Go Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Go developers for your organization.
Go
This Google-developed language has gained a strong following due to its superior performance, strong standard library, and cross-platform support.
According to the CoderPad 2024 Developer survey, Go is the 12th most in-demand language among technical recruiters and hiring managers.
We have created pragmatic programming tasks and interview inquiries designed to assess developers’ Go expertise during coding interviews. In addition, we have assembled a collection of best practices to guarantee that your interview questions effectively measure the candidates’ proficiency in Go.
Table of Contents
Go example question
Help us design a parking lot
Hey candidate! Welcome to your interview. Boilerplate is provided. Feel free to change the code as you see fit. To run the code at any time, please hit the run button located in the top left corner.
Goals: Design a parking lot using object-oriented principles
Here are a few methods that you should be able to run:
- Tell us how many spots are remaining
- Tell us how many total spots are in the parking lot
- Tell us when the parking lot is full
- Tell us when the parking lot is empty
- Tell us when certain spots are full e.g. when all motorcycle spots are taken
- Tell us how many spots vans are taking up
Assumptions:
- The parking lot can hold motorcycles, cars and vans
- The parking lot has motorcycle spots, car spots and large spots
- A motorcycle can park in any spot
- A car can park in a single compact spot, or a regular spot
- A van can park, but it will take up 3 regular spots
- These are just a few assumptions. Feel free to ask your interviewer about more assumptions as needed
Go skills to assess
Jobs using Go
Junior Go interview questions
Apologies for the misunderstanding. Here are 10 detailed questions for a junior Golang developer:
Question:
What is the purpose of a goroutine in Go, and how does it differ from a regular thread?
Answer:
A goroutine in Go is a lightweight, independently executing function that runs concurrently with other goroutines in the same address space. Goroutines are managed by the Go runtime and are cheaper to create and switch between compared to operating system threads. Goroutines are scheduled cooperatively, which means they yield control voluntarily, allowing other goroutines to run. In contrast, regular threads are managed by the operating system and have a higher memory and performance overhead.
Question:
How can you fix the following code to correctly calculate the sum of the numbers in the numbers
slice?
package main
import "fmt"
func main() {
numbers := []int{1, 2, 3, 4, 5}
sum := 0
for i := 0; i < len(numbers); i++ {
sum += numbers[i]
}
fmt.Println("Sum:", sum)
}
Code language: JavaScript (javascript)
Answer:
The provided code is already correct and will calculate the sum of the numbers in the numbers
slice correctly, resulting in the output “Sum: 15”.
Question:
What is the purpose of the defer
statement in Go, and how does it work?
Answer:
The defer
statement in Go is used to schedule a function call to be executed just before the surrounding function returns. It is commonly used for cleanup or resource release operations. The deferred function calls are executed in Last In, First Out (LIFO) order, meaning the most recently deferred function is executed first. The defer
statement is often used to ensure that resources are properly released even in the case of errors or early returns.
Question:
What is the output of the following code?
package main
import "fmt"
func main() {
str := "Hello, World!"
fmt.Println(len(str))
}
Code language: CSS (css)
Answer:
The code will output 13
because len(str)
returns the number of characters in the string str
, which is 13.
Question:
How can you fix the following code to correctly concatenate the two strings?
package main
import "fmt"
func main() {
str1 := "Hello"
str2 := "World"
result := str1 + " " + str2
fmt.Println(result)
}
Code language: JavaScript (javascript)
Answer:
The provided code is already correct and will concatenate the strings “Hello” and “World” correctly, resulting in the output “Hello World”.
Question:
Explain the purpose of a mutex in Go and how it helps in managing concurrent access to shared resources.
Answer:
A mutex (short for mutual exclusion) is a synchronization primitive in Go that allows multiple goroutines to coordinate and protect shared resources. It ensures that only one goroutine can access the protected resource at a time, preventing concurrent read or write conflicts. A mutex provides two main operations: Lock()
and Unlock()
. When a goroutine needs to access a shared resource, it first acquires the lock using Lock()
, performs its operation, and then releases the lock using Unlock()
. This way, the mutex ensures that only one goroutine can access the resource at any given time, maintaining data consistency and avoiding race conditions.
