PHP Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified PHP developers for your organization.
PHP
PHP was one of the first languages used in web development, and still has a strong showing in popular content management systems like WordPress and Drupal. Much of its popularity is in its low learning curve, embedded HTML support, and cross platform compatibility.
According to the CoderPad 2024 Developer survey, PHP is the 9th most in-demand language among technical recruiters and hiring managers.
We have developed hands-on coding tasks and interview queries below to evaluate developers’ PHP skills during coding interviews. Moreover, we have compiled a set of best practices to ensure that your interview questions accurately gauge the candidates’ PHP proficiency.
Table of Contents
PHP example question
Help us design a parking lot
Hey candidate! Welcome to your interview. Boilerplate is provided. Feel free to change the code as you see fit. To run the code at any time, please hit the run button located in the top left corner.
Goals: Design a parking lot using object-oriented principles
Here are a few methods that you should be able to run:
- Tell us how many spots are remaining
- Tell us how many total spots are in the parking lot
- Tell us when the parking lot is full
- Tell us when the parking lot is empty
- Tell us when certain spots are full e.g. when all motorcycle spots are taken
- Tell us how many spots vans are taking up
Assumptions:
- The parking lot can hold motorcycles, cars and vans
- The parking lot has motorcycle spots, car spots and large spots
- A motorcycle can park in any spot
- A car can park in a single compact spot, or a regular spot
- A van can park, but it will take up 3 regular spots
- These are just a few assumptions. Feel free to ask your interviewer about more assumptions as needed
Junior PHP interview questions
Question: Fix the code:
<?php
class Circle {
private $radius;
public function __construct($radius) {
$this->radius = $radius;
}
public function getArea() {
return pi() * pow($radius, 2);
}
}
$circle = new Circle(5);
echo $circle->getArea();
?>
Code language: PHP (php)
Answer: The issue with the code is that the $radius
variable in the getArea()
method is not properly referenced. It should be $this->radius
instead of $radius
. Here’s the corrected code:
<?php
class Circle {
private $radius;
public function __construct($radius) {
$this->radius = $radius;
}
public function getArea() {
return pi() * pow($this->radius, 2);
}
}
$circle = new Circle(5);
echo $circle->getArea();
?>
Code language: PHP (php)
Question: What is the difference between abstract classes and interfaces in PHP?
Answer: Abstract classes can have both defined methods and properties, whereas interfaces can only have method signatures (method names without any implementation). A class can extend only one abstract class, but it can implement multiple interfaces.
Question: What will be the output of the code below?
<?php
function multiplyByTwo($number) {
$number *= 2;
return $number;
}
$number = 5;
echo multiplyByTwo($number);
?>
Code language: PHP (php)
Answer: The output will be 10
.
Question: What is the purpose of autoloading in PHP, and how can it be implemented?
Answer: Autoloading eliminates the need to manually include PHP class files by automatically loading them when needed. In PHP, autoloading can be implemented using spl_autoload_register
or by using autoloading standards such as PSR-4.
Question: What is the issue with the above code, and how can it be fixed?
<?php
class Rectangle {
private $width;
private $height;
public function __construct($width, $height) {
$this->$width = $width;
$this->$height = $height;
}
public function getArea() {
return $this->width * $this->height;
}
}
$rectangle = new Rectangle(4, 5);
echo $rectangle->getArea();
?>
Code language: PHP (php)
Answer: The issue is that $width
and $height
variables are not properly referenced in the constructor. To fix the code, remove the extra $
symbols from $this->$width
and $this->$height
and change them to $this->width
and $this->height
respectively.
Question: What is the purpose of the final
keyword in PHP, and how is it used?
Answer: The final
keyword is used to indicate that a class or method cannot be overridden by any child class. When a class is marked as final
, it cannot be extended, and when a method is marked as final
, it cannot be overridden.
Question: What will be the output of the above code?
