Perl Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Perl developers for your organization.
Perl
Perl is a popular language for text processing, rapid prototyping, and ensuring cross-platform compatibility.
Larry Wall developed Perl as a general-purpose scripting language, with its first version released in 1987. It was initially designed for text manipulation and system administration tasks but has since evolved into a versatile language used in various domains.
https://www.perl.org/
To evaluate the skill level of developers in Perl during programming interviews, we provide a set of hands-on coding exercises and interview questions below.
Additionally, we have developed a range of suggested methods to ensure that your interview inquiries accurately measure the candidates’ Perl capabilities.
Table of Contents
Perl example question
Help us design a parking lot app
Hey candidate! Welcome to your interview. Boilerplate is provided. Feel free to change the code as you see fit. To run the code at any time, please hit the run button located in the top left corner.
Goals: Design a parking lot using object-oriented principles
Here are a few methods that you should be able to run:
- Tell us how many spots are remaining
- Tell us how many total spots are in the parking lot
- Tell us when the parking lot is full
- Tell us when the parking lot is empty
- Tell us when certain spots are full e.g. when all motorcycle spots are taken
- Tell us how many spots vans are taking up
Assumptions:
- The parking lot can hold motorcycles, cars and vans
- The parking lot has motorcycle spots, car spots and large spots
- A motorcycle can park in any spot
- A car can park in a single compact spot, or a regular spot
- A van can park, but it will take up 3 regular spots
- These are just a few assumptions. Feel free to ask your interviewer about more assumptions as needed
Perl skills to assess
Jobs using Perl
Junior Perl interview questions
Question:
What are scalars in Perl? Provide an example demonstrating the usage of scalars.
Answer:
In Perl, scalars are variables that hold a single value. They can store different types of data, such as numbers, strings, and references. Scalars are denoted by the $
sigil.
Here’s an example demonstrating the usage of scalars:
my $name = "John";
my $age = 25;
print "Name: $namen";
print "Age: $agen";
Code language: PHP (php)
In this example, two scalar variables, $name
and $age
, are declared and assigned values. The print
statement uses the scalar variables to display their values.
Scalars are versatile and widely used in Perl for storing and manipulating individual values. They can be used in mathematical operations, string concatenation, conditional statements, and much more.
Question:
Explain the concept of arrays in Perl. How are arrays used, and what are some common array operations?
Answer:
In Perl, arrays are ordered lists of scalar values. They allow you to store multiple values under a single variable name. Arrays are denoted by the @
sigil.
Arrays are used to group related data together. You can add, remove, and access elements in an array using their indices. Some common array operations include:
- Creating an array:
my @fruits = ("Apple", "Banana", "Orange");
Code language: CSS (css)
- Accessing elements:
print $fruits[0]; # Output: "Apple"
Code language: PHP (php)
- Modifying elements:
$fruits[1] = "Grapes";
Code language: PHP (php)
- Appending elements:
push @fruits, "Mango";
Code language: CSS (css)
- Getting the number of elements:
my $count = scalar @fruits;
Code language: PHP (php)
Arrays provide a convenient way to work with multiple values and perform operations like sorting, searching, and iterating over the elements.
Question:
What are hashes in Perl? How are hashes used, and what are some common hash operations?
Answer:
In Perl, hashes are unordered collections of key-value pairs. They allow you to associate values (called “values”) with unique identifiers (called “keys”). Hashes are denoted by the %
sigil.
Hashes are used to store and retrieve data based on specific identifiers. Each key in a hash is unique, and you can access its corresponding value. Some common hash operations include:
- Creating a hash:
my %student = (
"name" => "John",
"age" => 25,
"grade" => "A"
);
Code language: PHP (php)
- Accessing values:
print $student{"name"}; # Output: "John"
Code language: PHP (php)
- Modifying values:
$student{"age"} = 26;
Code language: PHP (php)
- Adding new key-value pairs:
$student{"city"} = "New York";
Code language: PHP (php)
- Checking if a key exists:
if (exists $student{"grade"}) {
# Do something
}
Code language: PHP (php)
Hashes provide a flexible data structure for organizing and accessing information. They are commonly used for tasks like storing configuration settings, creating lookup tables, and handling data with unique identifiers.
Question:
Explain the concept of control structures in Perl. What are conditional statements and loops, and how are they used in Perl programming? Provide examples.
Answer:
Control structures in Perl allow you to control the flow of program execution based on conditions and loops. They help in making decisions, repeating actions, and executing different parts of the code based on certain conditions.
