Python Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Python developers for your organization.
Python
Regularly considered one of the most in-demand languages across the world, Python continues to gain a dedicated following due to its superior support for data processing and manipulation.
According to the CoderPad 2024 Developer survey, Python is THE most in-demand language among technical recruiters and hiring managers.
Below you’ll see examples of realistic coding exercises and interview questions that will help you evaluate developers’ Python skills during coding interviews.
You’ll also find a set best practices to ensure your interview questions accurately assess the Python skills of your candidates.
Table of Contents
Python example question
Can you fix this Python function?
This function works as expected, but it’s very slow on large test cases. Can you figure out what’s wrong and fix it without changing its statistical distribution?
Make the generate_random_list
function run faster than 100ms for both tests.
Python skills to assess
Jobs using Python
Junior Python interview questions
Question: What is the difference between a tuple and a list in Python?
Answer: A list is a mutable sequence of elements, while a tuple is an immutable sequence of elements. This means that you can modify a list by adding, removing or changing elements, but you cannot do the same with a tuple.
Question: The following code is supposed to find the sum of the even numbers in a list. What is wrong with the code, and how can it be fixed?
Answer:
numbers = [1, 2, 3, 4, 5]
total = 0
for number in numbers:
if number % 2 == 0
total += number
print(total)
Code language: Python (python)
The code is missing a colon after the if statement. The fixed code is:
numbers = [1, 2, 3, 4, 5]
total = 0
for number in numbers:
if number % 2 == 0:
total += number
print(total)
Code language: PHP (php)
Question: What is the difference between a function and a method in Python?
Answer: A function is a standalone block of code that performs a specific task, while a method is a function that is associated with an object and can access and modify its data.
Question: The following code is supposed to create a dictionary with the keys and values reversed from the original dictionary. What is wrong with the code, and how can it be fixed?
Answer:
original_dict = {"one": 1, "two": 2, "three": 3}
reversed_dict = {}
for key, value in original_dict.items():
reversed_dict[value] = key
print(reversed_dict)
Code language: Python (python)
The code is correct.
Question: What is the difference between a while loop and a for loop in Python?
Answer: A for loop is used to iterate over a sequence, such as a list or a string, while a while loop is used to repeat a block of code as long as a certain condition is true.
Question: The following code is supposed to create a list of the squares of the first five integers. What is wrong with the code, and how can it be fixed?
Answer:
squares = []
for i in range(5)
squares.append(i ** 2)
print(squares)
Code language: Python (python)
The code is missing a colon after the for statement. The fixed code is:
squares = []
for i in range(5):
squares.append(i ** 2)
print(squares)
Code language: Python (python)
Question: What is the difference between the “is” operator and the “==” operator in Python?
Answer: The “is
” operator checks if two objects are the same object, while the “==
” operator checks if two objects have the same value.
Question: The following code is supposed to remove all the even numbers from a list. What is wrong with the code, and how can it be fixed?
Answer:
numbers = [1, 2, 3, 4, 5]
for number in numbers:
if number % 2 == 0
numbers.remove(number)
print(numbers)
Code language: Python (python)
The code is modifying the list while iterating over it, which can lead to unexpected results. The fixed code is to create a new list instead:
numbers = [1, 2, 3, 4, 5]
odd_numbers = []
for number in numbers:
if number % 2 != 0:
odd_numbers.append(number)
print(odd_numbers)
Code language: Python (python)
Question: What is the purpose of the pass
statement in Python?
Answer: The pass
statement is used as a placeholder when a statement is required syntactically, but no code needs to be executed.
Question: The following code is supposed to create a list of the first five even numbers. What is wrong with the code, and how can it be fixed?
Answer:
even_numbers = [0, 2, 4, 6, 8]
Code language: Python (python)
The code is correct.
Intermediate Python interview questions
Question: What is a generator in Python? How is it different from a regular function?
Answer: A generator is a special type of function in Python that returns an iterator object, which can be iterated over using a for
loop. The key difference between a generator and a regular function is that a generator can yield
values one at a time, whereas a regular function returns
all values at once. This makes generators more memory-efficient, as they do not need to generate all values at once and store them in memory.
Question: What is the difference between a class method and an instance method in Python?
Answer: A class method is a method that is bound to the class and not the instance of the class. It can be called on the class itself, without the need to create an instance of the class. An instance method, on the other hand, is bound to the instance of the class and can only be called on an instance of the class.
Question: What is the purpose of the __str__
method in Python classes? How is it different from the __repr__
method?
Answer: The __str__
method is used to define the string representation of an object, and is typically used for displaying the object to end-users. The __repr__
method, on the other hand, is used to define the “official” string representation of an object, and is typically used for debugging and development purposes. The main difference between the two is that __str__
is called when str()
is called on an object, while __repr__
is called when repr()
is called on an object.
