Haskell Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Haskell developers for your organization.
Haskell
A purely functional programming language, Haskell maintains popular usage due to its strong static typing and type inference, its use of lazy evaluation for more efficient code, and its use of algebraic data types and pattern matching.
Haskell was first conceived in the late 1980s during a series of meetings among researchers in functional programming languages. The goal was to create a standardized, open-source, purely functional programming language.
https://doi.org/10.1145/1238844.1238856
Our team has developed practical coding assignments and interview questions tailored to assess developers’ Haskell skills during coding interviews. Additionally, we have compiled a set of best practices to ensure your interview questions accurately evaluate the candidates’ proficiency in Haskell.
Table of Contents
Haskell example question
Help us design a parking lot
Hey candidate! Welcome to your interview. Boilerplate is provided. Feel free to change the code as you see fit. To run the code at any time, please hit the run button located in the top left corner.
Goals: Design a parking lot using object-oriented principles
Here are a few methods that you should be able to run:
- Tell us how many spots are remaining
- Tell us how many total spots are in the parking lot
- Tell us when the parking lot is full
- Tell us when the parking lot is empty
- Tell us when certain spots are full e.g. when all motorcycle spots are taken
- Tell us how many spots vans are taking up
Assumptions:
- The parking lot can hold motorcycles, cars and vans
- The parking lot has motorcycle spots, car spots and large spots
- A motorcycle can park in any spot
- A car can park in a single compact spot, or a regular spot
- A van can park, but it will take up 3 regular spots
- These are just a few assumptions. Feel free to ask your interviewer about more assumptions as needed
Haskell skills to assess
Jobs using Haskell
Junior Haskell interview questions
Question: The following Haskell code is intended to calculate the factorial of a given number. However, it contains a bug and throws an error. Identify the bug and fix the code.
factorial :: Integer -> Integer
factorial n = if n == 0 then 1 else n * factorial n-1
Code language: Haskell (haskell)
Answer:
The bug in the code is the incorrect placement of parentheses. The correct code is as follows:
factorial :: Integer -> Integer
factorial n = if n == 0 then 1 else n * factorial (n-1)
Code language: Haskell (haskell)
Question: Explain what lazy evaluation means in Haskell and how it differs from strict evaluation.
Answer:
Lazy evaluation in Haskell means that expressions are not evaluated until their values are actually needed. Haskell only evaluates expressions when the result is required to complete a computation. This allows for potentially infinite data structures and efficient handling of computations. In contrast, strict evaluation evaluates expressions immediately, even if their results are not used.
Question: The following Haskell code is intended to check if a given list is sorted in ascending order. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
isSorted :: Ord a => [a] -> Bool
isSorted [] = True
isSorted [_] = True
isSorted (x:y:xs) = x <= y && isSorted xs
Code language: Haskell (haskell)
Answer:
The logical error in the code is that it only checks adjacent elements for ordering, but it doesn’t consider the ordering of subsequent elements. The correct code is as follows:
isSorted :: Ord a => [a] -> Bool
isSorted [] = True
isSorted [_] = True
isSorted (x:y:xs) = x <= y && isSorted (y:xs)
Code language: PHP (php)
Question: What are higher-order functions in Haskell? Provide an example.
Answer:
Higher-order functions in Haskell are functions that can take other functions as arguments or return functions as results. They treat functions as first-class citizens. An example of a higher-order function is the map
function:
map :: (a -> b) -> [a] -> [b]
Code language: Haskell (haskell)
The map
function takes a function (a -> b)
and a list [a]
as arguments and applies the function to each element of the list, producing a new list of type [b]
.
Question: The following Haskell code is intended to calculate the sum of squares of even numbers in a given list. However, it contains a syntax error and doesn’t compile. Identify the error and fix the code.
sumOfEvenSquares :: [Integer] -> Integer
sumOfEvenSquares = sum . map ^2 . filter even
Answer:
The syntax error in the code is that the operator ^
for exponentiation needs to be surrounded by parentheses when used in the map
function. The correct code is as follows:
sumOfEvenSquares :: [Integer] -> Integer
sumOfEvenSquares = sum . map (^2) . filter even
Question: Explain what currying is in Haskell and provide an example.
Answer:
Currying in Haskell is the process of transforming a function that takes multiple arguments into a sequence of functions, each taking a single argument. It allows partial application of functions, where some arguments can be applied to produce a new function that takes the remaining arguments.
Here’s an example:
add :: Int -> Int -> Int
add x y = x + y
Code language: Haskell (haskell)
By calling add
with a single argument, it returns a new function that takes the second argument:
addTwo :: Int -> Int
addTwo = add 2
In this example, addTwo
is a curried function that adds 2 to its argument.
