Scala Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Scala developers for your organization.
Scala
Developed to address the weaknesses of Java, Scala maintains compatibility with the Java Virtual Machine (JVM) while having more concise syntax, embracing functional programming (in addition to object-oriented programming), and having more powerful type inference.
Drawing inspiration from multiple programming languages such as Java, Haskell, and ML, Scala combines the object-oriented and functional programming paradigms in an effort to offer the most advantageous aspects of each.
https://docs.scala-lang.org
We have developed practical coding assignments and interview questions tailored to evaluate developers’ Scala skills during coding interviews. Furthermore, we have compiled a set of best practices to ensure that your interview questions accurately gauge the candidates’ proficiency in Scala.
Table of Contents
Scala example question
Help us design a parking lot
Hey candidate! Welcome to your interview. Boilerplate is provided. Feel free to change the code as you see fit. To run the code at any time, please hit the run button located in the top left corner.
Goals: Design a parking lot using object-oriented principles
Here are a few methods that you should be able to run:
- Tell us how many spots are remaining
- Tell us how many total spots are in the parking lot
- Tell us when the parking lot is full
- Tell us when the parking lot is empty
- Tell us when certain spots are full e.g. when all motorcycle spots are taken
- Tell us how many spots vans are taking up
Assumptions:
- The parking lot can hold motorcycles, cars and vans
- The parking lot has motorcycle spots, car spots and large spots
- A motorcycle can park in any spot
- A car can park in a single compact spot, or a regular spot
- A van can park, but it will take up 3 regular spots
- These are just a few assumptions. Feel free to ask your interviewer about more assumptions as needed
Scala skills to assess
Jobs using Scala
Junior Scala interview questions
Question:
Explain the concept of immutability in Scala and why it is important in functional programming.
Answer:
In Scala, immutability refers to the property of an object or data structure that once created, cannot be modified. In other words, once a value is assigned to an immutable variable, it cannot be changed. Immutability is a fundamental concept in functional programming because it helps avoid side effects and makes programs easier to reason about and understand.
In functional programming, functions are expected to produce the same output for the same input, regardless of when or where they are called. Immutability ensures that data does not change unexpectedly, which is crucial for achieving referential transparency and making functions pure.
For example, consider the following Scala code using an immutable list:
val numbers = List(1, 2, 3)
val doubledNumbers = numbers.map(_ * 2)
Code language: PHP (php)
In this example, the original numbers
list remains unchanged after the map
operation, and a new list doubledNumbers
is created with the doubled values. This immutability ensures that the original data is preserved, making the code more predictable and reliable.
Question:
The following Scala code is intended to calculate the factorial of a given number using recursion. However, it contains a syntax error and doesn’t compile. Identify the error and fix the code.
def factorial(n: Int): Int = {
if (n == 0) {
1
} else {
n * factorial(n - 1)
}
Code language: JavaScript (javascript)
Answer:
The syntax error in the code is the missing closing brace }
for the factorial
function. The correct code is as follows:
def factorial(n: Int): Int = {
if (n == 0) {
1
} else {
n * factorial(n - 1)
}
}
Code language: JavaScript (javascript)
In this corrected code, the closing brace is added to properly close the factorial
function.
Question:
Explain what Option[T] is in Scala, and how it helps in handling null or missing values.
Answer:
In Scala, Option[T]
is a container type that represents an optional value. It can either hold a value of type T
(Some[T]) or be empty (None). This type is commonly used to handle situations where a value may be present or absent, such as when dealing with the possibility of null or missing values.
By using Option[T]
, developers can avoid null pointer exceptions and write safer and more robust code. When accessing the value from an Option
, developers need to handle both cases: when the Option
contains a value (Some[T]) and when it is empty (None).
For example, consider the following code using Option
:
val maybeName: Option[String] = Some("John")
// Safe access to the value using pattern matching
val name = maybeName match {
case Some(value) => value
case None => "Unknown"
}
Code language: JavaScript (javascript)
In this example, maybeName
is an Option[String]
containing the name “John.” By using pattern matching, we can safely access the value of maybeName
and handle the case when it is empty.
Question:
The following Scala code is intended to filter out even numbers from a list. However, it contains a logical error and does not produce the correct result. Identify the error and fix the code.
def filterEvenNumbers(numbers: List[Int]): List[Int] = {
for (num <- numbers) {
if (num % 2 == 0)
num :: numbers
}
}
Code language: PHP (php)
Answer:
The logical error in the code is that the filtered even numbers are not collected into a new list. The correct code is as follows:
def filterEvenNumbers(numbers: List[Int]): List[Int] = {
var result: List[Int] = List()
for (num <- numbers) {
if (num % 2 == 0)
result = num :: result
}
result.reverse
}
Code language: PHP (php)
In this corrected code, we initialize an empty list result
to collect the filtered even numbers. We then use the ::
operator to prepend the even numbers to the result
list. Since ::
adds elements at the beginning of the list, we need to reverse the list to maintain the original order.
