Rust Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Rust developers for your organization.
Rust
Rust is a versatile language commonly used for systems programming and noted for its memory safety, popularity with performance-critical applications, and its interoperability with C libraries.
Rust was created by Graydon Hoare, a Mozilla employee, as a personal project starting in 2006. It was later adopted by Mozilla and announced as an official project in 2010. The first stable release, Rust 1.0, was launched on May 15, 2015.
https://doc.rust-lang.org/book/
To evaluate the Rust expertise of developers in coding interviews, we have provided hands-on coding exercises and interview questions below.
Additionally, we have outlined a set of suggested best practices to ensure that your interview questions accurately measure the candidates’ Rust skills.
Table of Contents
Rust example question
Help us design a parking lot app
Hey candidate! Welcome to your interview. Boilerplate is provided. Feel free to change the code as you see fit. To run the code at any time, please hit the run button located in the top left corner.
Goals: Design a parking lot using object-oriented principles
Here are a few methods that you should be able to run:
- Tell us how many spots are remaining
- Tell us how many total spots are in the parking lot
- Tell us when the parking lot is full
- Tell us when the parking lot is empty
- Tell us when certain spots are full e.g. when all motorcycle spots are taken
- Tell us how many spots vans are taking up
Assumptions:
- The parking lot can hold motorcycles, cars and vans
- The parking lot has motorcycle spots, car spots and large spots
- A motorcycle can park in any spot
- A car can park in a single compact spot, or a regular spot
- A van can park, but it will take up 3 regular spots
- These are just a few assumptions. Feel free to ask your interviewer about more assumptions as needed
Rust skills to assess
Jobs using Rust
Junior Rust interview questions
Question:
Explain what ownership and borrowing are in Rust and why they are essential concepts in the language.
Answer:
In Rust, ownership and borrowing are fundamental concepts that govern memory safety and eliminate data races. They are critical to ensuring that memory is managed efficiently and securely without the need for a garbage collector.
Ownership in Rust refers to the idea that every piece of data has a single owner, and there can only be one owner at a time. When the owner goes out of scope, the data is automatically deallocated, avoiding memory leaks. Ownership allows Rust to manage resources efficiently without relying on a garbage collector, making it suitable for systems programming.
Borrowing, on the other hand, is the mechanism through which Rust allows temporary access to data without taking ownership. Borrowing is essential for enabling multiple references to data without introducing data races. Rust enforces strict borrowing rules at compile time, preventing dangling pointers and concurrent access to mutable data.
Overall, ownership and borrowing work together to provide memory safety and prevent common bugs like null pointer dereferences and use-after-free errors.
Question:
The following Rust code is intended to read a file and return its contents as a string. However, it contains a logical error and doesn’t compile correctly. Identify the error and fix the code.
use std::fs::File;
use std::io::Read;
fn read_file(path: &str) -> String {
let mut file = File::open(path)?;
let mut content = String::new();
file.read_to_string(&mut content)?;
content
}
Code language: PHP (php)
Answer:
The logical error in the code is the incorrect use of the ?
operator without specifying a return type that can handle the Result
type. The correct code is as follows:
use std::fs::File;
use std::io::Read;
use std::io::Result;
fn read_file(path: &str) -> Result<String> {
let mut file = File::open(path)?;
let mut content = String::new();
file.read_to_string(&mut content)?;
Ok(content)
}
Code language: PHP (php)
In this corrected code, the function now returns a Result<String>
type, indicating that it can return either a successful String
or an error.
Question:
Explain the concept of lifetimes in Rust and how they prevent dangling references.
Answer:
Lifetimes in Rust are annotations used to track the validity of references to data. They ensure that references do not outlive the data they are pointing to, preventing dangling references.
When you have a function or a data structure that takes references as arguments, Rust requires specifying the lifetimes of those references. Lifetimes are indicated using apostrophes (e.g., 'a
), and they represent the duration for which a reference is valid.
The Rust compiler uses the lifetime annotations to perform borrow checking, which involves analyzing the lifetimes of references to ensure they don’t violate any borrowing rules. If a reference’s lifetime extends beyond the data it points to (i.e., a dangling reference), the Rust compiler will reject the code at compile time.
Lifetimes are crucial for writing safe concurrent code, as they prevent the use of references to deallocated or invalid memory.
Question:
The following Rust code is intended to find the maximum value in a vector of integers. It works, but it is not completely correct according to “Rust philosophy”. Can you find the problem and propose a better code?
fn find_max(numbers: &mut Vec<i32>) -> i32 {
let mut max = numbers[0];
for num in numbers {
if *num > max {
max = *num;
}
}
max
}
fn main() {
let mut numbers = vec![1, 20, 3];
let max = find_max(&mut numbers);
println!("{}", max);
}
Code language: JavaScript (javascript)
Answer:
The function find_max has a parameter with the “mut” keyword, to explicitly states that it could modify its content. But it is obviously not necessary when searching for the max value. Here is a fixed code, in which find_max can not modify the content of the parameter “numbers”.
fn find_max(numbers: &Vec<i32>) -> i32 {
let mut max = numbers[0];
for &num in numbers {
if num > max {
max = num;
}
}
max
}
fn main() {
let numbers = vec![1, 20, 3];
let max = find_max(&numbers);
println!("{}", max);
}
Code language: JavaScript (javascript)
Question:
Explain the concept of Option and Result in Rust and their significance in error handling.