- Conditional Statements:
Conditional statements in Perl allow you to execute different blocks of code based on specific conditions. Theif-else
statement is commonly used for this purpose.
my $age = 18;
if ($age >= 18) {
print "You are eligible to voten";
} else {
print "You are not eligible to voten";
}
Code language: PHP (php)
In this example, the program checks if the $age
variable is greater than or equal to 18. Depending on the condition, it prints a corresponding message.
- Loops:
Loops in Perl allow you to repeat a block of code multiple times. The two common types of loops in Perl arefor
andwhile
loops.
# Looping with for
for my $i (1..5) {
print "$i ";
}
print "n";
# Looping with while
my $count = 0;
while ($count < 5) {
print "$count ";
$count++;
}
print "n";
Code language: PHP (php)
In this example, the for
loop prints numbers from 1 to 5, and the while
loop prints numbers from 0 to 4.
Control structures are essential in programming to make decisions, iterate over data, and create dynamic behavior. They help create flexible and interactive Perl programs.
Question:
What are regular expressions in Perl? How are regular expressions used, and what are some common applications of regular expressions?
Answer:
In Perl, regular expressions (regex) are patterns used to match and manipulate text based on certain rules. They provide a powerful way to search, extract, and manipulate strings using specific patterns.
Regular expressions are enclosed in forward slashes (/
) and can include metacharacters, literals, character classes, quantifiers, and more. Some common applications of regular expressions in Perl include:
- Pattern matching:
my $string = "The quick brown fox jumps over the lazy dog.";
if ($string =~ /quicks(.+)sfox/) {
print "Matched: $1n";
} else {
print "No matchn";
}
Code language: PHP (php)
In this example, the regular expression /quicks(.+)sfox/
is used to search for a pattern that starts with “quick”, followed by any number of characters, and ends with “fox”. The captured text within the parentheses is stored in the $1
variable and printed.
- Substitution:
Regular expressions can be used to replace specific patterns within a string.
my $string = "Hello, World!";
$string =~ s/Hello/Hi/;
print $string; # Output: "Hi, World!"
Code language: PHP (php)
In this example, the s/Hello/Hi/
regular expression is used to substitute the first occurrence of “Hello” with “Hi” in the string.
Regular expressions are widely used in Perl for tasks such as pattern matching, substitution, validation, data extraction, and text manipulation. They provide a concise and powerful way to work with textual data.
Question:
Explain the concept of subroutines in Perl. What are subroutines, and how are they used in Perl programming? Provide an example demonstrating the usage of subroutines.
Answer:
In Perl, subroutines (also known as functions) are reusable blocks of code that perform specific tasks. They allow you to encapsulate a set of instructions and execute them whenever needed.
Subroutines are defined using the sub
keyword, followed by the subroutine name and a block of code. They can have input parameters (arguments) and may return a value using the return
statement.
Here’s an example demonstrating the usage of subroutines:
sub
greet {
my ($name) = @_;
print "Hello, $name!n";
}
greet("John");
Code language: PHP (php)
In this example, a subroutine named greet
is defined to print a greeting message. The $name
variable is passed as an argument to the subroutine and is used to personalize the greeting.
Subroutines are beneficial in Perl programming as they promote code reusability, modularization, and maintainability. They allow you to break down complex tasks into smaller, manageable units.
Question:
What is the significance of the use strict
and use warnings
pragmas in Perl? How do they help in writing better Perl code?
Answer:
The use strict
and use warnings
pragmas are used in Perl to enforce stricter coding practices and enable additional warnings and error-checking during program execution.
use strict
:
Theuse strict
pragma enforces the use of strict variable declaration rules. It helps prevent the use of undeclared variables, enforces variable scoping, and promotes better coding practices.use warnings
:
Theuse warnings
pragma enables additional warning messages during program execution. It helps identify potential issues, such as uninitialized variables, ambiguous syntax, and other common mistakes.
By including use strict
and use warnings
at the beginning of Perl scripts, you can catch potential errors and enforce better programming practices. They contribute to writing cleaner, more reliable Perl code.
Question:
Explain the concept of file handling in Perl. How can you open, read, write, and close files in Perl? Provide an example demonstrating file operations.
Answer:
File handling in Perl allows you to work with files on the file system, including opening, reading, writing, and closing files. Perl provides various built-in functions and operators for file operations.