<?php
function calculateAverage($numbers) {
$sum = array_sum($numbers);
$average = $sum / count($numbers);
return $average;
}
$numbers = [2, 4, 6, 8, 10];
echo calculateAverage($numbers);
?>
Code language: PHP (php)
Answer: The output will be 6
.
Question: What is method chaining in PHP, and how does it work?
Answer: Method chaining is a technique that allows multiple methods to be called on an object in a single statement. Each method in the chain returns an instance of the object, which allows subsequent methods to be called on it. It enhances code readability and can make method calls more concise.
Question: What is the issue with the above code, and how can it be fixed?
<?php
class User {
private $name;
private $age;
public function __construct($name, $age) {
$this->name = $name;
$this->age = $age;
}
public function getName() {
return $name;
}
}
$user = new User("John", 25);
echo $user->getName();
?>
Code language: PHP (php)
Answer: The issue is that the $name
variable in the getName()
method is not properly referenced. To fix the code, change return $name
to return $this->name
in the getName()
method.
Question: What are namespaces in PHP, and why are they useful?
Answer: Namespaces in PHP are used to organize classes, interfaces, functions, and constants into a hierarchical structure, preventing naming conflicts between different components. They provide a way to group related code and make it more modular, reusable, and easier to maintain.
Intermediate PHP interview questions
Question: What is the issue with the code below, and how can it be fixed?
<?php
class User {
private $name;
public function __construct($name) {
$this->name = $name;
}
public function getName() {
return $this->$name;
}
}
$user = new User("John Doe");
echo $user->getName();
?>
Code language: PHP (php)
Answer: The issue is that the $name
variable in the getName()
method is not properly referenced. To fix the code, change return $this->$name
to return $this->name
in the getName()
method.
Question: What are the benefits of using dependency injection in PHP? Provide an example of how you would implement it.
Answer: Dependency injection promotes loose coupling between classes and improves testability, maintainability, and code reusability. An example of implementing dependency injection is by passing dependent objects or interfaces as constructor parameters or using setter methods to inject dependencies.
Question: What will be the output of the code below?
<?php
function calculateAverage($numbers) {
$sum = array_sum($numbers);
$average = $sum / count($numbers);
return $average;
}
$numbers = [2, 4, 6, 8, 10];
echo calculateAverage($numbers);
?>
Code language: PHP (php)
Answer: The output will be 6
.
Question: Explain the concept of polymorphism in object-oriented programming and provide an example in PHP.
Answer: Polymorphism allows objects of different classes to be treated as objects of a common superclass or interface. It enables methods to be overridden in child classes to provide different implementations. For example, in PHP, you can have a Shape
superclass with a calculateArea()
method, and different shapes like Circle
and Rectangle
can extend Shape
and implement their own calculateArea()
methods.
Question: What is missing in the code below, and how can it be fixed?
<?php
class Database {
private $connection;
public function __construct($host, $username, $password, $database) {
$this->connection = new mysqli($host, $username, $password, $database);
}
public function query($sql) {
return $this->connection->query($sql);
}
}
$database = new Database("localhost", "root", "password", "my_database");
$result = $database->query("SELECT * FROM users");
?>
Code language: PHP (php)
Answer: The code is missing error handling for database connection failures. To fix the code, you can add error handling logic to check for connection errors and handle them appropriately.
Question: Explain the concept of namespaces in PHP and how they help in organizing code.
Answer: Namespaces in PHP provide a way to organize classes, interfaces, functions, and constants into a hierarchical structure, preventing naming conflicts. They allow developers to group related code and make it more modular, reusable, and easier to maintain. Namespaces also enable autoloading and simplify class name resolution.
Question: What will be the output of the code below?
<?php
class Math {
public static function square($number) {
return $number * $number;
}
}
echo Math::square(5);
?>
Code language: PHP (php)
Answer: The output will be 25
.
Question: What are traits in PHP, and how are they different from classes and interfaces?
