Kotlin Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Kotlin developers for your organization.
Kotlin
Known primarily for its use in Android development — Google announced it as an official Android language in 2017 — Kotlin is also popular for its Java interoperability, concise syntax, and null safety type system.
According to the CoderPad 2024 Developer survey, Kotlin is the 13th most in-demand language among technical recruiters and hiring managers.
We have designed functional coding assignments and interview queries specifically aimed at assessing developers’ Kotlin expertise during coding interviews. Moreover, we have assembled a collection of recommended guidelines to guarantee that your interview questions effectively measure the candidates’ proficiency in Kotlin.
Table of Contents
Kotlin example question
Help us design a parking lot
Hey candidate! Welcome to your interview. Boilerplate is provided. Feel free to change the code as you see fit. To run the code at any time, please hit the run button located in the top left corner.
Goals: Design a parking lot using object-oriented principles
Here are a few methods that you should be able to run:
- Tell us how many spots are remaining
- Tell us how many total spots are in the parking lot
- Tell us when the parking lot is full
- Tell us when the parking lot is empty
- Tell us when certain spots are full e.g. when all motorcycle spots are taken
- Tell us how many spots vans are taking up
Assumptions:
- The parking lot can hold motorcycles, cars and vans
- The parking lot has motorcycle spots, car spots and large spots
- A motorcycle can park in any spot
- A car can park in a single compact spot, or a regular spot
- A van can park, but it will take up 3 regular spots
- These are just a few assumptions. Feel free to ask your interviewer about more assumptions as needed
Kotlin skills to assess
Jobs using Kotlin
Junior Kotlin interview questions
Question: What is the difference between val
and var
in Kotlin? Provide an example to demonstrate their usage.
Answer:
In Kotlin, val
is used to declare a read-only (immutable) variable, while var
is used to declare a mutable variable. Once initialized, the value of a val
cannot be changed, whereas a var
can be reassigned.
val pi = 3.14
var radius = 5.0
radius = 7.0 // Valid: radius is mutable and can be reassigned
pi = 3.14159 // Invalid: pi is read-only and cannot be reassigned
Code language: JavaScript (javascript)
Question: In the given Kotlin code, there is an error preventing the function from executing correctly. Identify the error and provide a fix.
fun printMessage() {
println(message)
val message = "Hello, World!"
}
printMessage()
Code language: JavaScript (javascript)
Answer:
The error is due to the usage of the message
variable before it is declared. To fix the error, move the declaration of message
before the println
statement.
fun printMessage() {
val message = "Hello, World!"
println(message)
}
printMessage()
Code language: JavaScript (javascript)
Question: Explain the concept of nullable types in Kotlin and how to safely handle null values.
Answer:
Nullable types in Kotlin allow variables to hold either a non-null value of the declared type or a special value called null
. To safely handle null values, Kotlin provides two main approaches: null checks and safe calls.
Null checks involve verifying if a nullable variable holds a non-null value before accessing its properties or calling its methods. This can be done using the safe access operator ?.
or the not-null assertion operator !!
.
Safe calls, denoted by the ?.
operator, enable executing a statement only if the variable is non-null. If the variable is null, the expression returns null without throwing an exception.
val nullableString: String? = null
val length = nullableString?.length // Returns null if nullableString is null
nullableString?.let { str ->
println(str.length) // Executed only if nullableString is non-null
}
Code language: JavaScript (javascript)
By utilizing null checks and safe calls, developers can handle null values safely and prevent NullPointerExceptions.
Question: Explain the purpose of the lateinit
modifier in Kotlin and provide an example of its usage.
Answer:
The lateinit
modifier is used in Kotlin for properties that are declared without an initial value but are guaranteed to be initialized before they are used. It allows the property to be accessed directly without nullable checks or assignment checks.
Here’s an example:
class Person {
lateinit var name: String
fun initializeName() {
name = "John Doe"
}
fun printName() {
if (::name.isInitialized) {
println(name)
}
}
}
val person = Person()
person.initializeName()
person.printName() // Output: John Doe
Code language: JavaScript (javascript)
In this example, the name
property is declared with lateinit
as it will be initialized later in the initializeName
function. The printName
function can directly access the name
property without null checks due to the guarantee that it will be initialized before use.
Question: Explain the difference between List
and MutableList
interfaces in Kotlin.
Answer:
In Kotlin, List
and MutableList
are interfaces representing read-only and mutable lists, respectively.
List
is an interface that provides read-only access to a collection of elements. Once created, the elements of a List
cannot be modified. The List
interface provides operations like accessing elements by index, checking size, iterating over elements, and more.
val readOnlyList: List<String> = listOf("Apple", "Banana", "Orange")
println(readOnlyList[0]) // Output: Apple
readOnlyList.add("Grapes") // Error: Cannot modify a read-only list
Code language: JavaScript (javascript)
MutableList
, on the other hand, is an interface that extends List
and provides additional operations to modify the collection, such as adding, removing, or updating elements.
val mutableList: MutableList<String> = mutableListOf("Apple", "Banana", "Orange")
mutableList.add("Grapes")
println(mutableList) // Output: [Apple, Banana, Orange, Grapes]
Code language: JavaScript (javascript)
Using MutableList
, you can dynamically modify the list by adding, removing, or updating elements after its creation.
