Objective-C Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Objective-C developers for your organization.
Objective-C
Despite the rise of Swift as the preferred language for macOS/iOS applications, Objective-C still remains popular due to its interoperability with C and C++, its dynamic runtime, and the mature ecosystem.
Objective-C is the language behind the Cocoa and Cocoa Touch frameworks, which are the primary frameworks for building macOS and iOS applications, respectively. These frameworks provide a rich set of APIs that enable developers to create complex applications with ease.
Apple’s Developer Documentation
Our group has designed functional programming tasks and discussion inquiries, customized to gauge the Objective-C abilities of developers throughout coding evaluations. Moreover, we have gathered a collection of optimal techniques to guarantee the precision of your interview questions in assessing candidates’ expertise in Objective-C.
Table of Contents
Objective-C example question
Help us design a parking lot
Hey candidate! Welcome to your interview. Boilerplate is provided. Feel free to change the code as you see fit. To run the code at any time, please hit the run button located in the top left corner.
Goals: Design a parking lot using object-oriented principles
Here are a few methods that you should be able to run:
- Tell us how many spots are remaining
- Tell us how many total spots are in the parking lot
- Tell us when the parking lot is full
- Tell us when the parking lot is empty
- Tell us when certain spots are full e.g. when all motorcycle spots are taken
- Tell us how many spots vans are taking up
Assumptions:
- The parking lot can hold motorcycles, cars and vans
- The parking lot has motorcycle spots, car spots and large spots
- A motorcycle can park in any spot
- A car can park in a single compact spot, or a regular spot
- A van can park, but it will take up 3 regular spots
- These are just a few assumptions. Feel free to ask your interviewer about more assumptions as needed
Objective-C skills to assess
Jobs using Objective-C
Junior Objective-C interview questions
Question:
Explain the concept of properties in Objective-C. What are the advantages of using properties over instance variables?
Answer:
In Objective-C, properties provide a convenient way to encapsulate the state of an object and define the getter and setter methods to access and modify that state. They are declared using the @property
syntax and can have attributes like nonatomic
, strong
, weak
, etc.
Advantages of using properties over instance variables include:
- Encapsulation: Properties allow for data encapsulation by providing controlled access to an object’s state.
- Automatic Getter and Setter Methods: Properties automatically generate getter and setter methods, reducing boilerplate code and improving code readability.
- Memory Management: Properties with appropriate memory management attributes (e.g.,
strong
,weak
,copy
) handle memory management automatically, simplifying memory management tasks. - Key-Value Observing (KVO): Properties can be observed using KVO, allowing other objects to be notified of changes to the property’s value.
- Dot Notation Syntax: Properties can be accessed using the dot notation syntax, making the code more concise and readable.
Question:
What is the difference between retain
, strong
, weak
, and copy
in Objective-C memory management? When would you use each one?
Answer:
retain
andstrong
both indicate that the object should be retained and keep a strong reference to it. The difference lies in the context:retain
is used in manual reference counting (MRC) for managing object ownership manually.strong
is used in automatic reference counting (ARC) to indicate a strong reference without manually managing retain counts. It is the default attribute in ARC and is commonly used for instance variables and properties.weak
indicates a weak reference that does not keep the object alive. It is typically used to break retain cycles and avoid strong reference cycles between objects.copy
creates a copy of an object when assigning it to the property. It is useful when you want to ensure that the object’s value remains unchanged.
The choice between retain
/strong
and weak
depends on the ownership and lifetime requirements of the object. copy
is used when you want to ensure the immutability of the object.
Question:
Explain the concept of protocols in Objective-C and their usage. Provide an example of creating a protocol and implementing it in a class.
Answer:
Protocols in Objective-C define a set of methods that a class can implement. They provide a way to define a contract or interface that multiple classes can conform to, enabling polymorphism and code reuse.
Here’s an example of creating a protocol and implementing it in a class:
@protocol Printable
- (void)print;
@end
@interface MyClass : NSObject <Printable>
@end
@implementation MyClass
- (void)print {
NSLog(@"Printing...");
}
@end
Code language: CSS (css)
In this example, the Printable
protocol is defined using the @protocol
directive. It declares a single method, print
. The MyClass
class adopts the Printable
protocol using the <Printable>
syntax. It must implement the required method print
defined in the protocol.
Protocols allow for polymorphism, where multiple classes can conform to the same protocol, enabling them to be used interchangeably based on the protocol contract.
Question:
What are blocks in Objective-C? How do they differ from regular methods or functions? Provide an example demonstrating the usage of blocks.
Answer:
Blocks in Objective-C are self-contained, anonymous segments of code that can be passed around and executed later. They are similar to closures in other programming languages. Blocks encapsulate both the code and the environment in which they are created, capturing variables and constants from the surrounding scope.
Blocks differ from regular methods or functions in that they can be used as first-class objects, allowing them to be stored in variables, passed as arguments, and returned from methods. They provide a way to create and pass behavior as data.
Here’s an example demonstrating the usage of blocks:
typedef void (^CompletionBlock)(BOOL success);
- (void)performTaskWithCompletion:(CompletionBlock)completion {
// Perform some task
BOOL success = YES;
// Invoke the completion block with the result
completion(success);
}
// Usage
[self performTaskWithCompletion:^(BOOL success) {
if (success) {
NSLog(@"Task completed successfully");
} else {
NSLog(@"Task failed");
}
}];
Code language: JavaScript (javascript)
In this example, a block type CompletionBlock
is defined using the typedef
keyword. The performTaskWithCompletion:
method takes a block as a parameter and executes it after performing a task. The block takes a BOOL
parameter indicating the success status of the task.
Blocks are commonly used for asynchronous operations, callback mechanisms, and implementing higher-order functions.
