Swift Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Swift developers for your organization.
Swift
The preferred language for macOS/iOS applications, Swift is also popular for its readability & simplicity, its fast and efficient performance, and its strong corporate backing by Apple.
In December 2015, Apple open-sourced Swift under the Apache License 2.0, allowing the broader developer community to contribute to its development and improvement. The Swift project is now available on GitHub, with ongoing enhancements and updates driven by both Apple and the community.
https://swift.org/blog/swift-is-now-open-source/
Our team has developed practical programming assignments and conversation prompts, tailored to measure the Swift proficiency of developers during coding assessments. Furthermore, we have compiled a set of best practices to ensure the accuracy of your interview questions in evaluating candidates’ skills in Swift.
Table of Contents
Swift example question
Help us design a parking lot
Hey candidate! Welcome to your interview. Boilerplate is provided. Feel free to change the code as you see fit. To run the code at any time, please hit the run button located in the top left corner.
Goals: Design a parking lot using object-oriented principles
Here are a few methods that you should be able to run:
- Tell us how many spots are remaining
- Tell us how many total spots are in the parking lot
- Tell us when the parking lot is full
- Tell us when the parking lot is empty
- Tell us when certain spots are full e.g. when all motorcycle spots are taken
- Tell us how many spots vans are taking up
Assumptions:
- The parking lot can hold motorcycles, cars and vans
- The parking lot has motorcycle spots, car spots and large spots
- A motorcycle can park in any spot
- A car can park in a single compact spot, or a regular spot
- A van can park, but it will take up 3 regular spots
- These are just a few assumptions. Feel free to ask your interviewer about more assumptions as needed
Swift skills to assess
Jobs using Swift
Junior Swift interview questions
Question:
Explain the concept of optionals in Swift and why they are important in the language. Provide an example of using an optional variable in Swift.
Answer:
Optionals in Swift are a fundamental feature that allows variables to either contain a value or be nil (no value). Optionals are essential in Swift to handle situations where a variable might not have a value, such as when reading data from a file or when a value is not available yet.
Here’s an example of using an optional variable in Swift:
var age: Int? = 25
// Using optional binding to check if the optional has a value before unwrapping
if let unwrappedAge = age {
print("The age is (unwrappedAge)")
} else {
print("Age is not available.")
}
Code language: PHP (php)
In this example, age
is an optional variable that can hold an integer value or be nil. We use optional binding with the if let
construct to safely unwrap the optional and check if it contains a value. If age
has a value, it is unwrapped and assigned to the constant unwrappedAge
, and we can use it safely within the scope of the if
block. If age
is nil, the else
block will be executed.
Question:
What is the difference between a class and a struct in Swift? When would you choose to use one over the other? Provide an example of a scenario where using a class is more appropriate than using a struct, and vice versa.
Answer:
In Swift, both classes and structs are used to define custom data types, but they have some key differences:
- Classes:
- Reference Types: Instances of classes are reference types, meaning they are passed by reference and multiple variables can refer to the same instance in memory.
- Inheritance: Classes support inheritance, allowing one class to inherit properties and methods from another class.
- Deinitializers: Classes can have deinitializers, which are code blocks executed when an instance is deallocated.
- Reference Counting: Classes use reference counting to manage memory, automatically releasing memory when no references to an instance exist.
2. Structs:
- Value Types: Instances of structs are value types, meaning they are copied when assigned to a new variable or passed to a function.
- No Inheritance: Structs do not support inheritance, so they cannot inherit properties or methods from other types.
- No Deinitializers: Structs do not have deinitializers since they don’t manage resources that need cleanup.
- No Reference Counting: Structs do not use reference counting; their memory management is handled automatically.
When to use classes over structs and vice versa depends on the situation:
- Use Classes: When you need reference semantics, inheritance, or when you want to manage shared mutable state. For example, classes are suitable for defining objects like a person, where instances may have shared properties and methods.
- Use Structs: When you need value semantics, immutability, or when the instance is relatively simple and does not require inheritance. For example, structs are suitable for defining simple data types like a point or a date, where instances are used for data representation.
Question:
Explain the concept of option chaining in Swift. How does it help in safely working with optionals? Provide an example of option chaining in Swift.
Answer:
Option chaining in Swift is a concise and safe way to work with optionals and their properties or methods. It allows you to access properties and call methods on an optional variable only if the variable has a value (i.e., it is not nil). If the optional is nil, the chain of operations short-circuits and returns nil without causing a runtime error.
Here’s an example of using option chaining in Swift:
struct Person {
var name: String?
var age: Int?
}
let john = Person(name: "John", age: 30)
let mary = Person(name: "Mary", age: nil)
// Using optional chaining to safely access the name property
if let johnName = john.name {
print("John's name is (johnName)")
} else {
print("John's name is not available.")
}
if let maryName = mary.name {
print("Mary's name is (maryName)")
} else {
print("Mary's name is not available.")
}
Code language: JavaScript (javascript)
In this example, the Person
struct has two optional properties: name
and age
. We use optional chaining to safely access the name
property. If the name
property is nil, the optional chaining will short-circuit, and the code in the else
block will be executed.
Question:
What is a guard statement in Swift, and how is it different from using an if-else statement? Provide an example of using a guard statement in Swift.
Answer:
A guard statement in Swift is used to check for a condition that must be true to continue executing the code. If the condition is false, the guard statement requires an early exit from the current scope using the return
, break
, continue
, or throw
keywords. Guard statements are particularly useful for reducing nesting levels and improving code readability.
Here’s an example of using a guard statement in Swift:
func divide(_ dividend: Int, by divisor: Int) -> Int? {
guard divisor != 0 else {
print("Error: Division by zero is not allowed.")
return nil
}
return dividend / divisor
}
if let result = divide(10, by: 2) {
print("Result: (result)")
}
if let result = divide(15, by: 0) {
print("Result: (result)")
}
Code language: PHP (php)
In this example, the divide(_:by:)
function uses a guard statement to check if the divisor
is not zero. If the divisor is zero, the guard statement will print an error message and return nil
, preventing the division by zero. Otherwise, the function proceeds to perform the division and returns the result.
