Elixir Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Elixir developers for your organization.
Elixir
This Erlang-based language is noted for its fault tolerance capabilities, its efficient scalability and concurrency, and its ease of use due to its expressive and concise syntax.
Elixir was created by José Valim, a Brazilian software engineer, in 2011. His goal was to develop a language that combined the strengths of the Erlang/OTP platform with a more modern and expressive syntax, improving developer productivity and making it more accessible.
https://changelog.com/podcast/179
In order to assess the proficiency of developers in Elixir during programming interviews, we offer a collection of practical coding tasks and interview queries below.
Moreover, we’ve devised a serie of recommended approaches to guarantee that your interview questions effectively gauge the candidates’ Elixir abilities.
Table of Contents
Elixir example question
Help us design a parking lot app
Hey candidate! Welcome to your interview. Boilerplate is provided. Feel free to change the code as you see fit. To run the code at any time, please hit the run button located in the top left corner.
Goals: Design a parking lot using object-oriented principles
Here are a few methods that you should be able to run:
- Tell us how many spots are remaining
- Tell us how many total spots are in the parking lot
- Tell us when the parking lot is full
- Tell us when the parking lot is empty
- Tell us when certain spots are full e.g. when all motorcycle spots are taken
- Tell us how many spots vans are taking up
Assumptions:
- The parking lot can hold motorcycles, cars and vans
- The parking lot has motorcycle spots, car spots and large spots
- A motorcycle can park in any spot
- A car can park in a single compact spot, or a regular spot
- A van can park, but it will take up 3 regular spots
- These are just a few assumptions. Feel free to ask your interviewer about more assumptions as needed
Elixir skills to assess
Jobs using Elixir
Junior Elixir interview questions
Question: Fix the code below:
defmodule MathFunctions do
def multiply(a, b) do
a + b
end
end
IO.puts(MathFunctions.multiply(3, 4)) # Expected output: 12
Code language: Elixir (elixir)
Answer:
defmodule MathFunctions do
def multiply(a, b) do
a * b
end
end
IO.puts(MathFunctions.multiply(3, 4)) # Output: 12
Code language: Elixir (elixir)
Question:
What is immutability in Elixir, and why is it important?
Answer:
In Elixir, immutability means that once a value is assigned to a variable, it cannot be changed. Instead, new values are created based on existing ones. Immutability is important because it guarantees that data remains consistent and avoids unexpected side effects. It enables safer concurrent programming and helps reason about code.
Question: Fix the code:
defmodule ListFunctions do
def subtract_one(list) do
Enum.map(list, &(&1 + 1))
end
end
IO.inspect(ListFunctions.subtract_one([1, 2, 3])) # Expected output: [0, 1, 2]
Code language: CSS (css)
Answer:
defmodule ListFunctions do
def subtract_one(list) do
Enum.map(list, &(&1 - 1))
end
end
IO.inspect(ListFunctions.subtract_one([1, 2, 3])) # Output: [0, 1, 2]
Code language: CSS (css)
Question:
What are OTP behaviors in Elixir, and how do they facilitate building concurrent systems?
Answer:
OTP (Open Telecom Platform) behaviors are higher-level abstractions provided by the OTP framework. They encapsulate common patterns for building concurrent and fault-tolerant systems. OTP behaviors, such as GenServer
and Supervisor
, provide ready-to-use implementations that handle message passing, state management, and supervision. They simplify the development of concurrent systems and ensure consistency and fault tolerance.
Question: Fix the code below:
defmodule StringFunctions do
def capitalize(string) do
string |> String.upcase()
end
end
IO.puts(StringFunctions.capitalize("elixir")) # Expected output: "Elixir"
Code language: PHP (php)
Answer:
defmodule StringFunctions do
def capitalize(string) do
string |> String.capitalize()
end
end
IO.puts(StringFunctions.capitalize("elixir")) # Output: "Elixir"
Code language: PHP (php)
Question:
What are Elixir processes, and how do they enable concurrency?
Answer:
Elixir processes are lightweight, isolated units of execution that run concurrently. They are managed by the Erlang VM and communicate with each other using message passing. Elixir processes allow programs to execute tasks concurrently, improving performance and responsiveness. They enable the development of highly concurrent systems by providing a scalable and fault-tolerant concurrency model.