Question:
What is the purpose of using the @synthesize
directive in Objective-C? When is it needed and when is it not required?
Answer:
The @synthesize
directive in Objective-C is used to automatically generate the getter and setter methods for properties. Prior to Objective-C 2.0, properties had to be explicitly synthesized in the implementation file using @synthesize propertyName = _propertyName;
. However, since Objective-C 2.0, the @synthesize
directive is no longer required in most cases.
By default, when a property is declared, the compiler automatically synthesizes the appropriate accessor methods, as well as an instance variable with the same name as the property preceded by an underscore (_
).
However, there are cases when explicit @synthesize
is still useful:
- When you want to use a different name for the instance variable backing the property, you can explicitly synthesize it using
@synthesize propertyName = _customName;
. - When you want to provide custom implementations of getter or setter methods, you can explicitly synthesize one method and implement the other manually.
In most cases, with modern Objective-C and the use of automatic synthesis, the @synthesize
directive is not required and can be omitted.
Question:
Explain the concept of exceptions in Objective-C. How are exceptions different from error handling using NSError
?
Answer:
Exceptions in Objective-C provide a mechanism to handle exceptional conditions and unexpected runtime errors. They are thrown using the @throw
statement and can be caught and handled using @try
, @catch
, and @finally
blocks.
Exceptions differ from error handling using NSError
in the following ways:
- Exceptions are used for exceptional conditions and unexpected runtime errors, while
NSError
is typically used for expected errors or recoverable conditions. - Exceptions are thrown and caught using
@throw
,@try
,@catch
, and@finally
, similar to exception handling in other programming languages.NSError
is typically propagated through return values or passed as an argument to indicate errors. - Exceptions can be caught at different levels of the call stack, allowing for centralized exception handling.
NSError
is usually handled at the caller level, providing explicit error handling and recovery options.
It is important to note that exceptions in Objective-C should be used sparingly and primarily for exceptional and unrecoverable errors, such as programming errors or invalid conditions. For expected errors and recoverable conditions, error handling using NSError
is the preferred approach.
Question:
What are the differences between NSMutableArray
and NSArray
in Objective-C? When would you use one over the other?
Answer:
NSArray
is an immutable array in Objective-C, meaning its contents cannot be modified after it is created. Once initialized, you cannot add, remove, or modify objects in anNSArray
.NSMutableArray
, on the other hand, is a mutable array that allows adding, removing, and modifying objects dynamically.
You would use NSArray
when you have a collection of objects that does not need to be modified. It provides better performance and memory efficiency since it does not need to support mutation operations.
NSMutableArray
is used when you need to dynamically modify the array’s contents. It provides methods to add, remove, and replace objects at specific indexes.
In general, it is good practice to use NSArray
whenever possible and switch to NSMutableArray
only when mutability is required to ensure immutability by default and reduce unintended side effects.
Question:
Explain the concept of key-value coding (KVC) in Objective-C. How does it simplify accessing and manipulating object properties?
Answer:
Key-Value Coding (KVC) is a mechanism in Objective-C that allows accessing and manipulating an object’s properties using keys as strings. Instead of directly invoking the getter and setter methods for properties, KVC provides a unified interface to read and write property values dynamically.
KVC simplifies accessing and manipulating object properties by providing the following benefits:
- Dynamic Access: KVC allows reading and writing properties without knowing their specific names at compile time. This enables generic code and reduces coupling between classes.
- Collection Operators: KVC supports collection operators, such as
@sum
,@avg
,@max
,@min
, and@distinctUnionOfObjects
, which simplify operations on collections of objects. - Key-Value Observing (KVO): KVC is the foundation for KVO, allowing objects to observe changes to specific properties of other objects.
- Reflection and Introspection: KVC enables reflection and introspection, making it possible to inspect an object’s properties dynamically.
To use KVC, you can use the methods provided by NSObject
such as valueForKey:
and setValue:forKey:
. Additionally, KVC-compliant properties can be accessed using dot notation or subscripting.
Question:
What is the role of the @autoreleasepool
block in Objective-C memory management? When should you use it?
Answer:
The @autoreleasepool
block in Objective-C is used to manage the autorelease pool, which is responsible for managing autoreleased objects. Autoreleased objects are those whose memory will be automatically released at the end of the current run loop iteration.
The @autoreleasepool
block creates a local autorelease pool, allowing you to control the lifetime of autoreleased objects within the block. When the block is exited, the autorelease pool releases the autoreleased objects, freeing up memory.
You should use the @autoreleasepool
block in situations where you are creating a large number of temporary objects, such as within a loop or a time-consuming operation. By creating a local autorelease pool, you can ensure that the memory used by the autoreleased objects is released more frequently, reducing peak memory usage.
It is important to note that with the introduction of Automatic Reference Counting (ARC), the need for manually managing autorelease pools has been significantly reduced. In most cases, ARC handles autorelease pools automatically, and explicit use of @autoreleasepool
blocks is not necessary. However, in specific scenarios where you have explicit control over memory management, such as in non-ARC code or performance-critical sections, using @autoreleasepool
blocks can still be beneficial.
Question:
Explain the difference between using dot notation and square bracket syntax for accessing object properties and invoking methods in Objective-C.
Answer:
In Objective-C, dot notation and square bracket syntax are two ways of accessing object properties and invoking methods, but they have different meanings and use cases.
Dot Notation:
- Dot notation is a shorthand syntax introduced in Objective-C 2.0 that allows direct access to properties using the dot (
.
) operator. - It provides a more concise and readable way to get or set property values, making the code more expressive.
- Dot notation is limited to properties defined with getter and setter methods.
- It is a compile-time feature and does not have any runtime implications.