Question:
Explain the concept of pattern matching in Scala and provide an example.
Answer:
Pattern matching in Scala is a powerful feature that allows developers to match and destructure data structures like tuples, case classes, and sealed classes based on their shape or content. It is similar to a switch or case statement found in other programming languages but with more expressive and flexible capabilities.
Here’s an example of pattern matching using a case class:
case class Person(name: String, age: Int)
def describePerson(person: Person): String = person match {
case Person("John", age) if age < 30 => "Young John"
case Person("John", age) => "John"
case Person(name, age) if age >= 18 => s"Adult $name"
case _ => "Unknown"
}
Code language: HTML, XML (xml)
In this example, we define a case class Person
with name
and age
fields. The describePerson
function takes a Person
object as an argument and matches it against different cases. If the person’s name is “John” and their age is less than 30, it returns “Young John.” If the name is “John” but the age is greater or equal to 30, it returns “John.” If the person is an adult (age >= 18), it returns “Adult {name}”. For any other cases, it returns “Unknown.”
Question:
The following Scala code is intended to calculate the sum of squares of elements in a list. However, it contains a syntax error and doesn’t compile. Identify the error and fix the code.
def sumOfSquares(numbers: List[Int]): Int = {
var sum = 0
for (num <- numbers) {
square = num * num
sum += square
}
sum
Code language: HTML, XML (xml)
Answer:
The syntax error in the code is the missing type annotation for the square
variable and the missing closing brace }
for the sumOfSquares
function. The correct code is as follows:
def sumOfSquares(numbers: List[Int]): Int = {
var sum = 0
for (num <- numbers) {
val square: Int = num * num
sum += square
}
sum
}
Code language: HTML, XML (xml)
In this corrected code, we add the type annotation : Int
for the square
variable to specify its type as Int
. Additionally, we add the closing brace }
to properly close the sumOfSquares
function.
Question:
Explain the concept of higher-order functions in Scala and provide an example.
Answer:
Higher-order functions in Scala are functions that can take other functions as arguments or return functions as results. They treat functions as first-class citizens, allowing developers to pass behaviors or functionality as values to other functions.
Here’s an example of a higher-order function in Scala:
def applyFunctionTwice(func: Int => Int, x: Int): Int = {
func(func(x))
}
val increment: Int => Int = (x: Int) => x + 1
val result = applyFunctionTwice(increment, 5)
Code language: JavaScript (javascript)
In this example, we define a higher-order function applyFunctionTwice
that takes two arguments: a function func
of type Int => Int
and an integer x
. The applyFunctionTwice
function applies the func
twice to the input x
and returns the result.
We also define a function increment
that takes an integer x
and returns x + 1
. We then call applyFunctionTwice
with increment
and 5
as arguments, resulting in 7
as the output (increment(increment(5))).
Question:
The following Scala code is intended to find the maximum element in a list using recursion. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
def findMax(numbers: List[Int]): Int = {
if (numbers.isEmpty)
return None
if (numbers.length == 1)
return numbers.head
val restMax = findMax(numbers.tail)
if (numbers.head > restMax)
numbers.head
else
restMax
Code language: PHP (php)
Answer:
The logical errors in the code are the incorrect return types for the base cases and the missing return keyword for the last comparison. The correct code is as follows:
def findMax(numbers: List[Int]): Int = {
if (numbers.isEmpty)
return Int.MinValue
if (numbers.length == 1)
return numbers.head
val restMax = findMax(numbers.tail)
if (numbers.head > restMax)
numbers.head
else
restMax
}
Code language: PHP (php)
In this corrected code, for the base case when the list is empty, we return Int.MinValue
, which acts as a sentinel value to ensure it does not affect the comparison with other integers in the list. For the single-element list, we correctly return numbers.head
. Additionally, we use the return
keyword to ensure that the correct value is returned in all cases.
Question:
Explain the concept of currying in Scala and why it is useful.
Answer:
Currying in Scala is a technique of transforming a function that takes multiple arguments into a series of functions, each taking a single argument. The resulting functions can be composed or partially applied, making the code more modular and flexible.
By currying functions, developers can easily create specialized versions of a function or create new functions by partially applying some arguments. This approach aligns well with functional programming paradigms and enables better code reuse and composition.
Here’s an example of currying in Scala:
def add(x: Int)(y: Int): Int = x + y
val addFive = add(5) _ // Partially applied function
val result = addFive(3) // result = 8
Code language: JavaScript (javascript)
In this example, we define a curried function add
that takes two integer arguments, x
and y
. We can partially apply the add
function by fixing the value of x
to 5, creating a new function addFive
that takes only y
. We can then call addFive
with a single argument 3
, resulting in 8
as the output.