Question: The following code is supposed to create a class for a basic calculator, but it is not working correctly. Fix the code.
class Calculator:
def __init__(self):
self.result = 0
def add(self, num1, num2):
self.result = num1 + num2
def subtract(self, num1, num2):
self.result = num1 - num2
Code language: Python (python)
Answer: The add
and subtract
methods are not actually returning any values, so they are not useful for external code that wants to use the calculator. To fix this, we can modify the methods to return the result instead of storing it as an attribute of the object:
class Calculator:
def __init__(self):
self.result = 0
def add(self, num1, num2):
return num1 + num2
def subtract(self, num1, num2):
return num1 - num2
Code language: Python (python)
Question: What is a lambda function in Python? How is it different from a regular function?
Answer: A lambda function is a small anonymous function in Python that can have any number of arguments, but can only have one expression. The main difference between a lambda function and a regular function is that a lambda function is defined inline, without a name, and is typically used as a simple, one-line function that is not reusable.
Question: The following code is supposed to create a generator function that returns a Fibonacci sequence, but it is not working correctly. Fix the code.
def fibonacci():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
Code language: Python (python)
Answer: The code looks correct, but the starting values for a
and b
should be 0
and 1
, respectively. The current code initializes a
as 1
and b
as 2
, which does not generate the correct Fibonacci sequence.
Question: What is a metaclass in Python? How is it different from a regular class?
Answer: A metaclass is a class that defines the behavior of other classes. It is used to customize the creation of classes and their instances, and can be used to enforce certain constraints or provide additional functionality to classes. The main difference between a metaclass and a regular class is that a metaclass is used to define the behavior of other classes, whereas a regular class is used to define objects that can be instantiated and used in a program.
Question: The following code is supposed to create a class that represents a bank account, but it is not working correctly. Fix the code.
class BankAccount:
def __init__(self, balance):
self.balance = balance
def deposit(self, amount):
self.balance += amount
def withdraw(self, amount):
self.balance -= amount
def check_balance(self):
print("Your balance is: ", self.balance)
Code language: Python (python)
Answer: The code looks correct, but there is no error handling for situations where the user tries to withdraw more money than they have in their account. To fix this, we can add a check in the withdraw
method to ensure that the amount being withdrawn does not exceed the current balance:
class BankAccount:
def __init__(self, balance):
self.balance = balance
def deposit(self, amount):
self.balance += amount
def withdraw(self, amount):
if amount > self.balance:
raise ValueError("Insufficient funds")
self.balance -= amount
def check_balance(self):
print("Your balance is: ", self.balance)
Code language: Python (python)
Question: The following code is supposed to create a function that takes a list of integers and returns only the even numbers, but it is not working correctly. Fix the code.
def get_even_numbers(numbers):
even_numbers = [x for x in numbers if x % 2 == 0]
return even_numbers
Code language: Python (python)
Answer: The code looks correct, but it can be improved by using a generator expression instead of a list comprehension to generate the even numbers on the fly and save memory:
def get_even_numbers(numbers):
even_numbers = (x for x in numbers if x % 2 == 0)
return even_numbers
Code language: Python (python)
Question: What is the difference between a generator function and a normal function in Python? Provide an example of a generator function.
Answer:
- Generator function is a type of function that yields a sequence of values instead of returning a single value.
- Normal function returns a single value and then exits.
- Generator functions use the
yield
keyword to return a value and pause the execution of the function. - Normal functions use the
return
keyword to return a value and immediately exit the function.
Example of a generator function:
def my_generator(n):
i = 0
while i < n:
yield i
i += 1
Code language: Python (python)
This generator function returns a sequence of numbers from 0 to n-1. It does not use the return
keyword but instead uses yield
to pause and resume the function.
Senior Python interview questions
Question: What is the purpose of lambda functions in Python, and how are they different from regular functions?
Answer: Lambda functions are used to create small, anonymous functions that can be used in place of regular functions. They are different from regular functions in that they do not have a name and can only contain a single expression.
Question: What is a generator function in Python, and how is it different from a regular function?
Answer: A generator function is a special type of function that can be used to generate a sequence of values on-the-fly. It is different from a regular function in that it uses the “yield” keyword to return values one-at-a-time instead of returning a list of all values at once.
Question: What is the purpose of the “yield” keyword in Python, and how is it used in generator functions?
Answer: The yield
keyword is used in generator functions to return a value and temporarily suspend the function’s execution. When the generator function is called again, it resumes execution from the point it left off and continues generating values one-at-a-time.
Question: What is meta-programming in Python, and how is it used?
Answer: Meta-programming is writing code that can manipulate code at runtime. It is used to create generic, reusable code, or to modify the behavior of existing code dynamically.