Question:
Explain the concept of Swift’s Automatic Reference Counting (ARC). How does ARC help manage memory in Swift? Provide an example of how ARC works in practice.
Answer:
Automatic Reference Counting (ARC) is a memory management mechanism in Swift that automatically tracks and manages the memory used by class instances. ARC ensures that instances are deallocated from memory when they are no longer needed, preventing memory leaks.
ARC works by keeping track of how many references (strong references) point to an instance. Whenever a new reference is created, ARC increments the reference count. When a reference goes out of scope or is set to nil, ARC decrements the reference count. When the reference count reaches zero, meaning no strong references point to the instance, ARC automatically deallocates the memory used by the instance.
Here’s an example of ARC in practice:
class Person {
var name: String
init(name: String) {
self.name = name
print("(name) is initialized.")
}
deinit {
print("(name) is deinitialized.")
}
}
var person1: Person? = Person(name: "John")
var person2: Person? = person1
person1 = nil
person2 = nil
Code language: PHP (php)
In this example, we define a Person
class with a name
property. The deinit
deinitializer is used to print a message when an instance of Person
is deallocated.
We create two optional references, person1
and person2
, and both point to the same Person
instance. When we set person1
and person2
to nil
, both references go out of scope, and their reference count decreases. Since there are no strong references pointing to the Person
instance anymore, it is deallocated, and the deinit
message is printed, indicating successful memory management by ARC.
Question:
Explain the concept of closures in Swift and why they are useful in the language. Provide an example of using a closure in Swift.
Answer:
Closures in Swift are self-contained blocks of functionality that can be assigned to variables, passed as arguments to functions, and returned from functions. Closures capture the surrounding context, including variables and constants, making them powerful and flexible.
Closures are useful in Swift for tasks like:
- Simplifying syntax: Closures allow for concise syntax when defining inline functions.
- Asynchronous operations: Closures are commonly used to handle asynchronous callbacks in Swift.
- Sorting: Closures are useful for defining custom sorting logic for collections.
- Higher-order functions: Many higher-order functions in Swift, like
map
,filter
, andreduce
, take closures as arguments.
Here’s an example of using a closure in Swift:
let numbers = [10, 5, 8, 3, 12]
// Sorting the array in ascending order using a closure
let sortedAscending = numbers.sorted { $0 < $1 }
print("Ascending order: (sortedAscending)")
// Sorting the array in descending order using a closure
let sortedDescending = numbers.sorted { $0 > $1 }
print("Descending order: (sortedDescending)")
// Using the map function to double each element in the array using a closure
let doubledNumbers = numbers.map { $0 * 2 }
print("Doubled numbers: (doubledNumbers)")
Code language: JavaScript (javascript)
In this example, we use closures to define custom sorting logic and to double each element in the array using the sorted
and map
functions, respectively.
Question:
What are option sets in Swift, and how do they provide a more efficient way to represent sets of related options? Provide an example of using an option set in Swift.
Answer:
Option sets in Swift are used to represent sets of related options as bitmasks. They provide an efficient and concise way to work with sets of distinct options where each option corresponds to a single bit in the underlying binary representation. This binary representation allows for fast bitwise operations to combine or check the presence of multiple options.
To define an option set, we create an enumeration that conforms to the OptionSet
protocol. The individual cases of the enumeration are assigned consecutive power-of-two values using bit shifting.
Here’s an example of using an option set in Swift:
struct Permissions: OptionSet {
let rawValue: Int
static let read = Permissions(rawValue: 1 << 0) // 1
static let write = Permissions(rawValue: 1 << 1) // 2
static let execute = Permissions(rawValue: 1 << 2) // 4
}
var myPermissions: Permissions = [.read, .write]
if myPermissions.contains(.read) {
print("Read permission is granted.")
}
if myPermissions.contains(.write) {
print("Write permission is granted.")
}
if myPermissions.contains(.execute) {
print("Execute permission is granted.")
} else {
print("Execute permission is not granted.")
}
Code language: JavaScript (javascript)
In this example, we define an OptionSet
called Permissions
with three cases: .read
, .write
, and .execute
. Each case is assigned a unique power-of-two value. We create an instance of Permissions
with read and write permissions and use the contains()
method to check if a specific permission is granted.
Question:
Explain the concept of generics in Swift and how they enhance code reusability and type safety. Provide an example of using generics in Swift.
Answer:
Generics in Swift allow you to write flexible and reusable functions and types that can work with a variety of data types. They enhance code reusability by enabling the creation of algorithms and data structures that work uniformly with any type, providing type safety without sacrificing performance.
Generics are particularly useful when you want to write functions or structures that can work with different data types while enforcing type constraints. They allow you to write code that is more abstract, efficient, and less prone to type-related bugs.
Here’s an example of using generics in Swift:
func swapValues<T>(_ a: inout T, _ b: inout T) {
let temp = a
a = b
b = temp
}
var x = 5
var y = 10
swapValues(&x, &y)
print("After swapping: x = (x), y = (y)")
var name1 = "Alice"
var name2 = "Bob"
swapValues(&name1, &name2)
print("After swapping: name1 = (name1), name2 = (name2)")
Code language: PHP (php)
In this example, we define a generic function swapValues(_:_:)
that can swap the values of two variables of any type T
. The function uses the inout
keyword to modify the original variables directly. We can call the swapValues
function with different types (Int and String) while ensuring type safety and code reusability.
Intermediate Swift interview questions
Question:
Explain Optionals in Swift and why they are important in handling nil values. Provide an example of using Optionals in Swift.
Answer:
Optionals in Swift are a powerful feature that allows you to represent the presence or absence of a value. They are used to handle situations where a variable may or may not have a value, including cases where the value is nil
. Optionals help to prevent runtime crashes that can occur due to accessing nil
values and provide a safer way to handle potential absence of data.