Question:
What will be the output of the following code?
package main
import "fmt"
func main() {
var numbers [5]int
fmt.Println(numbers)
}
Code language: JavaScript (javascript)
Answer:
The code will output [0 0 0 0 0]
because the declaration var numbers [5]int
creates an array of integers with a length of 5. Since the array
is not explicitly initialized, its elements are set to their respective zero values, which is 0
for integers.
Question:
How can you fix the following code to print the numbers from 1 to 5?
package main
import "fmt"
func main() {
for i := 0; i < 5; i++ {
fmt.Println(i + 1)
}
}
Code language: JavaScript (javascript)
Answer:
The provided code is already correct and will print the numbers from 1 to 5 correctly.
Question:
What is the purpose of a slice in Go, and how is it different from an array?
Answer:
A slice in Go is a flexible, dynamically-sized view into an underlying array. It provides a more powerful and convenient way to work with sequences of elements compared to arrays. Slices are references to arrays, allowing efficient manipulation of data without copying the entire sequence. Unlike arrays, slices can change in length dynamically, and their capacity can be extended by appending new elements. Slices are often used to represent and manipulate collections of data.
Question:
How can you fix the following code to print only the even numbers from the numbers
slice?
package main
import "fmt"
func main() {
numbers := []int{1, 2, 3, 4, 5, 6, 7, 8, 9, 10}
for _, num := range numbers {
if num%2 == 0 {
fmt.Println(num)
}
}
}
Code language: JavaScript (javascript)
Answer:
The provided code is already correct and will print only the even numbers from the numbers
slice, which are 2, 4, 6, 8, and 10.
Intermediate Go interview questions
Question:
What is the purpose of a Goroutine Pool in Go, and how can it be used to manage concurrent tasks efficiently?
Answer:
A Goroutine Pool, also known as a worker pool or task pool, is a design pattern that involves pre-creating a fixed number of Goroutines to handle tasks concurrently. The purpose of a Goroutine Pool is to limit the number of concurrent Goroutines and efficiently manage task execution. By reusing Goroutines, you can avoid the overhead of creating and destroying Goroutines for each task, resulting in better performance and resource management.
In Go, a Goroutine Pool can be implemented using a combination of Goroutines, channels, and synchronization mechanisms like WaitGroups or semaphores. Tasks are typically submitted to the pool through a channel, and the Goroutines in the pool continuously read from the channel, execute the tasks, and then loop back to read from the channel again. By controlling the number of Goroutines in the pool and the size of the task queue, you can fine-tune the level of concurrency and prevent resource exhaustion.
Question:
Explain the concept of a mutex in Go. How can a mutex be used to synchronize access to shared resources?
Answer:
In Go, a mutex (short for mutual exclusion) is a synchronization primitive used to protect shared resources from concurrent access. It provides a way to allow only one Goroutine to access the protected resource at a time, ensuring exclusive access and preventing data races.
A mutex has two main methods: Lock()
and Unlock()
. When a Goroutine wants to access a shared resource, it calls Lock()
on the mutex to acquire the lock. If the lock is already held by another Goroutine, the calling Goroutine will be blocked until the lock is released. Once the Goroutine finishes its work with the shared resource, it calls Unlock()
to release the lock, allowing other Goroutines to acquire it.
By appropriately placing Lock()
and Unlock()
calls around critical sections of code that access shared resources, you can enforce mutual exclusion and ensure that only one Goroutine can modify the shared resource at a time. This helps in preventing race conditions and maintaining data integrity in concurrent programs.
Question:
Explain the concept of defer in Go. How can defer be used to ensure the execution of cleanup tasks?
Answer:
In Go, defer
is a language feature that allows you to schedule a function call to be executed when the surrounding function completes, regardless of how it completes (whether it returns normally or panics). The deferred function call is executed just before the enclosing function returns.
Defer statements are typically used for performing cleanup tasks, such as closing files or releasing resources, that should always be executed regardless of the control flow. By deferring the cleanup function call, you ensure that it will be executed even if an error occurs or an early return statement is encountered.
The order of execution of deferred function calls is last-in, first-out (LIFO). This means that if multiple functions are deferred, the last one deferred will be the first one executed when the enclosing function returns.
