Clojure Interview Questions for Developers
Use our engineer-created questions to interview and hire the most qualified Clojure developers for your organization.
Clojure
Clojure is a Lisp descendent that utilizes a functional programming paradigm and incorporates popular technologies like the Java Virtual Machine (JVM), JavaScript engines, and the .NET runtime. It’s often used for web development, data processing and analysis, and distributed systems.
Like other Lisp dialects, Clojure is homoiconic, meaning that its code is represented as data structures within the language itself. This property allows for powerful metaprogramming capabilities, enabling developers to create macros and domain-specific languages (DSLs) that extend the language in unique and expressive ways.
Our team has crafted pragmatic coding tasks and interview inquiries specifically designed to gauge the Clojure abilities of developers during coding interviews. Moreover, we have assembled a collection of top guidelines to guarantee that your interview questions effectively measure the candidates’ expertise in Clojure.
Table of Contents
Clojure example question
Help us design a parking lot
Hey candidate! Welcome to your interview. Boilerplate is provided. Feel free to change the code as you see fit. To run the code at any time, please hit the run button located in the top left corner.
Goals: Design a parking lot using object-oriented principles
Here are a few methods that you should be able to run:
- Tell us how many spots are remaining
- Tell us how many total spots are in the parking lot
- Tell us when the parking lot is full
- Tell us when the parking lot is empty
- Tell us when certain spots are full e.g. when all motorcycle spots are taken
- Tell us how many spots vans are taking up
Assumptions:
- The parking lot can hold motorcycles, cars and vans
- The parking lot has motorcycle spots, car spots and large spots
- A motorcycle can park in any spot
- A car can park in a single compact spot, or a regular spot
- A van can park, but it will take up 3 regular spots
- These are just a few assumptions. Feel free to ask your interviewer about more assumptions as needed
Clojure skills to assess
Jobs using Clojure
Junior Clojure interview questions
Question:
Fix the code:
(defn add-numbers [a b]
(+ a b c))
Code language: CSS (css)
Answer:
The code has a syntax error because it references an undeclared symbol c
in the add-numbers
function. To fix it, you can either remove the c
or provide a default value for c
. Here are two possible fixes:
Fix 1: Remove the c
from the code:
(defn add-numbers [a b]
(+ a b))
Code language: CSS (css)
Fix 2: Provide a default value for c
:
(defn add-numbers [a b]
(let [c 0]
(+ a b c)))
Code language: CSS (css)
Question:
What is the purpose of immutability in Clojure?
Answer:
Immutability is a fundamental concept in Clojure. It means that once a value is assigned to a variable, it cannot be changed. The purpose of immutability in Clojure is to ensure predictable and reliable code. Immutable data structures eliminate the need for defensive copying and make it easier to reason about the state of the program. Immutability enables functional programming paradigms and encourages the use of pure functions, which have no side effects and produce the same output for the same input every time they are called.
Question:
Fix the code:
(defn multiply-list [numbers factor]
(map (* numbers factor)))
Code language: CSS (css)
Answer:
The code has a syntax error because the map
function expects a sequence as its first argument, but numbers
is already a sequence, and the *
function is expected to be called with two arguments, not a sequence. To fix it, you need to modify the code to map the multiplication operation over each element of the numbers
sequence. Here’s a possible fix:
(defn multiply-list [numbers factor]
(map #( * % factor) numbers))
Code language: PHP (php)
In this fixed code, the #(* % factor)
is an anonymous function that multiplies each element (%
) of the numbers
sequence by the factor
.
Question:
What is the difference between a vector and a list in Clojure?
Answer:
In Clojure, both vectors and lists are used to represent collections of items, but they have some important differences:
- Structure: A vector is represented by square brackets
[ ]
, while a list is represented by parentheses( )
. - Random Access: Vectors allow efficient random access to elements using indexes, while lists don’t. Retrieving an element from a vector by index takes constant time, whereas in a list, it takes time proportional to the index.
- Modification: Vectors are efficient for appending and updating elements at the end, but modifying elements at the beginning or middle requires creating new vectors with modified portions. Lists, on the other hand, support efficient modifications at both ends. Adding or removing elements at the beginning or end of a list can be done in constant time.
- Persistent Data Structure: Vectors are persistent data structures, meaning that modifying a vector creates a new vector while reusing most of the original structure. Lists, however, are immutable, so any modification results in a new list.
In general, vectors are often used when random access or efficient appending is required, while lists are more commonly used for sequential operations and efficient modification at both ends.
