Erlang Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Erlang developers for your organization.
Erlang
A solid language that features hot code swapping, fault tolerance, and functional programming, Erlang is a popular choice in the telecommunications and IoT industries.
Erlang was developed in the late 1980s by Ericsson, a Swedish telecommunications company, to address the need for a programming language that could handle massive concurrency, distributed systems, and fault tolerance. Joe Armstrong, Robert Virding, and Mike Williams were the key developers behind the language.
http://www.erlang.org/
To assess the proficiency of Erlang developers during coding interviews, we offer an assortment of practical coding tasks and interview queries listed below. Furthermore, we have devised a variety of recommended techniques to guarantee that your interview questions effectively gauge the candidates’ Erlang competencies.
Table of Contents
Erlang example question
Help us design a parking lot app
Hey candidate! Welcome to your interview. Boilerplate is provided. Feel free to change the code as you see fit. To run the code at any time, please hit the run button located in the top left corner.
Goals: Design a parking lot using object-oriented principles
Here are a few methods that you should be able to run:
- Tell us how many spots are remaining
- Tell us how many total spots are in the parking lot
- Tell us when the parking lot is full
- Tell us when the parking lot is empty
- Tell us when certain spots are full e.g. when all motorcycle spots are taken
- Tell us how many spots vans are taking up
Assumptions:
- The parking lot can hold motorcycles, cars and vans
- The parking lot has motorcycle spots, car spots and large spots
- A motorcycle can park in any spot
- A car can park in a single compact spot, or a regular spot
- A van can park, but it will take up 3 regular spots
- These are just a few assumptions. Feel free to ask your interviewer about more assumptions as needed
Erlang skills to assess
Jobs using Erlang
Junior Erlang interview questions
Question:
The following Erlang code is intended to calculate the factorial of a given number but has a bug. Identify and correct the error.
-module(factorial).
-export([factorial/1]).
factorial(0) -> 1;
factorial(N) -> N * factorial(N - 1).
Code language: JavaScript (javascript)
Answer:
The code has a missing base case for the factorial function. When N
is 0, the code should return 1 to terminate the recursive calls. The corrected code is as follows:
-module(factorial).
-export([factorial/1]).
factorial(0) -> 1;
factorial(N) when N > 0 -> N * factorial(N - 1).
Code language: JavaScript (javascript)
Question:
What is tail recursion in Erlang? How does it differ from general recursion?
Answer:
In Erlang, tail recursion is a form of recursion where the recursive call is the last operation performed in a function. In general recursion, the recursive call is followed by additional computation or operations.
Tail recursion is particularly useful because it allows the Erlang virtual machine to optimize the code by reusing the same stack frame for each recursive call, avoiding stack overflow errors and improving performance. This optimization is known as tail call optimization (TCO).
Question:
The following Erlang code attempts to concatenate two lists but contains a mistake. Identify and correct the error.
-module(concatenate).
-export([concat/2]).
concat([], L) -> L;
concat([L|T], H) -> [H|concat(T, L)].
Code language: CSS (css)
Answer:
The code has the arguments in the wrong order when making the recursive call. The corrected code is as follows:
-module(concatenate).
-export([concat/2]).
concat([], L) -> L;
concat([H|T], L) -> [H|concat(T, L)].
Code language: CSS (css)
Question:
What is a process in Erlang? How does it differ from an operating system process?
Answer:
In Erlang, a process is a lightweight concurrent execution unit. Processes in Erlang are not the same as operating system processes. Erlang processes are created and managed within the Erlang runtime system and are highly efficient in terms of memory consumption and context switching.
Erlang processes have their own memory space, stack, and message queues. They communicate with each other by sending and receiving messages. Erlang processes are preemptively scheduled by the runtime system, allowing for efficient concurrency and fault-tolerant distributed systems.
Question:
The following Erlang code is intended to find the maximum element in a list but contains a bug. Identify and correct the error.
-module(maximum).
-export([find_max/1]).
find_max([H|T]) -> find_max(H, T).
find_max(Max, []) -> Max;
find_max(Max, [H|T]) when H > Max -> find_max(H, T);
find_max(Max, [_|T]) -> find_max(Max, T).