Here’s an example:
func someFunction() {
resource := acquireResource()
defer releaseResource(resource) // Cleanup task
// Code that uses the resource
// ...
}
Code language: CSS (css)
In the example, releaseResource()
is deferred, ensuring that it will be called just before someFunction()
returns, regardless of the code flow within the function.
Question:
Explain the concept of middleware in the context of web applications. How can middleware be implemented in Go?
Answer:
In the context of web applications, middleware refers to a software layer placed between the web server and the actual application logic. It provides a way to intercept and modify requests and responses, add additional functionality, and enforce cross-cutting concerns.
Middleware functions in Go typically have the signature func(http.Handler) http.Handler
. They take an http.Handler
as input, which represents the next handler in the chain, and return a new http.Handler
that wraps the original handler with additional behavior. Middleware can perform tasks like authentication, request logging, error handling, caching, compression, and more.
Middleware functions can be chained together to form a middleware stack, where each middleware in the stack processes the request and invokes the next middleware in the chain. This allows for modular and reusable components to handle different aspects of the application’s functionality.
Go provides middleware support through the net/http
package, and there are also third-party middleware libraries like Negroni and Alice that simplify the implementation and composition of middleware in Go-based web applications.
Question:
Explain the concept of dependency injection in Go. How can dependency injection be implemented to achieve loose coupling and better testability?
Answer:
Dependency injection is a design pattern that allows the separation of concerns by providing dependencies to an object from the outside rather than creating them internally. The purpose of dependency injection is to achieve loose coupling between components and improve testability, reusability, and maintainability of the codebase.
In Go, dependency injection can be implemented using constructor injection or method injection. Constructor injection involves passing dependencies as parameters to a struct’s constructor function, while method injection involves passing dependencies as arguments to specific methods that require them.
By providing dependencies through interfaces rather than concrete types, you can easily swap implementations and write testable code. This allows you to replace real dependencies with mock or stub implementations during unit testing, making it easier to isolate and verify the behavior of individual components.
Here’s an example of constructor injection:
type MyService struct {
dependency Dependency
}
func NewMyService(dependency Dependency) *MyService {
return &MyService{
dependency: dependency,
}
}
// ...
Code language: JavaScript (javascript)
In the example, MyService
depends on Dependency
, which is passed through the constructor. This allows different implementations of Dependency
to be used interchangeably, promoting flexibility and testability.
Question:
Explain the concept of context in Go. How can context be used for managing deadlines, cancellations, and request-scoped values?
Answer:
In Go, the context
package provides a way to propagate request-scoped values, deadlines, and cancellations across Goroutines, allowing for better control and management of concurrent operations.
The context.Context
type represents a context, which can be created using the context.Background()
function or derived from an existing context using the context.WithXXX()
functions. A context carries values and deadlines across API boundaries, enabling the signaling of cancellation or timeout conditions.
Contexts are commonly used in scenarios where a Goroutine spawns child Goroutines to perform work, and there is a need to propagate certain information down the Goroutine tree. For example, in web applications, a request-scoped context can be used to carry request-specific data or manage timeouts and cancellations for long-running operations.
The context
package provides methods like context.WithDeadline()
, context.WithTimeout()
, and context.WithCancel()
to create derived contexts with specific deadlines or cancellation capabilities. These derived contexts can be passed to Goroutines, and the Goroutines can access the values or monitor the cancellation state through the context.
By utilizing context effectively, you can ensure proper resource cleanup, prevent Goroutine leaks, and handle timeouts or cancellations gracefully in your Go applications.
Question:
Explain the concept of an interface in Go. How can interfaces be used to achieve polym
orphism and decoupling in Go programs?
Answer:
In Go, an interface is a collection of method signatures that define a behavior. It specifies what methods a concrete type must implement to satisfy the interface contract. Interfaces provide a way to achieve polymorphism and decoupling in Go programs.
By defining an interface, you can write functions or methods that operate on values of any type that satisfies the interface. This allows you to write code that is agnostic to the specific concrete types but focuses on the behaviors they exhibit.
Polymorphism in Go is achieved through interface types. Any type that implements the methods defined in an interface is considered to satisfy that interface. This enables substitutability, where different concrete types can be used interchangeably as long as they satisfy the required interface.
