OCaml Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified OCaml developers for your organization.
OCaml
OCaml is widely used in various industries due to its strong static type system, functional programming paradigm, and high-performance capabilities, which together facilitate the development of reliable, efficient, and maintainable software applications.
OCaml, short for “Objective Caml,” was developed in the mid-1990s at the French Institute for Research in Computer Science and Automation (INRIA) by Xavier Leroy, Jérôme Vouillon, Damien Doligez, and others. It evolved from an earlier language called Caml, which itself was a dialect of the ML (Meta Language) family.
https://ocaml.org/learn/history.html
To assess the proficiency of OCaml developers in programming interviews, we offer an array of practical coding tasks and interview topics detailed below. Furthermore, we have devised various recommended approaches to guarantee that your interview questions effectively gauge the candidates’ OCaml competencies.
Table of Contents
OCaml example question
Help us design a parking lot app
Hey candidate! Welcome to your interview. Boilerplate is provided. Feel free to change the code as you see fit. To run the code at any time, please hit the run button located in the top left corner.
Goals: Design a parking lot using object-oriented principles
Here are a few methods that you should be able to run:
- Tell us how many spots are remaining
- Tell us how many total spots are in the parking lot
- Tell us when the parking lot is full
- Tell us when the parking lot is empty
- Tell us when certain spots are full e.g. when all motorcycle spots are taken
- Tell us how many spots vans are taking up
Assumptions:
- The parking lot can hold motorcycles, cars and vans
- The parking lot has motorcycle spots, car spots and large spots
- A motorcycle can park in any spot
- A car can park in a single compact spot, or a regular spot
- A van can park, but it will take up 3 regular spots
- These are just a few assumptions. Feel free to ask your interviewer about more assumptions as needed
OCaml skills to assess
Jobs using OCaml
Junior OCaml interview questions
Question:
Explain the basic syntax of defining a function in OCaml. Provide an example of a simple function and its usage.
Answer:
In OCaml, functions are defined using the let
keyword followed by the function name, argument(s), and the function body. The syntax for defining a function is as follows:
let function_name arg1 arg2 ... =
(* Function body *)
(* Return value *)
Code language: JavaScript (javascript)
Here’s an example of a simple function that adds two integers:
let add_numbers x y =
x + y
Code language: JavaScript (javascript)
This function takes two arguments, x
and y
, and returns their sum. To use this function, you can call it with appropriate arguments:
let result = add_numbers 3 5
Code language: JavaScript (javascript)
In this case, the add_numbers
function is called with arguments 3
and 5
, and the result is assigned to the result
variable. The value of result
would be 8
.
Question:
Explain the concept of pattern matching in OCaml. How does it work, and what are its benefits? Provide an example demonstrating the usage of pattern matching.
Answer:
Pattern matching is a powerful feature in OCaml that allows you to match and destructure values based on patterns. It provides a concise and elegant way to handle different cases and perform different actions based on the structure of values.
Pattern matching works by comparing the structure of a value against a set of patterns and executing the corresponding code block associated with the matching pattern. It enables you to handle different cases with different behaviors easily.
Here’s an example demonstrating the usage of pattern matching with a function that checks if a given number is even or odd:
let check_even_odd n =
match n with
| 0 -> "Even"
| 1 -> "Odd"
| _ -> "Unknown"
Code language: JavaScript (javascript)
In this example, the check_even_odd
function uses the match
keyword to match the value of n
against different patterns. If n
matches the pattern 0
, the function returns “Even”. If it matches the pattern 1
, the function returns “Odd”. If none of the patterns match, the _
pattern acts as a catch-all and returns “Unknown”.
Question:
Explain the concept of recursive functions in OCaml. How do recursive functions work, and why are they important in functional programming? Provide an example demonstrating the usage of a recursive function.
Answer:
Recursive functions in OCaml are functions that call themselves during their execution. They allow a function to solve a problem by breaking it down into smaller subproblems and solving each subproblem using the same function.
Recursive functions work by defining a base case that specifies when the recursion should terminate, and a recursive case that describes how to break down the problem into smaller parts and make progress towards the base case.
Recursive functions are important in functional programming because they provide a natural and elegant way to express computations that involve repetitive or self-referential patterns. They allow for concise and expressive code that aligns well with the mathematical foundations of functional programming.
Here’s an example demonstrating the usage of a recursive function to calculate the factorial of a number:
let rec factorial n =
if n <= 1 then
1
else
n * factorial (n - 1)
Code language: JavaScript (javascript)
In this example, the factorial
function calculates the factorial of a number n
. If n
is less than or equal to 1
, the base case is reached, and the function returns 1
. Otherwise, it recursively calls itself with n - 1
and multiplies the result by n
. This process continues until the base case is reached.
Question:
Explain the concept of immutable data in OCaml. What does it mean for data to be immutable, and why is it a fundamental concept in functional programming? Provide an example demonstrating the usage of immutable data in OCaml.
Answer:
In OCaml, immutable data refers to data that cannot be modified once it is created. Once a value is assigned to a variable, it cannot be changed, and any operation on the value creates a new value instead of modifying the original value.
Immutable data is a fundamental concept in functional programming because it ensures referential transparency and makes programs easier to reason about. It eliminates the complexity of shared mutable state and enables safe and parallel execution of code.