Code language: CSS (css)
Answer:
The code has a logical error in the last clause of the find_max/3
function. When comparing the current element H
with the maximum Max
, the greater value should be assigned to Max
instead of recursively calling find_max/3
. The corrected code is as follows:
-module(maximum).
-export([find_max/1]).
find_max([H|T]) -> find_max(H, T).
find_max(Max, []) -> Max;
find_max(Max, [H|T]) when H > Max -> find_max
(H, T);
find_max(Max, [_|T]) -> find_max(Max, T).
Code language: CSS (css)
Question:
What is pattern matching in Erlang? How is it used in function clauses?
Answer:
Pattern matching is a fundamental concept in Erlang that allows values to be matched against patterns. In function clauses, pattern matching is used to select the appropriate clause to execute based on the input arguments.
When a function is called, Erlang sequentially checks the clauses of the function in order. It tries to match the arguments provided with the patterns defined in each clause from top to bottom. When a matching pattern is found, the corresponding clause is executed, and its expressions are evaluated.
Pattern matching enables Erlang to handle different cases and execute different code paths based on the shape or contents of the data being processed.
Question:
The following Erlang code attempts to reverse a list but contains a mistake. Identify and correct the error.
-module(reverse).
-export([reverse_list/1]).
reverse_list([]) -> [];
reverse_list([H|T]) -> reverse_list(T), [H].
Code language: CSS (css)
Answer:
The code has a mistake in the second clause of the reverse_list/1
function. The recursive call reverse_list(T)
is not capturing the reversed list correctly. The corrected code is as follows:
-module(reverse).
-export([reverse_list/1]).
reverse_list([]) -> [];
reverse_list([H|T]) -> lists:append(reverse_list(T), [H]).
Code language: CSS (css)
Question:
What are OTP behaviors in Erlang? Give examples of some common OTP behaviors.
Answer:
OTP (Open Telecom Platform) behaviors in Erlang are reusable frameworks that provide high-level abstractions for building concurrent and fault-tolerant systems. These behaviors encapsulate common patterns and best practices for building reliable distributed applications.
Some common OTP behaviors in Erlang include:
gen_server
: Provides a client-server abstraction with synchronous and asynchronous message passing.gen_fsm
: Implements a finite state machine (FSM) behavior with states and state transitions.gen_event
: Enables event handling and dispatching among multiple event handlers.supervisor
: Manages the lifecycle of worker processes and restarts them in case of failures.application
: Facilitates the management and supervision of a collection of related processes.
OTP behaviors simplify the development of concurrent and distributed systems by providing a standard structure and functionality for common use cases.
Question:
The following Erlang code attempts to calculate the sum of a list of integers but contains an error. Identify and correct the mistake.
-module(sum).
-export([calculate_sum/1]).
calculate_sum([]) -> 0;
calculate_sum([H|T]) -> H + calculate_sum(T).
Code language: CSS (css)
Answer:
The code has a mistake in the second clause of the calculate_sum/1
function. The recursive call calculate_sum(T)
is missing the addition of the head element H
. The corrected code is as follows:
-module(sum).
-export([calculate_sum/1]).
calculate_sum([]) -> 0;
calculate_sum([H|T]) -> H + calculate_sum(T).
Code language: CSS (css)
Question:
What is hot code swapping in Erlang? How does it contribute to system availability?
Answer:
Hot code swapping is a feature in Erlang that allows the replacement of code in a running system without stopping or restarting it. It enables updates or bug fixes to be deployed to live systems without downtime.
In Erlang, hot code swapping is made possible by the isolation of processes and their independent memory spaces. New versions of code can be loaded into a running system, and processes can migrate to the new code version seamlessly during runtime.
Hot code swapping contributes to system availability by minimizing downtime and allowing for continuous operation. It is particularly valuable in systems where uninterrupted service is critical, such as telecommunication infrastructure and distributed applications.
Intermediate Erlang interview questions
Question:
The following Erlang code aims to read the contents of a file and return them as a list of lines. However, there is an error in the code. Identify and correct the mistake.
-module(file_reader).