Question: Explain the concept of higher-order functions in Kotlin and provide an example.
Answer:
Higher-order functions in Kotlin are functions that can accept other functions as parameters or return functions as results. They allow developers to treat functions as first-class citizens, enabling powerful functional programming techniques.
Here’s an example of a higher-order function:
fun calculate(a: Int, b: Int, operation: (Int, Int) -> Int): Int {
return operation(a, b)
}
val addition = { x: Int, y: Int -> x + y }
val result = calculate(5, 3, addition) // Result: 8
Code language: JavaScript (javascript)
In this example, the calculate
function accepts two integers (a
and b
) and a function (operation
) that takes two integers and returns an integer. The operation
function can be any function that matches the specified signature.
The higher-order function allows flexibility by accepting different operations, such as addition, subtraction, multiplication, or even custom functions, to be passed as arguments.
Question: In the given Kotlin code, there is a bug preventing the loop from executing correctly. Identify the bug and provide a fix.
val numbers = mutableListOf(1, 2, 3, 4, 5)
for (i in numbers.indices) {
if (i % 2 == 0) {
numbers.removeAt(i)
}
}
println(numbers) // Output: [2, 4]
Code language: JavaScript (javascript)
Answer:
The bug is due to modifying the numbers
list while iterating over it using indices
. When an
element is removed from the list, the indices shift, leading to incorrect removal of elements.
To fix the bug, iterate in reverse order or use a while
loop:
val numbers = mutableListOf(1, 2, 3, 4, 5)
var i = numbers.size - 1
while (i >= 0) {
if (i % 2 == 0) {
numbers.removeAt(i)
}
i--
}
println(numbers) // Output: [2, 4]
Code language: JavaScript (javascript)
By iterating in reverse order or using a while
loop, the removal of elements does not affect the subsequent indices.
Question: Explain the concept of extension functions in Kotlin and provide an example.
Answer:
Extension functions in Kotlin allow developers to add new functions to existing classes without modifying their source code. They provide a way to extend the functionality of classes, including third-party or built-in classes, without inheritance.
Here’s an example of an extension function:
fun String.removeSpaces(): String {
return this.replace(" ", "")
}
val text = "Hello World"
val result = text.removeSpaces() // Result: "HelloWorld"
Code language: JavaScript (javascript)
In this example, the removeSpaces
function is defined as an extension function for the String
class. It removes spaces from a string by utilizing the replace
function. The extension function can be called directly on any string object, providing a more concise and readable way to manipulate strings.
Question: Explain the concept of data classes in Kotlin and their benefits. Provide an example of a data class and its usage.
Answer:
Data classes in Kotlin are special classes that are primarily used to hold data/state rather than behavior. They provide several benefits, such as automatic generation of common methods, property accessors, and component functions.
Here’s an example of a data class:
data class Person(val name: String, val age: Int)
val person = Person("John Doe", 30)
println(person.name) // Output: John Doe
println(person.age) // Output: 30
In this example, the Person
class is declared as a data class with two properties: name
and age
. Kotlin automatically generates useful methods like toString
, equals
, hashCode
, and copy
for the data class.
Data classes are commonly used for modeling entities, transferring data between components, or representing JSON or database records. Their concise syntax and generated functionality simplify common operations involving data.
Question: Explain the concept of coroutines in Kotlin and their advantages over traditional thread-based concurrency.
Answer:
Coroutines in Kotlin provide a way to write asynchronous, non-blocking code that is more readable and easier to reason about than traditional thread-based concurrency approaches. They allow developers to write sequential code while executing operations concurrently.
Advantages of coroutines over traditional thread-based concurrency include:
- Lightweight: Coroutines are lighter than threads, allowing for highly scalable concurrency without the overhead of thread creation and management.
- Sequential Code Structure: Coroutines use sequential code structure with suspending functions, allowing developers to write asynchronous code that looks similar to synchronous code, enhancing readability and maintainability.
- Exception Handling: Coroutines provide structured exception handling, making it easier to handle and propagate exceptions within asynchronous operations.
- Integration with Libraries: Coroutines integrate well with existing libraries and frameworks, enabling asynchronous programming with familiar APIs.
Here’s an example of using coroutines with suspend
functions:
import kotlinx.coroutines.*
suspend fun fetchData(): String {
delay(1000)
return "Data fetched"
}
fun main() = runBlocking {
launch {
val data = fetchData()
println(data)
}
println("Coroutine is running")
}
Code language: JavaScript (javascript)
In this example, the fetchData
function is declared as a suspend
function, indicating that it can be suspended without blocking the underlying thread. The runBlocking
coroutine builder is used to create a coroutine scope, and the launch
function is used to launch a new coroutine. The code executes sequentially, and the delay in fetchData
does not block the main thread.
Coroutines provide a powerful and efficient way to handle asynchronous operations in Kotlin, making concurrency easier to implement and maintain.
Intermediate Kotlin interview questions
Question: Explain the concept of sealed classes in Kotlin and their purpose in modeling restricted class hierarchies. Provide an example to illustrate their usage.
