Dart Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Dart developers for your organization.
Dart
This Google-developed language was primarily created for building web, server-side, and mobile applications with an emphasis on performance, ease of use, and scalability.
Dart is a strongly typed language with a sound type system, which helps to catch potential errors during development. It also features automatic memory management through garbage collection, which simplifies memory handling for developers.
https://dart.dev/guides/language
In order to evaluate the skill level of developers in Dart during programming interviews, we provide a set of hands-on coding exercises and interview questions below. Additionally, we have developed a range of suggested methods to ensure that your interview inquiries accurately measure the candidates’ Dart capabilities.
Table of Contents
Dart example question
Help us design a parking lot app
Hey candidate! Welcome to your interview. Boilerplate is provided. Feel free to change the code as you see fit. To run the code at any time, please hit the run button located in the top left corner.
Goals: Design a parking lot using object-oriented principles
Here are a few methods that you should be able to run:
- Tell us how many spots are remaining
- Tell us how many total spots are in the parking lot
- Tell us when the parking lot is full
- Tell us when the parking lot is empty
- Tell us when certain spots are full e.g. when all motorcycle spots are taken
- Tell us how many spots vans are taking up
Assumptions:
- The parking lot can hold motorcycles, cars and vans
- The parking lot has motorcycle spots, car spots and large spots
- A motorcycle can park in any spot
- A car can park in a single compact spot, or a regular spot
- A van can park, but it will take up 3 regular spots
- These are just a few assumptions. Feel free to ask your interviewer about more assumptions as needed
Dart skills to assess
Jobs using Dart
Junior Dart interview questions
Question:
The following Dart code snippet is intended to print the numbers from 1 to 10. However, it is currently not working. Identify and fix the issue.
void main() {
for (var i = 1; i < 11; i++) {
print(i);
}
}
Code language: JavaScript (javascript)
Answer:
The code snippet provided is correct and will print the numbers from 1 to 10. There is no issue with it.
Question:
What is Dart’s main use case, and which platform does it primarily target?
Answer:
Dart is a general-purpose programming language primarily used for building web and mobile applications. It is the language used for developing Flutter applications, which is a UI framework primarily targeting mobile platforms like Android and iOS.
Question:
The following Dart code snippet is supposed to concatenate two strings and print the result. However, it is not producing the expected output. Identify and fix the issue.
void main() {
String firstName = "John";
String lastName = "Doe";
String fullName = firstName + lastName;
print(fullName);
}
Code language: JavaScript (javascript)
Answer:
To concatenate two strings in Dart, you can use the +
operator. However, in the given code snippet, the +
operator is not adding a space between the firstName
and lastName
. To fix this, you can add a space manually between the strings:
void main() {
String firstName = "John";
String lastName = "Doe";
String fullName = firstName + " " + lastName;
print(fullName);
}
Code language: JavaScript (javascript)
Question:
What is the purpose of using the async
and await
keywords in Dart?
Answer:
The async
and await
keywords in Dart are used in asynchronous programming to work with functions that perform potentially time-consuming tasks, such as making network requests or accessing databases. By marking a function as async
, it can use the await
keyword to wait for the completion of other asynchronous operations without blocking the execution. This allows for more efficient and responsive code, especially in UI-driven applications.
Question:
The following Dart code snippet is supposed to calculate the sum of all elements in the numbers
list. However, it is not returning the correct result. Identify and fix the issue.
void main() {
List<int> numbers = [1, 2, 3, 4, 5];
int sum = 0;
for (var i = 0; i <= numbers.length; i++) {
sum += numbers[i];
}
print(sum);
}
Code language: PHP (php)
Answer:
The issue in the code is with the loop condition in the for
loop. The condition should be i < numbers.length
instead of i <= numbers.length
to avoid accessing an index out of range. Here’s the corrected code:
void main() {
List<int> numbers = [1, 2, 3, 4, 5];
int sum = 0;
for (var i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
print(sum);
}
Code language: PHP (php)
Question:
What is the purpose of using the final
keyword in Dart?
Answer:
In Dart, the final
keyword is used to declare a variable whose value cannot be changed once assigned. It is similar to const
, but the value of a final
variable can be determined at runtime. Using final
allows you to ensure that a variable remains constant after its initialization, providing immutability and better performance in some cases.