-export([read_file/1]).
read_file(FilePath) ->
{ok, File} = file:open(FilePath, [read]),
Lines = read_lines(File, []),
file:close(File),
Lines.
read_lines(File, Acc) ->
case io:get_line(File, "") of
eof -> Acc;
Line -> read_lines(File, [Line | Acc])
end.
Code language: JavaScript (javascript)
Answer:
The code has an issue with the order of lines in the resulting list. Since the code is appending new lines at the beginning of the accumulator list, the lines will appear in reverse order. To correct this, we can either reverse the resulting list or append lines at the end. Here’s the corrected code with lines appended at the end:
-module(file_reader).
-export([read_file/1]).
read_file(FilePath) ->
{ok, File} = file:open(FilePath, [read]),
Lines = lists:reverse(read_lines(File, [])),
file:close(File),
Lines.
read_lines(File, Acc) ->
case io:get_line(File, "") of
eof -> Acc;
Line -> read_lines(File, Acc ++ [Line])
end.
Code language: JavaScript (javascript)
Question:
What is the purpose of a parse transform in Erlang? Give an example of a commonly used parse transform.
Answer:
A parse transform in Erlang is a compile-time mechanism that allows developers to intercept and modify the abstract syntax tree (AST) of Erlang source code before it gets compiled into bytecode. It enables code transformations and extensions beyond what is supported by the language itself.
A commonly used parse transform in Erlang is the lager
parse transform, which is part of the Lager logging framework. The lager
parse transform modifies the AST of functions to automatically add logging statements, simplifying the process of adding logging to Erlang applications.
Question:
The following Erlang code attempts to calculate the Fibonacci sequence but contains an error. Identify and correct the mistake.
-module(fibonacci).
-export([fib/1]).
fib(0) -> 0;
fib(1) -> 1;
fib(N) -> fib(N - 1) + fib(N - 2).
Code language: JavaScript (javascript)
Answer:
The code has a performance issue due to redundant recursive calls. When calculating the Fibonacci sequence, the current implementation recalculates the same values multiple times, leading to exponential time complexity. We can optimize it by using tail recursion and introducing accumulator variables to store the intermediate results. Here’s the corrected code:
-module(fibonacci).
-export([fib/1]).
fib(N) -> fib(N, 0, 1).
fib(0, Acc1, _) -> Acc1;
fib(N, Acc1, Acc2) -> fib(N - 1, Acc2, Acc1 + Acc2).
Code language: JavaScript (javascript)
Question:
What are ETS tables in Erlang? How are they different from regular Erlang data structures?
Answer:
ETS (Erlang Term Storage) tables are an in-memory key-value store provided by the Erlang runtime system. ETS tables offer fast and efficient access to shared data across Erlang processes.
ETS tables differ from regular Erl
ang data structures in a few ways:
- ETS tables are shared among multiple processes, allowing for data exchange and synchronization.
- ETS tables can hold large amounts of data without impacting garbage collection, as they are stored separately from the process heaps.
- ETS tables support different access patterns and concurrency control mechanisms (e.g., read/write locks, transactions) to handle concurrent access from multiple processes efficiently.
ETS tables are commonly used for caching, process communication, and managing shared data in Erlang applications.
Question:
The following Erlang code attempts to perform element-wise addition on two lists but contains a mistake. Identify and correct the error.
-module(list_addition).
-export([add_lists/2]).
add_lists([], []) -> [];
add_lists([H1|T1], [H2|T2]) -> [H1 + H2 | add_lists(T1, T2)].
Code language: CSS (css)
Answer:
The code has an issue with handling lists of unequal lengths. When one list becomes empty, but the other list still has elements, the code will throw a badmatch exception. To fix this, we need to add a clause to handle such cases gracefully. Here’s the corrected code:
-module(list_addition).
-export([add_lists/2]).
add_lists([], []) -> [];
add_lists([H1|T1], [H2|T2]) -> [H1 + H2 | add_lists(T1, T2)];
add_lists(_, _) -> error:badarg.
Code language: CSS (css)
The added clause matches any other combination of input lists and returns an error tuple to indicate an invalid argument.
Question:
What is a gen_server in Erlang? Explain its role and typical usage.
Answer:
A gen_server
is an OTP behavior in Erlang that provides a framework for building client-server-style concurrent processes. It serves as a generic server implementation with message-based communication and a predefined behavior.