Answer:
Sealed classes in Kotlin are used to represent restricted class hierarchies, where all possible subclasses of a sealed class are known and defined within the same file or module. Sealed classes are typically used to model data types that can take on a limited set of values.
Here’s an example of using a sealed class:
sealed class Result
data class Success(val data: String) : Result()
data class Error(val message: String) : Result()
fun processResult(result: Result) {
when (result) {
is Success -> println("Data: ${result.data}")
is Error -> println("Error: ${result.message}")
}
}
val successResult = Success("Some data")
val errorResult = Error("Something went wrong")
processResult(successResult) // Output: Data: Some data
processResult(errorResult) // Output: Error: Something went wrong
In this example, the Result
class is declared as a sealed class, and two subclasses, Success
and Error
, are defined within the same file. Sealed classes are used in the processResult
function to handle different types of results using a when
expression.
Question: In the given Kotlin code, there is a bug preventing the function from executing correctly. Identify the error and provide a fix.
fun fibonacci(n: Int): Int {
if (n == 0 || n == 1) {
return n
}
return fibonacci(n - 1) + fibonacci(n - 2)
}
val result = fibonacci(5)
println(result)
Code language: JavaScript (javascript)
Answer:
The bug in the code is that it is recursively calculating the Fibonacci sequence without any memoization or caching. This leads to exponential time complexity, resulting in slow performance for larger values of n
.
To fix this, you can introduce memoization to cache previously calculated values of the Fibonacci sequence using an array or a HashMap.
val fibCache = mutableMapOf<Int, Int>()
fun fibonacci(n: Int): Int {
if (n == 0 || n == 1) {
return n
}
if (fibCache.containsKey(n)) {
return fibCache[n]!!
}
val fib = fibonacci(n - 1) + fibonacci(n - 2)
fibCache[n] = fib
return fib
}
val result = fibonacci(5)
println(result)
Code language: JavaScript (javascript)
By caching the intermediate results in fibCache
, the function avoids redundant calculations and significantly improves performance.
Question: Explain the concept of dependency injection (DI) in Kotlin and its benefits in software development. Provide an example to demonstrate the usage of DI.
Answer:
Dependency Injection (DI) is a design pattern and technique used in software development to decouple dependencies between classes. It involves injecting the dependencies required by a class from external sources rather than having the class create or manage its dependencies.
DI provides several benefits, including:
- Modular and Testable Code: DI promotes modularity by allowing individual components to focus on their specific responsibilities. It also simplifies unit testing as dependencies can be easily mocked or replaced with test doubles.
- Loose Coupling: DI reduces the coupling between components, making the codebase more flexible and maintainable. Changes in one component do not affect the entire system, as long as the contract (interface) between components remains consistent.
- Inversion of Control (IoC): DI facilitates the Inversion of Control principle by shifting the responsibility of managing dependencies to external sources (e.g., DI containers or frameworks). This allows for better control and flexibility in configuring and managing the application’s dependencies.
Here’s an example of using DI in Kotlin with constructor injection:
class UserService(private val userRepository: UserRepository) {
// Class implementation
}
class UserRepository {
// Class implementation
}
fun main() {
val userRepository = UserRepository()
val userService = UserService(userRepository)
// Use the userService instance
}
In this example, the UserService
class has a dependency on the UserRepository
class. Instead of creating an instance of UserRepository
within UserService
, the dependency is injected through the constructor. This allows for better flexibility, testability, and loose coupling between the classes.
Question: Explain the concepts of covariance and contravariance in Kotlin’s type system. Provide examples to illustrate their usage.
Answer:
Covariance and contravariance are concepts related to type compatibility in Kotlin’s type system, specifically for generic types.
Covariance refers to a relationship between two generic types, where the subtype relationship between their type arguments is preserved. It allows a variable of a generic type with a specific subtype to be assigned to a variable of a more general generic type.
open class Animal
class Cat : Animal()
fun printAnimals(animals: List<Animal>) {
for (animal in animals) {
println(animal)
}
}
val cats: List<Cat> = listOf(Cat(), Cat())
printAnimals(cats) // Covariance: List<Cat> is assigned to List<Animal>
Code language: HTML, XML (xml)
In this example, the printAnimals
function expects a List<Animal>
. However, a List<Cat>
is assigned to it due to covariance. Since Cat
is a subtype of Animal
, the assignment is allowed.
Contravariance, on the other hand, refers to a relationship where the subtype relationship between type arguments is reversed. It allows a variable of a more general generic type to be assigned to a variable of a specific subtype.
interface Comparator<in T> {
fun compare(a: T, b: T): Int
}
val animalComparator: Comparator<Animal> = object : Comparator<Animal> {
override fun compare(a: Animal, b: Animal): Int {
// Comparison logic
}
}
val catComparator: Comparator<Cat> = animalComparator // Contravariance: Comparator<Animal> is assigned to Comparator<Cat>
Code language: HTML, XML (xml)
In this example, the Comparator<Animal>
instance is assigned to a variable of type Comparator<Cat>
due to contravariance. The assignment is allowed because Comparator<T>
is declared as contravariant by using the in
keyword.