Question:
The following Scala code is intended to calculate the sum of squares of even numbers in a list using the filter
and map
higher-order functions. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
def sumOfSquaresOfEvens(numbers: List[Int]): Int = {
numbers.filter(_ % 2 == 0)
.map(_ * _)
.sum
Code language: PHP (php)
Answer:
The logical error in the code is the incorrect usage of the anonymous function for multiplication in the map
operation. The correct code is as follows:
def sumOfSquaresOfEvens(numbers: List[Int]): Int = {
numbers.filter(_ % 2 == 0)
.map(num => num * num)
.sum
}
Code language: JavaScript (javascript)
In this corrected code, we use the lambda syntax (num => num * num)
to define an anonymous function that squares each element in the list during the map
operation. This ensures that the sum of squares of even numbers is correctly calculated.
Intermediate Scala interview questions
Question:
Explain the concept of immutability in Scala and why it is important in functional programming.
Answer:
Immutability in Scala refers to the property of objects whose state cannot be changed after they are created. In other words, once an object is instantiated, its data remains constant throughout its lifetime. Immutability is a fundamental concept in functional programming and brings several benefits:
- Thread Safety: Immutable data structures are inherently thread-safe because they cannot be modified concurrently. This eliminates the need for complex synchronization mechanisms and helps avoid common concurrency issues like race conditions.
- Predictable Behavior: Since immutable objects cannot change state, their behavior remains consistent and predictable. This simplifies reasoning about code and makes it easier to understand and maintain.
- Functional Purity: Immutability is a crucial aspect of functional purity, where functions depend only on their inputs and produce predictable outputs, without causing side effects.
- Easy Sharing and Caching: Immutable data can be safely shared across different parts of the application without fear of unintended modifications. This allows for efficient caching and memoization.
Question:
The following Scala code is intended to compute the factorial of a given number using recursion. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
def factorial(n: Int): Int = {
if (n == 0) 1
n * factorial(n - 1)
}
Answer:
The logical error in the code is that the base case of the recursion is not correctly specified. The correct code is as follows:
def factorial(n: Int): Int = {
if (n == 0) 1
else n * factorial(n - 1)
}
Code language: JavaScript (javascript)
In this corrected code, the base case is specified correctly with the else
keyword. Now the factorial function will correctly calculate the factorial of the given number.
Question:
Explain the concept of pattern matching in Scala and how it is used.
Answer:
Pattern matching in Scala is a powerful feature that allows you to match the structure of data against defined patterns. It is often used with match
expressions to conditionally execute code based on the matched pattern. Pattern matching can be applied to various data types, including case classes, tuples, lists, and more.
Here’s an example of pattern matching with a match
expression in Scala:
def matchExample(input: Any): String = input match {
case 1 => "One"
case "hello" => "Greetings!"
case lst: List[Int] => s"List of Integers: $lst"
case (x, y) => s"Tuple: $x, $y"
case _ => "Unrecognized input"
}
Code language: PHP (php)
In this example, the matchExample
function takes an input
parameter and uses pattern matching to handle different cases. If the input is 1, it returns “One.” If it is the string “hello,” it returns “Greetings!” For a list of integers, it returns a string indicating it’s a list of integers, and for a tuple, it returns a string representing the tuple’s values. The _
is used as a wildcard to handle any other unrecognized input.
Question:
The following Scala code is intended to calculate the sum of even numbers from a given list using a higher-order function. However, it contains a syntax error and doesn’t compile. Identify the error and fix the code.
val numbers = List(1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
val sumOfEvens = numbers.filter(_ % 2 == 0).reduce((a, b) => a + b)
Code language: JavaScript (javascript)
Answer:
The syntax error in the code is related to the parentheses around the lambda function used in the reduce
method. The correct code is as follows:
val numbers = List(1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
val sumOfEvens = numbers.filter(_ % 2 == 0).reduce((a, b) => a + b)
Code language: JavaScript (javascript)
In this corrected code, the parentheses around the lambda function (a, b) => a + b
are removed, and the sum of even numbers in the list is calculated correctly.
Question:
Explain the concept of higher-order functions in Scala and provide an example of their usage.
Answer:
Higher-order functions in Scala are functions that take other functions as arguments or return functions as results. They allow functions to be treated as first-class citizens, enabling powerful abstractions and functional programming paradigms.
Here’s an example of a higher-order function in Scala:
def applyOperation(operation: (Int, Int) => Int, a: Int, b: Int): Int = {
operation(a, b)
}
val add: (Int, Int) => Int = (x, y) => x + y
val multiply: (Int, Int) => Int = (x, y) => x * y
val result1 = applyOperation(add, 5, 3)
val result2 = applyOperation(multiply, 4, 7)
Code language: JavaScript (javascript)
In this example, the applyOperation
function is a higher-order function that takes an operation function and two integers as arguments. The add
and multiply
functions are examples of operation functions that can be passed to applyOperation
. Depending on the operation function used, result1
will be 8 (5 + 3), and result2
will be 28 (4 * 7).