Using interfaces promotes decoupling between components, as the components only depend on the interfaces they interact with rather than concrete implementations. This allows for better code extensibility, testability, and maintainability.
Here’s an example:
type Writer interface {
Write(data []byte) (int, error)
}
func Process(w Writer) {
// ...
w.Write(data)
// ...
}
Code language: PHP (php)
In the example, the Process
function operates on any type that satisfies the Writer
interface. This allows different types, such as a file writer or a network writer, to be passed to the function without modifying the function’s code.
Question:
Explain the concept of channels in Go. How can channels be used to facilitate communication and synchronization between Goroutines?
Answer:
In Go, channels are a core language feature used for communication and synchronization between Goroutines. They provide a way for Goroutines to send and receive values concurrently, ensuring safe communication and coordination.
A channel is created using the make()
function with the chan
keyword and a specified element type. Channels can be unbuffered or buffered. Unbuffered channels have no capacity and require both the sender and receiver to be ready to communicate at the same time. Buffered channels have a specific capacity and allow a certain number of values to be sent without requiring an immediate receiver.
Channels use the arrow operator <-
for sending and receiving values. The sender uses <-
to send a value to the channel, while the receiver uses <-
to receive a value from the channel. These operations block until both the sender and receiver are ready to communicate.
Channels can be used to coordinate Goroutines, synchronize concurrent operations, and safely pass data between Goroutines without the need for explicit locking mechanisms. They promote the Go concurrency model of “Do not communicate by sharing memory; instead, share memory by communicating.”
Here’s an example:
func worker(id int, jobs <-chan int, results chan<- int) {
for job := range jobs {
// Perform some work with job
result := process(job)
// Send result to results channel
results <- result
}
}
func main() {
jobs := make(chan int)
results := make(chan int)
// Spawn worker Goroutines
for i := 0; i < numWorkers; i++ {
go worker(i, jobs, results)
}
// Send jobs to the jobs channel
for _, job := range jobList {
jobs <- job
}
// Close the jobs channel to signal completion
close(jobs)
// Receive results from the results channel
for i := 0; i < len(jobList); i++ {
result := <-results
// Process the result
}
}
Code language: PHP (php)
In the example, the main Goroutine sends jobs to the jobs
channel, and the worker Goroutines receive jobs from the same channel. The worker Goroutines process the jobs and send the results to the results
channel. Finally, the main Goroutine receives the results from the results
channel. This enables concurrent job processing and proper synchronization between the Goroutines.
Question:
Identify and fix the issue in the following Go code snippet that is causing a data race:
package main
import (
"fmt"
"sync"
)
func main() {
counter := 0
var wg sync.WaitGroup
for i := 0; i < 10; i++ {
wg.Add(1)
go func() {
counter++
wg.Done()
}()
}
wg.Wait()
fmt.Println("Counter:", counter)
}
Code language: JavaScript (javascript)
Answer:
The issue in the code snippet is that multiple Goroutines are concurrently accessing and modifying the counter
variable without proper synchronization, leading to a data race.
To fix the issue, you can use a mutex to protect the access to the counter
variable and ensure exclusive access from Goroutines. The sync.Mutex
type from the sync
package can be used for this purpose.
Here’s the fixed code:
package main
import (
"fmt"
"sync"
)
func main() {
counter := 0
var wg sync.WaitGroup
var mu sync.Mutex
for i := 0; i < 10; i++ {
wg.Add(1)
go func() {
mu.Lock()
counter++
mu.Unlock()
wg.Done()
}()
}
wg.Wait()
fmt.Println("Counter:", counter)
}
Code language: JavaScript (javascript)
In the fixed code, a sync.Mutex
named mu
is introduced to protect the critical section where the counter
variable is modified. The mu.Lock()
call ensures exclusive access, and mu.Unlock()
releases the lock after the modification is done. This guarantees that only one Goroutine can modify the counter
variable at a time, preventing data races.
Senior Golang interview questions
Question:
What is the purpose of a Goroutine Pool in Go, and how can it be used to manage concurrent tasks efficiently?