Question: Explain the concept of extension properties in Kotlin and provide an example of their usage.
Answer:
Extension properties in Kotlin allow developers to add new properties to existing classes without modifying their source code. They provide a convenient way to extend the functionality of classes, similar to extension functions.
Here’s an example of an extension property:
val String.firstChar: Char
get() = if (isEmpty()) throw NoSuchElementException() else this[0]
fun main() {
val text = "Hello"
println(text.firstChar) // Output: H
}
Code language: JavaScript (javascript)
In this example, an extension property firstChar
is added to the String
class. It provides a getter implementation that returns the first character of the string. The extension property can be accessed directly on any string object.
Extension properties are useful for adding additional properties to existing classes, especially when the property relies on the state or behavior of the class.
Question: Explain the concept of delegates in Kotlin and provide an example of using the built-in Lazy
delegate.
Answer:
Delegates in Kotlin are a mechanism to delegate the implementation of a property or function to another object. They provide a way to implement common patterns, reduce boilerplate code, and separate concerns.
The built-in Lazy
delegate is commonly used to implement lazy initialization of properties. It ensures that the initialization code for a property is executed only once, the first time the property is accessed, and subsequent accesses return the cached value.
Here’s an example of using the Lazy
delegate:
val expensiveCalculation: Int by lazy {
println("Calculating...")
// Expensive calculation logic
42
}
fun main() {
println("Before accessing property")
println(expensiveCalculation)
println(expensiveCalculation)
}
Code language: JavaScript (javascript)
In this example, the expensiveCalculation
property is declared using the by lazy
syntax. The lambda expression provided to lazy
is the initialization logic, which is executed only when the property is first accessed.
The output of the code will be:
Before accessing property
Calculating...
42
42
The initialization code is executed only once, and subsequent accesses to the property return the cached value without re-executing the initialization logic.
Question: Explain the concept of higher-order functions with receiver (also known as extension function literals with receiver) in Kotlin. Provide an example to illustrate their usage.
Answer:
Higher-order functions with receiver (also known as extension function literals with receiver) are a powerful feature in Kotlin that allows defining function literals with a special receiver object. These functions can access properties and functions of the receiver object as if they were defined inside it.
Here’s an example of a higher-order function with receiver:
data class Person(val name: String, val age: Int)
fun Person.printDetails() {
println("Name: $name, Age: $age")
}
fun main() {
val person = Person("John Doe", 30)
person.printDetails() // Output: Name: John Doe, Age: 30
}
In this example, the printDetails
function is defined as an extension function with receiver for the Person
class. Inside the function, the properties name
and age
are accessed directly, as if they were part of the class itself.
Higher-order functions with receiver provide a concise and expressive way to define utility functions or DSLs (Domain-Specific Languages) by leveraging the context of a specific receiver object.
Question: Explain the concept of functional programming in Kotlin and its advantages. Provide examples of functional programming techniques in Kotlin.
Answer:
Functional programming is a programming paradigm that emphasizes the use of pure functions, immutability, and higher-order functions. Kotlin provides support for functional programming, enabling developers to write concise, readable, and maintainable code.
Advantages of functional programming in Kotlin include:
- Readability and Expressiveness: Functional programming techniques, such as using higher-order functions and function composition, can lead to code that is easier to read and understand.
- Immutability: Functional programming promotes immutability, reducing the complexity of state management and making code more predictable and thread-safe.
- Avoidance of Side Effects: Pure functions, which produce the same output for the same inputs and have no side effects, make code more reliable, testable, and debuggable.
- Concurrency and Parallelism: Functional programming facilitates writing code that is amenable to parallel and concurrent execution, leveraging techniques like immutability and pure functions.
Here are some examples of functional programming techniques in Kotlin:
- Higher-Order Functions: Functions that take other functions as parameters or return functions as results, allowing for behavior parameterization.
- Lambda Expressions: Concise function literals that can be passed as arguments or stored in variables, enabling anonymous function definitions.
- Immutable Data Structures: Using read-only collections (e.g.,
List
,Set
,Map
) and data classes to ensure immutability and avoid unintended modifications. - Function Composition: Combining multiple functions to create a new function by chaining their invocations, facilitating modular and reusable code.
Functional programming in Kotlin provides a powerful set of tools and techniques to write elegant and maintainable code, particularly when solving problems that involve transformations, aggregations, or concurrency.
Question: Explain the concept of delegation in Kotlin and provide an example of using the by
keyword for class delegation.
Answer:
Delegation in Kotlin is a design pattern that allows one class to delegate some of its responsibilities to another class. It provides a way to achieve code reuse, composition over inheritance, and separation of concerns.
In Kotlin, class delegation can be implemented using the by
keyword, which delegates the implementation of an interface or the behavior of a property to another object.
Here’s an example of class delegation using the by
keyword:
interface SoundProducer {
fun makeSound()
}
class Dog : SoundProducer {
override fun makeSound() {
println("Bark!")
}
}
class GuardDog(soundProducer: SoundProducer) : SoundProducer by soundProducer
fun main() {
val dog = Dog()
val guardDog = GuardDog(dog)
guardDog.makeSound() // Output: Bark!