Here’s an example demonstrating the usage of immutable data in OCaml:
let greet name =
let message = "Hello, " ^ name in
print_endline message
Code language: JavaScript (javascript)
In this example, the greet
function takes a name
parameter and creates an immutable message
variable by concatenating the string “Hello, ” with the name
. The message
is then printed using print_endline
. Once the message
is created, it cannot be modified. Any subsequent operations on the message
would create a new value.
Question:
Explain the concept of higher-order functions in OCaml. What are higher-order functions, and why are they important in functional programming? Provide an example demonstrating the usage of a higher-order function.
Answer:
Higher-order functions in OCaml are functions that take other functions as arguments or return functions as results. They treat functions as first-class values, allowing them to be passed around, composed, and manipulated like any other data.
Higher-order functions are important in functional programming because they enable code abstraction, modularity, and code reuse.
They provide a powerful way to express complex computations by composing and combining simpler functions.
Here’s an example demonstrating the usage of a higher-order function that applies a given function to each element of a list:
let rec map f lst =
match lst with
| [] -> []
| x :: xs -> f x :: map f xs
Code language: JavaScript (javascript)
In this example, the map
function takes a function f
and a list lst
. It applies the function f
to each element of the list using pattern matching. If the list is empty ([]
), an empty list is returned. Otherwise, the function f
is applied to the head of the list (x
) and recursively applied to the tail of the list (xs
), and the results are combined into a new list.
Question:
Explain the concept of variant types in OCaml. What are variant types, and how do they enable modeling of different possibilities? Provide an example demonstrating the usage of variant types.
Answer:
Variant types in OCaml are types that represent a set of distinct possibilities or cases. They allow you to define custom types with different variations, each representing a unique case or value.
Variant types enable modeling and handling of different possibilities in a concise and type-safe manner. They provide a powerful way to express and reason about complex data structures and program behaviors.
Here’s an example demonstrating the usage of variant types to represent different shapes:
type shape =
| Circle of float
| Rectangle of float * float
| Triangle of float * float * float
In this example, the shape
type is defined as a variant type. It has three possible cases: Circle
with a single parameter representing the radius, Rectangle
with two parameters representing the width and height, and Triangle
with three parameters representing the lengths of its sides.
You can create values of the shape
type by using the defined cases:
let circle = Circle 5.0
let rectangle = Rectangle (10.0, 20.0)
let triangle = Triangle (3.0, 4.0, 5.0)
Code language: JavaScript (javascript)
In this case, circle
represents a circle with a radius of 5.0
, rectangle
represents a rectangle with a width of 10.0
and height of 20.0
, and triangle
represents a triangle with side lengths 3.0
, 4.0
, and 5.0
.
Question:
Explain the concept of currying in OCaml. What is currying, and how does it enable the transformation of functions? Provide an example demonstrating the usage of currying.
Answer:
Currying in OCaml is a technique that allows functions with multiple arguments to be transformed into a sequence of functions, each taking a single argument. It enables partial application and the creation of specialized versions of functions.
Currying works by transforming a function that takes multiple arguments into a series of functions, each taking one argument and returning a new function that takes the next argument until all arguments are consumed and the final result is produced.
Here’s an example demonstrating the usage of currying to create a specialized version of a function:
let add x y = x + y
let add_to_five = add 5
Code language: JavaScript (javascript)
In this example, the add
function takes two arguments, x
and y
, and returns their sum. The add_to_five
is a specialized version of the add
function created by partially applying the add
function with the argument 5
. It represents a function that takes a single argument y
and adds it to 5
.
You can use the specialized function add_to_five
like any other function:
let result = add_to_five 3
Code language: JavaScript (javascript)
In this case, result
would be 8
because add_to_five
is equivalent to add 5
, which adds 5
to the provided argument 3
.
Currying provides flexibility and code reuse by allowing the creation of specialized functions from more general ones, reducing the need for explicit parameter passing.
Question:
Explain the concept of modules in OCaml. What are modules, and how do they enable organizing and encapsulating code? Provide an example demonstrating the usage of modules.
Answer:
Modules in OCaml are a way to organize and encapsulate related code and data structures. They allow you to group functions, types, and values together and provide a level of abstraction and modularity.
Modules enable code organization, information hiding, and separation of concerns. They provide a means to structure large codebases, promote code reuse, and ensure encapsulation and encapsulation.
Here’s an example demonstrating the usage of modules to define a module for handling geometric shapes:
module Shapes = struct
type shape =
| Circle of float
| Rectangle of float * float
| Triangle of float * float * float
let area shape =
match shape with
| Circle radius -> 3.14 *. radius *. radius
| Rectangle width height -> width *. height
| Triangle a b c ->
let s = (a +. b +. c) /. 2.0 in
sqrt (s *. (s -. a) *. (s -. b) *. (s
-. c))
end
Code language: JavaScript (javascript)
In this example, a module named Shapes
is defined using the module
keyword. Inside the module, a shape
type is defined as a variant type representing different geometric shapes. The area
function calculates the area of a given shape using pattern matching.
To use the module, you can access its values and functions using the dot notation:
let circle = Shapes.Circle 5.0
let area = Shapes.area circle
Code language: JavaScript (javascript)
In this case, circle
represents a circle with a radius of 5.0
, and area
is calculated using the Shapes.area
function.
Modules provide a powerful mechanism for organizing and structuring code in OCaml, promoting code reuse and modularity.
Question:
Explain the concept of records in OCaml. What are records, and how do they enable the creation of composite data structures? Provide an example demonstrating the usage of records.