Answer:
In Rust, Option
and Result
are two enums used for error handling and representing the presence or absence of a value.
Option<T>
represents an optional value that can either be Some(T)
to indicate that a value is present or None
to indicate the absence of a value. It is commonly used to handle situations where a function might return a value or nothing at all.
Result<T, E>
represents the outcome of a computation that may result in an error (Err(E)
) or a successful value (Ok(T)
). It is used to handle recoverable errors and to propagate errors up the call stack.
By using Option
and Result
, Rust enforces the practice of explicit error handling, preventing unexpected panics and encouraging developers to handle potential failure cases explicitly.
Question:
The following code creates an array containing 40 integers, each having a random value between 1 and 100. It works but it is not completely optimized. Is there a way to create the same array in a more efficient and idiomatic way?
use rand::Rng;
fn main() {
let mut rng = rand::thread_rng();
// Create an array of 40 numbers
let mut numbers = [0; 40];
// Initialize each element with a random value, between 1 and 100.
for num in numbers.iter_mut() {
*num = rng.gen_range(1..101);
}
println!("{:?}", numbers);
}
Code language: PHP (php)
Answer:
The array is first initialized with 40 zeros, by the instruction “numbers = [0; 40];”. Then, its content is modified with the randomly generated values. The first initialization is useless. But in Rust, it is not advisable to create an uninitialized array and fill it later. The best solution is to directly create the array with its random values. It can be done with the “from_fn” function. You may note that in the fixed code, the variable “numbers” is declared without the “mut” keyword. It is no longer necessary, as we do not have the intention to modify its content after the first initialization.
use rand::Rng;
fn main() {
let mut rng = rand::thread_rng();
let numbers: [usize; 40] = core::array::from_fn(|_| rng.gen_range(1..101));
println!("{:?}", numbers);
}
Code language: PHP (php)
Question:
Explain the concept of ownership and borrowing in the context of managing mutable data in Rust.
Answer:
In Rust, ownership and borrowing play a significant role in managing mutable data efficiently and safely.
Ownership ensures that a piece of data has a single owner at any given time. When a value is owned by a variable, that variable has exclusive control over the data. When the owner goes out of scope, Rust automatically deallocates the data, preventing memory leaks. To mutate data, you must have ownership of the data.
Borrowing, on the other hand, allows temporary and read-only access to data without transferring ownership. Borrowing allows multiple references to access the data simultaneously, but only one mutable reference can be active at a time. Borrowing is enforced through strict rules at compile-time, which prevent data races and other memory safety issues.
By combining ownership and borrowing, Rust enables safe and efficient mutation of data without the need for a garbage collector.
Question:
The following Rust code is intended to create a new vector containing only the even numbers from the original vector. However, it contains a logical error and doesn’t produce
the correct result. Identify the error and fix the code.
fn filter_even_numbers(numbers: &Vec<i32>) -> Vec<i32> {
let even_numbers = numbers.iter().filter(|&num| num % 2 == 0);
even_numbers.collect();
}
Code language: HTML, XML (xml)
Answer:
The logical error in the code is that the filter()
method returns an iterator, not a vector. The correct code is as follows:
fn filter_even_numbers(numbers: &Vec<i32>) -> Vec<i32> {
let even_numbers: Vec<i32> = numbers.iter().filter(|&num| num % 2 == 0).copied().collect();
even_numbers
}
Code language: HTML, XML (xml)
In this corrected code, the filter()
method is combined with the copied()
method to obtain an iterator of references, which is then collected into a new Vec<i32>
containing the even numbers.
Question:
Explain the concept of lifetimes in function parameters and return values, and how they relate to borrowing.
Answer:
In Rust, lifetimes are annotations used to specify the relationship between the lifetimes of references and the data they refer to. Lifetimes are essential for functions that take references as parameters and return references as values.
When a function takes references as parameters, the lifetimes of the input references must be explicitly specified to ensure that the references are valid for the entire duration of the function call. This prevents the function from using references that may go out of scope before the function finishes execution.
Similarly, when a function returns a reference as its output, the lifetime of the returned reference must be connected to the lifetime of the data used to generate it. This ensures that the returned reference remains valid after the function call.
By annotating lifetimes, Rust’s borrow checker can enforce strict rules to prevent dangling references, data races, and other memory safety issues. Lifetimes help ensure that references are used safely and consistently throughout the codebase.