}
In this example, the Dog
class implements the SoundProducer
interface. The GuardDog
class delegates the makeSound
function to an instance of a SoundProducer
passed in its constructor. Consequently, when makeSound
is called on guardDog
, it is delegated to the dog
instance, resulting in “Bark!” being printed.
By using delegation, classes can focus on their core responsibilities while delegating specific tasks or behavior to other classes, promoting code reuse and maintainability.
Question: Explain the concepts of coroutines in Kotlin and their advantages over traditional thread-based concurrency.
Answer:
Coroutines in Kotlin are a powerful feature that enables writing asynchronous, non-blocking code in a sequential and straightforward manner. Coroutines provide a way to perform concurrent and asynchronous programming without the complexities associated with traditional thread-based approaches.
Advantages of coroutines over traditional thread-based concurrency include:
- Sequential and Asynchronous Code: Coroutines allow developers to write asynchronous code in a sequential style, making it easier to understand, read, and reason about.
- Lightweight: Coroutines are lightweight and can be launched in large numbers without incurring the overhead associated with creating and managing threads.
- Structured Concurrency: Coroutines facilitate structured concurrency by providing mechanisms to ensure that all launched coroutines complete before the parent coroutine or scope completes. This simplifies resource management and error handling.
- Suspending Functions: Coroutines leverage suspending functions, which can be used to perform long-running or IO-bound operations without blocking the underlying thread. This allows for efficient utilization of system resources.
- Coroutine Builders and Scopes: Kotlin provides coroutine builders and scopes, such as
launch
,async
,runBlocking
, andcoroutineScope
, to control the lifecycle and execution of coroutines, enabling fine-grained control and composition.
Here’s an example of using coroutines to perform concurrent operations:
import kotlinx.coroutines.*
suspend fun fetchData(): String {
delay(1000) // Simulate a delay
return "Data fetched"
}
fun main() = runBlocking {
val job1 = launch {
val data = fetchData()
println(data)
}
val job2 = launch {
val data = fetchData()
println(data)
}
// Wait for both jobs to complete
job1.join()
job2.join()
}
Code language: JavaScript (javascript)
In this example, two coroutines (job1
and job2
) are launched to fetch data concurrently using the launch
coroutine builder. The runBlocking
coroutine scope is used to ensure the main
function does not complete until all launched coroutines finish execution.
Coroutines provide a structured and efficient approach to concurrent programming in Kotlin, offering improved readability, scalability, and performance compared to traditional thread-based concurrency models.
Senior Kotlin interview questions
Question: Explain the concept of DSL (Domain-Specific Language) in Kotlin and its benefits. Provide an example of creating a DSL in Kotlin.
Answer:
DSL (Domain-Specific Language) in Kotlin refers to a specialized language designed to address a specific problem domain. It allows developers to express solutions in a more concise, expressive, and readable manner, tailored to the specific domain’s requirements.
Benefits of DSL in Kotlin include:
- Readability and Expressiveness: DSLs provide a syntax that closely resembles the problem domain, making the code more readable and understandable, especially for non-technical domain experts.
- Enhanced Productivity: DSLs can simplify complex tasks by providing a higher-level abstraction, reducing the amount of code required and enabling faster development and maintenance.
- Domain-Specific Validation: DSLs can enforce domain-specific constraints and validations, leading to safer and more reliable code.
- Improved Collaboration: DSLs enable better collaboration between domain experts and developers, as the DSL syntax can bridge the gap between technical and non-technical stakeholders.
Here’s an example of creating a DSL for configuring a mock server:
class MockServerDSL {
private val routes = mutableListOf<Route>()
fun route(path: String, response: String) {
routes.add(Route(path, response))
}
fun start() {
// Code to start the mock server with configured routes
}
}
class Route(val path: String, val response: String)
fun mockServer(configuration: MockServerDSL.() -> Unit) {
val dsl = MockServerDSL()
configuration(dsl)
dsl.start()
}
fun main() {
mockServer {
route("/api/users", "[{ "name": "John" }]")
route("/api/products", "[{ "name": "Phone" }]")
}
}
Code language: HTML, XML (xml)
In this example, a DSL is created for configuring a mock server. The mockServer
function takes a lambda with a receiver of MockServerDSL
as its argument, allowing the configuration of routes using the DSL syntax. The route
function within the DSL adds routes to the MockServerDSL
instance.
By leveraging DSLs, the code becomes more readable and focused on the problem domain, providing a concise and expressive way to configure the mock server.
Question: Explain the concept of type-safe builders in Kotlin and their benefits. Provide an example of using type-safe builders to construct a complex object.
Answer:
Type-safe builders in Kotlin are a technique used to construct complex objects or data structures in a structured and type-safe manner. They provide a fluent and DSL-like syntax for building objects, ensuring type safety and compile-time checks.
Benefits of type-safe builders include:
- Readability and Expressiveness: Type-safe builders provide a structured and declarative way to construct objects, making the code more readable and self-explanatory.
- Type Safety and Compile-Time Checks: Builders leverage Kotlin’s static typing system to enforce type safety during the construction process. Compiler checks help catch errors early, reducing the risk of runtime exceptions.
