F# Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified F# developers for your organization.
F#
F# has gained popularity in recent years due to its unique combination of functional programming and object-oriented programming features, its concise and expressive syntax, and its integration with the .NET ecosystem.
F# was created by Don Syme at Microsoft Research in the early 2000s. It was initially designed as a .NET-compatible functional programming language with the goal of simplifying complex programming tasks and improving developer productivity. F# has since evolved into a general-purpose language and is now part of the .NET ecosystem.
https://learn.microsoft.com/en-us/dotnet/fsharp/
In order to evaluate the expertise of F# developers during programming interviews, we provide a collection of hands-on coding exercises and interview questions outlined below. Additionally, we have developed a range of suggested methods to ensure that your interview inquiries accurately measure the candidates’ F# capabilities.
Table of Contents
F# example question
Help us design a parking lot app
Hey candidate! Welcome to your interview. Boilerplate is provided. Feel free to change the code as you see fit. To run the code at any time, please hit the run button located in the top left corner.
Goals: Design a parking lot using object-oriented principles
Here are a few methods that you should be able to run:
- Tell us how many spots are remaining
- Tell us how many total spots are in the parking lot
- Tell us when the parking lot is full
- Tell us when the parking lot is empty
- Tell us when certain spots are full e.g. when all motorcycle spots are taken
- Tell us how many spots vans are taking up
Assumptions:
- The parking lot can hold motorcycles, cars and vans
- The parking lot has motorcycle spots, car spots and large spots
- A motorcycle can park in any spot
- A car can park in a single compact spot, or a regular spot
- A van can park, but it will take up 3 regular spots
- These are just a few assumptions. Feel free to ask your interviewer about more assumptions as needed
F# skills to assess
Junior F# interview questions
Question: How can you define a record type in F#?
Answer: In F#, a record type is defined using the type
keyword followed by the record name and a set of fields enclosed in curly braces. For example:
type Person = { Name: string; Age: int }
Question: What is pattern matching in F# and how is it used?
Answer: Pattern matching is a powerful feature in F# that allows you to match the structure of data and perform different actions based on the patterns. It is often used with the match
keyword. For example:
let rec factorial n =
match n with
| 0 -> 1
| _ -> n * factorial (n - 1)
Code language: JavaScript (javascript)
Question: How can you define an immutable list in F#?
Answer: In F#, an immutable list can be defined using square brackets and semicolons to separate the elements. For example:
let myList = [1; 2; 3]
Code language: JavaScript (javascript)
Question: What is the purpose of the async
keyword in F#?
Answer: The async
keyword is used in F# to define asynchronous computations. It allows you to write code that can perform I/O or long-running operations without blocking the execution. The result of an asynchronous computation is typically represented by the Async<'T>
type. Here’s an example:
let asyncExample = async {
// Asynchronous code here
}
Code language: JavaScript (javascript)
Question: How can you handle exceptions in F#?
Answer: Exceptions in F# can be handled using the try/with
expression. The code that may raise an exception is enclosed in the try
block, and specific exception handlers are defined in the with
block. For example:
try
// Code that may raise an exception
with
| ex -> printfn "An exception occurred: %s" ex.Message
Code language: JavaScript (javascript)
Question: What is currying in F# and how is it useful?
Answer: Currying in F# is the technique of transforming a function that takes multiple arguments into a sequence of functions, each taking a single argument. This allows partial application of functions and makes it easier to create specialized versions of functions. Here’s an example:
let add x y = x + y
let addCurried = add 5
Code language: JavaScript (javascript)
Question: How do you define a discriminated union type in F#?
Answer: In F#, a discriminated union type is defined using the type
keyword followed by the union name and a set of cases separated by the pipe |
symbol. Each case can optionally have associated data. For example:
type Shape =
| Circle of float
| Rectangle of float * float
Question: How can you define a recursive function in F#?