Question:
The following Rust code is intended to concatenate two strings and return the result. However, it contains a logical error and doesn’t compile correctly. Identify the error and fix the code.
fn concatenate_strings(s1: &str, s2: &str) -> &str {
let result = format!("{}{}", s1, s2);
&result
}
Code language: JavaScript (javascript)
Answer:
The logical error in the code is that the result
variable is a local variable whose lifetime ends at the end of the function, so returning a reference to it would lead to a dangling reference. The correct code is as follows:
fn concatenate_strings(s1: &str, s2: &str) -> String {
let result = format!("{}{}", s1, s2);
result
}
Code language: JavaScript (javascript)
In this corrected code, the function returns the concatenated String
directly instead of trying to return a reference to a local variable. This ensures that the returned value is valid beyond the function call.
Intermediate Rust interview questions
Question:
Explain ownership, borrowing, and lifetimes in Rust, and how they help prevent memory-related bugs.
Answer:
In Rust, ownership, borrowing, and lifetimes are key concepts that help ensure memory safety and prevent common memory-related bugs like null pointer dereferences, use-after-free, and data races.
Ownership: In Rust, each value has a unique owner, and there can only be one owner at a time. When a value goes out of scope, Rust automatically calls the drop
function to deallocate the memory associated with that value. Ownership ensures that memory is properly managed, and there are no dangling references.
Borrowing: Instead of transferring ownership, Rust allows borrowing references to values. Borrowing allows multiple read-only references (&T
) or a single mutable reference (&mut T
) to exist alongside the original owner. Borrowing is subject to strict rules that prevent simultaneous mutable and immutable references, ensuring data consistency and preventing data races.
Lifetimes: Lifetimes are annotations that define the scope for which a borrow is valid. They help the Rust compiler ensure that borrowed references do not outlive the data they refer to. By specifying lifetimes, Rust enforces that borrowed references are valid for as long as they are used, preventing use-after-free errors.
Together, ownership, borrowing, and lifetimes make up Rust’s ownership system, which provides compile-time guarantees for memory safety without relying on garbage collection or runtime checks.
Question:
Explain the difference between Vec<T>
and Box<T>
in Rust and when you would choose one over the other.
Answer:Vec<T>
and Box<T>
are both used to manage memory and store data in Rust, but they have different purposes and use cases.
Vec<T>
: Vec<T>
is a dynamic array or a growable array, represented by the Vec
type. It can hold a variable number of elements of type T
. The Vec
type is stored on the heap and automatically resizes itself when elements are added or removed. Ownership of the Vec
is typically managed through ownership and borrowing.
Use Vec<T>
when you need a collection of elements whose size may change dynamically during runtime. For example, use a Vec<String>
when you want to store a list of strings with an unknown number of entries.
Box<T>
: Box<T>
is a smart pointer that points to data stored on the heap. It provides a way to allocate memory on the heap and maintain a single owner for the data. When the Box
goes out of scope, its destructor is called, and the memory it points to is deallocated. Box
is used to store a single value and is useful when you need to allocate memory that has a fixed size and should have a clear ownership model.
Use Box<T>
when you need to store a single value on the heap, and you want to ensure that it is deallocated automatically when it goes out of scope. For example, use Box<i32>
when you want to store an integer on the heap and access it through a smart pointer with clear ownership semantics.
Question:
Explain how error handling is done in Rust, and compare the use of Result
and Option
for different scenarios.
Answer:
In Rust, error handling is a first-class language feature, and it encourages developers to handle errors explicitly. The two primary types used for error handling are Result<T, E>
and Option<T>
.
Result<T, E>
: The Result<T, E>
type represents either a successful value of type T
or an error of type E
. It is commonly used when an operation can result in either success or failure. Rust forces developers to handle the possible error cases, either through pattern matching with match
or by using combinators like unwrap()
(which will panic if the Result
is an Err
). This ensures that errors are explicitly addressed and not ignored.
Use Result<T, E>
when an operation can fail, and you want to communicate the error to the caller or handle it gracefully within the function.
Option<T>
: The Option<T>
type represents an optional value that can be either Some(T)
(a value is present) or None
(no value is present). It is commonly used when a function can return a valid value or nothing (null-like scenario). Rust encourages developers to handle the possibility of a None
case, either through pattern matching with match
or by using combinators like unwrap()
(which will panic if the Option
is a None
).
Use Option<T>
when a function may not be able to return a valid value in some scenarios, and you want to avoid null pointers or “null reference” errors.
In summary, use Result<T, E>
for operations that can fail with specific error information, and use Option<T>
for scenarios where a value may or may not be present (nullable values).
Question:
Explain the concept of lifetimes and how they are used in function signatures and data structures in Rust.
Answer:
Lifetimes in Rust are annotations that describe the relationships between references in the code and help the compiler ensure memory safety and prevent dangling references.
