R Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified R developers for your organization.
R
Popular in the statistical computing and graphics domain, R has a very active open source community and strong data visualization library that makes it popular in academic and scientific applications.
By statisticians, for statisticians — R was built specifically for statistical work by Ross Ihaka and Robert Gentleman of the University of Auckland, New Zealand in the early 1990’s.
http://www.stat.auckland.ac.nz/~ihaka/downloads/JCGS-1996.pdf
We have developed practical coding assignments and interview questions tailored to evaluate developers’ R skills during coding interviews. Furthermore, we have compiled a set of best practices to ensure that your interview questions accurately assess the candidates’ proficiency in R.
Table of Contents
R example question
Help us design a parking lot
Hey candidate! Welcome to your interview. Boilerplate is provided. Feel free to change the code as you see fit. To run the code at any time, please hit the run button located in the top left corner.
Goals: Design a parking lot using object-oriented principles
Here are a few methods that you should be able to run:
- Tell us how many spots are remaining
- Tell us how many total spots are in the parking lot
- Tell us when the parking lot is full
- Tell us when the parking lot is empty
- Tell us when certain spots are full e.g. when all motorcycle spots are taken
- Tell us how many spots vans are taking up
Assumptions:
- The parking lot can hold motorcycles, cars and vans
- The parking lot has motorcycle spots, car spots and large spots
- A motorcycle can park in any spot
- A car can park in a single compact spot, or a regular spot
- A van can park, but it will take up 3 regular spots
- These are just a few assumptions. Feel free to ask your interviewer about more assumptions as needed
R skills to assess
Jobs using R
Junior R interview questions
Question:
What is R and what is it commonly used for in data analysis and statistical computing?
Answer:
R is a programming language and environment specifically designed for statistical computing and data analysis. It provides a wide range of statistical and graphical techniques and is widely used in fields such as data science, biostatistics, finance, and social sciences. R provides extensive libraries and packages that allow for data manipulation, statistical modeling, visualization, and machine learning tasks.
Question:
Explain the concept of vectors in R. How are vectors created and manipulated? Provide an example demonstrating the usage of vector operations in R.
Answer:
In R, a vector is a basic data structure that stores elements of the same data type. Vectors can be created using the c()
function or by using the :
operator for creating sequences. For example, vec <- c(1, 2, 3, 4, 5)
creates a numeric vector with elements 1, 2, 3, 4, and 5.
Vector elements can be accessed using indexing, such as vec[2]
to access the second element. Vector operations, such as addition, subtraction, multiplication, and division, can be performed element-wise. For example, vec + 2
adds 2 to each element of the vector.
Question:
What are data frames in R? How do they differ from matrices? Provide an example demonstrating the creation and manipulation of data frames in R.
Answer:
A data frame in R is a two-dimensional tabular data structure that stores data in rows and columns, similar to a table in a database or a spreadsheet. It allows for the storage and manipulation of heterogeneous data types, such as numeric, character, and factor variables.
Data frames can be created using the data.frame()
function by combining vectors of equal length. For example, df <- data.frame(name = c("John", "Alice", "Bob"), age = c(25, 30, 35))
creates a data frame with two columns: “name” and “age”.
Data frames can be manipulated using various functions such as subset()
, filter()
, and mutate()
from the dplyr
package. These functions enable data selection, filtering, and transformation operations on data frames.
Question:
Explain the concept of control structures in R. What are the different types of control structures, and when would you use each type? Provide examples demonstrating the usage of control structures in R.