Answer:
Records in OCaml are composite data structures that allow you to group related values together. They are similar to structures or objects in other programming languages.
Records enable the creation of custom data types by defining a named collection of fields, each with a specific type. They provide a convenient way to organize and manipulate related data.
Here’s an example demonstrating the usage of records to represent a person:
type person = {
name: string;
age: int;
email: string option;
}
In this example, a person
type is defined as a record with three fields: name
of type string
, age
of type int
, and email
of type string option
, representing an optional email address.
You can create values of the person
type by specifying values for each field:
let john = { name = "John"; age = 25; email = Some "[email protected]" }
Code language: JavaScript (javascript)
In this case, john
represents a person with the name “John”, age 25
, and an email address “[email protected]”.
You can access and update the fields of a record using dot notation:
let name = john.name
let updated_john = { john with age = 26 }
Code language: JavaScript (javascript)
In this case, name
represents the value of the name
field in the john
record, and updated_john
represents a new record with the same fields as john
, but with the age
field updated to 26
.
Records provide a convenient way to represent and manipulate composite data structures in OCaml, enabling structured and type-safe data modeling.
Question:
Explain the concept of functors in OCaml. What are functors, and how do they enable code reuse and modularity? Provide an example demonstrating the usage of functors.
Answer:
Functors in OCaml are higher-order modules that take one or more modules as arguments and return a new module as a result. They enable code reuse and modularity by parameterizing module definitions and allowing the creation of generic modules.
Functors provide a way to define reusable patterns for modules, allowing the creation of module templates that can be instantiated with different implementations. They promote code abstraction, separation of concerns, and modular development.
Here’s an example demonstrating the usage of functors to create a generic stack module:
module type Stack = sig
type 'a stack
val create : unit -> 'a stack
val push : 'a -> 'a stack -> unit
val pop : 'a stack -> 'a option
end
module ListStack : Stack = struct
type 'a stack = 'a list ref
let create () = ref []
let push x s = s := x :: !s
let pop s =
match !s with
| [] -> None
| x :: xs ->
s := xs;
Some x
end
module ArrayStack : Stack = struct
type 'a stack = 'a array ref * int ref
let create () = (ref [||], ref 0)
let push x (arr, size) =
if !size < Array.length !arr then begin
!arr.(!size) <- x;
incr size
end
let pop (arr, size) =
if !size > 0 then begin
decr size;
Some !arr.(!size)
end else
None
end
Code language: PHP (php)
In this example, a Stack
module type is defined with a polymorphic stack type and three operations: create
to create a new stack, push
to push an element onto the stack, and pop
to pop an element from the stack.
Two modules, ListStack
and ArrayStack
, implement the Stack
module type. ListStack
uses a list as the underlying data structure, while ArrayStack
uses an array.
You can use the stack modules as follows:
module MyStack = ListStack
let stack = MyStack.create ()
MyStack.push 42 stack
let value = MyStack.pop stack
Code language: JavaScript (javascript)
In this case, MyStack
is an alias for ListStack
, and a stack is created using MyStack.create()
. An integer value 42
is pushed onto the stack, and then popped using MyStack.pop
.
Functors provide a powerful mechanism for creating reusable and parameterized modules in OCaml, promoting code reuse and modularity.
Intermediate OCaml interview questions
Question:
Explain the concept of algebraic data types (ADTs) in OCaml. What are ADTs, and how do they enable modeling complex data structures? Provide an example demonstrating the usage of ADTs.
Answer:
Algebraic Data Types (ADTs) in OCaml are composite types that allow you to define and model complex data structures. ADTs combine different types together using sum types (disjoint unions) and product types (records).
Sum types represent a choice between different alternatives, while product types represent a combination of multiple values.
Here’s an example demonstrating the usage of ADTs to model a binary tree:
type 'a tree =
| Leaf
| Node of 'a * 'a tree * 'a tree
Code language: PHP (php)
In this example, the tree
type is defined as an ADT. It has two cases: Leaf
, representing an empty tree, and Node
, representing a tree node with a value of type 'a
and two child trees.
You can create values of the tree
type by using the defined cases:
let tree1 = Node (1, Leaf, Leaf)
let tree2 = Node (2, Node (1, Leaf, Leaf), Node (3, Leaf, Leaf))
Code language: JavaScript (javascript)
In this case, tree1
represents a tree with a single node containing the value 1
and two empty subtrees, while tree2
represents a tree with a root node containing the value 2
, a left subtree with a node containing the value 1
, and a right subtree with a node containing the value 3
.
ADTs provide a powerful way to model and manipulate complex data structures in a type-safe manner, enabling expressive and robust programs.
Question:
Explain the concept of higher-order functions in OCaml. What are higher-order functions, and why are they important in functional programming? Provide an example demonstrating the usage of a higher-order function.
Answer:
Higher-order functions in OCaml are functions that can take other functions as arguments or return functions as results. They treat functions as first-class values, allowing them to be passed around, composed, and manipulated like any other data.
Higher-order functions are important in functional programming because they enable code abstraction, modularity, and code reuse. They provide a powerful way to express complex computations by composing and combining simpler functions.
Here’s an example demonstrating the usage of a higher-order function that applies a given function to each element of a list:
let rec map f lst =
match lst with
| [] -> []
| x :: xs -> f x :: map f xs
Code language: JavaScript (javascript)
In this example, the map
function takes a function f
and a list lst
. It applies the function f
to each element of the list using pattern matching. If the list is empty ([]
), an empty list is returned. Otherwise, the function f
is applied to the head of the list (x
) and recursively applied to the tail of the list (xs
), and the results are combined into a new list.