In function signatures, lifetimes specify how long a reference argument must live in relation to other reference arguments and the return value. This helps Rust ensure that borrowed references do not outlive the data they point to. Lifetimes are denoted by names preceded by an apostrophe ('
). For example:
fn foo<'a>(x: &'a i32, y: &'a i32) -> &'a i32 {
if x > y {
x
} else {
y
}
}
Code language: JavaScript (javascript)
In this example, the lifetime 'a
is used to specify that the returned reference must live at least as long as both x
and y
.
Lifetimes are also used in data structures to define the relationships between references stored in the structure. For example, in a struct
:
struct MyStruct<'a> {
data: &'a i32,
}
Code language: HTML, XML (xml)
In this case, the MyStruct
has a lifetime parameter 'a
, which means that any reference stored in data
must live at least as long as the instance of MyStruct
.
By using lifetimes, Rust ensures that borrowed references remain valid for as long as they are used and helps prevent common memory-related bugs like use-after-free and data races.
Question:
Explain the concept of ownership and borrowing rules in Rust and how they contribute to memory safety.
Answer:
Ownership and borrowing rules in Rust are key features that ensure memory safety and prevent common bugs like data races and null pointer dereferences.
Ownership Rules:
- Each value in Rust has a unique owner, and there can be only one owner at a time.
- When the owner of a value goes out of scope, Rust automatically calls the
drop
function to deallocate the memory associated with the value. This ensures that memory is released appropriately and there are no memory leaks. - Ownership can be transferred using move semantics, where ownership of a value is moved from one variable to another. This prevents multiple variables from accessing the same data at the same time, avoiding data races.
Borrowing Rules:
- Instead of transferring ownership, Rust allows borrowing references to values. Borrowing is used to enable multiple read-only references (
&T
) or a single mutable reference (&mut T
) to coexist alongside the original owner. - Borrowed references have a limited lifetime and cannot outlive the data they point to. This prevents dangling references and use-after-free bugs.
- Rust enforces strict rules for mutable references, allowing only one mutable reference at a time. This prevents data races because it ensures exclusive access to mutable data.
By combining ownership and borrowing rules, Rust’s compiler can perform compile-time checks to ensure that memory is correctly managed and accessed safely. These rules eliminate the need for garbage collection or runtime checks, providing memory safety without sacrificing performance.
Question:
Explain the async
and await
keywords in Rust and how they are used for asynchronous programming.
Answer:
The async
and await
keywords in Rust are used to define and work with asynchronous code, enabling asynchronous programming without blocking threads.
async
: The async
keyword is used to define an asynchronous function. An asynchronous function returns a future, which represents a computation that may not have completed yet. The async
keyword is used before the function signature, indicating that the function can be paused and resumed without blocking the thread.
await
: The await
keyword is used within an asynchronous function to await the completion of a future. When await
is encountered, the function will pause its execution and allow the event loop to perform other tasks. When the awaited future completes, the function resumes from where it left off.
Example of using async
and await
:
use tokio::time::Duration;
async fn my_async_function() {
println!("Starting asynchronous operation...");
tokio::time::sleep(Duration::from_secs(2)).await;
println!("Asynchronous operation completed!");
}
Code language: PHP (php)
In this example, the tokio::time::sleep()
function returns a future, which is awaited using await
. While waiting for the future to complete, the asynchronous function can perform other tasks without blocking the thread.
To run asynchronous functions, you need to use a runtime like tokio
or async-std
, which provides an event loop to manage asynchronous tasks.
Asynchronous programming in Rust allows you to efficiently handle I/O-bound tasks, such as network communication or file operations, without the need for multiple threads, leading to more scalable and performant applications.
Question:
Explain how Rust ensures thread safety and prevents data races in concurrent code.
Answer:
Rust ensures thread safety and prevents data races through its ownership and borrowing model, enforced by the borrow checker and the Sync
and Send
marker traits.
- Ownership and Borrowing: Rust’s ownership model enforces strict rules for mutable references, allowing only one mutable reference (
&mut T
) or multiple read-only references (&T
) to access data at any given time. This prevents data races because it ensures exclusive access to mutable data, and read-only access is safe and doesn’t interfere with other read-only accesses. - Borrow Checker: The Rust compiler has a borrow checker that analyzes the lifetimes of references to ensure that references are used safely and do not outlive the data they point to. The borrow checker disallows dangling references and ensures that borrowed references remain valid for as long as they are used, preventing use-after-free bugs.
Sync
andSend
Traits: Rust has two marker traits,Sync
andSend
, to ensure that types are thread-safe. A type implementingSync
indicates that it can be safely shared between threads, and a type implementingSend
indicates that it can be safely moved between threads. The compiler enforces that types implement these traits appropriately to prevent concurrent access to non-thread-safe data.Mutex
andArc
: To safely share mutable data between threads, Rust provides theMutex
(Mutual Exclusion) andArc
(Atomic Reference Counting) types.Mutex
ensures exclusive access to the data by only allowing one thread to hold the lock at a time, whileArc
provides shared ownership of data with atomic reference counting to prevent data races.