Question: The following Haskell code is intended to calculate the sum of all elements in a given list. However, it contains a syntax error and doesn’t compile. Identify the error and fix the code.
sumList :: Num a => [a] -> a
sumList = foldl (+) 0
Code language: PHP (php)
Answer:
The syntax error in the code is the incorrect placement of parentheses. The correct code is as follows:
sumList :: Num a => [a] -> a
sumList = foldl (+) 0
Code language: PHP (php)
Question: What is pattern matching in Haskell? Provide an example.
Answer:
Pattern matching in Haskell is a way to deconstruct data structures by matching their shape against specific patterns. It allows extracting and binding values from data structures, such as lists or tuples, based on their structure.
Here’s an example using pattern matching with a list:
sumList :: [Int] -> Int
sumList [] = 0
sumList (x:xs) = x + sumList xs
In this example, the function sumList
pattern matches on the list structure. If the list is empty, it returns 0. Otherwise, it extracts the first element x
and recursively sums the rest of the list xs
.
Question: The following Haskell code is intended to reverse a given list. However, it contains a logical error and doesn’t produce the correct result. Identify the error and fix the code.
reverseList [] = []
reverseList :: [a] -> [a]
reverseList (x:xs) = reverseList xs ++ [x]
Answer:
The logical error in the code is that it appends the elements of the list in the wrong order. The correct code is as follows:
reverseList :: [a] -> [a]
reverseList [] = []
reverseList (x:xs) = reverseList xs ++ [x]
Question: What are typeclasses
in Haskell? Provide an example.
Answer:
Typeclasses in Haskell define a set of functions or operations that can be implemented for different types. They define a common interface or behavior that types can adhere to. Typeclasses allow for polymorphism, where functions can be defined generically and work with different types that belong to the same typeclass.
An example of a typeclass is Eq
, which is used for types that can be compared for equality:
class Eq a where
(==) :: a -> a -> Bool
(/=) :: a -> a -> Bool
Code language: JavaScript (javascript)
Any type a
that implements the (==)
and (/=)
functions can be considered an instance of the Eq
typeclass, allowing for equality comparisons between values of that type.
Intermediate Haskell interview questions
Question: Consider the following Haskell code snippet:
data Person = Person
{ name :: String
, age :: Int
}
displayPerson :: Person -> String
displayPerson p = "Name: " ++ name p ++ ", Age: " ++ show (age p)
Code language: Haskell (haskell)
Explain the purpose of the code and how it can be used to create and display information about a person.
Answer:
The code snippet defines a data type Person
with two fields: name
of type String
and age
of type Int
. It also provides a function displayPerson
that takes a Person
as input and returns a formatted string containing the person’s name and age.
This code allows us to create instances of Person
with specific names and ages and display their information in a readable format. Here’s an example usage:
john :: Person
john = Person { name = "John Doe", age = 30 }
main :: IO ()
main = putStrLn (displayPerson john)
Code language: Haskell (haskell)
Running the main
function will print the following output:
Name: John Doe, Age: 30
Code language: HTTP (http)
Question:
You are working on a Haskell project that requires you to compute the Fibonacci sequence. Write a function fibonacci
that takes an integer n
as input and returns the n
th Fibonacci number. Ensure that your implementation is efficient and handles large values of n
.
Answer:
fibonacci :: Int -> Integer
fibonacci n = fibs !! n
where
fibs = 0 : 1 : zipWith (+) fibs (tail fibs)
This implementation uses an infinite list fibs
to memoize the Fibonacci sequence. By using zipWith
and tail
, we efficiently generate the Fibonacci numbers. The !!
operator retrieves the n
th Fibonacci number from the list. This approach ensures efficient computation and handles large values of n
due to memoization.
Question: You are working with a list of integers and need to find the maximum value in the list. Write a function findMax
that takes a list of integers and returns the maximum value using recursion.
Answer:
findMax :: [Int] -> Int
findMax [] = error "Empty list"
findMax [x] = x
findMax (x:xs) = max x (findMax xs)
Code language: JavaScript (javascript)
The findMax
function uses recursion to iterate through the list. The base cases are defined for an empty list (throws an error) and a list with a single element (returns that element). For lists with multiple elements, the function compares the current element x
with the maximum value of the rest of the list (findMax xs)
using the max
function.
Question:
You are implementing a basic calculator in Haskell. Write a function calculate
that takes a string representation of an arithmetic expression and returns the computed result. The expression can include addition, subtraction, multiplication, and division operations.