Here’s an example demonstrating file operations in Perl:
# Open a file for reading
open(my $file, '<', 'data.txt') or die "Could not open file: $!";
# Read file content
while (my $line = <$file>) {
chomp $line;
print "Line: $linen";
}
# Close the file
close($file);
Code language: PHP (php)
In this example, the open
function is used to open the file named data.txt
for reading (<
mode). The file is then read line by line using the while
loop. The chomp
function is used to remove the newline character from each line. Finally, the file is closed using the close
function.
Perl provides several modes for opening files (<
for reading, >
for writing, >>
for appending, etc.) and various file-related functions (like print
for writing to a file, seek
for file positioning, etc.) that enable efficient file handling.
Question:
What are modules in Perl? How are modules used, and why are they beneficial in Perl programming?
Answer:
Modules in Perl are collections of reusable code that provide additional functionality and extend the capabilities of the language. They encapsulate related subroutines, variables, and constants into a single unit.
Modules are used by including them in Perl scripts using the use
keyword. They provide a convenient way to organize code, promote code reuse, and simplify complex tasks. Perl has a rich ecosystem of modules available on CPAN (Comprehensive Perl Archive Network) that cover a wide range of functionalities.
Here’s an example demonstrating the usage of a module:
use strict;
use warnings;
use Math::Complex;
my $number = Math::Complex->make(3, 4);
print "Real part: " . $number->Re . "n";
print "Imaginary part: " . $number->Im . "n";
Code language: PHP (php)
In this example, the Math::Complex
module is used to create a complex number. The module provides the make
subroutine and accessors (Re
and Im
) to retrieve the real and imaginary parts of the complex number.
Modules are beneficial in Perl programming as they allow developers to leverage existing code, reduce development time, maintain code consistency, and promote code sharing within the Perl community.
Question:
Explain the concept of references in Perl. What are references, and how are they used in Perl programming? Provide an example demonstrating the usage of references.
Answer:
In Perl, references are scalar values that refer to other variables or data structures. They allow you to create complex data structures and pass them around efficiently.
References are created using the backslash () operator. They can refer to scalars, arrays, hashes, subroutines, and even other references. To access the value or data structure pointed to by a reference, you can use the dereference operator (
$
, @
, %
, &
).
Here’s an example demonstrating the usage of references:
my $array_ref = [1, 2, 3];
my $hash_ref = { name => "John", age => 25 };
print $array_ref->[0]; # Output: 1
print $hash_ref->{name}; # Output: "John"
Code language: PHP (php)
In this example, an array reference and a hash reference are created using the square brackets ([]
) and curly braces ({}
) syntax, respectively. The values within the brackets or braces define the elements of the array or the key-value pairs of the hash. To access the elements or values, the dereference operator is used (->
).
References are commonly used in Perl for creating and manipulating complex data structures, passing large data structures to subroutines efficiently, and working with nested data. They provide flexibility and memory efficiency in handling data in Perl.
These answers provide detailed explanations for each question. It is recommended to further explore the Perl documentation and additional resources for a more in-depth understanding of Perl programming.
Intermediate Perl interview questions
Question:
Explain the concept of modules in Perl. What are modules, and how are they used in Perl programming? Provide an example demonstrating the usage of modules.
Answer:
In Perl, modules are reusable collections of code that provide additional functionality and extend the capabilities of the language. They encapsulate related subroutines, variables, and constants into a single unit, allowing for easier code organization and code reuse.
Modules are typically stored in separate files with a .pm
extension and can be used by including them in Perl scripts using the use
keyword. They provide a convenient way to add new features, interact with external libraries, or solve specific problems.
Here’s an example demonstrating the usage of a module:
use strict;
use warnings;
use Math::Trig;
my $angle = 45;
my $radians = deg2rad($angle);
print "Sine of $angle degrees: " . sin($radians) . "n";
Code language: PHP (php)
In this example, the Math::Trig
module is used to perform trigonometric calculations. The deg2rad
function from the module is used to convert the angle from degrees to radians, and then the sin
function is called to calculate the sine of the angle.
Modules provide a way to organize and reuse code, making it easier to maintain and extend Perl programs. They also foster collaboration within the Perl community by allowing developers to share their code through CPAN (Comprehensive Perl Archive Network).
Question:
Explain the concept of object-oriented programming (OOP) in Perl. What is OOP, and how is it implemented in Perl? Provide an example demonstrating the usage of OOP in Perl.
Answer:
Object-oriented programming (OOP) is a programming paradigm that focuses on organizing code around objects, which are instances of classes. It provides a way to model real-world entities as objects, encapsulating data and behavior within them.
In Perl, OOP can be implemented using the built-in bless
function to associate a reference with a specific class. Classes are typically defined as packages, and objects are created by instantiating those classes.