Example:
NSString *name = person.name;
person.age = 25;
Square Bracket Syntax:
- Square bracket syntax is the traditional way of invoking methods and accessing properties in Objective-C.
- It is used to send messages to objects and allows calling any method, including those without getter or setter semantics.
- It is more flexible and can be used in various situations, such as passing multiple arguments, calling methods with selectors, or accessing elements from arrays.
- Square bracket syntax has runtime implications as the message dispatch happens at runtime.
Example:
NSString *name = [person name];
[person setName:@"John"];
Code language: JavaScript (javascript)
In general, dot notation is preferred for accessing properties when available since it enhances code readability. Square bracket syntax is used for general method invocation, passing arguments, or when interacting with objects dynamically at runtime.
Intermediate Objective-C interview questions
Question:
Explain the concept of categories in Objective-C and their usage. Provide an example demonstrating the usage of categories.
Answer:
Categories in Objective-C allow developers to extend existing classes by adding methods without modifying their original implementation. They provide a way to organize and modularize code by grouping related methods into separate files.
Here’s an example of creating a category and implementing it in a class:
// NSString+Utilities.h
@interface NSString (Utilities)
- (NSString *)reversedString;
- (NSString *)stringByRemovingWhitespace;
@end
// NSString+Utilities.m
@implementation NSString (Utilities)
- (NSString *)reversedString {
NSMutableString *result = [NSMutableString stringWithCapacity:self.length];
for (NSInteger i = self.length - 1; i >= 0; i--) {
[result appendFormat:@"%C", [self characterAtIndex:i]];
}
return result;
}
- (NSString *)stringByRemovingWhitespace {
return [self stringByReplacingOccurrencesOfString:@" " withString:@""];
}
@end
// Usage
NSString *originalString = @"Hello, World!";
NSString *reversedString = [originalString reversedString];
NSLog(@"Reversed string: %@", reversedString); // Output: !dlroW ,olleH
NSString *stringWithWhitespace = @"Hello, World!";
NSString *stringWithoutWhitespace = [stringWithWhitespace stringByRemovingWhitespace];
NSLog(@"String without whitespace: %@", stringWithoutWhitespace); // Output: HelloWorld!
Code language: JavaScript (javascript)
In this example, a category named Utilities
is created for the NSString
class. The category adds two utility methods, reversedString
and stringByRemovingWhitespace
, which can be called on any NSString
instance. The category is implemented in separate header and implementation files.
Categories are useful for adding utility methods, extending existing classes from frameworks, or organizing related functionality into separate files.
Question:
Explain the concept of Key-Value Observing (KVO) in Objective-C and its usage. Provide an example demonstrating the usage of KVO.
Answer:
Key-Value Observing (KVO) is a mechanism in Objective-C that allows objects to observe changes to specific properties of other objects. It provides a way to receive notifications when the observed properties change, enabling loosely coupled communication between objects.
Here’s an example demonstrating the usage of KVO:
// Observer.h
@interface Observer : NSObject
@end
// Observer.m
@implementation Observer
- (instancetype)init {
self = [super init];
if (self) {
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(propertyDidChange:)
name:@"PersonNameDidChangeNotification"
object:nil];
}
return self;
}
- (void)propertyDidChange:(NSNotification *)notification {
if ([notification.name isEqualToString:@"PersonNameDidChangeNotification"]) {
NSString *newName = notification.userInfo[@"newName"];
NSLog(@"Person's name changed: %@", newName);
}
}
- (void)dealloc {
[[NSNotificationCenter defaultCenter] removeObserver:self];
}
@end
// Person.h
@interface Person : NSObject
@property (nonatomic, copy) NSString *name;
@end
// Person.m
@implementation Person
- (void)setName:(NSString *)name {
_name = name;
[[NSNotificationCenter defaultCenter] postNotificationName:@"PersonNameDidChangeNotification"
object:self
userInfo:@{@"newName" : name}];
}
@end
// Usage
Person *person = [[Person alloc] init];
Observer *observer = [[Observer alloc] init];
person.name = @"John"; // Output: Person's name changed: John
Code language: JavaScript (javascript)
In this example, the Observer
class observes changes to the name
property of the Person
class using NSNotificationCenter
. When the name
property is set, a notification is posted with the updated value. The Observer
registers itself as an observer for the PersonNameDidChangeNotification
and responds to changes by invoking the propertyDidChange:
method.
KVO provides a way to establish relationships between objects and receive notifications about property changes, enabling decoupled communication and dynamic behavior.
Question:
What are the differences between assign
, weak
, and unsafe_unretained
in Objective-C property attributes? When would you use each one?
Answer:
In Objective-C, the assign
, weak
, and unsafe_unretained
property attributes are used to indicate the type of reference to be used for a particular property.
assign
: Theassign
attribute is used for primitive types (e.g.,int
,float
) and weakly-typed objects. It assigns the value directly without retaining or releasing it. This attribute is used in Manual Reference Counting (MRC) and is the default behavior for object properties.weak
: Theweak
attribute is used for object references and creates a weak reference to the object. It does not retain or release the object, and if the object is deallocated, the reference is automatically set tonil
. This attribute is used in Automatic Reference Counting (ARC) to avoid strong reference cycles.unsafe_unretained
: Theunsafe_unretained
attribute is similar toweak
, but it does not automatically set the reference tonil
when the object is deallocated. If the referenced object is deallocated, the reference becomes a dangling pointer. This attribute is used in MRC or when dealing with legacy code.
When to use each attribute:
- Use
assign
for primitive types or weakly-typed objects that do not require memory management. - Use
weak
for object references to avoid strong reference cycles or retain cycles. - Use
unsafe_unretained
only when necessary, such as in MRC or when dealing with legacy code where automatic setting of the reference tonil
is not required.