Question: This code is supposed to create a generator that yields the first n numbers in the Fibonacci sequence. Fix the code.
def fibonacci(n):
a, b = 0, 1
for i in range(n):
yield b
a, b = b, a+b
print(fibonacci(5))
Code language: Python (python)
Answer: The code is correct, but the print()
statement is printing the generator object instead of the sequence of numbers. To print the sequence of numbers, you can convert the generator to a list:
def fibonacci(n):
a, b = 0, 1
for i in range(n):
yield b
a, b = b, a+b
print(list(fibonacci(5)))
Code language: Python (python)
Question: This code is supposed to return a list of all odd numbers from the input list. Fix the code.
def get_odd_numbers(nums):
odd_nums = filter(lambda num: num % 2)
return list(odd_nums)
nums = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
print(get_odd_numbers(nums))
Code language: Python (python)
Answer: The lambda function inside the filter() function is missing the argument nums in the expression. The corrected code is:
def get_odd_numbers(nums):
odd_nums = filter(lambda num: num % 2, nums)
return list(odd_nums)
nums = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
print(get_odd_numbers(nums))
Code language: Python (python)
Question: What are decorators in Python, and how are they used?
Answer: Decorators are a way to modify the behavior of a function or class in Python without changing its source code. They are implemented as callable objects that take another function or class as an argument and return a modified version of it.
Question: This code is supposed to use a lambda function to sort a list of dictionaries by the value of a specific key. Fix the code.
data = [{'name': 'Alice', 'age': 28}, {'name': 'Bob', 'age': 21}, {'name': 'Charlie', 'age': 35}]
sorted_data = sorted(data, key=lambda x: x['age'])
print(sorted_data)
Code language: Python (python)
Answer:
The code is correct and will sort the list of dictionaries by the age key.
Question: This code is supposed to create a metaclass that allows classes to be initialized with a dictionary of attributes. Fix the code.
class DictMeta(type):
def __new__(mcs, name, bases, attrs):
obj = super().__new__(mcs, name, bases, attrs)
obj.__dict__.update(attrs)
return obj
class MyClass(metaclass=DictMeta):
def __init__(self, data):
self.data = data
my_instance = MyClass({'name': 'Alice', 'age': 28})
print(my_instance.name, my_instance.age)
Code language: Python (python)
Answer:
class DictMeta(type):
def __new__(mcs, name, bases, attrs, **kwargs):
new_attrs = {}
for key, value in attrs.items():
if isinstance(value, dict):
new_attrs.update(value)
else:
new_attrs[key] = value
return super().__new__(mcs, name, bases, new_attrs)
class MyClass(metaclass=DictMeta):
def __init__(self, name=None, age=None):
self.name = name
self.age = age
my_instance = MyClass({'name': 'Alice', 'age': 28})
print(my_instance.name, my_instance.age)
Code language: Python (python)
In the DictMeta
class, the __new__
method should call __init__
instead of obj.__dict__.update(attrs)
to properly initialize the attributes of the class. In the MyClass
class, the __init__
method should update the __dict__
attribute of the instance with the data
parameter to properly initialize the instance attributes.
Question: This code is supposed to use a generator function to yield all prime numbers up to a given limit. Fix the code.
def primes(limit):
i = 2
while i < limit:
for j in range(2, i):
if i % j == 0:
break
else:
yield i
i += 1
print(list(primes(20)))
Code language: Python (python)
Answer: The code is correct and will generate a list of prime numbers up to 20.
Question: This code is supposed to use a context manager to open a file, write some data to it, and then automatically close the file. Fix the code.
with open('output.txt', 'w') as f:
f.write('hello, world')
Code language: Python (python)
Answer:
with open('output.txt', 'w') as f:
f.write('hello, worldn')
Code language: JavaScript (javascript)
The code is correct, but it should include a newline character at the end of the string to properly format the output in the file.
More Python interview resources
For more guides on improving your knowledge of Python and acing interviews, we have outlined helpful blog posts below:
- Guide to Classes and Object-Oriented Programming in Python
- Python List Comprehension – The Comprehensive Guide
- An Introduction to Linked List Data Structures
- Testing in Python: Types of Tests and How to Write Them
- Code Challenge: Python Unit Testing
- Python Interview Questions by Codingame
1,000 Companies use CoderPad to Screen and Interview Developers
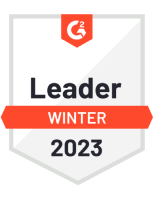
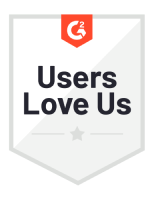
Interview best practices for Python roles
Python interviews can vary on everything from the engineering role involved to the level of candidate being interviewed. In order to get the best results from your Python interview questions, we recommend following these best practices when working with your candidates:
- Use technical questions that reflect actual business cases with your organization – this is more interesting for the candidate and will better showcase the skills match with your team.
- Encourage the candidate to ask questions. Make the exercise a collaborative experience.
- If you’re using Python for data analysis, make sure the candidate has a solid understanding of data structures.
Additionally, you’ll want to follow standard interview etiquette with your Python interviews – tailor the interview question complexity to the level of development skills of your candidates, ensure prompt feedback to the candidates about their status in the job search process, and make sure you leave time for candidates to ask questions either about the assessment or working with you and your team.