Question:
Explain the concept of currying in OCaml. What is currying, and how does it enable the transformation of functions? Provide an example demonstrating the usage of currying.
Answer:
Currying in OCaml is a technique that allows functions with multiple arguments to be transformed into a sequence of functions, each taking a single argument. It enables partial application and the creation of specialized versions of functions.
Currying works by transforming a function that takes multiple arguments into a series of functions, each taking one argument and returning a new function that takes the next argument until all arguments are consumed and the final result is produced.
Here’s an example demonstrating the usage of currying to create a specialized version of a function:
let add x y = x + y
let add_to_five = add 5
Code language: JavaScript (javascript)
In this example, the add
function takes two arguments, x
and y
, and returns their sum. The add_to_five
is a specialized version of the add
function created by partially applying the add
function with the argument 5
. It represents a function that takes a single argument y
and adds it to 5
.
You can use the specialized function add_to_five
like any other function:
let result = add_to_five 3
Code language: JavaScript (javascript)
In this case, result
would be 8
because add_to_five
is equivalent to add 5
, which adds 5
to the provided argument 3
.
Currying provides flexibility and code reuse by allowing the creation of specialized functions from more general ones, reducing the need for explicit parameter passing.
Question:
Explain the concept of pattern matching in OCaml. How does it work, and what are its benefits? Provide an example demonstrating the usage of pattern matching.
Answer:
Pattern matching is a powerful feature in OCaml that allows you to match and destructure values based on patterns. It provides a concise and elegant way to handle different cases and perform different actions based on the structure of values.
Pattern matching works by comparing the structure of a value against a set of patterns and executing the corresponding code block associated with the matching pattern. It enables you to handle different cases with different behaviors easily.
Here’s an example demonstrating the usage of pattern matching with a function that checks if a given number is even or odd:
let check_even_odd n =
match n with
| 0 -> "Even"
| 1 -> "Odd"
| _ -> "Unknown"
Code language: JavaScript (javascript)
In this example, the check_even_odd
function uses the match
keyword to match the value of n
against different patterns. If n
matches the pattern 0
, the function returns “Even”. If it matches the pattern 1
, the function returns “Odd”. If none of the patterns match, the _
pattern acts as a catch-all and returns “Unknown”.
Pattern matching simplifies conditional branching and enables concise and readable code, making it easier to handle different cases and perform appropriate actions based on the structure of values.
Question:
Explain the concept of modules in OCaml. What are modules, and how do they enable organizing and encapsulating code? Provide an example demonstrating the usage of modules.
Answer:
Modules in OCaml are a way to organize and encapsulate related code and data structures. They allow you to group functions, types, and values together and provide a level of abstraction and modularity.
Modules enable code organization, information hiding, and separation of concerns. They provide a means to structure large codebases, promote code reuse, and ensure encapsulation and encapsulation.
Here’s an example demonstrating the usage of modules to define a module for handling geometric shapes:
module Shapes = struct
type shape =
| Circle of float
| Rectangle of float * float
| Triangle of float * float * float
let area shape =
match shape with
| Circle radius -> 3.14 *. radius *. radius
| Rectangle width height -> width *. height
| Triangle a b c ->
let s = (a +. b +. c) /. 2.0 in
sqrt (s *. (s -. a) *. (s -. b)
*. (s -. c))
end
Code language: JavaScript (javascript)
In this example, a module named Shapes
is defined using the module
keyword. Inside the module, a shape
type is defined as a variant type representing different geometric shapes. The area
function calculates the area of a given shape using pattern matching.
To use the module, you can access its values and functions using the dot notation:
let circle = Shapes.Circle 5.0
let area = Shapes.area circle
Code language: JavaScript (javascript)
In this case, circle
represents a circle with a radius of 5.0
, and area
is calculated using the Shapes.area
function.
Modules provide a powerful mechanism for organizing and structuring code in OCaml, promoting code reuse and modularity.
Question:
Explain the concept of polymorphism in OCaml. What is polymorphism, and how does it enable code reuse and flexibility? Provide an example demonstrating the usage of polymorphic functions.
Answer:
Polymorphism in OCaml refers to the ability to write code that can operate on values of different types. It enables code reuse and flexibility by allowing functions to be defined and used with different types, promoting generic programming.
There are two types of polymorphism in OCaml: parametric polymorphism and ad hoc polymorphism.
Parametric polymorphism allows functions to be defined in a generic way, independently of specific types. It enables code to be reused across a wide range of types.
Here’s an example demonstrating the usage of polymorphic functions with parametric polymorphism:
let identity x = x
let length lst =
let rec aux acc = function
| [] -> acc
| _ :: xs -> aux (acc + 1) xs
in
aux 0 lst
In this example, the identity
function takes a single argument x
and returns it unchanged. The length
function calculates the length of a list by recursively traversing the list and incrementing an accumulator.
Both functions are polymorphic and can operate on values of any type. The identity
function maintains the type of its input, while the length
function can work with lists of any type.
This polymorphic behavior allows these functions to be reused across different types, providing code flexibility and avoiding code duplication.
Question:
Explain the concept of records in OCaml. What are records, and how do they enable the creation of composite data structures? Provide an example demonstrating the usage of records.