In general, it is recommended to use weak
instead of unsafe_unretained
whenever possible, as it provides additional safety by automatically setting the reference to nil
when the object is deallocated.
Question:
Explain the concept of singletons in Objective-C. How do you implement a singleton class? What are the potential issues with singletons?
Answer:
Singletons in Objective-C are classes that can have only one instance throughout the application’s lifecycle. They provide a globally accessible point of access to a shared instance, allowing data or functionality to be accessed consistently across multiple parts of the application.
Here’s an example of implementing a singleton class in Objective-C:
// MySingleton.h
@interface MySingleton : NSObject
+ (instancetype)sharedInstance;
@end
// MySingleton.m
@implementation MySingleton
+ (instancetype)sharedInstance {
static MySingleton *sharedInstance = nil;
static dispatch_once_t onceToken;
dispatch_once(&onceToken, ^{
sharedInstance = [[self alloc] init];
});
return sharedInstance;
}
@end
// Usage
MySingleton *singleton = [MySingleton sharedInstance];
Code language: JavaScript (javascript)
In this example, the sharedInstance
method is used to access the singleton instance. It uses the Grand Central Dispatch (GCD) function dispatch_once
to ensure that the initialization of the shared instance occurs only once, even in a multithreaded environment.
Potential issues with singletons include:
- Global State: Singletons introduce global state, which can make it harder to reason about the flow of data and can lead to dependencies between different parts of the codebase.
- Dependency Injection: Singleton classes often rely on a global point of access, making it difficult to inject dependencies or swap implementations during testing.
- Testing: Singletons can be challenging to test due to their global nature and shared state. Dependencies on the singleton instance can make unit testing more difficult.
- Tight Coupling: The use of singletons can lead to tight coupling between different parts of the application, making code maintenance and refactoring more challenging.
While singletons can be convenient in certain situations, it’s important to consider the potential drawbacks and carefully evaluate whether their use is appropriate in a given context. Alternatives such as dependency injection or using instance-based objects may be more flexible and maintainable in many cases.
Question:
Explain the concept of delegates in Objective-C. How do delegates enable communication between objects? Provide an example demonstrating the usage of delegates.
Answer:
Delegates in Objective-C enable communication and interaction between objects. They allow one object to delegate certain responsibilities or actions to another object, providing a way to decouple and customize behavior.
Here’s an example demonstrating the usage of delegates:
// MyProtocol.h
@protocol MyProtocol <NSObject>
- (void)didFinishTask;
@end
// MyObject.h
@interface MyObject : NSObject
@property (nonatomic, weak) id<MyProtocol> delegate;
- (void)performTask;
@end
// MyObject.m
@implementation MyObject
- (void)performTask {
// Perform the task
NSLog(@"Task performed");
// Notify the delegate
if ([self.delegate respondsToSelector:@selector(didFinishTask)]) {
[self.delegate didFinishTask];
}
}
@end
// ViewController.h
#import "MyProtocol.h"
@interface ViewController : UIViewController <MyProtocol>
@end
// ViewController.m
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
MyObject *object = [[MyObject alloc] init];
object.delegate = self;
[object performTask];
}
- (void)didFinishTask {
NSLog(@"Task finished delegate method called");
}
@end
// Usage
ViewController *viewController = [[ViewController alloc] init];
// Output: Task performed
// Output: Task finished delegate method called
Code language: HTML, XML (xml)
In this example, the MyProtocol
protocol defines a method didFinishTask
. The MyObject
class has a delegate property conforming to MyProtocol
. When performTask
is called on MyObject
, it performs the task and notifies the delegate by invoking the didFinishTask
method if it responds to it.
The ViewController
class adopts the MyProtocol
protocol and becomes the delegate of MyObject
. It implements the didFinishTask
method to handle the task completion.
Delegates enable objects to communicate and provide a way to customize behavior without tightly coupling the objects together. They are commonly used in frameworks, UI controls, and asynchronous operations.
Question:
Explain the concept of blocks in Objective-C. How do blocks enable capturing and using variables from the surrounding scope? Provide an example demonstrating the usage of blocks.
Answer:
Blocks in Objective-C are self-contained, anonymous segments of code that can be passed around and executed later. They are similar to closures in other programming languages. Blocks encapsulate both the code and the environment in which they are created, capturing variables and constants from the surrounding scope.
Here’s an example demonstrating the usage of blocks:
typedef void (^CompletionBlock)(BOOL success);
- (void)performTaskWithCompletion:(CompletionBlock)completion {
// Perform some task
BOOL success = YES;
// Invoke the completion block with the result
completion(success);
}
// Usage
[self performTaskWithCompletion:^(BOOL success) {
if (success) {
NSLog(@"Task completed successfully");
} else {
NSLog(@"Task failed");
}
}];
Code language: JavaScript (javascript)
In this example, a block type CompletionBlock
is defined using the typedef
keyword. The performTaskWithCompletion:
method takes a block as a parameter and executes it after performing a task. The block takes a BOOL
parameter indicating the success status of the task.
Blocks are commonly used for asynchronous operations, callback mechanisms, and implementing higher-order functions. They enable capturing variables from the surrounding scope, allowing the block to access and modify them even after the surrounding scope has exited.
Blocks can capture variables from the surrounding scope by automatically creating a copy of them. The captured variables retain their values even if they go out of scope, providing a powerful way to encapsulate behavior and data together.
Question:
Explain the concept of NSNotificationCenter in Objective-C. How does it enable communication between objects? Provide an example demonstrating the usage of NSNotificationCenter.
Answer:NSNotificationCenter
in Objective-C is a publish-subscribe mechanism that enables communication between objects using a notification-based system. It allows objects to post notifications and other objects to observe and respond to those notifications.