The gen_server
behavior abstracts away common patterns for server processes, including initialization, message handling, state management, and termination. By implementing a gen_server
, developers can focus on the specific business logic of their server application without worrying about the underlying infrastructure.
Typically, a gen_server
is used when building long-running server processes, such as database connectors, communication protocols, or other services requiring stateful interactions. It offers benefits such as built-in error handling, asynchronous and synchronous message handling, and supervision capabilities provided by OTP.
Question:
The following Erlang code aims to filter out odd numbers from a list but contains an error. Identify and correct the mistake.
-module(filter_odd).
-export([filter_odd/1]).
filter_odd([]) -> [];
filter_odd([H|T]) when H rem 2 == 1 -> [H | filter_odd(T)];
filter_odd([_|T]) -> filter_odd(T).
Code language: JavaScript (javascript)
Answer:
The code has a mistake in the second clause of the filter_odd/1
function. When the head element H
is an odd number, it should be included in the resulting list. However, the code uses the equality operator ==
instead of the not-equal operator /=
. Here’s the corrected code:
-module(filter_odd).
-export([filter_odd/1]).
filter_odd([]) -> [];
filter_odd([H|T]) when H rem 2 /= 0 -> [H | filter_odd(T)];
filter_odd([_|T]) -> filter_odd(T).
Code language: JavaScript (javascript)
Question:
What is a supervision tree in Erlang? How does it contribute to building fault-tolerant systems?
Answer:
A supervision tree is a hierarchical structure
of processes in Erlang that is managed by a supervisor process. It is a key component of building fault-tolerant systems in Erlang/OTP.
In a supervision tree, processes are organized into a parent-child relationship, where each child process is supervised by its parent. If a child process terminates abnormally, the supervisor can take appropriate actions, such as restarting the process, terminating other related processes, or performing any necessary recovery actions.
Supervision trees contribute to building fault-tolerant systems by providing automatic process monitoring, failure detection, and recovery mechanisms. When an error occurs, supervisors can quickly restart failed processes, isolate failures, and maintain system availability.
Question:
The following Erlang code attempts to capitalize the first letter of each word in a sentence but contains an error. Identify and correct the mistake.
-module(capitalize_words).
-export([capitalize/1]).
capitalize(Sentence) ->
Words = string:tokens(Sentence, " "),
[capitalize_word(Word) || Word <- Words].
capitalize_word(Word) ->
[string:to_upper(FirstChar) | Rest] = Word,
[FirstChar | Rest].
Code language: JavaScript (javascript)
Answer:
The code has an issue with handling empty words in the capitalize_word/1
function. When encountering an empty word, such as a double space between words, the code will throw a badmatch exception. To fix this, we can add a guard condition to check if the word is non-empty before performing the pattern matching. Here’s the corrected code:
-module(capitalize_words).
-export([capitalize/1]).
capitalize(Sentence) ->
Words = string:tokens(Sentence, " "),
[capitalize_word(Word) || Word <- Words].
capitalize_word(Word) when is_list(Word), Word /= [] ->
[string:to_upper(FirstChar) | Rest] = Word,
[FirstChar | Rest];
capitalize_word(Word) -> Word.
Code language: JavaScript (javascript)
The added guard condition ensures that the word is a non-empty list before performing pattern matching.
Question:
What is OTP release handling in Erlang? Explain its purpose and benefits.
Answer:
OTP release handling in Erlang refers to the process of bundling and deploying a complete Erlang/OTP application along with its dependencies as a self-contained unit. It involves packaging the application’s code, configuration, and necessary runtime components for easy deployment and system upgrades.
The purpose of OTP release handling is to simplify the deployment, version management, and upgrade processes of Erlang/OTP applications. It allows for consistent and controlled releases of applications in production environments, ensuring minimal disruption and easy rollback if needed.
Benefits of OTP release handling include:
- Atomic upgrades: An OTP release is deployed as a single unit, enabling atomic upgrades without impacting running processes.
- Version management: Releases allow for managing different versions of an application and its dependencies, facilitating side-by-side installations and gradual upgrades.