Example: calculate "3 + 5 * 2 - 10 / 2"
Answer:
import Text.Parsec
import Text.Parsec.String (Parser)
import Text.Parsec.Language (emptyDef)
import qualified Text.Parsec.Token as Token
lexer :: Token.TokenParser ()
lexer = Token.makeTokenParser emptyDef
integer :: Parser Integer
integer = Token.integer lexer
parens :: Parser a -> Parser a
parens = Token.parens lexer
symbol :: String -> Parser String
symbol = Token.symbol lexer
term :: Parser Integer
term = parens expr <|> integer
expr :: Parser Integer
expr = chainl1 term addop
addop :: Parser (Integer -> Integer -> Integer)
addop = (symbol "+" >> return (+))
<|> (symbol "-" >> return (-))
calculate :: String -> Either ParseError Integer
calculate input = parse expr "" input
Code language: JavaScript (javascript)
The calculate
function uses the Text.Parsec
library to parse and evaluate the arithmetic expression. It defines a lexer using Token.makeTokenParser
and provides parsers for integers, parentheses, and symbols. The term
parser handles nested expressions and integers. The expr
parser uses chainl1
to handle left-associative addition and subtraction operations. The addop
parser matches either addition or subtraction symbols and returns the corresponding function (+)
or (-)
.
To use the calculate
function, pass the arithmetic expression as a string. It returns either a ParseError
if the expression is invalid or the computed result as an Integer
.
Question:
You are working with a list of strings and need to concatenate them into a single string. However, you want to separate each string with a specific delimiter. Write a function joinWithDelimiter
that takes a delimiter string and a list of strings and returns a single string with the delimiter between each element.
Example: joinWithDelimiter ", " ["apple", "banana", "orange"]
Answer:
joinWithDelimiter :: String -> [String] -> String
joinWithDelimiter _ [] = ""
joinWithDelimiter _ [x] = x
joinWithDelimiter delimiter (x:xs) = x ++ delimiter ++ joinWithDelimiter delimiter xs
Code language: JavaScript (javascript)
The joinWithDelimiter
function uses recursion to concatenate the strings with the specified delimiter. The base cases are defined for an empty list (returns an empty string) and a list with a single element (returns that element). For lists with multiple elements, the function appends the current element x
, the delimiter, and the result of recursively joining the rest of the list (joinWithDelimiter delimiter xs)
.
For the example input joinWithDelimiter ", " ["apple", "banana", "orange"]
, the function would return the string "apple, banana, orange"
.
Question:
You are building a system that requires generating a random password. Write a function generatePassword
that takes an integer length
as input and returns a random password string of the specified length. The password should contain a combination of uppercase letters, lowercase letters, and digits.
Answer:
import System.Random (randomRIO)
import Data.List (shuffle)
generatePassword :: Int -> IO String
generatePassword length = do
let characters = ['A'..'Z'] ++ ['a'..'z'] ++ ['0'..'9']
shuffledCharacters <- shuffle characters
randomIndices <- take length <$> shuffle [0..length-1]
let password = map (shuffledCharacters !!) randomIndices
return password
Code language: JavaScript (javascript)
The generatePassword
function uses the System.Random
library and the shuffle
function from Data.List
to generate a random password. It first defines the characters
list, which contains all the possible characters for the password (uppercase letters, lowercase letters, and digits). It then shuffles the characters to create a random ordering.
Next, it generates length
random indices using shuffle [0..length-1]
. These indices will be used to select characters from the shuffled list. Finally, it maps the selected characters to their corresponding indices in the shuffled list to create the password.
The function returns an IO String
action, which means it performs I/O and returns a string. To use this function,
you can bind the result of generatePassword
in the IO
monad and execute it using main
or any suitable place in your program.
Question:
You are working with a list of integers and need to remove all occurrences of a specific element from the list. Write a function removeElement
that takes an element and a list of integers and returns a new list with all occurrences of the element removed.
Example: removeElement 2 [1, 2, 3, 2, 4, 2]
Answer:
removeElement :: Eq a => a -> [a] -> [a]
removeElement _ [] = []
removeElement x (y:ys)
| x == y = removeElement x ys
| otherwise = y : removeElement x ys
Code language: PHP (php)
The removeElement
function uses recursion to iterate through the list. The base cases are defined for an empty list (returns an empty list) and a non-empty list. If the current element y
is equal to the element to be removed x
, it recursively continues with the rest of the list ys
. Otherwise, it includes the current element y
in the result and continues recursively with the rest of the list ys
.
For the example input removeElement 2 [1, 2, 3, 2, 4, 2]
, the function would return the list [1, 3, 4]
.
Question:
You are building a web application and need to validate user input for an email address. Write a function isValidEmail
that takes a string as input and checks if it represents a valid email address according to a basic set of rules.
Answer:
import Text.Regex.Posix ((=~))
isValidEmail :: String -> Bool
isValidEmail email = email =~ "^[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,}$"
Code language: JavaScript (javascript)
The isValidEmail
function uses regular expressions to check if the input string matches the specified pattern. The pattern "^[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,}$"
represents a basic email address pattern.