Answer:
Control structures in R allow for controlling the flow of execution in a program. The main types of control structures in R are:
if
statements: Used to perform conditional execution of code based on a condition. For example:
if (x > 0) {
print("x is positive")
} else if (x < 0) {
print("x is negative")
} else {
print("x is zero")
}
Code language: PHP (php)
for
loops: Used to iterate over a sequence or a vector. For example:
for (i in 1:5) {
print(i)
}
Code language: PHP (php)
while
loops: Used to repeatedly execute a block of code while a condition is true. For example:
i <- 1
while (i <= 5) {
print(i)
i <- i + 1
}
Code language: PHP (php)
repeat
loops: Used to create an infinite loop that can be terminated using thebreak
statement. For example:
i <- 1
repeat {
print(i)
i <- i + 1
if (i > 5) {
break
}
}
Code language: PHP (php)
switch
statements: Used to select one of several code blocks to execute based on the value of an expression. For example:
day <- "Monday"
switch(day,
"Monday" = print("Start of the week"),
"Friday" = print("End of the week"),
print("Middle of the week"))
Code language: PHP (php)
Control structures allow for making decisions, iterating over data, and creating flexible code execution paths in R programs.
Question:
What are the primary data types in R? Explain the characteristics and usage of each data type, including numeric, character, logical, and factor.
Answer:
In R, the primary data types include:
- Numeric: Used to represent numeric values such as integers and floating-point numbers. Numeric values can be operated on using arithmetic operations.
- Character: Used to represent text strings. Character values are enclosed in quotes (” or “”). String manipulation functions can be used to modify and process character values.
- Logical: Used to represent boolean values (
TRUE
orFALSE
). Logical values are commonly used for conditional operations and comparisons. - Factor: Used to represent categorical variables with a limited number of distinct values. Factors are useful for data analysis and modeling. They can have levels that define the distinct values and their ordering.
Each data type has specific characteristics and is used in different contexts based on the type of data being handled.
Question:
Explain the concept of functions in R. How are functions defined and called? Provide an example demonstrating the creation and usage of a user-defined function in R.
Answer:
Functions in R are reusable blocks of code that perform specific tasks. They can take inputs (arguments) and return outputs (values or objects). Functions provide modularity and help organize code into logical units.
Functions in R are defined using the function()
keyword followed by the function name and arguments. For example:
# Function definition
addNumbers <- function(x, y) {
result <- x + y
return(result)
}
# Function call
sum <- addNumbers(3, 5)
print(sum) # Output: 8
Code language: PHP (php)
In this example, the addNumbers()
function takes two arguments x
and y
, adds them together, and returns the result. The function is called with arguments 3
and 5
, and the returned value is stored in the sum
variable and printed.
Question:
What is the purpose of the dplyr package in R? Explain some of the key functions provided by dplyr and how they can be used for data manipulation.
Answer:
The dplyr
package in R provides a grammar of data manipulation, enabling efficient and intuitive data manipulation operations. It offers a set of functions that perform common data manipulation tasks, such as filtering, selecting, arranging, grouping, and summarizing data.
Some key functions provided by dplyr include:
filter()
: Used to select rows based on specific conditions.select()
: Used to select specific columns from a data frame.arrange()
: Used to reorder rows based on one or more variables.mutate()
: Used to create new variables (columns) based on existing variables.group_by()
: Used to group data based on one or more variables for subsequent operations.summarize()
: Used to calculate summary statistics or aggregate data within groups.
These functions make data manipulation tasks more streamlined and expressive, allowing for cleaner and more efficient code.
Question:
Explain the concept of data visualization in R. What are some popular packages and functions used for creating visualizations? Provide an example demonstrating the creation of a basic plot in R.
Answer:
Data visualization in R involves creating graphical representations of data to gain insights and communicate findings effectively. R provides various packages and functions for data visualization.
Some popular packages for data visualization in R include ggplot2, plotly, and lattice. These packages provide functions for creating a wide range of plots, including bar plots, line plots, scatter plots, histograms, and more.
Here’s an example demonstrating the creation of a basic scatter plot using the ggplot2 package:
library(ggplot2)
# Create a data frame
data <- data.frame(x = c(1, 2, 3, 4, 5),
y = c(3, 5, 4, 6, 2))
# Create a scatter plot
ggplot(data, aes(x = x, y = y)) +
geom_point() +
labs(title = "Scatter Plot", x = "X-axis", y = "Y-axis")
Code language: HTML, XML (xml)
In this example, the ggplot2 package is loaded using library(ggplot2)
. A data frame is created with x and y variables. The scatter plot is created using ggplot()
and geom_point()
functions, specifying the x and y aesthetics. The labs()
function is used to provide a title and label the axes.
