CopyToClipboard
component and pass the short URL to be copied as props to it.
To do this, import the CopyToClipboard
component from thenreact-copy-to-clipboard
library and modify the div with the class name of shortener__viewShot
to the following version:
import {CopyToClipboard} from 'react-copy-to-clipboard';
...
<div className="shortener__viewShot">
{shortendUrl}
<CopyToClipboard text={shortendUrl}>
<button onClick={() => alert("The URL has been copied")}>copy</button>
</CopyToClipboard>
</div>
Code language: HTML, XML (xml)
Now when the copy button is clicked in our app, the short URL will be copied to the clipboard and the app will display an alert saying so.
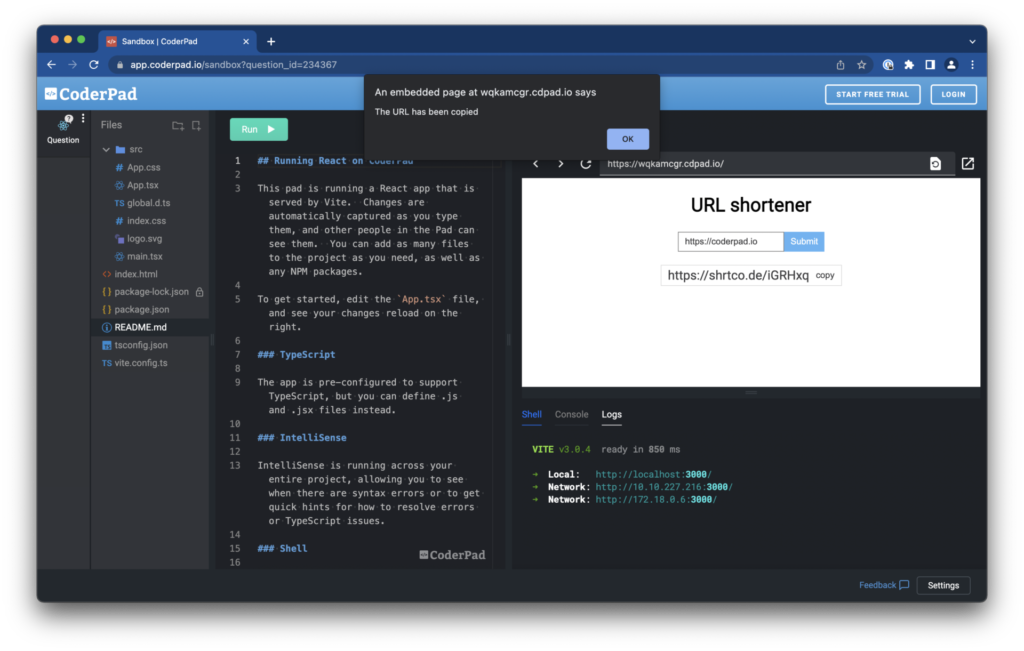
Try it out in the CoderPad sandbox
Now you should know how to add a URL shortener to your app with Shrtcode. This should come in handy whether you are building a social media app that automatically shortens links in posts just as LinkedIn does, a verification system where a link is also attached to the email as an alternative to the verification button or any other app where shorter links are preferred.
Shorten your URLs in the sandbox below:
Taminoturoko Briggs is an enthusiastic software developer and technical writer. Core languages include JavaScript and Python.