Explanation of the pattern:
^[A-Za-z0-9._%+-]+
: Matches one or more of the allowed characters for the local part of the email address.@
: Matches the “@” symbol.[A-Za-z0-9.-]+
: Matches one or more of the allowed characters for the domain part of the email address.\.
: Matches the dot (.) character, which needs to be escaped with backslash.[A-Za-z]{2,}
: Matches two or more alphabetic characters for the top-level domain.
If the input string matches the pattern, the function returns True
, indicating a valid email address. Otherwise, it returns False
.
Question:
You are working with a list of integers and need to find the second largest element in the list. Write a function findSecondLargest
that takes a list of integers and returns the second largest element. Assume that the list contains at least two distinct elements.
Answer:
import Data.List (sort)
findSecondLargest :: [Int] -> Int
findSecondLargest xs = last (take 2 (reverse (sort xs)))
Code language: JavaScript (javascript)
The findSecondLargest
function first sorts the list of integers in ascending order using sort
. It then reverses the sorted list using reverse
to obtain the elements in descending order. Finally, it takes the last two elements using take 2
and selects the second largest element using last
.
Note that this implementation assumes that the list contains at least two distinct elements. If the input list is not guaranteed to have at least two elements, additional checks should be added to handle such cases.
Question:
You are working with a list of tuples, where each tuple represents a person’s name and age. You need to filter out the people who are above a certain age threshold. Write a function filterByAge
that takes an age threshold and a list of tuples and returns a new list containing only the people who are above the age threshold.
Example: filterByAge 18 [("Alice", 20), ("Bob", 25), ("Charlie", 15), ("David", 30)]
Answer:
filterByAge :: Int -> [(String, Int)] -> [(String, Int)]
filterByAge threshold = filter ((_, age) -> age > threshold)
Code language: JavaScript (javascript)
The filterByAge
function uses the filter
higher-order function to remove elements from the list that do not satisfy the given predicate. In this case, the predicate is defined using a lambda function ((_, age) -> age > threshold)
.
The lambda function takes each tuple (name, age)
and checks if the age
is greater than the given threshold
. If the age is greater, the tuple is kept in the filtered list; otherwise, it is removed.
For the example input filterByAge 18 [("Alice", 20), ("Bob", 25), ("Charlie", 15), ("David", 30)]
, the function would return the list [("Alice", 20), ("Bob", 25), ("David", 30)]
.
Senior Haskell interview questions
Question:
In Haskell, what is a monad and how does it facilitate sequencing of computations? Provide an example of a monad in Haskell and explain how it can be used.
Answer:
A monad in Haskell is a computational context that facilitates sequencing of computations. It allows us to compose computations with side effects in a structured manner while maintaining referential transparency. Monads provide a set of operations, such as return
and (>>=)
, to work with values within the context and handle the sequencing of computations.
One example of a monad in Haskell is the Maybe
monad. The Maybe
monad represents a computation that can potentially produce a value or fail. It helps handle situations where a value may be missing (represented by Nothing
) or present (represented by Just a
).
Here’s an example usage of the Maybe
monad:
safeDivide :: Int -> Int -> Maybe Int
safeDivide x 0 = Nothing
safeDivide x y = Just (x `div` y)
performComputation :: Int -> Int -> Maybe Int
performComputation x y = do
a <- safeDivide x y
b <- safeDivide 10 a
return (a + b)
Code language: JavaScript (javascript)
In this example, safeDivide
is a function that divides two integers but returns Nothing
if the second argument is zero to avoid division by zero. The performComputation
function uses the Maybe
monad to handle the sequencing of computations. It first performs the division using safeDivide
and binds the result to a
. Then, it performs another division with 10
as the numerator and a
as the denominator, binding the result to b
. Finally, it returns the sum of a
and b
within the Maybe
monad context.
By using the Maybe
monad, the computations in performComputation
are sequenced such that if any step returns Nothing
, the subsequent steps are short-circuited, and the overall result becomes Nothing
. This allows for safe and concise handling of potentially failing computations.
Question:
You are building a web application in Haskell using a framework like Yesod or Scotty. Discuss the benefits and drawbacks of using a Haskell web framework compared to other languages like Python or Ruby.
Answer:
Using a Haskell web framework, such as Yesod or Scotty, offers several benefits and drawbacks compared to other languages like Python or Ruby.
Benefits of using a Haskell web framework:
- Strong static typing: Haskell’s static type system helps catch errors at compile time, reducing the likelihood of runtime errors in web applications. This leads to improved reliability and easier refactoring.