Question:
The following Scala code is intended to calculate the sum of squares of a given list. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
val numbers = List(1, 2, 3, 4, 5)
val sumOfSquares = numbers.map(n => n * n).reduce((a, b) => a + b)
Code language: JavaScript (javascript)
Answer:
The logical error in the code is that the parentheses are missing around the lambda function used in the map
method. The correct code is as follows:
val numbers = List(1, 2, 3, 4, 5)
val sumOfSquares = numbers.map(n => n * n).reduce((a, b) => a + b)
Code language: JavaScript (javascript)
In this corrected code, the parentheses around the lambda function (n => n * n)
are added, and the sum of squares is calculated correctly.
Question:
Explain the concept of implicits in Scala and provide an example of their usage.
Answer:
Implicits in Scala allow you to define additional operations on existing classes without modifying their original source code. They enable implicit conversions, implicit parameters, and type classes, providing a powerful mechanism for extending library classes and adding functionalities.
Here’s an example of using implicits to extend the functionality of an existing class:
class MyString(str: String) {
def reverse: String = str.reverse
}
object ImplicitsExample {
implicit def stringToMyString(str
: String): MyString = new MyString(str)
def main(args: Array[String]): Unit = {
val myStr: MyString = "Hello, Scala!" // Implicit conversion
val reversedStr: String = myStr.reverse // Using the extended functionality
println(reversedStr) // Output: "!alacS ,olleH"
}
}
In this example, we create a class MyString
that extends the functionality of the String
class by adding a reverse
method. The implicit conversion method stringToMyString
allows us to automatically convert a String
to a MyString
object. As a result, we can use the reverse
method on a regular String
as if it were a MyString
object.
Question:
The following Scala code is intended to define a case class representing a circle and calculate its area. However, it contains a syntax error and doesn’t compile. Identify the error and fix the code.
case class Circle(radius: Double)
object CircleExample {
def calculateArea(circle: Circle): Double = {
val area = Math.PI * radius * radius
area
}
def main(args: Array[String]): Unit = {
val circle = Circle(5.0)
val area = calculateArea(circle)
println(s"The area of the circle is: $area")
}
}
Answer:
The syntax error in the code is that the radius
variable is used without prefixing it with circle
, which is the name of the case class instance. The correct code is as follows:
case class Circle(radius: Double)
object CircleExample {
def calculateArea(circle: Circle): Double = {
val area = Math.PI * circle.radius * circle.radius
area
}
def main(args: Array[String]): Unit = {
val circle = Circle(5.0)
val area = calculateArea(circle)
println(s"The area of the circle is: $area")
}
}
In this corrected code, the radius
variable is accessed using the circle
instance of the Circle
case class, allowing the area of the circle to be calculated correctly.
Question:
Explain the concept of Option in Scala and its purpose. How does it help in handling null or absent values?
Answer:
Option in Scala is a container type used to represent the presence or absence of a value. It is commonly used to handle situations where a value may or may not be present, avoiding the use of null
references and reducing the risk of null pointer exceptions.
An Option
instance can have one of two possible values:
Some(value)
: Represents the presence of a value, wherevalue
is the actual value.None
: Represents the absence of a value.
Option
is often used as the return type of function that may or may not return a value. It enforces explicit handling of the absence of a value, promoting safer and more robust code.
Here’s an example of using Option
to handle null or absent values:
def findElement(arr: Array[Int], target: Int): Option[Int] = {
val index = arr.indexOf(target)
if (index != -1) Some(index)
else None
}
val array = Array(1, 3, 5, 7, 9)
val targetElement = 5
val result = findElement(array, targetElement)
result match {
case Some(index) => println(s"Element $targetElement found at index $index.")
case None => println(s"Element $targetElement not found.")
}
Code language: PHP (php)
In this example, the findElement
function returns an Option[Int]
, indicating whether the target element was found or not. The match
expression is used to handle the two possible cases: Some(index)
if the element is found, and None
if it is not found.
Question:
The following Scala code is intended to filter out duplicate elements from a given list. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
val numbers = List(1, 2, 2, 3, 4, 4, 5, 5, 5)
val uniqueNumbers = numbers.distinct
Code language: PHP (php)
Answer:
The logical error in the code is that the distinct
method removes all duplicate elements, leaving only unique elements in the list. The correct code is as follows:
val numbers = List(1, 2, 2, 3, 4, 4, 5, 5, 5)
val uniqueNumbers = numbers.toSet.toList
Code language: PHP (php)
In this corrected code, the toSet
method is used to convert the list to a set, which automatically removes duplicate elements. The resulting set is then converted back to a list using toList
, resulting in uniqueNumbers
containing only distinct elements.
Senior Scala interview questions
Question:
Explain the concept of immutability in Scala and its benefits in concurrent programming. Provide an example demonstrating how immutability is enforced in Scala.