- Configuration management: OTP releases include configuration files, making it easier to manage application-specific settings across different environments.
- System reliability: The self-contained nature of releases reduces dependencies on the development environment, making the system more resilient and reproducible.
OTP release handling is often used in production deployments of Erlang/OTP applications, particularly in cases where high availability, system reliability, and seamless upgrades are required.
Senior Erlang interview questions
Certainly! Here are 10 detailed questions containing equal parts of fix-the-code and theoretical questions and answers for a senior Erlang developer who has experience in building toolings:
Question:
The following Erlang code attempts to sort a list of integers in ascending order but contains an error. Identify and correct the mistake.
-module(sorting).
-export([sort_list/1]).
sort_list([]) -> [];
sort_list([Pivot|T]) ->
Smaller = [X || X <- T, X < Pivot],
Greater = [Y || Y <- T, Y >= Pivot],
sort_list(Smaller) ++ [Pivot] ++ sort_list(Greater).
Code language: JavaScript (javascript)
Answer:
The code has an issue with the comparison operators used in the list comprehensions. The condition X < Pivot
should be changed to X =< Pivot
in order to correctly handle duplicate elements. Similarly, Y >= Pivot
should be changed to Y > Pivot
. Here’s the corrected code:
-module(sorting).
-export([sort_list/1]).
sort_list([]) -> [];
sort_list([Pivot|T]) ->
Smaller = [X || X <- T, X =< Pivot],
Greater = [Y || Y <- T, Y > Pivot],
sort_list(Smaller) ++ [Pivot] ++ sort_list(Greater).
Code language: JavaScript (javascript)
Question:
What is the purpose of parse transforms in Erlang? How can they be used to extend the language?
Answer:
Parse transforms in Erlang allow developers to intercept and modify the abstract syntax tree (AST) of Erlang source code during compilation. They provide a mechanism to extend the language by enabling custom code transformations and introducing new syntax or constructs.
Parse transforms can be used for various purposes, including:
- Source code transformations: Parse transforms can be used to automate repetitive code transformations, enforce coding conventions, or generate boilerplate code.
- Domain-specific language (DSL) creation: Developers can define their own DSLs by implementing parse transforms that translate custom syntax into Erlang constructs.
- Aspect-oriented programming: Parse transforms can introduce cross-cutting concerns, such as logging or tracing, into existing code without modifying the original source files.
By leveraging parse transforms, Erlang developers can extend the language’s capabilities and tailor it to specific requirements.
Question:
The following Erlang code aims to calculate the factorial of a number but contains an error. Identify and correct the mistake.
-module(factorial).
-export([factorial/1]).
factorial(N) when N > 0 -> N * factorial(N - 1);
factorial(0) -> 1.
Code language: JavaScript (javascript)
Answer:
The code has a mistake in the first clause of the factorial/1
function. The recursive call factorial(N - 1)
should be guarded with the condition N > 0
to ensure termination when negative numbers are passed as arguments. Here’s the corrected code:
-module(factorial).
-export([factorial/1]).
factorial(N) when N > 0 -> N * factorial(N - 1);
factorial(0) -> 1;
factorial(_) -> error:badarg.
Code language: JavaScript (javascript)
The added clause matches any other argument and returns an error tuple to indicate an invalid argument.
Question:
What is an Erlang port? How can ports be used to interface with external systems?
Answer:
In Erlang, a port is a communication mechanism that allows Erlang processes to interact with external programs or systems. Ports enable bidirectional communication, allowing Erlang to send messages to external programs and receive messages back.
Ports can be used to interface with external systems in several ways:
- Interfacing with C or C++ code: Ports can be used to execute and communicate with native code written in C or C++ by creating a port program that interacts with the Erlang runtime via standard input and output.
- Integrating with existing applications: Ports can enable Erlang processes to communicate with existing applications or services by establishing a communication protocol or message format that both sides can understand.
- Calling external executables: Erlang can use ports to invoke external executables or command-line tools and capture their output for further processing.
By utilizing ports, Erlang developers can seamlessly integrate their applications with external systems and leverage the capabilities of other programming languages or existing software.
Question:
The following Erlang code attempts to calculate the sum of a list of numbers but contains an error. Identify and correct the mistake.