Question:
What is the purpose of the apply family of functions in R? How do these functions enable the application of a function to subsets of data? Provide an example demonstrating the usage of an apply function in R.
Answer:
The apply family of functions in R (e.g., apply()
, lapply()
, sapply()
) allows for applying a function to subsets of data or elements of an object, such as a matrix or a list. These functions simplify repetitive tasks and avoid the need for explicit loops.
The apply()
function is commonly used to apply a function across rows or columns of a matrix or data frame. For example, to calculate the column-wise mean of a matrix, you can use the apply()
function as follows:
# Create a matrix
matrix <- matrix(c(1, 2, 3, 4, 5, 6), nrow = 2)
# Apply column-wise mean
means <- apply(matrix, 2, mean)
print(means) # Output: 3 4 5
Code language: PHP (php)
In this example, the apply()
function is used to calculate the mean of each column (specified by 2
) of the matrix. The resulting means are stored in the means
variable and printed.
The lapply()
function is used to apply a function to each element of a list, while sapply()
simplifies the output and returns a vector or matrix if possible.
Question:
Explain the concept of data importing and exporting in R. What are some common file formats and packages used for importing and exporting data? Provide an example demonstrating the import of a CSV file in R.
Answer:
Data importing and exporting in R involves reading data from external files into R and saving R objects to external files. R supports various file formats and provides packages for handling different data sources.
Some common file formats for data import and export in R include CSV (Comma-Separated Values), Excel spreadsheets, SQL databases, and text files.
To import a CSV file into R, you can use the read.csv()
function. Here’s an example:
# Import a CSV file
data <- read.csv("data.csv")
Code language: PHP (php)
In this example, the read.csv()
function is used to read the “data.csv” file and store its contents in the data
object.
For exporting data, you can use functions such as write.csv()
to save data frames or matrices to a CSV file, or write.table()
to save data to a text file.
These functions, along with other specialized packages like readxl
for Excel files or DBI
for database connectivity, provide flexible options for importing and exporting data in R.
These answers provide detailed explanations for each question, covering the fundamental concepts in R programming. It’s essential to refer to R documentation and additional resources for a comprehensive understanding of the language and its applications.
Intermediate R interview questions
Question:
How would you read a CSV file in R and store its contents in a data frame?
Answer:
To read a CSV file in R and store its contents in a data frame, you can use the read.csv()
function. Here’s an example:
data <- read.csv("path/to/file.csv")
Code language: JavaScript (javascript)
In this example, the CSV file located at “path/to/file.csv” is read, and its contents are stored in the data
data frame.
Question:
What is the purpose of the apply()
function in R? Provide an example of its usage.
Answer:
The apply()
function in R is used to apply a function to a data structure, such as a matrix or a data frame, along a specific margin. It allows for the efficient application of a function to each row or column of a data structure.
Here’s an example:
matrix_data <- matrix(1:9, nrow = 3)
result <- apply(matrix_data, 1, sum)
In this example, a matrix matrix_data
is created with values 1 to 9. The apply()
function is then used to calculate the sum of each row (margin = 1
) of the matrix. The result is stored in the result
vector.
Question:
The following R code is intended to calculate the factorial of a given number. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
factorial <- function(n) {
result <- 1
for (i in n:1) {
result <- result * i
}
result
}
Code language: JavaScript (javascript)
Answer:
The logical error in the code is that the loop iterates in the wrong direction. The correct code is as follows:
factorial <- function(n) {
result <- 1
for (i in 1:n) {
result <- result * i
}
result
}
Code language: JavaScript (javascript)
In this corrected code, the loop iterates from 1 to n
in the correct order, ensuring the factorial is calculated accurately.
Question:
Explain what closures are in R and provide an example.
Answer:
Closures in R are functions bundled together with their lexical environment. They allow a function to access variables from its parent environment, even after the parent function has finished executing.