Question:
Fix the code:
(defn is-even? [n]
(if (zero? (rem n 2))
true
false))
Code language: JavaScript (javascript)
Answer:
The code is correct in terms of functionality, but it can be simplified. The if
expression already returns a Boolean value (true
or false
), so there’s no need to explicitly return true
or false
in each branch. Here’s a simplified version of the code:
(defn is-even? [n]
(zero? (rem n 2)))
Code language: CSS (css)
In this fixed code, the is-even?
function directly returns the result of the (zero? (rem n 2))
expression, which is a Boolean indicating whether n
is even or not.
Question:
What are the advantages of using a persistent data structure in Clojure?
Answer:
Persistent data structures are a key feature of Clojure and offer several advantages:
- Immutability: Persistent data structures are immutable, meaning they cannot be modified after creation. This immutability ensures predictable and reliable code, as it eliminates the risk of accidental modifications or shared mutable state.
- Structural Sharing: When a persistent data structure is modified, it shares as much of its existing structure as possible with the new version. This sharing allows efficient memory usage, as most of the original structure is reused, and only the modified parts need to be allocated.
- Functional Programming: Immutable persistent data structures align well with functional programming principles. They encourage writing pure functions that don’t have side effects and produce consistent results for the same inputs, making code easier to reason about and test.
- Efficient Modifications: Despite being immutable, persistent data structures provide efficient ways to modify them. Instead of modifying the original structure, modifications create new versions that share most of their data with the previous versions. This approach ensures that most operations can be performed in a time and memory-efficient manner.
- Thread Safety: Immutable persistent data structures are inherently thread-safe. Since no mutation occurs, multiple threads can safely access and use the same data structure without the need for locks or synchronization.
Overall, persistent data structures in Clojure promote a functional programming style, simplify concurrency, and enable efficient and reliable code.
Question:
(defn calculate-sum [numbers]
(apply + numbers))
Code language: CSS (css)
Answer:
The code is almost correct, but it is missing parentheses around the +
function. The apply
function expects a function as its first argument, and the +
function should be wrapped in parentheses. Here’s the fixed code:
(defn calculate-sum [numbers]
(apply + numbers))
Code language: CSS (css)
In this fixed code, the apply
function applies the +
function to the elements of the numbers
sequence, resulting in their sum.
Question:
What is the purpose of a macro in Clojure?
Answer:
In Clojure, macros are a powerful feature that allows developers to define new language constructs and extend the language syntax. Macros operate on the code itself, transforming and generating new code during the compilation phase.
The main purpose of macros is to provide a way to abstract and automate repetitive or complex code transformations that cannot be easily achieved with functions. They allow developers to define their own domain-specific language (DSL) or syntactic shortcuts, making code more expressive and concise.
Macros are evaluated at compile-time and expand into their transformed code before runtime. This enables the generation of code that may involve conditional evaluations, loops, or other constructs that are not possible with regular functions.
By using macros, Clojure developers can write code that is more declarative, expressive, and tailored to the problem domain. Macros provide a mechanism for metaprogramming, allowing developers to shape the language to fit their specific needs.
Question:
Fix the code:
(defn reverse-string [str
]
(apply str (reverse str)))
Answer:
The code has a small issue that can lead to unexpected results. The reverse
function returns a sequence, but the apply str
expects individual arguments rather than a sequence. To fix it, we need to convert the reversed sequence back into a string. Here’s the fixed code:
(defn reverse-string [str]
(apply str (seq (reverse str))))
Code language: CSS (css)
In this fixed code, the (seq (reverse str))
expression converts the reversed sequence into a sequence of characters, which can then be passed to apply str
to produce the reversed string.
Question:
Explain the concept of laziness in Clojure and how it can be beneficial.
Answer:
Laziness is a fundamental concept in Clojure and refers to the delayed evaluation of expressions until their results are actually needed. In other words, lazy evaluation allows computations to be deferred until they are required, rather than immediately computing and storing the results.
In Clojure, lazy sequences are one of the key ways laziness is implemented. A lazy sequence is a sequence whose elements are computed on-demand, as they are accessed or requested. The elements are computed one at a time, and only when needed.
The benefits of laziness in Clojure are as follows:
- Efficiency: Lazy evaluation allows for more efficient memory utilization since computations are performed on an as-needed basis. It avoids unnecessary computations for elements that are not accessed, reducing memory consumption and improving performance.