Answer:
A Goroutine Pool in Go is a mechanism for managing a fixed number of Goroutines (lightweight threads) to execute tasks concurrently. The purpose of a Goroutine Pool is to limit the number of Goroutines created to avoid overwhelming system resources and provide better control over concurrency.
By using a Goroutine Pool, you can reuse Goroutines instead of creating and destroying them for each task, which reduces the overhead of Goroutine creation and improves performance. It allows you to control the number of concurrent tasks being executed at any given time, preventing resource exhaustion.
To implement a Goroutine Pool, you can utilize a buffered channel to hold the tasks and a fixed number of Goroutines that consume tasks from the channel. This ensures that only a limited number of Goroutines are actively running, while the rest are waiting for new tasks.
By managing the Goroutine Pool, you can efficiently control concurrency, limit resource usage, and prevent bottlenecks in highly concurrent applications.
Question:
Explain the concept of context in Go. How can you use context to handle timeouts and cancellations in long-running operations?
Answer:
In Go, a context provides control over the lifecycle of a Goroutine and allows the propagation of cancellation signals and deadlines across Goroutines. It enables structured and safe termination of Goroutines and helps manage resources and handle timeouts.
A context is created using the context.Background()
or context.TODO()
functions, which serve as the root of a context tree. A context can be passed down to child Goroutines to ensure they share the same context and cancellation mechanism.
Timeouts and cancellations can be handled using contexts by utilizing the context.WithTimeout()
and context.WithCancel()
functions. context.WithTimeout()
creates a new context with an associated deadline, and the Goroutine using this context can detect when the deadline is exceeded. context.WithCancel()
creates a new context with a cancellation function that can be used to signal cancellation to Goroutines.
To handle timeouts, you can wrap the long-running operation in a Goroutine and monitor the context’s Done()
channel. If the context is canceled or the deadline is exceeded, the Done()
channel will be closed, indicating a timeout. You can then gracefully exit the Goroutine or perform any necessary cleanup.
Cancellations can be handled similarly. When cancellation is needed, call the cancellation function associated with the context. This will close the Done()
channel, signaling cancellation to all Goroutines using that context. Goroutines can check the Done()
channel and exit gracefully when cancellation is received.
By utilizing contexts, you can propagate cancellation signals and deadlines across Goroutines, ensuring proper cleanup and termination, and effectively handle timeouts in long-running operations.
Question:
The following Go code snippet is intended to read a CSV file and print its contents. However, it is not working as expected. Identify the issue and provide the corrected code:
func main() {
file, _ := os.Open("data.csv")
defer file.Close()
reader := csv.NewReader(file)
records, _ := reader.ReadAll()
for _, record := range records {
fmt.Println(record)
}
}
Code language: JavaScript (javascript)
Answer:
The issue in the code is that the errors returned by os.Open()
and reader.ReadAll()
are not being handled. It is important to handle errors to ensure proper error checking and handling. Here’s the corrected code:
func main() {
file, err := os.Open("data.csv")
if err != nil {
log.Fatal(err)
}
defer file.Close()
reader := csv.NewReader(file)
records, err := reader.ReadAll()
if err != nil {
log.Fatal(err)
}
for _, record := range records {
fmt.Println(record)
}
}
Code language: JavaScript (javascript)
In the corrected code, we check and handle the errors returned by os.Open()
and reader.ReadAll()
using the err
variable. If an error occurs, we log the error and exit the program using log.Fatal()
.
Question:
What are the benefits of using a message broker in a distributed system architecture? Can you recommend any popular message broker systems compatible with Go-based applications?
Answer:
Message brokers play a crucial role in distributed system architectures by enabling reliable communication and decoupling of components. Some benefits of using a message broker are:
- Asynchronous Communication: Message brokers facilitate asynchronous communication between different components of a distributed system. Producers can publish messages to the broker, and consumers can receive and process those messages at their own pace, promoting loose coupling and flexibility.
- Reliability: Message brokers provide durable storage for messages, ensuring that messages are not lost even if a consumer is temporarily unavailable. This reliability enables fault tolerance and guarantees message delivery.
- Scalability: Message brokers act as intermediaries, allowing the system to scale horizontally by adding more consumers or producers without directly impacting the other components. This decoupling enables better resource utilization and load balancing.