Question:
The following Dart code snippet is intended to calculate the factorial of a given number. However, it is currently not producing the correct result. Identify and fix the issue.
void main() {
int number = 5;
int factorial = 1;
for (var i = 2; i <= number; i++) {
factorial *= i;
}
print("The factorial of $number is $factorial");
}
Code language: JavaScript (javascript)
Answer:
The code snippet provided correctly calculates the factorial of a given number. There is no issue with it.
Question:
What is the difference between a List
and a Set
in Dart?
Answer:
In Dart, a List
is an ordered collection of elements that allows duplicate values. Elements in a list can be accessed using their index. On the other hand, a Set
is an unordered collection of unique elements. It does not allow duplicate values, and the order of elements is not guaranteed. Sets are useful when you need to ensure uniqueness and perform operations like union, intersection, and difference on collections.
Question:
The following Dart code snippet is intended to check if a given number is even or odd. However, it is not returning the correct result. Identify and fix the issue.
void main() {
int number = 7;
if (number % 2 = 0) {
print("$number is even");
} else {
print("$number is odd");
}
}
Code language: PHP (php)
Answer:
In the given code snippet, there is a mistake in the condition of the if
statement. Instead of using the assignment operator =
, the equality operator ==
should be used. Here’s the corrected code:
void main() {
int number = 7;
if (number % 2 == 0) {
print("$number is even");
} else {
print("$number is odd");
}
}
Code language: PHP (php)
Question:
What are some advantages of using Dart for mobile app development compared to other programming languages?
Answer:
Some advantages of using Dart for mobile app development, particularly with Flutter, include:
- Cross-platform development: Dart allows you to build mobile apps for both Android and iOS using a single codebase, reducing development time and effort.
- Hot Reload: Dart’s Hot Reload feature in Flutter enables developers to see the changes made in the code immediately reflected in the app, allowing for faster iteration and debugging.
- Performance: Dart’s Just-in-Time (JIT) compilation during development and Ahead-of-Time (AOT) compilation during production result in efficient and performant mobile apps.
- Rich UI and Customization: Dart and Flutter provide a rich set of customizable UI widgets, enabling developers to create visually appealing and highly interactive mobile apps.
- Community and Ecosystem: Dart and Flutter have a growing community and ecosystem, with a wide range of packages and libraries available, making it easier to leverage existing solutions for various app requirements.
Intermediate Dart interview questions
Question:
The following Dart code snippet is intended to calculate the sum of two numbers. However, it is not producing the correct result. Identify and fix the issue.
int calculateSum(int a, int b) {
int sum = a + b;
return sum;
}
void main() {
int result = calculateSum(2, 3);
print(result);
}
Code language: JavaScript (javascript)
Answer:
The code is correct. It correctly calculates the sum of two numbers. The output will be 5.
Question:
What is a constructor in Dart? Explain with an example.
Answer:
A constructor in Dart is a special method used for creating objects of a class. It initializes the object’s state when it is created. Dart supports two types of constructors: default constructors and named constructors.
Example:
class Person {
String name;
int age;
Person(this.name, this.age); // Default constructor
Person.fromBirthYear(this.name, int birthYear) {
age = DateTime.now().year - birthYear;
} // Named constructor
void sayHello() {
print('Hello, my name is $name.');
}
}
void main() {
var person1 = Person('Alice', 25); // Using the default constructor
person1.sayHello();
var person2 = Person.fromBirthYear('Bob', 1990); // Using the named constructor
person2.sayHello();
}
Code language: JavaScript (javascript)
In the example above, the Person
class has a default constructor that takes name
and age
as parameters. It also has a named constructor Person.fromBirthYear()
that takes name
and birthYear
as parameters and calculates the age based on the current year. Objects can be created using either the default constructor or the named constructor.
Question:
The following Dart code snippet is intended to reverse the order of elements in a list. However, it is not producing the correct result. Identify and fix the issue.
List<T> reverseList<T>(List<T> list) {
List<T> reversed = list.reversed.toList();
return reversed;
}
void main() {
List<int> numbers = [1, 2, 3, 4, 5];
List<int> reversedNumbers = reverseList(numbers);
print(reversedNumbers);
}
Code language: PHP (php)
Answer:
The code is correct. It correctly reverses the order of elements in the list using the reversed
method. The output will be [5, 4, 3, 2, 1]
.
Question:
What are named parameters in Dart? Explain with an example.