-module(summation).
-export([sum/1]).
sum([]) -> 0;
sum([H|T]) -> H + sum(T).
Code language: CSS (css)
Answer:
The code has a performance issue due to the use of the +
operator inside the recursion. The +
operator has a linear time complexity, resulting in quadratic time complexity for summing a long list. To optimize the code, we can use tail recursion and introduce an accumulator variable to store the running sum. Here’s the corrected code:
-module(summation).
-export([sum/1]).
sum(List) -> sum(List, 0).
sum([], Acc) -> Acc;
sum([H|T], Acc) -> sum(T, Acc + H).
Code language: CSS (css)
The accumulator variable Acc
accumulates the sum as the list is traversed, resulting in linear time complexity.
Question:
What are the advantages of using property-based testing in Erlang? How does it differ from example-based testing?
Answer:
Property-based testing is a testing methodology that focuses on generating and verifying properties or invariants of the system under test. It offers several advantages over example-based testing:
- Test coverage: Property-based testing generates a wide range of test cases automatically, providing better coverage of possible input variations and edge cases. This helps uncover corner cases that may not be apparent when writing individual example-based tests.
- Scalability: Property-based tests are easily scalable as they generate test cases based on properties or constraints. By specifying the desired properties, developers can generate an infinite number of test cases, ensuring comprehensive testing without the need to enumerate specific examples.
- Code documentation: Property-based tests serve as executable specifications of the system’s behavior. By explicitly defining the expected properties, they provide clear documentation and ensure that the intended behavior is properly tested.
- Bug detection: Property-based testing often uncovers subtle bugs and edge cases that example-based testing might miss. By randomly generating test cases, property-based testing can expose hidden errors and edge case failures that may have been overlooked in example-based tests.
- Test maintainability: Property-based tests are concise and require less maintenance compared to example-based tests. Changing the properties or constraints can easily result in new test cases being generated, reducing the need for manual test case modifications.
In summary, property-based testing in Erlang offers improved test coverage, scalability, code documentation, bug detection, and test maintainability compared to example-based testing.
Question:
The following Erlang code attempts to count the number of occurrences of each element in a list but contains an error. Identify and correct the mistake.
-module(element_counter).
-export([count_elements/1]).
count_elements(List) ->
count_elements(List, dict:new()).
count_elements([], Result) -> Result;
count_elements([H|
T], Result) ->
count_elements(T, dict:update_counter(H, 1, Result)).
Code language: JavaScript (javascript)
Answer:
The code has a mistake in the second clause of the count_elements/2
function. The function dict:update_counter/3
should be used to increment the counter for an existing key in the dictionary. However, the code incorrectly uses dict:update_counter/2
, which throws an exception when the key is not present. Here’s the corrected code:
-module(element_counter).
-export([count_elements/1]).
count_elements(List) ->
count_elements(List, dict:new()).
count_elements([], Result) -> Result;
count_elements([H|T], Result) ->
count_elements(T, dict:update_counter(H, 1, Result, {H, 0})).
Code language: JavaScript (javascript)
By providing a default value {H, 0}
as the fourth argument to dict:update_counter/4
, we ensure that a counter is initialized to 0 for any new element encountered.
Question:
What is hot code swapping in Erlang? Explain its significance in achieving zero-downtime upgrades.
Answer:
Hot code swapping is a unique feature of Erlang that allows the replacement of code in a running system without stopping or restarting the application. It enables zero-downtime upgrades, where new code can be introduced seamlessly while the system continues to process requests.
Significance of hot code swapping in achieving zero-downtime upgrades:
- Continuous availability: Hot code swapping ensures that critical systems remain available during the upgrade process, minimizing downtime and disruptions in service.
- Error correction: Hot code swapping enables quick fixes and error corrections in a running system without interrupting its operation. Bugs or issues can be addressed and patched in real-time, ensuring smooth and reliable system behavior.
- Feature enhancements: Hot code swapping allows for the introduction of new features and functionality in a live system. New code can be deployed incrementally, enabling continuous delivery and enabling agile development practices.