Here’s an example of using Optionals in Swift:
// Optional String
var username: String?
username = "John"
// Use optional binding to safely unwrap the optional
if let name = username {
print("Hello, (name)!")
} else {
print("No username provided.")
}
Code language: JavaScript (javascript)
In this example, the username
variable is declared as an Optional String (String?
). We assign the value "John"
to the username
variable. We use optional binding with if let
to safely unwrap the optional and print the greeting message if the username
has a value. If the username
is nil
, the “No username provided.” message will be printed.
Question:
What are closures in Swift, and why are they useful? Provide an example of using a closure in Swift.
Answer:
Closures in Swift are self-contained blocks of code that can be assigned to variables, passed as arguments to functions, or returned from functions. They capture their surrounding context, making them powerful and flexible for tasks like callbacks, sorting, and encapsulating behavior.
Here’s an example of using a closure in Swift for sorting an array of integers in ascending order:
let numbers = [5, 2, 8, 1, 3]
let sortedNumbers = numbers.sorted { (a, b) -> Bool in
return a < b
}
print(sortedNumbers) // Output: [1, 2, 3, 5, 8]
Code language: JavaScript (javascript)
In this example, we use the sorted
method of the array and pass a closure as an argument. The closure compares two integers a
and b
and returns true
if a
is less than b
, indicating that a
should come before b
in the sorted array.
Question:
Explain the difference between class inheritance and protocol adoption in Swift. When should you use one over the other? Provide an example of both class inheritance and protocol adoption.
Answer:
In Swift, class inheritance and protocol adoption are two ways to achieve code reuse and abstraction.
Class inheritance allows a class to inherit properties and methods from another class. It establishes an “is-a” relationship, where a subclass is a specialized version of its superclass. It promotes code reuse and allows the subclass to extend or override the behavior of the superclass.
Example of class inheritance:
class Shape {
func area() -> Double {
return 0.0
}
}
class Circle: Shape {
var radius: Double
init(radius: Double) {
self.radius = radius
}
override func area() -> Double {
return Double.pi * radius * radius
}
}
let circle = Circle(radius: 5.0)
print(circle.area()) // Output: 78.53981633974483
Protocol adoption, on the other hand, allows a class, struct, or enum to conform to a protocol, providing a set of rules or behaviors it must implement. It establishes a “can-do” relationship, where a type can do something without requiring a shared ancestor.
Example of protocol adoption:
protocol Playable {
func play()
}
class Song: Playable {
func play() {
print("Now playing: Song")
}
}
class Video: Playable {
func play() {
print("Now playing: Video")
}
}
let song = Song()
let video = Video()
song.play() // Output: Now playing: Song
video.play() // Output: Now playing: Video
Use class inheritance when you have a clear hierarchical relationship between classes, and you want to share common properties and behaviors. Use protocol adoption when you want to define a contract that multiple types can adhere to, regardless of their class hierarchy. Swift allows classes to inherit from only one superclass, but they can adopt multiple protocols, providing more flexibility in organizing and reusing code.
Question:
Explain the concept of memory management in Swift. What are the key principles Swift employs to manage memory effectively?
Answer:
Memory management in Swift is the process of allocating and deallocating memory for objects and variables during the program’s execution. Swift employs Automatic Reference Counting (ARC) as its memory management mechanism, which automatically keeps track of how many references point to an object. When the reference count drops to zero, the object is deallocated, and its memory is released.
Key principles of memory management in Swift:
- Strong References: When an object has at least one strong reference pointing to it, ARC keeps the object alive. Once all strong references are removed, the object is deallocated.
- Weak References: Weak references do not increase the reference count. They are used to avoid retain cycles, where two objects strongly reference each other and prevent deallocation.
- Unowned References: Similar to weak references, unowned references do not increase the reference count. However, they assume that the reference will always have a valid value and won’t become
nil
during the object’s lifetime. - Reference Cycles: Circular strong references between objects can lead to memory leaks, where objects are not deallocated as expected. To break such cycles, you can use weak or unowned references.
- Automatic Reference Counting (ARC): Swift automatically inserts memory management code based on the reference counts, ensuring objects are deallocated when no longer in use.
Example of using weak reference to break a reference cycle:
class Person {
var name: String
weak var car: Car?
init(name: String) {
self.name = name
}
}
class Car {
let model: String
var owner: Person?
init(model: String) {
self.model = model
}
}
var john: Person? = Person(name: "John")
var bmw: Car? = Car(model: "BMW")
john?.car = bmw
bmw?.owner = john
john = nil // The Person instance is deallocated because the strong reference count becomes zero.
bmw = nil // The Car instance is deallocated because the weak reference count becomes zero.
Code language: JavaScript (javascript)
In this example, the Person
and Car
classes have a circular strong reference through their properties car
and owner
. By using a weak reference for the car
property, the reference cycle is broken, and both Person
and Car
instances can be deallocated properly.
Question:
What is the guard
statement in Swift, and how does it differ from if-let
? Provide an example of using both guard
and if-let
in Swift.
Answer:
The guard
statement in Swift is used to ensure that a condition is true, and if not, it will exit the current scope using the return
, throw
, break
, or continue
statement. guard
is typically used to validate inputs, early exit from a function if conditions are
not met, and reduce nested code blocks.
Example of using guard
:
func divide(_ numerator: Int, _ denominator: Int) -> Int? {
guard denominator != 0 else {
return nil // Exit the function early if denominator is zero.
}
return numerator / denominator
}
if let result = divide(10, 2) {
print("Result:", result) // Output: Result: 5
}
if let result = divide(8, 0) {
print("Result:", result)
} else {
print("Division by zero is not allowed.") // Output: Division by zero is not allowed.