Answer:
In Scala, immutability refers to the property of objects that cannot be modified after they are created. Once an immutable object is created, its state cannot change, and any attempt to modify it will result in a new object being created with the updated values. Immutability ensures that data remains constant and cannot be accidentally altered, which has several benefits in concurrent programming.
Benefits of immutability in concurrent programming:
- Thread Safety: Since immutable objects cannot be modified, they are inherently thread-safe. Multiple threads can access and use immutable objects without the risk of concurrent modifications and data corruption.
- No Synchronization: Immutability eliminates the need for explicit synchronization mechanisms (e.g., locks) to manage concurrent access to shared data. This simplifies the code and reduces the likelihood of deadlocks and race conditions.
- Predictable Behavior: Immutable objects have a predictable state throughout their lifecycle, making it easier to reason about their behavior and ensuring consistent results in concurrent scenarios.
Example demonstrating immutability in Scala:
case class Person(name: String, age: Int)
// Create an immutable instance of Person
val person = Person("Alice", 30)
// Attempt to modify the person object
// This will create a new instance with the updated age
val updatedPerson = person.copy(age = 31)
println(s"Original Person: $person")
println(s"Updated Person: $updatedPerson")
In this example, we define a Person
case class with two fields: name
and age
. The Person
instance is created with the val
keyword, making it immutable. When we attempt to modify the age
field using the copy
method, it returns a new instance of Person
with the updated age. The original person
object remains unchanged. This behavior ensures that the state of the Person
object remains constant, enforcing immutability in Scala.
Question:
Explain the concept of Option in Scala and how it helps in handling null or absent values. Provide an example of using Option in Scala.
Answer:
In Scala, Option
is a container type that represents an optional value that may or may not exist. It is used as a safer alternative to handling null values and helps in avoiding null pointer exceptions. An Option
can either be Some(value)
if the value is present or None
if the value is absent.
Benefits of Option in handling null or absent values:
- Null Safety: Option provides a clear and explicit way to handle the possibility of null values without introducing null pointer exceptions.
- Avoiding NullPointerExceptions: By using Option, developers are encouraged to handle the absence of a value explicitly, reducing the likelihood of unintended null dereferences.
Example of using Option in Scala:
// Function to find an element in a list and return it as an Option
def findElement(list: List[Int], target: Int): Option[Int] = {
val result = list.find(_ == target)
result match {
case Some(value) => Some(value)
case None => None
}
}
val numbers = List(1, 2, 3, 4, 5)
val targetValue = 3
val foundElement = findElement(numbers, targetValue)
foundElement match {
case Some(value) => println(s"Element $targetValue found: $value")
case None => println(s"Element $targetValue not found.")
}
Code language: PHP (php)
In this example, the function findElement
takes a list of integers and a target value as input. It uses the find
method on the list to look for the target value. If the value is found, it returns Some(value)
wrapped in an Option. If the value is not found, it returns None
. The function demonstrates how Option can be used to handle the absence of a value safely.
Question:
Explain the concept of pattern matching in Scala and its significance in functional programming. Provide an example demonstrating pattern matching.
Answer:
Pattern matching is a powerful feature in Scala that allows you to match the structure of data against predefined patterns and extract values accordingly. It is widely used in functional programming to handle different cases or scenarios elegantly and concisely.
Significance of pattern matching in functional programming:
- Concise Control Flow: Pattern matching allows you to express complex control flows in a more concise and readable manner compared to traditional if-else or switch statements.
- Extensibility: Pattern matching is extensible, meaning you can define your custom pattern matching rules by implementing the unapply method in extractor objects. This gives you the flexibility to pattern match on your own data types.
- Exhaustiveness Checking: The Scala compiler performs exhaustiveness checking on pattern matching, ensuring that all possible cases are covered. This helps catch potential bugs and makes the code more robust.
Example demonstrating pattern matching in Scala:
def matchDayOfWeek(day: String): String = day match {
case "Monday" | "Tuesday" | "Wednesday" | "Thursday" | "Friday" => "Weekday"
case "Saturday" | "Sunday" => "Weekend"
case _ => "Invalid day"
}
val day1 = "Monday"
val day2 = "Saturday"
val day3 = "InvalidDay"
println(s"$day1 is a ${matchDayOfWeek(day1)}")
println(s"$day2 is a ${matchDayOfWeek(day2)}")
println(s"$day3 is a ${matchDayOfWeek(day3)}")
Code language: JavaScript (javascript)
In this example, the matchDayOfWeek
function takes a day as input and uses pattern matching to classify it as a weekday, weekend, or an invalid day. The first case matches any of the weekdays, the second case matches weekends, and the third case is the default (catch-all) case for any other input. The use of pattern matching makes the code concise and easy to understand.
Question:
Explain the concept of currying in Scala and its benefits in functional programming. Provide an example of using currying in Scala.
Answer:
Currying is a technique in functional programming where a function that takes multiple arguments is transformed into a series of functions, each taking a single argument. It allows for partial application of functions and creates a more modular and flexible codebase.