- Configuration Flexibility: Builders allow for flexible configuration options, enabling the customization of complex objects with various parameters and properties.
- Domain-Specific Expressiveness: Type-safe builders can be designed with a domain-specific vocabulary, making the code more expressive and closely aligned with the problem domain.
Here’s an example of using a type-safe builder to construct a complex object:
data class Person(val name: String, val age: Int, val address: String)
class PersonBuilder {
var name: String = ""
var age: Int = 0
var address: String = ""
fun build(): Person {
return Person(name, age, address)
}
}
fun person(block: PersonBuilder.() -> Unit): Person {
val builder = PersonBuilder()
block(builder)
return builder.build()
}
fun main() {
val person = person {
name = "John Doe"
age = 30
address = "123 Main St"
}
println(person)
}
In this example, the person
function acts as a type-safe builder for constructing a Person
object. The person
function takes a lambda with a receiver of PersonBuilder
as its argument, allowing the configuration of properties using a builder-style syntax. The build
function within the PersonBuilder
class constructs the Person
object based on the configured properties.
By using a type-safe builder, the construction of complex objects becomes more structured, readable, and error-resistant, with compile-time checks ensuring type safety throughout the process.
Question: Explain the concept of operator overloading in Kotlin and provide examples of using overloaded operators.
Answer:
Operator overloading in Kotlin allows developers to define the behavior of operators (e.g., arithmetic, comparison) for custom classes or types. It provides a way to make instances of a class behave like built-in types, enabling more intuitive and expressive code.
Here are some examples of using overloaded operators:
- Arithmetic Operators:
data class Vector(val x: Int, val y: Int) {
operator fun plus(other: Vector): Vector {
return Vector(x + other.x, y + other.y)
}
}
val v1 = Vector(1, 2)
val v2 = Vector(3, 4)
val sum = v1 + v2
println(sum) // Output: Vector(x=4, y=6)
In this example, the plus
operator is overloaded using the operator
keyword to define vector addition. The +
operator can now be used to add two Vector
instances.
- Comparison Operators:
data class Point(val x
: Int, val y: Int) : Comparable<Point> {
override fun compareTo(other: Point): Int {
return (x + y) - (other.x + other.y)
}
}
val p1 = Point(2, 3)
val p2 = Point(4, 5)
val result = p1 < p2
println(result) // Output: true
Code language: HTML, XML (xml)
In this example, the compareTo
function is implemented to compare Point
instances based on the sum of their coordinates. The <
operator is now overloaded, allowing direct comparison between Point
instances.
By overloading operators, custom classes can provide a more intuitive and expressive syntax, resembling the behavior of built-in types and enhancing code readability.
Question: Explain the concept of inline functions in Kotlin and their benefits. Provide an example demonstrating the usage of inline functions.
Answer:
Inline functions in Kotlin are a mechanism to optimize the performance of higher-order functions by inlining the function body at the call site during compilation. Inline functions help reduce the overhead of function calls and improve performance.
Benefits of inline functions include:
- Reduced Function Call Overhead: Inlining the function body eliminates the overhead of creating a function call stack frame, improving performance in scenarios where higher-order functions are used extensively.
- Improved Performance for Lambdas: Inlining can avoid the creation of lambda objects and capture contexts, reducing memory allocations and garbage collection overhead.
- Control Over Function Parameters: Inline functions allow developers to control the behavior of function parameters, such as enforcing non-local returns or inlining specific lambda expressions.
- Code Generation Flexibility: Inlining functions at the call site provides more opportunities for compiler optimizations, such as constant propagation, dead code elimination, and loop unrolling.
Here’s an example demonstrating the usage of an inline function:
inline fun measureTimeMillis(block: () -> Unit): Long {
val startTime = System.currentTimeMillis()
block()
return System.currentTimeMillis() - startTime
}
fun main() {
val duration = measureTimeMillis {
// Code block to measure execution time
Thread.sleep(1000)
}
println("Execution time: $duration ms")
}
Code language: JavaScript (javascript)
In this example, the measureTimeMillis
function is declared as an inline function. It takes a lambda parameter block
, which represents the code to be measured. The function measures the execution time using System.currentTimeMillis()
and returns the duration.
By inlining the measureTimeMillis
function, the code within the lambda is directly inserted at the call site during compilation, avoiding the function call overhead and providing more accurate time measurement.
Question: Explain the concept of reified types in Kotlin and their usage with inline functions. Provide an example demonstrating the usage of reified types.
Answer:
Reified types in Kotlin allow developers to access and manipulate type information at runtime. Reified types are used in combination with inline functions, providing a way to operate on generic types without losing type information due to type erasure.
Here’s an example demonstrating the usage of reified types:
inline fun <reified T> getTypeName(): String {
return T::class.simpleName ?: "Unknown"
}
fun main() {
val typeName = getTypeName<String>()
println("Type name: $typeName") // Output: Type name: String
}
Code language: PHP (php)
In this example, the getTypeName
function is declared as an inline function with a reified type parameter T
. Inside the function, T::class
is used to access the KClass
instance representing the type T
. The simpleName
property is then accessed to retrieve the type name as a string.