By leveraging these language features and constructs, Rust provides compile-time guarantees for thread safety and prevents data races, eliminating many common pitfalls of concurrent programming.
Question:
Explain the concept of lifetimes and how they are used in function signatures and data structures in Rust.
Answer:
Lifetimes in Rust are annotations that describe the relationships between references in the code and help the compiler ensure memory safety and prevent dangling references.
In function signatures, lifetimes specify how long a reference argument must live in relation to other reference arguments and the return value. This helps Rust ensure that borrowed references do not outlive the data they point to. Lifetimes are denoted by names preceded by an apostrophe ('
). For example:
fn foo<'a>(x: &'a i32, y: &'a i32) -> &'a i32 {
if x > y {
x
} else {
y
}
}
Code language: JavaScript (javascript)
In this example, the lifetime 'a
is used to specify that the returned reference must live at least as long as both x
and y
.
Lifetimes are also used in data structures to define the relationships between references stored in the structure. For example, in a struct
:
struct MyStruct<'a> {
data: &'a i32,
}
Code language: HTML, XML (xml)
In this case, the MyStruct
has a lifetime parameter 'a
, which means that any reference stored in data
must live at least as long as the instance of MyStruct
.
By using lifetimes, Rust ensures that borrowed references remain valid for as long as they are used and helps prevent common memory-related bugs like use-after-free and data races.
Question:
Explain the async
and await
keywords in Rust and how they are used for asynchronous programming.
Answer:
The async
and await
keywords in Rust are used to define and work with asynchronous code, enabling asynchronous programming without blocking threads.
async
: The async
keyword is used to define an asynchronous function. An asynchronous function returns a future, which represents a computation that may not have completed yet. The async
keyword is used before the function signature, indicating that the function can be paused and resumed without blocking the thread.
await
: The await
keyword is used within an asynchronous function to await the completion of a future. When await
is encountered, the function will pause its execution and allow the event loop to perform other tasks. When the awaited future completes, the function resumes from where it left off.
Example of using async
and await
:
use tokio::time::Duration;
async fn my_async_function() {
println!("Starting asynchronous operation...");
tokio::time::sleep(Duration::from_secs(2)).await;
println!("Asynchronous operation completed!");
}
Code language: PHP (php)
In this example, the tokio::time::sleep()
function returns a future, which is awaited using await
. While waiting for the future to complete, the asynchronous function can perform other tasks without blocking the thread.
To run asynchronous functions, you need to use a runtime like tokio
or async-std
, which provides an event loop to manage asynchronous tasks.
Asynchronous programming in Rust allows you to efficiently handle I/O-bound tasks, such as network communication or file operations, without the need for multiple threads, leading to more scalable and performant applications.
Question:
Explain how Rust ensures thread safety and prevents data races in concurrent code.
Answer:
Rust ensures thread safety and prevents data races through its ownership and borrowing model, enforced by the borrow checker and the Sync
and Send
marker traits.
- Ownership and Borrowing: Rust’s ownership model enforces strict rules for mutable references, allowing only one mutable reference (
&mut T
) or multiple read-only references (&T
) to access data at any given time. This prevents data races because it ensures exclusive access to mutable data, and read-only access is safe and doesn’t interfere with other read-only accesses. - Borrow Checker: The Rust compiler has a borrow checker that analyzes the lifetimes of references to ensure that references are used safely and do not outlive the data they point to. The borrow checker disallows dangling references and ensures that borrowed references remain valid for as long as they are used, preventing use-after-free bugs.
Sync
andSend
Traits: Rust has two marker traits,Sync
andSend
, to ensure that types are thread-safe. A type implementingSync
indicates that it can be safely shared between threads, and a type implementingSend
indicates that it can be safely moved between threads. The compiler enforces that types implement these traits appropriately to prevent concurrent access to non-thread-safe data.Mutex
andArc
: To safely share mutable data between threads, Rust provides theMutex
(Mutual Exclusion) andArc
(Atomic Reference Counting) types.Mutex
ensures exclusive access to the data by only allowing one thread to hold the lock at a time, whileArc
provides shared ownership of data with atomic reference counting to prevent data races.
By leveraging these language features and constructs, Rust provides compile-time guarantees for thread safety and prevents data races, eliminating many common pitfalls of concurrent programming.
Senior Rust interview questions
Question:
Explain ownership, borrowing, and lifetimes in Rust and how they contribute to memory safety.
Answer:
In Rust, ownership, borrowing, and lifetimes are fundamental concepts that enable memory safety without the need for a garbage collector.
Ownership: Every value in Rust has a unique owner, which is responsible for deallocating the memory when the owner goes out of scope. When a value is assigned to another variable or passed to a function, the ownership is transferred, and the previous owner no longer has access to that value. This prevents double frees or accessing memory after it has been deallocated, ensuring memory safety.
