Verilog Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Verilog developers for your organization.
Verilog
Verilog is a popular hardware description language (HDL) used extensively in the design of digital circuits and systems.
The Institute of Electrical and Electronics Engineers (IEEE) solidified Verilog’s status as an official HDL by adding it to IEEE Standard 1364 in 1995.
In order to assess the Verilog proficiency of developers during coding interviews, we have supplied practical coding tasks and interview queries below.
Furthermore, we have delineated a collection of recommended guidelines to guarantee that your interview inquiries effectively gauge the candidates’ Verilog capabilities.
Table of Contents
Verilog example question
Help us design a parking lot app
Hey candidate! Welcome to your interview. Boilerplate is provided. Feel free to change the code as you see fit. To run the code at any time, please hit the run button located in the top left corner.
Goals: Design a parking lot using object-oriented principles
Here are a few methods that you should be able to run:
- Tell us how many spots are remaining
- Tell us how many total spots are in the parking lot
- Tell us when the parking lot is full
- Tell us when the parking lot is empty
- Tell us when certain spots are full e.g. when all motorcycle spots are taken
- Tell us how many spots vans are taking up
Assumptions:
- The parking lot can hold motorcycles, cars and vans
- The parking lot has motorcycle spots, car spots and large spots
- A motorcycle can park in any spot
- A car can park in a single compact spot, or a regular spot
- A van can park, but it will take up 3 regular spots
- These are just a few assumptions. Feel free to ask your interviewer about more assumptions as needed
Verilog skills to assess
Jobs using Verilog
Junior Verilog interview questions
Question:
Explain the concept of a flip-flop in Verilog and provide an example of its usage in a real-world application.
Answer:
In Verilog, a flip-flop is a fundamental building block used to store and synchronize data in digital circuits. It is a sequential logic element that operates based on a clock signal. There are different types of flip-flops, such as D flip-flop, JK flip-flop, and T flip-flop, each with specific functionality.
Example usage: A common real-world application of a flip-flop is in the design of registers or memory elements. For instance, in a microcontroller or processor, flip-flops are used to store intermediate results, control signals, or temporary data during the execution of instructions.
Question:
What is the purpose of a testbench in Verilog, and why is it essential during the development process?
Answer:
A testbench in Verilog is a module or set of modules used to simulate and verify the functionality of a design. It is an essential part of the development process because it allows developers to create test scenarios and test the correctness of their designs before synthesis and implementation in hardware.
The testbench generates test stimuli to apply to the design and monitors the responses or outputs. By comparing the expected outputs with the actual outputs during simulation, developers can identify design issues, validate the correctness of the hardware description, and ensure the design meets the desired specifications. This verification process helps catch bugs and ensures the design behaves as expected in different scenarios.
Question:
Explain the difference between blocking and non-blocking assignments in Verilog.
Answer:
In Verilog, both blocking and non-blocking assignments are used to assign values to variables. The main difference between them lies in their evaluation timing and how they impact the simulation behavior.
- Blocking assignments (
=
): In a blocking assignment, the right-hand side (RHS) expression is evaluated immediately, and the assignment is executed in the same simulation time step. It means that the assignments are executed sequentially in the order they appear in the code, and the next statement has to wait for the current assignment to finish.
Example:
always @(posedge clk)
begin
a = b; // 'a' is immediately updated with the current value of 'b'
c = a; // 'c' is immediately updated with the current value of 'a' after the previous assignment is completed
end
Code language: JavaScript (javascript)
- Non-blocking assignments (
<=
): In a non-blocking assignment, the RHS expression is evaluated concurrently with other statements in the always block, and the assignments are scheduled to be executed in the next time step. This allows multiple assignments to happen simultaneously without any particular order.
Example:
always @(posedge clk)
begin
a <= b; // 'a' will be updated with the current value of 'b' in the next time step
c <= a; // 'c' will be updated with the current value of 'a' in the next time step as well, regardless of the previous assignment
end
Code language: JavaScript (javascript)
Using non-blocking assignments is common for modeling flip-flops or sequential logic, as it reflects the behavior of hardware elements that store data based on a clock signal.