Here’s an example demonstrating the usage of NSNotificationCenter
:
// MyObject.h
@interface MyObject : NSObject
@end
// MyObject.m
@implementation MyObject
- (void)doSomething {
// Do something
// Post a notification
[[NSNotificationCenter defaultCenter] postNotificationName:@"MyNotification"
object:nil
userInfo:@{@"key" : @"value"}];
}
@end
// MyObserver.h
@interface MyObserver : NSObject
@end
// MyObserver.m
@implementation MyObserver
- (instancetype)init {
self = [super init];
if (self) {
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(handleNotification:)
name:@"MyNotification"
object:nil];
}
return self;
}
- (void)handleNotification:(NSNotification *)notification {
if ([notification.name isEqualToString:@"MyNotification"]) {
NSDictionary *userInfo = notification.userInfo;
NSString *value = userInfo[@"key"];
NSLog(@"Received notification with value: %@", value);
}
}
- (void)dealloc {
[[NSNotificationCenter defaultCenter] removeObserver:self];
}
@end
// Usage
MyObserver *observer = [[MyObserver alloc] init];
MyObject *object = [[MyObject alloc] init];
[object doSomething]; // Output: Received notification with value: value
Code language: JavaScript (javascript)
In this example, the MyObject
class posts a notification using NSNotificationCenter
when doSomething
is called. The MyObserver
class registers itself as an observer for the "MyNotification"
and responds to the notification by invoking the handleNotification:
method.
NSNotificationCenter enables decoupled communication between objects by allowing them to broadcast and receive notifications. Multiple objects can observe the same notification, and notifications can carry additional data through the userInfo
dictionary.
Question:
Explain the concept of property attributes in Objective-C. What are some common property attributes, and what do they indicate?
Answer:
Property attributes in Objective-C provide additional information about properties, allowing developers to specify behavior and characteristics. They are placed inside parentheses before the property declaration.
Common property attributes include:
readonly
: Indicates that the property can be read but not written to. The compiler generates a getter method but no setter method.readwrite
: Indicates that the property can be both read and written to. This is the default attribute if none is specified.nonatomic
: Specifies that the property is not thread-safe and can be accessed from multiple threads simultaneously without synchronization. It improves performance but can lead to data corruption in a multithreaded environment.atomic
: Specifies that the property is thread-safe and ensures that access is synchronized. It provides data integrity in a multithreaded environment but can impact performance.weak
: Specifies a weak reference to the property. The reference is automatically set tonil
when the referenced object is deallocated. It helps to avoid strong reference cycles.strong
/retain
: Specifies a strong reference to the property, retaining the referenced object. The reference count of the object is increased.copy
: Specifies that the property should make a copy of the assigned value when set. It is commonly used for NSString and block properties to ensure immutability and avoid mutability issues.getter=
/setter=
: Allows customizing the getter and setter method names for the property.
These attributes provide control over memory management, thread-safety, and customization of property behavior. They allow developers to fine-tune the behavior of properties based on specific requirements.
Question:
What is the purpose of using the @autoreleasepool
block in Objective-C memory management? When should you use it?
Answer:
The @autoreleasepool
block in Objective-C is used to manage the autorelease pool, which is responsible for managing autoreleased objects. Autoreleased objects are those whose memory will be automatically released at the end of the current run loop iteration.
The @autoreleasepool
block creates a local autorelease pool, allowing you to control the lifetime of autoreleased objects within the block. When the block is exited, the autorelease pool releases the autoreleased objects, freeing up memory.
You should use the @autoreleasepool
block in situations where you are creating a large number of temporary objects, such as within a loop or a time-consuming operation. By creating a local autorelease pool, you can ensure that the memory used by the autoreleased objects is released more frequently, reducing peak memory usage.
It is important to note that with the introduction of Automatic Reference Counting (ARC), the need for manually managing autorelease pools has been significantly reduced. In most cases, ARC handles autorelease pools automatically, and explicit use of @autoreleasepool
blocks is not necessary. However, in specific scenarios where you have explicit control over memory management, such as in non-ARC code or performance-critical sections, using @autoreleasepool
blocks can still be beneficial.
Question:
Explain the concept of method swizzling in Objective-C. How does it work, and what are the potential use cases?
Answer:
Method swizzling in Objective-C is a technique that allows developers to exchange the implementations of methods at runtime. It involves swapping the implementations of two methods, typically belonging to the same class or different classes.
Method swizzling works by manipulating the Objective-C runtime and is primarily accomplished using two functions: class_getInstanceMethod
and method_exchangeImplementations
. These functions retrieve the method implementations and then swap them.
Potential use cases of method swizzling include:
- Adding Functionality: Method swizzling can be used to add additional behavior to existing methods without modifying the original implementation. This can be useful for logging, profiling, or debugging purposes.
- Method Overriding: Method swizzling can be used to override existing methods, providing an alternative implementation to the original method. This can be used to customize behavior or fix bugs in third-party libraries or system frameworks.
- A/B Testing: Method swizzling can be used to dynamically switch between different implementations of a method, allowing for A/B testing or feature toggling without modifying the codebase.
- Fixing Bugs: Method swizzling can be used to fix bugs or modify behavior in system classes or frameworks that are not under your control.
It’s important to use method swizzling judiciously and with caution. It can introduce complexity, make code harder to understand and debug, and potentially lead to unexpected behavior or conflicts with other swizzled methods. Care should be taken to ensure that swizzled methods are properly documented, tested, and comply with Apple’s guidelines.
These answers provide an overview of the concepts and explanations for each question. For further understanding, it is recommended to refer to the Objective-C documentation and additional resources.