}
Code language: PHP (php)
The if-let
construct is used to safely unwrap optionals. It checks if an optional has a value, and if it does, it assigns the unwrapped value to a new constant or variable within the scope of the if
block.
Example of using if-let
:
func greetUser(_ username: String?) {
if let name = username {
print("Hello, (name)!")
} else {
print("Hello, guest!")
}
}
greetUser("John") // Output: Hello, John!
greetUser(nil) // Output: Hello, guest!
Code language: JavaScript (javascript)
In this example, the greetUser
function uses if-let
to safely unwrap the username
optional. If the username
has a value, it is unwrapped and printed as part of the greeting message. If the username
is nil
, the “Hello, guest!” message is printed.
The key difference between guard
and if-let
is that guard
is used to ensure a condition is true and provides an early exit from the current scope, whereas if-let
is used to safely unwrap optionals and execute code based on the unwrapped value.
Question:
Explain Optionals in Swift and why they are essential in handling certain scenarios. Provide an example of using Optionals in Swift.
Answer:
Optionals in Swift are a way to represent the presence or absence of a value. They are essential in scenarios where a value may be missing, such as when dealing with data from user inputs or data parsing. Optionals help prevent runtime crashes that could occur if you try to access a value that doesn’t exist.
Here’s an example of using Optionals in Swift:
// Define an optional variable to store an integer value
var optionalValue: Int?
// Attempt to parse a user input as an integer
let userInput = "123"
if let intValue = Int(userInput) {
optionalValue = intValue
}
// Using optional binding to safely unwrap the optional
if let value = optionalValue {
print("The parsed value is: (value)")
} else {
print("Invalid input or nil value.")
}
Code language: JavaScript (javascript)
In this example, optionalValue
is an optional variable that may or may not hold an integer value. The Int()
constructor is used to attempt to parse the user input userInput
as an integer. If the parsing is successful, the value is assigned to optionalValue
. Later, the optional binding if let
is used to safely unwrap optionalValue
and print its contents if it exists, or print an error message if it is nil
.
Question:
Explain the concept of closures in Swift and why they are useful in asynchronous programming. Provide an example of using closures in Swift.
Answer:
Closures in Swift are self-contained blocks of code that can capture and store references to variables and constants from their surrounding context. They are similar to anonymous functions or lambda expressions in other programming languages. Closures are useful in asynchronous programming because they allow us to pass behavior as an argument to functions, making it easier to handle tasks that need to execute at a later time or on a different thread.
Here’s an example of using closures in Swift for asynchronous programming:
// Function to perform a task asynchronously and call a completion closure when done
func performAsyncTask(completion: @escaping (String) -> Void) {
DispatchQueue.global().async {
// Simulate a time-consuming task
sleep(2)
let result = "Task completed!"
DispatchQueue.main.async {
// Call the completion closure on the main thread
completion(result)
}
}
}
// Call the function with a closure to handle the result
performAsyncTask { result in
print(result)
}
Code language: JavaScript (javascript)
In this example, the function performAsyncTask(completion:)
takes a closure as an argument, which will be called when the asynchronous task is completed. Inside the function, a time-consuming task is simulated using DispatchQueue.global().async
. After the task is completed, the completion
closure is called on the main thread using DispatchQueue.main.async
, allowing UI updates or further processing to be safely executed on the main thread.
Question:
Explain the concept of protocols in Swift and why they are essential in building reusable and flexible code. Provide an example of using protocols in Swift.
Answer:
Protocols in Swift define a set of methods and properties that a class, struct, or enum must implement if it conforms to the protocol. They are essential in building reusable and flexible code because they allow for defining a common interface that multiple types can adopt. By conforming to a protocol, different types can provide their own implementation of the protocol requirements, enabling polymorphism and code abstraction.
Here’s an example of using protocols in Swift:
// Protocol defining the "Animal" interface
protocol Animal {
var name: String { get }
func makeSound()
}
// Struct conforming to the "Animal" protocol
struct Dog: Animal {
let name: String
func makeSound() {
print("(name) barks.")
}
}
// Struct conforming to the "Animal" protocol
struct Cat: Animal {
let name: String
func makeSound() {
print("(name) meows.")
}
}
// Function that takes an "Animal" type as a parameter
func animalSound(animal: Animal) {
animal.makeSound()
}
// Create instances of "Dog" and "Cat" and call the function
let dog = Dog(name: "Buddy")
let cat = Cat(name: "Whiskers")
animalSound(animal: dog) // Output: "Buddy barks."
animalSound(animal: cat) // Output: "Whiskers meows."
Code language: JavaScript (javascript)
In this example, we define a protocol named Animal
, which requires conforming types to have a name
property and a makeSound()
method. The Dog
and Cat
structs conform to the Animal
protocol by providing their own implementation of the required properties and methods. The function animalSound(animal:)
takes an Animal
type as a parameter, allowing it to work with any type that conforms to the Animal
protocol. This demonstrates how protocols enable code reusability and abstraction.
Question:
Explain the concept of memory management in Swift and how Swift uses Automatic Reference Counting (ARC) to manage memory. Provide an example to illustrate memory management with ARC.
Answer:
Memory management in Swift refers to the management of memory resources allocated to objects and data structures during program execution. Swift uses Automatic Reference Counting (ARC) to manage memory. ARC automatically tracks and manages the references to objects and deallocates objects when they are no longer referenced, ensuring that memory is efficiently utilized and preventing memory leaks.
Here’s an example to illustrate memory management with ARC:
class Person {
var name: String
init(name: String) {
self.name = name
print("(name) is initialized.")
}
deinit {
print("(name) is deallocated.")
}
}
func createPerson() {
let person1 = Person(name: "Alice")
let person2 = person1 // Strong reference to the same object
// The objects are still in memory since both variables hold strong references
}
createPerson() // Output: "Alice is initialized." "Alice is deallocated."