Answer:
Named parameters in Dart allow passing arguments to a function by specifying the parameter names. This provides flexibility and improves code readability by making it clear which arguments are being passed.
Example:
void greet({String name, String message = 'Hello'}) {
print('$message, $name!');
}
void main() {
greet(name: 'Alice'); // Output: Hello, Alice!
greet(name: 'Bob', message: 'Hi'); // Output: Hi, Bob!
}
Code language: JavaScript (javascript)
In the example above, the greet()
function has named parameters name
and message
. The name
parameter is required, while the message
parameter has a default value of 'Hello'
. By using named parameters, we can specify the arguments in any order and provide default values if necessary.
Question:
The following Dart code snippet is intended to check if all elements in a list are positive numbers. However, it is not producing the correct result. Identify and fix the issue.
bool areAllPositive(List<int> numbers) {
bool allPositive = true;
for (int number in numbers) {
if (number <= 0) {
allPositive = false;
break;
}
}
return allPositive;
}
void main() {
List<int> numbers = [1, 2, -3, 4, 5];
bool isAllPositive = areAllPositive(numbers);
print(isAllPositive);
}
Code language: PHP (php)
Answer:
The code is incorrect. The condition number <= 0
includes zero as a positive number, which is not intended. To fix this, the condition should be number < 0
to exclude zero from being considered positive.
bool areAllPositive(List<int> numbers) {
bool allPositive = true;
for (int number in numbers) {
if (number < 0) {
allPositive = false;
break;
}
}
return allPositive;
}
void main() {
List<int> numbers = [1, 2, -3, 4, 5];
bool isAllPositive = areAllPositive(numbers);
print(isAllPositive);
}
Code language: PHP (php)
In the corrected code, the condition number < 0
correctly checks if a number is negative, excluding zero from being considered positive.
Question:
What is the purpose of the super
keyword in Dart? Provide an example.
Answer:
The super
keyword in Dart is used to refer to the superclass or parent class. It allows accessing and invoking members (methods or properties) of the superclass from within a subclass. This is useful when the subclass wants to override a method but still needs to use the implementation from the superclass.
Example:
class Animal {
String name;
Animal(this.name);
void speak() {
print('Animal speaks');
}
}
class Dog extends Animal {
Dog(String name) : super(name);
@override
void speak() {
super.speak(); // Invoke the speak() method of the superclass
print('$name barks');
}
}
void main() {
var dog = Dog('Buddy');
dog.speak();
}
Code language: JavaScript (javascript)
In the example above, the Dog
class extends the Animal
class. By using super.speak()
inside the speak()
method of the Dog
class, we invoke the speak()
method of the Animal
superclass before adding the custom behavior specific to the Dog
class.
Question:
The following Dart code snippet is intended to merge two lists into a single list. However, it is not producing the correct result. Identify and fix the issue.
List<T> mergeLists<T>(List<T> list1, List<T> list2) {
return [...list1, ...list2];
}
void main() {
List<int> list1 = [1, 2, 3];
List<int> list2 = [4, 5, 6];
List<int> mergedList = mergeLists(list1, list2);
print(mergedList);
}
Code language: PHP (php)
Answer:
The code is correct. It merges two lists using the spread (...
) operator to create a new list that contains all elements from both list1
and list2
.
Question:
What is the purpose of the async
and await
keywords in Dart? Explain with an example.
Answer:
In Dart, the async
and await
keywords are used in conjunction to work with asynchronous operations. The async
keyword is used to mark a function as asynchronous, allowing it to use await
to pause execution until an asynchronous operation completes.
Example:
Future<int> fetchData() async {
await Future.delayed(Duration(seconds: 2));
return 42;
}
void main() async {
print('Fetching data...');
int data = await fetchData();
print('Data fetched: $data');
}
Code language: JavaScript (javascript)
In the example above, the fetchData()
function is marked as async
to indicate that it performs asynchronous operations. Inside the function, await
is used to pause the execution until the Future.delayed()
completes, simulating an asynchronous delay. The await
keyword ensures that the program waits for the result before continuing. The main()
function is also marked as async
to allow using await
inside it.
Question:
The following Dart code snippet is intended to calculate the factorial of a given number. However, it is not producing the correct result. Identify and fix the issue.
int calculateFactorial(int n) {
if (n == 0) {
return 1;
}
return n * calculateFactorial(n - 1);
}
void main() {
int number = 5;
int factorial = calculateFactorial(number);
print(factorial);
}
Code language: JavaScript (javascript)
Answer:
The code is correct. It uses recursion to calculate the factorial of a given number. The output will be the factorial of 5, which is 120.