Question:
Provide an example of a Verilog module that implements a 4-to-1 multiplexer (MUX) and explain its functionality.
Answer:
module mux_4to1 (
input [3:0] data_in, // 4-bit input data
input [1:0] select, // 2-bit select signal
output reg data_out // 1-bit output data
);
always @(*)
begin
case (select)
2'b00: data_out = data_in[0]; // select input 0
2'b01: data_out = data_in[1]; // select input 1
2'b10: data_out = data_in[2]; // select input 2
2'b11: data_out = data_in[3]; // select input 3
default: data_out = 1'b0; // default output value if select is invalid
endcase
end
endmodule
Code language: PHP (php)
The mux_4to1
module implements a 4-to-1 multiplexer with four 1-bit inputs (data_in[3:0]
) and two select signals (select[1:0]
). The data_out
output is 1-bit. The module uses a case statement to select one of the four input signals based on the select
input.
The behavior of the multiplexer is determined by the select
input. When select
is 2'b00
, the output is set to data_in[0]
, i.e., the first input. When select
is 2'b01
, the output is set to data_in[1]
, i.e., the second input, and so on. If select
has an invalid value, the default output is set to 1’b0. The always @(*)
block ensures that the output data_out
is updated whenever any of the inputs change.
Question:
Explain the purpose of the always
block in Verilog and the difference between always @(*)
and always @(posedge clk)
.
Answer:
The always
block in Verilog is used to define combinational or sequential logic. It is the fundamental construct used to describe how signals and variables are updated in response to changes in the sensitivity list (the part inside the parenthesis).
always @(*)
: This is a combinational always block, also known as a sensitivity list or sensitivity to all. The block executes whenever any signal inside the block changes. It is commonly used for combinational logic, where the output depends only on the current values of inputs and not on the past values.
Example:
always @(*)
begin
sum = a + b;
product = a * b;
end
always @(posedge clk)
: This is a sequential always block with a positive edge clock trigger. The block executes only when there is a positive edge (rising edge) on theclk
signal. It is used to model flip-flops or other sequential elements that change state only on clock edges.
Example:
always @(posedge clk)
begin
if (reset) // Reset the counter if 'reset' signal is asserted
counter <= 4'b0;
else
counter <= counter + 1; // Increment the counter on every clock edge
end
Code language: PHP (php)
The main difference between the two always blocks lies in their behavior:
always @(*)
executes whenever any signal inside the block changes and represents combinational logic.always @(posedge clk)
executes only on the positive edge of theclk
signal and is used for sequential logic elements like flip-flops.
Question:
Explain the concept of parameterized modules in Verilog and provide an example.
Answer:
Parameterized modules in Verilog allow developers to create reusable modules with customizable properties. Parameters are constants that can be passed as arguments when instantiating a module. This flexibility allows the same module to be used for different configurations without modifying its code.
Example:
module adder #(
parameter WIDTH = 8
) (
input [WIDTH-1:0] a
,
input [WIDTH-1:0] b,
output [WIDTH-1:0] sum
);
assign sum = a + b;
endmodule
Code language: PHP (php)
In this example, we define a parameterized module called adder
. The module takes a parameter WIDTH
, which represents the bit width of the inputs and output. Inside the module, the sum
output is calculated as the sum of a
and b
, both having a bit width of WIDTH
.
When instantiating the adder
module, you can specify the WIDTH
parameter based on your requirement:
module testbench;
// Instantiate the adder module with WIDTH = 16
adder #(WIDTH=16) adder_instance1 (
.a(a_input),
.b(b_input),
.sum(sum_output)
);
// Instantiate the adder module with WIDTH = 32
adder #(WIDTH=32) adder_instance2 (
.a(a_input),
.b(b_input),
.sum(sum_output)
);
endmodule
Code language: PHP (php)
By using parameterized modules, you can create adders with different bit widths, reusing the same module code for various configurations.
Question:
What is the purpose of the case
statement in Verilog, and how is it used? Provide an example.