Senior Objective-C interview questions
Question:
Explain the concept of blocks in Objective-C. How do blocks enable capturing and using variables from the surrounding scope? Provide an example demonstrating the usage of blocks.
Answer:
Blocks in Objective-C are self-contained, anonymous segments of code that can be passed around and executed later. They are similar to closures in other programming languages. Blocks encapsulate both the code and the environment in which they are created, capturing variables and constants from the surrounding scope.
Here’s an example demonstrating the usage of blocks:
typedef void (^CompletionBlock)(BOOL success);
- (void)performTaskWithCompletion:(CompletionBlock)completion {
// Perform some task
BOOL success = YES;
// Invoke the completion block with the result
completion(success);
}
// Usage
[self performTaskWithCompletion:^(BOOL success) {
if (success) {
NSLog(@"Task completed successfully");
} else {
NSLog(@"Task failed");
}
}];
Code language: JavaScript (javascript)
In this example, a block type CompletionBlock
is defined using the typedef
keyword. The performTaskWithCompletion:
method takes a block as a parameter and executes it after performing a task. The block takes a BOOL
parameter indicating the success status of the task.
Blocks are commonly used for asynchronous operations, callback mechanisms, and implementing higher-order functions. They enable capturing variables from the surrounding scope, allowing the block to access and modify them even after the surrounding scope has exited.
Question:
Explain the concept of method swizzling in Objective-C. How does it work, and what are the potential use cases?
Answer:
Method swizzling in Objective-C is a technique that allows developers to exchange the implementations of methods at runtime. It involves swapping the implementations of two methods, typically belonging to the same class or different classes.
Method swizzling works by manipulating the Objective-C runtime and is primarily accomplished using two functions: class_getInstanceMethod
and method_exchangeImplementations
. These functions retrieve the method implementations and then swap them.
Potential use cases of method swizzling include:
- Adding Functionality: Method swizzling can be used to add additional behavior to existing methods without modifying the original implementation. This can be useful for logging, profiling, or debugging purposes.
- Method Overriding: Method swizzling can be used to override existing methods, providing an alternative implementation to the original method. This can be used to customize behavior or fix bugs in third-party libraries or system frameworks.
- A/B Testing: Method swizzling can be used to dynamically switch between different implementations of a method, allowing for A/B testing or feature toggling without modifying the codebase.
- Fixing Bugs: Method swizzling can be used to fix bugs or modify behavior in system classes or frameworks that are not under your control.
It’s important to use method swizzling judiciously and with caution. It can introduce complexity, make code harder to understand and debug, and potentially lead to unexpected behavior or conflicts with other swizzled methods. Care should be taken to ensure that swizzled methods are properly documented, tested, and comply with Apple’s guidelines.
Question:
Explain the concept of Core Data in iOS development. What is Core Data, and how does it enable data persistence? Provide an example demonstrating the usage of Core Data.
Answer:
Core Data is a framework provided by Apple that enables developers to manage the model layer objects and their persistence in iOS and macOS applications. It provides an object-oriented interface for working with a data model and supports various storage options, including SQLite, XML, and in-memory stores.
Core Data enables data persistence by providing an abstraction layer between the data model and the underlying storage. It manages the loading, saving, querying, and undo/redo of data objects, allowing developers to focus on working with objects rather than dealing with low-level data storage operations.
Here’s an example demonstrating the usage of Core Data:
// Person.h
@interface Person : NSManagedObject
@property (nonatomic, strong) NSString *name;
@property (nonatomic, strong) NSNumber *age;
@end
// Person.m
@implementation Person
@dynamic name;
@dynamic age;
@end
// Usage
NSManagedObjectContext *context = ... // Get the managed object context
Person *person = [NSEntityDescription insertNewObjectForEntityForName:@"Person"
inManagedObjectContext:context];
person.name = @"John";
person.age = @(25);
NSError *error;
if (![context save:&error]) {
NSLog(@"Failed to save person: %@", error);
} else {
NSLog(@"Person saved successfully");
}
Code language: JavaScript (javascript)
In this example, a Core Data entity named “Person” is defined using the NSManagedObject
subclass. The entity has two properties, “name” and “age”. To create and save a person object, an instance of the managed object context is obtained, and a new person object is inserted into the context. The properties of the person object are set, and the context is saved to persist the changes.
Core Data provides powerful features such as data modeling, relationships, versioning, and faulting, making it a preferred choice for managing data persistence in iOS applications.
Question:
Explain the concept of GCD (Grand Central Dispatch) in iOS development. What is GCD, and how does it enable concurrent programming? Provide an example demonstrating the usage of GCD.
Answer:
GCD (Grand Central Dispatch) is a technology provided by Apple for performing concurrent and asynchronous tasks in iOS and macOS applications. It is a low-level API for managing work across multiple threads or queues.
GCD enables concurrent programming by providing a simple and efficient way to execute tasks concurrently. It abstracts away the complexities of thread management and provides a high-level interface for dispatching work to different queues.
Here’s an example demonstrating the usage of GCD:
dispatch_queue_t concurrentQueue = dispatch_queue_create("com.example.concurrent", DISPATCH_QUEUE_CONCURRENT);
dispatch_async(concurrentQueue, ^{
NSLog(@"Task 1 executed");
});
dispatch_async(concurrentQueue, ^{
NSLog(@"Task 2 executed");
});
dispatch_async(concurrentQueue, ^{
NSLog(@"Task 3 executed");
});
dispatch_barrier_async(concurrentQueue, ^{
NSLog(@"Barrier task executed");
});
dispatch_async(concurrentQueue, ^{
NSLog(@"Task 4 executed");
});
dispatch_async(concurrentQueue, ^{
NSLog(@"Task 5 executed");
});
Code language: JavaScript (javascript)
In this example, a concurrent queue named “com.example.concurrent” is created using dispatch_queue_create
. Multiple tasks are dispatched asynchronously to the concurrent queue using dispatch_async
. These tasks can execute concurrently and may complete in any order.