Code language: JavaScript (javascript)
In this example, we define a class Person
with a name
property and a deinitializer (deinit
) to print a message when an instance of the class is deallocated. Inside the createPerson()
function, we create a Person
instance named person1
. Then, we assign person1
to another variable person2
, creating another strong reference to the same object. When the createPerson()
function exits, both person1
and person2
go out of scope, and there are no more strong references to the Person
object. As a result, the Person
object is deallocated, and the deinitializer is called, printing "Alice is deallocated."
. This demonstrates how ARC automatically manages the memory of objects in Swift.
Question:
Explain the concept of generics in Swift and why they are essential in building reusable and type-safe code. Provide an example of using generics in Swift.
Answer:
Generics in Swift allow you to write flexible and reusable code that can work with different types. They enable you to create functions, structures, and classes that can accept parameters of any type without sacrificing type safety. Generics are essential in building reusable code because they allow you to avoid
code duplication and write algorithms that work with a wide range of data types.
Here’s an example of using generics in Swift:
// Generic function to swap two values of any type
func swapValues<T>(_ a: inout T, _ b: inout T) {
let temp = a
a = b
b = temp
}
// Usage of the generic swap function with different data types
var intValue1 = 5
var intValue2 = 10
swapValues(&intValue1, &intValue2)
print("Swapped int values: (intValue1), (intValue2)") // Output: "Swapped int values: 10, 5"
var stringValue1 = "Hello"
var stringValue2 = "World"
swapValues(&stringValue1, &stringValue2)
print("Swapped string values: (stringValue1), (stringValue2)") // Output: "Swapped string values: World, Hello"
Code language: PHP (php)
In this example, we define a generic function swapValues(_:_:)
that takes two parameters of type T
(generic type). The function swaps the values of the two parameters using a temporary variable. This function can be used with different data types, including integers and strings, without explicitly writing separate swap functions for each type. The generic syntax, <T>
, allows Swift to infer the appropriate type based on the provided arguments, ensuring type safety at compile time. This showcases the flexibility and reusability achieved by using generics in Swift.
Senior Swift interview questions
Question:
Explain what Swift Optionals are and why they are important in Swift development. Provide an example of how to use Optionals in Swift.
Answer:
Swift Optionals are a feature that allows variables to have a value or to be nil (empty). They are crucial in Swift development because they help handle situations where a variable may not have a valid value. Optionals prevent unexpected crashes due to accessing nil values and promote safer code by making developers explicitly handle the possibility of nil.
Here’s an example of using Optionals in Swift:
// Optional Int
var age: Int? = 25
// Trying to access the value of an Optional
if let validAge = age {
print("Age is (validAge)")
} else {
print("Age is not available")
}
// Assigning nil to an Optional
var name: String? = "John"
name = nil
// Using Optional Chaining
struct Person {
var name: String?
}
let person1 = Person(name: "Alice")
let person2 = Person(name: nil)
print(person1.name?.count ?? 0) // Output: 5
print(person2.name?.count ?? 0) // Output: 0
Code language: JavaScript (javascript)
In this example, the variable age
is an Optional Int, and it can either hold an integer value or be nil. The if let
statement checks if age
has a value and safely unwraps it to the constant validAge
. The variable name
is an Optional String, and it is assigned nil. The Optional chaining is demonstrated using the Person
struct, where name
is an Optional property, and the count
property is accessed safely even when name
is nil.
Question:
Explain the concept of ARC (Automatic Reference Counting) in Swift. How does it help manage memory in Swift? Provide an example to illustrate its functionality.
Answer:
ARC (Automatic Reference Counting) is a memory management technique used by Swift to automatically manage the memory allocation and deallocation for objects. It works by keeping track of the number of references to an object. When the reference count drops to zero, indicating that no one is using the object, the memory occupied by the object is deallocated.
Here’s an example to illustrate ARC in Swift:
class Person {
let name: String
init(name: String) {
self.name = name
print("(name) is being initialized.")
}
deinit {
print("(name) is being deinitialized.")
}
}
var person1: Person? = Person(name: "Alice")
var person2: Person? = person1 // person1 and person2 now both reference the same Person instance
person1 = nil // The reference count of the Person instance decreases to 1
person2 = nil // The reference count of the Person instance decreases to 0 and the instance is deallocated
Code language: PHP (php)
In this example, when person1
and person2
are assigned to the same Person
instance, the reference count becomes 2. When both person1
and person2
are set to nil, the reference count drops to 0, and the Person
instance is deallocated, triggering the deinitializer.
Question:
What is the difference between a value type and a reference type in Swift? Provide examples of each.
Answer:
In Swift, value types and reference types are two different ways of representing and handling data.
Value Types:
- Value types store their data directly in the memory location where the variable is defined.
- When a value type is assigned to a new variable or passed as a function argument, a copy of the value is created, and modifications to the copy do not affect the original value.
- Examples of value types in Swift include
Int
,Double
,String
,Array
,Struct
, andEnum
.
var x = 10
var y = x // y is a copy of x
y += 5 // Does not affect the value of x
print(x) // Output: 10
print(y) // Output: 15
Code language: PHP (php)
Reference Types:
- Reference types store a reference to the memory location where the actual data is stored.
- When a reference type is assigned to a new variable or passed as a function argument, only the reference is copied, not the actual data. As a result, both the original and the new variable point to the same data in memory.
- Examples of reference types in Swift include
Class
and functions that return closures.
class Person {
var name: String
init(name: String) {
self.name = name
}
}
var person1 = Person(name: "Alice")
var person2 = person1 // person2 is a reference to the same Person instance as person1
person2.name = "Bob"
print(person1.name) // Output: "Bob" - Changes in person2 also affect person1
print(person2.name) // Output: "Bob"
Code language: PHP (php)
Question:
Explain the concept of Protocol in Swift and how it is used. Provide an example of a custom protocol and its implementation.
Answer:
In Swift, a protocol is a blueprint that defines a set of methods, properties, and requirements that a class or struct must adopt. Protocols allow you to define common behavior and functionality that can be shared among different types without the need for inheritance.