Answer:
The case
statement in Verilog is used to implement multi-way decisions or conditional logic. It allows developers to describe multiple possible conditions and their corresponding actions in a concise and organized manner.
Example:
module traffic_light (
input [1:0] state,
output reg [2:0] signal
);
always @(*)
begin
case (state)
2'b00: signal = 3'b001; // Traffic light is green
2'b01: signal = 3'b010; // Traffic light is yellow
2'b10: signal = 3'b100; // Traffic light is red
default: signal = 3'b000; // Invalid state, turn off all signals
endcase
end
endmodule
Code language: PHP (php)
In this example, the traffic_light
module takes a two-bit state
input, representing different traffic light states. Based on the value of state
, the module sets the three-bit signal
output to indicate the corresponding traffic light color. When state
is 2'b00
, the output signal
is 3'b001
, indicating a green traffic light. When state
is 2'b01
, the output signal
is 3'b010
, indicating a yellow traffic light. Similarly, when state
is 2'b10
, the output signal
is 3'b100
, indicating a red traffic light. If an invalid state
is given, the default
branch sets the output signal
to 3'b000
, turning off all signals.
Question:
Explain the concept of a finite state machine (FSM) in Verilog and its significance in digital circuit design. Provide a simple example.
Answer:
A finite state machine (FSM) in Verilog is a model of a sequential digital circuit where the system can exist in a finite number of states, and the transition between states is controlled by a set of inputs. FSMs are widely used to describe complex control logic, protocols, and sequential processes in digital circuit design.
Example: A simple Verilog implementation of a 2-state FSM to debounce a push-button signal.
module debounce_fsm (
input clk,
input reset,
input button,
output reg debounced_button
);
// Define states using an enumeration
typedef enum logic [1:0] {
IDLE,
PRESSED
} state_t;
// Declare the current state variable
reg state_t current_state, next_state;
// FSM state transitions and outputs
always @(posedge clk or posedge reset)
begin
if (reset)
current_state <= IDLE;
else
current_state <= next_state;
end
always @(posedge clk)
begin
// Default next state is current state
next_state = current_state;
// State transitions and outputs
case (current_state)
IDLE:
if (!button)
next_state = PRESSED;
PRESSED:
if (button)
next_state = IDLE;
endcase
end
// Debounce the button signal in PRESSED state
always @(posedge clk)
begin
case (current_state)
PRESSED:
debounced_button <= 1;
default:
debounced_button <= 0;
endcase
end
endmodule
Code language: JavaScript (javascript)
In this example, we implement an FSM to debounce a push-button signal. The FSM has two states, IDLE
and PRESSED
. When the button is pressed, the FSM transitions from IDLE
to PRESSED
state. When the button is released, the FSM transitions back from PRESSED
to IDLE
state.
The debouncing of the button signal occurs in the PRESSED
state. While in the PRESSED
state, the output debounced_button
is set to 1
, indicating a stable button press. In other states, the output is set to 0
.
Question:
Explain the purpose of the generate
construct in Verilog and provide an example of its usage.
Answer:
The generate
construct in Verilog is used to create hardware structures or modules dynamically during elaboration (compilation) time. It allows developers to conditionally instantiate modules or generate repetitive structures based on parameters or conditions.
Example: Using the generate
construct to create a parameterized array of registers.
module register_array #(
parameter NUM_REGISTERS = 8,
parameter WIDTH = 8
) (
input [WIDTH-1:0] data_in,
output [WIDTH-1:0] data_out
);
generate
genvar i;
for (i = 0; i < NUM_REGISTERS; i = i + 1)
begin : reg_loop
reg [WIDTH-1:0] reg_data;
always @(posedge clk)
if (reset)
reg_data <= 0;
else
reg_data <= data_in;
assign data_out = reg_data;
end
endgenerate
endmodule
Code language: PHP (php)
In this example, the register_array
module generates an array of registers based on the parameters NUM_REGISTERS
and WIDTH
. Inside the generate
block, the for
loop iterates from 0
to NUM_REGISTERS - 1
, creating a separate register for each iteration.