- High performance: Haskell’s efficient runtime system and optimized execution model make it well-suited for building high-performance web applications.
- Concurrency and parallelism: Haskell’s functional nature and built-in support for concurrency and parallelism enable efficient utilization of multi-core processors and scalable handling of concurrent requests.
- Expressive and concise code: Haskell’s functional programming paradigm allows for expressive and concise code, making it easier to understand, maintain, and reason about complex web applications.
- Strong emphasis on purity and immutability: Haskell encourages pure functions and immutable data, which promotes modularity, testability, and easier reasoning about the behavior of web applications.
Drawbacks of using a Haskell web framework:
- Learning curve: Haskell has a steeper learning curve compared to more mainstream languages like Python or Ruby. Its unique concepts and functional programming paradigm may require developers to invest time in understanding the language and associated frameworks.
- Smaller ecosystem: Haskell’s ecosystem, although growing steadily, is still smaller compared to languages like Python or Ruby. This may result in fewer available libraries and resources for specific web development needs.
- Less community support: Haskell, being a niche language, may have relatively fewer community resources, online forums, and Stack Overflow discussions compared to more widely-used languages. Finding specific solutions or troubleshooting may require more effort.
- Tooling and IDE support: While Haskell has mature tooling and IDE support, it may not be as extensive or polished as in other languages. IDEs and development tools may have limitations or lack certain features.
- Limited availability of developers: Finding experienced Haskell developers for a team or project may be more challenging compared to more popular languages. This could potentially impact the scalability and availability of development resources.
When deciding to use a Haskell web framework, it’s important to consider these factors and assess whether the benefits align with the project requirements and team’s familiarity and expertise with the language.
Question:
You are working on a Haskell project that requires interacting with a relational database. Explain how you would typically connect to a database, execute queries, and handle the results in Haskell.
Answer:
When working with a relational database in Haskell, the typical approach involves using a library like persistent
or HDBC
to establish a connection, execute queries, and handle the results.
Here’s a step-by-step overview of the process:
- Dependency management: Add the necessary database library as a dependency in your project’s
cabal
orstack
configuration file. - Establish a connection: Use the library’s functions or methods to establish a connection to the database by providing the required connection details, such as the host, port, username, password, and database name. This step usually involves creating a connection pool for efficient resource management.
- Query execution: Use the library’s functions or methods to construct and execute SQL queries. Libraries often provide an embedded DSL (Domain-Specific Language) for building queries in a type-safe manner, or you can use raw SQL queries directly. For example,
persistent
provides an embedded DSL, whileHDBC
allows executing raw SQL queries. - Handling results: Depending on the library, you can handle the query results in different ways. Common approaches include:
- Retrieving the results as a list of records, where each record represents a row in the result set. The records can be mapped to Haskell data types for easier manipulation.
- Utilizing streaming or pagination to process large result sets efficiently, avoiding excessive memory consumption.
- Using specific functions or combinators to aggregate, filter, or transform the results as needed.
- Error handling: Properly handle exceptions or errors that may occur during the connection establishment, query execution, or result processing. Libraries often provide mechanisms to handle and propagate errors in a controlled manner.
- Resource management: Ensure the proper release of acquired resources, such as closing the database connection or returning connections to the connection pool, to prevent resource leaks.
Overall, the process involves establishing a connection to the database, executing queries using the library’s API, processing the results, handling errors, and managing resources effectively.
Question:
You are working on a Haskell project that requires interacting with external REST APIs. How would you typically make HTTP requests and handle the responses in Haskell?
Answer:
When interacting with external REST APIs in Haskell, the typical approach involves using a library like http-client
or req
to make HTTP requests and handle the responses.
Here’s an overview of the process:
- Dependency management: Add the necessary HTTP library as a dependency in your project’s
cabal
orstack
configuration file. - Making HTTP requests: Use the library’s functions or methods to construct and make HTTP requests. Specify the request method (GET, POST, PUT, DELETE, etc.), URL, headers, request body (if applicable), and other relevant parameters.
- Handling responses: The library typically provides functions or combinators to handle the HTTP response. Some common approaches include:
- Parsing the response body as JSON using libraries like
aeson
,json
orhttp-client
‘s built-in support for JSON response parsing. - Extracting specific information from the response headers, such as authentication tokens or pagination details.
- Checking the status code and handling different response statuses (success, client or server errors) accordingly.
- Handling errors or exceptions that may occur during the request execution, such as network failures or invalid responses.
- Serializing and deserializing data: If your project involves sending or receiving JSON data, use a library like
aeson
orjson
to serialize your Haskell data types to JSON and deserialize JSON responses to Haskell data types. - Error handling: Properly handle exceptions or errors that may occur during the HTTP request or response handling. Libraries often provide mechanisms to handle and propagate errors in a controlled manner.