Here’s an example:
makeMultiplier <- function(factor) {
function(x) {
x * factor
}
}
multiplyByTwo <- makeMultiplier(2)
result <- multiplyByTwo(5)
Code language: JavaScript (javascript)
In this example, the makeMultiplier()
function returns a closure that multiplies a given value by the factor
parameter. The closure retains access to the factor
variable even after makeMultiplier()
has finished executing. The multiplyByTwo
closure is created using makeMultiplier(2)
, and it multiplies its argument by 2. Finally, multiplyByTwo(5)
is called, resulting in the value 10.
Question:
The following R code is intended to calculate the sum of squares of even numbers in a given vector. However, it contains a syntax error and doesn’t produce the correct result. Identify the error and fix the code.
sum_of_even_squares <- function(numbers) {
sum(sapply(numbers, function(x) if (x %% 2 == 0) x^2))
}
Code language: JavaScript (javascript)
Answer:
The syntax error in the code is the missing else
branch in the if
statement. The correct code is as follows:
sum_of_even_squares <- function(numbers) {
sum(sapply(numbers, function(x) if (x %% 2 == 0) x^2 else 0))
}
Code language: JavaScript (javascript)
In this corrected code, the else
branch is added to the if
statement, providing a default value of 0 for non-even numbers.
Question 6:
What is recursion in R? Explain with an example.
Answer:
Recursion in R refers to the process where a function calls itself within its own body. It allows for solving problems by breaking them down into smaller subproblems that are solved in a similar manner.
Here’s an example of a recursive function that calculates the factorial of a number:
factorial <- function(n) {
if (n == 0) {
return(1)
} else {
return(n * factorial(n - 1))
}
}
result <- factorial(5)
Code language: JavaScript (javascript)
In this example, the factorial()
function recursively calls itself with a smaller value of n
until it reaches the base case where n
is 0. The function then returns 1, and the recursive calls unwind, multiplying the values to calculate the factorial.
Question:
The following R code is intended to reverse a given vector. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
reverse_vector <- function(vec) {
reversed <- vec
for (i in 1:length(vec)) {
reversed[i] <- vec[length(vec) - i + 1]
}
reversed
}
Code language: JavaScript (javascript)
Answer:
The logical error in the code is that it incorrectly assigns values to the reversed
vector. The correct code is as follows:
reverse_vector <- function(vec) {
reversed <- vector("numeric", length(vec))
for (i in 1:length(vec)) {
reversed[i] <- vec[length(vec) - i + 1]
}
reversed
}
Code language: JavaScript (javascript)
In this corrected code, a new vector reversed
is created with the same length as the input vector vec
. The values are then assigned correctly using the loop, resulting in the reversed vector.
Question:
What are anonymous functions in R? Provide an example of their usage.
Answer:
Anonymous functions in R, also known as lambda functions, are functions that are defined without a formal name. They are typically used in scenarios where a function is needed temporarily or as an argument to another function.
Here’s an example of an anonymous function in R:
squared <- function(x) x^2
result <- sapply(1:5, function(x) squared(x))
Code language: PHP (php)
In this example, an anonymous function is defined within the sapply()
function. It takes an argument x
and returns the square of x
. The sapply()
function then applies this anonymous function to each element of the vector 1:5
, resulting in the squared values stored in the result
vector.
Question:
The following R code is intended to check if a given list contains any duplicate elements. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
has_duplicates <- function(lst) {
for (i in 1:length(lst)) {
for (j in (i+1):length(lst)) {
if (lst[i] == lst[j]) {
return(TRUE)
}
}
}
return(FALSE)
}
Code language: PHP (php)
Answer:
The logical error in the code is that the second loop should start from `i+1
instead of
i`. The correct code is as follows:
has_duplicates <- function(lst) {
for (i in 1:length(lst)) {
for (j in (i+1):length(lst)) {
if (lst[i] == lst[j]) {
return(TRUE)
}
}
}
return(FALSE)
}
Code language: PHP (php)
In this corrected code, the second loop starts from i+1
, ensuring that duplicate elements are properly checked.
Question:
What are higher-order functions in R? Provide an example of their usage.