The registers are assigned the input data_in
value on the positive edge of the clk
signal, except during a reset condition (reset
is asserted), where all registers are cleared to zero. The output data_out
is then connected to the corresponding register’s data.
By using the generate
construct, developers can easily create parameterized and scalable hardware structures.
Question:
Explain the concept of clock domain crossing (CDC) in Verilog and the challenges associated with it. Provide an example of how to handle CDC.
Answer:
Clock domain crossing (CDC) in Verilog refers to the process of transferring data between different clock domains. In digital circuits, different components or modules may operate at different clock frequencies or be asynchronous to each other. Transferring data between such clock domains requires special handling to ensure reliable and glitch-free operation.
The challenges associated with CDC include:
- Metastability: When a signal crosses from one clock domain to another, it may be sampled at an uncertain time, leading to metastability. Metastability can cause unpredictable results or even circuit failure.
- Data Synchronization: Data arriving at different frequencies needs to be synchronized to avoid data loss or incorrect values.
Example: A simple Verilog synchronizer to handle CDC between two clock domains.
module synchronizer (
input clk1, clk2,
input [DATA_WIDTH-1:0] data_in,
output reg [DATA_WIDTH-1:0] data_out
);
reg [DATA_WIDTH-1:0] sync_reg;
always @(posedge clk1)
sync_reg <= data_in;
always @(posedge clk2)
data_out <= sync_reg;
endmodule
Code language: CSS (css)
In this example, we implement a basic synchronizer module to transfer data between clk1
and clk2
clock domains. The input data_in
is sampled on the positive edge of clk1
and stored in sync_reg
. On the positive edge of clk2
, the data is transferred from sync_reg
to the output data_out
.
Using a synchronizer like this helps in avoiding metastability and ensures proper data transfer between different clock domains. However, it is essential to consider the setup and hold time requirements to ensure reliable operation and minimize the risk of metastability. More advanced CDC techniques, such as FIFOs or handshake protocols, may be required in complex designs to handle data crossing multiple clock domains.
Intermediate Verilog interview questions
Question:
Explain the purpose of a Verilog testbench and its role in the hardware design process.
Answer:
A Verilog testbench is a separate Verilog code that is used to simulate and verify the functionality of a hardware design. Its main purpose is to apply stimulus (input) to the design and observe the responses (output) to check if the design behaves correctly according to the specified requirements.
The testbench plays a crucial role in the hardware design process as it enables the designer to validate the correctness of the design before its actual implementation in hardware. By running simulations with different test scenarios, the testbench helps identify and debug potential issues, ensuring that the final hardware design meets the desired functionality.
Question:
The following Verilog code is intended to implement a simple 2-to-1 multiplexer. However, it contains a syntax error and does not compile. Identify the error and fix the code.
module mux_2to1 (input a, b, sel, output y);
assign y = sel ? a : b;
endmodule
Code language: JavaScript (javascript)
Answer:
The syntax error in the code is that the input ports are not declared with proper data types. The correct code is as follows:
module mux_2to1 (input wire a, b, input wire sel, output reg y);
always @(sel, a, b)
if (sel)
y = a;
else
y = b;
endmodule
Code language: JavaScript (javascript)
In this corrected code, the input ports a
, b
, and sel
are declared as input wire
, and the output port y
is declared as output reg
. The always
block is used to describe the behavior of the multiplexer based on the value of sel
.
Question:
Explain the difference between blocking and non-blocking assignments in Verilog.
Answer:
In Verilog, blocking and non-blocking assignments are used to describe how values are assigned to variables within an always
block.
Blocking assignments (=
) are evaluated sequentially in the order they appear in the always
block. The right-hand side (RHS) expression is evaluated immediately, and the assigned value is immediately updated in the left-hand side (LHS) variable. This means that subsequent assignments in the same always
block will see the updated value.
Non-blocking assignments (<=
), on the other hand, are evaluated concurrently or in parallel for all assignments within the same time step of the always
block. The RHS expressions are evaluated for all non-blocking assignments before updating the LHS variables simultaneously. This models the behavior of hardware registers and is typically used to describe synchronous behavior.