- Resource management: Ensure the proper release of acquired resources, such as closing network connections or freeing memory, to prevent resource leaks.
Overall, the process involves constructing and making HTTP requests, handling the responses, parsing JSON data (if required), handling errors, and managing resources effectively.
Question:
You are working on a Haskell project and need to perform concurrent or parallel computations for improved performance. Explain how you would typically achieve concurrency or parallelism in Haskell and discuss the advantages and considerations of each approach.
Answer:
In Haskell, achieving concurrency or parallelism can be accomplished through several approaches, including using pure concurrency, parallel strategies, or libraries like async
or stm
.
- Pure concurrency: Haskell provides built-in support for lightweight threads known as “green threads” or “Haskell threads.” By utilizing functions like
forkIO
or libraries likeunliftio
, you can create concurrent computations that can run simultaneously and independently within the same Haskell process. Pure concurrency allows for fine-grained control and is well-suited for I/O-bound tasks or situations where shared mutable state is not a concern.
Advantages: Lightweight and easy to create; suitable for I/O-bound tasks; fine-grained control over concurrency.
Considerations: No automatic load balancing; shared mutable state can lead to race conditions and require explicit synchronization.
- Parallel strategies: Haskell’s
Control.Parallel.Strategies
module provides strategies to express parallel computations. By annotating computations with parallel combinators likeparMap
,parList
, orusing
, you can leverage multiple CPU cores to perform parallel computations. Parallel strategies work well for tasks that can be broken down into independent subtasks, such as parallel map or reduce operations.
Advantages: Simple to use with built-in strategies; suitable for data parallelism; automatic load balancing.
Considerations: Requires task decomposition into parallelizable subtasks; shared mutable state can lead to race conditions and require explicit synchronization.
- Libraries like
async
orstm
: Haskell offers libraries likeasync
andstm
(Software Transactional Memory) to handle more complex concurrent or parallel scenarios. These libraries provide higher-level abstractions for managing concurrency, synchronization, and composition of computations.async
allows managing concurrent computations with support for cancellation, whilestm
offers atomic transactions for shared state manipulation in a concurrent setting.
Advantages: Higher-level abstractions for concurrent programming; support for cancellation and shared state management.
Considerations: Learning curve for advanced features; potential overhead in certain scenarios; careful design required for shared mutable state.
The choice of concurrency or parallelism approach depends on the specific requirements and characteristics of the problem at hand. It is important to consider factors such as the nature of the computation, availability of independent tasks, requirements for shared state management, and performance considerations to determine the most suitable approach.
Question:
You are working on a Haskell project and need to handle and manipulate large amounts of data efficiently. Explain how you would typically approach memory management and optimization in Haskell to ensure good performance and avoid excessive memory consumption.
Answer:
When working with large amounts of data in Haskell, efficient memory management and optimization can be achieved through various approaches and techniques. Here are some common practices:
- Use strict data types: By default, Haskell uses lazy evaluation, which can lead to memory thunks and potential memory leaks when working with large data structures. To mitigate this, consider using strict data types (
Data.Text
instead ofString
,Vector
instead ofList
, etc.) or strict evaluation annotations (!
) to force strictness when necessary. - Employ streaming and incremental processing: Instead of loading the entire dataset into memory, consider using streaming libraries like
conduit
orpipes
to process data incrementally. These libraries allow you to process data in a streaming fashion, reducing memory footprint and enabling efficient processing of large datasets. - Utilize data compression techniques: If your data is compressible, consider using compression libraries like
zlib
orlz4
to compress data before storage or during transit. This can significantly reduce memory usage and I/O overhead, especially when dealing with large files or network communication. - Optimize memory allocations: Be mindful of memory allocations in performance-critical sections. Use techniques like array fusion (
vector
library) or custom allocation strategies to minimize unnecessary memory allocations. Avoid intermediate data structures when possible by utilizing efficient combinators or specialized functions. - Profile and benchmark your code: Utilize profiling tools (
+RTS -p
,ghc-prof
,ghc-events
, etc.) to identify memory hotspots and bottlenecks in your code. Profile-guided optimization can help pinpoint areas that require optimization efforts and guide performance improvements. - Employ memory-safe techniques: Haskell provides memory-safe constructs by default. However, when working with lower-level libraries or FFI, be cautious of potential memory leaks or memory-related bugs. Proper resource management (e.g., freeing memory when necessary) and careful use of memory-allocated functions or libraries are crucial to ensure memory safety.
- Consider using libraries for specific use cases: Haskell offers various specialized libraries tailored for efficient data processing and manipulation, such as
vector
,unordered-containers
, orbytestring
. Utilizing these libraries can provide optimized data structures and algorithms, minimizing memory consumption and improving performance.