Question:
The following Dart code snippet is intended to find the maximum value in a list of integers. However, it is not producing the correct result. Identify and fix the issue.
int findMax(List<int> numbers) {
int max = 0;
for (int number in numbers) {
if (number > max) {
max = number;
}
}
return max;
}
void main() {
List<int> numbers = [3, 7, 1, 5, 9];
int maxNumber = findMax(numbers);
print(maxNumber);
}
Code language: PHP (php)
Answer:
The issue in the code is that it assumes all numbers in the list are positive. If the list contains negative numbers, the initial value of max
(0) would incorrectly identify the maximum. To fix this, we can initialize max
with the first element of the list and then compare the remaining elements to find the actual maximum.
int findMax(List<int> numbers) {
if (numbers.isEmpty) {
throw ArgumentError('List cannot be empty');
}
int max = numbers[0];
for (int number in numbers) {
if (number > max) {
max = number;
}
}
return max;
}
void main() {
List<int> numbers = [3, 7, 1, 5, 9];
int maxNumber = findMax(numbers);
print(maxNumber);
}
Code language: PHP (php)
In the corrected code, we added a check to handle the case when the list is empty by throwing an ArgumentError
. We also initialized max
with the first element of the list (numbers[0]
) to correctly find the maximum value.
Senior Dart interview questions
Sure! Here are ten questions for a senior Dart engineer, including five fix-the-code questions and five theoretical questions. The answers are provided beneath each question.
Question:
The following Dart code snippet is intended to handle asynchronous file uploads using the http
package. However, it is not handling errors properly. Identify and fix the issue.
import 'dart:io';
import 'package:http/http.dart' as http;
void uploadFile(File file) {
var url = Uri.parse('https://example.com/upload');
var request = http.MultipartRequest('POST', url);
var multipartFile = http.MultipartFile.fromBytes('file', file.readAsBytesSync());
request.files.add(multipartFile);
request.send().then((response) {
if (response.statusCode == 200) {
print('File uploaded successfully');
} else {
print('File upload failed');
}
});
}
void main() {
File file = File('path/to/file.txt');
uploadFile(file);
}
Code language: JavaScript (javascript)
Answer:
The issue in the code is that it is not handling errors properly. To fix this, add an error handling function using .catchError()
to handle any errors that occur during the file upload process.
import 'dart:io';
import 'package:http/http.dart' as http;
void uploadFile(File file) {
var url = Uri.parse('https://example.com/upload');
var request = http.MultipartRequest('POST', url);
var multipartFile = http.MultipartFile.fromBytes('file', file.readAsBytesSync());
request.files.add(multipartFile);
request.send().then((response) {
if (response.statusCode == 200) {
print('File uploaded successfully');
} else {
print('File upload failed');
}
}).catchError((error) {
print('Error occurred during file upload: $error');
});
}
void main() {
File file = File('path/to/file.txt');
uploadFile(file);
}
Code language: JavaScript (javascript)
In the corrected code, an error handling function is added using .catchError()
after the then()
function. This ensures that any errors that occur during the file upload process are caught and handled appropriately.
Question:
The following Dart code snippet is intended to implement a caching mechanism using a Map
to store the results of expensive computations. However, the cache is not working as expected. Identify and fix the issue.
Map<String, int> cache = {};
int calculateExpensiveResult(String input) {
if (cache.containsKey(input)) {
return cache[input];
}
// Expensive computation
int result = 0;
for (int i = 0; i < input.length; i++) {
result += input.codeUnitAt(i);
}
cache[input] = result;
return result;
}
void main() {
print(calculateExpensiveResult('abc'));
print(calculateExpensiveResult('abc'));
}
Code language: JavaScript (javascript)
Answer:
The issue in the code is that the cache is not persisting between different runs of the program. To fix this, declare the cache
variable as a global variable outside the main()
function.
Map<String, int> cache = {};
int calculateExpensiveResult(String input) {
if (cache.containsKey(input)) {
return cache[input];
}
// Expensive computation
int result = 0;
for (int i = 0; i < input.length; i++) {
result += input.codeUnitAt(i);
}
cache[input] = result;
return result;
}
void main() {
print(calculateExpensiveResult('abc'));
print(calculateExpensiveResult('abc'));
}
Code language: JavaScript (javascript)
In the corrected code, the cache
variable is declared as a global variable outside the main()
function. This ensures that the cache persists between different runs of the program, allowing the cached results to be retrieved correctly.