Answer:
Higher-order functions in R are functions that can take other functions as arguments or return functions as results. They treat functions as first-class objects, allowing for flexible and modular programming.
Here’s an example of a higher-order function in R:
apply_operation <- function(operation, x, y) {
operation(x, y)
}
add <- function(a, b) {
a + b
}
subtract <- function(a, b) {
a - b
}
result1 <- apply_operation(add, 5, 3)
result2 <- apply_operation(subtract, 10, 6)
Code language: JavaScript (javascript)
In this example, the apply_operation()
function is a higher-order function that takes an operation
function as an argument, along with two numbers x
and y
. It applies the operation
function to x
and y
and returns the result.
The add()
and subtract()
functions are passed as arguments to apply_operation()
, resulting in result1
being 8 (5 + 3) and result2
being 4 (10 – 6).
Senior R interview questions
Question:
Explain the concept of data mining in the context of R and provide an example of a data mining technique commonly used in R.
Answer:
Data mining in the context of R involves extracting useful insights and patterns from large datasets. It is the process of discovering patterns, relationships, and anomalies in data to make informed decisions and predictions.
An example of a data mining technique commonly used in R is association rule mining. This technique is used to identify relationships or associations between items in a dataset. The arules
package in R provides functions for performing association rule mining.
Here’s an example of using association rule mining in R:
library(arules)
# Create a transaction dataset
transactions <- read.transactions("path/to/transaction_data.csv", format = "basket", sep = ",")
# Mine association rules
rules <- apriori(transactions, parameter = list(supp = 0.1, conf = 0.8))
# View the generated rules
inspect(rules)
Code language: PHP (php)
In this example, a transaction dataset is read from a CSV file using read.transactions()
. The apriori()
function is then used to mine association rules from the dataset, with support and confidence thresholds specified. Finally, the inspect()
function is used to view the generated association rules.
Question:
The following R code is intended to perform K-means clustering on a given dataset. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
kmeans_clustering <- function(data, k) {
set.seed(123)
result <- kmeans(data, centers = k)
return(result$cluster)
}
Code language: PHP (php)
Answer:
The logical error in the code is that it returns the cluster assignments instead of the entire k-means result. The correct code is as follows:
kmeans_clustering <- function(data, k) {
set.seed(123)
result <- kmeans(data, centers = k)
return(result)
}
Code language: JavaScript (javascript)
In this corrected code, the kmeans_clustering()
function returns the entire k-means result, including cluster assignments, cluster centroids, and other information.
Question:
Explain the concept of cross-validation in the context of machine learning using R.
Answer:
Cross-validation in the context of machine learning is a technique used to evaluate the performance and generalization of a model. It involves partitioning the available data into multiple subsets, training the model on a portion of the data, and evaluating its performance on the remaining data.
A commonly used method for cross-validation is k-fold cross-validation. In k-fold cross-validation, the data is divided into k equally sized subsets or folds. The model is trained and evaluated k times, with each fold serving as the validation set once, while the remaining k-1 folds are used for training.
Here’s an example of performing k-fold cross-validation in R using the caret
package:
library(caret)
# Load dataset
data <- read.csv("path/to/data.csv")
# Define control parameters for cross-validation
ctrl <- trainControl(method = "cv", number = 5)
# Train the model using k-fold cross-validation
model <- train(target_variable ~ ., data = data, trControl = ctrl, method = "lm")
# View the cross-validation results
print(model)
Code language: PHP (php)
In this example, the dataset is loaded using read.csv()
. The trainControl()
function is used to define the control parameters for cross-validation, specifying the number of folds (number = 5
). The train()
function is then used to train a linear regression model (method = "lm"
) using the k-fold cross-validation approach. The resulting model object contains the cross-validation results, including performance metrics and model parameters.
Question:
The following R code is intended to perform principal component analysis (PCA) on a given dataset. However, it contains a syntax error and doesn’t run correctly. Identify the error and fix the code.
pca_analysis <- function(data) {
result <- prcomp(data)
return(result$rotation)
}
Code language: PHP (php)
Answer:
The syntax error in the code is the incorrect usage of the prcomp()
function. The correct code is as follows:
pca_analysis <- function(data) {
result <- princomp(data)
return(result$loadings)
}
Code language: PHP (php)
In this corrected code, the princomp()
function is used instead of prcomp()
to perform principal component analysis. The resulting loadings (eigenvectors) are returned from the function.