The dispatch_barrier_async
function is used to schedule a barrier task, which ensures that all previously enqueued tasks finish executing before the barrier task starts. After the barrier task, more tasks are dispatched asynchronously.
GCD provides a powerful and efficient way to manage concurrent execution, handle synchronization, and improve the performance of iOS applications.
Question:
Explain the concept of memory management in Objective-C. What are the memory management mechanisms in Objective-C, and how do they work?
Answer:
Memory management in Objective-C involves managing the allocation and deallocation of objects to ensure efficient memory usage and prevent memory leaks.
Objective-C has two primary memory management mechanisms:
- Manual Reference Counting (MRC): In MRC, developers explicitly manage the reference counts of objects by calling
retain
,release
, andautorelease
methods. Each time an object is retained, its reference count increases, and when released, its reference count decreases. When the reference count reaches zero, the object is deallocated. MRC requires careful memory management to avoid retain cycles and dangling pointers. - Automatic Reference Counting (ARC): ARC automates the memory management process by automatically inserting
retain
,release
, andautorelease
calls at compile time. It keeps track of the reference counts of objects and inserts memory management code accordingly. ARC reduces the burden of manual memory management and helps prevent common memory-related bugs, such as over-releasing or memory leaks.
Both MRC and ARC are based on the concept of reference counting, where objects keep track of how many references they have. When an object’s reference count reaches zero, it is deallocated.
ARC is the preferred memory management mechanism in modern Objective-C development as it reduces the chances of memory-related bugs and simplifies memory management. However, developers should still understand memory management concepts, such as strong and weak references, retain cycles, and the use of strong
, weak
, and unsafe_unretained
attributes.
Question:
Explain the concept of Core Animation in iOS development. What is Core Animation, and how does it enable the creation of smooth animations? Provide an example demonstrating the usage of Core Animation.
Answer:
Core Animation is a framework provided by Apple that enables the creation and management of animations in iOS and macOS applications. It is a high-performance animation framework built on top of Core Graphics and Core Image.
Core Animation enables the creation of smooth animations by leveraging hardware acceleration and rendering techniques. It utilizes the graphics processing unit (GPU) to offload animation computations, resulting in faster and more efficient rendering.
Here’s an example demonstrating the usage of Core Animation:
CALayer *layer = [[CALayer alloc] init];
layer.bounds = CGRectMake(0, 0, 100, 100);
layer.position = CGPointMake(150, 150);
layer.backgroundColor = [UIColor redColor].CGColor;
CABasicAnimation *animation = [CABasicAnimation animationWithKeyPath:@"opacity"];
animation.fromValue = @(1.0);
animation.toValue = @(0.0);
animation.duration = 1.0;
animation.repeatCount = HUGE_VALF;
[layer addAnimation:animation forKey:@"opacityAnimation"];
[self.view.layer addSublayer:layer];
Code language: PHP (php)
In this example, a CALayer
object is created and configured with a size, position, and background color. A CABasicAnimation
is created to animate the opacity property of the layer from 1.0 to 0.0 over a duration of 1.0 second. The animation is set to repeat indefinitely. The animation is added to the layer using the addAnimation:forKey:
method.
The layer is then added to the view’s layer hierarchy, and the animation is automatically applied, creating a fade-out effect.
Core Animation provides a wide range of animation capabilities, including keyframe animations, transitions, and custom animations. It simplifies the creation of smooth and visually appealing animations in iOS applications.
Question:
Explain the concept of Autolayout in iOS development. What is Autolayout, and how does it enable building adaptive user interfaces? Provide an example demonstrating the usage of Autolayout.
Answer:
Autolayout is a layout system provided by Apple in iOS development that enables building adaptive and flexible user interfaces. It allows developers to define the relationships and constraints between UI elements, ensuring that the layout adapts to different screen sizes, orientations, and localization.
Autolayout enables building adaptive user interfaces by using a system of constraints. Constraints define the position, size, and alignment of UI elements relative to each other or to the parent view. Autolayout automatically adjusts the layout based on these constraints, ensuring that the UI remains visually consistent across different devices and orientations.
Here’s an example demonstrating the usage of Autolayout:
UIView *redView = [[UIView alloc] init];
redView.backgroundColor = [UIColor redColor];
redView.translatesAutoresizingMaskIntoConstraints = NO;
[self.view addSubview:redView];
// Add constraints
[NSLayoutConstraint activateConstraints:@[
[redView.leadingAnchor constraintEqualToAnchor:self.view.leadingAnchor constant:20.0],
[redView.topAnchor constraintEqualToAnchor:self.view.topAnchor constant:20.0],
[redView.widthAnchor constraintEqualToConstant:100.0],
[redView.heightAnchor constraintEqualToConstant:100.0]
]];
Code language: PHP (php)
In this example, a red-colored UIView
is created and added as a subview to the main view. Autolayout constraints are then created and activated to define the position and size of the red view. The red view is constrained to be 20 points from the leading and top edges of the main view, with a fixed width and height of 100 points.
Autolayout dynamically adjusts the position and size of the red view based on the constraints, ensuring that it remains consistent across different screen sizes and orientations.
Autolayout provides a powerful and flexible way to create adaptive user interfaces, enabling developers to build layouts that automatically adapt to different devices and localization requirements.
Question:
Explain the concept of NSURLSession in iOS development. What is NSURLSession, and how does it enable network communication? Provide an example demonstrating the usage of NSURLSession.