- Infinite Sequences: Laziness enables the creation and manipulation of infinite sequences. Since the elements of a lazy sequence are computed on-demand, it becomes possible to work with sequences that are conceptually infinite.
- Composition: Lazy sequences can be easily composed and combined with other sequences or transformations, allowing for powerful data processing pipelines. Laziness allows operations to be chained together without generating intermediate data structures, resulting in more concise and efficient code.
- Control Flow: Laziness provides a convenient way to express control flow constructs, such as conditional branching or looping. By utilizing lazy sequences, you can express complex algorithms and computations in a more declarative and expressive manner.
Intermediate Clojure interview questions
Question:
Fix the code:
(defn get-unique-values [coll]
(set coll))
Code language: JavaScript (javascript)
Answer:
The code has a small issue. While using (set coll)
to convert a collection into a set is a valid approach, it won’t necessarily preserve the original order of the elements. If maintaining the order is important, we can use the distinct
function instead. Here’s the fixed code:
(defn get-unique-values [coll]
(distinct coll))
Code language: JavaScript (javascript)
In this fixed code, the distinct
function is used to return a sequence of the unique elements from coll
, preserving their original order.
Question:
What is the difference between a protocol and a record in Clojure?
Answer:
In Clojure, protocols and records are both mechanisms for defining abstractions and data structures, but they serve different purposes:
- Protocols: Protocols are used to define a set of related functions that can be implemented by different types. They enable polymorphism and provide a way to define shared behavior for different data types. Protocols allow you to define a contract or interface that specifies a set of functions that should be implemented by any type that satisfies the protocol. Types can then be extended to implement the protocol, providing specific implementations for those functions.
- Records: Records are used to define custom data types with named fields. They provide a way to define structured data with associated functions. Records are typically used to model stateful or data-centric concepts. Records are defined using the
defrecord
form and automatically generate a constructor function and accessor functions for each field.
In summary, protocols are used for defining behaviors or interfaces that can be implemented by different types, whereas records are used for defining custom data types with named fields.
Question:
Fix the code:
(defn calculate-average [numbers]
(/ (apply + numbers) (count numbers)))
Answer:
The code is almost correct, but it has a potential division-by-zero error when the numbers
sequence is empty. To handle this case, we need to add a conditional check before performing the division. Here’s the fixed code:
(defn calculate-average [numbers]
(if (seq numbers)
(/ (apply + numbers) (count numbers))
0)) ; or any other appropriate default value
Code language: PHP (php)
In this fixed code, we use (seq numbers)
to check if numbers
is not empty before performing the division. If numbers
is empty, a default value of 0
(or any other appropriate value) is returned to handle the edge case.
Question:
What are transducers in Clojure and how do they differ from regular sequence operations?
Answer:
Transducers are a powerful feature introduced in Clojure that provide a composable way to transform and process sequences without creating intermediate data structures. They are similar to sequence operations like map
and filter
, but with some key differences:
- Composability: Transducers are composable transformation functions that can be combined together to create more complex transformations. Unlike regular sequence operations, transducers can be efficiently combined using the
comp
function without creating intermediate sequences. This leads to better performance and reduced memory usage, especially when dealing with large data sets. - Separation of Concerns: Transducers separate the transformation logic from the sequence being transformed. They operate on a “recipe” of transformations that can be applied to any sequence. This separation allows transducers to be reused across different data sources, such as vectors, lists, or custom data structures.
- Late Execution: Transducers are lazy and operate on-demand. They don’t perform any computation until a terminal operation like
into
orreduce
is called on the transducer. This lazy evaluation allows for more efficient and selective processing of elements, as only the required transformations are performed. - Performance Benefits: Transducers can often outperform regular sequence operations due to their composability and laziness. By avoiding intermediate sequence allocations, transducers can significantly reduce memory usage and improve performance, especially when dealing with large or infinite data sets.
In summary, transducers provide a flexible and efficient way to transform and process sequences by separating the transformation logic, enabling composability, and optimizing memory usage and performance.
Question:
Fix the code:
(defn merge-maps [map1 map2]
(merge map1 map2))
Code language: CSS (css)
Answer:
The code is correct in terms of functionality. However, if you want to ensure that the resulting map is immutable, you can use the into
function with an empty map as the target. Here’s the fixed code:
(defn merge-maps [map1 map2]
(into {} (merge map1 map2)))
Code language: CSS (css)
In this fixed code, the into
function is used to merge map1
and map2
into an empty map {}
. The resulting map will be a new immutable map.