Answer: Traits in PHP provide a way to reuse code in classes by enabling horizontal code sharing. Unlike classes, traits cannot be instantiated or inherited, and they cannot have properties. Traits are meant to group functionality that can be added to different classes, providing a form of multiple inheritance.
Question: What will be the output of the code below?
<?php
function reverseString($str) {
$reversed = '';
for ($i = strlen($str) - 1; $i >= 0; $i--) {
$reversed .= $str[$i];
}
return $reversed;
}
echo reverseString("Hello");
?>
Code language: PHP (php)
Answer: The output will be olleH
.
Question: What is the difference between include
and require
statements in PHP?
Answer: Both include
and require
statements are used to include and evaluate the content of external files in PHP scripts. The difference is that require
throws a fatal error if the specified file cannot be included, while include
generates a warning and continues execution. Therefore, require
is typically used for important files, like essential libraries, while include
is used for optional files, like templates or configurations.
Senior PHP interview questions
Question: The code below finds prime numbers up to a given limit ($n
). However, it is not optimized and can be improved. Can you optimize it?
<?php
function findPrimeNumbers($n) {
$primeNumbers = [];
for ($i = 2; $i <= $n; $i++) {
$isPrime = true;
for ($j = 2; $j < $i; $j++) {
if ($i % $j === 0) {
$isPrime = false;
break;
}
}
if ($isPrime) {
$primeNumbers[] = $i;
}
}
return $primeNumbers;
}
$primes = findPrimeNumbers(100);
foreach ($primes as $prime) {
echo $prime . " ";
}
?>
Code language: PHP (php)
Answer:
<?php
function findPrimeNumbers($n) {
if ($n < 2) {
return [];
}
$primeNumbers = [2];
$isComposite = [];
for ($i = 3; $i <= $n; $i += 2) {
if (!$isComposite[$i]) {
$primeNumbers[] = $i;
for ($j = $i * $i; $j <= $n; $j += 2 * $i) {
$isComposite[$j] = true;
}
}
}
return $primeNumbers;
}
$primes = findPrimeNumbers(100);
foreach ($primes as $prime) {
echo $prime . " ";
}
?>
Code language: HTML, XML (xml)
Optimization Improvements:
- Skipping even numbers: In the optimized code, we start with 3 and only check odd numbers. This is because all even numbers (except 2) are not prime.
- Skipping unnecessary checks: In the inner loop, we start from $i * $i instead of 2. This is because any smaller multiple of $i would have already been marked as composite by smaller prime numbers.
- Using an array for composite numbers: Instead of using a boolean variable for each number, we use an array
$isComposite
to store the composite numbers, marking them astrue
.
The optimized code reduces the number of iterations and unnecessary checks, resulting in improved performance for finding prime numbers.
Please note that the optimization techniques can vary depending on the specific use case and requirements. The example provided above focuses on improving the prime number finding algorithm.
Question: Describe a situation where you would use the Singleton pattern in PHP. Provide an example of how you would implement it.
Answer: The Singleton pattern is used when you need to ensure that only one instance of a class exists throughout the application. It is commonly used for database connections, loggers, and configuration settings. An example in PHP could be a DatabaseConnection
class where you want to restrict multiple connections and provide a single connection instance throughout the application.
Question: What will be the output of the code below?
<?php
function validateEmail($email) {
return filter_var($email, FILTER_VALIDATE_EMAIL);
}
$email = "[email protected]";
if (validateEmail($email)) {
echo "Valid email.";
} else {
echo "Invalid email.";
}
?>
Code language: PHP (php)
Answer: The output will be Valid email.
if the email "[email protected]"
is considered valid.
Question: Explain the concept of caching in PHP and describe a caching mechanism you have used in a previous project.