Answer:
NSURLSession is a networking API provided by Apple for performing network communication in iOS and macOS applications. It provides a powerful and flexible interface for making HTTP and HTTPS requests, handling authentication, managing background downloads/uploads, and more.
NSURLSession enables network communication by managing the entire lifecycle of network tasks. It handles the creation of requests, sending the requests, receiving responses, and managing the session and connection states. It supports various types of tasks, including data tasks, download tasks, and upload tasks.
Here’s an example demonstrating the usage of NSURLSession:
NSURL *url = [NSURL URLWithString:@"https://api.example.com/data"];
NSURLRequest *request = [NSURLRequest requestWithURL:url];
NSURLSessionDataTask *dataTask = [[NSURLSession sharedSession] dataTaskWithRequest:request completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"Error: %@", error);
} else {
// Process the received data
NSLog(@"Received data: %@", data);
}
}];
[dataTask resume];
Code language: JavaScript (javascript)
In this example, a URL and a URL request are created. A data task is then created using the shared session’s dataTaskWithRequest:completionHandler:
method. The completion handler is called when the data task finishes, providing the received data, response, and any error that occurred. Inside the completion handler, the received data is processed or logged.
NSURLSession provides a wide range of features and options for customizing network requests, handling authentication, managing cookies, and working with background transfers. It is a versatile and powerful framework for network communication in iOS applications.
Question:
Explain the concept of Core Graphics in iOS development. What is Core Graphics, and how does it enable drawing and rendering? Provide an example demonstrating the usage of Core Graphics.
Answer:
Core Graphics is a powerful framework provided by Apple in iOS development for drawing and rendering 2D graphics. It provides a set of low-level C-based APIs for creating and manipulating graphics contexts, paths, shapes, images, gradients, and more.
Core Graphics enables drawing and rendering by providing a comprehensive set of functions for creating and manipulating graphical elements. It allows developers to create custom views, generate PDF documents, perform image manipulation, apply transformations
, and perform complex rendering operations.
Here’s an example demonstrating the usage of Core Graphics to draw a rectangle:
- (void)drawRect:(CGRect)rect {
CGContextRef context = UIGraphicsGetCurrentContext();
CGContextSetFillColorWithColor(context, [UIColor redColor].CGColor);
CGContextFillRect(context, CGRectMake(50.0, 50.0, 100.0, 100.0));
}
Code language: JavaScript (javascript)
In this example, the drawRect:
method is overridden in a custom UIView
subclass. The current graphics context is obtained using UIGraphicsGetCurrentContext()
, and the fill color is set to red using CGContextSetFillColorWithColor()
. Finally, a rectangle is drawn on the graphics context using CGContextFillRect()
.
Core Graphics provides a wide range of functions and capabilities for creating and manipulating 2D graphics. It is commonly used for custom drawing, creating charts, rendering UI components, and generating complex graphical content in iOS applications.
Question:
Explain the concept of Core Data in iOS development. What is Core Data, and how does it enable data persistence? Provide an example demonstrating the usage of Core Data.
Answer:
Core Data is a framework provided by Apple that enables developers to manage the model layer objects and their persistence in iOS and macOS applications. It provides an object-oriented interface for working with a data model and supports various storage options, including SQLite, XML, and in-memory stores.
Core Data enables data persistence by providing an abstraction layer between the data model and the underlying storage. It manages the loading, saving, querying, and undo/redo of data objects, allowing developers to focus on working with objects rather than dealing with low-level data storage operations.
Here’s an example demonstrating the usage of Core Data:
// Person.h
@interface Person : NSManagedObject
@property (nonatomic, strong) NSString *name;
@property (nonatomic, strong) NSNumber *age;
@end
// Person.m
@implementation Person
@dynamic name;
@dynamic age;
@end
// Usage
NSManagedObjectContext *context = ... // Get the managed object context
Person *person = [NSEntityDescription insertNewObjectForEntityForName:@"Person"
inManagedObjectContext:context];
person.name = @"John";
person.age = @(25);
NSError *error;
if (![context save:&error]) {
NSLog(@"Failed to save person: %@", error);
} else {
NSLog(@"Person saved successfully");
}
Code language: JavaScript (javascript)
In this example, a Core Data entity named “Person” is defined using the NSManagedObject
subclass. The entity has two properties, “name” and “age”. To create and save a person object, an instance of the managed object context is obtained, and a new person object is inserted into the context. The properties of the person object are set, and the context is saved to persist the changes.
Core Data provides powerful features such as data modeling, relationships, versioning, and faulting, making it a preferred choice for managing data persistence in iOS applications.
These answers provide an overview of the concepts and explanations for each question. For further understanding, it is recommended to refer to the Objective-C and iOS documentation and additional resources.
1,000 Companies use CoderPad to Screen and Interview Developers
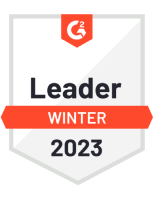
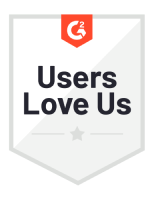
Best interview practices for Objective-C roles
To ensure successful Objective-C interviews, it is crucial to take into account various aspects, such as the applicant’s background and the specific engineering position. To foster a positive interview experience, we suggest implementing the following best practices:
- Develop technical questions that are based on real-world business situations within your organization. This strategy will effectively engage the applicant and assist in evaluating their fit with your team.
- Establish a cooperative atmosphere by encouraging candidates to ask questions throughout the interview.
- Candidates should have an understanding of Auto Layout and constraints for building responsive apps.
Moreover, adhering to standard interview practices is essential when conducting Objective-C interviews. This includes tailoring the question difficulty to suit the applicant’s capabilities, offering timely updates regarding their application status, and allowing them to inquire about the assessment process and cooperation with you and your team.