Benefits of currying in functional programming:
- Partial Function Application: Currying enables partial function application, where you can fix some arguments of a function and obtain a new function with the remaining arguments. This makes it easier to reuse and compose functions.
- Function Composition: Currying promotes function composition, where functions can be combined to create more complex and reusable operations.
- Flexibility and Reusability: Currying provides a higher level of flexibility, allowing functions to be reused in different contexts with varying numbers of arguments.
Example demonstrating currying in Scala:
// Uncurried function
def add(x: Int, y: Int): Int = x + y
// Curried function
def curriedAdd(x: Int)(y: Int): Int = x + y
// Partial application of curriedAdd
val addFive = curriedAdd(5) _
println(s"Uncurried add: ${add(3, 4)}")
println(s"Curried add: ${curriedAdd(3)(4)}")
println
(s"Partial application of curriedAdd: ${addFive(4)}")
Code language: JavaScript (javascript)
In this example, we define an add
function that takes two arguments and returns their sum. We then define a curriedAdd
function, which is the curried version of the add
function. The curried version allows us to call it with one argument to get a new function that expects the second argument.
The partial application of the curriedAdd
function is demonstrated using addFive
, which is a new function derived from curriedAdd
with x
fixed as 5. This partial function application creates a reusable function that only requires one argument to complete the calculation.
Question:
Explain the concept of higher-order functions in Scala and their significance in functional programming. Provide an example of using higher-order functions in Scala.
Answer:
Higher-order functions in Scala are functions that take one or more functions as arguments or return functions as results. They treat functions as first-class citizens, allowing them to be manipulated and composed like any other data type. Higher-order functions play a significant role in functional programming, enabling more concise and expressive code.
Significance of higher-order functions in functional programming:
- Abstraction: Higher-order functions abstract common patterns of computation, making the code more modular and reusable.
- Function Composition: Higher-order functions enable function composition, where multiple functions can be combined to create new functions that perform complex operations.
- Encapsulation of Behavior: Higher-order functions encapsulate behavior, allowing different behaviors to be passed to the same function, making it flexible and adaptable.
Example demonstrating higher-order functions in Scala:
// Higher-order function: map
def doubleNumbers(numbers: List[Int], f: Int => Int): List[Int] = numbers.map(f)
// Function to double a number
def double(x: Int): Int = x * 2
// Function to square a number
def square(x: Int): Int = x * x
val numbers = List(1, 2, 3, 4, 5)
val doubledNumbers = doubleNumbers(numbers, double)
val squaredNumbers = doubleNumbers(numbers, square)
println(s"Doubled numbers: $doubledNumbers")
println(s"Squared numbers: $squaredNumbers")
Code language: PHP (php)
In this example, we define a higher-order function doubleNumbers
that takes a list of numbers and a function f
as arguments. The map
function is used inside doubleNumbers
to apply f
to each element of the list, effectively doubling or squaring the numbers.
We define two simple functions double
and square
that double and square a given number, respectively. By passing these functions as arguments to doubleNumbers
, we can double or square the numbers in the numbers
list, demonstrating the use of higher-order functions to abstract behavior and create more flexible code.
Question:
Explain the concept of Futures in Scala and their role in asynchronous programming. Provide an example of using Futures in Scala to perform asynchronous tasks.
Answer:
Futures in Scala represent a computation that will be completed at some point in the future. They are used to perform asynchronous programming, where you can execute tasks concurrently without blocking the main thread. Futures allow you to perform non-blocking operations, making it ideal for tasks that may take some time to complete, such as network requests or file I/O.
Using Futures in Scala for asynchronous tasks:
import scala.concurrent.{Future, ExecutionContext}
import scala.util.{Success, Failure}
import scala.concurrent.ExecutionContext.Implicits.global
// Simulate an asynchronous task that returns a result after a delay
def performTask(): Future[String] = {
Future {
Thread.sleep(2000) // Simulating a delay of 2 seconds
"Task completed successfully!"
}
}
// Callbacks for handling the result of the Future
def onSuccess(result: String): Unit = {
println(s"Task result: $result")
}
def onFailure(ex: Throwable): Unit = {
println(s"Task failed with exception: ${ex.getMessage}")
}
// Main program
def main(args: Array[String]): Unit = {
val future = performTask()
// Register callbacks for success and failure
future.onComplete {
case Success(result) => onSuccess(result)
case Failure(ex) => onFailure(ex)
}
println("Waiting for the task to complete...")
Thread.sleep(3000) // Simulating some other work while waiting for the Future to complete
}
Code language: JavaScript (javascript)
In this example, the performTask
function returns a Future that simulates an asynchronous task with a 2-second delay. We use the Future
constructor to wrap the task’s code inside a Future. The onComplete
method is used to register callbacks for handling the result of the Future. The onSuccess
callback is invoked if the Future completes successfully, and the onFailure
callback is called if the Future fails with an exception.