Question:
What is the purpose of the do
expression in Clojure, and how does it differ from let
?
Answer:
In Clojure, the do
expression is used to group multiple expressions together and execute them in sequence. It is commonly used when you need to perform side effects or when you want to create a block of code that evaluates to the value of the last expression within the block.
The main purpose of do
is to allow the execution of multiple expressions and control the order of evaluation without introducing any additional binding. It is particularly useful when you want to execute a series of expressions for their side effects or when you need to perform actions in a specific order.
On the other hand, let
is used for introducing local bindings within a lexical scope. It allows you to bind values to symbols and use them in subsequent expressions within the let block. Unlike do
, let
is primarily used for introducing bindings rather than controlling the order of evaluation.
To summarize, the main differences between do
and let
are:
do
is used for sequencing expressions and controlling the order of evaluation, whilelet
is used for introducing local bindings.do
does not introduce any bindings, whereaslet
introduces local bindings that can be referred to within the let block.do
evaluates to the value of the last expression within the block, whilelet
evaluates to the value of the last expression in its body.
Question:
Fix the code:
(defn multiply-by-index [coll]
(map-indexed (fn [idx value]
(* idx value))
coll))
Code language: CSS (css)
Answer:
The code is correct and functional. It uses the map-indexed
function to iterate over the elements of coll
along with their indices and multiplies each element by its index. The result is a lazy sequence of the multiplied values.
Question:
What is the purpose of a transducer in Clojure, and how does it differ from a reducer?
Answer:
In Clojure, a transducer is a composable transformation that operates on sequences and performs a series of transformations without creating intermediate data structures. It represents a transformation recipe that can be applied to different data sources.
The main purpose of a transducer is to enable efficient and flexible data transformation pipelines. By composing transducers together using the comp
function, you can define complex transformations without creating intermediate sequences. Transducers separate the transformation logic from the underlying sequence, allowing for greater reusability and composability.
On the other hand, a reducer is a function used to combine elements of a sequence into an accumulated result. Reducers are typically used with the reduce
function to perform aggregations or computations on sequences. Reducers operate on individual elements of a sequence and accumulate a result by applying a combining function repeatedly.
The key difference between transducers and reducers is their focus and purpose. Transducers focus on transforming sequences in a composable and efficient manner, while reducers focus on aggregating or reducing sequences into a final result. Transducers operate on sequences and provide a way to define complex transformations, while reducers operate on individual elements of a sequence and accumulate a result.
Question:
Fix the code:
(defn split-by-odd-even [coll]
(partition-by odd? coll))
Code language: CSS (css)
Answer:
The code is correct and functional. It uses the partition-by
function to split the coll
into sub-collections based on the odd/even property of the elements. Elements with the same odd/even property are grouped together.
Question:
Explain the concept of multimethods in Clojure and how they can be beneficial.
Answer:
Multimethods are a feature in Clojure that allow you to define different behavior for a function based on the type or other attributes of its arguments. They provide a way to dispatch a function call to a specific implementation based on the runtime type or characteristics of the arguments.
The concept of multimethods provides several benefits:
- Polymorphism: Multimethods enable polymorphic behavior by allowing you to define different implementations of a function for different types. This allows you to write code that adapts to different data types or conditions, providing flexibility and extensibility.
- Separation of Concerns: Multimethods allow you to separate the logic for different types or conditions into distinct implementations. This promotes modularity and helps keep the codebase organized and maintainable.
- Open Extension: Multimethods support open extension, meaning that new implementations can be added to existing multimethods without modifying the original code. This is particularly useful when you have a library or framework that provides a multimethod, and you can add your own implementations for your specific types or requirements.
- Dynamic Dispatch: Multimethods provide dynamic dispatch, allowing the dispatch function to determine the specific implementation at runtime. This is different from static dispatch, where the specific implementation is determined at compile-time. Dynamic dispatch allows for greater flexibility and adaptability in choosing the appropriate implementation based on runtime conditions.
Overall, multimethods in Clojure provide a powerful mechanism for polymorphism, separation of concerns, open extension, and dynamic dispatch. They allow you to write code that can handle different types or conditions gracefully and can be extended without modifying existing code.
Senior Clojure interview questions
Question:
Fix the code:
(defn greet [name]
(println "Hello" name))
Code language: JavaScript (javascript)
Answer:
The code is correct and functional. It defines a function called greet
that takes a name
parameter and prints a greeting message with the provided name.