- Message Routing: Message brokers offer advanced routing mechanisms, such as topic-based or content-based routing, allowing messages to be selectively delivered to specific consumers based on predefined rules. This flexibility in routing helps build complex communication patterns.
- Protocol Transformation: Message brokers often support multiple communication protocols, allowing components built with different technologies and languages to communicate seamlessly. This interoperability is crucial in heterogeneous distributed systems.
For Go-based applications, some popular message broker systems that are compatible include:
- RabbitMQ: A feature-rich and widely-used message broker with support for various protocols and features like message queuing, routing, and topic-based messaging.
- Apache Kafka: A distributed streaming platform that provides high-throughput, fault-tolerant, and scalable messaging for real-time data streams.
- NATS: A lightweight and high-performance messaging system that offers publish-subscribe and request-reply patterns.
- ActiveMQ: An open-source message broker that supports the Java Message Service (JMS) API and multiple protocols.
- Redis Pub/Sub: A simple and fast messaging system provided by Redis, which supports publish-subscribe messaging patterns.
These message brokers offer robust solutions for building distributed systems and have extensive community support and integration libraries available for Go-based applications.
Question:
The following Go code snippet is intended to make a secure HTTPS request using a custom TLS configuration. However, it is not working as expected. Identify the issue and provide the corrected code:
func main() {
url := "https://api.example.com"
transport := http.Transport{
TLSClientConfig: &tls.Config{
InsecureSkipVerify: true,
},
}
client := http.Client{
Transport: &transport,
}
response, _ := client.Get(url)
defer response.Body.Close()
body, _ := ioutil.ReadAll(response.Body)
fmt.Println(string(body))
}
Code language: JavaScript (javascript)
Answer:
The issue in the code is that the http.Transport
struct should be passed as a pointer to the http.Client
struct. Here’s the corrected code:
func main() {
url := "https://api.example.com"
transport := &http.Transport{
TLSClientConfig: &tls.Config{
InsecureSkipVerify: true,
},
}
client := &http.Client{
Transport: transport,
}
response, err := client.Get(url)
if err != nil {
log.Fatal(err)
}
defer response.Body.Close()
body, err := ioutil.ReadAll(response.Body)
if err != nil {
log.Fatal(err)
}
fmt.Println(string(body))
}
Code language: JavaScript (javascript)
In the corrected code, we define transport
and client
as pointers to http.Transport
and http.Client
structs, respectively. This ensures that the modifications made to the transport
are reflected in the client
.
We also handle the errors returned by client.Get()
and ioutil.ReadAll()
using the err
variable. If an error occurs, we log the error and exit the program using log.Fatal()
.
Question:
Explain the concept of connection pooling in database systems. How can you leverage connection pooling in a Go-based application that interacts with a database?
Answer:
Connection pooling is a technique used in database systems to efficiently manage and reuse database connections. It helps reduce the overhead of establishing a new connection for each database operation and improves the performance of applications interacting with databases.
In a typical scenario without connection pooling, for every database operation, an application needs to establish a new connection, authenticate with the database server, and perform the operation. This process incurs significant overhead in terms of time and resources.
Connection pooling addresses this issue by creating a pool of pre-established database connections that are ready to be used by the application. When a database operation is required, the application borrows a connection from the pool, performs the operation, and returns the connection back to the pool for reuse.
By reusing existing connections, the application avoids the need to establish new connections for each operation. This eliminates the overhead of authentication and reduces the time required to complete each database operation. Additionally, connection pooling ensures that the number of active connections to the database is limited, preventing resource exhaustion and improving scalability.
In a Go-based application, you can leverage connection pooling using libraries such as “database/sql” and the respective database driver. The “database/sql” package provides an interface to work with databases, and most database drivers in Go already support connection pooling.
To use connection pooling, you typically set a maximum limit for the number of connections in the pool. When the application initializes, it establishes a number of connections up to the maximum limit and adds them to the pool. When a database operation is needed, the application requests a connection from the pool using the db.Acquire()
or db.Get()
method. After the operation, the connection is released back to the pool using the conn.Release()
or conn.Close()
method.
By utilizing connection pooling, Go-based applications can efficiently manage and reuse database connections, resulting in improved performance and resource utilization.