Here’s an example to illustrate the difference:
module blocking_nonblocking_example (input clk, reset, input data, output reg q);
always @(posedge clk or posedge reset)
if (reset)
q <= 0;
else
q = data;
endmodule
Code language: JavaScript (javascript)
In this example, q = data;
is a blocking assignment, and q <= 0;
is a non-blocking assignment. When the clock (clk
) rises, the non-blocking assignment (q <= 0;
) will update the value of q
concurrently with the blocking assignment (q = data;
).
Question:
The following Verilog code is intended to create a 4-bit counter that counts up on the rising edge of the clock. However, it contains a logical error and does not work as expected. Identify the error and fix the code.
module counter_4bit (input clk, input rst, output reg[3:0] count);
always @(posedge clk, posedge rst)
if (rst)
count <= 0;
else
count <= count + 1;
endmodule
Code language: JavaScript (javascript)
Answer:
The logical error in the code is the misuse of the sensitivity list in the always
block. The correct code is as follows:
module counter_4bit (input clk, input rst, output reg[3:0] count);
always @(posedge clk, posedge rst)
if (rst)
count <= 0;
else if (clk)
count <= count + 1;
endmodule
Code language: JavaScript (javascript)
In this corrected code, we use an else if (clk)
condition to ensure that the counter increments only on the rising edge of the clock (clk
). The use of else if
correctly separates the two actions (reset and increment) based on different triggering events.
Question:
Explain the concept of a finite-state machine (FSM) and its importance in digital circuit design.
Answer:
A finite-state machine (FSM) is a computational model used in digital circuit design and other sequential logic applications. It is a mathematical abstraction that consists of a set of states, an initial state, a set of inputs, a set of outputs, and a set of transition rules that determine the behavior of the machine.
FSMs are essential in digital circuit design because they allow designers to model and implement sequential logic behavior in hardware. By specifying the states, inputs, outputs, and transition rules, designers can create hardware circuits that can respond to different input sequences and perform specific tasks. FSMs are used in various digital design applications, such as control units, data path components, and communication protocols.
Question:
The following Verilog code is intended to implement a 2-bit binary counter with synchronous reset functionality. However, it contains a logical error and does not work as expected. Identify the error and fix the code.
module binary_counter_2bit (input clk, input rst, output reg[1:0] count);
always @(posedge clk)
if (rst)
count <= 0;
else
count <= count + 1;
endmodule
Code language: JavaScript (javascript)
Answer:
The logical error in the code is that the reset condition is not properly synchronized with the clock edge. The correct code is as follows:
module binary_counter_2bit (input clk, input rst, output reg[1:0] count);
always @(posedge clk)
if (rst)
count <= 2'b00;
else
count <= count + 1;
endmodule
In this corrected code, the reset condition (rst
) is synchronized with the clock edge (posedge clk
) to ensure that the counter is reset synchronously with the clock. When rst
is asserted, the counter is set to 2'b00
, representing a 2-bit binary value of 0.
Question:
Explain the concept of event-driven simulation in Verilog and its advantages in modeling digital circuits.
Answer:
Event-driven simulation is a technique used in Verilog to model and simulate digital circuits efficiently. In event-driven simulation, the simulator processes events or changes that occur in the circuit, rather than executing the entire code in a continuous loop.
Advantages of event-driven simulation in modeling digital circuits include:
- Efficiency: Event-driven simulation focuses on changes in signals, making it more efficient than continuously re-executing the code. It avoids redundant calculations and improves simulation speed.
- Modularity: It allows designers to model individual components and their interactions separately. Each component responds to events, which promotes modularity and ease of debugging.
- Parallelism: Event-driven simulation allows parallel execution of different blocks of code responding to different events. This makes it well-suited for parallel hardware behavior representation.
- Accurate timing: Event-driven simulation inherently captures signal changes and delays accurately, making it suitable for accurate timing analysis and verification.