- Fault tolerance: Hot code swapping works seamlessly with Erlang’s built-in fault-tolerant features, such as supervision trees. If the new code introduces errors or crashes, supervisors can automatically handle the failures and roll back to the previous version of the code, ensuring system stability.
Hot code swapping is a powerful tool that empowers Erlang developers to upgrade systems smoothly and dynamically, without disrupting critical operations or compromising system reliability.
Question:
The following Erlang code attempts to find the maximum element in a list but contains an error. Identify and correct the mistake.
-module(maximum).
-export([find_maximum/1]).
find_maximum([H|T]) -> find_maximum(T, H).
find_maximum([], Max) -> Max;
find_maximum([H|T], Max) when H > Max -> find_maximum(T, H);
find_maximum([_|T], Max) -> find_maximum(T, Max).
Code language: CSS (css)
Answer:
The code has an issue with the second clause of the find_maximum/2
function. When the current element H
is greater than the maximum Max
, the code should update the maximum value to H
. However, the code incorrectly continues using the previous maximum value. Here’s the corrected code:
-module(maximum).
-export([find_maximum/1]).
find_maximum([H|T]) -> find_maximum(T, H).
find_maximum([], Max) -> Max;
find_maximum([H|T], Max) when H > Max -> find_maximum(T, H);
find_maximum([_|T], Max) -> find_maximum(T, Max).
Code language: CSS (css)
By updating the recursive call to find_maximum(T, H)
, we ensure that the maximum value is correctly updated when a
larger element is found.
Question:
Explain the concept of distributed Erlang and its benefits in building scalable and fault-tolerant systems.
Answer:
Distributed Erlang is a feature of the Erlang runtime system that allows multiple Erlang nodes to communicate and collaborate in a networked environment. It enables the distribution of processes across multiple machines, facilitating the development of scalable and fault-tolerant systems.
Benefits of distributed Erlang in building scalable and fault-tolerant systems:
- Scalability: Distributed Erlang enables the distribution of processes across multiple nodes, allowing applications to scale horizontally by adding more computing resources. It enables systems to handle increased workloads and leverage the collective processing power of multiple machines.
- Fault tolerance: Distributed Erlang provides built-in fault tolerance mechanisms, such as process monitoring and supervision trees, that work across networked nodes. If a node or process fails, supervisors can detect the failure and take appropriate actions, such as restarting processes or redistributing work to other nodes.
- Location transparency: Distributed Erlang abstracts the underlying network communication details, allowing processes to communicate seamlessly regardless of their physical location. This enables developers to write distributed applications without explicit knowledge of network addresses or communication protocols.
- Transparent process interaction: Distributed Erlang provides a transparent mechanism for process interaction across nodes. Processes can send messages to each other as if they were on the same node, hiding the complexities of network communication.
- Code mobility: Distributed Erlang supports the movement of code across nodes, allowing system upgrades or code changes to be seamlessly propagated without interrupting the operation of the distributed system.
By leveraging distributed Erlang, developers can build highly scalable and fault-tolerant systems that can handle large workloads, distribute processing across multiple machines, and recover from failures with minimal disruption.
1,000 Companies use CoderPad to Screen and Interview Developers
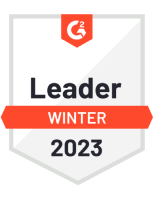
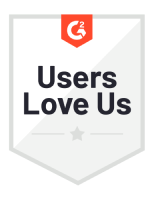
Interview best practices for Erlang roles
When conducting efficient Erlang interviews, it’s essential to take into account various aspects, such as the candidates’ experience levels and the specific engineering role they are pursuing. To make certain your Erlang interview questions generate optimal outcomes, we suggest adhering to these best practices during interactions with candidates:
- Formulate technical questions that reflect real-world situations within your organization. This method not only engages the candidate but also allows you to more accurately assess their suitability for your team.
- Foster a collaborative environment by permitting candidates to ask questions throughout the interview.
- Candidates should also have knowledge of designing and implementing distributed systems via nodes and global process registries.
Furthermore, it’s crucial to observe standard interview practices when conducting Erlang interviews. This involves tailoring question difficulty according to the candidate’s expertise, offering prompt feedback on their application status, and allowing candidates to inquire about the evaluation or collaborate with you and your team.