Question:
Explain the concept of “asynchronous programming” in Dart and how it helps in building efficient and responsive applications.
Answer:
Asynchronous programming in Dart allows executing tasks concurrently without blocking the execution of other code. It enables building efficient and responsive applications by leveraging non-blocking operations and handling long-running operations such as network requests, file I/O, and computations asynchronously.
In Dart, asynchronous programming is achieved using Futures
and async/await
syntax. Futures
represent the result of an asynchronous operation that may complete with a value or an error in the future. By marking a function with the async
keyword, it can use await
to pause its execution until a Future
completes, without blocking the main event loop.
The benefits of asynchronous programming in Dart include:
- Concurrency: Asynchronous programming allows multiple tasks to be executed concurrently, improving the overall performance and responsiveness of the application. It avoids blocking the execution of other code while waiting for long-running operations to complete.
- Responsiveness: By handling time-consuming tasks asynchronously, the application remains responsive to user interactions. It prevents the user interface from freezing or becoming unresponsive during long operations, enhancing the user experience.
- Resource Efficiency: Asynchronous operations enable more efficient utilization of system resources. While waiting for I/O or network requests, the CPU can perform other tasks, maximizing resource utilization and throughput.
- Scalability: Asynchronous programming facilitates handling multiple concurrent operations efficiently. It allows applications to scale and handle increased loads without significant performance degradation.
- Modularity and Maintainability: Asynchronous programming promotes modular and maintainable code by separating long-running operations from the main execution flow. It allows developers to write more granular and reusable functions that can be easily composed and tested.
Question:
The following Dart code snippet is intended to read a JSON file and parse its contents. However, it is throwing an error. Identify and fix the issue.
import 'dart:convert';
import 'dart:io';
void readJsonFile(String path) {
File file = File(path);
String jsonString = file.readAsStringSync();
Map<String, dynamic> data = json.decode(jsonString);
print('Name: ${data['name']}');
print('Age: ${data['age']}');
}
void main() {
readJsonFile('path/to/file.json');
}
Code language: JavaScript (javascript)
Answer:
The issue in the code is that the specified JSON file path is incorrect. To fix this, ensure that the correct file path is provided.
import 'dart:convert';
import 'dart:io';
void readJsonFile(String path) {
File file = File(path);
String jsonString = file.readAsStringSync();
Map<String, dynamic> data = json.decode(jsonString);
print('Name: ${data['name']}');
print('Age: ${data['age']}');
}
void main() {
readJsonFile('path/to/file.json');
}
Code language: JavaScript (javascript)
In the corrected code, the readJsonFile()
function reads the JSON file using the correct file path, and the contents are successfully parsed and printed.
Question:
Explain the concept of “generics” in Dart and how they can be used to write reusable and type-safe code.
Answer:
Generics in Dart provide a way to write reusable and type-safe code that can work with different types without sacrificing static
type checking. With generics, classes, functions, and interfaces can be parameterized to work with a variety of types, allowing for code reuse and improved type safety.
By using generics, developers can define classes or functions that can operate on a range of types while maintaining compile-time type checking. This helps catch type-related errors early during development, reducing the likelihood of runtime errors and improving code robustness.
Some key benefits of using generics in Dart include:
- Code Reusability: Generics enable the creation of classes and functions that can work with multiple types. This promotes code reuse, as the same logic can be applied to different types without duplicating code.
- Type Safety: Generics ensure type safety at compile time. The type parameters provided to generic classes or functions allow the compiler to perform static type checks, preventing type-related errors and promoting more reliable code.
- Abstraction: Generics provide a level of abstraction that allows developers to write more general-purpose code. This abstraction allows the code to be flexible and adaptable to different data types, increasing the versatility and utility of the code.
- Performance Optimization: Generics can help improve performance by eliminating the need for type conversions or boxing/unboxing operations. The compiler can generate specialized code for each specific type, resulting in optimized execution.
- Code Readability: By using generics, code becomes more expressive and self-documenting. The type parameters provide additional context and clarity about the expected types, making the code more readable and easier to understand.