Answer:
Records in OCaml are composite data structures that allow you to group related values together. They are similar to structures or objects in other programming languages.
Records enable the creation of custom data types by defining a named collection of fields, each with a specific type. They provide a convenient way to organize and manipulate related data.
Here’s an example demonstrating the usage of records to represent a person:
type person = {
name: string;
age: int;
email: string option;
}
In this example, a person
type is defined as a record with three fields: name
of type string
, age
of type int
, and email
of type string option
, representing an optional email address.
You can create values of the person
type by specifying values for each field:
let john = { name = "John"; age = 25; email = Some "[email protected]" }
Code language: JavaScript (javascript)
In this case, john
represents a person with the name “John”, age 25
, and an email address “[email protected]”.
You can access and update the fields of a record using dot notation:
let name = john.name
let updated_john = { john with age = 26 }
Code language: JavaScript (javascript)
In this case, name
represents the value of the name
field in the john
record, and updated_john
represents a new record with the same fields as john
, but with the age
field updated to 26
.
Records provide a convenient way to represent and manipulate composite data structures in OCaml, enabling structured and type-safe data modeling.
Question:
Explain the concept of exceptions in OCaml. What are exceptions, and how do they enable error handling? Provide an example demonstrating the usage of exceptions.
Answer:
Exceptions in OCaml are a mechanism for handling and propagating exceptional conditions or errors that occur during program execution. They allow you to gracefully handle error scenarios and control the program’s flow when exceptional situations arise.
Exceptions provide a way to separate the normal program execution path from error handling and recovery code, making it easier to manage and reason about exceptional conditions.
Here’s an example demonstrating the usage of exceptions:
exception DivisionByZero
let safe_divide x y =
if y = 0 then
raise DivisionByZero
else
x / y
Code language: JavaScript (javascript)
In this example, the DivisionByZero
exception is defined using the exception
keyword. The safe_divide
function takes two integers x
and y
and checks if y
is zero. If y
is zero, it raises the DivisionByZero
exception. Otherwise, it performs the division x / y
.
To handle exceptions, you can use the try...with
construct:
try
let result = safe_divide 10 0 in
print_int result
with
| DivisionByZero -> print_string "Division by zero error"
Code language: JavaScript (javascript)
In this case, an attempt to divide 10
by 0
would raise the DivisionByZero
exception. The exception is caught by the try...with
construct, and the associated error message is printed.
Exceptions provide a structured and controlled way to handle errors and exceptional situations, improving the reliability and robustness of OCaml programs.
Question:
Explain the concept of modules and functors in OCaml. How do modules and functors enable code reuse and modularity? Provide an example demonstrating the usage of modules and functors.
Answer:
Modules and functors in OCaml are powerful features that enable code reuse, encapsulation, and modularity. They allow you to organize and structure code, promote information hiding, and create reusable components.
Modules provide a way to group related functions, types, and values together, encapsulating them and providing a level of abstraction. They enable code organization, separation of concerns, and facilitate the creation of reusable code components.
Here’s an example demonstrating the usage of modules to create a module for handling mathematical operations:
module Math = struct
let square x = x * x
let cube x = x * x * x
end
Code language: JavaScript (javascript)
In this example, a module named Math
is defined using the module
keyword. It contains two functions, square
and cube
, which perform mathematical operations on their input.
To use the module, you can access its functions using the dot notation:
let result1 = Math.square 5
let result2 = Math.cube 3
Code language: JavaScript (javascript)
In this case, result1
would be 25
, and result2
would be 27
, obtained by calling the square
and cube
functions from the Math
module.
Functors, on the other hand, are higher-order modules that take one or
more modules as arguments and return a new module as a result. They enable parameterizing module definitions and creating generic and reusable modules.
Here’s an example demonstrating the usage of a functor to create a generic set module:
module type Set = sig
type 'a t
val empty : 'a t
val insert : 'a -> 'a t -> 'a t
val contains : 'a -> 'a t -> bool
end
module ListSet : Set = struct
type 'a t = 'a list
let empty = []
let insert x set = x :: set
let contains x set = List.mem x set
end
Code language: PHP (php)
In this example, a module type Set
is defined, representing a generic set. A ListSet
module is created using a functor that takes a module of type Set
as an argument and implements the set operations using a list.
You can use the set module as follows:
module MySet = ListSet
let set = MySet.insert 42 MySet.empty
let contains = MySet.contains 42 set
Code language: JavaScript (javascript)
In this case, MySet
is an alias for ListSet
, and a set is created by inserting 42
into an empty set. The contains
function checks if 42
is present in the set.
Modules and functors provide a powerful way to organize, reuse, and modularize code in OCaml, promoting code maintainability and extensibility.
Question:
Explain the concept of polymorphic variants in OCaml. What are polymorphic variants, and how do they enable flexible and extensible code? Provide an example demonstrating the usage of polymorphic variants.
Answer:
Polymorphic variants in OCaml are a feature that allows the creation of extensible and flexible types by combining different cases together. They provide a way to define open-ended and heterogeneous data structures.
Polymorphic variants enable the creation of types with varying sets of cases that can be extended or combined with other types, promoting code flexibility and adaptability.