Answer: In F#, a recursive function is defined using the let rec
keyword instead of just let
. This allows the function to refer to itself within its body. For example:
let rec factorial n =
if n <= 1 then 1
else n * factorial (n - 1)
Code language: JavaScript (javascript)
Question: How do you define an option type in F#?
Answer: In F#, the option type is defined using the Option<'T>
generic type. It represents a value that can either be Some
value of type 'T
or None
. For example:
let optionalValue : Option<int> = Some 42
Code language: HTML, XML (xml)
Question: What is partial function application in F# and how is it achieved?
Answer: Partial function application in F# allows you to create a new function by supplying some, but not all, of the arguments to an existing function. This can be achieved using the _
(underscore) placeholder for the arguments you want to partially apply. For example:
let multiply x y = x * y
let double = multiply 2
Code language: JavaScript (javascript)
In the above code, multiply
is a function that takes two arguments. By applying 2
to multiply
, we create a new function double
that multiplies its argument by 2
.
Intermediate F# interview questions
Question: How can you define a higher-order function in F#?
Answer:
let applyTwice f x = f (f x)
Code language: JavaScript (javascript)
Question: What are the benefits of using immutability in F#?
Answer: Immutable data promotes a functional programming style and offers benefits such as improved code clarity, better testability, easier concurrency and parallelism, and fewer bugs related to shared mutable state.
Question: How can you handle asynchronous operations in F#?
Answer:
let asyncOperation = async {
// Asynchronous code here
return result
}
let result = Async.RunSynchronously asyncOperation
Code language: JavaScript (javascript)
Question: What is the purpose of the Seq
module in F#?
Answer: The Seq
module provides functions for working with sequences (lazy lists) in F#. It offers operations like mapping, filtering, folding, and more. It enables efficient processing of large or infinite sequences.
Question: How can you implement memoization in F#?
Answer:
let memoize f =
let cache = System.Collections.Generic.Dictionary<_, _>()
fun x ->
if cache.ContainsKey(x) then
cache.[x]
else
let result = f x
cache.[x] <- result
result
Code language: JavaScript (javascript)
Question: How do you define an interface in F#?
Answer:
type IShape =
abstract member Area : float
abstract member Perimeter : float
Code language: PHP (php)
Question: How can you use F# computation expressions to work with custom types?
Answer: F# computation expressions, also known as workflows, allow you to define custom control flow for your types. By implementing specific computation builders, you can define specialized syntax and behavior for your types, making them easier to work with in specific domains.
Question: What is tail recursion optimization, and why is it important in functional programming?
Answer: Tail recursion optimization is a compiler optimization technique that eliminates unnecessary stack frames in recursive function calls. It prevents stack overflow errors and improves the performance of recursive functions, making them more efficient in functional programming where recursion is often used instead of loops.
Question: How can you write unit tests for F# code?
Answer: F# supports various unit testing frameworks such as NUnit, xUnit, and FsUnit. You can write test cases using frameworks like these, creating test functions that exercise your code and assert the expected behavior.
Question: How can you handle errors and propagate them in a functional way in F#?
Answer: In F#, you can handle errors using the Result<'T, 'Error>
type or the Option<'T>
type. You can propagate errors using match expressions or functions like Result.map
, Result.bind
, Option.map
, and Option.bind
. This functional error handling approach helps ensure code correctness and enables composability.
Senior F# interview questions
Question: Explain the concept of function composition in F# and provide an example. Also, fix the code snippet below to compose the functions addOne
and multiplyByTwo
to transform the input value.
let addOne x = x + 1
let multiplyByTwo x = x * 2
let result = addOne multiplyByTwo 3
Code language: JavaScript (javascript)
Answer:
Function composition in F# is the act of chaining multiple functions together to create a new function. It is achieved using the >>
operator. Here’s an example of fixing the code snippet:
let addOne x = x + 1
let multiplyByTwo x = x * 2
let composedFunction = addOne >> multiplyByTwo
let result = composedFunction 3
Code language: JavaScript (javascript)
Question: Discuss the advantages and disadvantages of using F# as a language for developing web applications. Additionally, fix the code snippet below that demonstrates a simple F# web API handler.
open System
open Microsoft.AspNetCore.Mvc
[<Route("api/hello")>]
type HelloController() =
inherit Controller()
[<HttpGet>]
member this.Get() =
"Hello, World!"