Remember that optimization should be performed based on actual profiling results rather than premature optimization. Measure, analyze, and iterate on your code to identify the critical areas for optimization and ensure that the optimizations align with the desired performance goals.
Question:
You are developing a Haskell project that requires handling asynchronous tasks or event-driven programming. Explain how you would typically handle asynchronous programming in Haskell and discuss the advantages and considerations of different approaches.
Answer:
Handling asynchronous tasks or event-driven programming in Haskell can be achieved through various approaches, including using async
library, STM
(Software Transactional Memory), or specialized event-driven frameworks like reactive-banana
or eventstore
.
Here are some common approaches with their advantages and considerations:
async
library: Theasync
library provides a lightweight concurrency abstraction with support for asynchronous tasks, cancellations, and composition. By utilizing theasync
functionsasync
,wait
, andcancel
, you can execute tasks concurrently and wait for their completion.
Advantages: Simple and lightweight; built-in cancellation support; good for managing concurrent tasks with fine-grained control.
Considerations: Manual
management of concurrency; requires explicit synchronization for shared mutable state.
STM
(Software Transactional Memory): STM is a built-in Haskell mechanism for concurrent programming with shared state. By usingTVar
(transactional variables) andSTM
monad, you can coordinate concurrent operations and ensure consistency.
Advantages: Provides a safe and composable way to handle shared state; automatic rollback of transactions on conflicts; built-in support for retry and orElse semantics.
Considerations: Requires understanding of transactional memory concepts; can be less performant than fine-grained concurrency in some cases.
- Event-driven frameworks: Haskell offers event-driven frameworks like
reactive-banana
oreventstore
, which provide abstractions for handling asynchronous events and managing event-driven architectures. These frameworks often utilize functional reactive programming (FRP) concepts.
Advantages: Higher-level abstractions for event-driven programming; declarative and compositional; well-suited for complex event handling and GUI applications.
Considerations: Learning curve for FRP concepts; specialized frameworks may have limited community support or require additional dependencies.
The choice of approach depends on the specific requirements and characteristics of the project. Consider factors such as the complexity of event handling, requirements for shared mutable state, need for cancellation or composition, and familiarity with the chosen approach to determine the most suitable solution.
Question:
You are building a Haskell project that requires interacting with a message queue system like RabbitMQ or Kafka. Explain how you would typically integrate and work with message queues in Haskell, including consuming and producing messages.
Answer:
Integrating and working with message queue systems like RabbitMQ or Kafka in Haskell involves using appropriate client libraries, such as amqp
for RabbitMQ or haskell-kafka-client
for Kafka. Here’s an overview of the typical process:
- Dependency management: Add the necessary message queue client library as a dependency in your project’s
cabal
orstack
configuration file. - Connecting to the message queue: Use the library’s functions or methods to establish a connection to the message queue system. Provide the required connection details, such as host, port, authentication credentials, and queue or topic names.
- Producing messages: Use the library’s functions or methods to publish or produce messages to the message queue. Specify the target queue or topic and the content of the message. Depending on the library, you may need to serialize the message content to a suitable format, such as JSON or binary.
- Consuming messages: Configure a message consumer to receive and process messages from the message queue. Depending on the library, you can define message handlers or callback functions that will be invoked when a new message arrives. The library handles the message retrieval and acknowledgment process.
- Message processing: Within the message handler or callback function, implement the necessary logic to process the received message. This could involve deserializing the message content, performing business operations based on the message, and optionally acknowledging the successful processing of the message to the message queue system.
- Error handling and retries: Handle exceptions or errors that may occur during message consumption or processing. Depending on the library, you may have options for retrying failed message processing or implementing error handling strategies.
- Resource management: Ensure the proper release of acquired resources, such as closing the connection to the message queue system or freeing any associated resources, to prevent leaks or connection limits.
It’s important to refer to the documentation and examples provided by the chosen message queue client library for specific implementation details and best practices.
Question:
You are working on a Haskell project that requires interacting with external APIs using OAuth for authentication and authorization. Explain how you would typically handle the OAuth flow in Haskell, including the steps involved and any necessary libraries or frameworks.
Answer:
Handling the OAuth flow in Haskell typically involves several steps, including obtaining access tokens, making authenticated requests, and handling the OAuth protocol. Here’s an overview of the process:
- Dependency management: Add the necessary OAuth library as a dependency in your project’s
cabal
orstack
configuration file. Common libraries for OAuth in Haskell includeoauth
andoauth2
. - Register your application: Before integrating with an OAuth provider, you need to register your application with the provider to obtain the necessary credentials, such as client ID and client secret. Each provider may have its own registration process.