Answer: Caching is the process of storing frequently accessed data in memory to improve performance and reduce the load on the database or other resources. A common caching mechanism used in PHP is the Memcached extension, which provides a distributed memory caching system. It allows storing key-value pairs in memory, which can significantly speed up data retrieval.
Question: What design pattern is used in the above below, and what is the purpose of using it?
<?php
class Logger {
private static $instance;
private function __construct() {
// Constructor logic
}
public static function getInstance() {
if (!self::$instance) {
self::$instance = new self();
}
return self::$instance;
}
public function log($message) {
echo $message;
}
}
$logger = Logger::getInstance();
$logger->log("Logging message.");
?>
Code language: PHP (php)
Answer: The design pattern used in the code is the Singleton pattern. It ensures that only one instance of the Logger class exists throughout the application. It is used to provide a global point of access to the logger object and control the instantiation process.
Question: Describe a scenario where you would use regular expressions (regex) in PHP. Provide an example of a regex pattern and its usage.
Answer: Regular expressions are used for pattern matching and manipulation of strings. A scenario where regex can be used is for validating and formatting user input, such as validating email addresses, phone numbers or extracting specific data from a string. For example, a regex pattern /^d{3}-d{3}-d{4}$/
can be used to validate a phone number in the format XXX-XXX-XXXX.
Question: What will be the output of the code below?
<?php
class Cache {
private $data = [];
public function set($key, $value, $expiration = 3600) {
$this->data[$key] = $value;
// Store expiration logic
}
public function get($key) {
if (isset($this->data[$key])) {
return $this->data[$key];
}
return null;
}
}
$cache = new Cache();
$cache->set("key", "value");
echo $cache->get("key");
?>
Code language: PHP (php)
Answer: The output will be "value"
.
Question: Explain the concept of lazy loading in PHP and provide an example of how it can be implemented.
Answer: Lazy loading is a technique where objects or resources are loaded only when they are actually needed. It helps improve performance and memory usage by deferring the initialization of expensive or resource-intensive operations until they are required. An example of lazy loading in PHP could be loading and initializing a database connection only when a database operation is performed.
Question: What will be the output of the code below?
<?php
function factorial($n) {
if ($n <= 1) {
return 1;
}
return $n * factorial($n - 1);
}
echo factorial(5);
?>
Code language: PHP (php)
Answer: The output will be 120
.
Question: Describe a situation where you would optimize PHP code for performance. Explain the optimization techniques you would consider.
Answer: One situation for optimizing PHP code for performance is when dealing with large datasets or high traffic applications. Optimization techniques that can be considered include implementing caching mechanisms, using efficient algorithms and data structures, optimizing database queries, utilizing opcode caching (e.g., APC, OPcache), minimizing database or file system accesses, and reducing code redundancy or unnecessary function calls.
More PHP interview resources
For more guides on improving your knowledge of PHP and acing interviews, we have outlined helpful resources:
1,000 Companies use CoderPad to Screen and Interview Developers
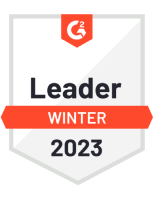
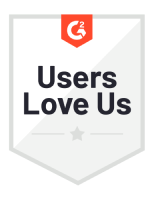
Best interview practices for PHP roles
In order to conduct successful PHP interviews, it is vital to consider various factors, including the candidate’s background and the specific engineering position. To ensure a fruitful interview experience, we recommend adopting the following best practices:
- Develop technical questions that reflect real-world business scenarios within your organization. This approach will effectively engage the candidate and assist in determining their compatibility with your team.
- Cultivate a cooperative environment by inviting the candidate to pose questions during the interview.
- As web development has the potential to expose lots of sensitive user data, make sure your candidates are well versed in security best practices.
Additionally, adhering to standard interview protocols is crucial when conducting PHP interviews. This involves tailoring the complexity of the questions to match the candidate’s capabilities, providing prompt updates on the status of their application, and giving them the opportunity to ask about the evaluation process and working with you and your team.