Here’s an example demonstrating the usage of OOP in Perl:
package Person;
sub new {
my ($class, $name, $age) = @_;
my $self = {
name => $name,
age => $age,
};
bless $self, $class;
return $self;
}
sub get_name {
my ($self) = @_;
return $self->{name};
}
sub get_age {
my ($self) = @_;
return $self->{age};
}
# Usage
my $person = Person->new("John", 25);
print "Name: " . $person->get_name() . "n";
print "Age: " . $person->get_age() . "n";
Code language: PHP (php)
In this example, a Person
class is defined within the Person
package. The new
method serves as a constructor to create a new Person
object and initialize its attributes. The get_name
and get_age
methods are used to retrieve the name and age of the person object, respectively.
Object-oriented programming allows for code modularity, reusability, and easier maintenance. It enables the creation of more complex and organized programs by representing entities as objects with their own data and behavior.
Question:
Explain the concept of exceptions in Perl. What are exceptions, and how are they handled in Perl programming? Provide an example demonstrating the usage of exceptions.
Answer:
Exceptions are runtime events that disrupt the normal flow of a program’s execution due to exceptional circumstances or errors. In Perl, exceptions are represented by objects and can be thrown using the die
function or the croak
function from the Carp module.
To handle exceptions, Perl provides the eval
block, which allows you to catch and handle exceptions gracefully.
Here’s an example demonstrating the usage of exceptions in Perl:
use strict;
use warnings;
eval {
open(my $file, '<', 'nonexistent.txt') or die "Could not open file: $!";
# Other code that depends on the file
close($file);
};
if ($@) {
print "An error occurred: $@n";
}
Code language: PHP (php)
In this example, the eval
block is used to catch any exception that may occur within it. The open
function attempts to open a file that does not exist, resulting in an error. The error message is captured in the special variable $@
, and if an exception occurs, the error message is printed.
Handling exceptions allows for more robust error management and graceful handling of exceptional situations in Perl programs.
Question:
Explain the concept of file handling in Perl. How can you open, read, write, and close files in Perl? Provide an example demonstrating file operations.
Answer:
File handling in Perl allows you to work with files on the file system, including opening, reading, writing, and closing files. Perl provides various built-in functions and operators for file operations.
Here’s an example demonstrating file operations in Perl:
use strict;
use warnings;
# Open a file for reading
open(my $file, '<', 'data.txt') or die "Could not open file: $!";
# Read file content
while (my $line = <$file>) {
chomp $
line;
print "Line: $linen";
}
# Close the file
close($file);
Code language: PHP (php)
In this example, the open
function is used to open the file named data.txt
for reading (<
mode). The file is then read line by line using the while
loop. The chomp
function is used to remove the newline character from each line. Finally, the file is closed using the close
function.
Perl provides several modes for opening files (<
for reading, >
for writing, >>
for appending, etc.) and various file-related functions (like print
for writing to a file, seek
for file positioning, etc.) that enable efficient file handling.
Question:
Explain the concept of regular expressions in Perl. What are regular expressions, and how are they used in Perl programming? Provide an example demonstrating the usage of regular expressions.
Answer:
In Perl, regular expressions (regex) are patterns used to match and manipulate text based on certain rules. They provide a powerful way to search, extract, and manipulate strings using specific patterns.
Regular expressions are enclosed in forward slashes (/
) and can include metacharacters, literals, character classes, quantifiers, and more. They are often used with built-in functions such as m//
, s///
, and qr//
.
Here’s an example demonstrating the usage of regular expressions in Perl:
use strict;
use warnings;
my $string = "The quick brown fox jumps over the lazy dog.";
if ($string =~ /quicks(.+)sfox/) {
print "Matched: $1n";
} else {
print "No matchn";
}
Code language: PHP (php)
In this example, the regular expression /quicks(.+)sfox/
is used to search for a pattern that starts with “quick”, followed by any number of characters, and ends with “fox”. The captured text within the parentheses is stored in the $1
variable and printed.
Regular expressions are widely used in Perl for tasks such as pattern matching, substitution, validation, and data extraction. They provide a concise and powerful way to work with textual data.
These answers provide detailed explanations for each question. It is recommended to further explore the Perl documentation and additional resources for a more in-depth understanding of Perl programming.
Certainly! Here are the answers to the remaining five questions:
Question:
Explain the concept of references in Perl. What are references, and how are they used in Perl programming? Provide an example demonstrating the usage of references.
Answer:
In Perl, references are scalar values that allow you to refer to other variables or data structures. They provide a way to create complex data structures and pass them around efficiently.