Borrowing: Instead of transferring ownership, Rust allows borrowing references to values. Borrowing enables temporary access to a value without taking ownership. There are two types of borrows: immutable borrows (&T
) and mutable borrows (&mut T
). Borrowing prevents data races and enforces a single-writer, multiple-reader (SWMR) model, ensuring thread safety.
Lifetimes: Lifetimes ensure that references remain valid and don’t outlive the data they point to. Rust’s borrow checker analyzes the lifetimes of references to prevent dangling or invalid references, guaranteeing memory safety at compile-time.
Together, these concepts help enforce strict rules at compile-time, preventing common memory-related bugs like null pointer dereferences, use-after-free, and data races, making Rust a memory-safe language.
Question:
The following Rust code is intended to read lines from a file and print the longest line. However, it contains a logical error and doesn’t compile. Identify the error and fix the code.
use std::fs::File;
use std::io::{BufRead, BufReader};
fn main() {
let file = File::open("data.txt").expect("Failed to open the file");
let reader = BufReader::new(file);
let mut longest_line = "";
for line in reader.lines() {
if line.len() > longest_line.len() {
longest_line = line;
}
}
println!("Longest line: {}", longest_line);
}
Code language: PHP (php)
Answer:
The logical error in the code is that longest_line
is initialized as an empty string (""
), which is an immutable reference to a string slice. We need to use an owned String
to hold the longest line. Here’s the corrected code:
use std::fs::File;
use std::io::{BufRead, BufReader};
fn main() {
let file = File::open("data.txt").expect("Failed to open the file");
let reader = BufReader::new(file);
let mut longest_line = String::new();
for line in reader.lines() {
if let Ok(line) = line {
if line.len() > longest_line.len() {
longest_line = line;
}
}
}
println!("Longest line: {}", longest_line);
}
Code language: JavaScript (javascript)
In this corrected code, longest_line
is now a mutable String
, and we use String::new()
to create an empty String
. Additionally, we use if let Ok(line) = line
to handle the Result returned by reader.lines()
, allowing us to access the content of the line safely.
Question:
Explain the concept of lifetimes in Rust and how they prevent dangling references.
Answer:
Lifetimes in Rust are annotations used to ensure that references to data remain valid for the entire duration they are being used. They prevent dangling references, which occur when a reference points to memory that has been deallocated or has gone out of scope.
In Rust, every reference has a lifetime, which describes the period during which the reference is valid. Lifetimes are denoted by apostrophes (‘a) and are associated with references and function signatures.
For example:
fn longest<'a>(x: &'a str, y: &'a str) -> &'a str {
if x.len() > y.len() {
x
} else {
y
}
}
Code language: JavaScript (javascript)
In this example, the function longest()
takes two string slices (&str
) as input arguments and returns a string slice with the same lifetime as the input references. The 'a
in the function signature denotes the lifetime parameter, stating that both input references and the return value must have the same lifetime 'a
.
By using lifetimes, Rust’s borrow checker can ensure that references don’t outlive the data they point to. This prevents dangling references because the compiler ensures that references are valid for the entire duration they are used, thereby guaranteeing memory safety.
Question:
The following Rust code is intended to find the unique elements in a vector. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
fn unique_elements(vec: Vec<i32>) -> Vec<i32> {
let mut unique_vec: Vec<i32> = vec![];
for &num in &vec {
if !unique_vec.contains(&num) {
unique_vec.push(num);
}
}
unique_vec
}
fn main() {
let vec = vec![1, 2, 3, 1, 2, 4, 5];
let unique_vec = unique_elements(vec);
println!("{:?}", unique_vec);
}
Code language: JavaScript (javascript)
Answer:
The logical error in the code is that the contains()
method is not comparing the elements properly. The elements in the unique_vec
are added as references (&num
), but when checking for containment, we should use the actual value of num
. Here’s the corrected code:
fn unique_elements(vec: Vec<i32>) -> Vec<i32> {
let mut unique_vec: Vec<i32> = vec![];
for num in vec {
if !unique_vec.contains(&num) {
unique_vec.push(num);
}
}
unique_vec
}
fn main() {
let vec = vec![1, 2, 3, 1, 2, 4, 5];
let unique_vec = unique_elements(vec);
println!("{:?}", unique_vec);
}
Code language: JavaScript (javascript)
In this corrected code, we remove the ampersand (&
) from the for
loop to directly move the elements from the original vector (vec
) into the unique_vec
. Additionally, when checking for containment, we use &num
to compare the reference to num
with the elements in unique_vec
.
Question:
What is the difference between Box<T>
, Rc<T>
, and Arc<T>
in Rust? When would you use each of them?
Answer:Box<T>
, Rc<T>
, and Arc<T>
are all smart pointers in Rust, but they have different use cases and behavior.