Here’s an example of a custom protocol called Vehicle
:
protocol Vehicle {
var numberOfWheels: Int { get }
func start()
func stop()
}
class Car: Vehicle {
var numberOfWheels: Int = 4
func start() {
print("Car started.")
}
func stop() {
print("Car stopped.")
}
}
class Bicycle: Vehicle {
var numberOfWheels: Int = 2
func start() {
print("Bicycle started.")
}
func stop() {
print("Bicycle stopped.")
}
}
let car = Car()
let bicycle = Bicycle()
car.start() // Output: "Car started."
bicycle.start() // Output: "Bicycle started."
In this example, the Vehicle
protocol defines two properties (numberOfWheels
) and two methods (start()
and stop()
). The Car
and Bicycle
classes conform to the Vehicle
protocol by implementing its requirements. The start()
and stop()
methods provide different implementations for each class while adhering to the same protocol.
Question:
What are the advantages of using Generics in Swift? Provide an example of a generic function.
Answer:
Generics in Swift allow you to write flexible and reusable code by defining functions, structures, and classes that can work with any type. They provide several advantages:
- Code Reusability: Generics enable you to write functions and data structures that can handle different types, avoiding code duplication.
- Type Safety: Swift’s type system ensures that generic code is type-safe at compile-time, preventing runtime errors.
- Performance: Generics enable efficient code by avoiding unnecessary type conversions and promoting inlining of specialized code for each type.
Here’s an example of a generic function that swaps two elements of any type:
func swapElements<T>(_ a: inout T, _ b: inout T) {
let temp = a
a = b
b = temp
}
var x = 5
var y = 10
swapElements(&x, &y)
print(x) // Output: 10
print(y) // Output: 5
var str1 = "Hello"
var str2 = "World"
swapElements(&str1, &str2)
print(str1) // Output: "World"
print(str2) // Output: "Hello"
Code language: PHP (php)
In this example, the swapElements()
function is generic and can work with any type T
. The <T>
syntax declares the type placeholder, and inout
is used to pass the variables by reference, allowing them to be modified within the function.
Question:
Explain the concept of DispatchQueue in Swift and how it is used to handle concurrency and asynchronous tasks. Provide an example of using DispatchQueue.
Answer:
DispatchQueue is a Swift framework for managing concurrent and asynchronous tasks. It allows you to execute tasks concurrently, either on the main thread or on a background thread, without worrying about low-level thread management.
Here’s an example of using DispatchQueue to perform a task asynchronously on a background thread:
import Foundation
func downloadDataFromServer(completion: @escaping (Data?) -> Void) {
DispatchQueue.global(qos: .background).async {
// Simulating a time-consuming task (e.g., downloading data from a server)
sleep(3)
// Data downloaded successfully
let data = Data()
completion(data)
}
}
print("Start downloading...")
downloadDataFromServer { data in
print("Download completed!")
// Process the downloaded data here
}
print("End of code.")
Code language: PHP (php)
In this example, the downloadDataFromServer()
function simulates a time-consuming task (e.g., downloading data from a server) by using sleep(3)
to pause execution for 3 seconds. The task is executed asynchronously on a background thread defined by DispatchQueue.global(qos: .background).async
.
The final output will be:
Start downloading...
End of code.
Download completed!
Notice that the “Download completed!” message is printed after “End of code.” because the task is executed asynchronously on a background thread.
Question:
Explain the concept of Swift’s Codable protocol and how it simplifies working with JSON data. Provide an example of using Codable to encode and decode JSON data.
Answer:
Swift’s Codable protocol is used for encoding and decoding data to and from different formats, such as JSON and property lists. By conforming to Codable, Swift types can be easily converted to and from their representation in JSON format, making working with JSON data much simpler and more efficient.
Here’s an example of using Codable to encode and decode JSON data:
struct Person: Codable {
var name: String
var age: Int
}
let jsonData = """
{
"name": "Alice",
"age": 30
}
""".data(using: .utf8)!
do {
// Decoding JSON data to Swift object
let person = try JSONDecoder().decode(Person.self, from: jsonData)
print(person) // Output: Person(name: "Alice", age: 30)
// Encoding Swift object to JSON data
let encodedData = try JSONEncoder().encode(person)
let jsonString = String(data: encodedData, encoding: .utf8)!
print(jsonString) // Output: "{"name":"Alice","age":30}"
} catch {
print("Error: (error)")
}
Code language: PHP (php)
In this example, the Person
struct conforms to Codable by adopting the protocol. The JSON data is represented as a Data
object, and the JSONDecoder
and JSONEncoder
classes are used to decode and encode the data, respectively. The decode()
method decodes JSON data into a Person
object, and the encode()
method encodes the Person
object back to JSON data.
Question:
What is the difference between weak
, unowned
, and strong
reference types in Swift? When would you use each of them?
Answer:
In Swift, reference cycles can lead to memory leaks, where objects are not deallocated as expected. To prevent strong reference cycles, developers can use weak
, unowned
, and strong
reference types:
weak
: Aweak
reference is a non-owning reference. It does not increase the reference count of the referenced object, and it is automatically set tonil
when the referenced object is deallocated. Useweak
to avoid strong reference cycles when there is a possibility that the referenced object might become nil.unowned
: Anunowned
reference is also a non-owning reference. Unlikeweak
, it is assumed that the referenced object always exists during the reference’s lifetime. If the referenced object is deallocated while theunowned
reference still exists, a runtime error will occur. Useunowned
when you are sure that the referenced object will not be deallocated before the reference is used.strong
: Astrong
reference is the default and is used to increase the reference count of the referenced object, keeping it alive as long as the reference exists.
Here’s an example of using weak
and unowned
references in Swift:
class Person {
let name: String
init(name: String) {
self.name = name
}
deinit {
print("(name) is being deinitialized.")