References are created using the backslash () operator. They can refer to scalars, arrays, hashes, subroutines, and even other references. To access the value or data structure pointed to by a reference, you can use the dereference operator (
$
, @
, %
, &
).
Here’s an example demonstrating the usage of references:
my $array_ref = [1, 2, 3];
my $hash_ref = { name => "John", age => 25 };
print $array_ref->[0]; # Output: 1
print $hash_ref->{name}; # Output: "John"
Code language: PHP (php)
In this example, an array reference and a hash reference are created using the square brackets ([]
) and curly braces ({}
) syntax, respectively. The values within the brackets or braces define the elements of the array or the key-value pairs of the hash. To access the elements or values, the dereference operator is used (->
).
References are commonly used in Perl for creating and manipulating complex data structures, passing large data structures to subroutines efficiently, and working with nested data. They provide flexibility and memory efficiency in handling data in Perl.
Question:
What is the purpose of the use strict
and use warnings
pragmas in Perl? How do they help in writing better Perl code? Provide an example demonstrating the usage of these pragmas.
Answer:
The use strict
and use warnings
pragmas in Perl serve as tools to enforce stricter coding practices and enable additional warnings and error-checking during program execution, resulting in better Perl code.
use strict
:
Theuse strict
pragma enforces the use of strict variable declaration rules. When enabled, it helps prevent the use of undeclared variables, enforces variable scoping, and promotes better coding practices. It catches common mistakes and ensures that variables are declared before use.use warnings
:
Theuse warnings
pragma enables additional warning messages during program execution. It helps identify potential issues such as uninitialized variables, ambiguous syntax, and other common mistakes. By enabling warnings, you get more information about possible problems in your code and can address them proactively.
Here’s an example demonstrating the usage of these pragmas:
use strict;
use warnings;
my $number = 42; # Warning: Use of uninitialized value $number
print $number; # Error: Global symbol "$number" requires explicit package name
Code language: PHP (php)
In this example, without the use strict
pragma, the uninitialized variable $number
would not have raised any warning or error. However, with use strict
and use warnings
, the program alerts you to the use of an uninitialized variable and the requirement to specify the package name for accessing variables.
By including use strict
and use warnings
at the beginning of Perl scripts, you can catch potential errors, enforce better programming practices, and produce cleaner, more reliable Perl code.
Question:
Explain the concept of subroutine references in Perl. What are subroutine references, and how are they used in Perl programming? Provide an example demonstrating the usage of subroutine references.
Answer:
In Perl, subroutine references are references to subroutines. They allow you to treat subroutines as data, enabling you to pass them as arguments to other subroutines, store them in data structures, and invoke them dynamically.
Subroutine references are created using the &
syntax. They can be dereferenced and called using the ->
operator.
Here’s an example demonstrating the usage of subroutine references:
sub greet {
my ($name) = @_;
print "Hello, $name!n";
}
my $greet_ref = &greet;
$greet_ref->("John"); # Output: Hello, John!
Code language: PHP (php)
In this example, the greet
subroutine is defined to print a greeting message. The subroutine reference $greet_ref
is created using the &
syntax, pointing to the greet
subroutine. It can then be invoked using the ->
operator, passing the name as an argument.
Subroutine references are useful when you want to pass subroutines as arguments to other subroutines, create callback mechanisms, or implement higher-order functions in Perl.
Question:
What are anonymous subroutines in Perl? How are they different from named subroutines? Provide an example demonstrating the usage of anonymous subroutines.
Answer:
In Perl, anonymous subroutines are subroutines that are not named and do not have a permanent identifier. They are defined on the fly and can be assigned to variables or used directly in code.
Anonymous subroutines are created using the sub {}
syntax. They can be stored in scalar variables or passed as arguments to other subroutines.
Here’s an example demonstrating the usage of anonymous subroutines:
my $greet = sub {
my ($name) = @_;
print "Hello, $name!n";
};
$greet->("John"); # Output: Hello, John!
Code language: PHP (php)
In this example, an anonymous subroutine is defined and assigned to the scalar variable $greet
. The subroutine takes a name as an argument and prints a greeting message. The subroutine is then invoked using the ->
operator, passing the name as an argument.
Anonymous subroutines are useful when you need to define small, one-time subroutines, create callbacks, or provide inline functionality without the need for named subroutines.
Question:
Explain the concept of object serialization in Perl. What is serialization, and how is it used in Perl programming? Provide an example demonstrating the serialization and deserialization of an object in Perl.