Question:
Explain the concept of regularization in machine learning and provide an example of a regularization technique commonly used in R.
Answer:
Regularization in machine learning is a technique used to prevent overfitting and improve the generalization of a model. It involves adding a penalty term to the model’s objective function to discourage large parameter values. By controlling the magnitude of the parameters, regularization helps in reducing the complexity of the model and prevents it from fitting the noise in the training data.
One commonly used regularization technique in R is Ridge regression. Ridge regression adds a penalty term to the sum of squared residuals, which is a function of the squared magnitude of the regression coefficients. This penalty term is controlled by a hyperparameter called the regularization parameter or lambda (λ).
Here’s an example of performing Ridge regression in R using the glmnet
package:
library(glmnet)
# Load dataset
data <- read.csv("path/to/data.csv")
# Separate predictors and target variable
X <- data[, -ncol(data)]
y <- data[, ncol(data)]
# Perform Ridge regression
ridge_model <- glmnet(X, y, alpha = 0, lambda = 0.5)
# View the coefficients
print(coef(ridge_model))
Code language: PHP (php)
In this example, the glmnet()
function is used to perform Ridge regression (alpha = 0
) with a regularization parameter of 0.5 (lambda = 0.5
). The resulting model object contains the coefficients, which can be viewed using the coef()
function.
Question:
The following R code is intended to perform feature scaling on a given dataset. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
feature_scaling <- function(data) {
scaled_data <- scale(data)
return(scaled_data)
}
Code language: JavaScript (javascript)
Answer:
The logical error in the code is that it returns the scaled data without converting it back to a data frame. The correct code is as follows:
feature_scaling <- function(data) {
scaled_data <- as.data.frame(scale(data))
return(scaled_data)
}
Code language: JavaScript (javascript)
In this corrected code, the as.data.frame()
function is used to convert the scaled data back to a data frame before returning it.
Question:
Explain the concept of ensemble learning in the context of machine learning using R.
Answer:
Ensemble learning in the context of machine learning is a technique that combines the predictions of multiple individual models to make a final prediction. It aims to improve predictive performance and generalization by leveraging the diversity and collective wisdom of the ensemble.
There are various ensemble learning methods, such as bagging, boosting, and stacking. Bagging (bootstrap aggregating) involves training multiple models on
different bootstrap samples of the data and combining their predictions through majority voting or averaging. Boosting, on the other hand, trains models sequentially, where each subsequent model focuses on correcting the mistakes of the previous models. Stacking combines the predictions of multiple models using another model, called a meta-model, which learns from the predictions of the individual models.
Here’s an example of using ensemble learning in R with the caret
package and bagging:
library(caret)
# Load dataset
data <- read.csv("path/to/data.csv")
# Define the training control parameters
ctrl <- trainControl(method = "repeatedcv", number = 5, repeats = 3)
# Train the ensemble model using bagging
model <- train(target_variable ~ ., data = data, trControl = ctrl, method = "treebag")
# Make predictions using the ensemble model
predictions <- predict(model, newdata = test_data)
Code language: PHP (php)
In this example, the trainControl()
function is used to define the training control parameters, specifying the number of cross-validation folds and repeats. The train()
function is then used to train an ensemble model using bagging (method = "treebag"
). Finally, the trained ensemble model is used to make predictions on new data.
Question:
The following R code is intended to perform feature selection using the LASSO (Least Absolute Shrinkage and Selection Operator) technique. However, it contains a syntax error and doesn’t run correctly. Identify the error and fix the code.
lasso_feature_selection <- function(X, y) {
result <- glmnet(X, y, family = "gaussian", alpha = 1, lambda = 0.5)
selected_features <- coef(result, s = 0.5)
return(selected_features)
}
Code language: JavaScript (javascript)
Answer:
The syntax error in the code is the incorrect usage of the glmnet()
function. The correct code is as follows:
lasso_feature_selection <- function(X, y) {
result <- glmnet(x = X, y = y, family = "gaussian", alpha = 1, lambda = 0.5)
selected_features <- coef(result, s = 0.5)
return(selected_features)
}
Code language: JavaScript (javascript)
In this corrected code, the x
argument is used instead of X
to pass the predictor variables to the glmnet()
function.