}
}
var strongReference: Person? = Person(name: "Alice")
// Using weak reference
weak var weakReference = strongReference
strongReference = nil
print(weakReference) // Output: nil
// Using unowned reference
unowned let unownedReference = strongReference!
strongReference = nil // Causes a runtime error: trying to access a deallocated instance
Code language: JavaScript (javascript)
In this example, strongReference
is a strong reference, and weakReference
is a weak reference. When strongReference
is set to nil, the weakReference
becomes nil, as the object it was pointing to is deallocated. The unownedReference
is an unowned reference and will cause a runtime error when strongReference
is set to nil because it assumes the referenced object will always exist.
Question:
Explain the concept of SwiftUI in Swift and how it simplifies the user interface development for iOS and macOS apps. Provide an example of creating a simple SwiftUI view.
Answer:
SwiftUI is a framework introduced by Apple that allows developers to build user interfaces declaratively using Swift code. It simplifies the process of creating user interfaces for iOS, macOS, watchOS, and tvOS apps by providing a modern and intuitive way to describe the UI hierarchy and behavior.
Here’s an example of creating a simple SwiftUI view that displays a text label and a button:
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
Text("Hello, SwiftUI!")
.font(.largeTitle)
.padding()
Button(action: {
print("Button tapped!")
}) {
Text("Tap Me!")
.font(.headline)
.foregroundColor(.white)
.padding()
.background(Color.blue)
.cornerRadius(8.0)
}
}
}
}
import PlaygroundSupport
PlaygroundPage.current.setLiveView(ContentView())
Code language: JavaScript (javascript)
In this example, the ContentView
struct
conforms to the View
protocol, and its body
property describes the layout of the UI using SwiftUI’s declarative syntax. The VStack
is used to vertically stack the Text
and Button
views. The Text
view displays a large title, and the Button
view is a clickable button with some styling. The Button
‘s action closure will be executed when the button is tapped, printing “Button tapped!” to the console. This SwiftUI code can be used in an Xcode project or even in a Swift Playground to visualize the UI.
Question:
Explain the concept of optionals in Swift and why they are important in handling null or missing values. Provide an example of using optionals in a real-world scenario.
Answer:
In Swift, optionals are a powerful feature used to handle the absence of a value, which is commonly referred to as a “null” or “nil” value in other programming languages. Optionals allow developers to indicate that a variable might have a value, or it might be nil. This distinction helps in writing safer code and avoiding runtime crashes due to unexpected nil values.
Real-world scenario example:
Let’s consider a weather app that fetches temperature data from a web API. Sometimes, the API might not return the temperature data due to a network issue or other reasons. In such cases, we can use optionals to handle the absence of data:
struct Weather {
var temperature: Double?
var condition: String?
}
func fetchWeatherData(completion: @escaping (Weather?) -> Void) {
// Simulate fetching data from API (e.g., using URLSession)
DispatchQueue.main.asyncAfter(deadline: .now() + 2) {
// Assume successful API call
completion(Weather(temperature: 25.0, condition: "Sunny"))
// If API call fails, we can pass nil to indicate the absence of data.
}
}
// Usage
fetchWeatherData { weather in
if let temperature = weather?.temperature {
print("Current temperature: (temperature)°C")
} else {
print("Failed to fetch temperature data.")
}
}
Code language: JavaScript (javascript)
In this example, the Weather
struct has optional properties temperature
and condition
, which may or may not have values. The fetchWeatherData
function simulates fetching weather data from an API. We pass a closure to the function, which will be called with the Weather
object (if data is available) or nil (if data is missing). By using optionals and conditional binding (if let
), we can safely handle the case when the weather data is missing, and our app won’t crash due to nil values.
Question:
The following Swift code is intended to read data from a file and decode it into a custom data structure using Codable. However, it contains a logical error and doesn’t compile correctly. Identify the error and fix the code.
struct Employee: Codable {
var name: String
var age: Int
var position: String
}
func readEmployeeDataFromFile() -> Employee? {
let fileURL = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask).first?.appendingPathComponent("employee_data.json")
if let fileURL = fileURL {
do {
let jsonData = try Data(contentsOf: fileURL)
let employee = try JSONDecoder().decode(Employee.self, from: jsonData)
return employee
} catch {
print("Error reading or decoding file:", error)
}
}
return nil
}
Code language: JavaScript (javascript)
Answer:
The logical error in the code is that it does not handle potential errors when reading data from the file or decoding JSON. The try
keyword is missing when calling Data(contentsOf:)
and JSONDecoder().decode()
.
Additionally, it is essential to use do-catch
blocks to handle errors correctly when performing operations that can throw exceptions.
Here’s the corrected code:
struct Employee: Codable {
var name: String
var age: Int
var position: String
}
func readEmployeeDataFromFile() -> Employee? {
let fileURL = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask).first?.appendingPathComponent("employee_data.json")
if let fileURL = fileURL {
do {
let jsonData = try Data(contentsOf: fileURL)
let employee = try JSONDecoder().decode(Employee.self, from: jsonData)
return employee
} catch {
print("Error reading or decoding file:", error)
return nil
}
}
return nil
}
Code language: JavaScript (javascript)
In the corrected code, we use try
keyword appropriately for the Data(contentsOf:)
and JSONDecoder().decode()
operations. Additionally, we handle the errors with a do-catch
block, and in case of any error, we return nil
.
Question:
Explain the concept of generics in Swift and how they contribute to writing reusable and type-safe code. Provide an example of using generics in Swift.
Answer:
Generics in Swift allow developers to create flexible and reusable functions, structures, and classes that can work with any data type. Instead of specifying the type of data explicitly, generics enable the use of placeholder types, allowing the code to handle various data types without duplication.
Benefits of generics:
- Code Reusability: Generics allow developers to write functions or data structures that can work with multiple data types, reducing code duplication.
- Type Safety: Generics ensure that the correct types are used during compile-time, reducing the chances of runtime errors.