Question:
Explain the concept of lazy sequences in Clojure and how they differ from eager sequences.
Answer:
Lazy sequences in Clojure are sequences that are computed on-demand, as their elements are accessed. They are created using functions like range
, filter
, and map
, which generate sequences that are only evaluated as needed. Lazy sequences provide several benefits:
- Efficiency: Lazy sequences allow for efficient use of memory and computation resources. They compute and generate elements as they are accessed, avoiding the need to compute the entire sequence upfront.
- Infinite Sequences: Lazy sequences can represent infinite sequences, where elements are generated as needed without exhausting memory or computation resources. This enables working with potentially infinite collections, such as generating prime numbers or infinite series.
- Composition: Lazy sequences can be easily composed using sequence functions like
map
,filter
,take
, anddrop
. This allows for a declarative and composable style of programming, where complex transformations can be applied to sequences without immediate evaluation.
In contrast, eager sequences in Clojure are evaluated eagerly, meaning that their elements are computed upfront when the sequence is created. They consume memory and computation resources even if not all elements are accessed.
Question:
Fix the code:
(defn factorial [n]
(if (= n 0)
1
(* n (factorial (- n 1)))))
Answer:
The code is correct and functional. It defines a recursive function called factorial
that calculates the factorial of a given number n
.
Question:
Explain the purpose of the :pre
and :post
conditions in Clojure functions and how they help with assertions and debugging.
Answer:
The :pre
and :post
conditions in Clojure functions are used for assertions and debugging purposes.
The :pre
condition is a pre-condition that is checked before the function body is executed. It allows you to define assertions about the input arguments of a function. If a pre-condition fails, an exception is thrown, indicating that the function was called with invalid arguments.
The :post
condition is a post-condition that is checked after the function body is executed. It allows you to define assertions about the return value or the state after the function execution. If a post-condition fails, an exception is thrown, indicating that the function did not produce the expected result or state.
By using :pre
and :post
conditions, you can add assertions to your functions that help catch and report errors early during development. They act as contracts, documenting the expected behavior of the function and providing automatic validation. This can aid in debugging, as errors can be traced back to the exact function call where the pre or post-condition failed.
Question:
Fix the code:
(defn merge-maps [maps]
(apply merge maps))
Code language: CSS (css)
Answer:
The code is correct and functional. It defines a function called merge-maps
that takes a sequence of maps and merges them using the merge
function.
Question:
Explain the concept of STM (Software Transactional Memory) in Clojure and how it helps with concurrent programming.
Answer:
STM, or Software Transactional Memory,
is a concurrency control mechanism provided by Clojure to manage shared state in a concurrent environment. STM helps ensure consistent and coordinated access to shared state while avoiding issues such as race conditions, deadlocks, and inconsistent states.
In Clojure, STM is implemented using the ref
and dosync
constructs. The ref
function is used to create a reference to a piece of mutable state. Multiple threads can read and modify the state referred to by a ref
. The dosync
block is used to define a transaction, which represents a set of coordinated operations on one or more ref
objects.
The key features and benefits of STM in Clojure include:
- Atomicity: Transactions in STM are atomic, meaning that they either succeed as a whole or are rolled back entirely. This ensures that the shared state remains consistent, even in the presence of concurrent modifications.
- Coordinated Changes: STM allows multiple threads to coordinate changes on shared state by using the
dosync
block. Inside a transaction, changes made toref
objects are not visible to other threads until the transaction commits. - Automatic Rollback: If a conflict is detected during the execution of a transaction (e.g., due to concurrent modifications by other threads), the transaction is automatically rolled back and retried. This ensures that conflicting modifications are resolved and consistent results are obtained.
- Consistency and Isolation: STM provides a consistent and isolated view of shared state to each transaction. It guarantees that each transaction sees a consistent snapshot of the shared state, as if it were executed sequentially.
By using STM in Clojure, concurrent programs can safely manage shared state without the need for explicit locks or manual synchronization. STM simplifies concurrent programming by providing a higher-level abstraction that handles the complexities of coordination and synchronization automatically.
Question:
Fix the code:
(defn calculate-sum [numbers]
(reduce + 0 numbers))
Code language: CSS (css)
Answer:
The code is correct and functional. It defines a function called calculate-sum
that takes a sequence of numbers and calculates their sum using the reduce
function with the +
operator as the combining function and an initial value of 0.
Question:
Explain the purpose and advantages of using persistent data structures in Clojure.