Box<T>
: A Box<T>
is a simple smart pointer that allows allocating data on the heap and provides ownership with fixed-size allocation. It is used to store data with a known, fixed size that needs to be transferred across ownership boundaries. Box<T>
is the most efficient and lightweight smart pointer, suitable for single-threaded scenarios.
Rc<T>
: An Rc<T>
(Reference Counted) is used for reference counting and shared ownership
across multiple ownership locations. It keeps track of the number of references to the data and deallocates the data when the last reference is dropped. It allows multiple immutable references (&T
) to the same data, but it cannot be used in multithreaded scenarios due to the lack of thread safety.
Arc<T>
: An Arc<T>
(Atomic Reference Counted) is similar to Rc<T>
but provides atomic reference counting, making it suitable for concurrent scenarios. It ensures thread safety and allows multiple threads to have shared ownership of the same data. Arc<T>
uses atomic operations to track the reference count and synchronize access, making it safe to share data across threads.
Use Box<T>
when you need single ownership and fixed-size allocation on the heap. Use Rc<T>
when you need shared ownership without thread safety and Arc<T>
when you need shared ownership with thread safety for concurrent access.
Question:
The following Rust code is intended to implement a simple concurrent task using threads. However, it contains a logical error and doesn’t work as expected. Identify the error and fix the code.
use std::thread;
fn main() {
let mut data = vec![1, 2, 3, 4, 5];
for i in 0..data.len() {
thread::spawn(|| {
data[i] *= 2;
});
}
thread::sleep(std::time::Duration::from_secs(2));
println!("{:?}", data);
}
Code language: PHP (php)
Answer:
The logical error in the code is that the closure passed to thread::spawn()
borrows data
immutably (&mut data
), which is not allowed as multiple threads are trying to access it concurrently. We should use move
to transfer ownership of data
to each thread. Here’s the corrected code:
use std::thread;
fn main() {
let mut data = vec![1, 2, 3, 4, 5];
for i in 0..data.len() {
thread::spawn(move || {
data[i] *= 2;
});
}
thread::sleep(std::time::Duration::from_secs(2));
println!("{:?}", data);
}
Code language: PHP (php)
In this corrected code, we use move
before the closure (move || { ... }
) to transfer ownership of data
to each thread. This ensures that each thread has its own copy of data
, allowing them to safely modify it in parallel.
Sure, here are the remaining four questions:
Question:
Explain the concept of lifetimes in relation to function arguments and return values. How can you specify lifetimes in function signatures?
Answer:
Lifetimes in Rust are used to track the validity of references and ensure that they remain valid for the entire duration they are being used. Lifetimes come into play when functions accept references as arguments or return references.
In function signatures, lifetimes are denoted by apostrophes (‘a) and are used as lifetime parameters. By specifying lifetimes in function signatures, you indicate that the references passed as arguments or returned from the function must have a certain lifetime that is tied to the lifetime of other references in the function.
For example:
fn get_longest<'a>(x: &'a str, y: &'a str) -> &'a str {
if x.len() > y.len() {
x
} else {
y
}
}
Code language: JavaScript (javascript)
In this example, the function get_longest()
takes two string slices (&str
) as input arguments and returns a string slice with the same lifetime as the input references. The lifetime parameter 'a
in the function signature indicates that both input references and the return value must have the same lifetime 'a
.
By specifying lifetimes, Rust’s borrow checker ensures that references remain valid for the entire duration they are used, preventing dangling references and ensuring memory safety.
Question:
The following Rust code is intended to read integers from the console and store them in a vector until the user enters 0. However, it contains a logical error and doesn’t work as expected. Identify the error and fix the code.
use std::io;
fn main() {
let mut numbers = Vec::new();
loop {
let mut input = String::new();
io::stdin().read_line(&mut input).expect("Failed to read input");
let number: i32 = input.trim().parse().expect("Invalid input");
if number == 0 {
break;
}
numbers.push(number);
}
println!("{:?}", numbers);
}
Code language: JavaScript (javascript)
Answer:
The logical error in the code is that the read_line()
method also reads the newline character (n
) when the user presses Enter after entering the number. This newline character is parsed as an invalid input, causing the program to panic. To fix the code, we need to remove the newline character before parsing the number. Here’s the corrected code:
use std::io;
fn main() {
let mut numbers = Vec::new();
loop {
let mut input = String::new();
io::stdin().read_line(&mut input).expect("Failed to read input");
let number: i32 = match input.trim().parse() {
Ok(0) => break,
Ok(num) => num,
Err(_) => {
println!("Invalid input. Please enter an integer.");
continue;
}
};
numbers.push(number);
}
println!("{:?}", numbers);
}
Code language: PHP (php)
In this corrected code, we use a match
statement to handle the parsing result. If the user enters 0, we break out of the loop. If the parsing succeeds, the valid number is pushed into the numbers
vector. If the parsing fails (e.g., when the input is not an integer), we print an error message and continue the loop to ask the user for a new input.