Question:
The following Go code snippet is intended to send an email using an SMTP server. However, it is not working as expected. Identify the issue and provide the corrected code:
func main() {
from := "[email protected]"
to := "[email protected]"
subject := "Hello"
body := "This is the email body."
message := fmt.Sprintf("From: %srnTo: %srnSubject: %srnrn%s", from, to, subject, body)
smtp.SendMail("smtp.example.com:587", nil, from, []string{to}, []byte(message))
}
Code language: CSS (css)
Answer:
The issue in the code is that the smtp.SendMail()
function requires an authentication mechanism to be specified. Additionally, error handling is missing in the code. Here’s the corrected code:
func main() {
from := "[email protected]"
password := "password" // The password for the email account
to := "[email protected]"
subject := "Hello"
body := "This is the email body."
message := fmt.Sprintf("From: %srnTo: %srnSubject: %srnrn%s", from, to, subject, body)
auth := smtp.PlainAuth("", from, password, "smtp.example.com")
err := smtp.SendMail("smtp.example.com:587", auth, from, []string{to}, []byte(message))
if err != nil {
log.Fatal(err)
}
}
Code language: CSS (css)
In the corrected code, we provide the email account’s password and create an authentication mechanism using smtp.PlainAuth()
. The password is passed as the second argument to smtp.PlainAuth()
. Additionally, we handle the error returned by smtp.SendMail()
using the err
variable. If an error occurs, we log the error and exit the program using log.Fatal()
.
Question:
Explain the concept of caching and its importance in web application development. Can you recommend any popular caching systems or libraries compatible with Go-based applications?
Answer:
Caching is the process of storing frequently accessed or computed data in a temporary storage location called a cache. In web application development, caching plays a crucial role in improving performance, reducing response times, and minimizing the load on backend systems.
When a request is made to a web application, the application can check if the requested data or the result of a computation is available in the cache. If it is, the application can retrieve the data from the cache instead of recalculating or fetching it from the original data source. This significantly reduces the time and resources required to serve the request, resulting in faster response times and improved user experience.
Caching is important in web application development for several reasons:
- Performance: Caching allows frequently accessed data or computation results to be served quickly from a cache, eliminating the need for expensive operations on each request. This improves overall application performance and responsiveness.
- Scalability: By reducing the load on backend systems, caching helps improve the scalability of web applications. Caching can handle a significant portion of read-heavy workloads, allowing backend resources to be used for more computationally intensive tasks.
- Reduced Latency: Caching brings data closer to the application or the user, reducing network latency. By minimizing the round trips to external data sources, caching enables faster data retrieval and improves overall system responsiveness.
- Resource Optimization: Caching reduces the workload on backend systems, such as databases or external APIs, by serving cached data. This optimizes resource utilization and prevents unnecessary strain on those systems.
For Go-based applications, some popular caching systems or libraries compatible with Go include:
- Redis: An in-memory data store that supports various caching patterns, such as key-value caching, distributed caching, and caching with expiration policies.
- Memcached: A high-performance, distributed memory caching system that provides a simple key-value interface for caching frequently accessed data.
- BigCache: A concurrent, memory-efficient cache library for Go that supports automatic cache eviction and data sharding.
- Groupcache: A caching library specifically designed for efficient caching in a distributed environment, with features like consistent hashing and automatic cache population.
These caching systems and libraries can be integrated into Go-based applications to implement caching strategies and improve performance and scalability.
Question:
The following Go code snippet is intended to read environment variables and print their values. However, it is not working as expected. Identify the issue and provide the corrected code:
func main() {
apiKey := os.Getenv("API_KEY
")
apiSecret := os.Getenv("API_SECRET")
authToken := os.Getenv("AUTH_TOKEN")
fmt.Println("API Key:", apiKey)
fmt.Println("API Secret:", apiSecret)
fmt.Println("Auth Token:", authToken)
}
Code language: PHP (php)
Answer:
The issue in the code is that the environment variables are not being properly set. Here’s the corrected code:
func main() {
err := godotenv.Load()
if err != nil {
log.Fatal("Error loading .env file")
}
apiKey := os.Getenv("API_KEY")
apiSecret := os.Getenv("API_SECRET")
authToken := os.Getenv("AUTH_TOKEN")
fmt.Println("API Key:", apiKey)
fmt.Println("API Secret:", apiSecret)
fmt.Println("Auth Token:", authToken)
}
Code language: JavaScript (javascript)
In the corrected code, we use the godotenv
package to load the environment variables from a .env
file. The godotenv.Load()
function is called to load the variables, and we handle any errors that occur during the loading process using the err
variable. If an error occurs, we log a fatal error message using log.Fatal()
.