Here’s an example demonstrating the usage of polymorphic variants to define and manipulate different shapes:
type shape =
[ `Circle of float
| `Rectangle of float * float
| `Triangle of float * float * float
]
let calculate_area shape =
match shape with
| `Circle radius -> 3.14 *. radius *. radius
| `Rectangle width height -> width *. height
| `Triangle a b c ->
let s = (a +. b +. c) /. 2.0 in
sqrt (s *. (s -. a) *. (s -. b) *. (s -. c))
Code language: JavaScript (javascript)
In this example, the shape
type is defined as a polymorphic variant. It has three cases: Circle
with a single parameter representing the radius, Rectangle
with two parameters representing the width and height, and Triangle
with three parameters representing the lengths of its sides.
The calculate_area
function takes a shape and calculates its area using pattern matching.
You can create values of the shape
type by using the defined cases:
let circle = `Circle 5.0
let rectangle = `Rectangle (10.0, 20.0)
let triangle = `Triangle (3.0, 4.0, 5.0)
Code language: JavaScript (javascript)
In this case, circle
represents a circle with a radius of 5.0
, rectangle
represents a rectangle with a width of 10.0
and height of 20.0
, and triangle
represents a triangle with side lengths 3.0
, 4.0
, and 5.0
.
Polymorphic variants provide a flexible and extensible way to define and manipulate heterogeneous data structures in OCaml, enabling dynamic and adaptable code.
Senior OCaml interview questions
Question:
Explain the concept of type inference in OCaml. How does type inference work, and what are its benefits? Provide an example demonstrating type inference in OCaml.
Answer:
Type inference in OCaml is the process of automatically deducing the types of expressions and variables in a program without the need for explicit type annotations. It allows the compiler to infer the types based on the structure and usage of the code.
Type inference works by analyzing the flow of values and expressions in the program, checking their compatibility, and deducing the most general type that satisfies all the constraints.
Here’s an example demonstrating type inference in OCaml:
let add x y = x + y
let result = add 3 4
Code language: JavaScript (javascript)
In this example, the add
function takes two integer arguments x
and y
and returns their sum. The result
variable is assigned the value returned by calling the add
function with the arguments 3
and 4
.
The OCaml compiler can infer that x
and y
are of type int
based on the usage of the +
operator and the provided arguments. Therefore, the add
function has the inferred type int -> int -> int
, meaning it takes two integers and returns an integer.
Type inference in OCaml eliminates the need for explicit type annotations in many cases, reducing the burden of type specification and allowing for more concise and readable code. It also provides static type checking and helps catch type-related errors at compile-time.
Question:
Explain the concept of polymorphic recursion in OCaml. What is polymorphic recursion, and how does it enable the definition of recursive functions on multiple types? Provide an example demonstrating the usage of polymorphic recursion.
Answer:
Polymorphic recursion in OCaml allows the definition of recursive functions that operate on multiple types, including recursive types. It enables the creation of generic and reusable recursive algorithms.
Polymorphic recursion works by allowing the types of the function arguments and return values to be polymorphic, meaning they can be instantiated to different types depending on the usage.
Here’s an example demonstrating the usage of polymorphic recursion to define a generic length function for lists:
let rec length : 'a. 'a list -> int = function
| [] -> 0
| _ :: xs -> 1 + length xs
In this example, the length
function takes a polymorphic argument 'a list
, representing a list of values of any type 'a
, and returns an integer representing the length of the list.
The recursive definition of length
calls itself on the tail of the list (xs
), enabling the computation of the length recursively.
Polymorphic recursion allows the length
function to be used with lists of any type, such as int list
, string list
, or even recursive types like ('a * 'a) list
. It provides a powerful mechanism for creating generic and reusable recursive algorithms that can operate on different types.
Question:
Explain the concept of abstract types in OCaml. What are abstract types, and how do they enable information hiding and encapsulation? Provide an example demonstrating the usage of abstract types.
Answer:
Abstract types in OCaml are types that are intentionally hidden or abstracted from the external code. They enable information hiding and encapsulation by allowing the definition of data structures with hidden implementation details.
Abstract types hide the internal representation of a data structure or module, exposing only a restricted interface or a set of operations through abstract types and signatures.
Here’s an example demonstrating the usage of abstract types to define and manipulate a stack module:
module type Stack = sig
type 'a t
val create : unit -> 'a t
val push : 'a -> 'a t -> unit
val pop : 'a t -> 'a option
end
module ListStack : Stack = struct
type 'a t = 'a list
let create () = []
let push x s = x :: s
let pop = function
| [] -> None
| x :: xs -> Some (x, xs)
end
In this example, a stack module is defined using the Stack
signature. The module provides operations to create a stack, push elements onto the stack, and pop elements from the stack.
The concrete implementation of the stack is hidden as an abstract type 'a t
within the ListStack
module. Users of the module can only interact with the stack through the defined operations, without direct access to the internal representation.
This abstraction allows the internal implementation of the stack to be changed without affecting the external code that relies on the abstract interface. It provides information hiding and encapsulation, promoting modularity and reducing code dependencies.
Question:
Explain the concept of GADTs (Generalized Algebraic Data Types) in OCaml. What are GADTs, and how do they enable the definition of types with refined constraints? Provide an example demonstrating the usage of GADTs.
Answer:
Generalized Algebraic Data Types (GADTs) in OCaml are an extension of algebraic data types that allow the definition of types with refined constraints
. They provide a way to express more precise type information and enable advanced type-based reasoning.
GADTs allow the refinement of types within pattern matching branches, enabling different cases to have distinct and more specific types. This refinement is based on the structure of the pattern being matched.