In the main
method, we call performTask
, and while the Future is executing, we simulate some other work with a 3-second delay. This demonstrates the non-blocking behavior of Futures, allowing other work to be done while waiting for the Future to complete.
Question:
Explain the concept of type classes in Scala and their role in providing ad-hoc polymorphism. Provide an example of using type classes in Scala.
Answer:
Type classes in Scala are a way of achieving ad-hoc polymorphism, where different data types can exhibit similar behavior without being related through a common inheritance hierarchy. Type classes enable adding behavior to existing classes without modifying their source code.
Role of type classes in providing ad-hoc polymorphism:
- Decoupling: Type classes allow adding functionality to a type without modifying it, promoting decoupling and avoiding the need for a common base class.
- Open for Extension: Type classes are open for extension, meaning new behaviors can be added for existing types without changing their definitions.
- Generic Programming: Type classes enable writing generic code that works with any type that satisfies the type class constraints, enhancing code reusability.
Example of using type classes in Scala:
// Type class definition for ordering
trait MyOrdering[A] {
def compare(x: A, y: A): Int
}
// Type class instance for ordering integers
implicit object IntOrdering extends MyOrdering[Int] {
def compare(x: Int, y: Int): Int = x - y
}
// Type class instance for ordering strings
implicit object StringOrdering extends MyOrdering[String] {
def compare(x: String, y: String): Int = x.compareTo(y)
}
// Generic function using type class
def findMax[A](list: List[A])(implicit ord: MyOrdering[A]): A = {
list.reduceLeft((x, y) => if (ord.compare(x, y) > 0) x else y)
}
val intList = List(5, 2, 8, 3)
val stringList = List("apple", "orange", "banana")
val maxInt = findMax(intList)
val maxString = findMax(stringList)
println(s"Max integer: $maxInt")
println(s"Max string: $maxString")
Code language: PHP (php)
In this example, we define a type class MyOrdering
, representing a comparison behavior. We create type class instances IntOrdering
and StringOrdering
to provide ordering capabilities for integers and
strings, respectively.
The findMax
function is a generic function that can find the maximum element in a list of any type that has a corresponding MyOrdering
instance. By using implicit parameters, Scala can automatically look up the appropriate type class instance for the given data type.
The findMax
function demonstrates how type classes enable generic programming by providing ad-hoc polymorphism, allowing the same function to work with different types as long as they satisfy the type class constraints.
Question:
Explain the concept of implicits in Scala and their role in automatic type conversions. Provide an example of using implicits for automatic type conversions.
Answer:
Implicits in Scala are a powerful feature used for automatic type conversions, parameter injection, and resolution of missing or default values. Implicits allow the compiler to insert code automatically, making the code more concise and expressive.
Role of implicits in automatic type conversions:
- Type Coercion: Implicits enable automatic type conversions between different data types when required, allowing smoother interoperability between different parts of the code.
- Implicit Parameters: Implicits can be used to define parameters that are automatically passed to functions without explicitly providing them, making function calls more concise.
- Companion Object Extensions: Implicits can be used to extend the functionality of a class by defining implicit methods in its companion object, making it possible to call those methods as if they were part of the class.
Example of using implicits for automatic type conversions:
// Define a class representing distances in meters
case class Meter(value: Double)
// Define an implicit conversion from meters to centimeters
implicit def meterToCentimeter(m: Meter): Centimeter = Centimeter(m.value * 100)
// Define a class representing distances in centimeters
case class Centimeter(value: Double)
// Function that accepts Centimeter as a parameter
def printDistanceInCentimeter(cm: Centimeter): Unit = {
println(s"Distance: ${cm.value} centimeters")
}
val distanceInMeters = Meter(5.5)
printDistanceInCentimeter(distanceInMeters) // The implicit conversion is automatically applied
In this example, we define two case classes Meter
and Centimeter
, representing distances in meters and centimeters, respectively. We define an implicit conversion from Meter
to Centimeter
by defining an implicit method meterToCentimeter
. This conversion allows Scala to automatically convert a Meter
object to a Centimeter
object when needed.
The printDistanceInCentimeter
function accepts a Centimeter
object as a parameter. When we call this function with a Meter
object, Scala automatically applies the implicit conversion defined in the companion object of Meter
, converting it to Centimeter
before passing it to the function.
Question:
Explain the concept of Algebraic Data Types (ADTs) in Scala and their significance in functional programming. Provide an example of defining and using an ADT in Scala.
Answer:
Algebraic Data Types (ADTs) in Scala are data types formed by combining other data types using algebraic operations. ADTs are crucial in functional programming as they enable modeling complex data structures with precision and type safety.
Significance of Algebraic Data Types in functional programming:
- Data Modeling: ADTs allow developers to model data structures with precision, clarity, and safety. They provide a clear definition of all possible cases or states that a data type can have.