Question: Fix the code below:
defmodule MathFunctions do
def subtract(a, b) do
a + b
end
end
IO.puts(MathFunctions.subtract(5, 3)) # Expected output: 2
Code language: PHP (php)
Answer:
defmodule MathFunctions do
def subtract(a, b) do
a - b
end
end
IO.puts(MathFunctions.subtract(5, 3)) # Output: 2
Code language: PHP (php)
Question:
What is the purpose of GenServer in Elixir, and how does it handle state and message passing?
Answer:
GenServer is a behavior in OTP that provides a client-server model for managing state and handling message passing. It allows developers to build concurrent, stateful processes that can receive and reply to messages. GenServer abstracts away the complexities of managing state and provides a standard interface for handling asynchronous requests and maintaining state consistency.
Question: Fix the code:
defmodule MathFunctions do
def divide(a, b) do
a / b
end
end
IO.puts(MathFunctions.divide(10, 2)) # Expected output: 5
Code language: PHP (php)
Answer:
defmodule MathFunctions do
def divide(a, b) do
div(a, b)
end
end
IO.puts(MathFunctions.divide(10, 2)) # Output: 5
Code language: PHP (php)
Question:
What is pattern matching in Elixir, and how is it used in function clauses?
Answer:
Pattern matching in Elixir allows matching against different patterns and executing the corresponding code block. In function clauses, pattern matching is used to define multiple function heads with different patterns of arguments. Elixir matches the input against the defined function clauses and invokes the one that matches. This enables polymorphism and the handling of different cases based on input patterns.
Intermediate Elixir interview questions
Question: How else can the function sum_list
below be rewritten?
defmodule MathFunctions do
def sum_list(list) do
Enum.reduce(list, 0, fn(x, acc) -> acc + x end)
end
end
IO.puts(MathFunctions.sum_list([1, 2, 3, 4])) # Expected output: 10
Code language: PHP (php)
Answer:
defmodule MathFunctions do
def sum_list(list) do
Enum.reduce(list, 0, &(&1 + &2))
end
end
IO.puts(MathFunctions.sum_list([1, 2, 3, 4])) # Output: 10
Code language: PHP (php)
Question:
Explain the concept of a GenStage in Elixir and how it can be used for backpressure in data processing pipelines.
Answer:
GenStage is a specification and set of behaviors in Elixir that allows building data processing pipelines with built-in backpressure support. It facilitates the flow of data between producers and consumers, ensuring that the rate of data production matches the consumption rate. Producers and consumers communicate through demand-driven protocols, enabling efficient and controlled data processing in systems that require flow control.
Question:
defmodule StringFunctions do
def split_string(string, separator) do
String.split(separator, string)
end
end
IO.inspect(StringFunctions.split_string("Hello, World!", ",")) # Expected output: ["Hello", " World!"]
Code language: CSS (css)
Answer:
defmodule StringFunctions do
def split_string(string, separator) do
String.split(string, separator)
end
end
IO.inspect(StringFunctions.split_string("Hello, World!", ",")) # Output: ["Hello", " World!"]
Code language: CSS (css)
Question:
What are the advantages of using ETS (Erlang Term Storage) tables in Elixir? Provide an example use case.
Answer:
ETS tables provide a way to store large amounts of data in-memory, accessible by multiple processes. The advantages of using ETS tables in Elixir include fast read and write operations, concurrent access, and reduced garbage collection overhead. An example use case is caching frequently accessed data, such as configuration settings or database query results, to improve performance and reduce external dependencies.
Question:
defmodule MathFunctions do
def calculate_average(list) do
sum = Enum.sum(list)
length = Enum.count(list)
sum / length + 1
end
end
IO.puts(MathFunctions.calculate_average([1, 2, 3, 4])) # Expected output: 2.5
Code language: PHP (php)
Answer:
defmodule MathFunctions do
def calculate_average(list) do
sum = Enum.sum(list)
length = length(list)
sum / length
end
end
IO.puts(MathFunctions.calculate_average([1, 2, 3, 4])) # Output: 2.5
Code language: PHP (php)
Question:
Explain the concept of fault tolerance in Elixir and how it is achieved through supervision trees.