By using a reified type, the type information is preserved at runtime, allowing functions like getTypeName
to operate on generic types without sacrificing type information due to type erasure.
Question: Explain the concept of sealed classes in Kotlin and their purpose in modeling restricted class hierarchies. Provide an example to illustrate their usage.
Answer:
Sealed classes in Kotlin are used to represent restricted class hierarchies, where all possible subclasses of a sealed class are known and defined within the same file or module. Sealed classes are typically used to model data types that can take on a limited set of values.
Here’s an example illustrating the usage of sealed classes:
sealed class Result
data class Success(val data: String) : Result()
data class Error(val message: String) : Result()
fun handleResult(result: Result) {
when (result) {
is Success -> println("Success: ${result.data}")
is Error -> println("Error: ${result.message}")
}
}
val successResult = Success("Data received")
val errorResult = Error("Failed to fetch data")
handleResult(successResult) // Output: Success: Data received
handleResult(errorResult) // Output: Error: Failed to fetch data
In this example, the Result
class is declared as a sealed class. Two subclasses, Success
and Error
, are defined within the same file. The handleResult
function uses a when
expression to handle different types of Result
objects based on their subclass.
Sealed classes provide a restricted hierarchy that ensures exhaustiveness checks in when
expressions, making the code more robust and less error-prone.
Question: Explain the concept of extension functions in Kotlin and their benefits. Provide an example demonstrating the usage of extension functions.
Answer:
Extension functions in Kotlin allow developers to add new functions to existing classes without modifying their source code. They provide a way to extend the functionality of classes, including third-party or built-in classes, without inheritance.
Benefits of extension functions include:
- Code Organization: Extension functions allow related functions to be grouped together, improving code organization and readability.
- Readability and Discoverability: Extension functions provide a more concise and natural way to express operations on objects, enhancing code readability and making the codebase more discoverable.
- Consistency and Interoperability: Extension functions enable developers to add missing functionality to existing classes, ensuring consistent usage and facilitating interoperability between different libraries or modules.
Here’s an example demonstrating the usage of extension functions:
fun String.removeSpaces(): String {
return this.replace(" ", "")
}
val text = "Hello World"
val result = text.removeSpaces()
println(result) // Output: HelloWorld
Code language: JavaScript (javascript)
In this example, the removeSpaces
function is defined as an extension function for the String
class. It removes spaces from a string by utilizing the replace
function. The extension function can be called directly on any string object, providing a more concise and readable way to manipulate strings.
Extension functions are a powerful feature in Kotlin that promotes code reuse, enhances code organization, and allows for the extension of existing classes with additional functionality.
Question: Explain the concept of reflection in Kotlin and its usage. Provide an example demonstrating the usage of reflection.
Answer:
Reflection in Kotlin refers to the ability of a program to examine its own structure, properties, and behaviors at runtime. It allows developers to inspect and manipulate classes, properties, functions, and other entities dynamically.
Reflection in Kotlin can be useful for various scenarios, such as:
- Dynamic Loading: Reflection enables loading classes and invoking methods dynamically based on runtime conditions or configurations.
- Frameworks and Libraries: Reflection is often used by frameworks and libraries to provide runtime extensibility and customization.
- Serialization and Deserialization: Reflection can be utilized to analyze and manipulate object properties during serialization and deserialization processes.
Here’s an example demonstrating the usage of reflection:
data class Person(val name: String, val age: Int)
fun main() {
val person = Person("John Doe", 30)
val clazz = person::class
val properties = clazz.members.filterIsInstance<KProperty1<Person, *>>()
properties.forEach { property ->
val value = property.get(person)
println("${property.name}: $value")
}
}
In this example, the Person
class is defined with name
and age
properties. Using reflection, the clazz
variable obtains the KClass
instance representing the Person
class. The properties
variable retrieves all properties of type KProperty1<Person, *>
, which are then iterated to print their names and values.
Reflection provides the ability to analyze and manipulate classes and their members at runtime, offering flexibility and dynamic behavior in Kotlin applications. However, it should be used judiciously, as it may introduce performance overhead and can be error-prone if used improperly.
Question: Explain the concept of Kotlin coroutines and their benefits over traditional thread-based concurrency. Provide examples illustrating the usage of coroutines.
Answer:
Kotlin coroutines are a lightweight concurrency framework that allows developers to write asynchronous and non-blocking code in a sequential and structured manner. Coroutines provide a way to perform concurrent operations without the complexities associated with traditional thread-based approaches.
Benefits of Kotlin coroutines over traditional thread-based concurrency include:
- Asynchronous and Non-Blocking: Coroutines enable writing asynchronous code in a sequential and straightforward style, avoiding callback hell and nested callbacks.
- Lightweight and Efficient: Coroutines are lightweight, allowing developers to launch thousands of concurrent coroutines without the resource overhead associated with threads.
- Structured Concurrency: Coroutines provide structured concurrency, ensuring that all launched coroutines complete before the parent coroutine or scope completes. This simplifies resource management and error handling.
- Suspend and Resume: Coroutines can suspend and resume their execution without blocking threads, making them ideal for I/O-bound and CPU-bound operations.
- Cancellation and Timeouts: Coroutines support cancellation and timeouts, allowing graceful termination of tasks and managing long-running operations.