Here’s an example demonstrating the usage of GADTs to define a type-safe representation of expressions:
type _ expr =
| IntLit : int -> int expr
| BoolLit : bool -> bool expr
| Add : int expr * int expr -> int expr
| And : bool expr * bool expr -> bool expr
let eval : type a. a expr -> a = function
| IntLit n -> n
| BoolLit b -> b
| Add (e1, e2) -> eval e1 + eval e2
| And (e1, e2) -> eval e1 && eval e2
Code language: JavaScript (javascript)
In this example, the expr
type is defined as a GADT representing different types of expressions. Each constructor has a specific type associated with it, capturing the refined constraints on the expression.
The eval
function takes an expression of type a expr
and returns a value of type a
. The pattern matching on the GADT constructors allows the type refinement to guide the evaluation process and enforce type safety.
GADTs enable more precise type checking, finer-grained control over types, and advanced type-based reasoning. They provide a powerful mechanism for expressing complex type constraints and ensuring type correctness.
Apologies for the incomplete response. Here’s the complete answer for question 5:
Question:
Explain the concept of modular programming in OCaml. What is modular programming, and how does it enable code organization and reusability? Provide an example demonstrating modular programming in OCaml.
Answer:
Modular programming in OCaml is a software development approach that emphasizes the organization of code into modules, promoting code reusability, maintainability, and modularity.
Modular programming involves breaking down a complex system into smaller, self-contained modules that encapsulate related functionality and data structures. Each module can be developed and tested independently and then composed together to form the complete system.
Modular programming enables code organization, separation of concerns, and promotes code reuse and modularity. It provides several benefits:
- Encapsulation: Modules allow you to encapsulate related functions, types, and values, hiding the implementation details and providing a well-defined interface. This encapsulation improves code maintainability and reduces the impact of changes within the module.
- Code Reusability: Modules can be reused across different projects or within the same project, promoting code reuse and reducing duplication. Modules provide a clear boundary for reusable components, allowing them to be easily imported and used in various parts of the system.
- Separation of Concerns: By dividing code into modules, you can separate different concerns or aspects of the system. Each module can focus on a specific functionality, making the codebase more manageable and easier to understand. This separation improves code organization and allows for easier debugging and testing.
- Modularity: Modules enable the system to be built and extended in a modular manner. New functionality can be added by creating new modules or extending existing ones. Modules can be combined and composed together to form larger systems, promoting extensibility and scalability.
Here’s an example demonstrating modular programming in OCaml:
(* Module for Math operations *)
module Math = struct
let add x y = x + y
let subtract x y = x - y
let multiply x y = x * y
let divide x y = x / y
end
(* Module for String operations *)
module StringUtils = struct
let capitalize str = String.capitalize_ascii str
let reverse str = String.rev str
let length str = String.length str
end
(* Main Program *)
let () =
let result1 = Math.add 5 3 in
let result2 = StringUtils.capitalize "hello" in
print_endline (string_of_int result1);
print_endline result2
Code language: JavaScript (javascript)
In this example, the code is divided into two modules: Math
and StringUtils
. The Math
module encapsulates mathematical operations, while the StringUtils
module encapsulates string-related operations.
The main program uses the functionality provided by the modules to perform calculations and manipulate strings. Each module serves a specific purpose and can be developed, tested, and maintained independently.
Modular programming allows for better code organization, reusability, and maintainability. It promotes the development of scalable systems by breaking down complex functionality into smaller, manageable modules.
Question:
Explain the concept of higher-order functions in OCaml. What are higher-order functions, and how do they enable functional programming paradigms? Provide an example demonstrating the usage of higher-order functions.
Answer:
Higher-order functions in OCaml are functions that can accept other functions as arguments or return functions as results. They enable functional programming paradigms such as function composition, abstraction, and encapsulation of behavior.
Higher-order functions allow functions to be treated as first-class citizens, enabling powerful and flexible programming techniques.
Here’s an example demonstrating the usage of higher-order functions:
let apply_twice f x = f (f x)
let increment x = x + 1
let square x = x * x
let result1 = apply_twice increment 5
let result2 = apply_twice square 3
Code language: JavaScript (javascript)
In this example, the apply_twice
function is a higher-order function that takes a function f
and a value x
. It applies the function f
twice to the value x
.
The increment
and square
functions are passed as arguments to the apply_twice
function, resulting in the increment of 5
twice and the squaring of 3
twice.
Higher-order functions enable code abstraction and reusability, as well as the composition of functions to create more complex behaviors. They are a fundamental concept in functional programming.
Question:
Explain the concept of monads in OCaml. What are monads, and how do they enable functional programming patterns such as handling side effects and managing state? Provide an example demonstrating the usage of monads.
Answer:
Monads in OCaml are a computational design pattern that enables managing complex computations, handling side effects, and encapsulating stateful computations in a pure functional way.
Monads provide a standardized interface for sequencing operations and controlling the flow of computations. They promote code modularity, maintainability, and enable separation of concerns.
Here’s an example demonstrating the usage of the Option
monad:
let divide x y =
if y = 0 then None
else Some (x / y)
let result =
Option.(
Some 10
>>= fun x -> divide x 2
>>= fun y -> divide y 5
)
Code language: JavaScript (javascript)
In this example, the divide
function performs division but returns an Option
type to handle the case of division by zero. The >>=
operator, called the bind operator, is used to sequence the computations within the Option
monad.