Code language: HTML, XML (xml)
Answer:
F# offers benefits like succinct syntax, immutability by default, and strong type inference, making it well-suited for web development. However, its ecosystem may have fewer resources and libraries compared to more mainstream languages. Here’s the fixed code snippet:
open System
open Microsoft.AspNetCore.Mvc
[<Route("api/hello")>]
type HelloController() =
inherit Controller()
[<HttpGet>]
member this.Get() : IActionResult =
this.Ok("Hello, World!")
Code language: HTML, XML (xml)
Question: Explain the concept of type providers in F#. Provide an example of using a type provider to work with external data sources. Additionally, fix the code snippet below that demonstrates loading CSV data using a type provider.
open FSharp.Data
type MyCsv = CsvProvider<"data.csv">
let data = MyCsv.Load("data.csv").Data
Code language: JavaScript (javascript)
Answer:
Type providers in F# are a powerful feature that enable the compiler to generate types based on external data sources. They provide compile-time checking and IntelliSense support. Here’s the fixed code snippet:
open FSharp.Data
type MyCsv = CsvProvider<"data.csv">
let data = MyCsv.Load("data.csv").Rows
Code language: JavaScript (javascript)
Question: Discuss the concept of active patterns in F# and provide an example of using an active pattern to match against different shapes. Additionally, fix the code snippet below that demonstrates using active patterns.
let (|Square|_|) x = if x % 2 = 0 then Some x else None
let (|Circle|_|) x = if x % 2 = 1 then Some x else None
let printShape shape =
match shape with
| Square x -> printfn "Square: %d" x
| Circle x -> printfn "Circle: %d" x
| _ -> printfn "Unknown shape"
Code language: JavaScript (javascript)
Answer:
Active patterns in F# allow custom pattern matching on values. Here’s the fixed code snippet:
let (|Square|_|) x = if x % 2 = 0 then Some x else None
let (|Circle|_|) x = if x % 2 = 1 then Some x else None
let printShape shape =
match shape with
| Square x -> printfn
"Square: %d" x
| Circle x -> printfn "Circle: %d" x
| _ -> printfn "Unknown shape"
let shape = 4
printShape (Square shape)
Code language: JavaScript (javascript)
Question: Explain the concept of computation expressions (workflows) in F#. Provide an example of creating a custom computation expression for error handling. Additionally, fix the code snippet below that demonstrates using a computation expression for error handling.
type ResultBuilder() =
member this.Bind(result, func) =
match result with
| Ok value -> func value
| Error err -> Error err
member this.Return(value) = Ok value
let result = result {
let! x = Ok 10
let! y = Ok 5
return x / y
}
Code language: JavaScript (javascript)
Answer:
Computation expressions, also known as workflows, provide a way to define custom control flow in F#. They enable concise and expressive syntax for working with monadic types. Here’s the fixed code snippet:
type ResultBuilder() =
member this.Bind(result, func) =
match result with
| Ok value -> func value
| Error err -> Error err
member this.Return(value) = Ok value
let result = ResultBuilder()
let computation =
result {
let! x = Ok 10
let! y = Ok 5
return x / y
}
Code language: JavaScript (javascript)
Question: Discuss the concept of agents in F# and their role in concurrent programming. Provide an example of using an agent to implement a concurrent task. Additionally, fix the code snippet below that demonstrates using an agent.
open System.Threading
let agent = MailboxProcessor.Start(fun inbox ->
let rec loop () =
async {
let! message = inbox.Receive()
printfn "Received: %s" message
return! loop ()
}
loop ())
agent.Post("Hello, World!")