Question:
Explain the concept of anomaly detection in the context of machine learning and provide an example of an anomaly detection technique commonly used in R.
Answer:
Anomaly detection in the context of machine learning involves identifying observations or instances in a dataset that deviate significantly from the expected behavior or patterns. Anomalies, also known as outliers, can provide valuable insights into unusual or unexpected occurrences, fraud detection, or system failures.
One commonly used anomaly detection technique in R is the Isolation Forest algorithm. The Isolation Forest algorithm uses the concept of randomly partitioning the data space to isolate anomalies efficiently. It constructs a random forest of isolation trees, where each tree recursively splits the data by randomly selecting a feature and a split point until each instance is isolated. The anomaly score is calculated based on the average path length required to isolate an instance across all trees.
Here’s an example of performing anomaly detection using the Isolation Forest algorithm in R:
library(dbscan)
# Load dataset
data <- read.csv("path/to/data.csv")
# Perform anomaly detection using Isolation Forest
isolation_forest <- iforest(data, ntrees = 100)
# Identify anomalies
anomaly_scores <- predict(isolation_forest, data)
anomalies <- data[anomaly_scores > threshold, ]
Code language: PHP (php)
In this example, the dbscan
package is used, which includes an implementation of the Isolation Forest algorithm (iforest()
function). The ntrees
parameter specifies the number of trees in the forest. Anomaly scores are obtained using the predict()
function, and anomalies are identified based on a predefined threshold.
Question 10:
The following R code is intended to perform text preprocessing on a given corpus of documents. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
text_preprocessing <- function(documents) {
cleaned_documents <- lapply(documents, function(doc) {
tolower(doc)
removePunctuation(doc)
removeNumbers(doc)
stripWhitespace(doc)
stemDocument(doc)
})
return(cleaned_documents)
}
Code language: JavaScript (javascript)
Answer:
The logical error in the code is that the intermediate steps of text preprocessing are not applied correctly. The correct code is as follows:
text_preprocessing <- function(documents) {
cleaned_documents <- lapply(documents, function(doc) {
doc <- tolower(doc)
doc <- removePunctuation(doc)
doc <- removeNumbers(doc)
doc <- stripWhitespace(doc)
doc <- stemDocument(doc)
return(doc)
})
return(cleaned_documents)
}
Code language: JavaScript (javascript)
In this corrected code, the intermediate steps of text preprocessing, such as converting to lowercase, removing punctuation, removing numbers, stripping whitespace, and stemming, are correctly applied to the doc
variable within the lapply()
function. The cleaned documents are then returned.
1,000 Companies use CoderPad to Screen and Interview Developers
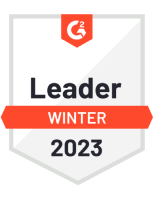
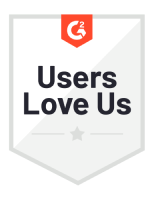
Best interview practices for R roles
For successful R interviews, it is essential to consider various factors, such as the candidate’s background and the specific engineering role. To facilitate a fruitful interview experience, we recommend adopting the following best practices:
- Develop technical questions that reflect real-world business scenarios within your organization. This approach will effectively engage the candidates and help determine their compatibility with your team.
- Cultivate a cooperative environment by inviting candidates to ask questions throughout the interview.
- Make sure your candidates know how to work with large datasets to show they can handle efficient data manipulation.
Moreover, adhering to standard interview practices is critical when conducting R interviews. This involves adjusting the difficulty of the questions to match the candidate’s abilities, providing timely updates on their application progress, and allowing them the opportunity to ask about the assessment process and collaborating with you and your team.