Answer:
Object serialization is the process of converting an object’s state into a format that can be stored, transmitted, or later reconstructed. In Perl, serialization is typically achieved using modules such as Storable
, JSON
, or Data::Dumper
.
Serialization allows you to save an object’s state to disk, send it over a network, or store it in a database. Later, the serialized data can be deserialized to recreate the original object.
Here’s an example demonstrating the serialization and deserialization of an object using the Storable
module:
use strict;
use warnings;
use Storable;
# Object creation
my $person = {
name => "John",
age => 25,
};
# Serialization
store $person, "person_data.bin";
# Deserialization
my $restored_person = retrieve("person_data.bin");
# Accessing restored object
print "Name: " . $restored_person->{name} . "n"; # Output: Name: John
print "Age: " . $restored_person->{age} . "n"; # Output: Age: 25
Code language: PHP (php)
In this example, a $person
hash reference is created to represent a person object. The store
function from the Storable
module is used to serialize
the object and save it to a file named “person_data.bin”. Later, the retrieve
function is used to deserialize the data from the file, restoring the object to its original state.
Serialization is valuable in scenarios where you need to persist object data, share data across different platforms or programming languages, or maintain object state between different program executions.
These answers provide detailed explanations for each question. It is recommended to further explore the Perl documentation and additional resources for a more in-depth understanding of Perl programming.
Senior Perl interview questions
Question:
Explain the concept of closures in Perl. What are closures, and how are they used in Perl programming? Provide an example demonstrating the usage of closures.
Answer:
In Perl, closures are anonymous subroutines that have access to variables outside of their own scope. They “close over” variables from the surrounding scope, retaining their values even after the surrounding scope has finished executing.
Closures are created using the combination of anonymous subroutines and lexical variables. They are useful for encapsulating data and behavior, creating callbacks, and maintaining private data within a subroutine.
Here’s an example demonstrating the usage of closures:
sub counter {
my ($start) = @_;
return sub {
return ++$start;
};
}
my $increment = counter(0);
print $increment->(); # Output: 1
print $increment->(); # Output: 2
Code language: PHP (php)
In this example, the counter
subroutine returns an anonymous subroutine that maintains its own copy of the $start
variable. Each time the anonymous subroutine is called using $increment->()
, it increments and returns the current value of $start
. The value of $start
is preserved between calls due to the closure.
Closures are powerful constructs in Perl that enable advanced programming techniques, such as memoization, currying, and maintaining stateful behavior within subroutines.
Question:
Explain the concept of tie in Perl. What is tying, and how is it used in Perl programming? Provide an example demonstrating the usage of tie.
Answer:
In Perl, tying is a mechanism that allows you to associate certain behaviors with variables. It enables you to control how variables are accessed, modified, and stored.
Tying is implemented using the tie
function and tie classes. When you tie a variable to a tie class, any operations on that variable are intercepted and processed by the associated tie class.
Here’s an example demonstrating the usage of tie:
use Tie::Scalar;
tie my $counter, 'Tie::Scalar';
$counter = 0;
print ++$counter; # Output: 1
print ++$counter; # Output: 2
Code language: PHP (php)
In this example, the Tie::Scalar
module is used to tie the scalar variable $counter
to a tie class. The tie
function establishes the connection between the variable and the tie class. After tying, any operations on $counter
are intercepted and handled by the tie class.
Tying is commonly used for implementing custom behaviors on variables, such as automatic validation, logging, or constraint enforcement. It allows for fine-grained control over how variables are accessed and modified.
Question:
Explain the concept of attributes in Perl. What are attributes, and how are they used in Perl programming? Provide an example demonstrating the usage of attributes.
Answer:
In Perl, attributes are a way to associate metadata or additional information with variables, subroutines, or classes. They provide a mechanism for adding extra meaning or behavior to Perl constructs.
Attributes are defined using the Attribute::Handlers
module and can be attached to various Perl constructs using the ATTRIBUTE
syntax. They can influence the behavior of subroutines, affect how variables are accessed, or modify class definitions.
Here’s an example demonstrating the usage of attributes:
use Attribute::Handlers;
sub my_attribute : Foo {
# Subroutine code here
}
my $var : Bar;
Code language: PHP (php)
In this example, the my_attribute
subroutine is defined with the Foo
attribute attached to it. The attribute can be used to modify the behavior of the subroutine, trigger additional actions, or provide additional context.
The $var
variable is declared with the Bar
attribute, allowing the attribute to affect how the variable is accessed or modified.