Code language: JavaScript (javascript)
Answer:
Agents in F# provide a high-level concurrency model for building concurrent programs. They encapsulate state and allow communication between multiple threads. Here’s the fixed code snippet:
open System.Threading
let agent = MailboxProcessor.Start(fun inbox ->
let rec loop () =
async {
let! message = inbox.Receive()
printfn "Received: %s" message
return! loop ()
}
loop ())
agent.Post("Hello, World!") |> ignore
Code language: JavaScript (javascript)
Question: Explain the concept of type providers for accessing databases in F#. Provide an example of using a type provider to interact with a SQL database. Additionally, fix the code snippet below that demonstrates using a type provider for database access.
open FSharp.Data.Sql
type Db = SqlDataProvider<ConnectionString = "Server=localhost;Database=mydb;User Id=myuser;Password=mypassword", DatabaseVendor = Common.DatabaseProviderTypes.POSTGRESQL>
let ctx = Db.GetDataContext()
let query =
query {
for row in ctx.Table do
select row
}
Code language: JavaScript (javascript)
Answer:
Type providers in F# can be used to access databases by providing compile-time checking and IntelliSense support. Here’s the fixed code snippet:
open FSharp.Data.Sql
type Db = SqlDataProvider<ConnectionString = "Server=localhost;Database=mydb;User Id=myuser;Password=mypassword", DatabaseVendor = Common.DatabaseProviderTypes.POSTGRESQL>
let ctx = Db.GetDataContext()
let query =
query {
for row in ctx.Dbo.MyTable do
select row
}
Code language: JavaScript (javascript)
Question: Discuss the concept of monads in functional programming and their significance in F#. Provide an example of using the Option
monad for handling nullable values. Additionally, fix the code snippet below that demonstrates using the Option
monad.
let divide x y =
if y = 0 then 1
else Some (x / y)
let result =
divide 10 2
|> Option.map (fun x -> x + 1)
Code language: JavaScript (javascript)
Answer:
Monads are a fundamental concept in functional programming and provide a way to structure computations. They encapsulate values and their associated operations. Here’s the fixed code snippet:
let divide x y =
if y = 0 then None
else Some (x / y)
let result =
divide 10 2
|> Option.map (fun x -> x + 1)
Code language: JavaScript (javascript)
Question: Explain the concept of currying in F# and its benefits. Provide an example of a curried function and demonstrate its usage. Additionally, fix the code snippet below that demonstrates currying.
let add x y z = x + y + z
let curriedAdd = add 1 2
let result = curriedAdd 3
Code language: JavaScript (javascript)
Answer:
Currying in F# is the technique of transforming a function with multiple arguments into a series of functions, each taking one argument. It allows for partial application and enhances composability. Here’s the fixed code snippet:
let add x y z = x + y + z
let curriedAdd = add 1 2
let result = curriedAdd 3
Code language: JavaScript (javascript)
1,000 Companies use CoderPad to Screen and Interview Developers
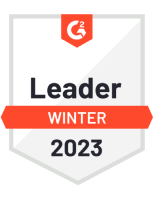
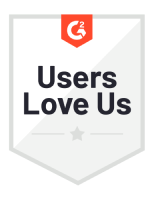
Interview best practices for F# roles
During effective F# interviews, it’s important to consider various factors, such as the candidates’ experience levels and the specific engineering role they are applying for. To ensure your F# interview questions produce the best results, we recommend following these best practices while engaging with candidates:
- Develop technical questions that mirror real-life scenarios within your organization. This approach not only keeps the candidate engaged but also enables you to more precisely evaluate their fit for your team.
- Encourage a cooperative atmosphere by allowing candidates to ask questions throughout the interview.
- Because of F#’s strong relationship to the .NET framework, candidates should also have an understanding of common .NET libraries.
Moreover, it’s essential to adhere to standard interview practices when carrying out F# interviews. This includes adjusting question difficulty based on the candidate’s skill level, providing timely feedback on their application status, and enabling candidates to ask about the assessment or collaborate with you and your team.