- Pattern Matching: ADTs work seamlessly with pattern matching, enabling concise and robust code for handling different cases.
- Immutability: ADTs are usually designed to be immutable, promoting safe and concurrent programming.
Example of defining and using an ADT in Scala:
sealed trait Shape
final case class Circle(radius: Double) extends Shape
final case class Rectangle(width: Double, height: Double) extends Shape
final case class Square(side: Double) extends Shape
def area(shape: Shape): Double = shape match {
case Circle(r) => math.Pi * r * r
case Rectangle(w, h) => w * h
case Square(s) => s * s
}
val circle = Circle(2.5)
val rectangle = Rectangle(3.0, 4.0)
val square = Square(2.0)
println(s"Area of Circle: ${area(circle)}")
println(s"Area of Rectangle: ${area(rectangle)}")
println(s"Area of Square: ${area(square)}")
In this example, we define an ADT Shape
, representing different geometric shapes. The ADT is defined using the sealed trait
keyword, which ensures that all possible shapes are defined within the same file.
We create three case classes Circle
, Rectangle
, and Square
, representing specific shapes. Each case class extends the Shape
trait, and each shape has its own specific properties.
The area
function is a pattern-matching function that calculates the area of each shape based on its type. Pattern matching allows us to handle different cases of the ADT efficiently.
When we call area
with different instances of Shape
, Scala automatically determines the appropriate case and calculates the area of the specific shape, demonstrating the power of ADTs and pattern matching in functional programming.
Question:
Explain the concept of Monads in Scala and their significance in functional programming. Provide an example of using a Monad in Scala.
Answer:
Monads in Scala are a design pattern used to represent sequences of computations and manage side effects in a functional and composable way. Monads encapsulate values and computations into a container, providing a set of operations to chain, transform, and combine computations.
Significance of Monads in functional programming:
- Composition: Monads enable sequential composition of computations, providing a clean and modular approach to manage complex sequences of operations.
- Error Handling: Monads can handle error scenarios elegantly by providing mechanisms to propagate errors through the computation chain without the need for explicit error handling.
- Side Effects: Monads help manage side effects in functional programming by encapsulating the side effects within the monadic context.
Example of using a Monad in Scala:
// Option Monad for handling optional values
object OptionMonad {
def apply[A](value: A): Option[A] = Some(value)
implicit class OptionOps[A](option: Option[A]) {
def flatMap[B](f: A => Option[B]): Option[B] = option match {
case Some(value) => f(value)
case None => None
}
def map[B](f: A => B): Option[B] = option match {
case Some(value) => Option(f(value))
case None => None
}
}
}
import OptionMonad._
def divide(a: Int, b: Int): Option[Double] =
if (b != 0) Option(a.toDouble / b) else None
val result1 = divide(10, 2).flatMap(r => divide(r.toInt, 3))
val result2 = divide(10, 0).flatMap(r => divide(r.toInt, 3))
println(s"Result 1: $result1")
println(s"Result 2: $result2")
In this example, we define a simple Monad for handling optional values, called OptionMonad
. The OptionOps
class provides flatMap
and map
operations, which are essential operations for any Monad.
The divide
function
returns an Option[Double]
representing the result of the division. If the denominator is not zero, it returns the result wrapped in a Some
, otherwise, it returns None
.
We use the Monad operations flatMap
to chain two division operations, where the second operation depends on the result of the first one. The map
operation is used to perform a computation on the result without unwrapping it from the Monad.
The use of Monads in this example demonstrates how to handle optional values and chain operations gracefully, while also handling error scenarios. Monads provide a powerful abstraction for working with various computation contexts in a consistent and composable way.
1,000 Companies use CoderPad to Screen and Interview Developers
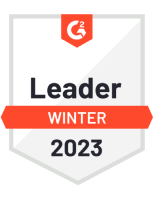
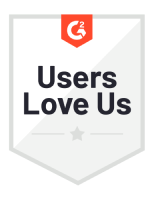
Best interview practices for Scala roles
To conduct successful Scala interviews, it’s essential to consider various factors, including the candidate’s background and the specific engineering role. To ensure a fruitful interview experience, we recommend adopting the following best practices:
- Develop technical questions that reflect real-world business scenarios within your organization. This approach will effectively engage the candidate and assist in evaluating their compatibility with your team.
- Cultivate a cooperative environment by encouraging candidates to ask questions throughout the interview.
- You’ll also want to ensure your candidates have an understanding of concurrent and parallel programming.
- Scala is very often used with Spark (a large-scale data processing engine). If needed, try to evaluate your candidates’ knowledge concerning this tool, beside their generic Scala knowledge.
Moreover, adhering to established interview procedures is critical when conducting Scala interviews. This encompasses adjusting the complexity of the questions to match the candidates’ skills, promptly informing them about the status of their application, and providing them with the opportunity to ask about the evaluation process and collaboration with you and your team.