After loading the environment variables, we retrieve their values using os.Getenv()
as before and print them to the console.
Question:
Explain the concept of goroutines in Go and how they differ from traditional threads. When and why would you use goroutines in your Go-based application?
Answer:
Goroutines are a fundamental concurrency feature in Go that allow concurrent execution of lightweight, independently scheduled functions or methods. They provide a simple and efficient way to achieve concurrency and parallelism in Go-based applications.
Unlike traditional threads, which are managed by the operating system’s kernel, goroutines are managed by the Go runtime. Goroutines are lightweight and have a small memory footprint, allowing thousands or even millions of goroutines to be created and executed concurrently without significant overhead.
Key differences between goroutines and traditional threads are:
- Cost: Creating and managing goroutines is much cheaper compared to traditional threads. Goroutines have minimal memory overhead and can be created and destroyed quickly. In contrast, threads typically require more memory and involve more expensive context switching.
- Concurrency Model: Goroutines utilize a concurrent programming model based on channels for communication and synchronization. This model promotes a simpler and safer approach to concurrent programming compared to managing shared memory and locks used in traditional thread-based programming.
- Scheduling: Goroutines are managed by the Go runtime’s scheduler, which multiplexes goroutines onto a smaller number of operating system threads. The scheduler uses techniques like preemption and work-stealing to efficiently schedule goroutines, ensuring optimal CPU utilization.
- Communication: Goroutines communicate through channels, which are built-in Go types that enable safe communication and synchronization between concurrent goroutines. Channels provide a powerful mechanism for passing data between goroutines without the need for explicit locking or coordination.
In Go-based applications, goroutines are used when concurrency is required to achieve parallelism or handle asynchronous tasks. Here are some common scenarios where goroutines are useful:
- Concurrent Processing: When performing CPU-bound tasks, such as heavy computations or data processing, goroutines can be used to parallelize the workload and utilize multiple CPU cores effectively.
- Asynchronous I/O: Goroutines are well-suited for handling I/O-bound tasks, such as network requests or file operations. By using goroutines, the application can asynchronously perform I/O operations without blocking the execution of other goroutines.
- Event-driven Programming: Goroutines can be used to handle event-driven programming models, such as handling incoming requests in a web server or processing messages from a message queue. Each request or message can be handled in its own goroutine, enabling high concurrency and responsiveness.
- Parallelizing Independent Tasks: When there are multiple independent tasks that can be executed concurrently, goroutines can be used to divide the workload and execute the tasks in parallel. This can lead to improved performance and reduced overall execution time.
By leveraging goroutines, Go-based applications can achieve high concurrency, parallelism, and responsiveness while maintaining a simple and efficient programming model.
1,000 Companies use CoderPad to Screen and Interview Developers
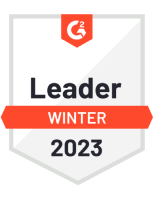
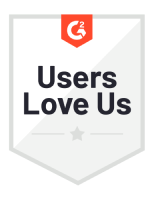
Best interview practices for Go roles
For successful Go interviews, it’s crucial to take into account various factors such as the candidate’s background and the specific engineering role. To guarantee a productive interview experience, we advise implementing these best practices:
- Craft technical questions that represent real-world business scenarios within your organization. This method will effectively engage the candidate and help assess their compatibility with your team.
- Foster a collaborative atmosphere by allowing candidates to ask questions throughout the interview.
- If you’re using Go as part of full-stack development, also ensure the candidate has a decent understanding of HTML and CSS.
Additionally, adhering to well-established interview procedures is vital when conducting Go interviews. This includes tailoring the complexity of the questions to the candidate’s skills, promptly updating them on their application status, and offering them a chance to inquire about the evaluation process and collaboration with you and your team.