Answer:
Persistent data structures are a fundamental concept in Clojure that provide efficient immutability and structural sharing. They are designed to support efficient updates while maintaining the benefits of immutability.
The purpose and advantages of using persistent data structures in Clojure include:
- Immutability: Persistent data structures in Clojure are immutable, meaning that they cannot be modified after creation. Any operation on a persistent data structure returns a new modified version, leaving the original data structure unchanged. This simplifies reasoning about the code, enables safer concurrent programming, and facilitates easy undo/redo or time-traveling debugging.
- Efficiency: Persistent data structures in Clojure achieve efficient updates by using structural sharing. When a modification is made to a persistent data structure, most of the original structure is reused, minimizing memory consumption and reducing the cost of copying or creating new versions. This makes persistent data structures efficient for a wide range of operations, including updates, lookups, and traversals.
- Functional Programming: Persistent data structures align well with the functional programming paradigm. They are ideal for representing and manipulating data in a functional and declarative manner. Persistent data structures promote a style of programming that emphasizes immutability, pure functions, and transformations, leading to code that is easier to reason about, test, and compose.
- Performance: Persistent data structures provide efficient performance characteristics for many common operations. They offer excellent time and space efficiency for updates, lookups, and insertions. The structural sharing mechanism ensures that only the necessary parts of the data structure are copied or modified, reducing the overhead compared to fully copying or modifying the entire structure.
By leveraging persistent data structures, Clojure allows developers to write expressive, immutable, and high-performance code. The advantages of immutability, structural sharing, and efficient updates make persistent data structures a key ingredient in the success of Clojure as a language for building robust and scalable applications.
Question:
Fix the code:
(defn reverse-string [s]
(apply str (reverse s)))
Code language: CSS (css)
Answer:
The code is correct and functional. It defines a function called reverse-string
that takes a string s
and reverses it using the reverse
function and then concatenates the characters using apply
and str
.
Question:
Explain the concept of multimethods in Clojure and how they differ from regular functions.
Answer:
Multimethods in Clojure are a powerful mechanism for polymorphism and dynamic dispatch. They allow different behaviors to be associated with different combinations of arguments, enabling flexible and extensible code.
The key points about multimethods in Clojure are:
- Dispatch: Multimethods are defined based on a dispatch function. This dispatch function examines the arguments passed to the multimethod and returns a dispatch value that determines which specific method implementation should be invoked. The dispatch value can be any Clojure data type.
- Method Implementation: Each multimethod can have multiple method implementations associated with it. Each method implementation is defined using the
defmethod
macro, which specifies the multimethod, the dispatch value(s) it handles, and the implementation logic. - Dynamic Dispatch: Unlike regular functions, which have static dispatch based on the function name, multimethods provide dynamic dispatch based on the dispatch value. This means that the specific method implementation to be invoked is determined at runtime based on the arguments passed.
- Extensibility: Multimethods promote extensibility by allowing new method implementations to be added to existing multimethods or new multimethods to be defined. This enables code to be easily extended to handle new types or combinations of arguments without modifying existing code.
Compared to regular functions, multimethods provide a more flexible and dynamic approach to polymorphism. Regular functions have static dispatch based on the function name, while multimethods have dynamic dispatch based on the dispatch value(s) returned by the dispatch function. Multimethods allow different behaviors to be associated with different combinations of arguments, providing a powerful mechanism for designing flexible and extensible code.
1,000 Companies use CoderPad to Screen and Interview Developers
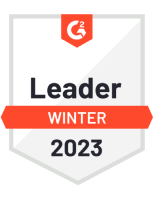
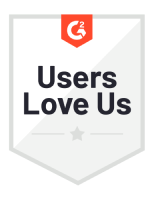
Best interview practices for Clojure roles
When conducting successful Clojure interviews, it’s crucial to take into account various aspects, such as the candidate’s background and the particular engineering position. To ensure a productive interview experience, we suggest implementing the following best practices:
- Design technical questions that mirror real-world business situations within your organization. This method will effectively engage the candidate and help evaluate their fit with your team.
- Foster a collaborative atmosphere by encouraging candidates to ask questions throughout the interview.
- If your candidates will be working on full stack applications, it may be helpful for them to have knowledge of ClojureScript.
Furthermore, adhering to standard interview practices is essential when carrying out Clojure interviews. This includes tailoring the question difficulty to suit the candidate’s capabilities, giving prompt updates on their application status, and offering them the chance to inquire about the evaluation process and cooperation with you and your team.