Answer:
Fault tolerance in Elixir refers to the ability of a system to recover from failures and continue operating. It is achieved through supervision trees, where processes are organized hierarchically under supervisors. Supervisors monitor their child processes and handle failures by restarting or terminating them. By using supervision trees, Elixir applications can automatically recover from errors and maintain system stability.
Question:
defmodule Counter do
defstruct count: 0
def increment(counter) do
%{counter | count: counter.count + 1}
end
end
counter = %Counter{}
counter = Counter.increment(counter)
IO.inspect(counter.count) # Expected output: 1
Code language: PHP (php)
Answer:
defmodule Counter do
defstruct count: 0
def increment(counter) do
%{counter | count: counter.count + 1}
end
end
counter = %Counter{}
counter = Counter.increment(counter)
IO.inspect(counter.count) # Output: 1
Code language: PHP (php)
Question:
What is the purpose of OTP applications in Elixir, and how are they structured?
Answer:
OTP (Open Telecom Platform) applications in Elixir are a way to package and manage reusable components of an Elixir system. They provide a structured way to organize code, configuration, and dependencies. OTP applications consist of a mix.exs file specifying dependencies and metadata, lib directory containing the application’s modules, and other optional directories for configuration, documentation, and tests. OTP applications can be easily started, stopped, and managed using tools like mix and releases.
Question:
defmodule StringFunctions do
defp is_palindrome?(string) do
string == String.reverse(string)
end
end
IO.puts(StringFunctions.is_palindrome?("racecar")) # Expected output: true
Code language: PHP (php)
Answer:
defmodule StringFunctions do
def is_palindrome?(string) do
string == String.reverse(string)
end
end
IO.puts(StringFunctions.is_palindrome?("racecar")) # Output: true
Code language: PHP (php)
Question:
What is the role of the BEAM (Erlang virtual machine) in Elixir, and how does it contribute to Elixir’s scalability and fault tolerance?
Answer:
The BEAM is the virtual machine on which Elixir runs, inheriting its characteristics from Erlang. It provides features like lightweight processes, preemptive scheduling, and message passing. These features enable Elixir to handle massive concurrency, distribute work across multiple cores and machines, and build fault-tolerant systems. The BEAM’s built-in mechanisms for process isolation, error handling, and supervision make it well-suited for building scalable and resilient applications.
Senior Elixir interview questions
Question:
The code snippet below attempts to calculate the sum of a list of numbers. However, when invoking MathFunctions.sum([1, 2, 3, 4, 5])
, it doesn’t produce the expected output. Fix the code to correctly calculate the sum and return the expected output.
defmodule MathFunctions do
def sum(list) do
Enum.reduce(list, 0, &+/2)
end
end
IO.puts(MathFunctions.sum([1, 2, 3, 4, 5])) # Expected output: 15
Code language: PHP (php)
Answer:
defmodule MathFunctions do
def sum(list) do
Enum.sum(list)
end
end
IO.puts(MathFunctions.sum([1, 2, 3, 4, 5])) # Output: 15
Code language: PHP (php)
Question:
Explain the concept of concurrency in Elixir. How does Elixir handle concurrency, and what are the benefits of concurrent programming in Elixir?
Answer:
Concurrency in Elixir refers to the ability to execute multiple computations simultaneously. Elixir handles concurrency through lightweight processes, also known as “actors.” These processes are isolated and communicate with each other through message passing.
Elixir uses the Actor Model, where each process has its own memory and executes independently. Processes communicate by sending and receiving messages, allowing for loose coupling and fault-tolerant systems.
The benefits of concurrent programming in Elixir include:
- Scalability: Elixir’s concurrency model allows developers to easily distribute work across multiple processes and utilize multiple CPU cores. This enables building scalable systems that can handle high loads and efficiently utilize available resources.
- Fault Tolerance: Elixir’s supervision mechanisms and isolated processes provide built-in fault tolerance. If a process fails, it can be restarted by a supervisor without affecting other parts of the system. This makes it easier to build robust and fault-tolerant applications.