Question:
What are the key differences between Rust and C++? As a senior Rust developer, how would you advocate for choosing Rust over C++ for a project?
Answer:
Rust and C++ are both systems programming languages that aim to provide performance, low-level control, and memory safety. However, they have several key differences:
- Memory Safety: Rust enforces strict memory safety guarantees at compile-time through its ownership and borrowing system and lifetimes. C++, on the other hand, relies on developers to manage memory manually, making it more prone to memory-related bugs like null pointer dereferences and use-after-free.
- Ownership Model: Rust’s ownership model ensures that each value has a unique owner and prevents data races in concurrent scenarios. C++ uses a combination of smart pointers and raw pointers for memory management, which can be error-prone and lead to memory leaks.
- Concurrency: Rust has built-in support for safe concurrency through its
async/await
model andArc<T>
for shared ownership across threads. C++ requires third-party libraries or manual implementation for similar functionality, making concurrent programming in C++ more challenging. - Compilation Speed: Rust’s borrow checker and strict type system may lead to slower compilation times compared to C++. However, this trade-off ensures safety and eliminates certain classes of bugs during development.
- Ecosystem: C++ has a mature and extensive ecosystem with numerous libraries and frameworks developed over several decades. Rust’s ecosystem is rapidly growing but might not yet have the same level of maturity and breadth as C++.
As a senior Rust developer, advocating for choosing Rust over C++ for a project can be based on the following points:
- Memory Safety: Rust’s strict memory safety guarantees eliminate entire classes of bugs, reducing the risk of security vulnerabilities and providing greater confidence in the codebase’s reliability.
- Concurrency and Parallelism: Rust’s built-in concurrency support makes it easier to write safe and efficient concurrent programs, making it an excellent choice for projects with high-performance requirements.
- Future-Proofing: Rust’s focus on safety and maintainability can lead to a more maintainable codebase in the long run, reducing technical debt and making it easier to extend and evolve the project.
- Developer Productivity: Rust’s strong type system and expressive syntax can improve developer productivity by catching errors at compile-time, leading to faster development cycles.
- Growing Community: Rust’s community is highly active and collaborative, with a growing number of libraries and tools that continue to enhance the language’s capabilities and ecosystem.
Ultimately, the decision
between Rust and C++ depends on the specific requirements and constraints of the project. Rust excels in projects that prioritize safety, concurrency, and long-term maintainability, while C++ remains a solid choice for well-established projects with a significant existing C++ codebase and when low-level control and performance are paramount.
Question:
The following Rust code is intended to implement a simple function that checks if a given string is a palindrome. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
fn is_palindrome(s: &str) -> bool {
s.chars().eq(s.chars().rev())
}
fn main() {
let word = "level";
if is_palindrome(word) {
println!("'{}' is a palindrome.", word);
} else {
println!("'{}' is not a palindrome.", word);
}
}
Code language: JavaScript (javascript)
Answer:
The logical error in the code is that s.chars().rev()
creates an iterator over the characters of the string in reverse order, but it doesn’t collect the reversed characters into a new string. Therefore, eq()
is comparing two iterators, not the actual reversed string. To fix the code, we need to collect the reversed characters into a new string before comparing. Here’s the corrected code:
fn is_palindrome(s: &str) -> bool {
let reversed: String = s.chars().rev().collect();
s == reversed
}
fn main() {
let word = "level";
if is_palindrome(word) {
println!("'{}' is a palindrome.", word);
} else {
println!("'{}' is not a palindrome.", word);
}
}
Code language: JavaScript (javascript)
In this corrected code, s.chars().rev().collect()
collects the reversed characters into a new String
, and then we use the ==
operator to compare the original string s
with the reversed string. This properly checks whether the input string is a palindrome.
1,000 Companies use CoderPad to Screen and Interview Developers
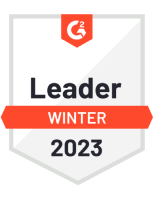
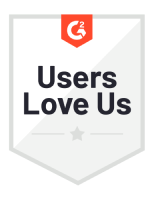
Interview best practices for Rust roles
For successful Rust interviews, it’s important to consider various factors, such as the candidates’ experience level and the specific engineering role they’re applying for. To ensure that your Rust interview questions yield the best results, we recommend adhering to the following best practices when interacting with candidates:
- Devise technical questions that correspond to real-world scenarios within your organization. This approach will not only engage the candidate but also allow you to better evaluate their fit for your team.
- Encourage candidates to ask questions during the interview and cultivate a cooperative atmosphere.
- You may want to consider assessing the candidate’s knowledge of popular Rust libraries like Tokio, Serde, and Actix/Rocket.
Furthermore, it’s crucial to observe standard interview practices when conducting Rust interviews. This includes adjusting the question difficulty based on the candidate’s skill level, providing timely feedback on their application status, and allowing candidates to ask about the assessment or collaborate with you and your team.