Attributes provide a flexible way to extend Perl constructs with additional functionality or metadata. They can be used to enforce constraints, define custom behaviors, or implement domain-specific features.
Question:
Explain the concept of autovivification in Perl. What is autovivification, and how does it occur in Perl programming? Provide an example demonstrating autovivification.
Answer:
Autovivification is a Perl feature where data structures (such as arrays and hashes) are automatically created or expanded when they are accessed or assigned a value. It allows for convenient and dynamic data structure manipulation without the need for explicit initialization.
Autovivification occurs when a nonexistent array element or hash key is accessed or assigned. Perl automatically creates the missing elements or keys, expanding the data structure as needed.
Here’s an example demonstrating autovivification:
my %data;
$data{person}{name} = "John";
$data{person}{age} = 25;
print $data{person}{name}; # Output: John
Code language: PHP (php)
In this example, %data
is a hash that is autovivified when accessed with nested keys. When the $data{person}{name}
and $data{person}{age}
assignments are made, Perl automatically creates the nested hash references (person
and its subkeys) as necessary.
Autovivification simplifies the manipulation of complex data structures by automatically creating the required elements on the fly. However, it’s important to be aware of autovivification’s behavior, as it can sometimes lead to unexpected results if not handled carefully.
Question:
Explain the concept of function signatures in Perl. What are function signatures, and how are they used in Perl programming? Provide an example demonstrating the usage of function signatures.
Answer:
Function signatures in Perl are a language feature that allows you to define formal parameter lists for subroutines. They provide a way to specify the expected number and types of arguments passed to a subroutine.
Function signatures are implemented using the signatures
feature, which can be enabled with the use feature 'signatures'
pragma or by using the use v5.20
pragma.
Here’s an example demonstrating the usage of function signatures:
use feature 'signatures';
sub greet($name) {
print "Hello, $name!n";
}
greet("John"); # Output: Hello, John!
Code language: PHP (php)
In this example, the greet
subroutine is defined with a function signature specifying a single parameter $name
. The function signature allows Perl to enforce the presence and type of the argument passed to the subroutine.
Function signatures provide a more expressive way to define subroutines and communicate their expected parameters. They help improve code readability and catch potential errors at compile-time.
Question:
Explain the concept of the @INC
array in Perl. What is @INC
, and how is it used in Perl programming? Provide an example demonstrating the usage of @INC
.
Answer:
In Perl, @INC
is a special array that contains a list of directories where Perl searches for module files during runtime. It is part of Perl’s module loading system and plays a crucial role in locating and including Perl modules.
When Perl encounters a use
statement, it searches for the specified module in the directories listed in @INC
. If the module is found, it is loaded and made available for use.
Here’s an example demonstrating the usage of @INC
:
use strict;
use warnings;
foreach my $dir (@INC) {
print "$dirn";
}
Code language: PHP (php)
In this example, the `@
INCarray is iterated using a
foreachloop, and each directory in
@INC` is printed. The directories represent locations where Perl searches for modules.
By default, @INC
contains the current directory (.
) and other directories specific to the Perl installation. You can modify @INC
at runtime to include additional directories or manipulate the search order for modules.
Understanding and utilizing @INC
is crucial for managing Perl modules and ensuring that Perl can locate the required module files during program execution.
Question:
Explain the concept of source filters in Perl. What are source filters, and how are they used in Perl programming? Provide an example demonstrating the usage of source filters.
Answer:
In Perl, source filters are a mechanism for transforming Perl source code before it is executed. They allow you to modify or extend Perl syntax, add language features, or perform source code preprocessing.
Source filters are implemented using the Filter::Simple
module or other filter modules available on CPAN. They provide a way to modify the lexical structure of Perl programs at compile-time.
Here’s an example demonstrating the usage of source filters:
use Filter::Simple;
FILTER {
s/awesome/great/g;
};
print "Perl is awesome!n"; # Output: Perl is great!
Code language: PHP (php)
In this example, the Filter::Simple
module is used to define a source filter. The FILTER
block contains a substitution (s/awesome/great/g
), which replaces all occurrences of “awesome” with “great” in the source code.
When the Perl program is executed, the source filter is applied, modifying the code before it is executed.
Source filters can be useful for implementing domain-specific languages (DSLs), modifying Perl syntax, or performing source code transformations. However, caution should be exercised when using source filters, as they can introduce complexity and make code harder to maintain.
Question:
Explain the concept of multithreading in Perl. What is multithreading, and how is it used in Perl programming? Provide an example demonstrating the usage of multithreading.