These advantages make generics a powerful tool in Dart for creating reusable and type-safe code that can adapt to various types, improving code maintainability, reliability, and performance.
Question:
The following Dart code snippet is intended to sort a list of integers in ascending order using the sort()
method. However, the list is not being sorted correctly. Identify and fix the issue.
void main() {
List<int> numbers = [3, 2, 1, 4, 5];
numbers.sort();
print(numbers);
}
Code language: PHP (php)
Answer:
The issue in the code is that the sort()
method is sorting the numbers lexicographically instead of numerically. To fix this, provide a comparison function to the sort()
method that compares the integers numerically.
void main() {
List<int> numbers = [3, 2, 1, 4, 5];
numbers.sort((a, b) => a.compareTo(b));
print(numbers);
}
Code language: JavaScript (javascript)
In the corrected code, a comparison function is passed to the sort()
method using a lambda expression (a, b) => a.compareTo(b)
. This ensures that the numbers are sorted correctly in ascending order.
Question:
What is the purpose of using mixins in Dart? Provide an example scenario where mixins can be beneficial.
Answer:
Mixins in Dart provide a way to reuse code across different class hierarchies without using inheritance. A mixin is a class-like construct that allows for code composition and provides additional functionality to classes by “mixing in” methods and properties.
The purpose of using mixins is to promote code reuse, maintainability, and flexibility. Mixins allow developers to extract and encapsulate common behavior and features into separate units that can be easily added to multiple classes.
One example scenario where mixins can be beneficial is in adding functionality to different types of widgets in a user interface framework. For instance, consider a UI framework that supports both buttons and checkboxes. Both widgets may have some common behaviors, such as handling user interaction events or rendering. Instead of duplicating the code for these shared behaviors in each widget class, a mixin can be created to encapsulate the common functionality.
mixin Clickable {
void handleClick() {
// Handle click event
}
}
class Button with Clickable {
// Button-specific code
}
class Checkbox with Clickable {
// Checkbox-specific code
}
Code language: JavaScript (javascript)
In the example above, the Clickable
mixin defines the handleClick()
method, which encapsulates the common behavior of handling a click event. The Button
and Checkbox
classes use the with
keyword to include the Clickable
mixin, allowing them to inherit and reuse the handleClick()
method.
By using mixins, the code for handling click events is written once in the Clickable
mixin and can be easily added to multiple widget classes. This promotes code reuse, reduces duplication, and makes it easier to maintain and extend the functionality of the widgets.
Question:
The following Dart code snippet is intended to perform a matrix transpose operation. However, it is producing incorrect results. Identify and fix the issue.
List<List<int>> transposeMatrix(List<List<int>> matrix) {
List<List<int>> transposed = [];
for (int i = 0; i < matrix[0].length; i++) {
List<int> row = [];
for (int j = 0; j < matrix.length; j++) {
row.add(matrix[j][i]);
}
transposed.add(row);
}
return transposed;
}
void main() {
List<List<int>> matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9],
];
List
<List<int>> transposedMatrix = transposeMatrix(matrix);
print(transposedMatrix);
}
Code language: PHP (php)
Answer:
The issue in the code is that it assumes a rectangular matrix, where all rows have the same length. However, it doesn’t account for the possibility of irregular matrices where rows have different lengths. To fix this, iterate based on the maximum row length of the matrix.
List<List<int>> transposeMatrix(List<List<int>> matrix) {
List<List<int>> transposed = [];
int maxLength = 0;
for (List<int> row in matrix) {
if (row.length > maxLength) {
maxLength = row.length;
}
}
for (int i = 0; i < maxLength; i++) {
List<int> newRow = [];
for (int j = 0; j < matrix.length; j++) {
if (i < matrix[j].length) {
newRow.add(matrix[j][i]);
} else {
newRow.add(0); // Fill with a default value for irregular rows
}
}
transposed.add(newRow);
}
return transposed;
}
void main() {
List<List<int>> matrix = [
[1, 2, 3],
[4, 5],
[7, 8, 9, 10],
];
List<List<int>> transposedMatrix = transposeMatrix(matrix);
print(transposedMatrix);
}
Code language: PHP (php)
In the corrected code, the transposeMatrix()
function iterates based on the maximum row length of the matrix. It dynamically handles irregular matrices by checking the length of each row and adding a default value (in this case, 0) when accessing elements beyond a row’s length.