Example of using generics:
func swapTwoValues<T>(_ a: inout T, _ b: inout T) {
let temp = a
a = b
b = temp
}
var a = 5
var b = 10
swapTwoValues(&a, &b)
print("a:", a, "b:", b) // Output: a: 10 b: 5
var x = "Hello"
var y = "World"
swapTwoValues(&x, &y)
print("x:", x, "y:", y) // Output: x: World y: Hello
Code language: PHP (php)
In this example, the swapTwoValues
function uses a generic placeholder type T
to swap two values of any data type. The function can be used with integers, strings, or any other data type without the need to create separate swap functions for each type, making the code more reusable and type-safe.
Question:
The following Swift code is intended to perform a network request using URLSession and handle the response using a completion handler. However, it contains a logical error and doesn’t work correctly. Identify the error and fix the code.
struct Post: Codable {
var id: Int
var title: String
var body: String
}
func fetchPosts(completion: @escaping ([Post]?) -> Void) {
let url = URL(string: "https://jsonplaceholder.typicode.com/posts")!
let task = URLSession.shared.dataTask(with: url) { data, _, error in
if let error = error {
print("Error fetching posts:", error)
completion(nil)
return
}
if let data = data {
do {
let posts = try JSONDecoder().decode([Post].self, from: data)
completion(posts)
} catch {
print("Error decoding JSON:", error)
completion(nil)
}
}
}
task.resume()
}
Code language: JavaScript (javascript)
Answer:
The logical error in the code is that the completion
handler is not called on the main queue, which can lead to unexpected behavior or UI issues.
To fix this, we need to dispatch the completion handler on the main queue, as shown in the corrected code:
struct Post: Codable {
var
id: Int
var title: String
var body: String
}
func fetchPosts(completion: @escaping ([Post]?) -> Void) {
let url = URL(string: "https://jsonplaceholder.typicode.com/posts")!
let task = URLSession.shared.dataTask(with: url) { data, _, error in
if let error = error {
print("Error fetching posts:", error)
DispatchQueue.main.async {
completion(nil)
}
return
}
if let data = data {
do {
let posts = try JSONDecoder().decode([Post].self, from: data)
DispatchQueue.main.async {
completion(posts)
}
} catch {
print("Error decoding JSON:", error)
DispatchQueue.main.async {
completion(nil)
}
}
}
}
task.resume()
}
Code language: JavaScript (javascript)
In the corrected code, we use DispatchQueue.main.async
to dispatch the completion handler on the main queue, ensuring that any UI updates or interactions are done on the main thread, as required by UIKit and other UI frameworks.
Question:
Explain the concept of memory management in Swift and how Automatic Reference Counting (ARC) works. Describe common scenarios that can lead to retain cycles and how to avoid them.
Answer:
Memory management in Swift involves handling the allocation and deallocation of objects to efficiently manage system memory. Swift uses Automatic Reference Counting (ARC) to manage memory automatically. ARC automatically keeps track of the number of strong references to an object, and when there are no strong references remaining, the object is deallocated to free up memory.
How ARC works:
- When an object is created (e.g., using
init()
ornew
), its reference count is set to 1. - When a strong reference to the object is established (e.g., assigning it to a variable), the reference count is incremented by 1.
- When a strong reference to the object is removed (e.g., setting the variable to nil), the reference count is decremented by 1.
- When the reference count reaches 0 (i.e., no strong references exist), the object is deallocated, and its memory is released.
Common scenarios leading to retain cycles:
Retain cycles occur when objects hold strong references to each other, forming a closed loop, and preventing ARC from deallocating them, even when they are no longer needed. Common scenarios that can lead to retain cycles are:
- Strong reference cycles between two objects referencing each other.
- Strong reference cycles involving closures that capture the instance they are defined in.
How to avoid retain cycles:
To avoid retain cycles, you can use weak or unowned references instead of strong references when appropriate. Both weak and unowned references do not increment the reference count and allow objects to be deallocated when there are no strong references remaining.
- Use
weak
when the reference can become nil (e.g., in a delegate pattern). - Use
unowned
when the reference will always have a value throughout its lifetime (e.g., in a closure capturing self in an escaping context).
Example:
class Person {
let name: String
var pet: Pet?
init(name: String) {
self.name = name
}
deinit {
print("(name) is being deallocated.")
}
}
class Pet {
let name: String
weak var owner: Person?
init(name: String) {
self.name = name
}
deinit {
print("(name) is being deallocated.")
}
}
var john: Person? = Person(name: "John")
var dog: Pet? = Pet(name: "Buddy")
john?.pet = dog
dog?.owner = john
// Setting references to nil to break the retain cycle
john = nil
dog = nil
Code language: JavaScript (javascript)
In this example, the Person
and Pet
classes have a strong reference to each other. By using weak references for the owner
property in the Pet
class, we break the retain cycle and ensure that both Person
and Pet
instances can be deallocated properly when their strong references are set to nil.
1,000 Companies use CoderPad to Screen and Interview Developers
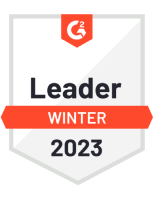
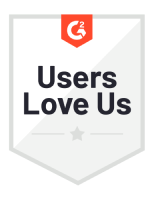
Best interview practices for Swift roles
To ensure successful Swift interviews, it’s important to consider various factors, such as the candidate’s background and the specific engineering role. To create a positive interview experience, we recommend adopting the following best practices:
- Formulate technical questions that reflect real-world business scenarios within your organization. This approach will effectively engage the candidate and help in assessing their compatibility with your team.
- Foster a collaborative environment by inviting candidates to ask questions throughout the interview.
- Ensure candidates possess knowledge of Auto Layout and constraints for creating responsive apps.
Additionally, it’s important to follow standard interview practices when conducting Swift interviews. This involves adjusting question difficulty based on the candidate’s abilities, providing prompt updates about their application status, and allowing them to inquire about the assessment process and collaboration with you and your team.