Question:
The following Verilog code is intended to implement a 3-input AND gate. However, it contains a syntax error and does not compile. Identify the error and fix the code.
module and_gate_3input (input a, b, c, output y);
assign y = a && b && c;
endmodule
Code language: JavaScript (javascript)
Answer:
The syntax error in the code is the incorrect usage of the &&
operator. The correct code is as follows:
module and_gate_3input (input a, b, c, output y);
assign y = a & b & c;
endmodule
Code language: JavaScript (javascript)
In this corrected code, the &
operator is used to perform the logical AND operation on inputs a
, b
, and c
.
Question:
Explain the concept of RTL (Register-Transfer Level) design in Verilog and its importance in hardware design.
Answer:
RTL (Register-Transfer Level) design in Verilog is a level of abstraction that describes a digital hardware design in terms of registers and the transfer of data between registers. It specifies how data is processed and transferred between registers in a sequential manner, capturing the behavior of the hardware at the register level.
RTL design is essential in hardware design because it allows designers to model complex digital systems efficiently and concisely. By describing the design in terms of registers and data transfers, RTL enables designers to focus on the functionality of the design rather than its implementation details. This abstraction level serves as a bridge between the high-level behavioral description of the design and the low-level gate-level implementation.
RTL descriptions are widely used for simulation, verification, and synthesis of digital circuits. They provide a clear representation of the design’s functionality, making it easier to understand, verify, and modify complex hardware systems.
Question:
The following Verilog code is intended to implement a 2-input OR gate. However, it contains a logical error and does not produce the correct output. Identify the error and fix the code.
module or_gate_2input (input a, b, output y);
assign y = a & b;
endmodule
Code language: JavaScript (javascript)
Answer:
The logical error in the code is the incorrect usage of the &
operator, which performs a bitwise AND operation. The correct code is as follows:
module or_gate_2input (input a, b, output y);
assign y = a | b;
endmodule
Code language: JavaScript (javascript)
In this corrected code, the |
operator is used to perform the logical OR operation on inputs a
and b
, producing the correct output for the 2-input OR gate.
Senior Verilog interview questions
Question:
Explain the concept of a finite-state machine (FSM) in Verilog and provide an example of its practical application.
Answer:
In Verilog, a finite-state machine (FSM) is a digital circuit that can be in one of a finite number of states at any given time. The FSM transitions from one state to another based on its inputs and current state, following a predefined set of rules or state transition table. FSMs are commonly used to design sequential circuits and control units in digital systems.
Practical Application Example: One practical application of an FSM in Verilog is designing a traffic light controller. The FSM can have states like “Red,” “Yellow,” and “Green,” and it transitions between these states based on timing requirements and input signals from sensors and timers.
Question:
The following Verilog code is intended to implement a 4-to-1 multiplexer. However, it contains a syntax error and doesn’t work as expected. Identify the error and fix the code.
module mux_4to1(output reg out, input [3:0] data, input [1:0] sel);
always @(sel)
case(sel)
2'b00: out = data[0];
2'b01: out = data[1];
2'b10: out = data[2];
2'b11: out = data[3];
endcase
endmodule
Code language: PHP (php)
Answer:
The syntax error in the code is the incorrect placement of semicolons in the case statement. The correct code is as follows:
module mux_4to1(output reg out, input [3:0] data, input [1:0] sel);
always @(sel)
case(sel)
2'b00: out = data[0];
2'b01: out = data[1];
2'b10: out = data[2];
2'b11: out = data[3];
endcase
endmodule
Code language: PHP (php)
In this corrected code, the semicolons are removed, and the 4-to-1 multiplexer is implemented correctly.
Question:
Explain the concept of blocking and non-blocking assignments in Verilog and when to use each.
Answer:
In Verilog, blocking assignments (=
) and non-blocking assignments (<=
) are used to update the values of variables within always blocks.
Blocking Assignment (=
): A blocking assignment is used for combinational logic. It means that the assignment on the left side is executed immediately, and the next statement in the always block will wait for the assignment to complete before proceeding. It is suitable for describing combinational logic, such as conditional statements or assignments.