The result is a computation that performs the division by 2
and then divides the result by 5
. If any division operation returns None
, the subsequent computations are short-circuited, and the final result is None
.
Monads enable the encapsulation of complex computation flows, error handling, and the management of stateful computations while maintaining the purity and composability of functional programming.
Question:
Explain the concept of lazy evaluation in OCaml. What is lazy evaluation, and how does it enable efficient and on-demand computation? Provide an example demonstrating the usage of lazy evaluation.
Answer:
Lazy evaluation in OCaml is a evaluation strategy where expressions are not evaluated immediately but instead deferred until their values are needed. It enables efficient computation by avoiding unnecessary computations and evaluating only what is necessary.
Lazy evaluation works by wrapping an expression in a lazy container, delaying its evaluation until its value is explicitly requested.
Here’s an example demonstrating the usage of lazy evaluation:
let expensive_computation () =
print_endline "Performing expensive computation";
42
let result =
let lazy_value = lazy (expensive_computation ()) in
if Random.bool () then Lazy.force lazy_value else 0
Code language: JavaScript (javascript)
In this example, the expensive_computation
function represents a computationally expensive operation. The result of this computation is wrapped in a lazy container using the lazy
keyword.
The lazy_value
is then conditionally evaluated using Lazy.force
. If a random boolean value is true
, the value is forced, and the expensive computation is performed. Otherwise, if the boolean value is false
, the computation is not performed, and the result is 0
.
Lazy evaluation allows for more efficient computation by deferring expensive computations until their values are actually needed. It can significantly improve performance in situations where not all computations are required.
Question:
Explain the concept of higher-kinded types (HKT) in OCaml. What are higher-kinded types, and how do they enable abstraction over type constructors? Provide an example demonstrating the usage of higher-kinded types.
Answer:
Higher-kinded types (HKT) in OCaml are types that abstract over type constructors, allowing generic programming over different kinds of types.
OCaml’s type system natively supports higher-kinded types through the use of polymorphic variant types and modules. HKT enables the creation of generic type definitions and functions that can operate on a wide range of type constructors.
Here’s an example demonstrating the usage of higher-kinded types:
module type Functor = sig
type 'a f
val map : ('a -> 'b) -> 'a f -> 'b f
end
module ListFunctor : Functor = struct
type 'a f = 'a list
let map f lst = List.map f lst
end
let increment = (+) 1
let result = ListFunctor.map increment [1; 2; 3]
Code language: PHP (php)
In this example, a Functor
module type is defined, representing a higher-kinded type. It includes an abstract type 'a f
that represents a type constructor and a map
function that operates on the type constructor.
The ListFunctor
module implements the Functor
module type for lists. The map
function applies a given function f
to each element of the list.
The increment
function is passed as an argument to the map
function, resulting in the incrementation of each element in the list.
Higher-kinded types enable the creation of generic abstractions that operate on various type constructors, providing flexibility and code reuse.
Question:
Explain the concept of first-class modules in OCaml. What are first-class modules, and how do they enable dynamic code composition and abstraction? Provide an example demonstrating the usage of first-class modules.
Answer:
First-class modules in OCaml allow modules to be treated as first-class values, enabling dynamic code composition and abstraction. They provide a powerful mechanism for modular programming and code reuse.
First-class modules allow modules to be created, passed as arguments, returned as results, and stored in data structures, similar to functions or values.
Here’s an example demonstrating the usage of first-class modules:
module type MathOperations = sig
val add : int -> int -> int
val subtract : int -> int -> int
end
let create_math_module () : (module MathOperations) =
let module M : MathOperations = struct
let add x y = x + y
let subtract x y = x - y
end
in
(module M : MathOperations)
let math_module = create_math_module ()
let result = let module M = (val math_module
: MathOperations) in M.add 3 4
Code language: JavaScript (javascript)
In this example, the MathOperations
module type defines a set of mathematical operations. The create_math_module
function creates a dynamic instance of a module that implements the MathOperations
interface.
The math_module
variable stores the dynamically created module, and the result
variable uses the module to perform an addition operation.
First-class modules enable dynamic code composition and abstraction. They provide a flexible way to create and manipulate modules at runtime, promoting modularity, code reuse, and dynamic system construction.
1,000 Companies use CoderPad to Screen and Interview Developers
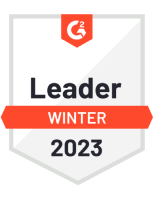
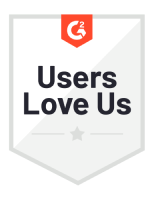
Interview best practices for OCaml roles
For efficient OCaml interviews, it’s crucial to take into account various aspects, such as the applicants’ experience levels and the specific engineering position they’re interviewing for. To ensure your OCaml interview questions yield optimal outcomes, we suggest adhering to these best practices when interacting with candidates:
- Create technical queries reflecting real-world situations in your organization. This method maintains the candidate’s engagement and allows for a more accurate assessment of their suitability for your team.
- Foster a collaborative environment by permitting candidates to pose questions throughout the interview.
- Assessing familiarity with common OCaml libraries such as Core, Lwt, and Async, will also be beneficial in evaluating a candidate’s OCaml skills.
Additionally, it’s vital to follow standard interview procedures when conducting OCaml interviews. This encompasses tailoring question difficulty based on the applicant’s expertise, offering prompt feedback on their application status, and allowing candidates to inquire about the evaluation or collaborate with you and your team.