- Responsiveness: Concurrency enables handling multiple requests or events simultaneously. By leveraging lightweight processes, Elixir can handle concurrent tasks efficiently, resulting in responsive systems that can handle high throughput and low latency requirements.
Question:
The code snippet below attempts to find the maximum element in a list of numbers. However, when invoking MathFunctions.max([3, 7, 2, 9, 5])
, it doesn’t produce the expected output. Fix the code to correctly find the maximum number and return the expected output.
defmodule MathFunctions do
def max(list) do
Enum.reduce(list, 0, fn(x, acc) -> if x < acc, do: x, else: acc end)
end
end
IO.puts(MathFunctions.max([3, 7, 2, 9, 5])) # Expected output: 9
Code language: PHP (php)
Answer:
defmodule MathFunctions do
def max(list) do
Enum.max(list)
end
end
IO.puts(MathFunctions.max([3, 7, 2, 9, 5])) # Output: 9
Code language: PHP (php)
Question:
What is metaprogramming in Elixir? Explain the concept and the role of macros in Elixir.
Answer:
Metaprogramming in Elixir refers to the ability to write code that generates or manipulates other code at compile-time. Elixir provides macros as a powerful metaprogramming tool.
Macros in Elixir are functions that transform and generate code. They operate on the abstract syntax tree (AST) of the code and are expanded during compilation. Macros are defined using the defmacro
construct and can be invoked using the macro
keyword.
Macros allow developers to define new constructs, domain-specific languages (DSLs), and abstractions specific to their application’s needs. They provide a way to extend the language itself without modifying the compiler.
Question:
The code snippet below attempts to reverse a given string. However, when invoking StringFunctions.reverse("Hello, World!")
, it doesn’t produce the expected output. Fix the code to correctly reverse the string and return the expected output.
defmodule StringFunctions do
def reverse(string) do
Enum.reduce(string, "", fn(x, acc) -> acc <> x end)
end
end
IO.puts(StringFunctions.reverse("Hello, World!")) # Expected output: "!dlroW ,olleH"
Code language: PHP (php)
Answer:
defmodule StringFunctions do
def reverse(string) do
String.reverse(string)
end
end
IO.puts(StringFunctions.reverse("Hello, World!")) # Output: "!dlroW ,olleH"
Code language: PHP (php)
Question:
What is OTP (Open Telecom Platform) in Elixir? Explain its purpose and the key components it provides for building fault-tolerant systems.
Answer:
OTP (Open Telecom Platform) in Elixir is a set of libraries, tools, and design principles that facilitate the building of robust, fault-tolerant, and scalable systems. It is a part of the Erlang ecosystem and extends to Elixir.
The purpose of OTP is to provide abstractions and standardized patterns for building concurrent and distributed applications. OTP promotes the “Let it crash” philosophy, where processes are isolated and allowed to fail while supervisors monitor and handle the failures.
Key components of OTP include:
- Supervisors: Supervisors are responsible for starting, stopping, and restarting processes in a hierarchical manner. They define restart strategies and supervise the overall system’s health.
- GenServers: GenServers are generic servers that encapsulate state, handle requests, and provide a callback interface for message handling. They are used for building stateful components.
- Applications: OTP applications provide a way to organize and manage the codebase. Applications define the structure, dependencies, and configuration of a system.
- Behaviors: OTP behaviors are predefined sets of callbacks that help developers implement common patterns. Examples include GenServer, Supervisor, and Application behaviors.
By leveraging OTP, developers can build fault-tolerant systems with built-in error handling, scalability, and distribution capabilities.
Question:
The code snippet below attempts to filter out even numbers from a given list. However, when invoking ListFunctions.filter_even([1, 2, 3, 4, 5])
, it doesn’t produce the expected output. Fix the code to correctly filter the even numbers and return the expected output.
defmodule ListFunctions do
def filter_even(list) do
Enum.filter(list, fn(x) -> rem(x, 2) == 0 end)
end
end
IO.inspect(ListFunctions.filter_even([1, 2, 3, 4, 5])) # Expected output: [2, 4]
Code language: PHP (php)
Answer:
defmodule ListFunctions do
def filter_even(list) do
Enum.filter(list, &rem(&1, 2) == 0)
end
end
IO.inspect(ListFunctions.filter_even([1, 2, 3, 4, 5])) # Output: [2, 4]
Code language: PHP (php)
Question:
What is OTP (Open Telecom Platform) in Elixir? Explain its purpose and the key components it provides for building fault-tolerant systems.