Non-blocking Assignment (<=
): A non-blocking assignment is used for sequential logic, especially for modeling flip-flops and state machines. It means that the assignment on the left side is scheduled to occur after all the other blocking assignments in the always block. The non-blocking assignment allows multiple updates to happen concurrently in a simulation time step, similar to how flip-flops work in hardware.
In summary, use blocking assignments for combinational logic and non-blocking assignments for sequential logic and flip-flop updates in Verilog.
Question:
The following Verilog code is intended to implement a 3-bit up-counter. However, it contains a logical error and doesn’t produce the correct count sequence. Identify the error and fix the code.
module counter_3bit(output reg [2:0] count, input clk, input reset);
always @(posedge clk, posedge reset)
if (reset)
count <= 3'b0;
else
count <= count + 1;
endmodule
Answer:
The logical error in the code is the incorrect sensitivity list in the always block. The reset condition should be checked separately from the clock edge. The correct code is as follows:
module counter_3bit(output reg [2:0] count, input clk, input reset);
always @(posedge clk)
if (reset)
count <= 3'b0;
else
count <= count + 1;
endmodule
In this corrected code, the reset condition is checked only on the positive edge of the clock, and the 3-bit up-counter works as expected.
Question:
What is RTL (Register Transfer Level) design in Verilog, and why is it important in hardware description?
Answer:
RTL (Register Transfer Level) design in Verilog is a high-level abstraction used to describe the behavior and interconnections of digital systems in terms of registers and the transfer of data between them. It represents the data flow and control flow of a digital system, making it easier to design and verify complex hardware structures.
Importance of RTL Design: RTL design is crucial in hardware description because it bridges the gap between the high-level functional specification and the low-level gate-level description. It allows designers to focus on the functionality and behavior of the digital system without getting into the details of gate-level implementation. RTL code is also synthesizable, meaning it can be synthesized into hardware gates and implemented on an FPGA or ASIC.
By designing at the RTL level, hardware designers can perform functional verification, optimization, and synthesis to generate efficient hardware implementations while maintaining portability and ease of design modification.
Question:
The following Verilog code is intended to implement a 2-input AND gate using assign
statements. However, it contains a syntax error and doesn’t work as expected. Identify the error and fix the code.
module and_gate(output reg out, input a, input b);
assign out reg = a & b;
endmodule
Code language: JavaScript (javascript)
Answer:
The syntax error in the code is the incorrect usage of the assign
statement. The correct code is as follows:
module and_gate(output reg out, input a, input b);
assign out = a & b;
endmodule
Code language: JavaScript (javascript)
In this corrected code, the assign
statement is used correctly to implement the 2-input AND gate.
Question:
Explain the concept of pipelining in Verilog and its advantages in hardware design.
Answer:
Pipelining in Verilog is a technique used to improve the throughput and performance of a digital system by breaking down complex tasks into a series of smaller stages (pipelines). Each stage performs a part of the computation, and the results are passed from one stage to another. Pipelining introduces a delay between input and output but increases the overall throughput of the system.
Advantages of Pipelining:
- Increased Throughput: Pipelining allows multiple tasks to be processed simultaneously, improving the overall system throughput. It enables more operations to be performed in a given time frame.
- Reduced Critical Path: By dividing tasks into smaller stages, the critical path (longest delay) of the circuit is reduced, enabling the system to operate at higher clock frequencies.
- Resource Sharing: Pipelining enables resource sharing between pipeline stages. As each stage works on a specific part of the computation, the same hardware can be used repeatedly for different tasks, reducing area and power consumption.
- Parallelism: Pipelining introduces a form of parallelism in hardware design. Different pipeline stages can work on different inputs simultaneously, leading to improved overall performance.
Overall, pipelining is a powerful technique for optimizing the performance of digital systems and is commonly used in processors, graphics pipelines, and communication systems.