- Obtain authorization URL: Construct the authorization URL by providing the appropriate parameters, including the client ID, redirect URI, and scopes. The authorization URL is used to initiate the OAuth flow and obtain user authorization.
- Redirect and authorization code: After the user grants authorization, the OAuth provider redirects the user back to your application’s redirect URI, along with an authorization code. Capture and store this code for the next step.
- Exchange authorization code for access token: Use the library’s functions or methods to exchange the authorization code for an access token. This involves making a request to the OAuth provider’s token endpoint, providing the authorization code, client ID, client secret, and redirect URI. The OAuth library typically handles this token exchange process.
- Store and manage access tokens: Once you obtain an access token, store it securely (e.g., in a session, database, or environment variable) for subsequent authenticated requests. The OAuth library often provides functions to manage token storage and retrieval.
- Make authenticated requests: Use the access token to make authenticated requests to the external API. Include the access token in the request headers or query parameters, depending on the API requirements. Libraries like
http-client
or specialized OAuth clients often provide utilities for adding authorization headers. - Token refresh: If the access token expires, handle the token refresh process. This involves making a request to the OAuth provider’s token endpoint using a refresh token (if provided) or repeating the initial token acquisition process.
- Error handling and token revocation: Handle OAuth-related errors, such as token expiration, invalid credentials, or unauthorized requests. Consider implementing error handling strategies and mechanisms for token revocation if needed.
It’s important to consult the documentation and examples provided by the chosen OAuth library for specific implementation details and best practices, as the steps and methods may vary depending on the library and OAuth provider.
Question:
You are developing a Haskell project that requires interacting with a relational database, performing complex queries, and mapping the results to Haskell data types. Explain how you would typically handle query composition, result mapping, and abstraction layers in Haskell to achieve a clean and maintainable database interaction.
Answer:
Handling query composition, result mapping, and abstraction layers in Haskell when interacting with a relational database can be achieved through the use of libraries like persistent
, Opaleye
, or HDBC
. Here’s an overview of the typical approach:
- Choose a database library: Select a suitable database library based on your project requirements and preferences. Libraries like
persistent
provide high-level abstractions, while libraries likeOpaleye
offer a lower-level approach with greater control over SQL queries. - Define the database schema: Use the library’s DSL or declarative approaches to define the database schema. This involves defining Haskell data types that represent the tables and columns in the database, along with any relationships or constraints.
- Query composition: Use the library’s DSL or functions to compose database queries. Libraries like
persistent
provide a rich DSL for type-safe query composition using combinators or operators. Libraries likeOpaleye
allow you to construct queries directly using functions. - Result mapping: Define mapping functions or type classes to map the query results to Haskell data types. This typically involves defining instances of type classes like
FromRow
orToRow
forpersistent
, or providing mapping functions forOpaleye
. These mapping functions or instances handle the conversion between the database representation and Haskell data types. - Abstracting database operations: Consider creating an abstraction layer or repository pattern to encapsulate database operations and provide a clean interface for interacting with the database. This can involve defining functions or methods that encapsulate common CRUD operations, complex queries, or transaction handling.
- Handling migrations: If your project involves evolving the database schema, consider using migration tools like
persistent-migration
ormigrant
to manage schema changes and versioning. These tools allow you to define incremental changes to the schema and apply them in a controlled manner. - Error handling and transactions: Handle exceptions or errors that may occur during database operations. Ensure proper transaction handling using the library’s built-in transaction management mechanisms or by explicitly managing transactions.
By following these practices, you can achieve a clean and maintainable database interaction layer in Haskell. It allows for type-safe query composition, separation of concerns, and abstraction of database operations, leading to more maintainable code and improved readability.
1,000 Companies use CoderPad to Screen and Interview Developers
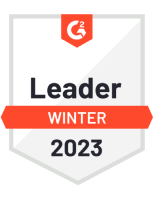
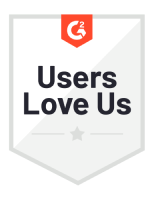
Best interview practices for Haskell roles
To conduct successful Haskell interviews, it is important to consider various factors, such as the candidate’s background and the specific engineering role. To ensure a positive interview experience, we recommend adopting the following best practices:
- Craft technical questions that reflect real-world business scenarios within your organization. This approach will effectively engage the candidate and help assess their compatibility with your team.
- Create a collaborative environment by inviting candidates to ask questions during the interview.
- It’s also helpful if candidates can demonstrate knowledge of useful Haskell libraries like Cabal, Stack, and Hackage.
In addition, adhering to standard interview practices is vital when conducting Haskell interviews. This involves adjusting the question difficulty to match the candidate’s abilities, providing timely updates on their application status, and giving them the opportunity to ask about the evaluation process and collaboration with you and your team.