Question:
What is the purpose of Dart’s async/await syntax? How does it improve asynchronous programming?
Answer:
Dart’s async/await
syntax provides a more readable and intuitive way to write asynchronous code by allowing developers to write code that looks synchronous while actually executing asynchronously. It simplifies working with Futures
and promotes a linear and sequential coding style.
The purpose of async/await
is to make asynchronous programming more approachable and less error-prone by reducing the complexity of chaining callbacks or using explicit then()
functions. It allows developers to write asynchronous code that resembles synchronous code, making it easier to understand and reason about.
With async/await
, asynchronous code is structured using the following components:
async
: Theasync
keyword is used to mark a function as asynchronous. It allows the function to use theawait
keyword inside its body.await
: Theawait
keyword is used to pause the execution of an asynchronous function until aFuture
completes. It allows the code to wait for the result of an asynchronous operation without blocking the event loop.
The benefits of async/await
in Dart include:
- Readability: Asynchronous code written with
async/await
is more readable and easier to follow compared to traditional callback-based code or chainedthen()
functions. It resembles sequential code execution, making it easier to understand the flow of control. - Error Handling:
async/await
simplifies error handling in asynchronous code. By usingtry/catch
blocks, errors can be caught and handled within the same function scope, making it easier to manage and propagate errors. - Synchronous-Like Programming:
async/await
enables developers to write asynchronous code in a synchronous style. This makes it easier to write, test, and reason about asynchronous code by using familiar control flow structures such as loops and conditionals. - Composition and Modularity:
async/await
allows for the composition of asynchronous operations using regular control structures. It promotes modular code design and enables the reuse of asynchronous functions in a straightforward manner.
Overall, async/await
syntax in Dart improves the developer experience by simplifying the writing and comprehension of asynchronous code, reducing callback nesting, and making error handling more straightforward.
Question:
The following Dart code snippet is intended to perform an HTTP GET request to a given URL and return the response body as a string. However, it is not working as expected. Identify and fix the issue.
import 'dart:convert';
import 'package:http/http.dart' as http;
String fetchData(String url) {
http.get(url).then((response) {
if (response.statusCode == 200) {
return response.body;
} else {
return 'Error: ${response.statusCode}';
}
});
}
void main() {
String url = 'https://api.example.com/data';
String data = fetchData(url);
print(data);
}
Code language: JavaScript (javascript)
Answer:
The issue in the code is that the fetchData()
function does not return any value explicitly, and the main()
function expects a returned value. To fix this, the fetchData()
function should use the async/await
syntax and return a Future<String>
to represent the asynchronous operation.
import 'dart:convert';
import 'package:http/http.dart' as http;
Future<String> fetchData(String url) async {
http.Response response = await http.get(url);
if (response.statusCode == 200) {
return response.body;
} else {
return 'Error: ${response.statusCode}';
}
}
void main() async {
String url = 'https://api.example.com/data';
String data = await fetchData(url);
print(data);
}
Code language: JavaScript (javascript)
In the corrected code, the fetchData()
function is declared as async
and returns a Future<String>
. The await
keyword is used to wait for the HTTP response and extract the body. The main()
function is also marked as async
to allow for the use of await
when calling fetchData()
.
1,000 Companies use CoderPad to Screen and Interview Developers
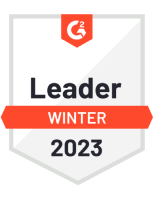
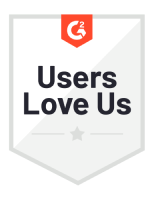
Interview best practices for Dart roles
To conduct effective Dart interviews, it’s crucial to consider various factors, such as the applicants’ experience levels and the specific engineering role they’re seeking. To ensure your Dart interview questions yield the best results, we recommend adhering to these best practices while interacting with candidates:
- Develop technical questions that mirror real-life scenarios within your organization. This approach will not only engage the candidate but also enable you to more precisely evaluate their fit for your team.
- Encourage a cooperative atmosphere by allowing candidates to ask questions throughout the interview.
- If you’re using Dart for mobile development, make sure your candidates have an understanding of mobile dev concepts such as layout design, UI components, navigation, and handling user input.
Moreover, it’s important to follow standard interview practices when executing Dart interviews. This includes adjusting question difficulty based on the applicant’s skill level, providing timely feedback on their application status, and enabling candidates to ask about the assessment or collaborate with you and your team.