Question:
The following Verilog code is intended to implement a 2-to-4 decoder using case statements. However, it contains a logical error and doesn’t produce the correct output. Identify the error and fix the code.
module decoder_2to4(output reg [3:0] dec_out, input [1:0] select);
always @(*)
case (select)
2'b00: dec_out = 4'b0001;
2'b01: dec_out = 4'b0010;
2'b10: dec_out = 4'b0100;
2'b11: dec_out = 4'b1000;
endcase
endmodule
Code language: JavaScript (javascript)
Answer:
The logical error in the code is the missing default assignment in the case statement. The correct code is as follows:
module decoder_2to4(output reg [3:0] dec_out, input [1:0] select);
always @(*)
case (select)
2'b00: dec_out = 4'b0001;
2'b01: dec_out = 4'b0010;
2'b10: dec_out = 4'b0100;
2'b11: dec_out = 4'b1000;
default: dec_out = 4'b0000;
endcase
endmodule
Code language: PHP (php)
In this corrected code, the default
case is added to handle any other input values not covered by the previous cases, and the 2-to-4 decoder works as expected.
Question:
Explain the concept of Verilog testbenches and their role in the verification process.
Answer:
In Verilog, a testbench is a separate module designed to verify the correctness of a design or module under different test scenarios. It provides stimulus (input) to the design and checks the resulting outputs against expected values. Testbenches are an essential part of the verification process in hardware design.
Role of Testbenches:
- Functional Verification: Testbenches help ensure that the design functions correctly as per the specified requirements and functionality. They apply a variety of test cases to validate that the design meets its intended purpose.
- Coverage Analysis: Testbenches are used to track the coverage of the design’s functionality. They help identify which parts of the design have been exercised by the test cases and which parts need additional testing.
- Debugging: Testbenches aid in identifying and diagnosing issues in the design. By comparing the actual outputs to expected outputs, they can help pinpoint errors and aid in debugging the design.
- Performance Evaluation: Testbenches allow designers to evaluate the performance of the design under various conditions, ensuring that it meets timing and other performance requirements.
- Regression Testing: Testbenches are reusable, making it easy to perform regression testing when the design or its specifications change. They help ensure that the design still behaves correctly after modifications.
In summary, Verilog testbenches play a crucial role in the verification process, helping designers validate the functionality, performance, and correctness of their hardware designs.
Question:
The following Verilog code is intended to implement a 4-bit binary adder. However, it contains a syntax error and doesn’t work as expected. Identify the error and fix the code.
module binary_adder(output reg [3:0] sum, input [3:0] a, input [3:0] b);
always @(*)
sum[3:0] = a[3:0] + b[3:0];
endmodule
Code language: CSS (css)
Answer:
The syntax error in the code is the incorrect usage of the reg
keyword with output
in the module declaration. The correct code is as follows:
module binary_adder(output [3:0] sum, input [3:0] a, input [3:0] b);
always @(*)
sum[3:0] = a[3:0] + b[3:0];
endmodule
Code language: CSS (css)
In this corrected code, the reg
keyword is removed from the module declaration, and the 4-bit binary adder works as expected.
1,000 Companies use CoderPad to Screen and Interview Developers
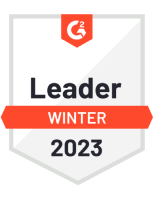
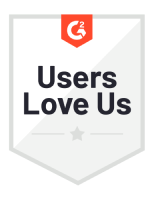
Interview best practices for Verilog roles
For effective Verilog interviews, it’s crucial to take into account multiple aspects, including the applicants experience levels and the engineering position they’re interviewing for. To guarantee that your Verilog interview inquiries produce optimal outcomes, we suggest adhering to the following best practices when engaging with candidates:
- Create technical questions that align with actual business cases within your organization. This will not only be more engaging for the candidate but also enable you to better assess the candidate’s suitability for your team.
- Encourage the candidate to ask questions during the interview and foster a collaborative environment.
- Make sure your candidates can also demonstrate proficiency in defining and examining timing constraints to guarantee that the design satisfies the necessary performance criteria.
Moreover, it is essential to follow conventional interview practices when carrying out Verilog interviews. This encompasses tailoring the question difficulty according to the applicant’s development skill level, offering prompt feedback regarding their application status, and permitting candidates to inquire about the evaluation or collaborating with you and your team.