Here are some examples illustrating the usage of Kotlin coroutines:
- Launching a Coroutine:
import kotlinx.coroutines.*
fun main() {
GlobalScope.launch {
delay(1000)
println("Hello from coroutine!")
}
println("Hello from main!")
Thread.sleep(2000)
}
Code language: JavaScript (javascript)
In this example, a coroutine is launched using GlobalScope.launch
. Inside the coroutine, a delay of 1000 milliseconds is introduced, and then a message is printed. While the coroutine is suspended, the main thread continues executing the next statement. After a sleep of 2000 milliseconds, the program terminates.
- Asynchronous Coroutine:
import kotlinx.coroutines.*
import java.util.concurrent.Executors
fun main() {
val executor = Executors.newFixedThreadPool(4).asCoroutineDispatcher()
val deferredResults = mutableListOf<Deferred<Int>>()
runBlocking {
repeat(10) { index ->
val deferred = async(executor) {
delay(1000)
println("Coroutine $index completed")
index
}
deferredResults.add(deferred)
}
val sum = deferredResults.awaitAll().sum()
println("Sum of results: $sum")
}
executor.close()
}
Code language: JavaScript (javascript)
In this example, multiple coroutines are launched concurrently using async
and awaitAll
. Each coroutine introduces a delay of 1000 milliseconds, prints a message, and returns its index. The awaitAll
function waits for all coroutines to complete and returns their results. Finally, the sum of the results is calculated and printed.
Kotlin coroutines provide a powerful and structured approach to concurrency, enabling developers to write efficient and non-blocking code with ease.
Question: Explain the concept of Kotlin delegates and their usage. Provide examples of using built-in delegates and creating custom delegates.
Answer:
Delegates in Kotlin provide a mechanism to delegate the implementation of properties and functions to another object. They allow developers to reuse common behavior, separate concerns, and enhance code readability.
Kotlin provides built-in delegates, such as lazy
, observable
, and vetoable
, which handle common scenarios. Additionally, developers can create custom delegates to encapsulate specific logic.
Here are examples of using built-in and custom delegates:
- Using the
lazy
Delegate:
val expensiveCalculation: Int by lazy {
println("Performing expensive calculation...")
// Expensive calculation logic
42
}
fun main() {
println("Before accessing property")
println(expensiveCalculation)
println(expensiveCalculation)
}
Code language: JavaScript (javascript)
In this example, the lazy
delegate is used to perform lazy initialization of the expensiveCalculation
property. The calculation is executed only when the property is first accessed, and subsequent accesses return the cached value.
- Using the
observable
Delegate:
import kotlin.properties.Delegates
var counter by Delegates.observable(0) { _, oldValue, newValue ->
println("Counter changed: $oldValue -> $newValue")
}
fun main() {
counter = 5 // Output: Counter changed: 0 -> 5
counter = 10 // Output: Counter changed: 5 -> 10
}
Code language: JavaScript (javascript)
In this example, the observable
delegate is used to monitor changes to the counter
property. Whenever the property is assigned a new value, the associated callback is triggered, printing the old and new values.
- Creating a Custom Delegate:
import kotlin.reflect.KProperty
class UpperCaseDelegate {
private var value: String = ""
operator fun getValue(thisRef: Any?, property: KProperty<*>): String {
return value.toUpperCase()
}
operator fun setValue(thisRef: Any?, property: KProperty<*>, newValue: String) {
value = newValue.toUpperCase()
}
}
class Person {
var name: String by UpperCaseDelegate()
}
fun main() {
val person = Person()
person.name = "John Doe"
println(person.name) // Output: JOHN DOE
}
Code language: JavaScript (javascript)
In this example, a custom delegate UpperCaseDelegate
is created to convert the assigned value to uppercase. The delegate implements the getValue
and setValue
operators, allowing it to intercept property access and modification. The name
property of the Person
class uses this custom delegate.
Delegates in Kotlin provide a flexible way to add behavior to properties and functions, allowing for code reuse, separation of concerns, and enhanced readability.
1,000 Companies use CoderPad to Screen and Interview Developers
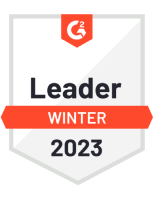
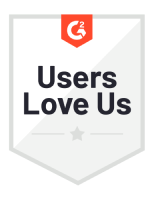
Best interview practices for Kotlin roles
In order to conduct effective Kotlin interviews, it is crucial to take into account multiple aspects, such as the applicant’s background and the specific engineering position. To guarantee a productive interview experience, we suggest implementing the following best practices:
- Design technical questions that mirror real-life business situations within your organization. This method will efficiently engage the applicant and help in assessing their compatibility with your team.
- Foster a collaborative atmosphere by motivating candidates to pose questions during the interview.
- Knowledge of coroutines and asynchronous programming may be useful for building non-blocking programs.
Furthermore, compliance with proven interview procedures is critical when conducting Kotlin interviews. This includes tailoring the difficulty of the questions to align with the applicant’s abilities, promptly updating them on the status of their application, and offering them the chance to inquire about the evaluation process and cooperation with you and your team.