Answer:
OTP (Open Telecom Platform) in Elixir is a set of libraries, tools, and design principles that facilitate the building of robust, fault-tolerant, and scalable systems. It is a part of the Erlang ecosystem and extends to Elixir.
The purpose of OTP is to provide abstractions and standardized patterns for building concurrent and distributed applications. OTP promotes the “Let it crash” philosophy, where processes are isolated and allowed to fail while supervisors monitor and handle the failures.
Key components of OTP include:
- Supervisors: Supervisors are responsible for starting, stopping, and restarting processes in a hierarchical manner. They define restart strategies and supervise the overall system’s health.
- GenServers: GenServers are generic servers that encapsulate state, handle requests, and provide a callback interface for message handling. They are used for building stateful components.
- Applications: OTP applications provide a way to organize and manage the codebase. Applications define the structure, dependencies, and configuration of a system.
- Behaviors: OTP behaviors are predefined sets of callbacks that help developers implement common patterns. Examples include GenServer, Supervisor, and Application behaviors.
By leveraging OTP, developers can build fault-tolerant systems with built-in error handling, scalability, and distribution capabilities.
Question:
The code snippet below attempts to calculate the average of a list of numbers. However, when invoking MathFunctions.average([1, 2, 3, 4, 5])
, it doesn’t produce the expected output. Fix the code to correctly calculate the average and return the expected output.
defmodule MathFunctions do
def average(list) do
sum = Enum.reduce(list, 0, &+/2)
sum / length(list)
end
end
IO.puts(MathFunctions.average([1, 2, 3, 4, 5])) # Expected output: 3.0
Code language: PHP (php)
Answer:
defmodule MathFunctions do
def average(list) do
sum = Enum.sum(list)
length = length(list)
sum / length
end
end
IO.puts(MathFunctions.average([1, 2, 3, 4, 5])) # Output: 3.0
Code language: PHP (php)
Question:
What is metaprogramming in Elixir? Explain the concept and the role of macros in Elixir.
Answer:
Metaprogramming in Elixir refers to the ability to write code that generates or manipulates other code at compile-time. Elixir provides Macros in Elixir are functions that transform and generate code at compile-time. They operate on the abstract syntax tree (AST) of the code and are expanded during compilation. Macros are defined using the defmacro
construct and can be invoked using the macro
keyword.
Macros allow developers to define new constructs, domain-specific languages (DSLs), and abstractions specific to their application’s needs. They provide a way to extend the language itself without modifying the compiler. Macros enable code generation, code reuse, and domain-specific optimizations.
By using macros, developers can write expressive, concise, and flexible code that adapts to different scenarios and simplifies complex tasks.
1,000 Companies use CoderPad to Screen and Interview Developers
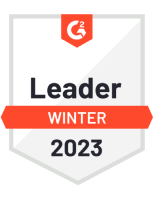
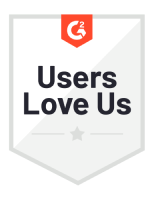
Interview best practices for Elixir roles
To conduct effective Elixir interviews, it’s essential to take into account various factors, such as the candidates’ experience levels and the specific engineering role they’re pursuing. To guarantee that your Elixir interview questions generate optimal outcomes, we suggest following these best practices when engaging with candidates:
- Create technical questions that reflect real-world situations within your organization. This strategy will not only captivate the candidate but also enable you to more accurately assess their suitability for your team.
- Foster a collaborative environment by inviting candidates to ask questions throughout the interview.
- Your candidates should be familiar with concurrency and distributed systems.
Additionally, it’s vital to adhere to conventional interview practices when carrying out Elixir interviews. This involves tailoring question difficulty according to the candidate’s ability, offering prompt feedback on their application status, and permitting candidates to inquire about the evaluation or collaborate with you and your team.