Answer:
Multithreading in Perl refers to the execution of multiple threads concurrently within a Perl program. Each thread represents a separate flow of control, allowing for concurrent execution of different tasks.
Multithreading in Perl is typically achieved using the threads
module, which provides facilities for creating and managing threads.
Here’s an example demonstrating the usage of multithreading:
use threads;
sub greet {
my ($name) = @_;
print "Hello, $name!n";
}
my $thread = threads->create('greet', 'John');
$thread->join();
Code language: PHP (php)
In this example, a thread is created using the threads
module’s create
method, specifying the subroutine greet
and the argument 'John'
. The thread runs concurrently with the main program, executing the greet
subroutine.
Multithreading allows for parallel execution of code and can be useful for performing computationally intensive tasks, handling multiple I/O operations simultaneously, or achieving better performance in certain scenarios.
Question:
Explain the concept of XS in Perl. What is XS, and how is it used in Perl programming? Provide an example demonstrating the usage of XS.
Answer:
XS is a mechanism in Perl that allows you to write and use C or C++ code directly within Perl programs. It provides an interface between Perl and native compiled code, enabling the development of Perl extensions with improved performance or access to existing C libraries.
XS code consists of C or C++ code that is compiled into a shared library and loaded into Perl at runtime. It allows Perl to call functions, manipulate data structures, and interact with C or C++ libraries.
Here’s an example demonstrating the usage of XS:
use Inline 'C';
my $result = calculate(10, 20);
print "Result: $resultn";
__END__
__C__
int calculate(int a, int b) {
return a + b;
}
Code language: PHP (php)
In this example, the Inline
module is used to define an XS subroutine called calculate
. The XS code is written between __C__
markers and contains the C implementation of the calculate
function, which performs a simple addition.
When the Perl program is executed, the XS code is compiled into a shared library and loaded into Perl. The calculate
function can then be called as a regular Perl subroutine.
XS provides a way to enhance Perl programs with high-performance C or C++ code, interact with existing libraries, or access system-level functionality not available directly in Perl.
Question:
Explain the concept of memoization in Perl. What is memoization, and how is it used in Perl programming? Provide an example demonstrating the usage of memoization.
Answer:
Memoization is a technique in Perl used to optimize the performance of functions by caching their return values based on the input arguments. It allows functions to avoid redundant calculations by storing and reusing previously computed results.
Memoization is typically implemented using hash tables or caching modules such as Memoize
. The cached results are looked up based on the function arguments, and if a matching result is found, it is returned directly instead of recomputing the result.
Here’s an example demonstrating the usage of memoization:
use Memoize;
sub fibonacci {
my ($n) = @_;
return $n if $n < 2;
return fibonacci($n - 1) + fibonacci($n - 2);
}
memoize('fibonacci');
print fibonacci(10); # Output: 55
Code language: PHP (php)
In this example, the fibonacci
function calculates the Fibonacci number recursively. By applying memoization with the memoize
function, the intermediate results are cached, preventing redundant calculations for the same inputs.
Memoization can significantly improve the performance of recursive or computationally expensive functions by avoiding unnecessary work. It is particularly beneficial for functions with repeated calculations or a large number of recursive calls.
These answers provide detailed explanations for each question. It is recommended to further explore the Perl documentation and additional resources for a more in-depth understanding of Perl programming.
1,000 Companies use CoderPad to Screen and Interview Developers
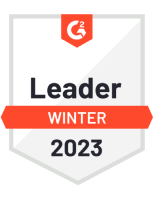
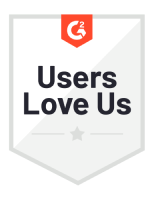
Interview best practices for Perl roles
When conducting effective Perl interviews, it’s crucial to consider various factors, such as the applicants’ experience levels and the specific engineering role they’re seeking. To ensure your Perl interview questions yield the best results, we recommend adhering to these best practices while interacting with candidates:
- Develop technical questions that mirror real-life scenarios within your organization. This approach will not only engage the candidate but also enable you to more precisely evaluate their fit for your team.
- Encourage a cooperative atmosphere by allowing candidates to ask questions throughout the interview.
- If you’re using Perl for web development, also ensure candidates have an understanding of HTML, CSS, and JavaScript.
- You may also check if candidates have any interest on Raku, a “sister language” created by the Perl Foundation, which aims to improve the original design of the Perl language.
Moreover, it’s important to follow standard interview practices when executing Perl interviews. This includes adjusting question difficulty based on the applicant’s skill level, providing timely feedback on their application status, and enabling candidates to ask about the assessment or collaborate with you and your team.